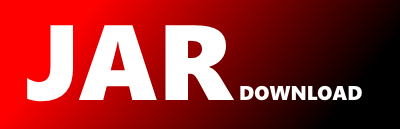
odata.msgraph.client.beta.entity.Customer Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Optional;
import odata.msgraph.client.beta.complex.PostalAddressType;
import odata.msgraph.client.beta.entity.collection.request.PictureCollectionRequest;
import odata.msgraph.client.beta.entity.request.CurrencyRequest;
import odata.msgraph.client.beta.entity.request.PaymentMethodRequest;
import odata.msgraph.client.beta.entity.request.PaymentTermRequest;
import odata.msgraph.client.beta.entity.request.ShipmentMethodRequest;
/**
*
* Org.OData.Capabilities.V1.DeleteRestrictions
*
* Deletable = true
*
* Org.OData.Capabilities.V1.InsertRestrictions
*
* Insertable = true
*
* Org.OData.Capabilities.V1.UpdateRestrictions
*
* Updatable = true
*/@JsonPropertyOrder({
"@odata.type",
"address",
"blocked",
"currencyCode",
"currencyId",
"displayName",
"email",
"lastModifiedDateTime",
"number",
"paymentMethodId",
"paymentTermsId",
"phoneNumber",
"shipmentMethodId",
"taxAreaDisplayName",
"taxAreaId",
"taxLiable",
"taxRegistrationNumber",
"type",
"website"})
@JsonInclude(Include.NON_NULL)
public class Customer extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.customer";
}
@JsonProperty("address")
protected PostalAddressType address;
@JsonProperty("blocked")
protected String blocked;
@JsonProperty("currencyCode")
protected String currencyCode;
@JsonProperty("currencyId")
protected String currencyId;
@JsonProperty("displayName")
protected String displayName;
@JsonProperty("email")
protected String email;
@JsonProperty("lastModifiedDateTime")
protected OffsetDateTime lastModifiedDateTime;
@JsonProperty("number")
protected String number;
@JsonProperty("paymentMethodId")
protected String paymentMethodId;
@JsonProperty("paymentTermsId")
protected String paymentTermsId;
@JsonProperty("phoneNumber")
protected String phoneNumber;
@JsonProperty("shipmentMethodId")
protected String shipmentMethodId;
@JsonProperty("taxAreaDisplayName")
protected String taxAreaDisplayName;
@JsonProperty("taxAreaId")
protected String taxAreaId;
@JsonProperty("taxLiable")
protected Boolean taxLiable;
@JsonProperty("taxRegistrationNumber")
protected String taxRegistrationNumber;
@JsonProperty("type")
protected String type;
@JsonProperty("website")
protected String website;
protected Customer() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderCustomer() {
return new Builder();
}
public static final class Builder {
private String id;
private PostalAddressType address;
private String blocked;
private String currencyCode;
private String currencyId;
private String displayName;
private String email;
private OffsetDateTime lastModifiedDateTime;
private String number;
private String paymentMethodId;
private String paymentTermsId;
private String phoneNumber;
private String shipmentMethodId;
private String taxAreaDisplayName;
private String taxAreaId;
private Boolean taxLiable;
private String taxRegistrationNumber;
private String type;
private String website;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder address(PostalAddressType address) {
this.address = address;
this.changedFields = changedFields.add("address");
return this;
}
public Builder blocked(String blocked) {
this.blocked = blocked;
this.changedFields = changedFields.add("blocked");
return this;
}
public Builder currencyCode(String currencyCode) {
this.currencyCode = currencyCode;
this.changedFields = changedFields.add("currencyCode");
return this;
}
public Builder currencyId(String currencyId) {
this.currencyId = currencyId;
this.changedFields = changedFields.add("currencyId");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder email(String email) {
this.email = email;
this.changedFields = changedFields.add("email");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder number(String number) {
this.number = number;
this.changedFields = changedFields.add("number");
return this;
}
public Builder paymentMethodId(String paymentMethodId) {
this.paymentMethodId = paymentMethodId;
this.changedFields = changedFields.add("paymentMethodId");
return this;
}
public Builder paymentTermsId(String paymentTermsId) {
this.paymentTermsId = paymentTermsId;
this.changedFields = changedFields.add("paymentTermsId");
return this;
}
public Builder phoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
this.changedFields = changedFields.add("phoneNumber");
return this;
}
public Builder shipmentMethodId(String shipmentMethodId) {
this.shipmentMethodId = shipmentMethodId;
this.changedFields = changedFields.add("shipmentMethodId");
return this;
}
public Builder taxAreaDisplayName(String taxAreaDisplayName) {
this.taxAreaDisplayName = taxAreaDisplayName;
this.changedFields = changedFields.add("taxAreaDisplayName");
return this;
}
public Builder taxAreaId(String taxAreaId) {
this.taxAreaId = taxAreaId;
this.changedFields = changedFields.add("taxAreaId");
return this;
}
public Builder taxLiable(Boolean taxLiable) {
this.taxLiable = taxLiable;
this.changedFields = changedFields.add("taxLiable");
return this;
}
public Builder taxRegistrationNumber(String taxRegistrationNumber) {
this.taxRegistrationNumber = taxRegistrationNumber;
this.changedFields = changedFields.add("taxRegistrationNumber");
return this;
}
public Builder type(String type) {
this.type = type;
this.changedFields = changedFields.add("type");
return this;
}
public Builder website(String website) {
this.website = website;
this.changedFields = changedFields.add("website");
return this;
}
public Customer build() {
Customer _x = new Customer();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.customer";
_x.id = id;
_x.address = address;
_x.blocked = blocked;
_x.currencyCode = currencyCode;
_x.currencyId = currencyId;
_x.displayName = displayName;
_x.email = email;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.number = number;
_x.paymentMethodId = paymentMethodId;
_x.paymentTermsId = paymentTermsId;
_x.phoneNumber = phoneNumber;
_x.shipmentMethodId = shipmentMethodId;
_x.taxAreaDisplayName = taxAreaDisplayName;
_x.taxAreaId = taxAreaId;
_x.taxLiable = taxLiable;
_x.taxRegistrationNumber = taxRegistrationNumber;
_x.type = type;
_x.website = website;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
@Property(name="address")
@JsonIgnore
public Optional getAddress() {
return Optional.ofNullable(address);
}
public Customer withAddress(PostalAddressType address) {
Customer _x = _copy();
_x.changedFields = changedFields.add("address");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.address = address;
return _x;
}
@Property(name="blocked")
@JsonIgnore
public Optional getBlocked() {
return Optional.ofNullable(blocked);
}
public Customer withBlocked(String blocked) {
Customer _x = _copy();
_x.changedFields = changedFields.add("blocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.blocked = blocked;
return _x;
}
@Property(name="currencyCode")
@JsonIgnore
public Optional getCurrencyCode() {
return Optional.ofNullable(currencyCode);
}
public Customer withCurrencyCode(String currencyCode) {
Customer _x = _copy();
_x.changedFields = changedFields.add("currencyCode");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.currencyCode = currencyCode;
return _x;
}
@Property(name="currencyId")
@JsonIgnore
public Optional getCurrencyId() {
return Optional.ofNullable(currencyId);
}
public Customer withCurrencyId(String currencyId) {
Customer _x = _copy();
_x.changedFields = changedFields.add("currencyId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.currencyId = currencyId;
return _x;
}
@Property(name="displayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
public Customer withDisplayName(String displayName) {
Customer _x = _copy();
_x.changedFields = changedFields.add("displayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.displayName = displayName;
return _x;
}
@Property(name="email")
@JsonIgnore
public Optional getEmail() {
return Optional.ofNullable(email);
}
public Customer withEmail(String email) {
Customer _x = _copy();
_x.changedFields = changedFields.add("email");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.email = email;
return _x;
}
@Property(name="lastModifiedDateTime")
@JsonIgnore
public Optional getLastModifiedDateTime() {
return Optional.ofNullable(lastModifiedDateTime);
}
public Customer withLastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
Customer _x = _copy();
_x.changedFields = changedFields.add("lastModifiedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.lastModifiedDateTime = lastModifiedDateTime;
return _x;
}
@Property(name="number")
@JsonIgnore
public Optional getNumber() {
return Optional.ofNullable(number);
}
public Customer withNumber(String number) {
Customer _x = _copy();
_x.changedFields = changedFields.add("number");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.number = number;
return _x;
}
@Property(name="paymentMethodId")
@JsonIgnore
public Optional getPaymentMethodId() {
return Optional.ofNullable(paymentMethodId);
}
public Customer withPaymentMethodId(String paymentMethodId) {
Customer _x = _copy();
_x.changedFields = changedFields.add("paymentMethodId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.paymentMethodId = paymentMethodId;
return _x;
}
@Property(name="paymentTermsId")
@JsonIgnore
public Optional getPaymentTermsId() {
return Optional.ofNullable(paymentTermsId);
}
public Customer withPaymentTermsId(String paymentTermsId) {
Customer _x = _copy();
_x.changedFields = changedFields.add("paymentTermsId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.paymentTermsId = paymentTermsId;
return _x;
}
@Property(name="phoneNumber")
@JsonIgnore
public Optional getPhoneNumber() {
return Optional.ofNullable(phoneNumber);
}
public Customer withPhoneNumber(String phoneNumber) {
Customer _x = _copy();
_x.changedFields = changedFields.add("phoneNumber");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.phoneNumber = phoneNumber;
return _x;
}
@Property(name="shipmentMethodId")
@JsonIgnore
public Optional getShipmentMethodId() {
return Optional.ofNullable(shipmentMethodId);
}
public Customer withShipmentMethodId(String shipmentMethodId) {
Customer _x = _copy();
_x.changedFields = changedFields.add("shipmentMethodId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.shipmentMethodId = shipmentMethodId;
return _x;
}
@Property(name="taxAreaDisplayName")
@JsonIgnore
public Optional getTaxAreaDisplayName() {
return Optional.ofNullable(taxAreaDisplayName);
}
public Customer withTaxAreaDisplayName(String taxAreaDisplayName) {
Customer _x = _copy();
_x.changedFields = changedFields.add("taxAreaDisplayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.taxAreaDisplayName = taxAreaDisplayName;
return _x;
}
@Property(name="taxAreaId")
@JsonIgnore
public Optional getTaxAreaId() {
return Optional.ofNullable(taxAreaId);
}
public Customer withTaxAreaId(String taxAreaId) {
Customer _x = _copy();
_x.changedFields = changedFields.add("taxAreaId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.taxAreaId = taxAreaId;
return _x;
}
@Property(name="taxLiable")
@JsonIgnore
public Optional getTaxLiable() {
return Optional.ofNullable(taxLiable);
}
public Customer withTaxLiable(Boolean taxLiable) {
Customer _x = _copy();
_x.changedFields = changedFields.add("taxLiable");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.taxLiable = taxLiable;
return _x;
}
@Property(name="taxRegistrationNumber")
@JsonIgnore
public Optional getTaxRegistrationNumber() {
return Optional.ofNullable(taxRegistrationNumber);
}
public Customer withTaxRegistrationNumber(String taxRegistrationNumber) {
Customer _x = _copy();
_x.changedFields = changedFields.add("taxRegistrationNumber");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.taxRegistrationNumber = taxRegistrationNumber;
return _x;
}
@Property(name="type")
@JsonIgnore
public Optional getType() {
return Optional.ofNullable(type);
}
public Customer withType(String type) {
Customer _x = _copy();
_x.changedFields = changedFields.add("type");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.type = type;
return _x;
}
@Property(name="website")
@JsonIgnore
public Optional getWebsite() {
return Optional.ofNullable(website);
}
public Customer withWebsite(String website) {
Customer _x = _copy();
_x.changedFields = changedFields.add("website");
_x.odataType = Util.nvl(odataType, "microsoft.graph.customer");
_x.website = website;
return _x;
}
public Customer withUnmappedField(String name, String value) {
Customer _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="currency")
@JsonIgnore
public CurrencyRequest getCurrency() {
return new CurrencyRequest(contextPath.addSegment("currency"), RequestHelper.getValue(unmappedFields, "currency"));
}
@NavigationProperty(name="paymentMethod")
@JsonIgnore
public PaymentMethodRequest getPaymentMethod() {
return new PaymentMethodRequest(contextPath.addSegment("paymentMethod"), RequestHelper.getValue(unmappedFields, "paymentMethod"));
}
@NavigationProperty(name="paymentTerm")
@JsonIgnore
public PaymentTermRequest getPaymentTerm() {
return new PaymentTermRequest(contextPath.addSegment("paymentTerm"), RequestHelper.getValue(unmappedFields, "paymentTerm"));
}
@NavigationProperty(name="picture")
@JsonIgnore
public PictureCollectionRequest getPicture() {
return new PictureCollectionRequest(
contextPath.addSegment("picture"), RequestHelper.getValue(unmappedFields, "picture"));
}
@NavigationProperty(name="shipmentMethod")
@JsonIgnore
public ShipmentMethodRequest getShipmentMethod() {
return new ShipmentMethodRequest(contextPath.addSegment("shipmentMethod"), RequestHelper.getValue(unmappedFields, "shipmentMethod"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Customer patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Customer _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Customer put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Customer _x = _copy();
_x.changedFields = null;
return _x;
}
private Customer _copy() {
Customer _x = new Customer();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.address = address;
_x.blocked = blocked;
_x.currencyCode = currencyCode;
_x.currencyId = currencyId;
_x.displayName = displayName;
_x.email = email;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.number = number;
_x.paymentMethodId = paymentMethodId;
_x.paymentTermsId = paymentTermsId;
_x.phoneNumber = phoneNumber;
_x.shipmentMethodId = shipmentMethodId;
_x.taxAreaDisplayName = taxAreaDisplayName;
_x.taxAreaId = taxAreaId;
_x.taxLiable = taxLiable;
_x.taxRegistrationNumber = taxRegistrationNumber;
_x.type = type;
_x.website = website;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Customer[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("address=");
b.append(this.address);
b.append(", ");
b.append("blocked=");
b.append(this.blocked);
b.append(", ");
b.append("currencyCode=");
b.append(this.currencyCode);
b.append(", ");
b.append("currencyId=");
b.append(this.currencyId);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("email=");
b.append(this.email);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("number=");
b.append(this.number);
b.append(", ");
b.append("paymentMethodId=");
b.append(this.paymentMethodId);
b.append(", ");
b.append("paymentTermsId=");
b.append(this.paymentTermsId);
b.append(", ");
b.append("phoneNumber=");
b.append(this.phoneNumber);
b.append(", ");
b.append("shipmentMethodId=");
b.append(this.shipmentMethodId);
b.append(", ");
b.append("taxAreaDisplayName=");
b.append(this.taxAreaDisplayName);
b.append(", ");
b.append("taxAreaId=");
b.append(this.taxAreaId);
b.append(", ");
b.append("taxLiable=");
b.append(this.taxLiable);
b.append(", ");
b.append("taxRegistrationNumber=");
b.append(this.taxRegistrationNumber);
b.append(", ");
b.append("type=");
b.append(this.type);
b.append(", ");
b.append("website=");
b.append(this.website);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}