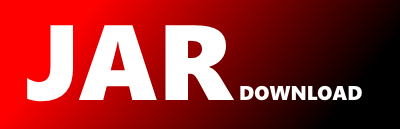
odata.msgraph.client.beta.entity.AndroidDeviceOwnerVpnConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.complex.AppListItem;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleDeviceMode;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsEdition;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsVersion;
import odata.msgraph.client.beta.complex.KeyValue;
import odata.msgraph.client.beta.complex.KeyValuePair;
import odata.msgraph.client.beta.complex.VpnProxyServer;
import odata.msgraph.client.beta.complex.VpnServer;
import odata.msgraph.client.beta.entity.request.AndroidDeviceOwnerCertificateProfileBaseRequest;
import odata.msgraph.client.beta.entity.request.DeviceManagementDerivedCredentialSettingsRequest;
import odata.msgraph.client.beta.enums.AndroidVpnConnectionType;
import odata.msgraph.client.beta.enums.VpnAuthenticationMethod;
/**
* “By providing the configurations in this profile you can instruct the Android
* Fully Managed device to connect to desired VPN endpoint. By specifying the
* authentication method and security types expected by VPN endpoint you can make
* the VPN connection seamless for end user.”
*/@JsonPropertyOrder({
"@odata.type",
"alwaysOn",
"alwaysOnLockdown",
"connectionType",
"customData",
"customKeyValueData",
"microsoftTunnelSiteId",
"proxyServer",
"targetedMobileApps",
"targetedPackageIds"})
@JsonInclude(Include.NON_NULL)
public class AndroidDeviceOwnerVpnConfiguration extends VpnConfiguration implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.androidDeviceOwnerVpnConfiguration";
}
@JsonProperty("alwaysOn")
protected Boolean alwaysOn;
@JsonProperty("alwaysOnLockdown")
protected Boolean alwaysOnLockdown;
@JsonProperty("connectionType")
protected AndroidVpnConnectionType connectionType;
@JsonProperty("customData")
protected List customData;
@JsonProperty("customData@nextLink")
protected String customDataNextLink;
@JsonProperty("customKeyValueData")
protected List customKeyValueData;
@JsonProperty("customKeyValueData@nextLink")
protected String customKeyValueDataNextLink;
@JsonProperty("microsoftTunnelSiteId")
protected String microsoftTunnelSiteId;
@JsonProperty("proxyServer")
protected VpnProxyServer proxyServer;
@JsonProperty("targetedMobileApps")
protected List targetedMobileApps;
@JsonProperty("targetedMobileApps@nextLink")
protected String targetedMobileAppsNextLink;
@JsonProperty("targetedPackageIds")
protected List targetedPackageIds;
@JsonProperty("targetedPackageIds@nextLink")
protected String targetedPackageIdsNextLink;
protected AndroidDeviceOwnerVpnConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderAndroidDeviceOwnerVpnConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode;
private DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition;
private DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private Boolean supportsScopeTags;
private Integer version;
private VpnAuthenticationMethod authenticationMethod;
private String connectionName;
private String realm;
private String role;
private List servers;
private String serversNextLink;
private Boolean alwaysOn;
private Boolean alwaysOnLockdown;
private AndroidVpnConnectionType connectionType;
private List customData;
private String customDataNextLink;
private List customKeyValueData;
private String customKeyValueDataNextLink;
private String microsoftTunnelSiteId;
private VpnProxyServer proxyServer;
private List targetedMobileApps;
private String targetedMobileAppsNextLink;
private List targetedPackageIds;
private String targetedPackageIdsNextLink;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder deviceManagementApplicabilityRuleDeviceMode(DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode) {
this.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleDeviceMode");
return this;
}
public Builder deviceManagementApplicabilityRuleOsEdition(DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition) {
this.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsEdition");
return this;
}
public Builder deviceManagementApplicabilityRuleOsVersion(DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion) {
this.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsVersion");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder supportsScopeTags(Boolean supportsScopeTags) {
this.supportsScopeTags = supportsScopeTags;
this.changedFields = changedFields.add("supportsScopeTags");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
public Builder authenticationMethod(VpnAuthenticationMethod authenticationMethod) {
this.authenticationMethod = authenticationMethod;
this.changedFields = changedFields.add("authenticationMethod");
return this;
}
public Builder connectionName(String connectionName) {
this.connectionName = connectionName;
this.changedFields = changedFields.add("connectionName");
return this;
}
public Builder realm(String realm) {
this.realm = realm;
this.changedFields = changedFields.add("realm");
return this;
}
public Builder role(String role) {
this.role = role;
this.changedFields = changedFields.add("role");
return this;
}
public Builder servers(List servers) {
this.servers = servers;
this.changedFields = changedFields.add("servers");
return this;
}
public Builder servers(VpnServer... servers) {
return servers(Arrays.asList(servers));
}
public Builder serversNextLink(String serversNextLink) {
this.serversNextLink = serversNextLink;
this.changedFields = changedFields.add("servers");
return this;
}
/**
* “Whether or not to enable always-on VPN connection.”
*
* @param alwaysOn
* value of {@code alwaysOn} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder alwaysOn(Boolean alwaysOn) {
this.alwaysOn = alwaysOn;
this.changedFields = changedFields.add("alwaysOn");
return this;
}
/**
* “If always-on VPN connection is enabled, whether or not to lock network traffic
* when that VPN is disconnected.”
*
* @param alwaysOnLockdown
* value of {@code alwaysOnLockdown} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder alwaysOnLockdown(Boolean alwaysOnLockdown) {
this.alwaysOnLockdown = alwaysOnLockdown;
this.changedFields = changedFields.add("alwaysOnLockdown");
return this;
}
/**
* “Connection type.”
*
* @param connectionType
* value of {@code connectionType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder connectionType(AndroidVpnConnectionType connectionType) {
this.connectionType = connectionType;
this.changedFields = changedFields.add("connectionType");
return this;
}
/**
* “Custom data to define key/value pairs specific to a VPN provider. This
* collection can contain a maximum of 25 elements.”
*
* @param customData
* value of {@code customData} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder customData(List customData) {
this.customData = customData;
this.changedFields = changedFields.add("customData");
return this;
}
/**
* “Custom data to define key/value pairs specific to a VPN provider. This
* collection can contain a maximum of 25 elements.”
*
* @param customData
* value of {@code customData} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder customData(KeyValue... customData) {
return customData(Arrays.asList(customData));
}
/**
* “Custom data to define key/value pairs specific to a VPN provider. This
* collection can contain a maximum of 25 elements.”
*
* @param customDataNextLink
* value of {@code customData@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder customDataNextLink(String customDataNextLink) {
this.customDataNextLink = customDataNextLink;
this.changedFields = changedFields.add("customData");
return this;
}
/**
* “Custom data to define key/value pairs specific to a VPN provider. This
* collection can contain a maximum of 25 elements.”
*
* @param customKeyValueData
* value of {@code customKeyValueData} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder customKeyValueData(List customKeyValueData) {
this.customKeyValueData = customKeyValueData;
this.changedFields = changedFields.add("customKeyValueData");
return this;
}
/**
* “Custom data to define key/value pairs specific to a VPN provider. This
* collection can contain a maximum of 25 elements.”
*
* @param customKeyValueData
* value of {@code customKeyValueData} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder customKeyValueData(KeyValuePair... customKeyValueData) {
return customKeyValueData(Arrays.asList(customKeyValueData));
}
/**
* “Custom data to define key/value pairs specific to a VPN provider. This
* collection can contain a maximum of 25 elements.”
*
* @param customKeyValueDataNextLink
* value of {@code customKeyValueData@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder customKeyValueDataNextLink(String customKeyValueDataNextLink) {
this.customKeyValueDataNextLink = customKeyValueDataNextLink;
this.changedFields = changedFields.add("customKeyValueData");
return this;
}
/**
* “Microsoft Tunnel site ID.”
*
* @param microsoftTunnelSiteId
* value of {@code microsoftTunnelSiteId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder microsoftTunnelSiteId(String microsoftTunnelSiteId) {
this.microsoftTunnelSiteId = microsoftTunnelSiteId;
this.changedFields = changedFields.add("microsoftTunnelSiteId");
return this;
}
/**
* “Proxy server.”
*
* @param proxyServer
* value of {@code proxyServer} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder proxyServer(VpnProxyServer proxyServer) {
this.proxyServer = proxyServer;
this.changedFields = changedFields.add("proxyServer");
return this;
}
/**
* “Targeted mobile apps. This collection can contain a maximum of 500 elements.”
*
* @param targetedMobileApps
* value of {@code targetedMobileApps} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder targetedMobileApps(List targetedMobileApps) {
this.targetedMobileApps = targetedMobileApps;
this.changedFields = changedFields.add("targetedMobileApps");
return this;
}
/**
* “Targeted mobile apps. This collection can contain a maximum of 500 elements.”
*
* @param targetedMobileApps
* value of {@code targetedMobileApps} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder targetedMobileApps(AppListItem... targetedMobileApps) {
return targetedMobileApps(Arrays.asList(targetedMobileApps));
}
/**
* “Targeted mobile apps. This collection can contain a maximum of 500 elements.”
*
* @param targetedMobileAppsNextLink
* value of {@code targetedMobileApps@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder targetedMobileAppsNextLink(String targetedMobileAppsNextLink) {
this.targetedMobileAppsNextLink = targetedMobileAppsNextLink;
this.changedFields = changedFields.add("targetedMobileApps");
return this;
}
/**
* “Targeted App package IDs.”
*
* @param targetedPackageIds
* value of {@code targetedPackageIds} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder targetedPackageIds(List targetedPackageIds) {
this.targetedPackageIds = targetedPackageIds;
this.changedFields = changedFields.add("targetedPackageIds");
return this;
}
/**
* “Targeted App package IDs.”
*
* @param targetedPackageIds
* value of {@code targetedPackageIds} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder targetedPackageIds(String... targetedPackageIds) {
return targetedPackageIds(Arrays.asList(targetedPackageIds));
}
/**
* “Targeted App package IDs.”
*
* @param targetedPackageIdsNextLink
* value of {@code targetedPackageIds@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder targetedPackageIdsNextLink(String targetedPackageIdsNextLink) {
this.targetedPackageIdsNextLink = targetedPackageIdsNextLink;
this.changedFields = changedFields.add("targetedPackageIds");
return this;
}
public AndroidDeviceOwnerVpnConfiguration build() {
AndroidDeviceOwnerVpnConfiguration _x = new AndroidDeviceOwnerVpnConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.androidDeviceOwnerVpnConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.authenticationMethod = authenticationMethod;
_x.connectionName = connectionName;
_x.realm = realm;
_x.role = role;
_x.servers = servers;
_x.serversNextLink = serversNextLink;
_x.alwaysOn = alwaysOn;
_x.alwaysOnLockdown = alwaysOnLockdown;
_x.connectionType = connectionType;
_x.customData = customData;
_x.customDataNextLink = customDataNextLink;
_x.customKeyValueData = customKeyValueData;
_x.customKeyValueDataNextLink = customKeyValueDataNextLink;
_x.microsoftTunnelSiteId = microsoftTunnelSiteId;
_x.proxyServer = proxyServer;
_x.targetedMobileApps = targetedMobileApps;
_x.targetedMobileAppsNextLink = targetedMobileAppsNextLink;
_x.targetedPackageIds = targetedPackageIds;
_x.targetedPackageIdsNextLink = targetedPackageIdsNextLink;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Whether or not to enable always-on VPN connection.”
*
* @return property alwaysOn
*/
@Property(name="alwaysOn")
@JsonIgnore
public Optional getAlwaysOn() {
return Optional.ofNullable(alwaysOn);
}
/**
* Returns an immutable copy of {@code this} with just the {@code alwaysOn} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Whether or not to enable always-on VPN connection.”
*
* @param alwaysOn
* new value of {@code alwaysOn} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code alwaysOn} field changed
*/
public AndroidDeviceOwnerVpnConfiguration withAlwaysOn(Boolean alwaysOn) {
AndroidDeviceOwnerVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("alwaysOn");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidDeviceOwnerVpnConfiguration");
_x.alwaysOn = alwaysOn;
return _x;
}
/**
* “If always-on VPN connection is enabled, whether or not to lock network traffic
* when that VPN is disconnected.”
*
* @return property alwaysOnLockdown
*/
@Property(name="alwaysOnLockdown")
@JsonIgnore
public Optional getAlwaysOnLockdown() {
return Optional.ofNullable(alwaysOnLockdown);
}
/**
* Returns an immutable copy of {@code this} with just the {@code alwaysOnLockdown}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “If always-on VPN connection is enabled, whether or not to lock network traffic
* when that VPN is disconnected.”
*
* @param alwaysOnLockdown
* new value of {@code alwaysOnLockdown} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code alwaysOnLockdown} field changed
*/
public AndroidDeviceOwnerVpnConfiguration withAlwaysOnLockdown(Boolean alwaysOnLockdown) {
AndroidDeviceOwnerVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("alwaysOnLockdown");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidDeviceOwnerVpnConfiguration");
_x.alwaysOnLockdown = alwaysOnLockdown;
return _x;
}
/**
* “Connection type.”
*
* @return property connectionType
*/
@Property(name="connectionType")
@JsonIgnore
public Optional getConnectionType() {
return Optional.ofNullable(connectionType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code connectionType}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Connection type.”
*
* @param connectionType
* new value of {@code connectionType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code connectionType} field changed
*/
public AndroidDeviceOwnerVpnConfiguration withConnectionType(AndroidVpnConnectionType connectionType) {
AndroidDeviceOwnerVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("connectionType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidDeviceOwnerVpnConfiguration");
_x.connectionType = connectionType;
return _x;
}
/**
* “Custom data to define key/value pairs specific to a VPN provider. This
* collection can contain a maximum of 25 elements.”
*
* @return property customData
*/
@Property(name="customData")
@JsonIgnore
public CollectionPage getCustomData() {
return new CollectionPage(contextPath, KeyValue.class, this.customData, Optional.ofNullable(customDataNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code customData} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Custom data to define key/value pairs specific to a VPN provider. This
* collection can contain a maximum of 25 elements.”
*
* @param customData
* new value of {@code customData} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code customData} field changed
*/
public AndroidDeviceOwnerVpnConfiguration withCustomData(List customData) {
AndroidDeviceOwnerVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("customData");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidDeviceOwnerVpnConfiguration");
_x.customData = customData;
return _x;
}
/**
* “Custom data to define key/value pairs specific to a VPN provider. This
* collection can contain a maximum of 25 elements.”
*
* @param options
* specify connect and read timeouts
* @return property customData
*/
@Property(name="customData")
@JsonIgnore
public CollectionPage getCustomData(HttpRequestOptions options) {
return new CollectionPage(contextPath, KeyValue.class, this.customData, Optional.ofNullable(customDataNextLink), Collections.emptyList(), options);
}
/**
* “Custom data to define key/value pairs specific to a VPN provider. This
* collection can contain a maximum of 25 elements.”
*
* @return property customKeyValueData
*/
@Property(name="customKeyValueData")
@JsonIgnore
public CollectionPage getCustomKeyValueData() {
return new CollectionPage(contextPath, KeyValuePair.class, this.customKeyValueData, Optional.ofNullable(customKeyValueDataNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* customKeyValueData} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Custom data to define key/value pairs specific to a VPN provider. This
* collection can contain a maximum of 25 elements.”
*
* @param customKeyValueData
* new value of {@code customKeyValueData} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code customKeyValueData} field changed
*/
public AndroidDeviceOwnerVpnConfiguration withCustomKeyValueData(List customKeyValueData) {
AndroidDeviceOwnerVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("customKeyValueData");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidDeviceOwnerVpnConfiguration");
_x.customKeyValueData = customKeyValueData;
return _x;
}
/**
* “Custom data to define key/value pairs specific to a VPN provider. This
* collection can contain a maximum of 25 elements.”
*
* @param options
* specify connect and read timeouts
* @return property customKeyValueData
*/
@Property(name="customKeyValueData")
@JsonIgnore
public CollectionPage getCustomKeyValueData(HttpRequestOptions options) {
return new CollectionPage(contextPath, KeyValuePair.class, this.customKeyValueData, Optional.ofNullable(customKeyValueDataNextLink), Collections.emptyList(), options);
}
/**
* “Microsoft Tunnel site ID.”
*
* @return property microsoftTunnelSiteId
*/
@Property(name="microsoftTunnelSiteId")
@JsonIgnore
public Optional getMicrosoftTunnelSiteId() {
return Optional.ofNullable(microsoftTunnelSiteId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* microsoftTunnelSiteId} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Microsoft Tunnel site ID.”
*
* @param microsoftTunnelSiteId
* new value of {@code microsoftTunnelSiteId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code microsoftTunnelSiteId} field changed
*/
public AndroidDeviceOwnerVpnConfiguration withMicrosoftTunnelSiteId(String microsoftTunnelSiteId) {
AndroidDeviceOwnerVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("microsoftTunnelSiteId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidDeviceOwnerVpnConfiguration");
_x.microsoftTunnelSiteId = microsoftTunnelSiteId;
return _x;
}
/**
* “Proxy server.”
*
* @return property proxyServer
*/
@Property(name="proxyServer")
@JsonIgnore
public Optional getProxyServer() {
return Optional.ofNullable(proxyServer);
}
/**
* Returns an immutable copy of {@code this} with just the {@code proxyServer}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Proxy server.”
*
* @param proxyServer
* new value of {@code proxyServer} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code proxyServer} field changed
*/
public AndroidDeviceOwnerVpnConfiguration withProxyServer(VpnProxyServer proxyServer) {
AndroidDeviceOwnerVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("proxyServer");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidDeviceOwnerVpnConfiguration");
_x.proxyServer = proxyServer;
return _x;
}
/**
* “Targeted mobile apps. This collection can contain a maximum of 500 elements.”
*
* @return property targetedMobileApps
*/
@Property(name="targetedMobileApps")
@JsonIgnore
public CollectionPage getTargetedMobileApps() {
return new CollectionPage(contextPath, AppListItem.class, this.targetedMobileApps, Optional.ofNullable(targetedMobileAppsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* targetedMobileApps} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Targeted mobile apps. This collection can contain a maximum of 500 elements.”
*
* @param targetedMobileApps
* new value of {@code targetedMobileApps} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code targetedMobileApps} field changed
*/
public AndroidDeviceOwnerVpnConfiguration withTargetedMobileApps(List targetedMobileApps) {
AndroidDeviceOwnerVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("targetedMobileApps");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidDeviceOwnerVpnConfiguration");
_x.targetedMobileApps = targetedMobileApps;
return _x;
}
/**
* “Targeted mobile apps. This collection can contain a maximum of 500 elements.”
*
* @param options
* specify connect and read timeouts
* @return property targetedMobileApps
*/
@Property(name="targetedMobileApps")
@JsonIgnore
public CollectionPage getTargetedMobileApps(HttpRequestOptions options) {
return new CollectionPage(contextPath, AppListItem.class, this.targetedMobileApps, Optional.ofNullable(targetedMobileAppsNextLink), Collections.emptyList(), options);
}
/**
* “Targeted App package IDs.”
*
* @return property targetedPackageIds
*/
@Property(name="targetedPackageIds")
@JsonIgnore
public CollectionPage getTargetedPackageIds() {
return new CollectionPage(contextPath, String.class, this.targetedPackageIds, Optional.ofNullable(targetedPackageIdsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* targetedPackageIds} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Targeted App package IDs.”
*
* @param targetedPackageIds
* new value of {@code targetedPackageIds} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code targetedPackageIds} field changed
*/
public AndroidDeviceOwnerVpnConfiguration withTargetedPackageIds(List targetedPackageIds) {
AndroidDeviceOwnerVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("targetedPackageIds");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidDeviceOwnerVpnConfiguration");
_x.targetedPackageIds = targetedPackageIds;
return _x;
}
/**
* “Targeted App package IDs.”
*
* @param options
* specify connect and read timeouts
* @return property targetedPackageIds
*/
@Property(name="targetedPackageIds")
@JsonIgnore
public CollectionPage getTargetedPackageIds(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.targetedPackageIds, Optional.ofNullable(targetedPackageIdsNextLink), Collections.emptyList(), options);
}
public AndroidDeviceOwnerVpnConfiguration withUnmappedField(String name, String value) {
AndroidDeviceOwnerVpnConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “Tenant level settings for the Derived Credentials to be used for authentication.”
*
* @return navigational property derivedCredentialSettings
*/
@NavigationProperty(name="derivedCredentialSettings")
@JsonIgnore
public DeviceManagementDerivedCredentialSettingsRequest getDerivedCredentialSettings() {
return new DeviceManagementDerivedCredentialSettingsRequest(contextPath.addSegment("derivedCredentialSettings"), RequestHelper.getValue(unmappedFields, "derivedCredentialSettings"));
}
/**
* “Identity certificate for client authentication when authentication method is
* certificate.”
*
* @return navigational property identityCertificate
*/
@NavigationProperty(name="identityCertificate")
@JsonIgnore
public AndroidDeviceOwnerCertificateProfileBaseRequest getIdentityCertificate() {
return new AndroidDeviceOwnerCertificateProfileBaseRequest(contextPath.addSegment("identityCertificate"), RequestHelper.getValue(unmappedFields, "identityCertificate"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public AndroidDeviceOwnerVpnConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
AndroidDeviceOwnerVpnConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public AndroidDeviceOwnerVpnConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
AndroidDeviceOwnerVpnConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private AndroidDeviceOwnerVpnConfiguration _copy() {
AndroidDeviceOwnerVpnConfiguration _x = new AndroidDeviceOwnerVpnConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.authenticationMethod = authenticationMethod;
_x.connectionName = connectionName;
_x.realm = realm;
_x.role = role;
_x.servers = servers;
_x.alwaysOn = alwaysOn;
_x.alwaysOnLockdown = alwaysOnLockdown;
_x.connectionType = connectionType;
_x.customData = customData;
_x.customKeyValueData = customKeyValueData;
_x.microsoftTunnelSiteId = microsoftTunnelSiteId;
_x.proxyServer = proxyServer;
_x.targetedMobileApps = targetedMobileApps;
_x.targetedPackageIds = targetedPackageIds;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("AndroidDeviceOwnerVpnConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("deviceManagementApplicabilityRuleDeviceMode=");
b.append(this.deviceManagementApplicabilityRuleDeviceMode);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsEdition=");
b.append(this.deviceManagementApplicabilityRuleOsEdition);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsVersion=");
b.append(this.deviceManagementApplicabilityRuleOsVersion);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("supportsScopeTags=");
b.append(this.supportsScopeTags);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("authenticationMethod=");
b.append(this.authenticationMethod);
b.append(", ");
b.append("connectionName=");
b.append(this.connectionName);
b.append(", ");
b.append("realm=");
b.append(this.realm);
b.append(", ");
b.append("role=");
b.append(this.role);
b.append(", ");
b.append("servers=");
b.append(this.servers);
b.append(", ");
b.append("alwaysOn=");
b.append(this.alwaysOn);
b.append(", ");
b.append("alwaysOnLockdown=");
b.append(this.alwaysOnLockdown);
b.append(", ");
b.append("connectionType=");
b.append(this.connectionType);
b.append(", ");
b.append("customData=");
b.append(this.customData);
b.append(", ");
b.append("customKeyValueData=");
b.append(this.customKeyValueData);
b.append(", ");
b.append("microsoftTunnelSiteId=");
b.append(this.microsoftTunnelSiteId);
b.append(", ");
b.append("proxyServer=");
b.append(this.proxyServer);
b.append(", ");
b.append("targetedMobileApps=");
b.append(this.targetedMobileApps);
b.append(", ");
b.append("targetedPackageIds=");
b.append(this.targetedPackageIds);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}