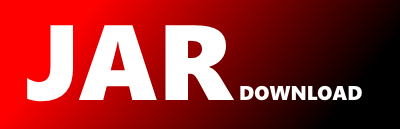
odata.msgraph.client.beta.entity.DeviceManagementComplexSettingDefinition Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.complex.DeviceManagementConstraint;
import odata.msgraph.client.beta.complex.DeviceManagementSettingDependency;
import odata.msgraph.client.beta.enums.DeviceManangementIntentValueType;
/**
* “Entity representing the defintion for a complex setting”
*/@JsonPropertyOrder({
"@odata.type",
"propertyDefinitionIds"})
@JsonInclude(Include.NON_NULL)
public class DeviceManagementComplexSettingDefinition extends DeviceManagementSettingDefinition implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.deviceManagementComplexSettingDefinition";
}
@JsonProperty("propertyDefinitionIds")
protected List propertyDefinitionIds;
@JsonProperty("propertyDefinitionIds@nextLink")
protected String propertyDefinitionIdsNextLink;
protected DeviceManagementComplexSettingDefinition() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderDeviceManagementComplexSettingDefinition() {
return new Builder();
}
public static final class Builder {
private String id;
private List constraints;
private String constraintsNextLink;
private List dependencies;
private String dependenciesNextLink;
private String description;
private String displayName;
private String documentationUrl;
private String headerSubtitle;
private String headerTitle;
private Boolean isTopLevel;
private List keywords;
private String keywordsNextLink;
private String placeholderText;
private DeviceManangementIntentValueType valueType;
private List propertyDefinitionIds;
private String propertyDefinitionIdsNextLink;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder constraints(List constraints) {
this.constraints = constraints;
this.changedFields = changedFields.add("constraints");
return this;
}
public Builder constraints(DeviceManagementConstraint... constraints) {
return constraints(Arrays.asList(constraints));
}
public Builder constraintsNextLink(String constraintsNextLink) {
this.constraintsNextLink = constraintsNextLink;
this.changedFields = changedFields.add("constraints");
return this;
}
public Builder dependencies(List dependencies) {
this.dependencies = dependencies;
this.changedFields = changedFields.add("dependencies");
return this;
}
public Builder dependencies(DeviceManagementSettingDependency... dependencies) {
return dependencies(Arrays.asList(dependencies));
}
public Builder dependenciesNextLink(String dependenciesNextLink) {
this.dependenciesNextLink = dependenciesNextLink;
this.changedFields = changedFields.add("dependencies");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder documentationUrl(String documentationUrl) {
this.documentationUrl = documentationUrl;
this.changedFields = changedFields.add("documentationUrl");
return this;
}
public Builder headerSubtitle(String headerSubtitle) {
this.headerSubtitle = headerSubtitle;
this.changedFields = changedFields.add("headerSubtitle");
return this;
}
public Builder headerTitle(String headerTitle) {
this.headerTitle = headerTitle;
this.changedFields = changedFields.add("headerTitle");
return this;
}
public Builder isTopLevel(Boolean isTopLevel) {
this.isTopLevel = isTopLevel;
this.changedFields = changedFields.add("isTopLevel");
return this;
}
public Builder keywords(List keywords) {
this.keywords = keywords;
this.changedFields = changedFields.add("keywords");
return this;
}
public Builder keywords(String... keywords) {
return keywords(Arrays.asList(keywords));
}
public Builder keywordsNextLink(String keywordsNextLink) {
this.keywordsNextLink = keywordsNextLink;
this.changedFields = changedFields.add("keywords");
return this;
}
public Builder placeholderText(String placeholderText) {
this.placeholderText = placeholderText;
this.changedFields = changedFields.add("placeholderText");
return this;
}
public Builder valueType(DeviceManangementIntentValueType valueType) {
this.valueType = valueType;
this.changedFields = changedFields.add("valueType");
return this;
}
/**
* “The definitions of each property of the complex setting”
*
* @param propertyDefinitionIds
* value of {@code propertyDefinitionIds} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder propertyDefinitionIds(List propertyDefinitionIds) {
this.propertyDefinitionIds = propertyDefinitionIds;
this.changedFields = changedFields.add("propertyDefinitionIds");
return this;
}
/**
* “The definitions of each property of the complex setting”
*
* @param propertyDefinitionIds
* value of {@code propertyDefinitionIds} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder propertyDefinitionIds(String... propertyDefinitionIds) {
return propertyDefinitionIds(Arrays.asList(propertyDefinitionIds));
}
/**
* “The definitions of each property of the complex setting”
*
* @param propertyDefinitionIdsNextLink
* value of {@code propertyDefinitionIds@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder propertyDefinitionIdsNextLink(String propertyDefinitionIdsNextLink) {
this.propertyDefinitionIdsNextLink = propertyDefinitionIdsNextLink;
this.changedFields = changedFields.add("propertyDefinitionIds");
return this;
}
public DeviceManagementComplexSettingDefinition build() {
DeviceManagementComplexSettingDefinition _x = new DeviceManagementComplexSettingDefinition();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.deviceManagementComplexSettingDefinition";
_x.id = id;
_x.constraints = constraints;
_x.constraintsNextLink = constraintsNextLink;
_x.dependencies = dependencies;
_x.dependenciesNextLink = dependenciesNextLink;
_x.description = description;
_x.displayName = displayName;
_x.documentationUrl = documentationUrl;
_x.headerSubtitle = headerSubtitle;
_x.headerTitle = headerTitle;
_x.isTopLevel = isTopLevel;
_x.keywords = keywords;
_x.keywordsNextLink = keywordsNextLink;
_x.placeholderText = placeholderText;
_x.valueType = valueType;
_x.propertyDefinitionIds = propertyDefinitionIds;
_x.propertyDefinitionIdsNextLink = propertyDefinitionIdsNextLink;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “The definitions of each property of the complex setting”
*
* @return property propertyDefinitionIds
*/
@Property(name="propertyDefinitionIds")
@JsonIgnore
public CollectionPage getPropertyDefinitionIds() {
return new CollectionPage(contextPath, String.class, this.propertyDefinitionIds, Optional.ofNullable(propertyDefinitionIdsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* propertyDefinitionIds} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The definitions of each property of the complex setting”
*
* @param propertyDefinitionIds
* new value of {@code propertyDefinitionIds} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code propertyDefinitionIds} field changed
*/
public DeviceManagementComplexSettingDefinition withPropertyDefinitionIds(List propertyDefinitionIds) {
DeviceManagementComplexSettingDefinition _x = _copy();
_x.changedFields = changedFields.add("propertyDefinitionIds");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagementComplexSettingDefinition");
_x.propertyDefinitionIds = propertyDefinitionIds;
return _x;
}
/**
* “The definitions of each property of the complex setting”
*
* @param options
* specify connect and read timeouts
* @return property propertyDefinitionIds
*/
@Property(name="propertyDefinitionIds")
@JsonIgnore
public CollectionPage getPropertyDefinitionIds(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.propertyDefinitionIds, Optional.ofNullable(propertyDefinitionIdsNextLink), Collections.emptyList(), options);
}
public DeviceManagementComplexSettingDefinition withUnmappedField(String name, String value) {
DeviceManagementComplexSettingDefinition _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public DeviceManagementComplexSettingDefinition patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
DeviceManagementComplexSettingDefinition _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public DeviceManagementComplexSettingDefinition put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
DeviceManagementComplexSettingDefinition _x = _copy();
_x.changedFields = null;
return _x;
}
private DeviceManagementComplexSettingDefinition _copy() {
DeviceManagementComplexSettingDefinition _x = new DeviceManagementComplexSettingDefinition();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.constraints = constraints;
_x.dependencies = dependencies;
_x.description = description;
_x.displayName = displayName;
_x.documentationUrl = documentationUrl;
_x.headerSubtitle = headerSubtitle;
_x.headerTitle = headerTitle;
_x.isTopLevel = isTopLevel;
_x.keywords = keywords;
_x.placeholderText = placeholderText;
_x.valueType = valueType;
_x.propertyDefinitionIds = propertyDefinitionIds;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("DeviceManagementComplexSettingDefinition[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("constraints=");
b.append(this.constraints);
b.append(", ");
b.append("dependencies=");
b.append(this.dependencies);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("documentationUrl=");
b.append(this.documentationUrl);
b.append(", ");
b.append("headerSubtitle=");
b.append(this.headerSubtitle);
b.append(", ");
b.append("headerTitle=");
b.append(this.headerTitle);
b.append(", ");
b.append("isTopLevel=");
b.append(this.isTopLevel);
b.append(", ");
b.append("keywords=");
b.append(this.keywords);
b.append(", ");
b.append("placeholderText=");
b.append(this.placeholderText);
b.append(", ");
b.append("valueType=");
b.append(this.valueType);
b.append(", ");
b.append("propertyDefinitionIds=");
b.append(this.propertyDefinitionIds);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}