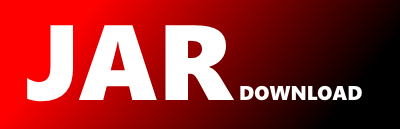
odata.msgraph.client.beta.entity.GroupPolicyConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.CollectionPageNonEntityRequest;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import odata.msgraph.client.beta.entity.collection.request.GroupPolicyConfigurationAssignmentCollectionRequest;
import odata.msgraph.client.beta.entity.collection.request.GroupPolicyDefinitionValueCollectionRequest;
/**
* “The group policy configuration entity contains the configured values for one or
* more group policy definitions.”
*/@JsonPropertyOrder({
"@odata.type",
"createdDateTime",
"description",
"displayName",
"lastModifiedDateTime",
"roleScopeTagIds"})
@JsonInclude(Include.NON_NULL)
public class GroupPolicyConfiguration extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.groupPolicyConfiguration";
}
@JsonProperty("createdDateTime")
protected OffsetDateTime createdDateTime;
@JsonProperty("description")
protected String description;
@JsonProperty("displayName")
protected String displayName;
@JsonProperty("lastModifiedDateTime")
protected OffsetDateTime lastModifiedDateTime;
@JsonProperty("roleScopeTagIds")
protected List roleScopeTagIds;
@JsonProperty("roleScopeTagIds@nextLink")
protected String roleScopeTagIdsNextLink;
protected GroupPolicyConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderGroupPolicyConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
/**
* “The date and time the object was created.”
*
* @param createdDateTime
* value of {@code createdDateTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
/**
* “User provided description for the resource object.”
*
* @param description
* value of {@code description} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
/**
* “User provided name for the resource object.”
*
* @param displayName
* value of {@code displayName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
/**
* “The date and time the entity was last modified.”
*
* @param lastModifiedDateTime
* value of {@code lastModifiedDateTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
/**
* “The list of scope tags for the configuration.”
*
* @param roleScopeTagIds
* value of {@code roleScopeTagIds} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
/**
* “The list of scope tags for the configuration.”
*
* @param roleScopeTagIds
* value of {@code roleScopeTagIds} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
/**
* “The list of scope tags for the configuration.”
*
* @param roleScopeTagIdsNextLink
* value of {@code roleScopeTagIds@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public GroupPolicyConfiguration build() {
GroupPolicyConfiguration _x = new GroupPolicyConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.groupPolicyConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “The date and time the object was created.”
*
* @return property createdDateTime
*/
@Property(name="createdDateTime")
@JsonIgnore
public Optional getCreatedDateTime() {
return Optional.ofNullable(createdDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code createdDateTime}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The date and time the object was created.”
*
* @param createdDateTime
* new value of {@code createdDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code createdDateTime} field changed
*/
public GroupPolicyConfiguration withCreatedDateTime(OffsetDateTime createdDateTime) {
GroupPolicyConfiguration _x = _copy();
_x.changedFields = changedFields.add("createdDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.groupPolicyConfiguration");
_x.createdDateTime = createdDateTime;
return _x;
}
/**
* “User provided description for the resource object.”
*
* @return property description
*/
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
/**
* Returns an immutable copy of {@code this} with just the {@code description}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “User provided description for the resource object.”
*
* @param description
* new value of {@code description} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code description} field changed
*/
public GroupPolicyConfiguration withDescription(String description) {
GroupPolicyConfiguration _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "microsoft.graph.groupPolicyConfiguration");
_x.description = description;
return _x;
}
/**
* “User provided name for the resource object.”
*
* @return property displayName
*/
@Property(name="displayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code displayName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “User provided name for the resource object.”
*
* @param displayName
* new value of {@code displayName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code displayName} field changed
*/
public GroupPolicyConfiguration withDisplayName(String displayName) {
GroupPolicyConfiguration _x = _copy();
_x.changedFields = changedFields.add("displayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.groupPolicyConfiguration");
_x.displayName = displayName;
return _x;
}
/**
* “The date and time the entity was last modified.”
*
* @return property lastModifiedDateTime
*/
@Property(name="lastModifiedDateTime")
@JsonIgnore
public Optional getLastModifiedDateTime() {
return Optional.ofNullable(lastModifiedDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* lastModifiedDateTime} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The date and time the entity was last modified.”
*
* @param lastModifiedDateTime
* new value of {@code lastModifiedDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code lastModifiedDateTime} field changed
*/
public GroupPolicyConfiguration withLastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
GroupPolicyConfiguration _x = _copy();
_x.changedFields = changedFields.add("lastModifiedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.groupPolicyConfiguration");
_x.lastModifiedDateTime = lastModifiedDateTime;
return _x;
}
/**
* “The list of scope tags for the configuration.”
*
* @return property roleScopeTagIds
*/
@Property(name="roleScopeTagIds")
@JsonIgnore
public CollectionPage getRoleScopeTagIds() {
return new CollectionPage(contextPath, String.class, this.roleScopeTagIds, Optional.ofNullable(roleScopeTagIdsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code roleScopeTagIds}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The list of scope tags for the configuration.”
*
* @param roleScopeTagIds
* new value of {@code roleScopeTagIds} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code roleScopeTagIds} field changed
*/
public GroupPolicyConfiguration withRoleScopeTagIds(List roleScopeTagIds) {
GroupPolicyConfiguration _x = _copy();
_x.changedFields = changedFields.add("roleScopeTagIds");
_x.odataType = Util.nvl(odataType, "microsoft.graph.groupPolicyConfiguration");
_x.roleScopeTagIds = roleScopeTagIds;
return _x;
}
/**
* “The list of scope tags for the configuration.”
*
* @param options
* specify connect and read timeouts
* @return property roleScopeTagIds
*/
@Property(name="roleScopeTagIds")
@JsonIgnore
public CollectionPage getRoleScopeTagIds(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.roleScopeTagIds, Optional.ofNullable(roleScopeTagIdsNextLink), Collections.emptyList(), options);
}
public GroupPolicyConfiguration withUnmappedField(String name, String value) {
GroupPolicyConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “The list of group assignments for the configuration.”
*
* @return navigational property assignments
*/
@NavigationProperty(name="assignments")
@JsonIgnore
public GroupPolicyConfigurationAssignmentCollectionRequest getAssignments() {
return new GroupPolicyConfigurationAssignmentCollectionRequest(
contextPath.addSegment("assignments"), RequestHelper.getValue(unmappedFields, "assignments"));
}
/**
* “The list of enabled or disabled group policy definition values for the
* configuration.”
*
* @return navigational property definitionValues
*/
@NavigationProperty(name="definitionValues")
@JsonIgnore
public GroupPolicyDefinitionValueCollectionRequest getDefinitionValues() {
return new GroupPolicyDefinitionValueCollectionRequest(
contextPath.addSegment("definitionValues"), RequestHelper.getValue(unmappedFields, "definitionValues"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public GroupPolicyConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
GroupPolicyConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public GroupPolicyConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
GroupPolicyConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private GroupPolicyConfiguration _copy() {
GroupPolicyConfiguration _x = new GroupPolicyConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
return _x;
}
@Action(name = "assign")
@JsonIgnore
public CollectionPageNonEntityRequest assign(List assignments) {
Map _parameters = ParameterMap
.put("assignments", "Collection(microsoft.graph.groupPolicyConfigurationAssignment)", assignments)
.build();
return CollectionPageNonEntityRequest.forAction(this.contextPath.addActionOrFunctionSegment("microsoft.graph.assign"), GroupPolicyConfigurationAssignment.class, _parameters);
}
@Action(name = "updateDefinitionValues")
@JsonIgnore
public ActionRequestNoReturn updateDefinitionValues(List added, List updated, List deletedIds) {
Map _parameters = ParameterMap
.put("added", "Collection(microsoft.graph.groupPolicyDefinitionValue)", added)
.put("updated", "Collection(microsoft.graph.groupPolicyDefinitionValue)", updated)
.put("deletedIds", "Collection(Edm.String)", Checks.checkIsAscii(deletedIds))
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("microsoft.graph.updateDefinitionValues"), _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("GroupPolicyConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}