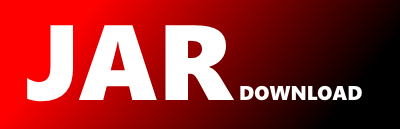
odata.msgraph.client.beta.entity.IosUpdateDeviceStatus Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Optional;
import odata.msgraph.client.beta.enums.ComplianceStatus;
import odata.msgraph.client.beta.enums.IosUpdatesInstallStatus;
@JsonPropertyOrder({
"@odata.type",
"complianceGracePeriodExpirationDateTime",
"deviceDisplayName",
"deviceId",
"deviceModel",
"installStatus",
"lastReportedDateTime",
"osVersion",
"platform",
"status",
"userId",
"userName",
"userPrincipalName"})
@JsonInclude(Include.NON_NULL)
public class IosUpdateDeviceStatus extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.iosUpdateDeviceStatus";
}
@JsonProperty("complianceGracePeriodExpirationDateTime")
protected OffsetDateTime complianceGracePeriodExpirationDateTime;
@JsonProperty("deviceDisplayName")
protected String deviceDisplayName;
@JsonProperty("deviceId")
protected String deviceId;
@JsonProperty("deviceModel")
protected String deviceModel;
@JsonProperty("installStatus")
protected IosUpdatesInstallStatus installStatus;
@JsonProperty("lastReportedDateTime")
protected OffsetDateTime lastReportedDateTime;
@JsonProperty("osVersion")
protected String osVersion;
@JsonProperty("platform")
protected Integer platform;
@JsonProperty("status")
protected ComplianceStatus status;
@JsonProperty("userId")
protected String userId;
@JsonProperty("userName")
protected String userName;
@JsonProperty("userPrincipalName")
protected String userPrincipalName;
protected IosUpdateDeviceStatus() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderIosUpdateDeviceStatus() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime complianceGracePeriodExpirationDateTime;
private String deviceDisplayName;
private String deviceId;
private String deviceModel;
private IosUpdatesInstallStatus installStatus;
private OffsetDateTime lastReportedDateTime;
private String osVersion;
private Integer platform;
private ComplianceStatus status;
private String userId;
private String userName;
private String userPrincipalName;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
/**
* “The DateTime when device compliance grace period expires”
*
* @param complianceGracePeriodExpirationDateTime
* value of {@code complianceGracePeriodExpirationDateTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder complianceGracePeriodExpirationDateTime(OffsetDateTime complianceGracePeriodExpirationDateTime) {
this.complianceGracePeriodExpirationDateTime = complianceGracePeriodExpirationDateTime;
this.changedFields = changedFields.add("complianceGracePeriodExpirationDateTime");
return this;
}
/**
* “Device name of the DevicePolicyStatus.”
*
* @param deviceDisplayName
* value of {@code deviceDisplayName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceDisplayName(String deviceDisplayName) {
this.deviceDisplayName = deviceDisplayName;
this.changedFields = changedFields.add("deviceDisplayName");
return this;
}
/**
* “The device id that is being reported.”
*
* @param deviceId
* value of {@code deviceId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceId(String deviceId) {
this.deviceId = deviceId;
this.changedFields = changedFields.add("deviceId");
return this;
}
/**
* “The device model that is being reported”
*
* @param deviceModel
* value of {@code deviceModel} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceModel(String deviceModel) {
this.deviceModel = deviceModel;
this.changedFields = changedFields.add("deviceModel");
return this;
}
/**
* “The installation status of the policy report.”
*
* @param installStatus
* value of {@code installStatus} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder installStatus(IosUpdatesInstallStatus installStatus) {
this.installStatus = installStatus;
this.changedFields = changedFields.add("installStatus");
return this;
}
/**
* “Last modified date time of the policy report.”
*
* @param lastReportedDateTime
* value of {@code lastReportedDateTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder lastReportedDateTime(OffsetDateTime lastReportedDateTime) {
this.lastReportedDateTime = lastReportedDateTime;
this.changedFields = changedFields.add("lastReportedDateTime");
return this;
}
/**
* “The device version that is being reported.”
*
* @param osVersion
* value of {@code osVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder osVersion(String osVersion) {
this.osVersion = osVersion;
this.changedFields = changedFields.add("osVersion");
return this;
}
/**
* “Platform of the device that is being reported”
*
* @param platform
* value of {@code platform} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder platform(Integer platform) {
this.platform = platform;
this.changedFields = changedFields.add("platform");
return this;
}
/**
* “Compliance status of the policy report.”
*
* @param status
* value of {@code status} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder status(ComplianceStatus status) {
this.status = status;
this.changedFields = changedFields.add("status");
return this;
}
/**
* “The User id that is being reported.”
*
* @param userId
* value of {@code userId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userId(String userId) {
this.userId = userId;
this.changedFields = changedFields.add("userId");
return this;
}
/**
* “The User Name that is being reported”
*
* @param userName
* value of {@code userName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userName(String userName) {
this.userName = userName;
this.changedFields = changedFields.add("userName");
return this;
}
/**
* “UserPrincipalName.”
*
* @param userPrincipalName
* value of {@code userPrincipalName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userPrincipalName(String userPrincipalName) {
this.userPrincipalName = userPrincipalName;
this.changedFields = changedFields.add("userPrincipalName");
return this;
}
public IosUpdateDeviceStatus build() {
IosUpdateDeviceStatus _x = new IosUpdateDeviceStatus();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.iosUpdateDeviceStatus";
_x.id = id;
_x.complianceGracePeriodExpirationDateTime = complianceGracePeriodExpirationDateTime;
_x.deviceDisplayName = deviceDisplayName;
_x.deviceId = deviceId;
_x.deviceModel = deviceModel;
_x.installStatus = installStatus;
_x.lastReportedDateTime = lastReportedDateTime;
_x.osVersion = osVersion;
_x.platform = platform;
_x.status = status;
_x.userId = userId;
_x.userName = userName;
_x.userPrincipalName = userPrincipalName;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “The DateTime when device compliance grace period expires”
*
* @return property complianceGracePeriodExpirationDateTime
*/
@Property(name="complianceGracePeriodExpirationDateTime")
@JsonIgnore
public Optional getComplianceGracePeriodExpirationDateTime() {
return Optional.ofNullable(complianceGracePeriodExpirationDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* complianceGracePeriodExpirationDateTime} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “The DateTime when device compliance grace period expires”
*
* @param complianceGracePeriodExpirationDateTime
* new value of {@code complianceGracePeriodExpirationDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code complianceGracePeriodExpirationDateTime} field changed
*/
public IosUpdateDeviceStatus withComplianceGracePeriodExpirationDateTime(OffsetDateTime complianceGracePeriodExpirationDateTime) {
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = changedFields.add("complianceGracePeriodExpirationDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosUpdateDeviceStatus");
_x.complianceGracePeriodExpirationDateTime = complianceGracePeriodExpirationDateTime;
return _x;
}
/**
* “Device name of the DevicePolicyStatus.”
*
* @return property deviceDisplayName
*/
@Property(name="deviceDisplayName")
@JsonIgnore
public Optional getDeviceDisplayName() {
return Optional.ofNullable(deviceDisplayName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceDisplayName
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Device name of the DevicePolicyStatus.”
*
* @param deviceDisplayName
* new value of {@code deviceDisplayName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceDisplayName} field changed
*/
public IosUpdateDeviceStatus withDeviceDisplayName(String deviceDisplayName) {
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = changedFields.add("deviceDisplayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosUpdateDeviceStatus");
_x.deviceDisplayName = deviceDisplayName;
return _x;
}
/**
* “The device id that is being reported.”
*
* @return property deviceId
*/
@Property(name="deviceId")
@JsonIgnore
public Optional getDeviceId() {
return Optional.ofNullable(deviceId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceId} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The device id that is being reported.”
*
* @param deviceId
* new value of {@code deviceId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceId} field changed
*/
public IosUpdateDeviceStatus withDeviceId(String deviceId) {
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = changedFields.add("deviceId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosUpdateDeviceStatus");
_x.deviceId = deviceId;
return _x;
}
/**
* “The device model that is being reported”
*
* @return property deviceModel
*/
@Property(name="deviceModel")
@JsonIgnore
public Optional getDeviceModel() {
return Optional.ofNullable(deviceModel);
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceModel}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The device model that is being reported”
*
* @param deviceModel
* new value of {@code deviceModel} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceModel} field changed
*/
public IosUpdateDeviceStatus withDeviceModel(String deviceModel) {
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = changedFields.add("deviceModel");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosUpdateDeviceStatus");
_x.deviceModel = deviceModel;
return _x;
}
/**
* “The installation status of the policy report.”
*
* @return property installStatus
*/
@Property(name="installStatus")
@JsonIgnore
public Optional getInstallStatus() {
return Optional.ofNullable(installStatus);
}
/**
* Returns an immutable copy of {@code this} with just the {@code installStatus}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The installation status of the policy report.”
*
* @param installStatus
* new value of {@code installStatus} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code installStatus} field changed
*/
public IosUpdateDeviceStatus withInstallStatus(IosUpdatesInstallStatus installStatus) {
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = changedFields.add("installStatus");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosUpdateDeviceStatus");
_x.installStatus = installStatus;
return _x;
}
/**
* “Last modified date time of the policy report.”
*
* @return property lastReportedDateTime
*/
@Property(name="lastReportedDateTime")
@JsonIgnore
public Optional getLastReportedDateTime() {
return Optional.ofNullable(lastReportedDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* lastReportedDateTime} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Last modified date time of the policy report.”
*
* @param lastReportedDateTime
* new value of {@code lastReportedDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code lastReportedDateTime} field changed
*/
public IosUpdateDeviceStatus withLastReportedDateTime(OffsetDateTime lastReportedDateTime) {
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = changedFields.add("lastReportedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosUpdateDeviceStatus");
_x.lastReportedDateTime = lastReportedDateTime;
return _x;
}
/**
* “The device version that is being reported.”
*
* @return property osVersion
*/
@Property(name="osVersion")
@JsonIgnore
public Optional getOsVersion() {
return Optional.ofNullable(osVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code osVersion} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The device version that is being reported.”
*
* @param osVersion
* new value of {@code osVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code osVersion} field changed
*/
public IosUpdateDeviceStatus withOsVersion(String osVersion) {
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = changedFields.add("osVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosUpdateDeviceStatus");
_x.osVersion = osVersion;
return _x;
}
/**
* “Platform of the device that is being reported”
*
* @return property platform
*/
@Property(name="platform")
@JsonIgnore
public Optional getPlatform() {
return Optional.ofNullable(platform);
}
/**
* Returns an immutable copy of {@code this} with just the {@code platform} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Platform of the device that is being reported”
*
* @param platform
* new value of {@code platform} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code platform} field changed
*/
public IosUpdateDeviceStatus withPlatform(Integer platform) {
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = changedFields.add("platform");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosUpdateDeviceStatus");
_x.platform = platform;
return _x;
}
/**
* “Compliance status of the policy report.”
*
* @return property status
*/
@Property(name="status")
@JsonIgnore
public Optional getStatus() {
return Optional.ofNullable(status);
}
/**
* Returns an immutable copy of {@code this} with just the {@code status} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Compliance status of the policy report.”
*
* @param status
* new value of {@code status} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code status} field changed
*/
public IosUpdateDeviceStatus withStatus(ComplianceStatus status) {
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = changedFields.add("status");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosUpdateDeviceStatus");
_x.status = status;
return _x;
}
/**
* “The User id that is being reported.”
*
* @return property userId
*/
@Property(name="userId")
@JsonIgnore
public Optional getUserId() {
return Optional.ofNullable(userId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userId} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The User id that is being reported.”
*
* @param userId
* new value of {@code userId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userId} field changed
*/
public IosUpdateDeviceStatus withUserId(String userId) {
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = changedFields.add("userId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosUpdateDeviceStatus");
_x.userId = userId;
return _x;
}
/**
* “The User Name that is being reported”
*
* @return property userName
*/
@Property(name="userName")
@JsonIgnore
public Optional getUserName() {
return Optional.ofNullable(userName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userName} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The User Name that is being reported”
*
* @param userName
* new value of {@code userName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userName} field changed
*/
public IosUpdateDeviceStatus withUserName(String userName) {
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = changedFields.add("userName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosUpdateDeviceStatus");
_x.userName = userName;
return _x;
}
/**
* “UserPrincipalName.”
*
* @return property userPrincipalName
*/
@Property(name="userPrincipalName")
@JsonIgnore
public Optional getUserPrincipalName() {
return Optional.ofNullable(userPrincipalName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userPrincipalName
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “UserPrincipalName.”
*
* @param userPrincipalName
* new value of {@code userPrincipalName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userPrincipalName} field changed
*/
public IosUpdateDeviceStatus withUserPrincipalName(String userPrincipalName) {
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = changedFields.add("userPrincipalName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosUpdateDeviceStatus");
_x.userPrincipalName = userPrincipalName;
return _x;
}
public IosUpdateDeviceStatus withUnmappedField(String name, String value) {
IosUpdateDeviceStatus _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public IosUpdateDeviceStatus patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public IosUpdateDeviceStatus put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
IosUpdateDeviceStatus _x = _copy();
_x.changedFields = null;
return _x;
}
private IosUpdateDeviceStatus _copy() {
IosUpdateDeviceStatus _x = new IosUpdateDeviceStatus();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.complianceGracePeriodExpirationDateTime = complianceGracePeriodExpirationDateTime;
_x.deviceDisplayName = deviceDisplayName;
_x.deviceId = deviceId;
_x.deviceModel = deviceModel;
_x.installStatus = installStatus;
_x.lastReportedDateTime = lastReportedDateTime;
_x.osVersion = osVersion;
_x.platform = platform;
_x.status = status;
_x.userId = userId;
_x.userName = userName;
_x.userPrincipalName = userPrincipalName;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("IosUpdateDeviceStatus[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("complianceGracePeriodExpirationDateTime=");
b.append(this.complianceGracePeriodExpirationDateTime);
b.append(", ");
b.append("deviceDisplayName=");
b.append(this.deviceDisplayName);
b.append(", ");
b.append("deviceId=");
b.append(this.deviceId);
b.append(", ");
b.append("deviceModel=");
b.append(this.deviceModel);
b.append(", ");
b.append("installStatus=");
b.append(this.installStatus);
b.append(", ");
b.append("lastReportedDateTime=");
b.append(this.lastReportedDateTime);
b.append(", ");
b.append("osVersion=");
b.append(this.osVersion);
b.append(", ");
b.append("platform=");
b.append(this.platform);
b.append(", ");
b.append("status=");
b.append(this.status);
b.append(", ");
b.append("userId=");
b.append(this.userId);
b.append(", ");
b.append("userName=");
b.append(this.userName);
b.append(", ");
b.append("userPrincipalName=");
b.append(this.userPrincipalName);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}