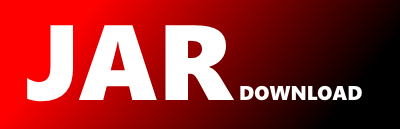
odata.msgraph.client.beta.entity.DeviceManagementIntentDeviceSettingStateSummary Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
/**
* “Entity that represents device setting state summary for an intent”
*/@JsonPropertyOrder({
"@odata.type",
"compliantCount",
"conflictCount",
"errorCount",
"nonCompliantCount",
"notApplicableCount",
"remediatedCount",
"settingName"})
@JsonInclude(Include.NON_NULL)
public class DeviceManagementIntentDeviceSettingStateSummary extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.deviceManagementIntentDeviceSettingStateSummary";
}
@JsonProperty("compliantCount")
protected Integer compliantCount;
@JsonProperty("conflictCount")
protected Integer conflictCount;
@JsonProperty("errorCount")
protected Integer errorCount;
@JsonProperty("nonCompliantCount")
protected Integer nonCompliantCount;
@JsonProperty("notApplicableCount")
protected Integer notApplicableCount;
@JsonProperty("remediatedCount")
protected Integer remediatedCount;
@JsonProperty("settingName")
protected String settingName;
protected DeviceManagementIntentDeviceSettingStateSummary() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderDeviceManagementIntentDeviceSettingStateSummary() {
return new Builder();
}
public static final class Builder {
private String id;
private Integer compliantCount;
private Integer conflictCount;
private Integer errorCount;
private Integer nonCompliantCount;
private Integer notApplicableCount;
private Integer remediatedCount;
private String settingName;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
/**
* “Number of compliant devices”
*
* @param compliantCount
* value of {@code compliantCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder compliantCount(Integer compliantCount) {
this.compliantCount = compliantCount;
this.changedFields = changedFields.add("compliantCount");
return this;
}
/**
* “Number of devices in conflict”
*
* @param conflictCount
* value of {@code conflictCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder conflictCount(Integer conflictCount) {
this.conflictCount = conflictCount;
this.changedFields = changedFields.add("conflictCount");
return this;
}
/**
* “Number of error devices”
*
* @param errorCount
* value of {@code errorCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder errorCount(Integer errorCount) {
this.errorCount = errorCount;
this.changedFields = changedFields.add("errorCount");
return this;
}
/**
* “Number of non compliant devices”
*
* @param nonCompliantCount
* value of {@code nonCompliantCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder nonCompliantCount(Integer nonCompliantCount) {
this.nonCompliantCount = nonCompliantCount;
this.changedFields = changedFields.add("nonCompliantCount");
return this;
}
/**
* “Number of not applicable devices”
*
* @param notApplicableCount
* value of {@code notApplicableCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder notApplicableCount(Integer notApplicableCount) {
this.notApplicableCount = notApplicableCount;
this.changedFields = changedFields.add("notApplicableCount");
return this;
}
/**
* “Number of remediated devices”
*
* @param remediatedCount
* value of {@code remediatedCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder remediatedCount(Integer remediatedCount) {
this.remediatedCount = remediatedCount;
this.changedFields = changedFields.add("remediatedCount");
return this;
}
/**
* “Name of a setting”
*
* @param settingName
* value of {@code settingName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder settingName(String settingName) {
this.settingName = settingName;
this.changedFields = changedFields.add("settingName");
return this;
}
public DeviceManagementIntentDeviceSettingStateSummary build() {
DeviceManagementIntentDeviceSettingStateSummary _x = new DeviceManagementIntentDeviceSettingStateSummary();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.deviceManagementIntentDeviceSettingStateSummary";
_x.id = id;
_x.compliantCount = compliantCount;
_x.conflictCount = conflictCount;
_x.errorCount = errorCount;
_x.nonCompliantCount = nonCompliantCount;
_x.notApplicableCount = notApplicableCount;
_x.remediatedCount = remediatedCount;
_x.settingName = settingName;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Number of compliant devices”
*
* @return property compliantCount
*/
@Property(name="compliantCount")
@JsonIgnore
public Optional getCompliantCount() {
return Optional.ofNullable(compliantCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code compliantCount}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Number of compliant devices”
*
* @param compliantCount
* new value of {@code compliantCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code compliantCount} field changed
*/
public DeviceManagementIntentDeviceSettingStateSummary withCompliantCount(Integer compliantCount) {
DeviceManagementIntentDeviceSettingStateSummary _x = _copy();
_x.changedFields = changedFields.add("compliantCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagementIntentDeviceSettingStateSummary");
_x.compliantCount = compliantCount;
return _x;
}
/**
* “Number of devices in conflict”
*
* @return property conflictCount
*/
@Property(name="conflictCount")
@JsonIgnore
public Optional getConflictCount() {
return Optional.ofNullable(conflictCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code conflictCount}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Number of devices in conflict”
*
* @param conflictCount
* new value of {@code conflictCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code conflictCount} field changed
*/
public DeviceManagementIntentDeviceSettingStateSummary withConflictCount(Integer conflictCount) {
DeviceManagementIntentDeviceSettingStateSummary _x = _copy();
_x.changedFields = changedFields.add("conflictCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagementIntentDeviceSettingStateSummary");
_x.conflictCount = conflictCount;
return _x;
}
/**
* “Number of error devices”
*
* @return property errorCount
*/
@Property(name="errorCount")
@JsonIgnore
public Optional getErrorCount() {
return Optional.ofNullable(errorCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code errorCount} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Number of error devices”
*
* @param errorCount
* new value of {@code errorCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code errorCount} field changed
*/
public DeviceManagementIntentDeviceSettingStateSummary withErrorCount(Integer errorCount) {
DeviceManagementIntentDeviceSettingStateSummary _x = _copy();
_x.changedFields = changedFields.add("errorCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagementIntentDeviceSettingStateSummary");
_x.errorCount = errorCount;
return _x;
}
/**
* “Number of non compliant devices”
*
* @return property nonCompliantCount
*/
@Property(name="nonCompliantCount")
@JsonIgnore
public Optional getNonCompliantCount() {
return Optional.ofNullable(nonCompliantCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code nonCompliantCount
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Number of non compliant devices”
*
* @param nonCompliantCount
* new value of {@code nonCompliantCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code nonCompliantCount} field changed
*/
public DeviceManagementIntentDeviceSettingStateSummary withNonCompliantCount(Integer nonCompliantCount) {
DeviceManagementIntentDeviceSettingStateSummary _x = _copy();
_x.changedFields = changedFields.add("nonCompliantCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagementIntentDeviceSettingStateSummary");
_x.nonCompliantCount = nonCompliantCount;
return _x;
}
/**
* “Number of not applicable devices”
*
* @return property notApplicableCount
*/
@Property(name="notApplicableCount")
@JsonIgnore
public Optional getNotApplicableCount() {
return Optional.ofNullable(notApplicableCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* notApplicableCount} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Number of not applicable devices”
*
* @param notApplicableCount
* new value of {@code notApplicableCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code notApplicableCount} field changed
*/
public DeviceManagementIntentDeviceSettingStateSummary withNotApplicableCount(Integer notApplicableCount) {
DeviceManagementIntentDeviceSettingStateSummary _x = _copy();
_x.changedFields = changedFields.add("notApplicableCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagementIntentDeviceSettingStateSummary");
_x.notApplicableCount = notApplicableCount;
return _x;
}
/**
* “Number of remediated devices”
*
* @return property remediatedCount
*/
@Property(name="remediatedCount")
@JsonIgnore
public Optional getRemediatedCount() {
return Optional.ofNullable(remediatedCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code remediatedCount}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Number of remediated devices”
*
* @param remediatedCount
* new value of {@code remediatedCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code remediatedCount} field changed
*/
public DeviceManagementIntentDeviceSettingStateSummary withRemediatedCount(Integer remediatedCount) {
DeviceManagementIntentDeviceSettingStateSummary _x = _copy();
_x.changedFields = changedFields.add("remediatedCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagementIntentDeviceSettingStateSummary");
_x.remediatedCount = remediatedCount;
return _x;
}
/**
* “Name of a setting”
*
* @return property settingName
*/
@Property(name="settingName")
@JsonIgnore
public Optional getSettingName() {
return Optional.ofNullable(settingName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code settingName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Name of a setting”
*
* @param settingName
* new value of {@code settingName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code settingName} field changed
*/
public DeviceManagementIntentDeviceSettingStateSummary withSettingName(String settingName) {
DeviceManagementIntentDeviceSettingStateSummary _x = _copy();
_x.changedFields = changedFields.add("settingName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagementIntentDeviceSettingStateSummary");
_x.settingName = settingName;
return _x;
}
public DeviceManagementIntentDeviceSettingStateSummary withUnmappedField(String name, String value) {
DeviceManagementIntentDeviceSettingStateSummary _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public DeviceManagementIntentDeviceSettingStateSummary patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
DeviceManagementIntentDeviceSettingStateSummary _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public DeviceManagementIntentDeviceSettingStateSummary put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
DeviceManagementIntentDeviceSettingStateSummary _x = _copy();
_x.changedFields = null;
return _x;
}
private DeviceManagementIntentDeviceSettingStateSummary _copy() {
DeviceManagementIntentDeviceSettingStateSummary _x = new DeviceManagementIntentDeviceSettingStateSummary();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.compliantCount = compliantCount;
_x.conflictCount = conflictCount;
_x.errorCount = errorCount;
_x.nonCompliantCount = nonCompliantCount;
_x.notApplicableCount = notApplicableCount;
_x.remediatedCount = remediatedCount;
_x.settingName = settingName;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("DeviceManagementIntentDeviceSettingStateSummary[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("compliantCount=");
b.append(this.compliantCount);
b.append(", ");
b.append("conflictCount=");
b.append(this.conflictCount);
b.append(", ");
b.append("errorCount=");
b.append(this.errorCount);
b.append(", ");
b.append("nonCompliantCount=");
b.append(this.nonCompliantCount);
b.append(", ");
b.append("notApplicableCount=");
b.append(this.notApplicableCount);
b.append(", ");
b.append("remediatedCount=");
b.append(this.remediatedCount);
b.append(", ");
b.append("settingName=");
b.append(this.settingName);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}