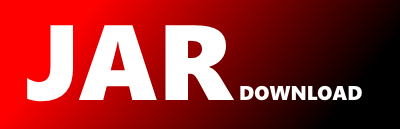
odata.msgraph.client.beta.entity.ConversationThread Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.guavamini.Preconditions;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import odata.msgraph.client.beta.complex.Recipient;
import odata.msgraph.client.beta.entity.collection.request.PostCollectionRequest;
@JsonPropertyOrder({
"@odata.type",
"ccRecipients",
"hasAttachments",
"isLocked",
"lastDeliveredDateTime",
"preview",
"topic",
"toRecipients",
"uniqueSenders"})
@JsonInclude(Include.NON_NULL)
public class ConversationThread extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.conversationThread";
}
@JsonProperty("ccRecipients")
protected List ccRecipients;
@JsonProperty("ccRecipients@nextLink")
protected String ccRecipientsNextLink;
@JsonProperty("hasAttachments")
protected Boolean hasAttachments;
@JsonProperty("isLocked")
protected Boolean isLocked;
@JsonProperty("lastDeliveredDateTime")
protected OffsetDateTime lastDeliveredDateTime;
@JsonProperty("preview")
protected String preview;
@JsonProperty("topic")
protected String topic;
@JsonProperty("toRecipients")
protected List toRecipients;
@JsonProperty("toRecipients@nextLink")
protected String toRecipientsNextLink;
@JsonProperty("uniqueSenders")
protected List uniqueSenders;
@JsonProperty("uniqueSenders@nextLink")
protected String uniqueSendersNextLink;
protected ConversationThread() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderConversationThread() {
return new Builder();
}
public static final class Builder {
private String id;
private List ccRecipients;
private String ccRecipientsNextLink;
private Boolean hasAttachments;
private Boolean isLocked;
private OffsetDateTime lastDeliveredDateTime;
private String preview;
private String topic;
private List toRecipients;
private String toRecipientsNextLink;
private List uniqueSenders;
private String uniqueSendersNextLink;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder ccRecipients(List ccRecipients) {
this.ccRecipients = ccRecipients;
this.changedFields = changedFields.add("ccRecipients");
return this;
}
public Builder ccRecipients(Recipient... ccRecipients) {
return ccRecipients(Arrays.asList(ccRecipients));
}
public Builder ccRecipientsNextLink(String ccRecipientsNextLink) {
this.ccRecipientsNextLink = ccRecipientsNextLink;
this.changedFields = changedFields.add("ccRecipients");
return this;
}
public Builder hasAttachments(Boolean hasAttachments) {
this.hasAttachments = hasAttachments;
this.changedFields = changedFields.add("hasAttachments");
return this;
}
public Builder isLocked(Boolean isLocked) {
this.isLocked = isLocked;
this.changedFields = changedFields.add("isLocked");
return this;
}
public Builder lastDeliveredDateTime(OffsetDateTime lastDeliveredDateTime) {
this.lastDeliveredDateTime = lastDeliveredDateTime;
this.changedFields = changedFields.add("lastDeliveredDateTime");
return this;
}
public Builder preview(String preview) {
this.preview = preview;
this.changedFields = changedFields.add("preview");
return this;
}
public Builder topic(String topic) {
this.topic = topic;
this.changedFields = changedFields.add("topic");
return this;
}
public Builder toRecipients(List toRecipients) {
this.toRecipients = toRecipients;
this.changedFields = changedFields.add("toRecipients");
return this;
}
public Builder toRecipients(Recipient... toRecipients) {
return toRecipients(Arrays.asList(toRecipients));
}
public Builder toRecipientsNextLink(String toRecipientsNextLink) {
this.toRecipientsNextLink = toRecipientsNextLink;
this.changedFields = changedFields.add("toRecipients");
return this;
}
public Builder uniqueSenders(List uniqueSenders) {
this.uniqueSenders = uniqueSenders;
this.changedFields = changedFields.add("uniqueSenders");
return this;
}
public Builder uniqueSenders(String... uniqueSenders) {
return uniqueSenders(Arrays.asList(uniqueSenders));
}
public Builder uniqueSendersNextLink(String uniqueSendersNextLink) {
this.uniqueSendersNextLink = uniqueSendersNextLink;
this.changedFields = changedFields.add("uniqueSenders");
return this;
}
public ConversationThread build() {
ConversationThread _x = new ConversationThread();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.conversationThread";
_x.id = id;
_x.ccRecipients = ccRecipients;
_x.ccRecipientsNextLink = ccRecipientsNextLink;
_x.hasAttachments = hasAttachments;
_x.isLocked = isLocked;
_x.lastDeliveredDateTime = lastDeliveredDateTime;
_x.preview = preview;
_x.topic = topic;
_x.toRecipients = toRecipients;
_x.toRecipientsNextLink = toRecipientsNextLink;
_x.uniqueSenders = uniqueSenders;
_x.uniqueSendersNextLink = uniqueSendersNextLink;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
@Property(name="ccRecipients")
@JsonIgnore
public CollectionPage getCcRecipients() {
return new CollectionPage(contextPath, Recipient.class, this.ccRecipients, Optional.ofNullable(ccRecipientsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public ConversationThread withCcRecipients(List ccRecipients) {
ConversationThread _x = _copy();
_x.changedFields = changedFields.add("ccRecipients");
_x.odataType = Util.nvl(odataType, "microsoft.graph.conversationThread");
_x.ccRecipients = ccRecipients;
return _x;
}
@Property(name="ccRecipients")
@JsonIgnore
public CollectionPage getCcRecipients(HttpRequestOptions options) {
return new CollectionPage(contextPath, Recipient.class, this.ccRecipients, Optional.ofNullable(ccRecipientsNextLink), Collections.emptyList(), options);
}
@Property(name="hasAttachments")
@JsonIgnore
public Optional getHasAttachments() {
return Optional.ofNullable(hasAttachments);
}
public ConversationThread withHasAttachments(Boolean hasAttachments) {
ConversationThread _x = _copy();
_x.changedFields = changedFields.add("hasAttachments");
_x.odataType = Util.nvl(odataType, "microsoft.graph.conversationThread");
_x.hasAttachments = hasAttachments;
return _x;
}
@Property(name="isLocked")
@JsonIgnore
public Optional getIsLocked() {
return Optional.ofNullable(isLocked);
}
public ConversationThread withIsLocked(Boolean isLocked) {
ConversationThread _x = _copy();
_x.changedFields = changedFields.add("isLocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.conversationThread");
_x.isLocked = isLocked;
return _x;
}
@Property(name="lastDeliveredDateTime")
@JsonIgnore
public Optional getLastDeliveredDateTime() {
return Optional.ofNullable(lastDeliveredDateTime);
}
public ConversationThread withLastDeliveredDateTime(OffsetDateTime lastDeliveredDateTime) {
ConversationThread _x = _copy();
_x.changedFields = changedFields.add("lastDeliveredDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.conversationThread");
_x.lastDeliveredDateTime = lastDeliveredDateTime;
return _x;
}
@Property(name="preview")
@JsonIgnore
public Optional getPreview() {
return Optional.ofNullable(preview);
}
public ConversationThread withPreview(String preview) {
ConversationThread _x = _copy();
_x.changedFields = changedFields.add("preview");
_x.odataType = Util.nvl(odataType, "microsoft.graph.conversationThread");
_x.preview = preview;
return _x;
}
@Property(name="topic")
@JsonIgnore
public Optional getTopic() {
return Optional.ofNullable(topic);
}
public ConversationThread withTopic(String topic) {
ConversationThread _x = _copy();
_x.changedFields = changedFields.add("topic");
_x.odataType = Util.nvl(odataType, "microsoft.graph.conversationThread");
_x.topic = topic;
return _x;
}
@Property(name="toRecipients")
@JsonIgnore
public CollectionPage getToRecipients() {
return new CollectionPage(contextPath, Recipient.class, this.toRecipients, Optional.ofNullable(toRecipientsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public ConversationThread withToRecipients(List toRecipients) {
ConversationThread _x = _copy();
_x.changedFields = changedFields.add("toRecipients");
_x.odataType = Util.nvl(odataType, "microsoft.graph.conversationThread");
_x.toRecipients = toRecipients;
return _x;
}
@Property(name="toRecipients")
@JsonIgnore
public CollectionPage getToRecipients(HttpRequestOptions options) {
return new CollectionPage(contextPath, Recipient.class, this.toRecipients, Optional.ofNullable(toRecipientsNextLink), Collections.emptyList(), options);
}
@Property(name="uniqueSenders")
@JsonIgnore
public CollectionPage getUniqueSenders() {
return new CollectionPage(contextPath, String.class, this.uniqueSenders, Optional.ofNullable(uniqueSendersNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public ConversationThread withUniqueSenders(List uniqueSenders) {
ConversationThread _x = _copy();
_x.changedFields = changedFields.add("uniqueSenders");
_x.odataType = Util.nvl(odataType, "microsoft.graph.conversationThread");
_x.uniqueSenders = uniqueSenders;
return _x;
}
@Property(name="uniqueSenders")
@JsonIgnore
public CollectionPage getUniqueSenders(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.uniqueSenders, Optional.ofNullable(uniqueSendersNextLink), Collections.emptyList(), options);
}
public ConversationThread withUnmappedField(String name, String value) {
ConversationThread _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
*
* Org.OData.Capabilities.V1.ChangeTracking
*
* Supported = false
*
* Org.OData.Capabilities.V1.DeleteRestrictions
*
* Deletable = false
*
* Org.OData.Capabilities.V1.InsertRestrictions
*
* Insertable = false
*
* Org.OData.Capabilities.V1.SearchRestrictions
*
* Searchable = false
*
* @return navigational property posts
*/
@NavigationProperty(name="posts")
@JsonIgnore
public PostCollectionRequest getPosts() {
return new PostCollectionRequest(
contextPath.addSegment("posts"), RequestHelper.getValue(unmappedFields, "posts"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ConversationThread patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
ConversationThread _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ConversationThread put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
ConversationThread _x = _copy();
_x.changedFields = null;
return _x;
}
private ConversationThread _copy() {
ConversationThread _x = new ConversationThread();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.ccRecipients = ccRecipients;
_x.hasAttachments = hasAttachments;
_x.isLocked = isLocked;
_x.lastDeliveredDateTime = lastDeliveredDateTime;
_x.preview = preview;
_x.topic = topic;
_x.toRecipients = toRecipients;
_x.uniqueSenders = uniqueSenders;
return _x;
}
@Action(name = "reply")
@JsonIgnore
public ActionRequestNoReturn reply(Post post) {
Preconditions.checkNotNull(post, "post cannot be null");
Map _parameters = ParameterMap
.put("Post", "microsoft.graph.post", post)
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("microsoft.graph.reply"), _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ConversationThread[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("ccRecipients=");
b.append(this.ccRecipients);
b.append(", ");
b.append("hasAttachments=");
b.append(this.hasAttachments);
b.append(", ");
b.append("isLocked=");
b.append(this.isLocked);
b.append(", ");
b.append("lastDeliveredDateTime=");
b.append(this.lastDeliveredDateTime);
b.append(", ");
b.append("preview=");
b.append(this.preview);
b.append(", ");
b.append("topic=");
b.append(this.topic);
b.append(", ");
b.append("toRecipients=");
b.append(this.toRecipients);
b.append(", ");
b.append("uniqueSenders=");
b.append(this.uniqueSenders);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}