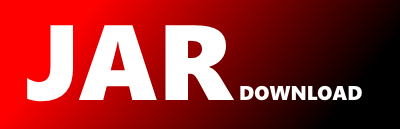
odata.msgraph.client.beta.entity.IosEasEmailProfileConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleDeviceMode;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsEdition;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsVersion;
import odata.msgraph.client.beta.entity.request.DeviceManagementDerivedCredentialSettingsRequest;
import odata.msgraph.client.beta.entity.request.IosCertificateProfileBaseRequest;
import odata.msgraph.client.beta.entity.request.IosCertificateProfileRequest;
import odata.msgraph.client.beta.enums.DomainNameSource;
import odata.msgraph.client.beta.enums.EasAuthenticationMethod;
import odata.msgraph.client.beta.enums.EasServices;
import odata.msgraph.client.beta.enums.EmailCertificateType;
import odata.msgraph.client.beta.enums.EmailSyncDuration;
import odata.msgraph.client.beta.enums.UserEmailSource;
import odata.msgraph.client.beta.enums.UsernameSource;
/**
* “By providing configurations in this profile you can instruct the native email
* client on iOS devices to communicate with an Exchange server and get email,
* contacts, calendar, reminders, and notes. Furthermore, you can also specify how
* much email to sync and how often the device should sync.”
*/@JsonPropertyOrder({
"@odata.type",
"accountName",
"authenticationMethod",
"blockMovingMessagesToOtherEmailAccounts",
"blockSendingEmailFromThirdPartyApps",
"blockSyncingRecentlyUsedEmailAddresses",
"durationOfEmailToSync",
"easServices",
"easServicesUserOverrideEnabled",
"emailAddressSource",
"encryptionCertificateType",
"hostName",
"perAppVPNProfileId",
"requireSmime",
"requireSsl",
"signingCertificateType",
"smimeEnablePerMessageSwitch",
"smimeEncryptByDefaultEnabled",
"smimeEncryptByDefaultUserOverrideEnabled",
"smimeEncryptionCertificateUserOverrideEnabled",
"smimeSigningCertificateUserOverrideEnabled",
"smimeSigningEnabled",
"smimeSigningUserOverrideEnabled",
"useOAuth"})
@JsonInclude(Include.NON_NULL)
public class IosEasEmailProfileConfiguration extends EasEmailProfileConfigurationBase implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.iosEasEmailProfileConfiguration";
}
@JsonProperty("accountName")
protected String accountName;
@JsonProperty("authenticationMethod")
protected EasAuthenticationMethod authenticationMethod;
@JsonProperty("blockMovingMessagesToOtherEmailAccounts")
protected Boolean blockMovingMessagesToOtherEmailAccounts;
@JsonProperty("blockSendingEmailFromThirdPartyApps")
protected Boolean blockSendingEmailFromThirdPartyApps;
@JsonProperty("blockSyncingRecentlyUsedEmailAddresses")
protected Boolean blockSyncingRecentlyUsedEmailAddresses;
@JsonProperty("durationOfEmailToSync")
protected EmailSyncDuration durationOfEmailToSync;
@JsonProperty("easServices")
protected EasServices easServices;
@JsonProperty("easServicesUserOverrideEnabled")
protected Boolean easServicesUserOverrideEnabled;
@JsonProperty("emailAddressSource")
protected UserEmailSource emailAddressSource;
@JsonProperty("encryptionCertificateType")
protected EmailCertificateType encryptionCertificateType;
@JsonProperty("hostName")
protected String hostName;
@JsonProperty("perAppVPNProfileId")
protected String perAppVPNProfileId;
@JsonProperty("requireSmime")
protected Boolean requireSmime;
@JsonProperty("requireSsl")
protected Boolean requireSsl;
@JsonProperty("signingCertificateType")
protected EmailCertificateType signingCertificateType;
@JsonProperty("smimeEnablePerMessageSwitch")
protected Boolean smimeEnablePerMessageSwitch;
@JsonProperty("smimeEncryptByDefaultEnabled")
protected Boolean smimeEncryptByDefaultEnabled;
@JsonProperty("smimeEncryptByDefaultUserOverrideEnabled")
protected Boolean smimeEncryptByDefaultUserOverrideEnabled;
@JsonProperty("smimeEncryptionCertificateUserOverrideEnabled")
protected Boolean smimeEncryptionCertificateUserOverrideEnabled;
@JsonProperty("smimeSigningCertificateUserOverrideEnabled")
protected Boolean smimeSigningCertificateUserOverrideEnabled;
@JsonProperty("smimeSigningEnabled")
protected Boolean smimeSigningEnabled;
@JsonProperty("smimeSigningUserOverrideEnabled")
protected Boolean smimeSigningUserOverrideEnabled;
@JsonProperty("useOAuth")
protected Boolean useOAuth;
protected IosEasEmailProfileConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderIosEasEmailProfileConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode;
private DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition;
private DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private Boolean supportsScopeTags;
private Integer version;
private String customDomainName;
private DomainNameSource userDomainNameSource;
private UsernameSource usernameAADSource;
private UserEmailSource usernameSource;
private String accountName;
private EasAuthenticationMethod authenticationMethod;
private Boolean blockMovingMessagesToOtherEmailAccounts;
private Boolean blockSendingEmailFromThirdPartyApps;
private Boolean blockSyncingRecentlyUsedEmailAddresses;
private EmailSyncDuration durationOfEmailToSync;
private EasServices easServices;
private Boolean easServicesUserOverrideEnabled;
private UserEmailSource emailAddressSource;
private EmailCertificateType encryptionCertificateType;
private String hostName;
private String perAppVPNProfileId;
private Boolean requireSmime;
private Boolean requireSsl;
private EmailCertificateType signingCertificateType;
private Boolean smimeEnablePerMessageSwitch;
private Boolean smimeEncryptByDefaultEnabled;
private Boolean smimeEncryptByDefaultUserOverrideEnabled;
private Boolean smimeEncryptionCertificateUserOverrideEnabled;
private Boolean smimeSigningCertificateUserOverrideEnabled;
private Boolean smimeSigningEnabled;
private Boolean smimeSigningUserOverrideEnabled;
private Boolean useOAuth;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder deviceManagementApplicabilityRuleDeviceMode(DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode) {
this.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleDeviceMode");
return this;
}
public Builder deviceManagementApplicabilityRuleOsEdition(DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition) {
this.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsEdition");
return this;
}
public Builder deviceManagementApplicabilityRuleOsVersion(DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion) {
this.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsVersion");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder supportsScopeTags(Boolean supportsScopeTags) {
this.supportsScopeTags = supportsScopeTags;
this.changedFields = changedFields.add("supportsScopeTags");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
public Builder customDomainName(String customDomainName) {
this.customDomainName = customDomainName;
this.changedFields = changedFields.add("customDomainName");
return this;
}
public Builder userDomainNameSource(DomainNameSource userDomainNameSource) {
this.userDomainNameSource = userDomainNameSource;
this.changedFields = changedFields.add("userDomainNameSource");
return this;
}
public Builder usernameAADSource(UsernameSource usernameAADSource) {
this.usernameAADSource = usernameAADSource;
this.changedFields = changedFields.add("usernameAADSource");
return this;
}
public Builder usernameSource(UserEmailSource usernameSource) {
this.usernameSource = usernameSource;
this.changedFields = changedFields.add("usernameSource");
return this;
}
/**
* “Account name.”
*
* @param accountName
* value of {@code accountName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder accountName(String accountName) {
this.accountName = accountName;
this.changedFields = changedFields.add("accountName");
return this;
}
/**
* “Authentication method for this Email profile.”
*
* @param authenticationMethod
* value of {@code authenticationMethod} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder authenticationMethod(EasAuthenticationMethod authenticationMethod) {
this.authenticationMethod = authenticationMethod;
this.changedFields = changedFields.add("authenticationMethod");
return this;
}
/**
* “Indicates whether or not to block moving messages to other email accounts.”
*
* @param blockMovingMessagesToOtherEmailAccounts
* value of {@code blockMovingMessagesToOtherEmailAccounts} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder blockMovingMessagesToOtherEmailAccounts(Boolean blockMovingMessagesToOtherEmailAccounts) {
this.blockMovingMessagesToOtherEmailAccounts = blockMovingMessagesToOtherEmailAccounts;
this.changedFields = changedFields.add("blockMovingMessagesToOtherEmailAccounts");
return this;
}
/**
* “Indicates whether or not to block sending email from third party apps.”
*
* @param blockSendingEmailFromThirdPartyApps
* value of {@code blockSendingEmailFromThirdPartyApps} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder blockSendingEmailFromThirdPartyApps(Boolean blockSendingEmailFromThirdPartyApps) {
this.blockSendingEmailFromThirdPartyApps = blockSendingEmailFromThirdPartyApps;
this.changedFields = changedFields.add("blockSendingEmailFromThirdPartyApps");
return this;
}
/**
* “Indicates whether or not to block syncing recently used email addresses, for
* instance - when composing new email.”
*
* @param blockSyncingRecentlyUsedEmailAddresses
* value of {@code blockSyncingRecentlyUsedEmailAddresses} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder blockSyncingRecentlyUsedEmailAddresses(Boolean blockSyncingRecentlyUsedEmailAddresses) {
this.blockSyncingRecentlyUsedEmailAddresses = blockSyncingRecentlyUsedEmailAddresses;
this.changedFields = changedFields.add("blockSyncingRecentlyUsedEmailAddresses");
return this;
}
/**
* “Duration of time email should be synced back to.”
*
* @param durationOfEmailToSync
* value of {@code durationOfEmailToSync} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder durationOfEmailToSync(EmailSyncDuration durationOfEmailToSync) {
this.durationOfEmailToSync = durationOfEmailToSync;
this.changedFields = changedFields.add("durationOfEmailToSync");
return this;
}
/**
* “Exchange data to sync.”
*
* @param easServices
* value of {@code easServices} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder easServices(EasServices easServices) {
this.easServices = easServices;
this.changedFields = changedFields.add("easServices");
return this;
}
/**
* “Allow users to change sync settings.”
*
* @param easServicesUserOverrideEnabled
* value of {@code easServicesUserOverrideEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder easServicesUserOverrideEnabled(Boolean easServicesUserOverrideEnabled) {
this.easServicesUserOverrideEnabled = easServicesUserOverrideEnabled;
this.changedFields = changedFields.add("easServicesUserOverrideEnabled");
return this;
}
/**
* “Email attribute that is picked from AAD and injected into this profile before
* installing on the device.”
*
* @param emailAddressSource
* value of {@code emailAddressSource} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder emailAddressSource(UserEmailSource emailAddressSource) {
this.emailAddressSource = emailAddressSource;
this.changedFields = changedFields.add("emailAddressSource");
return this;
}
/**
* “Encryption Certificate type for this Email profile.”
*
* @param encryptionCertificateType
* value of {@code encryptionCertificateType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder encryptionCertificateType(EmailCertificateType encryptionCertificateType) {
this.encryptionCertificateType = encryptionCertificateType;
this.changedFields = changedFields.add("encryptionCertificateType");
return this;
}
/**
* “Exchange location that (URL) that the native mail app connects to.”
*
* @param hostName
* value of {@code hostName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder hostName(String hostName) {
this.hostName = hostName;
this.changedFields = changedFields.add("hostName");
return this;
}
/**
* “Profile ID of the Per-App VPN policy to be used to access emails from the native
* Mail client”
*
* @param perAppVPNProfileId
* value of {@code perAppVPNProfileId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder perAppVPNProfileId(String perAppVPNProfileId) {
this.perAppVPNProfileId = perAppVPNProfileId;
this.changedFields = changedFields.add("perAppVPNProfileId");
return this;
}
/**
* “Indicates whether or not to use S/MIME certificate.”
*
* @param requireSmime
* value of {@code requireSmime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder requireSmime(Boolean requireSmime) {
this.requireSmime = requireSmime;
this.changedFields = changedFields.add("requireSmime");
return this;
}
/**
* “Indicates whether or not to use SSL.”
*
* @param requireSsl
* value of {@code requireSsl} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder requireSsl(Boolean requireSsl) {
this.requireSsl = requireSsl;
this.changedFields = changedFields.add("requireSsl");
return this;
}
/**
* “Signing Certificate type for this Email profile.”
*
* @param signingCertificateType
* value of {@code signingCertificateType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder signingCertificateType(EmailCertificateType signingCertificateType) {
this.signingCertificateType = signingCertificateType;
this.changedFields = changedFields.add("signingCertificateType");
return this;
}
/**
* “Indicates whether or not to allow unencrypted emails.”
*
* @param smimeEnablePerMessageSwitch
* value of {@code smimeEnablePerMessageSwitch} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder smimeEnablePerMessageSwitch(Boolean smimeEnablePerMessageSwitch) {
this.smimeEnablePerMessageSwitch = smimeEnablePerMessageSwitch;
this.changedFields = changedFields.add("smimeEnablePerMessageSwitch");
return this;
}
/**
* “If set to true S/MIME encryption is enabled by default.”
*
* @param smimeEncryptByDefaultEnabled
* value of {@code smimeEncryptByDefaultEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder smimeEncryptByDefaultEnabled(Boolean smimeEncryptByDefaultEnabled) {
this.smimeEncryptByDefaultEnabled = smimeEncryptByDefaultEnabled;
this.changedFields = changedFields.add("smimeEncryptByDefaultEnabled");
return this;
}
/**
* “If set to true, the user can toggle the encryption by default setting.”
*
* @param smimeEncryptByDefaultUserOverrideEnabled
* value of {@code smimeEncryptByDefaultUserOverrideEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder smimeEncryptByDefaultUserOverrideEnabled(Boolean smimeEncryptByDefaultUserOverrideEnabled) {
this.smimeEncryptByDefaultUserOverrideEnabled = smimeEncryptByDefaultUserOverrideEnabled;
this.changedFields = changedFields.add("smimeEncryptByDefaultUserOverrideEnabled");
return this;
}
/**
* “If set to true the user can select the S/MIME encryption identity.”
*
* @param smimeEncryptionCertificateUserOverrideEnabled
* value of {@code smimeEncryptionCertificateUserOverrideEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder smimeEncryptionCertificateUserOverrideEnabled(Boolean smimeEncryptionCertificateUserOverrideEnabled) {
this.smimeEncryptionCertificateUserOverrideEnabled = smimeEncryptionCertificateUserOverrideEnabled;
this.changedFields = changedFields.add("smimeEncryptionCertificateUserOverrideEnabled");
return this;
}
/**
* “If set to true, the user can select the signing identity.”
*
* @param smimeSigningCertificateUserOverrideEnabled
* value of {@code smimeSigningCertificateUserOverrideEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder smimeSigningCertificateUserOverrideEnabled(Boolean smimeSigningCertificateUserOverrideEnabled) {
this.smimeSigningCertificateUserOverrideEnabled = smimeSigningCertificateUserOverrideEnabled;
this.changedFields = changedFields.add("smimeSigningCertificateUserOverrideEnabled");
return this;
}
/**
* “If set to true S/MIME signing is enabled for this account”
*
* @param smimeSigningEnabled
* value of {@code smimeSigningEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder smimeSigningEnabled(Boolean smimeSigningEnabled) {
this.smimeSigningEnabled = smimeSigningEnabled;
this.changedFields = changedFields.add("smimeSigningEnabled");
return this;
}
/**
* “If set to true, the user can toggle S/MIME signing on or off.”
*
* @param smimeSigningUserOverrideEnabled
* value of {@code smimeSigningUserOverrideEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder smimeSigningUserOverrideEnabled(Boolean smimeSigningUserOverrideEnabled) {
this.smimeSigningUserOverrideEnabled = smimeSigningUserOverrideEnabled;
this.changedFields = changedFields.add("smimeSigningUserOverrideEnabled");
return this;
}
/**
* “Specifies whether the connection should use OAuth for authentication.”
*
* @param useOAuth
* value of {@code useOAuth} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder useOAuth(Boolean useOAuth) {
this.useOAuth = useOAuth;
this.changedFields = changedFields.add("useOAuth");
return this;
}
public IosEasEmailProfileConfiguration build() {
IosEasEmailProfileConfiguration _x = new IosEasEmailProfileConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.iosEasEmailProfileConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.customDomainName = customDomainName;
_x.userDomainNameSource = userDomainNameSource;
_x.usernameAADSource = usernameAADSource;
_x.usernameSource = usernameSource;
_x.accountName = accountName;
_x.authenticationMethod = authenticationMethod;
_x.blockMovingMessagesToOtherEmailAccounts = blockMovingMessagesToOtherEmailAccounts;
_x.blockSendingEmailFromThirdPartyApps = blockSendingEmailFromThirdPartyApps;
_x.blockSyncingRecentlyUsedEmailAddresses = blockSyncingRecentlyUsedEmailAddresses;
_x.durationOfEmailToSync = durationOfEmailToSync;
_x.easServices = easServices;
_x.easServicesUserOverrideEnabled = easServicesUserOverrideEnabled;
_x.emailAddressSource = emailAddressSource;
_x.encryptionCertificateType = encryptionCertificateType;
_x.hostName = hostName;
_x.perAppVPNProfileId = perAppVPNProfileId;
_x.requireSmime = requireSmime;
_x.requireSsl = requireSsl;
_x.signingCertificateType = signingCertificateType;
_x.smimeEnablePerMessageSwitch = smimeEnablePerMessageSwitch;
_x.smimeEncryptByDefaultEnabled = smimeEncryptByDefaultEnabled;
_x.smimeEncryptByDefaultUserOverrideEnabled = smimeEncryptByDefaultUserOverrideEnabled;
_x.smimeEncryptionCertificateUserOverrideEnabled = smimeEncryptionCertificateUserOverrideEnabled;
_x.smimeSigningCertificateUserOverrideEnabled = smimeSigningCertificateUserOverrideEnabled;
_x.smimeSigningEnabled = smimeSigningEnabled;
_x.smimeSigningUserOverrideEnabled = smimeSigningUserOverrideEnabled;
_x.useOAuth = useOAuth;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Account name.”
*
* @return property accountName
*/
@Property(name="accountName")
@JsonIgnore
public Optional getAccountName() {
return Optional.ofNullable(accountName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code accountName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Account name.”
*
* @param accountName
* new value of {@code accountName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code accountName} field changed
*/
public IosEasEmailProfileConfiguration withAccountName(String accountName) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("accountName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.accountName = accountName;
return _x;
}
/**
* “Authentication method for this Email profile.”
*
* @return property authenticationMethod
*/
@Property(name="authenticationMethod")
@JsonIgnore
public Optional getAuthenticationMethod() {
return Optional.ofNullable(authenticationMethod);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* authenticationMethod} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Authentication method for this Email profile.”
*
* @param authenticationMethod
* new value of {@code authenticationMethod} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code authenticationMethod} field changed
*/
public IosEasEmailProfileConfiguration withAuthenticationMethod(EasAuthenticationMethod authenticationMethod) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("authenticationMethod");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.authenticationMethod = authenticationMethod;
return _x;
}
/**
* “Indicates whether or not to block moving messages to other email accounts.”
*
* @return property blockMovingMessagesToOtherEmailAccounts
*/
@Property(name="blockMovingMessagesToOtherEmailAccounts")
@JsonIgnore
public Optional getBlockMovingMessagesToOtherEmailAccounts() {
return Optional.ofNullable(blockMovingMessagesToOtherEmailAccounts);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* blockMovingMessagesToOtherEmailAccounts} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Indicates whether or not to block moving messages to other email accounts.”
*
* @param blockMovingMessagesToOtherEmailAccounts
* new value of {@code blockMovingMessagesToOtherEmailAccounts} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code blockMovingMessagesToOtherEmailAccounts} field changed
*/
public IosEasEmailProfileConfiguration withBlockMovingMessagesToOtherEmailAccounts(Boolean blockMovingMessagesToOtherEmailAccounts) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("blockMovingMessagesToOtherEmailAccounts");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.blockMovingMessagesToOtherEmailAccounts = blockMovingMessagesToOtherEmailAccounts;
return _x;
}
/**
* “Indicates whether or not to block sending email from third party apps.”
*
* @return property blockSendingEmailFromThirdPartyApps
*/
@Property(name="blockSendingEmailFromThirdPartyApps")
@JsonIgnore
public Optional getBlockSendingEmailFromThirdPartyApps() {
return Optional.ofNullable(blockSendingEmailFromThirdPartyApps);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* blockSendingEmailFromThirdPartyApps} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Indicates whether or not to block sending email from third party apps.”
*
* @param blockSendingEmailFromThirdPartyApps
* new value of {@code blockSendingEmailFromThirdPartyApps} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code blockSendingEmailFromThirdPartyApps} field changed
*/
public IosEasEmailProfileConfiguration withBlockSendingEmailFromThirdPartyApps(Boolean blockSendingEmailFromThirdPartyApps) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("blockSendingEmailFromThirdPartyApps");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.blockSendingEmailFromThirdPartyApps = blockSendingEmailFromThirdPartyApps;
return _x;
}
/**
* “Indicates whether or not to block syncing recently used email addresses, for
* instance - when composing new email.”
*
* @return property blockSyncingRecentlyUsedEmailAddresses
*/
@Property(name="blockSyncingRecentlyUsedEmailAddresses")
@JsonIgnore
public Optional getBlockSyncingRecentlyUsedEmailAddresses() {
return Optional.ofNullable(blockSyncingRecentlyUsedEmailAddresses);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* blockSyncingRecentlyUsedEmailAddresses} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Indicates whether or not to block syncing recently used email addresses, for
* instance - when composing new email.”
*
* @param blockSyncingRecentlyUsedEmailAddresses
* new value of {@code blockSyncingRecentlyUsedEmailAddresses} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code blockSyncingRecentlyUsedEmailAddresses} field changed
*/
public IosEasEmailProfileConfiguration withBlockSyncingRecentlyUsedEmailAddresses(Boolean blockSyncingRecentlyUsedEmailAddresses) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("blockSyncingRecentlyUsedEmailAddresses");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.blockSyncingRecentlyUsedEmailAddresses = blockSyncingRecentlyUsedEmailAddresses;
return _x;
}
/**
* “Duration of time email should be synced back to.”
*
* @return property durationOfEmailToSync
*/
@Property(name="durationOfEmailToSync")
@JsonIgnore
public Optional getDurationOfEmailToSync() {
return Optional.ofNullable(durationOfEmailToSync);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* durationOfEmailToSync} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Duration of time email should be synced back to.”
*
* @param durationOfEmailToSync
* new value of {@code durationOfEmailToSync} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code durationOfEmailToSync} field changed
*/
public IosEasEmailProfileConfiguration withDurationOfEmailToSync(EmailSyncDuration durationOfEmailToSync) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("durationOfEmailToSync");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.durationOfEmailToSync = durationOfEmailToSync;
return _x;
}
/**
* “Exchange data to sync.”
*
* @return property easServices
*/
@Property(name="easServices")
@JsonIgnore
public Optional getEasServices() {
return Optional.ofNullable(easServices);
}
/**
* Returns an immutable copy of {@code this} with just the {@code easServices}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Exchange data to sync.”
*
* @param easServices
* new value of {@code easServices} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code easServices} field changed
*/
public IosEasEmailProfileConfiguration withEasServices(EasServices easServices) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("easServices");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.easServices = easServices;
return _x;
}
/**
* “Allow users to change sync settings.”
*
* @return property easServicesUserOverrideEnabled
*/
@Property(name="easServicesUserOverrideEnabled")
@JsonIgnore
public Optional getEasServicesUserOverrideEnabled() {
return Optional.ofNullable(easServicesUserOverrideEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* easServicesUserOverrideEnabled} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Allow users to change sync settings.”
*
* @param easServicesUserOverrideEnabled
* new value of {@code easServicesUserOverrideEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code easServicesUserOverrideEnabled} field changed
*/
public IosEasEmailProfileConfiguration withEasServicesUserOverrideEnabled(Boolean easServicesUserOverrideEnabled) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("easServicesUserOverrideEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.easServicesUserOverrideEnabled = easServicesUserOverrideEnabled;
return _x;
}
/**
* “Email attribute that is picked from AAD and injected into this profile before
* installing on the device.”
*
* @return property emailAddressSource
*/
@Property(name="emailAddressSource")
@JsonIgnore
public Optional getEmailAddressSource() {
return Optional.ofNullable(emailAddressSource);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* emailAddressSource} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Email attribute that is picked from AAD and injected into this profile before
* installing on the device.”
*
* @param emailAddressSource
* new value of {@code emailAddressSource} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code emailAddressSource} field changed
*/
public IosEasEmailProfileConfiguration withEmailAddressSource(UserEmailSource emailAddressSource) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("emailAddressSource");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.emailAddressSource = emailAddressSource;
return _x;
}
/**
* “Encryption Certificate type for this Email profile.”
*
* @return property encryptionCertificateType
*/
@Property(name="encryptionCertificateType")
@JsonIgnore
public Optional getEncryptionCertificateType() {
return Optional.ofNullable(encryptionCertificateType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* encryptionCertificateType} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Encryption Certificate type for this Email profile.”
*
* @param encryptionCertificateType
* new value of {@code encryptionCertificateType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code encryptionCertificateType} field changed
*/
public IosEasEmailProfileConfiguration withEncryptionCertificateType(EmailCertificateType encryptionCertificateType) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("encryptionCertificateType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.encryptionCertificateType = encryptionCertificateType;
return _x;
}
/**
* “Exchange location that (URL) that the native mail app connects to.”
*
* @return property hostName
*/
@Property(name="hostName")
@JsonIgnore
public Optional getHostName() {
return Optional.ofNullable(hostName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code hostName} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Exchange location that (URL) that the native mail app connects to.”
*
* @param hostName
* new value of {@code hostName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code hostName} field changed
*/
public IosEasEmailProfileConfiguration withHostName(String hostName) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("hostName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.hostName = hostName;
return _x;
}
/**
* “Profile ID of the Per-App VPN policy to be used to access emails from the native
* Mail client”
*
* @return property perAppVPNProfileId
*/
@Property(name="perAppVPNProfileId")
@JsonIgnore
public Optional getPerAppVPNProfileId() {
return Optional.ofNullable(perAppVPNProfileId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* perAppVPNProfileId} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Profile ID of the Per-App VPN policy to be used to access emails from the native
* Mail client”
*
* @param perAppVPNProfileId
* new value of {@code perAppVPNProfileId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code perAppVPNProfileId} field changed
*/
public IosEasEmailProfileConfiguration withPerAppVPNProfileId(String perAppVPNProfileId) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("perAppVPNProfileId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.perAppVPNProfileId = perAppVPNProfileId;
return _x;
}
/**
* “Indicates whether or not to use S/MIME certificate.”
*
* @return property requireSmime
*/
@Property(name="requireSmime")
@JsonIgnore
public Optional getRequireSmime() {
return Optional.ofNullable(requireSmime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code requireSmime}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates whether or not to use S/MIME certificate.”
*
* @param requireSmime
* new value of {@code requireSmime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code requireSmime} field changed
*/
public IosEasEmailProfileConfiguration withRequireSmime(Boolean requireSmime) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("requireSmime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.requireSmime = requireSmime;
return _x;
}
/**
* “Indicates whether or not to use SSL.”
*
* @return property requireSsl
*/
@Property(name="requireSsl")
@JsonIgnore
public Optional getRequireSsl() {
return Optional.ofNullable(requireSsl);
}
/**
* Returns an immutable copy of {@code this} with just the {@code requireSsl} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Indicates whether or not to use SSL.”
*
* @param requireSsl
* new value of {@code requireSsl} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code requireSsl} field changed
*/
public IosEasEmailProfileConfiguration withRequireSsl(Boolean requireSsl) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("requireSsl");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.requireSsl = requireSsl;
return _x;
}
/**
* “Signing Certificate type for this Email profile.”
*
* @return property signingCertificateType
*/
@Property(name="signingCertificateType")
@JsonIgnore
public Optional getSigningCertificateType() {
return Optional.ofNullable(signingCertificateType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* signingCertificateType} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Signing Certificate type for this Email profile.”
*
* @param signingCertificateType
* new value of {@code signingCertificateType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code signingCertificateType} field changed
*/
public IosEasEmailProfileConfiguration withSigningCertificateType(EmailCertificateType signingCertificateType) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("signingCertificateType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.signingCertificateType = signingCertificateType;
return _x;
}
/**
* “Indicates whether or not to allow unencrypted emails.”
*
* @return property smimeEnablePerMessageSwitch
*/
@Property(name="smimeEnablePerMessageSwitch")
@JsonIgnore
public Optional getSmimeEnablePerMessageSwitch() {
return Optional.ofNullable(smimeEnablePerMessageSwitch);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* smimeEnablePerMessageSwitch} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether or not to allow unencrypted emails.”
*
* @param smimeEnablePerMessageSwitch
* new value of {@code smimeEnablePerMessageSwitch} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code smimeEnablePerMessageSwitch} field changed
*/
public IosEasEmailProfileConfiguration withSmimeEnablePerMessageSwitch(Boolean smimeEnablePerMessageSwitch) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("smimeEnablePerMessageSwitch");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.smimeEnablePerMessageSwitch = smimeEnablePerMessageSwitch;
return _x;
}
/**
* “If set to true S/MIME encryption is enabled by default.”
*
* @return property smimeEncryptByDefaultEnabled
*/
@Property(name="smimeEncryptByDefaultEnabled")
@JsonIgnore
public Optional getSmimeEncryptByDefaultEnabled() {
return Optional.ofNullable(smimeEncryptByDefaultEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* smimeEncryptByDefaultEnabled} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “If set to true S/MIME encryption is enabled by default.”
*
* @param smimeEncryptByDefaultEnabled
* new value of {@code smimeEncryptByDefaultEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code smimeEncryptByDefaultEnabled} field changed
*/
public IosEasEmailProfileConfiguration withSmimeEncryptByDefaultEnabled(Boolean smimeEncryptByDefaultEnabled) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("smimeEncryptByDefaultEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.smimeEncryptByDefaultEnabled = smimeEncryptByDefaultEnabled;
return _x;
}
/**
* “If set to true, the user can toggle the encryption by default setting.”
*
* @return property smimeEncryptByDefaultUserOverrideEnabled
*/
@Property(name="smimeEncryptByDefaultUserOverrideEnabled")
@JsonIgnore
public Optional getSmimeEncryptByDefaultUserOverrideEnabled() {
return Optional.ofNullable(smimeEncryptByDefaultUserOverrideEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* smimeEncryptByDefaultUserOverrideEnabled} field changed. Field description below
* . The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “If set to true, the user can toggle the encryption by default setting.”
*
* @param smimeEncryptByDefaultUserOverrideEnabled
* new value of {@code smimeEncryptByDefaultUserOverrideEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code smimeEncryptByDefaultUserOverrideEnabled} field changed
*/
public IosEasEmailProfileConfiguration withSmimeEncryptByDefaultUserOverrideEnabled(Boolean smimeEncryptByDefaultUserOverrideEnabled) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("smimeEncryptByDefaultUserOverrideEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.smimeEncryptByDefaultUserOverrideEnabled = smimeEncryptByDefaultUserOverrideEnabled;
return _x;
}
/**
* “If set to true the user can select the S/MIME encryption identity.”
*
* @return property smimeEncryptionCertificateUserOverrideEnabled
*/
@Property(name="smimeEncryptionCertificateUserOverrideEnabled")
@JsonIgnore
public Optional getSmimeEncryptionCertificateUserOverrideEnabled() {
return Optional.ofNullable(smimeEncryptionCertificateUserOverrideEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* smimeEncryptionCertificateUserOverrideEnabled} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “If set to true the user can select the S/MIME encryption identity.”
*
* @param smimeEncryptionCertificateUserOverrideEnabled
* new value of {@code smimeEncryptionCertificateUserOverrideEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code smimeEncryptionCertificateUserOverrideEnabled} field changed
*/
public IosEasEmailProfileConfiguration withSmimeEncryptionCertificateUserOverrideEnabled(Boolean smimeEncryptionCertificateUserOverrideEnabled) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("smimeEncryptionCertificateUserOverrideEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.smimeEncryptionCertificateUserOverrideEnabled = smimeEncryptionCertificateUserOverrideEnabled;
return _x;
}
/**
* “If set to true, the user can select the signing identity.”
*
* @return property smimeSigningCertificateUserOverrideEnabled
*/
@Property(name="smimeSigningCertificateUserOverrideEnabled")
@JsonIgnore
public Optional getSmimeSigningCertificateUserOverrideEnabled() {
return Optional.ofNullable(smimeSigningCertificateUserOverrideEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* smimeSigningCertificateUserOverrideEnabled} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “If set to true, the user can select the signing identity.”
*
* @param smimeSigningCertificateUserOverrideEnabled
* new value of {@code smimeSigningCertificateUserOverrideEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code smimeSigningCertificateUserOverrideEnabled} field changed
*/
public IosEasEmailProfileConfiguration withSmimeSigningCertificateUserOverrideEnabled(Boolean smimeSigningCertificateUserOverrideEnabled) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("smimeSigningCertificateUserOverrideEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.smimeSigningCertificateUserOverrideEnabled = smimeSigningCertificateUserOverrideEnabled;
return _x;
}
/**
* “If set to true S/MIME signing is enabled for this account”
*
* @return property smimeSigningEnabled
*/
@Property(name="smimeSigningEnabled")
@JsonIgnore
public Optional getSmimeSigningEnabled() {
return Optional.ofNullable(smimeSigningEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* smimeSigningEnabled} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “If set to true S/MIME signing is enabled for this account”
*
* @param smimeSigningEnabled
* new value of {@code smimeSigningEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code smimeSigningEnabled} field changed
*/
public IosEasEmailProfileConfiguration withSmimeSigningEnabled(Boolean smimeSigningEnabled) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("smimeSigningEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.smimeSigningEnabled = smimeSigningEnabled;
return _x;
}
/**
* “If set to true, the user can toggle S/MIME signing on or off.”
*
* @return property smimeSigningUserOverrideEnabled
*/
@Property(name="smimeSigningUserOverrideEnabled")
@JsonIgnore
public Optional getSmimeSigningUserOverrideEnabled() {
return Optional.ofNullable(smimeSigningUserOverrideEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* smimeSigningUserOverrideEnabled} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “If set to true, the user can toggle S/MIME signing on or off.”
*
* @param smimeSigningUserOverrideEnabled
* new value of {@code smimeSigningUserOverrideEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code smimeSigningUserOverrideEnabled} field changed
*/
public IosEasEmailProfileConfiguration withSmimeSigningUserOverrideEnabled(Boolean smimeSigningUserOverrideEnabled) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("smimeSigningUserOverrideEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.smimeSigningUserOverrideEnabled = smimeSigningUserOverrideEnabled;
return _x;
}
/**
* “Specifies whether the connection should use OAuth for authentication.”
*
* @return property useOAuth
*/
@Property(name="useOAuth")
@JsonIgnore
public Optional getUseOAuth() {
return Optional.ofNullable(useOAuth);
}
/**
* Returns an immutable copy of {@code this} with just the {@code useOAuth} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Specifies whether the connection should use OAuth for authentication.”
*
* @param useOAuth
* new value of {@code useOAuth} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code useOAuth} field changed
*/
public IosEasEmailProfileConfiguration withUseOAuth(Boolean useOAuth) {
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = changedFields.add("useOAuth");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosEasEmailProfileConfiguration");
_x.useOAuth = useOAuth;
return _x;
}
public IosEasEmailProfileConfiguration withUnmappedField(String name, String value) {
IosEasEmailProfileConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “Tenant level settings for the Derived Credentials to be used for authentication.”
*
* @return navigational property derivedCredentialSettings
*/
@NavigationProperty(name="derivedCredentialSettings")
@JsonIgnore
public DeviceManagementDerivedCredentialSettingsRequest getDerivedCredentialSettings() {
return new DeviceManagementDerivedCredentialSettingsRequest(contextPath.addSegment("derivedCredentialSettings"), RequestHelper.getValue(unmappedFields, "derivedCredentialSettings"));
}
/**
* “Identity certificate.”
*
* @return navigational property identityCertificate
*/
@NavigationProperty(name="identityCertificate")
@JsonIgnore
public IosCertificateProfileBaseRequest getIdentityCertificate() {
return new IosCertificateProfileBaseRequest(contextPath.addSegment("identityCertificate"), RequestHelper.getValue(unmappedFields, "identityCertificate"));
}
/**
* “S/MIME encryption certificate.”
*
* @return navigational property smimeEncryptionCertificate
*/
@NavigationProperty(name="smimeEncryptionCertificate")
@JsonIgnore
public IosCertificateProfileRequest getSmimeEncryptionCertificate() {
return new IosCertificateProfileRequest(contextPath.addSegment("smimeEncryptionCertificate"), RequestHelper.getValue(unmappedFields, "smimeEncryptionCertificate"));
}
/**
* “S/MIME signing certificate.”
*
* @return navigational property smimeSigningCertificate
*/
@NavigationProperty(name="smimeSigningCertificate")
@JsonIgnore
public IosCertificateProfileRequest getSmimeSigningCertificate() {
return new IosCertificateProfileRequest(contextPath.addSegment("smimeSigningCertificate"), RequestHelper.getValue(unmappedFields, "smimeSigningCertificate"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public IosEasEmailProfileConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public IosEasEmailProfileConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
IosEasEmailProfileConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private IosEasEmailProfileConfiguration _copy() {
IosEasEmailProfileConfiguration _x = new IosEasEmailProfileConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.customDomainName = customDomainName;
_x.userDomainNameSource = userDomainNameSource;
_x.usernameAADSource = usernameAADSource;
_x.usernameSource = usernameSource;
_x.accountName = accountName;
_x.authenticationMethod = authenticationMethod;
_x.blockMovingMessagesToOtherEmailAccounts = blockMovingMessagesToOtherEmailAccounts;
_x.blockSendingEmailFromThirdPartyApps = blockSendingEmailFromThirdPartyApps;
_x.blockSyncingRecentlyUsedEmailAddresses = blockSyncingRecentlyUsedEmailAddresses;
_x.durationOfEmailToSync = durationOfEmailToSync;
_x.easServices = easServices;
_x.easServicesUserOverrideEnabled = easServicesUserOverrideEnabled;
_x.emailAddressSource = emailAddressSource;
_x.encryptionCertificateType = encryptionCertificateType;
_x.hostName = hostName;
_x.perAppVPNProfileId = perAppVPNProfileId;
_x.requireSmime = requireSmime;
_x.requireSsl = requireSsl;
_x.signingCertificateType = signingCertificateType;
_x.smimeEnablePerMessageSwitch = smimeEnablePerMessageSwitch;
_x.smimeEncryptByDefaultEnabled = smimeEncryptByDefaultEnabled;
_x.smimeEncryptByDefaultUserOverrideEnabled = smimeEncryptByDefaultUserOverrideEnabled;
_x.smimeEncryptionCertificateUserOverrideEnabled = smimeEncryptionCertificateUserOverrideEnabled;
_x.smimeSigningCertificateUserOverrideEnabled = smimeSigningCertificateUserOverrideEnabled;
_x.smimeSigningEnabled = smimeSigningEnabled;
_x.smimeSigningUserOverrideEnabled = smimeSigningUserOverrideEnabled;
_x.useOAuth = useOAuth;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("IosEasEmailProfileConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("deviceManagementApplicabilityRuleDeviceMode=");
b.append(this.deviceManagementApplicabilityRuleDeviceMode);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsEdition=");
b.append(this.deviceManagementApplicabilityRuleOsEdition);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsVersion=");
b.append(this.deviceManagementApplicabilityRuleOsVersion);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("supportsScopeTags=");
b.append(this.supportsScopeTags);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("customDomainName=");
b.append(this.customDomainName);
b.append(", ");
b.append("userDomainNameSource=");
b.append(this.userDomainNameSource);
b.append(", ");
b.append("usernameAADSource=");
b.append(this.usernameAADSource);
b.append(", ");
b.append("usernameSource=");
b.append(this.usernameSource);
b.append(", ");
b.append("accountName=");
b.append(this.accountName);
b.append(", ");
b.append("authenticationMethod=");
b.append(this.authenticationMethod);
b.append(", ");
b.append("blockMovingMessagesToOtherEmailAccounts=");
b.append(this.blockMovingMessagesToOtherEmailAccounts);
b.append(", ");
b.append("blockSendingEmailFromThirdPartyApps=");
b.append(this.blockSendingEmailFromThirdPartyApps);
b.append(", ");
b.append("blockSyncingRecentlyUsedEmailAddresses=");
b.append(this.blockSyncingRecentlyUsedEmailAddresses);
b.append(", ");
b.append("durationOfEmailToSync=");
b.append(this.durationOfEmailToSync);
b.append(", ");
b.append("easServices=");
b.append(this.easServices);
b.append(", ");
b.append("easServicesUserOverrideEnabled=");
b.append(this.easServicesUserOverrideEnabled);
b.append(", ");
b.append("emailAddressSource=");
b.append(this.emailAddressSource);
b.append(", ");
b.append("encryptionCertificateType=");
b.append(this.encryptionCertificateType);
b.append(", ");
b.append("hostName=");
b.append(this.hostName);
b.append(", ");
b.append("perAppVPNProfileId=");
b.append(this.perAppVPNProfileId);
b.append(", ");
b.append("requireSmime=");
b.append(this.requireSmime);
b.append(", ");
b.append("requireSsl=");
b.append(this.requireSsl);
b.append(", ");
b.append("signingCertificateType=");
b.append(this.signingCertificateType);
b.append(", ");
b.append("smimeEnablePerMessageSwitch=");
b.append(this.smimeEnablePerMessageSwitch);
b.append(", ");
b.append("smimeEncryptByDefaultEnabled=");
b.append(this.smimeEncryptByDefaultEnabled);
b.append(", ");
b.append("smimeEncryptByDefaultUserOverrideEnabled=");
b.append(this.smimeEncryptByDefaultUserOverrideEnabled);
b.append(", ");
b.append("smimeEncryptionCertificateUserOverrideEnabled=");
b.append(this.smimeEncryptionCertificateUserOverrideEnabled);
b.append(", ");
b.append("smimeSigningCertificateUserOverrideEnabled=");
b.append(this.smimeSigningCertificateUserOverrideEnabled);
b.append(", ");
b.append("smimeSigningEnabled=");
b.append(this.smimeSigningEnabled);
b.append(", ");
b.append("smimeSigningUserOverrideEnabled=");
b.append(this.smimeSigningUserOverrideEnabled);
b.append(", ");
b.append("useOAuth=");
b.append(this.useOAuth);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}