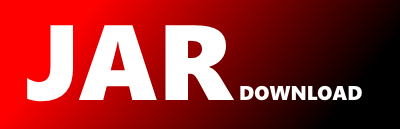
odata.msgraph.client.beta.entity.AndroidForWorkNineWorkEasConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleDeviceMode;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsEdition;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsVersion;
import odata.msgraph.client.beta.enums.AndroidUsernameSource;
import odata.msgraph.client.beta.enums.EasAuthenticationMethod;
import odata.msgraph.client.beta.enums.EmailSyncDuration;
import odata.msgraph.client.beta.enums.UserEmailSource;
/**
* “By providing configurations in this profile you can instruct the Nine Work email
* client on Android For Work devices to communicate with an Exchange server and
* get email, contacts, calendar, tasks, and notes. Furthermore, you can also
* specify how much email to sync and how often the device should sync.”
*/@JsonPropertyOrder({
"@odata.type",
"syncCalendar",
"syncContacts",
"syncTasks"})
@JsonInclude(Include.NON_NULL)
public class AndroidForWorkNineWorkEasConfiguration extends AndroidForWorkEasEmailProfileBase implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.androidForWorkNineWorkEasConfiguration";
}
@JsonProperty("syncCalendar")
protected Boolean syncCalendar;
@JsonProperty("syncContacts")
protected Boolean syncContacts;
@JsonProperty("syncTasks")
protected Boolean syncTasks;
protected AndroidForWorkNineWorkEasConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderAndroidForWorkNineWorkEasConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode;
private DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition;
private DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private Boolean supportsScopeTags;
private Integer version;
private EasAuthenticationMethod authenticationMethod;
private EmailSyncDuration durationOfEmailToSync;
private UserEmailSource emailAddressSource;
private String hostName;
private Boolean requireSsl;
private AndroidUsernameSource usernameSource;
private Boolean syncCalendar;
private Boolean syncContacts;
private Boolean syncTasks;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder deviceManagementApplicabilityRuleDeviceMode(DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode) {
this.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleDeviceMode");
return this;
}
public Builder deviceManagementApplicabilityRuleOsEdition(DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition) {
this.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsEdition");
return this;
}
public Builder deviceManagementApplicabilityRuleOsVersion(DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion) {
this.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsVersion");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder supportsScopeTags(Boolean supportsScopeTags) {
this.supportsScopeTags = supportsScopeTags;
this.changedFields = changedFields.add("supportsScopeTags");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
public Builder authenticationMethod(EasAuthenticationMethod authenticationMethod) {
this.authenticationMethod = authenticationMethod;
this.changedFields = changedFields.add("authenticationMethod");
return this;
}
public Builder durationOfEmailToSync(EmailSyncDuration durationOfEmailToSync) {
this.durationOfEmailToSync = durationOfEmailToSync;
this.changedFields = changedFields.add("durationOfEmailToSync");
return this;
}
public Builder emailAddressSource(UserEmailSource emailAddressSource) {
this.emailAddressSource = emailAddressSource;
this.changedFields = changedFields.add("emailAddressSource");
return this;
}
public Builder hostName(String hostName) {
this.hostName = hostName;
this.changedFields = changedFields.add("hostName");
return this;
}
public Builder requireSsl(Boolean requireSsl) {
this.requireSsl = requireSsl;
this.changedFields = changedFields.add("requireSsl");
return this;
}
public Builder usernameSource(AndroidUsernameSource usernameSource) {
this.usernameSource = usernameSource;
this.changedFields = changedFields.add("usernameSource");
return this;
}
/**
* “Toggles syncing the calendar. If set to false the calendar is turned off on the
* device.”
*
* @param syncCalendar
* value of {@code syncCalendar} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder syncCalendar(Boolean syncCalendar) {
this.syncCalendar = syncCalendar;
this.changedFields = changedFields.add("syncCalendar");
return this;
}
/**
* “Toggles syncing contacts. If set to false contacts are turned off on the device.”
*
* @param syncContacts
* value of {@code syncContacts} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder syncContacts(Boolean syncContacts) {
this.syncContacts = syncContacts;
this.changedFields = changedFields.add("syncContacts");
return this;
}
/**
* “Toggles syncing tasks. If set to false tasks are turned off on the device.”
*
* @param syncTasks
* value of {@code syncTasks} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder syncTasks(Boolean syncTasks) {
this.syncTasks = syncTasks;
this.changedFields = changedFields.add("syncTasks");
return this;
}
public AndroidForWorkNineWorkEasConfiguration build() {
AndroidForWorkNineWorkEasConfiguration _x = new AndroidForWorkNineWorkEasConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.androidForWorkNineWorkEasConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.authenticationMethod = authenticationMethod;
_x.durationOfEmailToSync = durationOfEmailToSync;
_x.emailAddressSource = emailAddressSource;
_x.hostName = hostName;
_x.requireSsl = requireSsl;
_x.usernameSource = usernameSource;
_x.syncCalendar = syncCalendar;
_x.syncContacts = syncContacts;
_x.syncTasks = syncTasks;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Toggles syncing the calendar. If set to false the calendar is turned off on the
* device.”
*
* @return property syncCalendar
*/
@Property(name="syncCalendar")
@JsonIgnore
public Optional getSyncCalendar() {
return Optional.ofNullable(syncCalendar);
}
/**
* Returns an immutable copy of {@code this} with just the {@code syncCalendar}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Toggles syncing the calendar. If set to false the calendar is turned off on the
* device.”
*
* @param syncCalendar
* new value of {@code syncCalendar} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code syncCalendar} field changed
*/
public AndroidForWorkNineWorkEasConfiguration withSyncCalendar(Boolean syncCalendar) {
AndroidForWorkNineWorkEasConfiguration _x = _copy();
_x.changedFields = changedFields.add("syncCalendar");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkNineWorkEasConfiguration");
_x.syncCalendar = syncCalendar;
return _x;
}
/**
* “Toggles syncing contacts. If set to false contacts are turned off on the device.”
*
* @return property syncContacts
*/
@Property(name="syncContacts")
@JsonIgnore
public Optional getSyncContacts() {
return Optional.ofNullable(syncContacts);
}
/**
* Returns an immutable copy of {@code this} with just the {@code syncContacts}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Toggles syncing contacts. If set to false contacts are turned off on the device.”
*
* @param syncContacts
* new value of {@code syncContacts} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code syncContacts} field changed
*/
public AndroidForWorkNineWorkEasConfiguration withSyncContacts(Boolean syncContacts) {
AndroidForWorkNineWorkEasConfiguration _x = _copy();
_x.changedFields = changedFields.add("syncContacts");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkNineWorkEasConfiguration");
_x.syncContacts = syncContacts;
return _x;
}
/**
* “Toggles syncing tasks. If set to false tasks are turned off on the device.”
*
* @return property syncTasks
*/
@Property(name="syncTasks")
@JsonIgnore
public Optional getSyncTasks() {
return Optional.ofNullable(syncTasks);
}
/**
* Returns an immutable copy of {@code this} with just the {@code syncTasks} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Toggles syncing tasks. If set to false tasks are turned off on the device.”
*
* @param syncTasks
* new value of {@code syncTasks} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code syncTasks} field changed
*/
public AndroidForWorkNineWorkEasConfiguration withSyncTasks(Boolean syncTasks) {
AndroidForWorkNineWorkEasConfiguration _x = _copy();
_x.changedFields = changedFields.add("syncTasks");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidForWorkNineWorkEasConfiguration");
_x.syncTasks = syncTasks;
return _x;
}
public AndroidForWorkNineWorkEasConfiguration withUnmappedField(String name, String value) {
AndroidForWorkNineWorkEasConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public AndroidForWorkNineWorkEasConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
AndroidForWorkNineWorkEasConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public AndroidForWorkNineWorkEasConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
AndroidForWorkNineWorkEasConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private AndroidForWorkNineWorkEasConfiguration _copy() {
AndroidForWorkNineWorkEasConfiguration _x = new AndroidForWorkNineWorkEasConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.authenticationMethod = authenticationMethod;
_x.durationOfEmailToSync = durationOfEmailToSync;
_x.emailAddressSource = emailAddressSource;
_x.hostName = hostName;
_x.requireSsl = requireSsl;
_x.usernameSource = usernameSource;
_x.syncCalendar = syncCalendar;
_x.syncContacts = syncContacts;
_x.syncTasks = syncTasks;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("AndroidForWorkNineWorkEasConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("deviceManagementApplicabilityRuleDeviceMode=");
b.append(this.deviceManagementApplicabilityRuleDeviceMode);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsEdition=");
b.append(this.deviceManagementApplicabilityRuleOsEdition);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsVersion=");
b.append(this.deviceManagementApplicabilityRuleOsVersion);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("supportsScopeTags=");
b.append(this.supportsScopeTags);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("authenticationMethod=");
b.append(this.authenticationMethod);
b.append(", ");
b.append("durationOfEmailToSync=");
b.append(this.durationOfEmailToSync);
b.append(", ");
b.append("emailAddressSource=");
b.append(this.emailAddressSource);
b.append(", ");
b.append("hostName=");
b.append(this.hostName);
b.append(", ");
b.append("requireSsl=");
b.append(this.requireSsl);
b.append(", ");
b.append("usernameSource=");
b.append(this.usernameSource);
b.append(", ");
b.append("syncCalendar=");
b.append(this.syncCalendar);
b.append(", ");
b.append("syncContacts=");
b.append(this.syncContacts);
b.append(", ");
b.append("syncTasks=");
b.append(this.syncTasks);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}