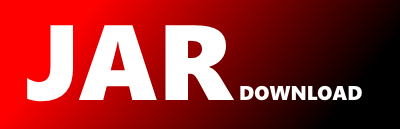
odata.msgraph.client.beta.entity.AndroidForWorkGeneralDeviceConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleDeviceMode;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsEdition;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsVersion;
import odata.msgraph.client.beta.enums.AndroidForWorkCrossProfileDataSharingType;
import odata.msgraph.client.beta.enums.AndroidForWorkDefaultAppPermissionPolicyType;
import odata.msgraph.client.beta.enums.AndroidForWorkRequiredPasswordType;
/**
* “Android For Work general device configuration.”
*/@JsonPropertyOrder({
"@odata.type",
"passwordBlockFaceUnlock",
"passwordBlockFingerprintUnlock",
"passwordBlockIrisUnlock",
"passwordBlockTrustAgents",
"passwordExpirationDays",
"passwordMinimumLength",
"passwordMinutesOfInactivityBeforeScreenTimeout",
"passwordPreviousPasswordBlockCount",
"passwordRequiredType",
"passwordSignInFailureCountBeforeFactoryReset",
"securityRequireVerifyApps",
"vpnAlwaysOnPackageIdentifier",
"vpnEnableAlwaysOnLockdownMode",
"workProfileAllowWidgets",
"workProfileBlockAddingAccounts",
"workProfileBlockCamera",
"workProfileBlockCrossProfileCallerId",
"workProfileBlockCrossProfileContactsSearch",
"workProfileBlockCrossProfileCopyPaste",
"workProfileBlockNotificationsWhileDeviceLocked",
"workProfileBlockPersonalAppInstallsFromUnknownSources",
"workProfileBlockScreenCapture",
"workProfileBluetoothEnableContactSharing",
"workProfileDataSharingType",
"workProfileDefaultAppPermissionPolicy",
"workProfilePasswordBlockFaceUnlock",
"workProfilePasswordBlockFingerprintUnlock",
"workProfilePasswordBlockIrisUnlock",
"workProfilePasswordBlockTrustAgents",
"workProfilePasswordExpirationDays",
"workProfilePasswordMinimumLength",
"workProfilePasswordMinLetterCharacters",
"workProfilePasswordMinLowerCaseCharacters",
"workProfilePasswordMinNonLetterCharacters",
"workProfilePasswordMinNumericCharacters",
"workProfilePasswordMinSymbolCharacters",
"workProfilePasswordMinUpperCaseCharacters",
"workProfilePasswordMinutesOfInactivityBeforeScreenTimeout",
"workProfilePasswordPreviousPasswordBlockCount",
"workProfilePasswordRequiredType",
"workProfilePasswordSignInFailureCountBeforeFactoryReset",
"workProfileRequirePassword"})
@JsonInclude(Include.NON_NULL)
public class AndroidForWorkGeneralDeviceConfiguration extends DeviceConfiguration implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.androidForWorkGeneralDeviceConfiguration";
}
@JsonProperty("passwordBlockFaceUnlock")
protected Boolean passwordBlockFaceUnlock;
@JsonProperty("passwordBlockFingerprintUnlock")
protected Boolean passwordBlockFingerprintUnlock;
@JsonProperty("passwordBlockIrisUnlock")
protected Boolean passwordBlockIrisUnlock;
@JsonProperty("passwordBlockTrustAgents")
protected Boolean passwordBlockTrustAgents;
@JsonProperty("passwordExpirationDays")
protected Integer passwordExpirationDays;
@JsonProperty("passwordMinimumLength")
protected Integer passwordMinimumLength;
@JsonProperty("passwordMinutesOfInactivityBeforeScreenTimeout")
protected Integer passwordMinutesOfInactivityBeforeScreenTimeout;
@JsonProperty("passwordPreviousPasswordBlockCount")
protected Integer passwordPreviousPasswordBlockCount;
@JsonProperty("passwordRequiredType")
protected AndroidForWorkRequiredPasswordType passwordRequiredType;
@JsonProperty("passwordSignInFailureCountBeforeFactoryReset")
protected Integer passwordSignInFailureCountBeforeFactoryReset;
@JsonProperty("securityRequireVerifyApps")
protected Boolean securityRequireVerifyApps;
@JsonProperty("vpnAlwaysOnPackageIdentifier")
protected String vpnAlwaysOnPackageIdentifier;
@JsonProperty("vpnEnableAlwaysOnLockdownMode")
protected Boolean vpnEnableAlwaysOnLockdownMode;
@JsonProperty("workProfileAllowWidgets")
protected Boolean workProfileAllowWidgets;
@JsonProperty("workProfileBlockAddingAccounts")
protected Boolean workProfileBlockAddingAccounts;
@JsonProperty("workProfileBlockCamera")
protected Boolean workProfileBlockCamera;
@JsonProperty("workProfileBlockCrossProfileCallerId")
protected Boolean workProfileBlockCrossProfileCallerId;
@JsonProperty("workProfileBlockCrossProfileContactsSearch")
protected Boolean workProfileBlockCrossProfileContactsSearch;
@JsonProperty("workProfileBlockCrossProfileCopyPaste")
protected Boolean workProfileBlockCrossProfileCopyPaste;
@JsonProperty("workProfileBlockNotificationsWhileDeviceLocked")
protected Boolean workProfileBlockNotificationsWhileDeviceLocked;
@JsonProperty("workProfileBlockPersonalAppInstallsFromUnknownSources")
protected Boolean workProfileBlockPersonalAppInstallsFromUnknownSources;
@JsonProperty("workProfileBlockScreenCapture")
protected Boolean workProfileBlockScreenCapture;
@JsonProperty("workProfileBluetoothEnableContactSharing")
protected Boolean workProfileBluetoothEnableContactSharing;
@JsonProperty("workProfileDataSharingType")
protected AndroidForWorkCrossProfileDataSharingType workProfileDataSharingType;
@JsonProperty("workProfileDefaultAppPermissionPolicy")
protected AndroidForWorkDefaultAppPermissionPolicyType workProfileDefaultAppPermissionPolicy;
@JsonProperty("workProfilePasswordBlockFaceUnlock")
protected Boolean workProfilePasswordBlockFaceUnlock;
@JsonProperty("workProfilePasswordBlockFingerprintUnlock")
protected Boolean workProfilePasswordBlockFingerprintUnlock;
@JsonProperty("workProfilePasswordBlockIrisUnlock")
protected Boolean workProfilePasswordBlockIrisUnlock;
@JsonProperty("workProfilePasswordBlockTrustAgents")
protected Boolean workProfilePasswordBlockTrustAgents;
@JsonProperty("workProfilePasswordExpirationDays")
protected Integer workProfilePasswordExpirationDays;
@JsonProperty("workProfilePasswordMinimumLength")
protected Integer workProfilePasswordMinimumLength;
@JsonProperty("workProfilePasswordMinLetterCharacters")
protected Integer workProfilePasswordMinLetterCharacters;
@JsonProperty("workProfilePasswordMinLowerCaseCharacters")
protected Integer workProfilePasswordMinLowerCaseCharacters;
@JsonProperty("workProfilePasswordMinNonLetterCharacters")
protected Integer workProfilePasswordMinNonLetterCharacters;
@JsonProperty("workProfilePasswordMinNumericCharacters")
protected Integer workProfilePasswordMinNumericCharacters;
@JsonProperty("workProfilePasswordMinSymbolCharacters")
protected Integer workProfilePasswordMinSymbolCharacters;
@JsonProperty("workProfilePasswordMinUpperCaseCharacters")
protected Integer workProfilePasswordMinUpperCaseCharacters;
@JsonProperty("workProfilePasswordMinutesOfInactivityBeforeScreenTimeout")
protected Integer workProfilePasswordMinutesOfInactivityBeforeScreenTimeout;
@JsonProperty("workProfilePasswordPreviousPasswordBlockCount")
protected Integer workProfilePasswordPreviousPasswordBlockCount;
@JsonProperty("workProfilePasswordRequiredType")
protected AndroidForWorkRequiredPasswordType workProfilePasswordRequiredType;
@JsonProperty("workProfilePasswordSignInFailureCountBeforeFactoryReset")
protected Integer workProfilePasswordSignInFailureCountBeforeFactoryReset;
@JsonProperty("workProfileRequirePassword")
protected Boolean workProfileRequirePassword;
protected AndroidForWorkGeneralDeviceConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderAndroidForWorkGeneralDeviceConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode;
private DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition;
private DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private Boolean supportsScopeTags;
private Integer version;
private Boolean passwordBlockFaceUnlock;
private Boolean passwordBlockFingerprintUnlock;
private Boolean passwordBlockIrisUnlock;
private Boolean passwordBlockTrustAgents;
private Integer passwordExpirationDays;
private Integer passwordMinimumLength;
private Integer passwordMinutesOfInactivityBeforeScreenTimeout;
private Integer passwordPreviousPasswordBlockCount;
private AndroidForWorkRequiredPasswordType passwordRequiredType;
private Integer passwordSignInFailureCountBeforeFactoryReset;
private Boolean securityRequireVerifyApps;
private String vpnAlwaysOnPackageIdentifier;
private Boolean vpnEnableAlwaysOnLockdownMode;
private Boolean workProfileAllowWidgets;
private Boolean workProfileBlockAddingAccounts;
private Boolean workProfileBlockCamera;
private Boolean workProfileBlockCrossProfileCallerId;
private Boolean workProfileBlockCrossProfileContactsSearch;
private Boolean workProfileBlockCrossProfileCopyPaste;
private Boolean workProfileBlockNotificationsWhileDeviceLocked;
private Boolean workProfileBlockPersonalAppInstallsFromUnknownSources;
private Boolean workProfileBlockScreenCapture;
private Boolean workProfileBluetoothEnableContactSharing;
private AndroidForWorkCrossProfileDataSharingType workProfileDataSharingType;
private AndroidForWorkDefaultAppPermissionPolicyType workProfileDefaultAppPermissionPolicy;
private Boolean workProfilePasswordBlockFaceUnlock;
private Boolean workProfilePasswordBlockFingerprintUnlock;
private Boolean workProfilePasswordBlockIrisUnlock;
private Boolean workProfilePasswordBlockTrustAgents;
private Integer workProfilePasswordExpirationDays;
private Integer workProfilePasswordMinimumLength;
private Integer workProfilePasswordMinLetterCharacters;
private Integer workProfilePasswordMinLowerCaseCharacters;
private Integer workProfilePasswordMinNonLetterCharacters;
private Integer workProfilePasswordMinNumericCharacters;
private Integer workProfilePasswordMinSymbolCharacters;
private Integer workProfilePasswordMinUpperCaseCharacters;
private Integer workProfilePasswordMinutesOfInactivityBeforeScreenTimeout;
private Integer workProfilePasswordPreviousPasswordBlockCount;
private AndroidForWorkRequiredPasswordType workProfilePasswordRequiredType;
private Integer workProfilePasswordSignInFailureCountBeforeFactoryReset;
private Boolean workProfileRequirePassword;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder deviceManagementApplicabilityRuleDeviceMode(DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode) {
this.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleDeviceMode");
return this;
}
public Builder deviceManagementApplicabilityRuleOsEdition(DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition) {
this.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsEdition");
return this;
}
public Builder deviceManagementApplicabilityRuleOsVersion(DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion) {
this.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsVersion");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder supportsScopeTags(Boolean supportsScopeTags) {
this.supportsScopeTags = supportsScopeTags;
this.changedFields = changedFields.add("supportsScopeTags");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
/**
* “Indicates whether or not to block face unlock.”
*
* @param passwordBlockFaceUnlock
* value of {@code passwordBlockFaceUnlock} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordBlockFaceUnlock(Boolean passwordBlockFaceUnlock) {
this.passwordBlockFaceUnlock = passwordBlockFaceUnlock;
this.changedFields = changedFields.add("passwordBlockFaceUnlock");
return this;
}
/**
* “Indicates whether or not to block fingerprint unlock.”
*
* @param passwordBlockFingerprintUnlock
* value of {@code passwordBlockFingerprintUnlock} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordBlockFingerprintUnlock(Boolean passwordBlockFingerprintUnlock) {
this.passwordBlockFingerprintUnlock = passwordBlockFingerprintUnlock;
this.changedFields = changedFields.add("passwordBlockFingerprintUnlock");
return this;
}
/**
* “Indicates whether or not to block iris unlock.”
*
* @param passwordBlockIrisUnlock
* value of {@code passwordBlockIrisUnlock} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordBlockIrisUnlock(Boolean passwordBlockIrisUnlock) {
this.passwordBlockIrisUnlock = passwordBlockIrisUnlock;
this.changedFields = changedFields.add("passwordBlockIrisUnlock");
return this;
}
/**
* “Indicates whether or not to block Smart Lock and other trust agents.”
*
* @param passwordBlockTrustAgents
* value of {@code passwordBlockTrustAgents} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordBlockTrustAgents(Boolean passwordBlockTrustAgents) {
this.passwordBlockTrustAgents = passwordBlockTrustAgents;
this.changedFields = changedFields.add("passwordBlockTrustAgents");
return this;
}
/**
* “Number of days before the password expires. Valid values 1 to 365”
*
* @param passwordExpirationDays
* value of {@code passwordExpirationDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordExpirationDays(Integer passwordExpirationDays) {
this.passwordExpirationDays = passwordExpirationDays;
this.changedFields = changedFields.add("passwordExpirationDays");
return this;
}
/**
* “Minimum length of passwords. Valid values 4 to 16”
*
* @param passwordMinimumLength
* value of {@code passwordMinimumLength} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordMinimumLength(Integer passwordMinimumLength) {
this.passwordMinimumLength = passwordMinimumLength;
this.changedFields = changedFields.add("passwordMinimumLength");
return this;
}
/**
* “Minutes of inactivity before the screen times out.”
*
* @param passwordMinutesOfInactivityBeforeScreenTimeout
* value of {@code passwordMinutesOfInactivityBeforeScreenTimeout} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordMinutesOfInactivityBeforeScreenTimeout(Integer passwordMinutesOfInactivityBeforeScreenTimeout) {
this.passwordMinutesOfInactivityBeforeScreenTimeout = passwordMinutesOfInactivityBeforeScreenTimeout;
this.changedFields = changedFields.add("passwordMinutesOfInactivityBeforeScreenTimeout");
return this;
}
/**
* “Number of previous passwords to block. Valid values 0 to 24”
*
* @param passwordPreviousPasswordBlockCount
* value of {@code passwordPreviousPasswordBlockCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordPreviousPasswordBlockCount(Integer passwordPreviousPasswordBlockCount) {
this.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
this.changedFields = changedFields.add("passwordPreviousPasswordBlockCount");
return this;
}
/**
* “Type of password that is required.”
*
* @param passwordRequiredType
* value of {@code passwordRequiredType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordRequiredType(AndroidForWorkRequiredPasswordType passwordRequiredType) {
this.passwordRequiredType = passwordRequiredType;
this.changedFields = changedFields.add("passwordRequiredType");
return this;
}
/**
* “Number of sign in failures allowed before factory reset. Valid values 1 to 16”
*
* @param passwordSignInFailureCountBeforeFactoryReset
* value of {@code passwordSignInFailureCountBeforeFactoryReset} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordSignInFailureCountBeforeFactoryReset(Integer passwordSignInFailureCountBeforeFactoryReset) {
this.passwordSignInFailureCountBeforeFactoryReset = passwordSignInFailureCountBeforeFactoryReset;
this.changedFields = changedFields.add("passwordSignInFailureCountBeforeFactoryReset");
return this;
}
/**
* “Require the Android Verify apps feature is turned on.”
*
* @param securityRequireVerifyApps
* value of {@code securityRequireVerifyApps} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder securityRequireVerifyApps(Boolean securityRequireVerifyApps) {
this.securityRequireVerifyApps = securityRequireVerifyApps;
this.changedFields = changedFields.add("securityRequireVerifyApps");
return this;
}
/**
* “Enable lockdown mode for always-on VPN.”
*
* @param vpnAlwaysOnPackageIdentifier
* value of {@code vpnAlwaysOnPackageIdentifier} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder vpnAlwaysOnPackageIdentifier(String vpnAlwaysOnPackageIdentifier) {
this.vpnAlwaysOnPackageIdentifier = vpnAlwaysOnPackageIdentifier;
this.changedFields = changedFields.add("vpnAlwaysOnPackageIdentifier");
return this;
}
/**
* “Enable lockdown mode for always-on VPN.”
*
* @param vpnEnableAlwaysOnLockdownMode
* value of {@code vpnEnableAlwaysOnLockdownMode} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder vpnEnableAlwaysOnLockdownMode(Boolean vpnEnableAlwaysOnLockdownMode) {
this.vpnEnableAlwaysOnLockdownMode = vpnEnableAlwaysOnLockdownMode;
this.changedFields = changedFields.add("vpnEnableAlwaysOnLockdownMode");
return this;
}
/**
* “Allow widgets from work profile apps.”
*
* @param workProfileAllowWidgets
* value of {@code workProfileAllowWidgets} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileAllowWidgets(Boolean workProfileAllowWidgets) {
this.workProfileAllowWidgets = workProfileAllowWidgets;
this.changedFields = changedFields.add("workProfileAllowWidgets");
return this;
}
/**
* “Block users from adding/removing accounts in work profile.”
*
* @param workProfileBlockAddingAccounts
* value of {@code workProfileBlockAddingAccounts} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileBlockAddingAccounts(Boolean workProfileBlockAddingAccounts) {
this.workProfileBlockAddingAccounts = workProfileBlockAddingAccounts;
this.changedFields = changedFields.add("workProfileBlockAddingAccounts");
return this;
}
/**
* “Block work profile camera.”
*
* @param workProfileBlockCamera
* value of {@code workProfileBlockCamera} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileBlockCamera(Boolean workProfileBlockCamera) {
this.workProfileBlockCamera = workProfileBlockCamera;
this.changedFields = changedFields.add("workProfileBlockCamera");
return this;
}
/**
* “Block display work profile caller ID in personal profile.”
*
* @param workProfileBlockCrossProfileCallerId
* value of {@code workProfileBlockCrossProfileCallerId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileBlockCrossProfileCallerId(Boolean workProfileBlockCrossProfileCallerId) {
this.workProfileBlockCrossProfileCallerId = workProfileBlockCrossProfileCallerId;
this.changedFields = changedFields.add("workProfileBlockCrossProfileCallerId");
return this;
}
/**
* “Block work profile contacts availability in personal profile.”
*
* @param workProfileBlockCrossProfileContactsSearch
* value of {@code workProfileBlockCrossProfileContactsSearch} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileBlockCrossProfileContactsSearch(Boolean workProfileBlockCrossProfileContactsSearch) {
this.workProfileBlockCrossProfileContactsSearch = workProfileBlockCrossProfileContactsSearch;
this.changedFields = changedFields.add("workProfileBlockCrossProfileContactsSearch");
return this;
}
/**
* “Boolean that indicates if the setting disallow cross profile copy/paste is
* enabled.”
*
* @param workProfileBlockCrossProfileCopyPaste
* value of {@code workProfileBlockCrossProfileCopyPaste} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileBlockCrossProfileCopyPaste(Boolean workProfileBlockCrossProfileCopyPaste) {
this.workProfileBlockCrossProfileCopyPaste = workProfileBlockCrossProfileCopyPaste;
this.changedFields = changedFields.add("workProfileBlockCrossProfileCopyPaste");
return this;
}
/**
* “Indicates whether or not to block notifications while device locked.”
*
* @param workProfileBlockNotificationsWhileDeviceLocked
* value of {@code workProfileBlockNotificationsWhileDeviceLocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileBlockNotificationsWhileDeviceLocked(Boolean workProfileBlockNotificationsWhileDeviceLocked) {
this.workProfileBlockNotificationsWhileDeviceLocked = workProfileBlockNotificationsWhileDeviceLocked;
this.changedFields = changedFields.add("workProfileBlockNotificationsWhileDeviceLocked");
return this;
}
/**
* “Prevent app installations from unknown sources in the personal profile.”
*
* @param workProfileBlockPersonalAppInstallsFromUnknownSources
* value of {@code workProfileBlockPersonalAppInstallsFromUnknownSources} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileBlockPersonalAppInstallsFromUnknownSources(Boolean workProfileBlockPersonalAppInstallsFromUnknownSources) {
this.workProfileBlockPersonalAppInstallsFromUnknownSources = workProfileBlockPersonalAppInstallsFromUnknownSources;
this.changedFields = changedFields.add("workProfileBlockPersonalAppInstallsFromUnknownSources");
return this;
}
/**
* “Block screen capture in work profile.”
*
* @param workProfileBlockScreenCapture
* value of {@code workProfileBlockScreenCapture} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileBlockScreenCapture(Boolean workProfileBlockScreenCapture) {
this.workProfileBlockScreenCapture = workProfileBlockScreenCapture;
this.changedFields = changedFields.add("workProfileBlockScreenCapture");
return this;
}
/**
* “Allow bluetooth devices to access enterprise contacts.”
*
* @param workProfileBluetoothEnableContactSharing
* value of {@code workProfileBluetoothEnableContactSharing} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileBluetoothEnableContactSharing(Boolean workProfileBluetoothEnableContactSharing) {
this.workProfileBluetoothEnableContactSharing = workProfileBluetoothEnableContactSharing;
this.changedFields = changedFields.add("workProfileBluetoothEnableContactSharing");
return this;
}
/**
* “Type of data sharing that is allowed.”
*
* @param workProfileDataSharingType
* value of {@code workProfileDataSharingType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileDataSharingType(AndroidForWorkCrossProfileDataSharingType workProfileDataSharingType) {
this.workProfileDataSharingType = workProfileDataSharingType;
this.changedFields = changedFields.add("workProfileDataSharingType");
return this;
}
/**
* “Type of password that is required.”
*
* @param workProfileDefaultAppPermissionPolicy
* value of {@code workProfileDefaultAppPermissionPolicy} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileDefaultAppPermissionPolicy(AndroidForWorkDefaultAppPermissionPolicyType workProfileDefaultAppPermissionPolicy) {
this.workProfileDefaultAppPermissionPolicy = workProfileDefaultAppPermissionPolicy;
this.changedFields = changedFields.add("workProfileDefaultAppPermissionPolicy");
return this;
}
/**
* “Indicates whether or not to block face unlock for work profile.”
*
* @param workProfilePasswordBlockFaceUnlock
* value of {@code workProfilePasswordBlockFaceUnlock} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordBlockFaceUnlock(Boolean workProfilePasswordBlockFaceUnlock) {
this.workProfilePasswordBlockFaceUnlock = workProfilePasswordBlockFaceUnlock;
this.changedFields = changedFields.add("workProfilePasswordBlockFaceUnlock");
return this;
}
/**
* “Indicates whether or not to block fingerprint unlock for work profile.”
*
* @param workProfilePasswordBlockFingerprintUnlock
* value of {@code workProfilePasswordBlockFingerprintUnlock} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordBlockFingerprintUnlock(Boolean workProfilePasswordBlockFingerprintUnlock) {
this.workProfilePasswordBlockFingerprintUnlock = workProfilePasswordBlockFingerprintUnlock;
this.changedFields = changedFields.add("workProfilePasswordBlockFingerprintUnlock");
return this;
}
/**
* “Indicates whether or not to block iris unlock for work profile.”
*
* @param workProfilePasswordBlockIrisUnlock
* value of {@code workProfilePasswordBlockIrisUnlock} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordBlockIrisUnlock(Boolean workProfilePasswordBlockIrisUnlock) {
this.workProfilePasswordBlockIrisUnlock = workProfilePasswordBlockIrisUnlock;
this.changedFields = changedFields.add("workProfilePasswordBlockIrisUnlock");
return this;
}
/**
* “Indicates whether or not to block Smart Lock and other trust agents for work
* profile.”
*
* @param workProfilePasswordBlockTrustAgents
* value of {@code workProfilePasswordBlockTrustAgents} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordBlockTrustAgents(Boolean workProfilePasswordBlockTrustAgents) {
this.workProfilePasswordBlockTrustAgents = workProfilePasswordBlockTrustAgents;
this.changedFields = changedFields.add("workProfilePasswordBlockTrustAgents");
return this;
}
/**
* “Number of days before the work profile password expires. Valid values 1 to 365”
*
* @param workProfilePasswordExpirationDays
* value of {@code workProfilePasswordExpirationDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordExpirationDays(Integer workProfilePasswordExpirationDays) {
this.workProfilePasswordExpirationDays = workProfilePasswordExpirationDays;
this.changedFields = changedFields.add("workProfilePasswordExpirationDays");
return this;
}
/**
* “Minimum length of work profile password. Valid values 4 to 16”
*
* @param workProfilePasswordMinimumLength
* value of {@code workProfilePasswordMinimumLength} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordMinimumLength(Integer workProfilePasswordMinimumLength) {
this.workProfilePasswordMinimumLength = workProfilePasswordMinimumLength;
this.changedFields = changedFields.add("workProfilePasswordMinimumLength");
return this;
}
/**
* “Minimum # of letter characters required in work profile password. Valid values 1
* to 10”
*
* @param workProfilePasswordMinLetterCharacters
* value of {@code workProfilePasswordMinLetterCharacters} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordMinLetterCharacters(Integer workProfilePasswordMinLetterCharacters) {
this.workProfilePasswordMinLetterCharacters = workProfilePasswordMinLetterCharacters;
this.changedFields = changedFields.add("workProfilePasswordMinLetterCharacters");
return this;
}
/**
* “Minimum # of lower-case characters required in work profile password. Valid
* values 1 to 10”
*
* @param workProfilePasswordMinLowerCaseCharacters
* value of {@code workProfilePasswordMinLowerCaseCharacters} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordMinLowerCaseCharacters(Integer workProfilePasswordMinLowerCaseCharacters) {
this.workProfilePasswordMinLowerCaseCharacters = workProfilePasswordMinLowerCaseCharacters;
this.changedFields = changedFields.add("workProfilePasswordMinLowerCaseCharacters");
return this;
}
/**
* “Minimum # of non-letter characters required in work profile password. Valid
* values 1 to 10”
*
* @param workProfilePasswordMinNonLetterCharacters
* value of {@code workProfilePasswordMinNonLetterCharacters} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordMinNonLetterCharacters(Integer workProfilePasswordMinNonLetterCharacters) {
this.workProfilePasswordMinNonLetterCharacters = workProfilePasswordMinNonLetterCharacters;
this.changedFields = changedFields.add("workProfilePasswordMinNonLetterCharacters");
return this;
}
/**
* “Minimum # of numeric characters required in work profile password. Valid values
* 1 to 10”
*
* @param workProfilePasswordMinNumericCharacters
* value of {@code workProfilePasswordMinNumericCharacters} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordMinNumericCharacters(Integer workProfilePasswordMinNumericCharacters) {
this.workProfilePasswordMinNumericCharacters = workProfilePasswordMinNumericCharacters;
this.changedFields = changedFields.add("workProfilePasswordMinNumericCharacters");
return this;
}
/**
* “Minimum # of symbols required in work profile password. Valid values 1 to 10”
*
* @param workProfilePasswordMinSymbolCharacters
* value of {@code workProfilePasswordMinSymbolCharacters} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordMinSymbolCharacters(Integer workProfilePasswordMinSymbolCharacters) {
this.workProfilePasswordMinSymbolCharacters = workProfilePasswordMinSymbolCharacters;
this.changedFields = changedFields.add("workProfilePasswordMinSymbolCharacters");
return this;
}
/**
* “Minimum # of upper-case characters required in work profile password. Valid
* values 1 to 10”
*
* @param workProfilePasswordMinUpperCaseCharacters
* value of {@code workProfilePasswordMinUpperCaseCharacters} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordMinUpperCaseCharacters(Integer workProfilePasswordMinUpperCaseCharacters) {
this.workProfilePasswordMinUpperCaseCharacters = workProfilePasswordMinUpperCaseCharacters;
this.changedFields = changedFields.add("workProfilePasswordMinUpperCaseCharacters");
return this;
}
/**
* “Minutes of inactivity before the screen times out.”
*
* @param workProfilePasswordMinutesOfInactivityBeforeScreenTimeout
* value of {@code workProfilePasswordMinutesOfInactivityBeforeScreenTimeout} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordMinutesOfInactivityBeforeScreenTimeout(Integer workProfilePasswordMinutesOfInactivityBeforeScreenTimeout) {
this.workProfilePasswordMinutesOfInactivityBeforeScreenTimeout = workProfilePasswordMinutesOfInactivityBeforeScreenTimeout;
this.changedFields = changedFields.add("workProfilePasswordMinutesOfInactivityBeforeScreenTimeout");
return this;
}
/**
* “Number of previous work profile passwords to block. Valid values 0 to 24”
*
* @param workProfilePasswordPreviousPasswordBlockCount
* value of {@code workProfilePasswordPreviousPasswordBlockCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordPreviousPasswordBlockCount(Integer workProfilePasswordPreviousPasswordBlockCount) {
this.workProfilePasswordPreviousPasswordBlockCount = workProfilePasswordPreviousPasswordBlockCount;
this.changedFields = changedFields.add("workProfilePasswordPreviousPasswordBlockCount");
return this;
}
/**
* “Type of work profile password that is required.”
*
* @param workProfilePasswordRequiredType
* value of {@code workProfilePasswordRequiredType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordRequiredType(AndroidForWorkRequiredPasswordType workProfilePasswordRequiredType) {
this.workProfilePasswordRequiredType = workProfilePasswordRequiredType;
this.changedFields = changedFields.add("workProfilePasswordRequiredType");
return this;
}
/**
* “Number of sign in failures allowed before work profile is removed and all
* corporate data deleted. Valid values 1 to 16”
*
* @param workProfilePasswordSignInFailureCountBeforeFactoryReset
* value of {@code workProfilePasswordSignInFailureCountBeforeFactoryReset} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfilePasswordSignInFailureCountBeforeFactoryReset(Integer workProfilePasswordSignInFailureCountBeforeFactoryReset) {
this.workProfilePasswordSignInFailureCountBeforeFactoryReset = workProfilePasswordSignInFailureCountBeforeFactoryReset;
this.changedFields = changedFields.add("workProfilePasswordSignInFailureCountBeforeFactoryReset");
return this;
}
/**
* “Password is required or not for work profile”
*
* @param workProfileRequirePassword
* value of {@code workProfileRequirePassword} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder workProfileRequirePassword(Boolean workProfileRequirePassword) {
this.workProfileRequirePassword = workProfileRequirePassword;
this.changedFields = changedFields.add("workProfileRequirePassword");
return this;
}
public AndroidForWorkGeneralDeviceConfiguration build() {
AndroidForWorkGeneralDeviceConfiguration _x = new AndroidForWorkGeneralDeviceConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.androidForWorkGeneralDeviceConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.passwordBlockFaceUnlock = passwordBlockFaceUnlock;
_x.passwordBlockFingerprintUnlock = passwordBlockFingerprintUnlock;
_x.passwordBlockIrisUnlock = passwordBlockIrisUnlock;
_x.passwordBlockTrustAgents = passwordBlockTrustAgents;
_x.passwordExpirationDays = passwordExpirationDays;
_x.passwordMinimumLength = passwordMinimumLength;
_x.passwordMinutesOfInactivityBeforeScreenTimeout = passwordMinutesOfInactivityBeforeScreenTimeout;
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
_x.passwordRequiredType = passwordRequiredType;
_x.passwordSignInFailureCountBeforeFactoryReset = passwordSignInFailureCountBeforeFactoryReset;
_x.securityRequireVerifyApps = securityRequireVerifyApps;
_x.vpnAlwaysOnPackageIdentifier = vpnAlwaysOnPackageIdentifier;
_x.vpnEnableAlwaysOnLockdownMode = vpnEnableAlwaysOnLockdownMode;
_x.workProfileAllowWidgets = workProfileAllowWidgets;
_x.workProfileBlockAddingAccounts = workProfileBlockAddingAccounts;
_x.workProfileBlockCamera = workProfileBlockCamera;
_x.workProfileBlockCrossProfileCallerId = workProfileBlockCrossProfileCallerId;
_x.workProfileBlockCrossProfileContactsSearch = workProfileBlockCrossProfileContactsSearch;
_x.workProfileBlockCrossProfileCopyPaste = workProfileBlockCrossProfileCopyPaste;
_x.workProfileBlockNotificationsWhileDeviceLocked = workProfileBlockNotificationsWhileDeviceLocked;
_x.workProfileBlockPersonalAppInstallsFromUnknownSources = workProfileBlockPersonalAppInstallsFromUnknownSources;
_x.workProfileBlockScreenCapture = workProfileBlockScreenCapture;
_x.workProfileBluetoothEnableContactSharing = workProfileBluetoothEnableContactSharing;
_x.workProfileDataSharingType = workProfileDataSharingType;
_x.workProfileDefaultAppPermissionPolicy = workProfileDefaultAppPermissionPolicy;
_x.workProfilePasswordBlockFaceUnlock = workProfilePasswordBlockFaceUnlock;
_x.workProfilePasswordBlockFingerprintUnlock = workProfilePasswordBlockFingerprintUnlock;
_x.workProfilePasswordBlockIrisUnlock = workProfilePasswordBlockIrisUnlock;
_x.workProfilePasswordBlockTrustAgents = workProfilePasswordBlockTrustAgents;
_x.workProfilePasswordExpirationDays = workProfilePasswordExpirationDays;
_x.workProfilePasswordMinimumLength = workProfilePasswordMinimumLength;
_x.workProfilePasswordMinLetterCharacters = workProfilePasswordMinLetterCharacters;
_x.workProfilePasswordMinLowerCaseCharacters = workProfilePasswordMinLowerCaseCharacters;
_x.workProfilePasswordMinNonLetterCharacters = workProfilePasswordMinNonLetterCharacters;
_x.workProfilePasswordMinNumericCharacters = workProfilePasswordMinNumericCharacters;
_x.workProfilePasswordMinSymbolCharacters = workProfilePasswordMinSymbolCharacters;
_x.workProfilePasswordMinUpperCaseCharacters = workProfilePasswordMinUpperCaseCharacters;
_x.workProfilePasswordMinutesOfInactivityBeforeScreenTimeout = workProfilePasswordMinutesOfInactivityBeforeScreenTimeout;
_x.workProfilePasswordPreviousPasswordBlockCount = workProfilePasswordPreviousPasswordBlockCount;
_x.workProfilePasswordRequiredType = workProfilePasswordRequiredType;
_x.workProfilePasswordSignInFailureCountBeforeFactoryReset = workProfilePasswordSignInFailureCountBeforeFactoryReset;
_x.workProfileRequirePassword = workProfileRequirePassword;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Indicates whether or not to block face unlock.”
*
* @return property passwordBlockFaceUnlock
*/
@Property(name="passwordBlockFaceUnlock")
@JsonIgnore
public Optional getPasswordBlockFaceUnlock() {
return Optional.ofNullable(passwordBlockFaceUnlock);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordBlockFaceUnlock} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to block face unlock.”
*
* @param passwordBlockFaceUnlock
* new value of {@code passwordBlockFaceUnlock} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordBlockFaceUnlock} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withPasswordBlockFaceUnlock(Boolean passwordBlockFaceUnlock) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordBlockFaceUnlock");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.passwordBlockFaceUnlock = passwordBlockFaceUnlock;
return _x;
}
/**
* “Indicates whether or not to block fingerprint unlock.”
*
* @return property passwordBlockFingerprintUnlock
*/
@Property(name="passwordBlockFingerprintUnlock")
@JsonIgnore
public Optional getPasswordBlockFingerprintUnlock() {
return Optional.ofNullable(passwordBlockFingerprintUnlock);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordBlockFingerprintUnlock} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Indicates whether or not to block fingerprint unlock.”
*
* @param passwordBlockFingerprintUnlock
* new value of {@code passwordBlockFingerprintUnlock} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordBlockFingerprintUnlock} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withPasswordBlockFingerprintUnlock(Boolean passwordBlockFingerprintUnlock) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordBlockFingerprintUnlock");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.passwordBlockFingerprintUnlock = passwordBlockFingerprintUnlock;
return _x;
}
/**
* “Indicates whether or not to block iris unlock.”
*
* @return property passwordBlockIrisUnlock
*/
@Property(name="passwordBlockIrisUnlock")
@JsonIgnore
public Optional getPasswordBlockIrisUnlock() {
return Optional.ofNullable(passwordBlockIrisUnlock);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordBlockIrisUnlock} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to block iris unlock.”
*
* @param passwordBlockIrisUnlock
* new value of {@code passwordBlockIrisUnlock} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordBlockIrisUnlock} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withPasswordBlockIrisUnlock(Boolean passwordBlockIrisUnlock) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordBlockIrisUnlock");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.passwordBlockIrisUnlock = passwordBlockIrisUnlock;
return _x;
}
/**
* “Indicates whether or not to block Smart Lock and other trust agents.”
*
* @return property passwordBlockTrustAgents
*/
@Property(name="passwordBlockTrustAgents")
@JsonIgnore
public Optional getPasswordBlockTrustAgents() {
return Optional.ofNullable(passwordBlockTrustAgents);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordBlockTrustAgents} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to block Smart Lock and other trust agents.”
*
* @param passwordBlockTrustAgents
* new value of {@code passwordBlockTrustAgents} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordBlockTrustAgents} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withPasswordBlockTrustAgents(Boolean passwordBlockTrustAgents) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordBlockTrustAgents");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.passwordBlockTrustAgents = passwordBlockTrustAgents;
return _x;
}
/**
* “Number of days before the password expires. Valid values 1 to 365”
*
* @return property passwordExpirationDays
*/
@Property(name="passwordExpirationDays")
@JsonIgnore
public Optional getPasswordExpirationDays() {
return Optional.ofNullable(passwordExpirationDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordExpirationDays} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Number of days before the password expires. Valid values 1 to 365”
*
* @param passwordExpirationDays
* new value of {@code passwordExpirationDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordExpirationDays} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withPasswordExpirationDays(Integer passwordExpirationDays) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordExpirationDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.passwordExpirationDays = passwordExpirationDays;
return _x;
}
/**
* “Minimum length of passwords. Valid values 4 to 16”
*
* @return property passwordMinimumLength
*/
@Property(name="passwordMinimumLength")
@JsonIgnore
public Optional getPasswordMinimumLength() {
return Optional.ofNullable(passwordMinimumLength);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordMinimumLength} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Minimum length of passwords. Valid values 4 to 16”
*
* @param passwordMinimumLength
* new value of {@code passwordMinimumLength} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordMinimumLength} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withPasswordMinimumLength(Integer passwordMinimumLength) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordMinimumLength");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.passwordMinimumLength = passwordMinimumLength;
return _x;
}
/**
* “Minutes of inactivity before the screen times out.”
*
* @return property passwordMinutesOfInactivityBeforeScreenTimeout
*/
@Property(name="passwordMinutesOfInactivityBeforeScreenTimeout")
@JsonIgnore
public Optional getPasswordMinutesOfInactivityBeforeScreenTimeout() {
return Optional.ofNullable(passwordMinutesOfInactivityBeforeScreenTimeout);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordMinutesOfInactivityBeforeScreenTimeout} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Minutes of inactivity before the screen times out.”
*
* @param passwordMinutesOfInactivityBeforeScreenTimeout
* new value of {@code passwordMinutesOfInactivityBeforeScreenTimeout} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordMinutesOfInactivityBeforeScreenTimeout} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withPasswordMinutesOfInactivityBeforeScreenTimeout(Integer passwordMinutesOfInactivityBeforeScreenTimeout) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordMinutesOfInactivityBeforeScreenTimeout");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.passwordMinutesOfInactivityBeforeScreenTimeout = passwordMinutesOfInactivityBeforeScreenTimeout;
return _x;
}
/**
* “Number of previous passwords to block. Valid values 0 to 24”
*
* @return property passwordPreviousPasswordBlockCount
*/
@Property(name="passwordPreviousPasswordBlockCount")
@JsonIgnore
public Optional getPasswordPreviousPasswordBlockCount() {
return Optional.ofNullable(passwordPreviousPasswordBlockCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordPreviousPasswordBlockCount} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Number of previous passwords to block. Valid values 0 to 24”
*
* @param passwordPreviousPasswordBlockCount
* new value of {@code passwordPreviousPasswordBlockCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordPreviousPasswordBlockCount} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withPasswordPreviousPasswordBlockCount(Integer passwordPreviousPasswordBlockCount) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordPreviousPasswordBlockCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
return _x;
}
/**
* “Type of password that is required.”
*
* @return property passwordRequiredType
*/
@Property(name="passwordRequiredType")
@JsonIgnore
public Optional getPasswordRequiredType() {
return Optional.ofNullable(passwordRequiredType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordRequiredType} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Type of password that is required.”
*
* @param passwordRequiredType
* new value of {@code passwordRequiredType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordRequiredType} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withPasswordRequiredType(AndroidForWorkRequiredPasswordType passwordRequiredType) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordRequiredType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.passwordRequiredType = passwordRequiredType;
return _x;
}
/**
* “Number of sign in failures allowed before factory reset. Valid values 1 to 16”
*
* @return property passwordSignInFailureCountBeforeFactoryReset
*/
@Property(name="passwordSignInFailureCountBeforeFactoryReset")
@JsonIgnore
public Optional getPasswordSignInFailureCountBeforeFactoryReset() {
return Optional.ofNullable(passwordSignInFailureCountBeforeFactoryReset);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordSignInFailureCountBeforeFactoryReset} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Number of sign in failures allowed before factory reset. Valid values 1 to 16”
*
* @param passwordSignInFailureCountBeforeFactoryReset
* new value of {@code passwordSignInFailureCountBeforeFactoryReset} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordSignInFailureCountBeforeFactoryReset} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withPasswordSignInFailureCountBeforeFactoryReset(Integer passwordSignInFailureCountBeforeFactoryReset) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("passwordSignInFailureCountBeforeFactoryReset");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.passwordSignInFailureCountBeforeFactoryReset = passwordSignInFailureCountBeforeFactoryReset;
return _x;
}
/**
* “Require the Android Verify apps feature is turned on.”
*
* @return property securityRequireVerifyApps
*/
@Property(name="securityRequireVerifyApps")
@JsonIgnore
public Optional getSecurityRequireVerifyApps() {
return Optional.ofNullable(securityRequireVerifyApps);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* securityRequireVerifyApps} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Require the Android Verify apps feature is turned on.”
*
* @param securityRequireVerifyApps
* new value of {@code securityRequireVerifyApps} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code securityRequireVerifyApps} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withSecurityRequireVerifyApps(Boolean securityRequireVerifyApps) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("securityRequireVerifyApps");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.securityRequireVerifyApps = securityRequireVerifyApps;
return _x;
}
/**
* “Enable lockdown mode for always-on VPN.”
*
* @return property vpnAlwaysOnPackageIdentifier
*/
@Property(name="vpnAlwaysOnPackageIdentifier")
@JsonIgnore
public Optional getVpnAlwaysOnPackageIdentifier() {
return Optional.ofNullable(vpnAlwaysOnPackageIdentifier);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* vpnAlwaysOnPackageIdentifier} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Enable lockdown mode for always-on VPN.”
*
* @param vpnAlwaysOnPackageIdentifier
* new value of {@code vpnAlwaysOnPackageIdentifier} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code vpnAlwaysOnPackageIdentifier} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withVpnAlwaysOnPackageIdentifier(String vpnAlwaysOnPackageIdentifier) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("vpnAlwaysOnPackageIdentifier");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.vpnAlwaysOnPackageIdentifier = vpnAlwaysOnPackageIdentifier;
return _x;
}
/**
* “Enable lockdown mode for always-on VPN.”
*
* @return property vpnEnableAlwaysOnLockdownMode
*/
@Property(name="vpnEnableAlwaysOnLockdownMode")
@JsonIgnore
public Optional getVpnEnableAlwaysOnLockdownMode() {
return Optional.ofNullable(vpnEnableAlwaysOnLockdownMode);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* vpnEnableAlwaysOnLockdownMode} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Enable lockdown mode for always-on VPN.”
*
* @param vpnEnableAlwaysOnLockdownMode
* new value of {@code vpnEnableAlwaysOnLockdownMode} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code vpnEnableAlwaysOnLockdownMode} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withVpnEnableAlwaysOnLockdownMode(Boolean vpnEnableAlwaysOnLockdownMode) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("vpnEnableAlwaysOnLockdownMode");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.vpnEnableAlwaysOnLockdownMode = vpnEnableAlwaysOnLockdownMode;
return _x;
}
/**
* “Allow widgets from work profile apps.”
*
* @return property workProfileAllowWidgets
*/
@Property(name="workProfileAllowWidgets")
@JsonIgnore
public Optional getWorkProfileAllowWidgets() {
return Optional.ofNullable(workProfileAllowWidgets);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileAllowWidgets} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Allow widgets from work profile apps.”
*
* @param workProfileAllowWidgets
* new value of {@code workProfileAllowWidgets} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileAllowWidgets} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileAllowWidgets(Boolean workProfileAllowWidgets) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileAllowWidgets");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileAllowWidgets = workProfileAllowWidgets;
return _x;
}
/**
* “Block users from adding/removing accounts in work profile.”
*
* @return property workProfileBlockAddingAccounts
*/
@Property(name="workProfileBlockAddingAccounts")
@JsonIgnore
public Optional getWorkProfileBlockAddingAccounts() {
return Optional.ofNullable(workProfileBlockAddingAccounts);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileBlockAddingAccounts} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Block users from adding/removing accounts in work profile.”
*
* @param workProfileBlockAddingAccounts
* new value of {@code workProfileBlockAddingAccounts} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileBlockAddingAccounts} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileBlockAddingAccounts(Boolean workProfileBlockAddingAccounts) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileBlockAddingAccounts");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileBlockAddingAccounts = workProfileBlockAddingAccounts;
return _x;
}
/**
* “Block work profile camera.”
*
* @return property workProfileBlockCamera
*/
@Property(name="workProfileBlockCamera")
@JsonIgnore
public Optional getWorkProfileBlockCamera() {
return Optional.ofNullable(workProfileBlockCamera);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileBlockCamera} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Block work profile camera.”
*
* @param workProfileBlockCamera
* new value of {@code workProfileBlockCamera} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileBlockCamera} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileBlockCamera(Boolean workProfileBlockCamera) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileBlockCamera");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileBlockCamera = workProfileBlockCamera;
return _x;
}
/**
* “Block display work profile caller ID in personal profile.”
*
* @return property workProfileBlockCrossProfileCallerId
*/
@Property(name="workProfileBlockCrossProfileCallerId")
@JsonIgnore
public Optional getWorkProfileBlockCrossProfileCallerId() {
return Optional.ofNullable(workProfileBlockCrossProfileCallerId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileBlockCrossProfileCallerId} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Block display work profile caller ID in personal profile.”
*
* @param workProfileBlockCrossProfileCallerId
* new value of {@code workProfileBlockCrossProfileCallerId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileBlockCrossProfileCallerId} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileBlockCrossProfileCallerId(Boolean workProfileBlockCrossProfileCallerId) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileBlockCrossProfileCallerId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileBlockCrossProfileCallerId = workProfileBlockCrossProfileCallerId;
return _x;
}
/**
* “Block work profile contacts availability in personal profile.”
*
* @return property workProfileBlockCrossProfileContactsSearch
*/
@Property(name="workProfileBlockCrossProfileContactsSearch")
@JsonIgnore
public Optional getWorkProfileBlockCrossProfileContactsSearch() {
return Optional.ofNullable(workProfileBlockCrossProfileContactsSearch);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileBlockCrossProfileContactsSearch} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Block work profile contacts availability in personal profile.”
*
* @param workProfileBlockCrossProfileContactsSearch
* new value of {@code workProfileBlockCrossProfileContactsSearch} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileBlockCrossProfileContactsSearch} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileBlockCrossProfileContactsSearch(Boolean workProfileBlockCrossProfileContactsSearch) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileBlockCrossProfileContactsSearch");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileBlockCrossProfileContactsSearch = workProfileBlockCrossProfileContactsSearch;
return _x;
}
/**
* “Boolean that indicates if the setting disallow cross profile copy/paste is
* enabled.”
*
* @return property workProfileBlockCrossProfileCopyPaste
*/
@Property(name="workProfileBlockCrossProfileCopyPaste")
@JsonIgnore
public Optional getWorkProfileBlockCrossProfileCopyPaste() {
return Optional.ofNullable(workProfileBlockCrossProfileCopyPaste);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileBlockCrossProfileCopyPaste} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Boolean that indicates if the setting disallow cross profile copy/paste is
* enabled.”
*
* @param workProfileBlockCrossProfileCopyPaste
* new value of {@code workProfileBlockCrossProfileCopyPaste} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileBlockCrossProfileCopyPaste} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileBlockCrossProfileCopyPaste(Boolean workProfileBlockCrossProfileCopyPaste) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileBlockCrossProfileCopyPaste");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileBlockCrossProfileCopyPaste = workProfileBlockCrossProfileCopyPaste;
return _x;
}
/**
* “Indicates whether or not to block notifications while device locked.”
*
* @return property workProfileBlockNotificationsWhileDeviceLocked
*/
@Property(name="workProfileBlockNotificationsWhileDeviceLocked")
@JsonIgnore
public Optional getWorkProfileBlockNotificationsWhileDeviceLocked() {
return Optional.ofNullable(workProfileBlockNotificationsWhileDeviceLocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileBlockNotificationsWhileDeviceLocked} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Indicates whether or not to block notifications while device locked.”
*
* @param workProfileBlockNotificationsWhileDeviceLocked
* new value of {@code workProfileBlockNotificationsWhileDeviceLocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileBlockNotificationsWhileDeviceLocked} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileBlockNotificationsWhileDeviceLocked(Boolean workProfileBlockNotificationsWhileDeviceLocked) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileBlockNotificationsWhileDeviceLocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileBlockNotificationsWhileDeviceLocked = workProfileBlockNotificationsWhileDeviceLocked;
return _x;
}
/**
* “Prevent app installations from unknown sources in the personal profile.”
*
* @return property workProfileBlockPersonalAppInstallsFromUnknownSources
*/
@Property(name="workProfileBlockPersonalAppInstallsFromUnknownSources")
@JsonIgnore
public Optional getWorkProfileBlockPersonalAppInstallsFromUnknownSources() {
return Optional.ofNullable(workProfileBlockPersonalAppInstallsFromUnknownSources);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileBlockPersonalAppInstallsFromUnknownSources} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “Prevent app installations from unknown sources in the personal profile.”
*
* @param workProfileBlockPersonalAppInstallsFromUnknownSources
* new value of {@code workProfileBlockPersonalAppInstallsFromUnknownSources} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileBlockPersonalAppInstallsFromUnknownSources} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileBlockPersonalAppInstallsFromUnknownSources(Boolean workProfileBlockPersonalAppInstallsFromUnknownSources) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileBlockPersonalAppInstallsFromUnknownSources");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileBlockPersonalAppInstallsFromUnknownSources = workProfileBlockPersonalAppInstallsFromUnknownSources;
return _x;
}
/**
* “Block screen capture in work profile.”
*
* @return property workProfileBlockScreenCapture
*/
@Property(name="workProfileBlockScreenCapture")
@JsonIgnore
public Optional getWorkProfileBlockScreenCapture() {
return Optional.ofNullable(workProfileBlockScreenCapture);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileBlockScreenCapture} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Block screen capture in work profile.”
*
* @param workProfileBlockScreenCapture
* new value of {@code workProfileBlockScreenCapture} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileBlockScreenCapture} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileBlockScreenCapture(Boolean workProfileBlockScreenCapture) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileBlockScreenCapture");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileBlockScreenCapture = workProfileBlockScreenCapture;
return _x;
}
/**
* “Allow bluetooth devices to access enterprise contacts.”
*
* @return property workProfileBluetoothEnableContactSharing
*/
@Property(name="workProfileBluetoothEnableContactSharing")
@JsonIgnore
public Optional getWorkProfileBluetoothEnableContactSharing() {
return Optional.ofNullable(workProfileBluetoothEnableContactSharing);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileBluetoothEnableContactSharing} field changed. Field description below
* . The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Allow bluetooth devices to access enterprise contacts.”
*
* @param workProfileBluetoothEnableContactSharing
* new value of {@code workProfileBluetoothEnableContactSharing} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileBluetoothEnableContactSharing} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileBluetoothEnableContactSharing(Boolean workProfileBluetoothEnableContactSharing) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileBluetoothEnableContactSharing");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileBluetoothEnableContactSharing = workProfileBluetoothEnableContactSharing;
return _x;
}
/**
* “Type of data sharing that is allowed.”
*
* @return property workProfileDataSharingType
*/
@Property(name="workProfileDataSharingType")
@JsonIgnore
public Optional getWorkProfileDataSharingType() {
return Optional.ofNullable(workProfileDataSharingType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileDataSharingType} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Type of data sharing that is allowed.”
*
* @param workProfileDataSharingType
* new value of {@code workProfileDataSharingType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileDataSharingType} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileDataSharingType(AndroidForWorkCrossProfileDataSharingType workProfileDataSharingType) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileDataSharingType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileDataSharingType = workProfileDataSharingType;
return _x;
}
/**
* “Type of password that is required.”
*
* @return property workProfileDefaultAppPermissionPolicy
*/
@Property(name="workProfileDefaultAppPermissionPolicy")
@JsonIgnore
public Optional getWorkProfileDefaultAppPermissionPolicy() {
return Optional.ofNullable(workProfileDefaultAppPermissionPolicy);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileDefaultAppPermissionPolicy} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Type of password that is required.”
*
* @param workProfileDefaultAppPermissionPolicy
* new value of {@code workProfileDefaultAppPermissionPolicy} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileDefaultAppPermissionPolicy} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileDefaultAppPermissionPolicy(AndroidForWorkDefaultAppPermissionPolicyType workProfileDefaultAppPermissionPolicy) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileDefaultAppPermissionPolicy");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileDefaultAppPermissionPolicy = workProfileDefaultAppPermissionPolicy;
return _x;
}
/**
* “Indicates whether or not to block face unlock for work profile.”
*
* @return property workProfilePasswordBlockFaceUnlock
*/
@Property(name="workProfilePasswordBlockFaceUnlock")
@JsonIgnore
public Optional getWorkProfilePasswordBlockFaceUnlock() {
return Optional.ofNullable(workProfilePasswordBlockFaceUnlock);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordBlockFaceUnlock} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Indicates whether or not to block face unlock for work profile.”
*
* @param workProfilePasswordBlockFaceUnlock
* new value of {@code workProfilePasswordBlockFaceUnlock} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordBlockFaceUnlock} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordBlockFaceUnlock(Boolean workProfilePasswordBlockFaceUnlock) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordBlockFaceUnlock");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordBlockFaceUnlock = workProfilePasswordBlockFaceUnlock;
return _x;
}
/**
* “Indicates whether or not to block fingerprint unlock for work profile.”
*
* @return property workProfilePasswordBlockFingerprintUnlock
*/
@Property(name="workProfilePasswordBlockFingerprintUnlock")
@JsonIgnore
public Optional getWorkProfilePasswordBlockFingerprintUnlock() {
return Optional.ofNullable(workProfilePasswordBlockFingerprintUnlock);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordBlockFingerprintUnlock} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Indicates whether or not to block fingerprint unlock for work profile.”
*
* @param workProfilePasswordBlockFingerprintUnlock
* new value of {@code workProfilePasswordBlockFingerprintUnlock} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordBlockFingerprintUnlock} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordBlockFingerprintUnlock(Boolean workProfilePasswordBlockFingerprintUnlock) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordBlockFingerprintUnlock");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordBlockFingerprintUnlock = workProfilePasswordBlockFingerprintUnlock;
return _x;
}
/**
* “Indicates whether or not to block iris unlock for work profile.”
*
* @return property workProfilePasswordBlockIrisUnlock
*/
@Property(name="workProfilePasswordBlockIrisUnlock")
@JsonIgnore
public Optional getWorkProfilePasswordBlockIrisUnlock() {
return Optional.ofNullable(workProfilePasswordBlockIrisUnlock);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordBlockIrisUnlock} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Indicates whether or not to block iris unlock for work profile.”
*
* @param workProfilePasswordBlockIrisUnlock
* new value of {@code workProfilePasswordBlockIrisUnlock} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordBlockIrisUnlock} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordBlockIrisUnlock(Boolean workProfilePasswordBlockIrisUnlock) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordBlockIrisUnlock");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordBlockIrisUnlock = workProfilePasswordBlockIrisUnlock;
return _x;
}
/**
* “Indicates whether or not to block Smart Lock and other trust agents for work
* profile.”
*
* @return property workProfilePasswordBlockTrustAgents
*/
@Property(name="workProfilePasswordBlockTrustAgents")
@JsonIgnore
public Optional getWorkProfilePasswordBlockTrustAgents() {
return Optional.ofNullable(workProfilePasswordBlockTrustAgents);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordBlockTrustAgents} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Indicates whether or not to block Smart Lock and other trust agents for work
* profile.”
*
* @param workProfilePasswordBlockTrustAgents
* new value of {@code workProfilePasswordBlockTrustAgents} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordBlockTrustAgents} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordBlockTrustAgents(Boolean workProfilePasswordBlockTrustAgents) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordBlockTrustAgents");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordBlockTrustAgents = workProfilePasswordBlockTrustAgents;
return _x;
}
/**
* “Number of days before the work profile password expires. Valid values 1 to 365”
*
* @return property workProfilePasswordExpirationDays
*/
@Property(name="workProfilePasswordExpirationDays")
@JsonIgnore
public Optional getWorkProfilePasswordExpirationDays() {
return Optional.ofNullable(workProfilePasswordExpirationDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordExpirationDays} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Number of days before the work profile password expires. Valid values 1 to 365”
*
* @param workProfilePasswordExpirationDays
* new value of {@code workProfilePasswordExpirationDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordExpirationDays} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordExpirationDays(Integer workProfilePasswordExpirationDays) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordExpirationDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordExpirationDays = workProfilePasswordExpirationDays;
return _x;
}
/**
* “Minimum length of work profile password. Valid values 4 to 16”
*
* @return property workProfilePasswordMinimumLength
*/
@Property(name="workProfilePasswordMinimumLength")
@JsonIgnore
public Optional getWorkProfilePasswordMinimumLength() {
return Optional.ofNullable(workProfilePasswordMinimumLength);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordMinimumLength} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Minimum length of work profile password. Valid values 4 to 16”
*
* @param workProfilePasswordMinimumLength
* new value of {@code workProfilePasswordMinimumLength} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordMinimumLength} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordMinimumLength(Integer workProfilePasswordMinimumLength) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordMinimumLength");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordMinimumLength = workProfilePasswordMinimumLength;
return _x;
}
/**
* “Minimum # of letter characters required in work profile password. Valid values 1
* to 10”
*
* @return property workProfilePasswordMinLetterCharacters
*/
@Property(name="workProfilePasswordMinLetterCharacters")
@JsonIgnore
public Optional getWorkProfilePasswordMinLetterCharacters() {
return Optional.ofNullable(workProfilePasswordMinLetterCharacters);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordMinLetterCharacters} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Minimum # of letter characters required in work profile password. Valid values 1
* to 10”
*
* @param workProfilePasswordMinLetterCharacters
* new value of {@code workProfilePasswordMinLetterCharacters} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordMinLetterCharacters} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordMinLetterCharacters(Integer workProfilePasswordMinLetterCharacters) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordMinLetterCharacters");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordMinLetterCharacters = workProfilePasswordMinLetterCharacters;
return _x;
}
/**
* “Minimum # of lower-case characters required in work profile password. Valid
* values 1 to 10”
*
* @return property workProfilePasswordMinLowerCaseCharacters
*/
@Property(name="workProfilePasswordMinLowerCaseCharacters")
@JsonIgnore
public Optional getWorkProfilePasswordMinLowerCaseCharacters() {
return Optional.ofNullable(workProfilePasswordMinLowerCaseCharacters);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordMinLowerCaseCharacters} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Minimum # of lower-case characters required in work profile password. Valid
* values 1 to 10”
*
* @param workProfilePasswordMinLowerCaseCharacters
* new value of {@code workProfilePasswordMinLowerCaseCharacters} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordMinLowerCaseCharacters} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordMinLowerCaseCharacters(Integer workProfilePasswordMinLowerCaseCharacters) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordMinLowerCaseCharacters");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordMinLowerCaseCharacters = workProfilePasswordMinLowerCaseCharacters;
return _x;
}
/**
* “Minimum # of non-letter characters required in work profile password. Valid
* values 1 to 10”
*
* @return property workProfilePasswordMinNonLetterCharacters
*/
@Property(name="workProfilePasswordMinNonLetterCharacters")
@JsonIgnore
public Optional getWorkProfilePasswordMinNonLetterCharacters() {
return Optional.ofNullable(workProfilePasswordMinNonLetterCharacters);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordMinNonLetterCharacters} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Minimum # of non-letter characters required in work profile password. Valid
* values 1 to 10”
*
* @param workProfilePasswordMinNonLetterCharacters
* new value of {@code workProfilePasswordMinNonLetterCharacters} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordMinNonLetterCharacters} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordMinNonLetterCharacters(Integer workProfilePasswordMinNonLetterCharacters) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordMinNonLetterCharacters");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordMinNonLetterCharacters = workProfilePasswordMinNonLetterCharacters;
return _x;
}
/**
* “Minimum # of numeric characters required in work profile password. Valid values
* 1 to 10”
*
* @return property workProfilePasswordMinNumericCharacters
*/
@Property(name="workProfilePasswordMinNumericCharacters")
@JsonIgnore
public Optional getWorkProfilePasswordMinNumericCharacters() {
return Optional.ofNullable(workProfilePasswordMinNumericCharacters);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordMinNumericCharacters} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Minimum # of numeric characters required in work profile password. Valid values
* 1 to 10”
*
* @param workProfilePasswordMinNumericCharacters
* new value of {@code workProfilePasswordMinNumericCharacters} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordMinNumericCharacters} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordMinNumericCharacters(Integer workProfilePasswordMinNumericCharacters) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordMinNumericCharacters");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordMinNumericCharacters = workProfilePasswordMinNumericCharacters;
return _x;
}
/**
* “Minimum # of symbols required in work profile password. Valid values 1 to 10”
*
* @return property workProfilePasswordMinSymbolCharacters
*/
@Property(name="workProfilePasswordMinSymbolCharacters")
@JsonIgnore
public Optional getWorkProfilePasswordMinSymbolCharacters() {
return Optional.ofNullable(workProfilePasswordMinSymbolCharacters);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordMinSymbolCharacters} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Minimum # of symbols required in work profile password. Valid values 1 to 10”
*
* @param workProfilePasswordMinSymbolCharacters
* new value of {@code workProfilePasswordMinSymbolCharacters} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordMinSymbolCharacters} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordMinSymbolCharacters(Integer workProfilePasswordMinSymbolCharacters) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordMinSymbolCharacters");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordMinSymbolCharacters = workProfilePasswordMinSymbolCharacters;
return _x;
}
/**
* “Minimum # of upper-case characters required in work profile password. Valid
* values 1 to 10”
*
* @return property workProfilePasswordMinUpperCaseCharacters
*/
@Property(name="workProfilePasswordMinUpperCaseCharacters")
@JsonIgnore
public Optional getWorkProfilePasswordMinUpperCaseCharacters() {
return Optional.ofNullable(workProfilePasswordMinUpperCaseCharacters);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordMinUpperCaseCharacters} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Minimum # of upper-case characters required in work profile password. Valid
* values 1 to 10”
*
* @param workProfilePasswordMinUpperCaseCharacters
* new value of {@code workProfilePasswordMinUpperCaseCharacters} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordMinUpperCaseCharacters} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordMinUpperCaseCharacters(Integer workProfilePasswordMinUpperCaseCharacters) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordMinUpperCaseCharacters");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordMinUpperCaseCharacters = workProfilePasswordMinUpperCaseCharacters;
return _x;
}
/**
* “Minutes of inactivity before the screen times out.”
*
* @return property workProfilePasswordMinutesOfInactivityBeforeScreenTimeout
*/
@Property(name="workProfilePasswordMinutesOfInactivityBeforeScreenTimeout")
@JsonIgnore
public Optional getWorkProfilePasswordMinutesOfInactivityBeforeScreenTimeout() {
return Optional.ofNullable(workProfilePasswordMinutesOfInactivityBeforeScreenTimeout);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordMinutesOfInactivityBeforeScreenTimeout} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “Minutes of inactivity before the screen times out.”
*
* @param workProfilePasswordMinutesOfInactivityBeforeScreenTimeout
* new value of {@code workProfilePasswordMinutesOfInactivityBeforeScreenTimeout} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordMinutesOfInactivityBeforeScreenTimeout} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordMinutesOfInactivityBeforeScreenTimeout(Integer workProfilePasswordMinutesOfInactivityBeforeScreenTimeout) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordMinutesOfInactivityBeforeScreenTimeout");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordMinutesOfInactivityBeforeScreenTimeout = workProfilePasswordMinutesOfInactivityBeforeScreenTimeout;
return _x;
}
/**
* “Number of previous work profile passwords to block. Valid values 0 to 24”
*
* @return property workProfilePasswordPreviousPasswordBlockCount
*/
@Property(name="workProfilePasswordPreviousPasswordBlockCount")
@JsonIgnore
public Optional getWorkProfilePasswordPreviousPasswordBlockCount() {
return Optional.ofNullable(workProfilePasswordPreviousPasswordBlockCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordPreviousPasswordBlockCount} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Number of previous work profile passwords to block. Valid values 0 to 24”
*
* @param workProfilePasswordPreviousPasswordBlockCount
* new value of {@code workProfilePasswordPreviousPasswordBlockCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordPreviousPasswordBlockCount} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordPreviousPasswordBlockCount(Integer workProfilePasswordPreviousPasswordBlockCount) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordPreviousPasswordBlockCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordPreviousPasswordBlockCount = workProfilePasswordPreviousPasswordBlockCount;
return _x;
}
/**
* “Type of work profile password that is required.”
*
* @return property workProfilePasswordRequiredType
*/
@Property(name="workProfilePasswordRequiredType")
@JsonIgnore
public Optional getWorkProfilePasswordRequiredType() {
return Optional.ofNullable(workProfilePasswordRequiredType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordRequiredType} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Type of work profile password that is required.”
*
* @param workProfilePasswordRequiredType
* new value of {@code workProfilePasswordRequiredType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordRequiredType} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordRequiredType(AndroidForWorkRequiredPasswordType workProfilePasswordRequiredType) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordRequiredType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordRequiredType = workProfilePasswordRequiredType;
return _x;
}
/**
* “Number of sign in failures allowed before work profile is removed and all
* corporate data deleted. Valid values 1 to 16”
*
* @return property workProfilePasswordSignInFailureCountBeforeFactoryReset
*/
@Property(name="workProfilePasswordSignInFailureCountBeforeFactoryReset")
@JsonIgnore
public Optional getWorkProfilePasswordSignInFailureCountBeforeFactoryReset() {
return Optional.ofNullable(workProfilePasswordSignInFailureCountBeforeFactoryReset);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfilePasswordSignInFailureCountBeforeFactoryReset} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “Number of sign in failures allowed before work profile is removed and all
* corporate data deleted. Valid values 1 to 16”
*
* @param workProfilePasswordSignInFailureCountBeforeFactoryReset
* new value of {@code workProfilePasswordSignInFailureCountBeforeFactoryReset} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfilePasswordSignInFailureCountBeforeFactoryReset} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfilePasswordSignInFailureCountBeforeFactoryReset(Integer workProfilePasswordSignInFailureCountBeforeFactoryReset) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfilePasswordSignInFailureCountBeforeFactoryReset");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfilePasswordSignInFailureCountBeforeFactoryReset = workProfilePasswordSignInFailureCountBeforeFactoryReset;
return _x;
}
/**
* “Password is required or not for work profile”
*
* @return property workProfileRequirePassword
*/
@Property(name="workProfileRequirePassword")
@JsonIgnore
public Optional getWorkProfileRequirePassword() {
return Optional.ofNullable(workProfileRequirePassword);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* workProfileRequirePassword} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Password is required or not for work profile”
*
* @param workProfileRequirePassword
* new value of {@code workProfileRequirePassword} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code workProfileRequirePassword} field changed
*/
public AndroidForWorkGeneralDeviceConfiguration withWorkProfileRequirePassword(Boolean workProfileRequirePassword) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = changedFields.add("workProfileRequirePassword");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkGeneralDeviceConfiguration");
_x.workProfileRequirePassword = workProfileRequirePassword;
return _x;
}
public AndroidForWorkGeneralDeviceConfiguration withUnmappedField(String name, String value) {
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public AndroidForWorkGeneralDeviceConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public AndroidForWorkGeneralDeviceConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
AndroidForWorkGeneralDeviceConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private AndroidForWorkGeneralDeviceConfiguration _copy() {
AndroidForWorkGeneralDeviceConfiguration _x = new AndroidForWorkGeneralDeviceConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.passwordBlockFaceUnlock = passwordBlockFaceUnlock;
_x.passwordBlockFingerprintUnlock = passwordBlockFingerprintUnlock;
_x.passwordBlockIrisUnlock = passwordBlockIrisUnlock;
_x.passwordBlockTrustAgents = passwordBlockTrustAgents;
_x.passwordExpirationDays = passwordExpirationDays;
_x.passwordMinimumLength = passwordMinimumLength;
_x.passwordMinutesOfInactivityBeforeScreenTimeout = passwordMinutesOfInactivityBeforeScreenTimeout;
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
_x.passwordRequiredType = passwordRequiredType;
_x.passwordSignInFailureCountBeforeFactoryReset = passwordSignInFailureCountBeforeFactoryReset;
_x.securityRequireVerifyApps = securityRequireVerifyApps;
_x.vpnAlwaysOnPackageIdentifier = vpnAlwaysOnPackageIdentifier;
_x.vpnEnableAlwaysOnLockdownMode = vpnEnableAlwaysOnLockdownMode;
_x.workProfileAllowWidgets = workProfileAllowWidgets;
_x.workProfileBlockAddingAccounts = workProfileBlockAddingAccounts;
_x.workProfileBlockCamera = workProfileBlockCamera;
_x.workProfileBlockCrossProfileCallerId = workProfileBlockCrossProfileCallerId;
_x.workProfileBlockCrossProfileContactsSearch = workProfileBlockCrossProfileContactsSearch;
_x.workProfileBlockCrossProfileCopyPaste = workProfileBlockCrossProfileCopyPaste;
_x.workProfileBlockNotificationsWhileDeviceLocked = workProfileBlockNotificationsWhileDeviceLocked;
_x.workProfileBlockPersonalAppInstallsFromUnknownSources = workProfileBlockPersonalAppInstallsFromUnknownSources;
_x.workProfileBlockScreenCapture = workProfileBlockScreenCapture;
_x.workProfileBluetoothEnableContactSharing = workProfileBluetoothEnableContactSharing;
_x.workProfileDataSharingType = workProfileDataSharingType;
_x.workProfileDefaultAppPermissionPolicy = workProfileDefaultAppPermissionPolicy;
_x.workProfilePasswordBlockFaceUnlock = workProfilePasswordBlockFaceUnlock;
_x.workProfilePasswordBlockFingerprintUnlock = workProfilePasswordBlockFingerprintUnlock;
_x.workProfilePasswordBlockIrisUnlock = workProfilePasswordBlockIrisUnlock;
_x.workProfilePasswordBlockTrustAgents = workProfilePasswordBlockTrustAgents;
_x.workProfilePasswordExpirationDays = workProfilePasswordExpirationDays;
_x.workProfilePasswordMinimumLength = workProfilePasswordMinimumLength;
_x.workProfilePasswordMinLetterCharacters = workProfilePasswordMinLetterCharacters;
_x.workProfilePasswordMinLowerCaseCharacters = workProfilePasswordMinLowerCaseCharacters;
_x.workProfilePasswordMinNonLetterCharacters = workProfilePasswordMinNonLetterCharacters;
_x.workProfilePasswordMinNumericCharacters = workProfilePasswordMinNumericCharacters;
_x.workProfilePasswordMinSymbolCharacters = workProfilePasswordMinSymbolCharacters;
_x.workProfilePasswordMinUpperCaseCharacters = workProfilePasswordMinUpperCaseCharacters;
_x.workProfilePasswordMinutesOfInactivityBeforeScreenTimeout = workProfilePasswordMinutesOfInactivityBeforeScreenTimeout;
_x.workProfilePasswordPreviousPasswordBlockCount = workProfilePasswordPreviousPasswordBlockCount;
_x.workProfilePasswordRequiredType = workProfilePasswordRequiredType;
_x.workProfilePasswordSignInFailureCountBeforeFactoryReset = workProfilePasswordSignInFailureCountBeforeFactoryReset;
_x.workProfileRequirePassword = workProfileRequirePassword;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("AndroidForWorkGeneralDeviceConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("deviceManagementApplicabilityRuleDeviceMode=");
b.append(this.deviceManagementApplicabilityRuleDeviceMode);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsEdition=");
b.append(this.deviceManagementApplicabilityRuleOsEdition);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsVersion=");
b.append(this.deviceManagementApplicabilityRuleOsVersion);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("supportsScopeTags=");
b.append(this.supportsScopeTags);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("passwordBlockFaceUnlock=");
b.append(this.passwordBlockFaceUnlock);
b.append(", ");
b.append("passwordBlockFingerprintUnlock=");
b.append(this.passwordBlockFingerprintUnlock);
b.append(", ");
b.append("passwordBlockIrisUnlock=");
b.append(this.passwordBlockIrisUnlock);
b.append(", ");
b.append("passwordBlockTrustAgents=");
b.append(this.passwordBlockTrustAgents);
b.append(", ");
b.append("passwordExpirationDays=");
b.append(this.passwordExpirationDays);
b.append(", ");
b.append("passwordMinimumLength=");
b.append(this.passwordMinimumLength);
b.append(", ");
b.append("passwordMinutesOfInactivityBeforeScreenTimeout=");
b.append(this.passwordMinutesOfInactivityBeforeScreenTimeout);
b.append(", ");
b.append("passwordPreviousPasswordBlockCount=");
b.append(this.passwordPreviousPasswordBlockCount);
b.append(", ");
b.append("passwordRequiredType=");
b.append(this.passwordRequiredType);
b.append(", ");
b.append("passwordSignInFailureCountBeforeFactoryReset=");
b.append(this.passwordSignInFailureCountBeforeFactoryReset);
b.append(", ");
b.append("securityRequireVerifyApps=");
b.append(this.securityRequireVerifyApps);
b.append(", ");
b.append("vpnAlwaysOnPackageIdentifier=");
b.append(this.vpnAlwaysOnPackageIdentifier);
b.append(", ");
b.append("vpnEnableAlwaysOnLockdownMode=");
b.append(this.vpnEnableAlwaysOnLockdownMode);
b.append(", ");
b.append("workProfileAllowWidgets=");
b.append(this.workProfileAllowWidgets);
b.append(", ");
b.append("workProfileBlockAddingAccounts=");
b.append(this.workProfileBlockAddingAccounts);
b.append(", ");
b.append("workProfileBlockCamera=");
b.append(this.workProfileBlockCamera);
b.append(", ");
b.append("workProfileBlockCrossProfileCallerId=");
b.append(this.workProfileBlockCrossProfileCallerId);
b.append(", ");
b.append("workProfileBlockCrossProfileContactsSearch=");
b.append(this.workProfileBlockCrossProfileContactsSearch);
b.append(", ");
b.append("workProfileBlockCrossProfileCopyPaste=");
b.append(this.workProfileBlockCrossProfileCopyPaste);
b.append(", ");
b.append("workProfileBlockNotificationsWhileDeviceLocked=");
b.append(this.workProfileBlockNotificationsWhileDeviceLocked);
b.append(", ");
b.append("workProfileBlockPersonalAppInstallsFromUnknownSources=");
b.append(this.workProfileBlockPersonalAppInstallsFromUnknownSources);
b.append(", ");
b.append("workProfileBlockScreenCapture=");
b.append(this.workProfileBlockScreenCapture);
b.append(", ");
b.append("workProfileBluetoothEnableContactSharing=");
b.append(this.workProfileBluetoothEnableContactSharing);
b.append(", ");
b.append("workProfileDataSharingType=");
b.append(this.workProfileDataSharingType);
b.append(", ");
b.append("workProfileDefaultAppPermissionPolicy=");
b.append(this.workProfileDefaultAppPermissionPolicy);
b.append(", ");
b.append("workProfilePasswordBlockFaceUnlock=");
b.append(this.workProfilePasswordBlockFaceUnlock);
b.append(", ");
b.append("workProfilePasswordBlockFingerprintUnlock=");
b.append(this.workProfilePasswordBlockFingerprintUnlock);
b.append(", ");
b.append("workProfilePasswordBlockIrisUnlock=");
b.append(this.workProfilePasswordBlockIrisUnlock);
b.append(", ");
b.append("workProfilePasswordBlockTrustAgents=");
b.append(this.workProfilePasswordBlockTrustAgents);
b.append(", ");
b.append("workProfilePasswordExpirationDays=");
b.append(this.workProfilePasswordExpirationDays);
b.append(", ");
b.append("workProfilePasswordMinimumLength=");
b.append(this.workProfilePasswordMinimumLength);
b.append(", ");
b.append("workProfilePasswordMinLetterCharacters=");
b.append(this.workProfilePasswordMinLetterCharacters);
b.append(", ");
b.append("workProfilePasswordMinLowerCaseCharacters=");
b.append(this.workProfilePasswordMinLowerCaseCharacters);
b.append(", ");
b.append("workProfilePasswordMinNonLetterCharacters=");
b.append(this.workProfilePasswordMinNonLetterCharacters);
b.append(", ");
b.append("workProfilePasswordMinNumericCharacters=");
b.append(this.workProfilePasswordMinNumericCharacters);
b.append(", ");
b.append("workProfilePasswordMinSymbolCharacters=");
b.append(this.workProfilePasswordMinSymbolCharacters);
b.append(", ");
b.append("workProfilePasswordMinUpperCaseCharacters=");
b.append(this.workProfilePasswordMinUpperCaseCharacters);
b.append(", ");
b.append("workProfilePasswordMinutesOfInactivityBeforeScreenTimeout=");
b.append(this.workProfilePasswordMinutesOfInactivityBeforeScreenTimeout);
b.append(", ");
b.append("workProfilePasswordPreviousPasswordBlockCount=");
b.append(this.workProfilePasswordPreviousPasswordBlockCount);
b.append(", ");
b.append("workProfilePasswordRequiredType=");
b.append(this.workProfilePasswordRequiredType);
b.append(", ");
b.append("workProfilePasswordSignInFailureCountBeforeFactoryReset=");
b.append(this.workProfilePasswordSignInFailureCountBeforeFactoryReset);
b.append(", ");
b.append("workProfileRequirePassword=");
b.append(this.workProfileRequirePassword);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}