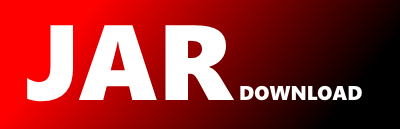
odata.msgraph.client.beta.entity.IosVpnConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.complex.AppListItem;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleDeviceMode;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsEdition;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsVersion;
import odata.msgraph.client.beta.complex.KeyValue;
import odata.msgraph.client.beta.complex.KeyValuePair;
import odata.msgraph.client.beta.complex.VpnOnDemandRule;
import odata.msgraph.client.beta.complex.VpnProxyServer;
import odata.msgraph.client.beta.complex.VpnServer;
import odata.msgraph.client.beta.entity.request.DeviceManagementDerivedCredentialSettingsRequest;
import odata.msgraph.client.beta.entity.request.IosCertificateProfileBaseRequest;
import odata.msgraph.client.beta.enums.AppleVpnConnectionType;
import odata.msgraph.client.beta.enums.VpnAuthenticationMethod;
import odata.msgraph.client.beta.enums.VpnProviderType;
/**
* “By providing the configurations in this profile you can instruct the iOS device
* to connect to desired VPN endpoint. By specifying the authentication method and
* security types expected by VPN endpoint you can make the VPN connection seamless
* for end user.”
*/@JsonPropertyOrder({
"@odata.type",
"cloudName",
"excludeList",
"microsoftTunnelSiteId",
"strictEnforcement",
"targetedMobileApps",
"userDomain"})
@JsonInclude(Include.NON_NULL)
public class IosVpnConfiguration extends AppleVpnConfiguration implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.iosVpnConfiguration";
}
@JsonProperty("cloudName")
protected String cloudName;
@JsonProperty("excludeList")
protected List excludeList;
@JsonProperty("excludeList@nextLink")
protected String excludeListNextLink;
@JsonProperty("microsoftTunnelSiteId")
protected String microsoftTunnelSiteId;
@JsonProperty("strictEnforcement")
protected Boolean strictEnforcement;
@JsonProperty("targetedMobileApps")
protected List targetedMobileApps;
@JsonProperty("targetedMobileApps@nextLink")
protected String targetedMobileAppsNextLink;
@JsonProperty("userDomain")
protected String userDomain;
protected IosVpnConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderIosVpnConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode;
private DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition;
private DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private Boolean supportsScopeTags;
private Integer version;
private List associatedDomains;
private String associatedDomainsNextLink;
private VpnAuthenticationMethod authenticationMethod;
private String connectionName;
private AppleVpnConnectionType connectionType;
private List customData;
private String customDataNextLink;
private List customKeyValueData;
private String customKeyValueDataNextLink;
private Boolean disableOnDemandUserOverride;
private Boolean disconnectOnIdle;
private Integer disconnectOnIdleTimerInSeconds;
private Boolean enablePerApp;
private Boolean enableSplitTunneling;
private List excludedDomains;
private String excludedDomainsNextLink;
private String identifier;
private String loginGroupOrDomain;
private List onDemandRules;
private String onDemandRulesNextLink;
private Boolean optInToDeviceIdSharing;
private VpnProviderType providerType;
private VpnProxyServer proxyServer;
private String realm;
private String role;
private List safariDomains;
private String safariDomainsNextLink;
private VpnServer server;
private String cloudName;
private List excludeList;
private String excludeListNextLink;
private String microsoftTunnelSiteId;
private Boolean strictEnforcement;
private List targetedMobileApps;
private String targetedMobileAppsNextLink;
private String userDomain;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder deviceManagementApplicabilityRuleDeviceMode(DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode) {
this.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleDeviceMode");
return this;
}
public Builder deviceManagementApplicabilityRuleOsEdition(DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition) {
this.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsEdition");
return this;
}
public Builder deviceManagementApplicabilityRuleOsVersion(DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion) {
this.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsVersion");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder supportsScopeTags(Boolean supportsScopeTags) {
this.supportsScopeTags = supportsScopeTags;
this.changedFields = changedFields.add("supportsScopeTags");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
public Builder associatedDomains(List associatedDomains) {
this.associatedDomains = associatedDomains;
this.changedFields = changedFields.add("associatedDomains");
return this;
}
public Builder associatedDomains(String... associatedDomains) {
return associatedDomains(Arrays.asList(associatedDomains));
}
public Builder associatedDomainsNextLink(String associatedDomainsNextLink) {
this.associatedDomainsNextLink = associatedDomainsNextLink;
this.changedFields = changedFields.add("associatedDomains");
return this;
}
public Builder authenticationMethod(VpnAuthenticationMethod authenticationMethod) {
this.authenticationMethod = authenticationMethod;
this.changedFields = changedFields.add("authenticationMethod");
return this;
}
public Builder connectionName(String connectionName) {
this.connectionName = connectionName;
this.changedFields = changedFields.add("connectionName");
return this;
}
public Builder connectionType(AppleVpnConnectionType connectionType) {
this.connectionType = connectionType;
this.changedFields = changedFields.add("connectionType");
return this;
}
public Builder customData(List customData) {
this.customData = customData;
this.changedFields = changedFields.add("customData");
return this;
}
public Builder customData(KeyValue... customData) {
return customData(Arrays.asList(customData));
}
public Builder customDataNextLink(String customDataNextLink) {
this.customDataNextLink = customDataNextLink;
this.changedFields = changedFields.add("customData");
return this;
}
public Builder customKeyValueData(List customKeyValueData) {
this.customKeyValueData = customKeyValueData;
this.changedFields = changedFields.add("customKeyValueData");
return this;
}
public Builder customKeyValueData(KeyValuePair... customKeyValueData) {
return customKeyValueData(Arrays.asList(customKeyValueData));
}
public Builder customKeyValueDataNextLink(String customKeyValueDataNextLink) {
this.customKeyValueDataNextLink = customKeyValueDataNextLink;
this.changedFields = changedFields.add("customKeyValueData");
return this;
}
public Builder disableOnDemandUserOverride(Boolean disableOnDemandUserOverride) {
this.disableOnDemandUserOverride = disableOnDemandUserOverride;
this.changedFields = changedFields.add("disableOnDemandUserOverride");
return this;
}
public Builder disconnectOnIdle(Boolean disconnectOnIdle) {
this.disconnectOnIdle = disconnectOnIdle;
this.changedFields = changedFields.add("disconnectOnIdle");
return this;
}
public Builder disconnectOnIdleTimerInSeconds(Integer disconnectOnIdleTimerInSeconds) {
this.disconnectOnIdleTimerInSeconds = disconnectOnIdleTimerInSeconds;
this.changedFields = changedFields.add("disconnectOnIdleTimerInSeconds");
return this;
}
public Builder enablePerApp(Boolean enablePerApp) {
this.enablePerApp = enablePerApp;
this.changedFields = changedFields.add("enablePerApp");
return this;
}
public Builder enableSplitTunneling(Boolean enableSplitTunneling) {
this.enableSplitTunneling = enableSplitTunneling;
this.changedFields = changedFields.add("enableSplitTunneling");
return this;
}
public Builder excludedDomains(List excludedDomains) {
this.excludedDomains = excludedDomains;
this.changedFields = changedFields.add("excludedDomains");
return this;
}
public Builder excludedDomains(String... excludedDomains) {
return excludedDomains(Arrays.asList(excludedDomains));
}
public Builder excludedDomainsNextLink(String excludedDomainsNextLink) {
this.excludedDomainsNextLink = excludedDomainsNextLink;
this.changedFields = changedFields.add("excludedDomains");
return this;
}
public Builder identifier(String identifier) {
this.identifier = identifier;
this.changedFields = changedFields.add("identifier");
return this;
}
public Builder loginGroupOrDomain(String loginGroupOrDomain) {
this.loginGroupOrDomain = loginGroupOrDomain;
this.changedFields = changedFields.add("loginGroupOrDomain");
return this;
}
public Builder onDemandRules(List onDemandRules) {
this.onDemandRules = onDemandRules;
this.changedFields = changedFields.add("onDemandRules");
return this;
}
public Builder onDemandRules(VpnOnDemandRule... onDemandRules) {
return onDemandRules(Arrays.asList(onDemandRules));
}
public Builder onDemandRulesNextLink(String onDemandRulesNextLink) {
this.onDemandRulesNextLink = onDemandRulesNextLink;
this.changedFields = changedFields.add("onDemandRules");
return this;
}
public Builder optInToDeviceIdSharing(Boolean optInToDeviceIdSharing) {
this.optInToDeviceIdSharing = optInToDeviceIdSharing;
this.changedFields = changedFields.add("optInToDeviceIdSharing");
return this;
}
public Builder providerType(VpnProviderType providerType) {
this.providerType = providerType;
this.changedFields = changedFields.add("providerType");
return this;
}
public Builder proxyServer(VpnProxyServer proxyServer) {
this.proxyServer = proxyServer;
this.changedFields = changedFields.add("proxyServer");
return this;
}
public Builder realm(String realm) {
this.realm = realm;
this.changedFields = changedFields.add("realm");
return this;
}
public Builder role(String role) {
this.role = role;
this.changedFields = changedFields.add("role");
return this;
}
public Builder safariDomains(List safariDomains) {
this.safariDomains = safariDomains;
this.changedFields = changedFields.add("safariDomains");
return this;
}
public Builder safariDomains(String... safariDomains) {
return safariDomains(Arrays.asList(safariDomains));
}
public Builder safariDomainsNextLink(String safariDomainsNextLink) {
this.safariDomainsNextLink = safariDomainsNextLink;
this.changedFields = changedFields.add("safariDomains");
return this;
}
public Builder server(VpnServer server) {
this.server = server;
this.changedFields = changedFields.add("server");
return this;
}
/**
* “Zscaler only. Zscaler cloud which the user is assigned to.”
*
* @param cloudName
* value of {@code cloudName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder cloudName(String cloudName) {
this.cloudName = cloudName;
this.changedFields = changedFields.add("cloudName");
return this;
}
/**
* “Zscaler only. List of network addresses which are not sent through the Zscaler
* cloud.”
*
* @param excludeList
* value of {@code excludeList} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder excludeList(List excludeList) {
this.excludeList = excludeList;
this.changedFields = changedFields.add("excludeList");
return this;
}
/**
* “Zscaler only. List of network addresses which are not sent through the Zscaler
* cloud.”
*
* @param excludeList
* value of {@code excludeList} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder excludeList(String... excludeList) {
return excludeList(Arrays.asList(excludeList));
}
/**
* “Zscaler only. List of network addresses which are not sent through the Zscaler
* cloud.”
*
* @param excludeListNextLink
* value of {@code excludeList@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder excludeListNextLink(String excludeListNextLink) {
this.excludeListNextLink = excludeListNextLink;
this.changedFields = changedFields.add("excludeList");
return this;
}
/**
* “Microsoft Tunnel site ID.”
*
* @param microsoftTunnelSiteId
* value of {@code microsoftTunnelSiteId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder microsoftTunnelSiteId(String microsoftTunnelSiteId) {
this.microsoftTunnelSiteId = microsoftTunnelSiteId;
this.changedFields = changedFields.add("microsoftTunnelSiteId");
return this;
}
/**
* “Zscaler only. Blocks network traffic until the user signs into Zscaler app. "
* True" means traffic is blocked.”
*
* @param strictEnforcement
* value of {@code strictEnforcement} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder strictEnforcement(Boolean strictEnforcement) {
this.strictEnforcement = strictEnforcement;
this.changedFields = changedFields.add("strictEnforcement");
return this;
}
/**
* “Targeted mobile apps. This collection can contain a maximum of 500 elements.”
*
* @param targetedMobileApps
* value of {@code targetedMobileApps} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder targetedMobileApps(List targetedMobileApps) {
this.targetedMobileApps = targetedMobileApps;
this.changedFields = changedFields.add("targetedMobileApps");
return this;
}
/**
* “Targeted mobile apps. This collection can contain a maximum of 500 elements.”
*
* @param targetedMobileApps
* value of {@code targetedMobileApps} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder targetedMobileApps(AppListItem... targetedMobileApps) {
return targetedMobileApps(Arrays.asList(targetedMobileApps));
}
/**
* “Targeted mobile apps. This collection can contain a maximum of 500 elements.”
*
* @param targetedMobileAppsNextLink
* value of {@code targetedMobileApps@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder targetedMobileAppsNextLink(String targetedMobileAppsNextLink) {
this.targetedMobileAppsNextLink = targetedMobileAppsNextLink;
this.changedFields = changedFields.add("targetedMobileApps");
return this;
}
/**
* “Zscaler only. Enter a static domain to pre-populate the login field with in the
* Zscaler app. If this is left empty, the user's Azure Active Directory domain
* will be used instead.”
*
* @param userDomain
* value of {@code userDomain} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userDomain(String userDomain) {
this.userDomain = userDomain;
this.changedFields = changedFields.add("userDomain");
return this;
}
public IosVpnConfiguration build() {
IosVpnConfiguration _x = new IosVpnConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.iosVpnConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.associatedDomains = associatedDomains;
_x.associatedDomainsNextLink = associatedDomainsNextLink;
_x.authenticationMethod = authenticationMethod;
_x.connectionName = connectionName;
_x.connectionType = connectionType;
_x.customData = customData;
_x.customDataNextLink = customDataNextLink;
_x.customKeyValueData = customKeyValueData;
_x.customKeyValueDataNextLink = customKeyValueDataNextLink;
_x.disableOnDemandUserOverride = disableOnDemandUserOverride;
_x.disconnectOnIdle = disconnectOnIdle;
_x.disconnectOnIdleTimerInSeconds = disconnectOnIdleTimerInSeconds;
_x.enablePerApp = enablePerApp;
_x.enableSplitTunneling = enableSplitTunneling;
_x.excludedDomains = excludedDomains;
_x.excludedDomainsNextLink = excludedDomainsNextLink;
_x.identifier = identifier;
_x.loginGroupOrDomain = loginGroupOrDomain;
_x.onDemandRules = onDemandRules;
_x.onDemandRulesNextLink = onDemandRulesNextLink;
_x.optInToDeviceIdSharing = optInToDeviceIdSharing;
_x.providerType = providerType;
_x.proxyServer = proxyServer;
_x.realm = realm;
_x.role = role;
_x.safariDomains = safariDomains;
_x.safariDomainsNextLink = safariDomainsNextLink;
_x.server = server;
_x.cloudName = cloudName;
_x.excludeList = excludeList;
_x.excludeListNextLink = excludeListNextLink;
_x.microsoftTunnelSiteId = microsoftTunnelSiteId;
_x.strictEnforcement = strictEnforcement;
_x.targetedMobileApps = targetedMobileApps;
_x.targetedMobileAppsNextLink = targetedMobileAppsNextLink;
_x.userDomain = userDomain;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Zscaler only. Zscaler cloud which the user is assigned to.”
*
* @return property cloudName
*/
@Property(name="cloudName")
@JsonIgnore
public Optional getCloudName() {
return Optional.ofNullable(cloudName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code cloudName} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Zscaler only. Zscaler cloud which the user is assigned to.”
*
* @param cloudName
* new value of {@code cloudName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code cloudName} field changed
*/
public IosVpnConfiguration withCloudName(String cloudName) {
IosVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("cloudName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosVpnConfiguration");
_x.cloudName = cloudName;
return _x;
}
/**
* “Zscaler only. List of network addresses which are not sent through the Zscaler
* cloud.”
*
* @return property excludeList
*/
@Property(name="excludeList")
@JsonIgnore
public CollectionPage getExcludeList() {
return new CollectionPage(contextPath, String.class, this.excludeList, Optional.ofNullable(excludeListNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code excludeList}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Zscaler only. List of network addresses which are not sent through the Zscaler
* cloud.”
*
* @param excludeList
* new value of {@code excludeList} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code excludeList} field changed
*/
public IosVpnConfiguration withExcludeList(List excludeList) {
IosVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("excludeList");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosVpnConfiguration");
_x.excludeList = excludeList;
return _x;
}
/**
* “Zscaler only. List of network addresses which are not sent through the Zscaler
* cloud.”
*
* @param options
* specify connect and read timeouts
* @return property excludeList
*/
@Property(name="excludeList")
@JsonIgnore
public CollectionPage getExcludeList(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.excludeList, Optional.ofNullable(excludeListNextLink), Collections.emptyList(), options);
}
/**
* “Microsoft Tunnel site ID.”
*
* @return property microsoftTunnelSiteId
*/
@Property(name="microsoftTunnelSiteId")
@JsonIgnore
public Optional getMicrosoftTunnelSiteId() {
return Optional.ofNullable(microsoftTunnelSiteId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* microsoftTunnelSiteId} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Microsoft Tunnel site ID.”
*
* @param microsoftTunnelSiteId
* new value of {@code microsoftTunnelSiteId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code microsoftTunnelSiteId} field changed
*/
public IosVpnConfiguration withMicrosoftTunnelSiteId(String microsoftTunnelSiteId) {
IosVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("microsoftTunnelSiteId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosVpnConfiguration");
_x.microsoftTunnelSiteId = microsoftTunnelSiteId;
return _x;
}
/**
* “Zscaler only. Blocks network traffic until the user signs into Zscaler app. "
* True" means traffic is blocked.”
*
* @return property strictEnforcement
*/
@Property(name="strictEnforcement")
@JsonIgnore
public Optional getStrictEnforcement() {
return Optional.ofNullable(strictEnforcement);
}
/**
* Returns an immutable copy of {@code this} with just the {@code strictEnforcement
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Zscaler only. Blocks network traffic until the user signs into Zscaler app. "
* True" means traffic is blocked.”
*
* @param strictEnforcement
* new value of {@code strictEnforcement} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code strictEnforcement} field changed
*/
public IosVpnConfiguration withStrictEnforcement(Boolean strictEnforcement) {
IosVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("strictEnforcement");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosVpnConfiguration");
_x.strictEnforcement = strictEnforcement;
return _x;
}
/**
* “Targeted mobile apps. This collection can contain a maximum of 500 elements.”
*
* @return property targetedMobileApps
*/
@Property(name="targetedMobileApps")
@JsonIgnore
public CollectionPage getTargetedMobileApps() {
return new CollectionPage(contextPath, AppListItem.class, this.targetedMobileApps, Optional.ofNullable(targetedMobileAppsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* targetedMobileApps} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Targeted mobile apps. This collection can contain a maximum of 500 elements.”
*
* @param targetedMobileApps
* new value of {@code targetedMobileApps} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code targetedMobileApps} field changed
*/
public IosVpnConfiguration withTargetedMobileApps(List targetedMobileApps) {
IosVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("targetedMobileApps");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosVpnConfiguration");
_x.targetedMobileApps = targetedMobileApps;
return _x;
}
/**
* “Targeted mobile apps. This collection can contain a maximum of 500 elements.”
*
* @param options
* specify connect and read timeouts
* @return property targetedMobileApps
*/
@Property(name="targetedMobileApps")
@JsonIgnore
public CollectionPage getTargetedMobileApps(HttpRequestOptions options) {
return new CollectionPage(contextPath, AppListItem.class, this.targetedMobileApps, Optional.ofNullable(targetedMobileAppsNextLink), Collections.emptyList(), options);
}
/**
* “Zscaler only. Enter a static domain to pre-populate the login field with in the
* Zscaler app. If this is left empty, the user's Azure Active Directory domain
* will be used instead.”
*
* @return property userDomain
*/
@Property(name="userDomain")
@JsonIgnore
public Optional getUserDomain() {
return Optional.ofNullable(userDomain);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userDomain} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Zscaler only. Enter a static domain to pre-populate the login field with in the
* Zscaler app. If this is left empty, the user's Azure Active Directory domain
* will be used instead.”
*
* @param userDomain
* new value of {@code userDomain} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userDomain} field changed
*/
public IosVpnConfiguration withUserDomain(String userDomain) {
IosVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("userDomain");
_x.odataType = Util.nvl(odataType, "microsoft.graph.iosVpnConfiguration");
_x.userDomain = userDomain;
return _x;
}
public IosVpnConfiguration withUnmappedField(String name, String value) {
IosVpnConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “Tenant level settings for the Derived Credentials to be used for authentication.”
*
* @return navigational property derivedCredentialSettings
*/
@NavigationProperty(name="derivedCredentialSettings")
@JsonIgnore
public DeviceManagementDerivedCredentialSettingsRequest getDerivedCredentialSettings() {
return new DeviceManagementDerivedCredentialSettingsRequest(contextPath.addSegment("derivedCredentialSettings"), RequestHelper.getValue(unmappedFields, "derivedCredentialSettings"));
}
/**
* “Identity certificate for client authentication when authentication method is
* certificate.”
*
* @return navigational property identityCertificate
*/
@NavigationProperty(name="identityCertificate")
@JsonIgnore
public IosCertificateProfileBaseRequest getIdentityCertificate() {
return new IosCertificateProfileBaseRequest(contextPath.addSegment("identityCertificate"), RequestHelper.getValue(unmappedFields, "identityCertificate"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public IosVpnConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
IosVpnConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public IosVpnConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
IosVpnConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private IosVpnConfiguration _copy() {
IosVpnConfiguration _x = new IosVpnConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.associatedDomains = associatedDomains;
_x.authenticationMethod = authenticationMethod;
_x.connectionName = connectionName;
_x.connectionType = connectionType;
_x.customData = customData;
_x.customKeyValueData = customKeyValueData;
_x.disableOnDemandUserOverride = disableOnDemandUserOverride;
_x.disconnectOnIdle = disconnectOnIdle;
_x.disconnectOnIdleTimerInSeconds = disconnectOnIdleTimerInSeconds;
_x.enablePerApp = enablePerApp;
_x.enableSplitTunneling = enableSplitTunneling;
_x.excludedDomains = excludedDomains;
_x.identifier = identifier;
_x.loginGroupOrDomain = loginGroupOrDomain;
_x.onDemandRules = onDemandRules;
_x.optInToDeviceIdSharing = optInToDeviceIdSharing;
_x.providerType = providerType;
_x.proxyServer = proxyServer;
_x.realm = realm;
_x.role = role;
_x.safariDomains = safariDomains;
_x.server = server;
_x.cloudName = cloudName;
_x.excludeList = excludeList;
_x.microsoftTunnelSiteId = microsoftTunnelSiteId;
_x.strictEnforcement = strictEnforcement;
_x.targetedMobileApps = targetedMobileApps;
_x.userDomain = userDomain;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("IosVpnConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("deviceManagementApplicabilityRuleDeviceMode=");
b.append(this.deviceManagementApplicabilityRuleDeviceMode);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsEdition=");
b.append(this.deviceManagementApplicabilityRuleOsEdition);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsVersion=");
b.append(this.deviceManagementApplicabilityRuleOsVersion);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("supportsScopeTags=");
b.append(this.supportsScopeTags);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("associatedDomains=");
b.append(this.associatedDomains);
b.append(", ");
b.append("authenticationMethod=");
b.append(this.authenticationMethod);
b.append(", ");
b.append("connectionName=");
b.append(this.connectionName);
b.append(", ");
b.append("connectionType=");
b.append(this.connectionType);
b.append(", ");
b.append("customData=");
b.append(this.customData);
b.append(", ");
b.append("customKeyValueData=");
b.append(this.customKeyValueData);
b.append(", ");
b.append("disableOnDemandUserOverride=");
b.append(this.disableOnDemandUserOverride);
b.append(", ");
b.append("disconnectOnIdle=");
b.append(this.disconnectOnIdle);
b.append(", ");
b.append("disconnectOnIdleTimerInSeconds=");
b.append(this.disconnectOnIdleTimerInSeconds);
b.append(", ");
b.append("enablePerApp=");
b.append(this.enablePerApp);
b.append(", ");
b.append("enableSplitTunneling=");
b.append(this.enableSplitTunneling);
b.append(", ");
b.append("excludedDomains=");
b.append(this.excludedDomains);
b.append(", ");
b.append("identifier=");
b.append(this.identifier);
b.append(", ");
b.append("loginGroupOrDomain=");
b.append(this.loginGroupOrDomain);
b.append(", ");
b.append("onDemandRules=");
b.append(this.onDemandRules);
b.append(", ");
b.append("optInToDeviceIdSharing=");
b.append(this.optInToDeviceIdSharing);
b.append(", ");
b.append("providerType=");
b.append(this.providerType);
b.append(", ");
b.append("proxyServer=");
b.append(this.proxyServer);
b.append(", ");
b.append("realm=");
b.append(this.realm);
b.append(", ");
b.append("role=");
b.append(this.role);
b.append(", ");
b.append("safariDomains=");
b.append(this.safariDomains);
b.append(", ");
b.append("server=");
b.append(this.server);
b.append(", ");
b.append("cloudName=");
b.append(this.cloudName);
b.append(", ");
b.append("excludeList=");
b.append(this.excludeList);
b.append(", ");
b.append("microsoftTunnelSiteId=");
b.append(this.microsoftTunnelSiteId);
b.append(", ");
b.append("strictEnforcement=");
b.append(this.strictEnforcement);
b.append(", ");
b.append("targetedMobileApps=");
b.append(this.targetedMobileApps);
b.append(", ");
b.append("userDomain=");
b.append(this.userDomain);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}