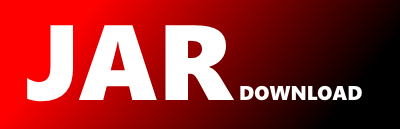
odata.msgraph.client.beta.entity.IntuneBrandingProfile Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import odata.msgraph.client.beta.complex.CompanyPortalBlockedAction;
import odata.msgraph.client.beta.complex.MimeContent;
import odata.msgraph.client.beta.complex.RgbColor;
import odata.msgraph.client.beta.entity.collection.request.IntuneBrandingProfileAssignmentCollectionRequest;
import odata.msgraph.client.beta.enums.EnrollmentAvailabilityOptions;
/**
* “This entity contains data which is used in customizing the tenant level
* appearance of the Company Portal applications as well as the end user web portal
* .”
*/@JsonPropertyOrder({
"@odata.type",
"companyPortalBlockedActions",
"contactITEmailAddress",
"contactITName",
"contactITNotes",
"contactITPhoneNumber",
"createdDateTime",
"customCanSeePrivacyMessage",
"customCantSeePrivacyMessage",
"customPrivacyMessage",
"disableClientTelemetry",
"displayName",
"enrollmentAvailability",
"isDefaultProfile",
"isFactoryResetDisabled",
"isRemoveDeviceDisabled",
"landingPageCustomizedImage",
"lastModifiedDateTime",
"lightBackgroundLogo",
"onlineSupportSiteName",
"onlineSupportSiteUrl",
"privacyUrl",
"profileDescription",
"profileName",
"roleScopeTagIds",
"sendDeviceOwnershipChangePushNotification",
"showAzureADEnterpriseApps",
"showDisplayNameNextToLogo",
"showLogo",
"showOfficeWebApps",
"themeColor",
"themeColorLogo"})
@JsonInclude(Include.NON_NULL)
public class IntuneBrandingProfile extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.intuneBrandingProfile";
}
@JsonProperty("companyPortalBlockedActions")
protected List companyPortalBlockedActions;
@JsonProperty("companyPortalBlockedActions@nextLink")
protected String companyPortalBlockedActionsNextLink;
@JsonProperty("contactITEmailAddress")
protected String contactITEmailAddress;
@JsonProperty("contactITName")
protected String contactITName;
@JsonProperty("contactITNotes")
protected String contactITNotes;
@JsonProperty("contactITPhoneNumber")
protected String contactITPhoneNumber;
@JsonProperty("createdDateTime")
protected OffsetDateTime createdDateTime;
@JsonProperty("customCanSeePrivacyMessage")
protected String customCanSeePrivacyMessage;
@JsonProperty("customCantSeePrivacyMessage")
protected String customCantSeePrivacyMessage;
@JsonProperty("customPrivacyMessage")
protected String customPrivacyMessage;
@JsonProperty("disableClientTelemetry")
protected Boolean disableClientTelemetry;
@JsonProperty("displayName")
protected String displayName;
@JsonProperty("enrollmentAvailability")
protected EnrollmentAvailabilityOptions enrollmentAvailability;
@JsonProperty("isDefaultProfile")
protected Boolean isDefaultProfile;
@JsonProperty("isFactoryResetDisabled")
protected Boolean isFactoryResetDisabled;
@JsonProperty("isRemoveDeviceDisabled")
protected Boolean isRemoveDeviceDisabled;
@JsonProperty("landingPageCustomizedImage")
protected MimeContent landingPageCustomizedImage;
@JsonProperty("lastModifiedDateTime")
protected OffsetDateTime lastModifiedDateTime;
@JsonProperty("lightBackgroundLogo")
protected MimeContent lightBackgroundLogo;
@JsonProperty("onlineSupportSiteName")
protected String onlineSupportSiteName;
@JsonProperty("onlineSupportSiteUrl")
protected String onlineSupportSiteUrl;
@JsonProperty("privacyUrl")
protected String privacyUrl;
@JsonProperty("profileDescription")
protected String profileDescription;
@JsonProperty("profileName")
protected String profileName;
@JsonProperty("roleScopeTagIds")
protected List roleScopeTagIds;
@JsonProperty("roleScopeTagIds@nextLink")
protected String roleScopeTagIdsNextLink;
@JsonProperty("sendDeviceOwnershipChangePushNotification")
protected Boolean sendDeviceOwnershipChangePushNotification;
@JsonProperty("showAzureADEnterpriseApps")
protected Boolean showAzureADEnterpriseApps;
@JsonProperty("showDisplayNameNextToLogo")
protected Boolean showDisplayNameNextToLogo;
@JsonProperty("showLogo")
protected Boolean showLogo;
@JsonProperty("showOfficeWebApps")
protected Boolean showOfficeWebApps;
@JsonProperty("themeColor")
protected RgbColor themeColor;
@JsonProperty("themeColorLogo")
protected MimeContent themeColorLogo;
protected IntuneBrandingProfile() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderIntuneBrandingProfile() {
return new Builder();
}
public static final class Builder {
private String id;
private List companyPortalBlockedActions;
private String companyPortalBlockedActionsNextLink;
private String contactITEmailAddress;
private String contactITName;
private String contactITNotes;
private String contactITPhoneNumber;
private OffsetDateTime createdDateTime;
private String customCanSeePrivacyMessage;
private String customCantSeePrivacyMessage;
private String customPrivacyMessage;
private Boolean disableClientTelemetry;
private String displayName;
private EnrollmentAvailabilityOptions enrollmentAvailability;
private Boolean isDefaultProfile;
private Boolean isFactoryResetDisabled;
private Boolean isRemoveDeviceDisabled;
private MimeContent landingPageCustomizedImage;
private OffsetDateTime lastModifiedDateTime;
private MimeContent lightBackgroundLogo;
private String onlineSupportSiteName;
private String onlineSupportSiteUrl;
private String privacyUrl;
private String profileDescription;
private String profileName;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private Boolean sendDeviceOwnershipChangePushNotification;
private Boolean showAzureADEnterpriseApps;
private Boolean showDisplayNameNextToLogo;
private Boolean showLogo;
private Boolean showOfficeWebApps;
private RgbColor themeColor;
private MimeContent themeColorLogo;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
/**
* “Collection of blocked actions on the company portal as per platform and device
* ownership types.”
*
* @param companyPortalBlockedActions
* value of {@code companyPortalBlockedActions} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder companyPortalBlockedActions(List companyPortalBlockedActions) {
this.companyPortalBlockedActions = companyPortalBlockedActions;
this.changedFields = changedFields.add("companyPortalBlockedActions");
return this;
}
/**
* “Collection of blocked actions on the company portal as per platform and device
* ownership types.”
*
* @param companyPortalBlockedActions
* value of {@code companyPortalBlockedActions} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder companyPortalBlockedActions(CompanyPortalBlockedAction... companyPortalBlockedActions) {
return companyPortalBlockedActions(Arrays.asList(companyPortalBlockedActions));
}
/**
* “Collection of blocked actions on the company portal as per platform and device
* ownership types.”
*
* @param companyPortalBlockedActionsNextLink
* value of {@code companyPortalBlockedActions@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder companyPortalBlockedActionsNextLink(String companyPortalBlockedActionsNextLink) {
this.companyPortalBlockedActionsNextLink = companyPortalBlockedActionsNextLink;
this.changedFields = changedFields.add("companyPortalBlockedActions");
return this;
}
/**
* “E-mail address of the person/organization responsible for IT support”
*
* @param contactITEmailAddress
* value of {@code contactITEmailAddress} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contactITEmailAddress(String contactITEmailAddress) {
this.contactITEmailAddress = contactITEmailAddress;
this.changedFields = changedFields.add("contactITEmailAddress");
return this;
}
/**
* “Name of the person/organization responsible for IT support”
*
* @param contactITName
* value of {@code contactITName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contactITName(String contactITName) {
this.contactITName = contactITName;
this.changedFields = changedFields.add("contactITName");
return this;
}
/**
* “Text comments regarding the person/organization responsible for IT support”
*
* @param contactITNotes
* value of {@code contactITNotes} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contactITNotes(String contactITNotes) {
this.contactITNotes = contactITNotes;
this.changedFields = changedFields.add("contactITNotes");
return this;
}
/**
* “Phone number of the person/organization responsible for IT support”
*
* @param contactITPhoneNumber
* value of {@code contactITPhoneNumber} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contactITPhoneNumber(String contactITPhoneNumber) {
this.contactITPhoneNumber = contactITPhoneNumber;
this.changedFields = changedFields.add("contactITPhoneNumber");
return this;
}
/**
* “Time when the BrandingProfile was created”
*
* @param createdDateTime
* value of {@code createdDateTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
/**
* “Text comments regarding what the admin has access to on the device”
*
* @param customCanSeePrivacyMessage
* value of {@code customCanSeePrivacyMessage} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder customCanSeePrivacyMessage(String customCanSeePrivacyMessage) {
this.customCanSeePrivacyMessage = customCanSeePrivacyMessage;
this.changedFields = changedFields.add("customCanSeePrivacyMessage");
return this;
}
/**
* “Text comments regarding what the admin doesn't have access to on the device”
*
* @param customCantSeePrivacyMessage
* value of {@code customCantSeePrivacyMessage} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder customCantSeePrivacyMessage(String customCantSeePrivacyMessage) {
this.customCantSeePrivacyMessage = customCantSeePrivacyMessage;
this.changedFields = changedFields.add("customCantSeePrivacyMessage");
return this;
}
/**
* “Text comments regarding what the admin doesn't have access to on the device”
*
* @param customPrivacyMessage
* value of {@code customPrivacyMessage} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder customPrivacyMessage(String customPrivacyMessage) {
this.customPrivacyMessage = customPrivacyMessage;
this.changedFields = changedFields.add("customPrivacyMessage");
return this;
}
/**
* “Applies to telemetry sent from all clients to the Intune service. When disabled,
* all proactive troubleshooting and issue warnings within the client are turned
* off, and telemetry settings appear inactive or hidden to the device user.”
*
* @param disableClientTelemetry
* value of {@code disableClientTelemetry} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder disableClientTelemetry(Boolean disableClientTelemetry) {
this.disableClientTelemetry = disableClientTelemetry;
this.changedFields = changedFields.add("disableClientTelemetry");
return this;
}
/**
* “Company/organization name that is displayed to end users”
*
* @param displayName
* value of {@code displayName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
/**
* “Customized device enrollment flow displayed to the end user”
*
* @param enrollmentAvailability
* value of {@code enrollmentAvailability} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder enrollmentAvailability(EnrollmentAvailabilityOptions enrollmentAvailability) {
this.enrollmentAvailability = enrollmentAvailability;
this.changedFields = changedFields.add("enrollmentAvailability");
return this;
}
/**
* “Boolean that represents whether the profile is used as default or not”
*
* @param isDefaultProfile
* value of {@code isDefaultProfile} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder isDefaultProfile(Boolean isDefaultProfile) {
this.isDefaultProfile = isDefaultProfile;
this.changedFields = changedFields.add("isDefaultProfile");
return this;
}
/**
* “Boolean that represents whether the adminsistrator has disabled the 'Factory
* Reset' action on corporate owned devices.”
*
* @param isFactoryResetDisabled
* value of {@code isFactoryResetDisabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder isFactoryResetDisabled(Boolean isFactoryResetDisabled) {
this.isFactoryResetDisabled = isFactoryResetDisabled;
this.changedFields = changedFields.add("isFactoryResetDisabled");
return this;
}
/**
* “Boolean that represents whether the adminsistrator has disabled the 'Remove
* Device' action on corporate owned devices.”
*
* @param isRemoveDeviceDisabled
* value of {@code isRemoveDeviceDisabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder isRemoveDeviceDisabled(Boolean isRemoveDeviceDisabled) {
this.isRemoveDeviceDisabled = isRemoveDeviceDisabled;
this.changedFields = changedFields.add("isRemoveDeviceDisabled");
return this;
}
/**
* “Customized image displayed in Company Portal apps landing page”
*
* @param landingPageCustomizedImage
* value of {@code landingPageCustomizedImage} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder landingPageCustomizedImage(MimeContent landingPageCustomizedImage) {
this.landingPageCustomizedImage = landingPageCustomizedImage;
this.changedFields = changedFields.add("landingPageCustomizedImage");
return this;
}
/**
* “Time when the BrandingProfile was last modified”
*
* @param lastModifiedDateTime
* value of {@code lastModifiedDateTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
/**
* “Logo image displayed in Company Portal apps which have a light background behind
* the logo”
*
* @param lightBackgroundLogo
* value of {@code lightBackgroundLogo} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder lightBackgroundLogo(MimeContent lightBackgroundLogo) {
this.lightBackgroundLogo = lightBackgroundLogo;
this.changedFields = changedFields.add("lightBackgroundLogo");
return this;
}
/**
* “Display name of the company/organization’s IT helpdesk site”
*
* @param onlineSupportSiteName
* value of {@code onlineSupportSiteName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder onlineSupportSiteName(String onlineSupportSiteName) {
this.onlineSupportSiteName = onlineSupportSiteName;
this.changedFields = changedFields.add("onlineSupportSiteName");
return this;
}
/**
* “URL to the company/organization’s IT helpdesk site”
*
* @param onlineSupportSiteUrl
* value of {@code onlineSupportSiteUrl} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder onlineSupportSiteUrl(String onlineSupportSiteUrl) {
this.onlineSupportSiteUrl = onlineSupportSiteUrl;
this.changedFields = changedFields.add("onlineSupportSiteUrl");
return this;
}
/**
* “URL to the company/organization’s privacy policy”
*
* @param privacyUrl
* value of {@code privacyUrl} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder privacyUrl(String privacyUrl) {
this.privacyUrl = privacyUrl;
this.changedFields = changedFields.add("privacyUrl");
return this;
}
/**
* “Description of the profile”
*
* @param profileDescription
* value of {@code profileDescription} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder profileDescription(String profileDescription) {
this.profileDescription = profileDescription;
this.changedFields = changedFields.add("profileDescription");
return this;
}
/**
* “Name of the profile”
*
* @param profileName
* value of {@code profileName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder profileName(String profileName) {
this.profileName = profileName;
this.changedFields = changedFields.add("profileName");
return this;
}
/**
* “List of scope tags assigned to the branding profile”
*
* @param roleScopeTagIds
* value of {@code roleScopeTagIds} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
/**
* “List of scope tags assigned to the branding profile”
*
* @param roleScopeTagIds
* value of {@code roleScopeTagIds} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
/**
* “List of scope tags assigned to the branding profile”
*
* @param roleScopeTagIdsNextLink
* value of {@code roleScopeTagIds@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
/**
* “Boolean that indicates if a push notification is sent to users when their device
* ownership type changes from personal to corporate”
*
* @param sendDeviceOwnershipChangePushNotification
* value of {@code sendDeviceOwnershipChangePushNotification} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder sendDeviceOwnershipChangePushNotification(Boolean sendDeviceOwnershipChangePushNotification) {
this.sendDeviceOwnershipChangePushNotification = sendDeviceOwnershipChangePushNotification;
this.changedFields = changedFields.add("sendDeviceOwnershipChangePushNotification");
return this;
}
/**
* “Boolean that indicates if AzureAD Enterprise Apps will be shown in Company
* Portal”
*
* @param showAzureADEnterpriseApps
* value of {@code showAzureADEnterpriseApps} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder showAzureADEnterpriseApps(Boolean showAzureADEnterpriseApps) {
this.showAzureADEnterpriseApps = showAzureADEnterpriseApps;
this.changedFields = changedFields.add("showAzureADEnterpriseApps");
return this;
}
/**
* “Boolean that represents whether the administrator-supplied display name will be
* shown next to the logo image or not”
*
* @param showDisplayNameNextToLogo
* value of {@code showDisplayNameNextToLogo} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder showDisplayNameNextToLogo(Boolean showDisplayNameNextToLogo) {
this.showDisplayNameNextToLogo = showDisplayNameNextToLogo;
this.changedFields = changedFields.add("showDisplayNameNextToLogo");
return this;
}
/**
* “Boolean that represents whether the administrator-supplied logo images are shown
* or not”
*
* @param showLogo
* value of {@code showLogo} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder showLogo(Boolean showLogo) {
this.showLogo = showLogo;
this.changedFields = changedFields.add("showLogo");
return this;
}
/**
* “Boolean that indicates if Office WebApps will be shown in Company Portal”
*
* @param showOfficeWebApps
* value of {@code showOfficeWebApps} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder showOfficeWebApps(Boolean showOfficeWebApps) {
this.showOfficeWebApps = showOfficeWebApps;
this.changedFields = changedFields.add("showOfficeWebApps");
return this;
}
/**
* “Primary theme color used in the Company Portal applications and web portal”
*
* @param themeColor
* value of {@code themeColor} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder themeColor(RgbColor themeColor) {
this.themeColor = themeColor;
this.changedFields = changedFields.add("themeColor");
return this;
}
/**
* “Logo image displayed in Company Portal apps which have a theme color background
* behind the logo”
*
* @param themeColorLogo
* value of {@code themeColorLogo} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder themeColorLogo(MimeContent themeColorLogo) {
this.themeColorLogo = themeColorLogo;
this.changedFields = changedFields.add("themeColorLogo");
return this;
}
public IntuneBrandingProfile build() {
IntuneBrandingProfile _x = new IntuneBrandingProfile();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.intuneBrandingProfile";
_x.id = id;
_x.companyPortalBlockedActions = companyPortalBlockedActions;
_x.companyPortalBlockedActionsNextLink = companyPortalBlockedActionsNextLink;
_x.contactITEmailAddress = contactITEmailAddress;
_x.contactITName = contactITName;
_x.contactITNotes = contactITNotes;
_x.contactITPhoneNumber = contactITPhoneNumber;
_x.createdDateTime = createdDateTime;
_x.customCanSeePrivacyMessage = customCanSeePrivacyMessage;
_x.customCantSeePrivacyMessage = customCantSeePrivacyMessage;
_x.customPrivacyMessage = customPrivacyMessage;
_x.disableClientTelemetry = disableClientTelemetry;
_x.displayName = displayName;
_x.enrollmentAvailability = enrollmentAvailability;
_x.isDefaultProfile = isDefaultProfile;
_x.isFactoryResetDisabled = isFactoryResetDisabled;
_x.isRemoveDeviceDisabled = isRemoveDeviceDisabled;
_x.landingPageCustomizedImage = landingPageCustomizedImage;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.lightBackgroundLogo = lightBackgroundLogo;
_x.onlineSupportSiteName = onlineSupportSiteName;
_x.onlineSupportSiteUrl = onlineSupportSiteUrl;
_x.privacyUrl = privacyUrl;
_x.profileDescription = profileDescription;
_x.profileName = profileName;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
_x.sendDeviceOwnershipChangePushNotification = sendDeviceOwnershipChangePushNotification;
_x.showAzureADEnterpriseApps = showAzureADEnterpriseApps;
_x.showDisplayNameNextToLogo = showDisplayNameNextToLogo;
_x.showLogo = showLogo;
_x.showOfficeWebApps = showOfficeWebApps;
_x.themeColor = themeColor;
_x.themeColorLogo = themeColorLogo;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Collection of blocked actions on the company portal as per platform and device
* ownership types.”
*
* @return property companyPortalBlockedActions
*/
@Property(name="companyPortalBlockedActions")
@JsonIgnore
public CollectionPage getCompanyPortalBlockedActions() {
return new CollectionPage(contextPath, CompanyPortalBlockedAction.class, this.companyPortalBlockedActions, Optional.ofNullable(companyPortalBlockedActionsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* companyPortalBlockedActions} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Collection of blocked actions on the company portal as per platform and device
* ownership types.”
*
* @param companyPortalBlockedActions
* new value of {@code companyPortalBlockedActions} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code companyPortalBlockedActions} field changed
*/
public IntuneBrandingProfile withCompanyPortalBlockedActions(List companyPortalBlockedActions) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("companyPortalBlockedActions");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.companyPortalBlockedActions = companyPortalBlockedActions;
return _x;
}
/**
* “Collection of blocked actions on the company portal as per platform and device
* ownership types.”
*
* @param options
* specify connect and read timeouts
* @return property companyPortalBlockedActions
*/
@Property(name="companyPortalBlockedActions")
@JsonIgnore
public CollectionPage getCompanyPortalBlockedActions(HttpRequestOptions options) {
return new CollectionPage(contextPath, CompanyPortalBlockedAction.class, this.companyPortalBlockedActions, Optional.ofNullable(companyPortalBlockedActionsNextLink), Collections.emptyList(), options);
}
/**
* “E-mail address of the person/organization responsible for IT support”
*
* @return property contactITEmailAddress
*/
@Property(name="contactITEmailAddress")
@JsonIgnore
public Optional getContactITEmailAddress() {
return Optional.ofNullable(contactITEmailAddress);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contactITEmailAddress} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “E-mail address of the person/organization responsible for IT support”
*
* @param contactITEmailAddress
* new value of {@code contactITEmailAddress} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contactITEmailAddress} field changed
*/
public IntuneBrandingProfile withContactITEmailAddress(String contactITEmailAddress) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("contactITEmailAddress");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.contactITEmailAddress = contactITEmailAddress;
return _x;
}
/**
* “Name of the person/organization responsible for IT support”
*
* @return property contactITName
*/
@Property(name="contactITName")
@JsonIgnore
public Optional getContactITName() {
return Optional.ofNullable(contactITName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code contactITName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Name of the person/organization responsible for IT support”
*
* @param contactITName
* new value of {@code contactITName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contactITName} field changed
*/
public IntuneBrandingProfile withContactITName(String contactITName) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("contactITName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.contactITName = contactITName;
return _x;
}
/**
* “Text comments regarding the person/organization responsible for IT support”
*
* @return property contactITNotes
*/
@Property(name="contactITNotes")
@JsonIgnore
public Optional getContactITNotes() {
return Optional.ofNullable(contactITNotes);
}
/**
* Returns an immutable copy of {@code this} with just the {@code contactITNotes}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Text comments regarding the person/organization responsible for IT support”
*
* @param contactITNotes
* new value of {@code contactITNotes} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contactITNotes} field changed
*/
public IntuneBrandingProfile withContactITNotes(String contactITNotes) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("contactITNotes");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.contactITNotes = contactITNotes;
return _x;
}
/**
* “Phone number of the person/organization responsible for IT support”
*
* @return property contactITPhoneNumber
*/
@Property(name="contactITPhoneNumber")
@JsonIgnore
public Optional getContactITPhoneNumber() {
return Optional.ofNullable(contactITPhoneNumber);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contactITPhoneNumber} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Phone number of the person/organization responsible for IT support”
*
* @param contactITPhoneNumber
* new value of {@code contactITPhoneNumber} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contactITPhoneNumber} field changed
*/
public IntuneBrandingProfile withContactITPhoneNumber(String contactITPhoneNumber) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("contactITPhoneNumber");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.contactITPhoneNumber = contactITPhoneNumber;
return _x;
}
/**
* “Time when the BrandingProfile was created”
*
* @return property createdDateTime
*/
@Property(name="createdDateTime")
@JsonIgnore
public Optional getCreatedDateTime() {
return Optional.ofNullable(createdDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code createdDateTime}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Time when the BrandingProfile was created”
*
* @param createdDateTime
* new value of {@code createdDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code createdDateTime} field changed
*/
public IntuneBrandingProfile withCreatedDateTime(OffsetDateTime createdDateTime) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("createdDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.createdDateTime = createdDateTime;
return _x;
}
/**
* “Text comments regarding what the admin has access to on the device”
*
* @return property customCanSeePrivacyMessage
*/
@Property(name="customCanSeePrivacyMessage")
@JsonIgnore
public Optional getCustomCanSeePrivacyMessage() {
return Optional.ofNullable(customCanSeePrivacyMessage);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* customCanSeePrivacyMessage} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Text comments regarding what the admin has access to on the device”
*
* @param customCanSeePrivacyMessage
* new value of {@code customCanSeePrivacyMessage} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code customCanSeePrivacyMessage} field changed
*/
public IntuneBrandingProfile withCustomCanSeePrivacyMessage(String customCanSeePrivacyMessage) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("customCanSeePrivacyMessage");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.customCanSeePrivacyMessage = customCanSeePrivacyMessage;
return _x;
}
/**
* “Text comments regarding what the admin doesn't have access to on the device”
*
* @return property customCantSeePrivacyMessage
*/
@Property(name="customCantSeePrivacyMessage")
@JsonIgnore
public Optional getCustomCantSeePrivacyMessage() {
return Optional.ofNullable(customCantSeePrivacyMessage);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* customCantSeePrivacyMessage} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Text comments regarding what the admin doesn't have access to on the device”
*
* @param customCantSeePrivacyMessage
* new value of {@code customCantSeePrivacyMessage} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code customCantSeePrivacyMessage} field changed
*/
public IntuneBrandingProfile withCustomCantSeePrivacyMessage(String customCantSeePrivacyMessage) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("customCantSeePrivacyMessage");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.customCantSeePrivacyMessage = customCantSeePrivacyMessage;
return _x;
}
/**
* “Text comments regarding what the admin doesn't have access to on the device”
*
* @return property customPrivacyMessage
*/
@Property(name="customPrivacyMessage")
@JsonIgnore
public Optional getCustomPrivacyMessage() {
return Optional.ofNullable(customPrivacyMessage);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* customPrivacyMessage} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Text comments regarding what the admin doesn't have access to on the device”
*
* @param customPrivacyMessage
* new value of {@code customPrivacyMessage} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code customPrivacyMessage} field changed
*/
public IntuneBrandingProfile withCustomPrivacyMessage(String customPrivacyMessage) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("customPrivacyMessage");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.customPrivacyMessage = customPrivacyMessage;
return _x;
}
/**
* “Applies to telemetry sent from all clients to the Intune service. When disabled,
* all proactive troubleshooting and issue warnings within the client are turned
* off, and telemetry settings appear inactive or hidden to the device user.”
*
* @return property disableClientTelemetry
*/
@Property(name="disableClientTelemetry")
@JsonIgnore
public Optional getDisableClientTelemetry() {
return Optional.ofNullable(disableClientTelemetry);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* disableClientTelemetry} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Applies to telemetry sent from all clients to the Intune service. When disabled,
* all proactive troubleshooting and issue warnings within the client are turned
* off, and telemetry settings appear inactive or hidden to the device user.”
*
* @param disableClientTelemetry
* new value of {@code disableClientTelemetry} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code disableClientTelemetry} field changed
*/
public IntuneBrandingProfile withDisableClientTelemetry(Boolean disableClientTelemetry) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("disableClientTelemetry");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.disableClientTelemetry = disableClientTelemetry;
return _x;
}
/**
* “Company/organization name that is displayed to end users”
*
* @return property displayName
*/
@Property(name="displayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code displayName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Company/organization name that is displayed to end users”
*
* @param displayName
* new value of {@code displayName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code displayName} field changed
*/
public IntuneBrandingProfile withDisplayName(String displayName) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("displayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.displayName = displayName;
return _x;
}
/**
* “Customized device enrollment flow displayed to the end user”
*
* @return property enrollmentAvailability
*/
@Property(name="enrollmentAvailability")
@JsonIgnore
public Optional getEnrollmentAvailability() {
return Optional.ofNullable(enrollmentAvailability);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* enrollmentAvailability} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Customized device enrollment flow displayed to the end user”
*
* @param enrollmentAvailability
* new value of {@code enrollmentAvailability} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code enrollmentAvailability} field changed
*/
public IntuneBrandingProfile withEnrollmentAvailability(EnrollmentAvailabilityOptions enrollmentAvailability) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("enrollmentAvailability");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.enrollmentAvailability = enrollmentAvailability;
return _x;
}
/**
* “Boolean that represents whether the profile is used as default or not”
*
* @return property isDefaultProfile
*/
@Property(name="isDefaultProfile")
@JsonIgnore
public Optional getIsDefaultProfile() {
return Optional.ofNullable(isDefaultProfile);
}
/**
* Returns an immutable copy of {@code this} with just the {@code isDefaultProfile}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Boolean that represents whether the profile is used as default or not”
*
* @param isDefaultProfile
* new value of {@code isDefaultProfile} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code isDefaultProfile} field changed
*/
public IntuneBrandingProfile withIsDefaultProfile(Boolean isDefaultProfile) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("isDefaultProfile");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.isDefaultProfile = isDefaultProfile;
return _x;
}
/**
* “Boolean that represents whether the adminsistrator has disabled the 'Factory
* Reset' action on corporate owned devices.”
*
* @return property isFactoryResetDisabled
*/
@Property(name="isFactoryResetDisabled")
@JsonIgnore
public Optional getIsFactoryResetDisabled() {
return Optional.ofNullable(isFactoryResetDisabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* isFactoryResetDisabled} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Boolean that represents whether the adminsistrator has disabled the 'Factory
* Reset' action on corporate owned devices.”
*
* @param isFactoryResetDisabled
* new value of {@code isFactoryResetDisabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code isFactoryResetDisabled} field changed
*/
public IntuneBrandingProfile withIsFactoryResetDisabled(Boolean isFactoryResetDisabled) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("isFactoryResetDisabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.isFactoryResetDisabled = isFactoryResetDisabled;
return _x;
}
/**
* “Boolean that represents whether the adminsistrator has disabled the 'Remove
* Device' action on corporate owned devices.”
*
* @return property isRemoveDeviceDisabled
*/
@Property(name="isRemoveDeviceDisabled")
@JsonIgnore
public Optional getIsRemoveDeviceDisabled() {
return Optional.ofNullable(isRemoveDeviceDisabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* isRemoveDeviceDisabled} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Boolean that represents whether the adminsistrator has disabled the 'Remove
* Device' action on corporate owned devices.”
*
* @param isRemoveDeviceDisabled
* new value of {@code isRemoveDeviceDisabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code isRemoveDeviceDisabled} field changed
*/
public IntuneBrandingProfile withIsRemoveDeviceDisabled(Boolean isRemoveDeviceDisabled) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("isRemoveDeviceDisabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.isRemoveDeviceDisabled = isRemoveDeviceDisabled;
return _x;
}
/**
* “Customized image displayed in Company Portal apps landing page”
*
* @return property landingPageCustomizedImage
*/
@Property(name="landingPageCustomizedImage")
@JsonIgnore
public Optional getLandingPageCustomizedImage() {
return Optional.ofNullable(landingPageCustomizedImage);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* landingPageCustomizedImage} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Customized image displayed in Company Portal apps landing page”
*
* @param landingPageCustomizedImage
* new value of {@code landingPageCustomizedImage} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code landingPageCustomizedImage} field changed
*/
public IntuneBrandingProfile withLandingPageCustomizedImage(MimeContent landingPageCustomizedImage) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("landingPageCustomizedImage");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.landingPageCustomizedImage = landingPageCustomizedImage;
return _x;
}
/**
* “Time when the BrandingProfile was last modified”
*
* @return property lastModifiedDateTime
*/
@Property(name="lastModifiedDateTime")
@JsonIgnore
public Optional getLastModifiedDateTime() {
return Optional.ofNullable(lastModifiedDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* lastModifiedDateTime} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Time when the BrandingProfile was last modified”
*
* @param lastModifiedDateTime
* new value of {@code lastModifiedDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code lastModifiedDateTime} field changed
*/
public IntuneBrandingProfile withLastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("lastModifiedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.lastModifiedDateTime = lastModifiedDateTime;
return _x;
}
/**
* “Logo image displayed in Company Portal apps which have a light background behind
* the logo”
*
* @return property lightBackgroundLogo
*/
@Property(name="lightBackgroundLogo")
@JsonIgnore
public Optional getLightBackgroundLogo() {
return Optional.ofNullable(lightBackgroundLogo);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* lightBackgroundLogo} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Logo image displayed in Company Portal apps which have a light background behind
* the logo”
*
* @param lightBackgroundLogo
* new value of {@code lightBackgroundLogo} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code lightBackgroundLogo} field changed
*/
public IntuneBrandingProfile withLightBackgroundLogo(MimeContent lightBackgroundLogo) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("lightBackgroundLogo");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.lightBackgroundLogo = lightBackgroundLogo;
return _x;
}
/**
* “Display name of the company/organization’s IT helpdesk site”
*
* @return property onlineSupportSiteName
*/
@Property(name="onlineSupportSiteName")
@JsonIgnore
public Optional getOnlineSupportSiteName() {
return Optional.ofNullable(onlineSupportSiteName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* onlineSupportSiteName} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Display name of the company/organization’s IT helpdesk site”
*
* @param onlineSupportSiteName
* new value of {@code onlineSupportSiteName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code onlineSupportSiteName} field changed
*/
public IntuneBrandingProfile withOnlineSupportSiteName(String onlineSupportSiteName) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("onlineSupportSiteName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.onlineSupportSiteName = onlineSupportSiteName;
return _x;
}
/**
* “URL to the company/organization’s IT helpdesk site”
*
* @return property onlineSupportSiteUrl
*/
@Property(name="onlineSupportSiteUrl")
@JsonIgnore
public Optional getOnlineSupportSiteUrl() {
return Optional.ofNullable(onlineSupportSiteUrl);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* onlineSupportSiteUrl} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “URL to the company/organization’s IT helpdesk site”
*
* @param onlineSupportSiteUrl
* new value of {@code onlineSupportSiteUrl} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code onlineSupportSiteUrl} field changed
*/
public IntuneBrandingProfile withOnlineSupportSiteUrl(String onlineSupportSiteUrl) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("onlineSupportSiteUrl");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.onlineSupportSiteUrl = onlineSupportSiteUrl;
return _x;
}
/**
* “URL to the company/organization’s privacy policy”
*
* @return property privacyUrl
*/
@Property(name="privacyUrl")
@JsonIgnore
public Optional getPrivacyUrl() {
return Optional.ofNullable(privacyUrl);
}
/**
* Returns an immutable copy of {@code this} with just the {@code privacyUrl} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “URL to the company/organization’s privacy policy”
*
* @param privacyUrl
* new value of {@code privacyUrl} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code privacyUrl} field changed
*/
public IntuneBrandingProfile withPrivacyUrl(String privacyUrl) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("privacyUrl");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.privacyUrl = privacyUrl;
return _x;
}
/**
* “Description of the profile”
*
* @return property profileDescription
*/
@Property(name="profileDescription")
@JsonIgnore
public Optional getProfileDescription() {
return Optional.ofNullable(profileDescription);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* profileDescription} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Description of the profile”
*
* @param profileDescription
* new value of {@code profileDescription} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code profileDescription} field changed
*/
public IntuneBrandingProfile withProfileDescription(String profileDescription) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("profileDescription");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.profileDescription = profileDescription;
return _x;
}
/**
* “Name of the profile”
*
* @return property profileName
*/
@Property(name="profileName")
@JsonIgnore
public Optional getProfileName() {
return Optional.ofNullable(profileName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code profileName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Name of the profile”
*
* @param profileName
* new value of {@code profileName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code profileName} field changed
*/
public IntuneBrandingProfile withProfileName(String profileName) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("profileName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.profileName = profileName;
return _x;
}
/**
* “List of scope tags assigned to the branding profile”
*
* @return property roleScopeTagIds
*/
@Property(name="roleScopeTagIds")
@JsonIgnore
public CollectionPage getRoleScopeTagIds() {
return new CollectionPage(contextPath, String.class, this.roleScopeTagIds, Optional.ofNullable(roleScopeTagIdsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code roleScopeTagIds}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “List of scope tags assigned to the branding profile”
*
* @param roleScopeTagIds
* new value of {@code roleScopeTagIds} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code roleScopeTagIds} field changed
*/
public IntuneBrandingProfile withRoleScopeTagIds(List roleScopeTagIds) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("roleScopeTagIds");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.roleScopeTagIds = roleScopeTagIds;
return _x;
}
/**
* “List of scope tags assigned to the branding profile”
*
* @param options
* specify connect and read timeouts
* @return property roleScopeTagIds
*/
@Property(name="roleScopeTagIds")
@JsonIgnore
public CollectionPage getRoleScopeTagIds(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.roleScopeTagIds, Optional.ofNullable(roleScopeTagIdsNextLink), Collections.emptyList(), options);
}
/**
* “Boolean that indicates if a push notification is sent to users when their device
* ownership type changes from personal to corporate”
*
* @return property sendDeviceOwnershipChangePushNotification
*/
@Property(name="sendDeviceOwnershipChangePushNotification")
@JsonIgnore
public Optional getSendDeviceOwnershipChangePushNotification() {
return Optional.ofNullable(sendDeviceOwnershipChangePushNotification);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* sendDeviceOwnershipChangePushNotification} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Boolean that indicates if a push notification is sent to users when their device
* ownership type changes from personal to corporate”
*
* @param sendDeviceOwnershipChangePushNotification
* new value of {@code sendDeviceOwnershipChangePushNotification} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code sendDeviceOwnershipChangePushNotification} field changed
*/
public IntuneBrandingProfile withSendDeviceOwnershipChangePushNotification(Boolean sendDeviceOwnershipChangePushNotification) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("sendDeviceOwnershipChangePushNotification");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.sendDeviceOwnershipChangePushNotification = sendDeviceOwnershipChangePushNotification;
return _x;
}
/**
* “Boolean that indicates if AzureAD Enterprise Apps will be shown in Company
* Portal”
*
* @return property showAzureADEnterpriseApps
*/
@Property(name="showAzureADEnterpriseApps")
@JsonIgnore
public Optional getShowAzureADEnterpriseApps() {
return Optional.ofNullable(showAzureADEnterpriseApps);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* showAzureADEnterpriseApps} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Boolean that indicates if AzureAD Enterprise Apps will be shown in Company
* Portal”
*
* @param showAzureADEnterpriseApps
* new value of {@code showAzureADEnterpriseApps} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code showAzureADEnterpriseApps} field changed
*/
public IntuneBrandingProfile withShowAzureADEnterpriseApps(Boolean showAzureADEnterpriseApps) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("showAzureADEnterpriseApps");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.showAzureADEnterpriseApps = showAzureADEnterpriseApps;
return _x;
}
/**
* “Boolean that represents whether the administrator-supplied display name will be
* shown next to the logo image or not”
*
* @return property showDisplayNameNextToLogo
*/
@Property(name="showDisplayNameNextToLogo")
@JsonIgnore
public Optional getShowDisplayNameNextToLogo() {
return Optional.ofNullable(showDisplayNameNextToLogo);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* showDisplayNameNextToLogo} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Boolean that represents whether the administrator-supplied display name will be
* shown next to the logo image or not”
*
* @param showDisplayNameNextToLogo
* new value of {@code showDisplayNameNextToLogo} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code showDisplayNameNextToLogo} field changed
*/
public IntuneBrandingProfile withShowDisplayNameNextToLogo(Boolean showDisplayNameNextToLogo) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("showDisplayNameNextToLogo");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.showDisplayNameNextToLogo = showDisplayNameNextToLogo;
return _x;
}
/**
* “Boolean that represents whether the administrator-supplied logo images are shown
* or not”
*
* @return property showLogo
*/
@Property(name="showLogo")
@JsonIgnore
public Optional getShowLogo() {
return Optional.ofNullable(showLogo);
}
/**
* Returns an immutable copy of {@code this} with just the {@code showLogo} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Boolean that represents whether the administrator-supplied logo images are shown
* or not”
*
* @param showLogo
* new value of {@code showLogo} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code showLogo} field changed
*/
public IntuneBrandingProfile withShowLogo(Boolean showLogo) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("showLogo");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.showLogo = showLogo;
return _x;
}
/**
* “Boolean that indicates if Office WebApps will be shown in Company Portal”
*
* @return property showOfficeWebApps
*/
@Property(name="showOfficeWebApps")
@JsonIgnore
public Optional getShowOfficeWebApps() {
return Optional.ofNullable(showOfficeWebApps);
}
/**
* Returns an immutable copy of {@code this} with just the {@code showOfficeWebApps
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Boolean that indicates if Office WebApps will be shown in Company Portal”
*
* @param showOfficeWebApps
* new value of {@code showOfficeWebApps} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code showOfficeWebApps} field changed
*/
public IntuneBrandingProfile withShowOfficeWebApps(Boolean showOfficeWebApps) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("showOfficeWebApps");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.showOfficeWebApps = showOfficeWebApps;
return _x;
}
/**
* “Primary theme color used in the Company Portal applications and web portal”
*
* @return property themeColor
*/
@Property(name="themeColor")
@JsonIgnore
public Optional getThemeColor() {
return Optional.ofNullable(themeColor);
}
/**
* Returns an immutable copy of {@code this} with just the {@code themeColor} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Primary theme color used in the Company Portal applications and web portal”
*
* @param themeColor
* new value of {@code themeColor} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code themeColor} field changed
*/
public IntuneBrandingProfile withThemeColor(RgbColor themeColor) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("themeColor");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.themeColor = themeColor;
return _x;
}
/**
* “Logo image displayed in Company Portal apps which have a theme color background
* behind the logo”
*
* @return property themeColorLogo
*/
@Property(name="themeColorLogo")
@JsonIgnore
public Optional getThemeColorLogo() {
return Optional.ofNullable(themeColorLogo);
}
/**
* Returns an immutable copy of {@code this} with just the {@code themeColorLogo}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Logo image displayed in Company Portal apps which have a theme color background
* behind the logo”
*
* @param themeColorLogo
* new value of {@code themeColorLogo} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code themeColorLogo} field changed
*/
public IntuneBrandingProfile withThemeColorLogo(MimeContent themeColorLogo) {
IntuneBrandingProfile _x = _copy();
_x.changedFields = changedFields.add("themeColorLogo");
_x.odataType = Util.nvl(odataType, "microsoft.graph.intuneBrandingProfile");
_x.themeColorLogo = themeColorLogo;
return _x;
}
public IntuneBrandingProfile withUnmappedField(String name, String value) {
IntuneBrandingProfile _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “The list of group assignments for the branding profile”
*
* @return navigational property assignments
*/
@NavigationProperty(name="assignments")
@JsonIgnore
public IntuneBrandingProfileAssignmentCollectionRequest getAssignments() {
return new IntuneBrandingProfileAssignmentCollectionRequest(
contextPath.addSegment("assignments"), RequestHelper.getValue(unmappedFields, "assignments"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public IntuneBrandingProfile patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
IntuneBrandingProfile _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public IntuneBrandingProfile put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
IntuneBrandingProfile _x = _copy();
_x.changedFields = null;
return _x;
}
private IntuneBrandingProfile _copy() {
IntuneBrandingProfile _x = new IntuneBrandingProfile();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.companyPortalBlockedActions = companyPortalBlockedActions;
_x.contactITEmailAddress = contactITEmailAddress;
_x.contactITName = contactITName;
_x.contactITNotes = contactITNotes;
_x.contactITPhoneNumber = contactITPhoneNumber;
_x.createdDateTime = createdDateTime;
_x.customCanSeePrivacyMessage = customCanSeePrivacyMessage;
_x.customCantSeePrivacyMessage = customCantSeePrivacyMessage;
_x.customPrivacyMessage = customPrivacyMessage;
_x.disableClientTelemetry = disableClientTelemetry;
_x.displayName = displayName;
_x.enrollmentAvailability = enrollmentAvailability;
_x.isDefaultProfile = isDefaultProfile;
_x.isFactoryResetDisabled = isFactoryResetDisabled;
_x.isRemoveDeviceDisabled = isRemoveDeviceDisabled;
_x.landingPageCustomizedImage = landingPageCustomizedImage;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.lightBackgroundLogo = lightBackgroundLogo;
_x.onlineSupportSiteName = onlineSupportSiteName;
_x.onlineSupportSiteUrl = onlineSupportSiteUrl;
_x.privacyUrl = privacyUrl;
_x.profileDescription = profileDescription;
_x.profileName = profileName;
_x.roleScopeTagIds = roleScopeTagIds;
_x.sendDeviceOwnershipChangePushNotification = sendDeviceOwnershipChangePushNotification;
_x.showAzureADEnterpriseApps = showAzureADEnterpriseApps;
_x.showDisplayNameNextToLogo = showDisplayNameNextToLogo;
_x.showLogo = showLogo;
_x.showOfficeWebApps = showOfficeWebApps;
_x.themeColor = themeColor;
_x.themeColorLogo = themeColorLogo;
return _x;
}
@Action(name = "assign")
@JsonIgnore
public ActionRequestNoReturn assign(List assignments) {
Map _parameters = ParameterMap
.put("assignments", "Collection(microsoft.graph.intuneBrandingProfileAssignment)", assignments)
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("microsoft.graph.assign"), _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("IntuneBrandingProfile[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("companyPortalBlockedActions=");
b.append(this.companyPortalBlockedActions);
b.append(", ");
b.append("contactITEmailAddress=");
b.append(this.contactITEmailAddress);
b.append(", ");
b.append("contactITName=");
b.append(this.contactITName);
b.append(", ");
b.append("contactITNotes=");
b.append(this.contactITNotes);
b.append(", ");
b.append("contactITPhoneNumber=");
b.append(this.contactITPhoneNumber);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("customCanSeePrivacyMessage=");
b.append(this.customCanSeePrivacyMessage);
b.append(", ");
b.append("customCantSeePrivacyMessage=");
b.append(this.customCantSeePrivacyMessage);
b.append(", ");
b.append("customPrivacyMessage=");
b.append(this.customPrivacyMessage);
b.append(", ");
b.append("disableClientTelemetry=");
b.append(this.disableClientTelemetry);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("enrollmentAvailability=");
b.append(this.enrollmentAvailability);
b.append(", ");
b.append("isDefaultProfile=");
b.append(this.isDefaultProfile);
b.append(", ");
b.append("isFactoryResetDisabled=");
b.append(this.isFactoryResetDisabled);
b.append(", ");
b.append("isRemoveDeviceDisabled=");
b.append(this.isRemoveDeviceDisabled);
b.append(", ");
b.append("landingPageCustomizedImage=");
b.append(this.landingPageCustomizedImage);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("lightBackgroundLogo=");
b.append(this.lightBackgroundLogo);
b.append(", ");
b.append("onlineSupportSiteName=");
b.append(this.onlineSupportSiteName);
b.append(", ");
b.append("onlineSupportSiteUrl=");
b.append(this.onlineSupportSiteUrl);
b.append(", ");
b.append("privacyUrl=");
b.append(this.privacyUrl);
b.append(", ");
b.append("profileDescription=");
b.append(this.profileDescription);
b.append(", ");
b.append("profileName=");
b.append(this.profileName);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("sendDeviceOwnershipChangePushNotification=");
b.append(this.sendDeviceOwnershipChangePushNotification);
b.append(", ");
b.append("showAzureADEnterpriseApps=");
b.append(this.showAzureADEnterpriseApps);
b.append(", ");
b.append("showDisplayNameNextToLogo=");
b.append(this.showDisplayNameNextToLogo);
b.append(", ");
b.append("showLogo=");
b.append(this.showLogo);
b.append(", ");
b.append("showOfficeWebApps=");
b.append(this.showOfficeWebApps);
b.append(", ");
b.append("themeColor=");
b.append(this.themeColor);
b.append(", ");
b.append("themeColorLogo=");
b.append(this.themeColorLogo);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}