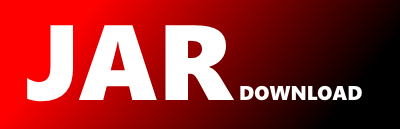
odata.msgraph.client.beta.entity.MacOSCompliancePolicy Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.enums.DeviceThreatProtectionLevel;
import odata.msgraph.client.beta.enums.MacOSGatekeeperAppSources;
import odata.msgraph.client.beta.enums.RequiredPasswordType;
/**
* “This class contains compliance settings for Mac OS.”
*/@JsonPropertyOrder({
"@odata.type",
"advancedThreatProtectionRequiredSecurityLevel",
"deviceThreatProtectionEnabled",
"deviceThreatProtectionRequiredSecurityLevel",
"firewallBlockAllIncoming",
"firewallEnabled",
"firewallEnableStealthMode",
"gatekeeperAllowedAppSource",
"osMaximumBuildVersion",
"osMaximumVersion",
"osMinimumBuildVersion",
"osMinimumVersion",
"passwordBlockSimple",
"passwordExpirationDays",
"passwordMinimumCharacterSetCount",
"passwordMinimumLength",
"passwordMinutesOfInactivityBeforeLock",
"passwordPreviousPasswordBlockCount",
"passwordRequired",
"passwordRequiredType",
"storageRequireEncryption",
"systemIntegrityProtectionEnabled"})
@JsonInclude(Include.NON_NULL)
public class MacOSCompliancePolicy extends DeviceCompliancePolicy implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.macOSCompliancePolicy";
}
@JsonProperty("advancedThreatProtectionRequiredSecurityLevel")
protected DeviceThreatProtectionLevel advancedThreatProtectionRequiredSecurityLevel;
@JsonProperty("deviceThreatProtectionEnabled")
protected Boolean deviceThreatProtectionEnabled;
@JsonProperty("deviceThreatProtectionRequiredSecurityLevel")
protected DeviceThreatProtectionLevel deviceThreatProtectionRequiredSecurityLevel;
@JsonProperty("firewallBlockAllIncoming")
protected Boolean firewallBlockAllIncoming;
@JsonProperty("firewallEnabled")
protected Boolean firewallEnabled;
@JsonProperty("firewallEnableStealthMode")
protected Boolean firewallEnableStealthMode;
@JsonProperty("gatekeeperAllowedAppSource")
protected MacOSGatekeeperAppSources gatekeeperAllowedAppSource;
@JsonProperty("osMaximumBuildVersion")
protected String osMaximumBuildVersion;
@JsonProperty("osMaximumVersion")
protected String osMaximumVersion;
@JsonProperty("osMinimumBuildVersion")
protected String osMinimumBuildVersion;
@JsonProperty("osMinimumVersion")
protected String osMinimumVersion;
@JsonProperty("passwordBlockSimple")
protected Boolean passwordBlockSimple;
@JsonProperty("passwordExpirationDays")
protected Integer passwordExpirationDays;
@JsonProperty("passwordMinimumCharacterSetCount")
protected Integer passwordMinimumCharacterSetCount;
@JsonProperty("passwordMinimumLength")
protected Integer passwordMinimumLength;
@JsonProperty("passwordMinutesOfInactivityBeforeLock")
protected Integer passwordMinutesOfInactivityBeforeLock;
@JsonProperty("passwordPreviousPasswordBlockCount")
protected Integer passwordPreviousPasswordBlockCount;
@JsonProperty("passwordRequired")
protected Boolean passwordRequired;
@JsonProperty("passwordRequiredType")
protected RequiredPasswordType passwordRequiredType;
@JsonProperty("storageRequireEncryption")
protected Boolean storageRequireEncryption;
@JsonProperty("systemIntegrityProtectionEnabled")
protected Boolean systemIntegrityProtectionEnabled;
protected MacOSCompliancePolicy() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderMacOSCompliancePolicy() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private Integer version;
private DeviceThreatProtectionLevel advancedThreatProtectionRequiredSecurityLevel;
private Boolean deviceThreatProtectionEnabled;
private DeviceThreatProtectionLevel deviceThreatProtectionRequiredSecurityLevel;
private Boolean firewallBlockAllIncoming;
private Boolean firewallEnabled;
private Boolean firewallEnableStealthMode;
private MacOSGatekeeperAppSources gatekeeperAllowedAppSource;
private String osMaximumBuildVersion;
private String osMaximumVersion;
private String osMinimumBuildVersion;
private String osMinimumVersion;
private Boolean passwordBlockSimple;
private Integer passwordExpirationDays;
private Integer passwordMinimumCharacterSetCount;
private Integer passwordMinimumLength;
private Integer passwordMinutesOfInactivityBeforeLock;
private Integer passwordPreviousPasswordBlockCount;
private Boolean passwordRequired;
private RequiredPasswordType passwordRequiredType;
private Boolean storageRequireEncryption;
private Boolean systemIntegrityProtectionEnabled;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
/**
* “MDATP Require Mobile Threat Protection minimum risk level to report
* noncompliance.”
*
* @param advancedThreatProtectionRequiredSecurityLevel
* value of {@code advancedThreatProtectionRequiredSecurityLevel} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionRequiredSecurityLevel(DeviceThreatProtectionLevel advancedThreatProtectionRequiredSecurityLevel) {
this.advancedThreatProtectionRequiredSecurityLevel = advancedThreatProtectionRequiredSecurityLevel;
this.changedFields = changedFields.add("advancedThreatProtectionRequiredSecurityLevel");
return this;
}
/**
* “Require that devices have enabled device threat protection.”
*
* @param deviceThreatProtectionEnabled
* value of {@code deviceThreatProtectionEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceThreatProtectionEnabled(Boolean deviceThreatProtectionEnabled) {
this.deviceThreatProtectionEnabled = deviceThreatProtectionEnabled;
this.changedFields = changedFields.add("deviceThreatProtectionEnabled");
return this;
}
/**
* “Require Mobile Threat Protection minimum risk level to report noncompliance.”
*
* @param deviceThreatProtectionRequiredSecurityLevel
* value of {@code deviceThreatProtectionRequiredSecurityLevel} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceThreatProtectionRequiredSecurityLevel(DeviceThreatProtectionLevel deviceThreatProtectionRequiredSecurityLevel) {
this.deviceThreatProtectionRequiredSecurityLevel = deviceThreatProtectionRequiredSecurityLevel;
this.changedFields = changedFields.add("deviceThreatProtectionRequiredSecurityLevel");
return this;
}
/**
* “Corresponds to the “Block all incoming connections” option.”
*
* @param firewallBlockAllIncoming
* value of {@code firewallBlockAllIncoming} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder firewallBlockAllIncoming(Boolean firewallBlockAllIncoming) {
this.firewallBlockAllIncoming = firewallBlockAllIncoming;
this.changedFields = changedFields.add("firewallBlockAllIncoming");
return this;
}
/**
* “Whether the firewall should be enabled or not.”
*
* @param firewallEnabled
* value of {@code firewallEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder firewallEnabled(Boolean firewallEnabled) {
this.firewallEnabled = firewallEnabled;
this.changedFields = changedFields.add("firewallEnabled");
return this;
}
/**
* “Corresponds to “Enable stealth mode.””
*
* @param firewallEnableStealthMode
* value of {@code firewallEnableStealthMode} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder firewallEnableStealthMode(Boolean firewallEnableStealthMode) {
this.firewallEnableStealthMode = firewallEnableStealthMode;
this.changedFields = changedFields.add("firewallEnableStealthMode");
return this;
}
/**
* “System and Privacy setting that determines which download locations apps can be
* run from on a macOS device.”
*
* @param gatekeeperAllowedAppSource
* value of {@code gatekeeperAllowedAppSource} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder gatekeeperAllowedAppSource(MacOSGatekeeperAppSources gatekeeperAllowedAppSource) {
this.gatekeeperAllowedAppSource = gatekeeperAllowedAppSource;
this.changedFields = changedFields.add("gatekeeperAllowedAppSource");
return this;
}
/**
* “Maximum MacOS build version.”
*
* @param osMaximumBuildVersion
* value of {@code osMaximumBuildVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder osMaximumBuildVersion(String osMaximumBuildVersion) {
this.osMaximumBuildVersion = osMaximumBuildVersion;
this.changedFields = changedFields.add("osMaximumBuildVersion");
return this;
}
/**
* “Maximum MacOS version.”
*
* @param osMaximumVersion
* value of {@code osMaximumVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder osMaximumVersion(String osMaximumVersion) {
this.osMaximumVersion = osMaximumVersion;
this.changedFields = changedFields.add("osMaximumVersion");
return this;
}
/**
* “Minimum MacOS build version.”
*
* @param osMinimumBuildVersion
* value of {@code osMinimumBuildVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder osMinimumBuildVersion(String osMinimumBuildVersion) {
this.osMinimumBuildVersion = osMinimumBuildVersion;
this.changedFields = changedFields.add("osMinimumBuildVersion");
return this;
}
/**
* “Minimum MacOS version.”
*
* @param osMinimumVersion
* value of {@code osMinimumVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder osMinimumVersion(String osMinimumVersion) {
this.osMinimumVersion = osMinimumVersion;
this.changedFields = changedFields.add("osMinimumVersion");
return this;
}
/**
* “Indicates whether or not to block simple passwords.”
*
* @param passwordBlockSimple
* value of {@code passwordBlockSimple} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordBlockSimple(Boolean passwordBlockSimple) {
this.passwordBlockSimple = passwordBlockSimple;
this.changedFields = changedFields.add("passwordBlockSimple");
return this;
}
/**
* “Number of days before the password expires. Valid values 1 to 65535”
*
* @param passwordExpirationDays
* value of {@code passwordExpirationDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordExpirationDays(Integer passwordExpirationDays) {
this.passwordExpirationDays = passwordExpirationDays;
this.changedFields = changedFields.add("passwordExpirationDays");
return this;
}
/**
* “The number of character sets required in the password.”
*
* @param passwordMinimumCharacterSetCount
* value of {@code passwordMinimumCharacterSetCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordMinimumCharacterSetCount(Integer passwordMinimumCharacterSetCount) {
this.passwordMinimumCharacterSetCount = passwordMinimumCharacterSetCount;
this.changedFields = changedFields.add("passwordMinimumCharacterSetCount");
return this;
}
/**
* “Minimum length of password. Valid values 4 to 14”
*
* @param passwordMinimumLength
* value of {@code passwordMinimumLength} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordMinimumLength(Integer passwordMinimumLength) {
this.passwordMinimumLength = passwordMinimumLength;
this.changedFields = changedFields.add("passwordMinimumLength");
return this;
}
/**
* “Minutes of inactivity before a password is required.”
*
* @param passwordMinutesOfInactivityBeforeLock
* value of {@code passwordMinutesOfInactivityBeforeLock} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordMinutesOfInactivityBeforeLock(Integer passwordMinutesOfInactivityBeforeLock) {
this.passwordMinutesOfInactivityBeforeLock = passwordMinutesOfInactivityBeforeLock;
this.changedFields = changedFields.add("passwordMinutesOfInactivityBeforeLock");
return this;
}
/**
* “Number of previous passwords to block. Valid values 1 to 24”
*
* @param passwordPreviousPasswordBlockCount
* value of {@code passwordPreviousPasswordBlockCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordPreviousPasswordBlockCount(Integer passwordPreviousPasswordBlockCount) {
this.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
this.changedFields = changedFields.add("passwordPreviousPasswordBlockCount");
return this;
}
/**
* “Whether or not to require a password.”
*
* @param passwordRequired
* value of {@code passwordRequired} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordRequired(Boolean passwordRequired) {
this.passwordRequired = passwordRequired;
this.changedFields = changedFields.add("passwordRequired");
return this;
}
/**
* “The required password type.”
*
* @param passwordRequiredType
* value of {@code passwordRequiredType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordRequiredType(RequiredPasswordType passwordRequiredType) {
this.passwordRequiredType = passwordRequiredType;
this.changedFields = changedFields.add("passwordRequiredType");
return this;
}
/**
* “Require encryption on Mac OS devices.”
*
* @param storageRequireEncryption
* value of {@code storageRequireEncryption} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder storageRequireEncryption(Boolean storageRequireEncryption) {
this.storageRequireEncryption = storageRequireEncryption;
this.changedFields = changedFields.add("storageRequireEncryption");
return this;
}
/**
* “Require that devices have enabled system integrity protection.”
*
* @param systemIntegrityProtectionEnabled
* value of {@code systemIntegrityProtectionEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder systemIntegrityProtectionEnabled(Boolean systemIntegrityProtectionEnabled) {
this.systemIntegrityProtectionEnabled = systemIntegrityProtectionEnabled;
this.changedFields = changedFields.add("systemIntegrityProtectionEnabled");
return this;
}
public MacOSCompliancePolicy build() {
MacOSCompliancePolicy _x = new MacOSCompliancePolicy();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.macOSCompliancePolicy";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
_x.version = version;
_x.advancedThreatProtectionRequiredSecurityLevel = advancedThreatProtectionRequiredSecurityLevel;
_x.deviceThreatProtectionEnabled = deviceThreatProtectionEnabled;
_x.deviceThreatProtectionRequiredSecurityLevel = deviceThreatProtectionRequiredSecurityLevel;
_x.firewallBlockAllIncoming = firewallBlockAllIncoming;
_x.firewallEnabled = firewallEnabled;
_x.firewallEnableStealthMode = firewallEnableStealthMode;
_x.gatekeeperAllowedAppSource = gatekeeperAllowedAppSource;
_x.osMaximumBuildVersion = osMaximumBuildVersion;
_x.osMaximumVersion = osMaximumVersion;
_x.osMinimumBuildVersion = osMinimumBuildVersion;
_x.osMinimumVersion = osMinimumVersion;
_x.passwordBlockSimple = passwordBlockSimple;
_x.passwordExpirationDays = passwordExpirationDays;
_x.passwordMinimumCharacterSetCount = passwordMinimumCharacterSetCount;
_x.passwordMinimumLength = passwordMinimumLength;
_x.passwordMinutesOfInactivityBeforeLock = passwordMinutesOfInactivityBeforeLock;
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
_x.passwordRequired = passwordRequired;
_x.passwordRequiredType = passwordRequiredType;
_x.storageRequireEncryption = storageRequireEncryption;
_x.systemIntegrityProtectionEnabled = systemIntegrityProtectionEnabled;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “MDATP Require Mobile Threat Protection minimum risk level to report
* noncompliance.”
*
* @return property advancedThreatProtectionRequiredSecurityLevel
*/
@Property(name="advancedThreatProtectionRequiredSecurityLevel")
@JsonIgnore
public Optional getAdvancedThreatProtectionRequiredSecurityLevel() {
return Optional.ofNullable(advancedThreatProtectionRequiredSecurityLevel);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* advancedThreatProtectionRequiredSecurityLevel} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “MDATP Require Mobile Threat Protection minimum risk level to report
* noncompliance.”
*
* @param advancedThreatProtectionRequiredSecurityLevel
* new value of {@code advancedThreatProtectionRequiredSecurityLevel} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code advancedThreatProtectionRequiredSecurityLevel} field changed
*/
public MacOSCompliancePolicy withAdvancedThreatProtectionRequiredSecurityLevel(DeviceThreatProtectionLevel advancedThreatProtectionRequiredSecurityLevel) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("advancedThreatProtectionRequiredSecurityLevel");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.advancedThreatProtectionRequiredSecurityLevel = advancedThreatProtectionRequiredSecurityLevel;
return _x;
}
/**
* “Require that devices have enabled device threat protection.”
*
* @return property deviceThreatProtectionEnabled
*/
@Property(name="deviceThreatProtectionEnabled")
@JsonIgnore
public Optional getDeviceThreatProtectionEnabled() {
return Optional.ofNullable(deviceThreatProtectionEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceThreatProtectionEnabled} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Require that devices have enabled device threat protection.”
*
* @param deviceThreatProtectionEnabled
* new value of {@code deviceThreatProtectionEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceThreatProtectionEnabled} field changed
*/
public MacOSCompliancePolicy withDeviceThreatProtectionEnabled(Boolean deviceThreatProtectionEnabled) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("deviceThreatProtectionEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.deviceThreatProtectionEnabled = deviceThreatProtectionEnabled;
return _x;
}
/**
* “Require Mobile Threat Protection minimum risk level to report noncompliance.”
*
* @return property deviceThreatProtectionRequiredSecurityLevel
*/
@Property(name="deviceThreatProtectionRequiredSecurityLevel")
@JsonIgnore
public Optional getDeviceThreatProtectionRequiredSecurityLevel() {
return Optional.ofNullable(deviceThreatProtectionRequiredSecurityLevel);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceThreatProtectionRequiredSecurityLevel} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Require Mobile Threat Protection minimum risk level to report noncompliance.”
*
* @param deviceThreatProtectionRequiredSecurityLevel
* new value of {@code deviceThreatProtectionRequiredSecurityLevel} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceThreatProtectionRequiredSecurityLevel} field changed
*/
public MacOSCompliancePolicy withDeviceThreatProtectionRequiredSecurityLevel(DeviceThreatProtectionLevel deviceThreatProtectionRequiredSecurityLevel) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("deviceThreatProtectionRequiredSecurityLevel");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.deviceThreatProtectionRequiredSecurityLevel = deviceThreatProtectionRequiredSecurityLevel;
return _x;
}
/**
* “Corresponds to the “Block all incoming connections” option.”
*
* @return property firewallBlockAllIncoming
*/
@Property(name="firewallBlockAllIncoming")
@JsonIgnore
public Optional getFirewallBlockAllIncoming() {
return Optional.ofNullable(firewallBlockAllIncoming);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* firewallBlockAllIncoming} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Corresponds to the “Block all incoming connections” option.”
*
* @param firewallBlockAllIncoming
* new value of {@code firewallBlockAllIncoming} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code firewallBlockAllIncoming} field changed
*/
public MacOSCompliancePolicy withFirewallBlockAllIncoming(Boolean firewallBlockAllIncoming) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("firewallBlockAllIncoming");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.firewallBlockAllIncoming = firewallBlockAllIncoming;
return _x;
}
/**
* “Whether the firewall should be enabled or not.”
*
* @return property firewallEnabled
*/
@Property(name="firewallEnabled")
@JsonIgnore
public Optional getFirewallEnabled() {
return Optional.ofNullable(firewallEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code firewallEnabled}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Whether the firewall should be enabled or not.”
*
* @param firewallEnabled
* new value of {@code firewallEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code firewallEnabled} field changed
*/
public MacOSCompliancePolicy withFirewallEnabled(Boolean firewallEnabled) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("firewallEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.firewallEnabled = firewallEnabled;
return _x;
}
/**
* “Corresponds to “Enable stealth mode.””
*
* @return property firewallEnableStealthMode
*/
@Property(name="firewallEnableStealthMode")
@JsonIgnore
public Optional getFirewallEnableStealthMode() {
return Optional.ofNullable(firewallEnableStealthMode);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* firewallEnableStealthMode} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Corresponds to “Enable stealth mode.””
*
* @param firewallEnableStealthMode
* new value of {@code firewallEnableStealthMode} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code firewallEnableStealthMode} field changed
*/
public MacOSCompliancePolicy withFirewallEnableStealthMode(Boolean firewallEnableStealthMode) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("firewallEnableStealthMode");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.firewallEnableStealthMode = firewallEnableStealthMode;
return _x;
}
/**
* “System and Privacy setting that determines which download locations apps can be
* run from on a macOS device.”
*
* @return property gatekeeperAllowedAppSource
*/
@Property(name="gatekeeperAllowedAppSource")
@JsonIgnore
public Optional getGatekeeperAllowedAppSource() {
return Optional.ofNullable(gatekeeperAllowedAppSource);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* gatekeeperAllowedAppSource} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “System and Privacy setting that determines which download locations apps can be
* run from on a macOS device.”
*
* @param gatekeeperAllowedAppSource
* new value of {@code gatekeeperAllowedAppSource} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code gatekeeperAllowedAppSource} field changed
*/
public MacOSCompliancePolicy withGatekeeperAllowedAppSource(MacOSGatekeeperAppSources gatekeeperAllowedAppSource) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("gatekeeperAllowedAppSource");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.gatekeeperAllowedAppSource = gatekeeperAllowedAppSource;
return _x;
}
/**
* “Maximum MacOS build version.”
*
* @return property osMaximumBuildVersion
*/
@Property(name="osMaximumBuildVersion")
@JsonIgnore
public Optional getOsMaximumBuildVersion() {
return Optional.ofNullable(osMaximumBuildVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* osMaximumBuildVersion} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Maximum MacOS build version.”
*
* @param osMaximumBuildVersion
* new value of {@code osMaximumBuildVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code osMaximumBuildVersion} field changed
*/
public MacOSCompliancePolicy withOsMaximumBuildVersion(String osMaximumBuildVersion) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("osMaximumBuildVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.osMaximumBuildVersion = osMaximumBuildVersion;
return _x;
}
/**
* “Maximum MacOS version.”
*
* @return property osMaximumVersion
*/
@Property(name="osMaximumVersion")
@JsonIgnore
public Optional getOsMaximumVersion() {
return Optional.ofNullable(osMaximumVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code osMaximumVersion}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Maximum MacOS version.”
*
* @param osMaximumVersion
* new value of {@code osMaximumVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code osMaximumVersion} field changed
*/
public MacOSCompliancePolicy withOsMaximumVersion(String osMaximumVersion) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("osMaximumVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.osMaximumVersion = osMaximumVersion;
return _x;
}
/**
* “Minimum MacOS build version.”
*
* @return property osMinimumBuildVersion
*/
@Property(name="osMinimumBuildVersion")
@JsonIgnore
public Optional getOsMinimumBuildVersion() {
return Optional.ofNullable(osMinimumBuildVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* osMinimumBuildVersion} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Minimum MacOS build version.”
*
* @param osMinimumBuildVersion
* new value of {@code osMinimumBuildVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code osMinimumBuildVersion} field changed
*/
public MacOSCompliancePolicy withOsMinimumBuildVersion(String osMinimumBuildVersion) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("osMinimumBuildVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.osMinimumBuildVersion = osMinimumBuildVersion;
return _x;
}
/**
* “Minimum MacOS version.”
*
* @return property osMinimumVersion
*/
@Property(name="osMinimumVersion")
@JsonIgnore
public Optional getOsMinimumVersion() {
return Optional.ofNullable(osMinimumVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code osMinimumVersion}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Minimum MacOS version.”
*
* @param osMinimumVersion
* new value of {@code osMinimumVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code osMinimumVersion} field changed
*/
public MacOSCompliancePolicy withOsMinimumVersion(String osMinimumVersion) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("osMinimumVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.osMinimumVersion = osMinimumVersion;
return _x;
}
/**
* “Indicates whether or not to block simple passwords.”
*
* @return property passwordBlockSimple
*/
@Property(name="passwordBlockSimple")
@JsonIgnore
public Optional getPasswordBlockSimple() {
return Optional.ofNullable(passwordBlockSimple);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordBlockSimple} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Indicates whether or not to block simple passwords.”
*
* @param passwordBlockSimple
* new value of {@code passwordBlockSimple} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordBlockSimple} field changed
*/
public MacOSCompliancePolicy withPasswordBlockSimple(Boolean passwordBlockSimple) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordBlockSimple");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.passwordBlockSimple = passwordBlockSimple;
return _x;
}
/**
* “Number of days before the password expires. Valid values 1 to 65535”
*
* @return property passwordExpirationDays
*/
@Property(name="passwordExpirationDays")
@JsonIgnore
public Optional getPasswordExpirationDays() {
return Optional.ofNullable(passwordExpirationDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordExpirationDays} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Number of days before the password expires. Valid values 1 to 65535”
*
* @param passwordExpirationDays
* new value of {@code passwordExpirationDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordExpirationDays} field changed
*/
public MacOSCompliancePolicy withPasswordExpirationDays(Integer passwordExpirationDays) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordExpirationDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.passwordExpirationDays = passwordExpirationDays;
return _x;
}
/**
* “The number of character sets required in the password.”
*
* @return property passwordMinimumCharacterSetCount
*/
@Property(name="passwordMinimumCharacterSetCount")
@JsonIgnore
public Optional getPasswordMinimumCharacterSetCount() {
return Optional.ofNullable(passwordMinimumCharacterSetCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordMinimumCharacterSetCount} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The number of character sets required in the password.”
*
* @param passwordMinimumCharacterSetCount
* new value of {@code passwordMinimumCharacterSetCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordMinimumCharacterSetCount} field changed
*/
public MacOSCompliancePolicy withPasswordMinimumCharacterSetCount(Integer passwordMinimumCharacterSetCount) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordMinimumCharacterSetCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.passwordMinimumCharacterSetCount = passwordMinimumCharacterSetCount;
return _x;
}
/**
* “Minimum length of password. Valid values 4 to 14”
*
* @return property passwordMinimumLength
*/
@Property(name="passwordMinimumLength")
@JsonIgnore
public Optional getPasswordMinimumLength() {
return Optional.ofNullable(passwordMinimumLength);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordMinimumLength} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Minimum length of password. Valid values 4 to 14”
*
* @param passwordMinimumLength
* new value of {@code passwordMinimumLength} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordMinimumLength} field changed
*/
public MacOSCompliancePolicy withPasswordMinimumLength(Integer passwordMinimumLength) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordMinimumLength");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.passwordMinimumLength = passwordMinimumLength;
return _x;
}
/**
* “Minutes of inactivity before a password is required.”
*
* @return property passwordMinutesOfInactivityBeforeLock
*/
@Property(name="passwordMinutesOfInactivityBeforeLock")
@JsonIgnore
public Optional getPasswordMinutesOfInactivityBeforeLock() {
return Optional.ofNullable(passwordMinutesOfInactivityBeforeLock);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordMinutesOfInactivityBeforeLock} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Minutes of inactivity before a password is required.”
*
* @param passwordMinutesOfInactivityBeforeLock
* new value of {@code passwordMinutesOfInactivityBeforeLock} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordMinutesOfInactivityBeforeLock} field changed
*/
public MacOSCompliancePolicy withPasswordMinutesOfInactivityBeforeLock(Integer passwordMinutesOfInactivityBeforeLock) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordMinutesOfInactivityBeforeLock");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.passwordMinutesOfInactivityBeforeLock = passwordMinutesOfInactivityBeforeLock;
return _x;
}
/**
* “Number of previous passwords to block. Valid values 1 to 24”
*
* @return property passwordPreviousPasswordBlockCount
*/
@Property(name="passwordPreviousPasswordBlockCount")
@JsonIgnore
public Optional getPasswordPreviousPasswordBlockCount() {
return Optional.ofNullable(passwordPreviousPasswordBlockCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordPreviousPasswordBlockCount} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Number of previous passwords to block. Valid values 1 to 24”
*
* @param passwordPreviousPasswordBlockCount
* new value of {@code passwordPreviousPasswordBlockCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordPreviousPasswordBlockCount} field changed
*/
public MacOSCompliancePolicy withPasswordPreviousPasswordBlockCount(Integer passwordPreviousPasswordBlockCount) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordPreviousPasswordBlockCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
return _x;
}
/**
* “Whether or not to require a password.”
*
* @return property passwordRequired
*/
@Property(name="passwordRequired")
@JsonIgnore
public Optional getPasswordRequired() {
return Optional.ofNullable(passwordRequired);
}
/**
* Returns an immutable copy of {@code this} with just the {@code passwordRequired}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Whether or not to require a password.”
*
* @param passwordRequired
* new value of {@code passwordRequired} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordRequired} field changed
*/
public MacOSCompliancePolicy withPasswordRequired(Boolean passwordRequired) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordRequired");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.passwordRequired = passwordRequired;
return _x;
}
/**
* “The required password type.”
*
* @return property passwordRequiredType
*/
@Property(name="passwordRequiredType")
@JsonIgnore
public Optional getPasswordRequiredType() {
return Optional.ofNullable(passwordRequiredType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordRequiredType} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The required password type.”
*
* @param passwordRequiredType
* new value of {@code passwordRequiredType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordRequiredType} field changed
*/
public MacOSCompliancePolicy withPasswordRequiredType(RequiredPasswordType passwordRequiredType) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordRequiredType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.passwordRequiredType = passwordRequiredType;
return _x;
}
/**
* “Require encryption on Mac OS devices.”
*
* @return property storageRequireEncryption
*/
@Property(name="storageRequireEncryption")
@JsonIgnore
public Optional getStorageRequireEncryption() {
return Optional.ofNullable(storageRequireEncryption);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* storageRequireEncryption} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Require encryption on Mac OS devices.”
*
* @param storageRequireEncryption
* new value of {@code storageRequireEncryption} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code storageRequireEncryption} field changed
*/
public MacOSCompliancePolicy withStorageRequireEncryption(Boolean storageRequireEncryption) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("storageRequireEncryption");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.storageRequireEncryption = storageRequireEncryption;
return _x;
}
/**
* “Require that devices have enabled system integrity protection.”
*
* @return property systemIntegrityProtectionEnabled
*/
@Property(name="systemIntegrityProtectionEnabled")
@JsonIgnore
public Optional getSystemIntegrityProtectionEnabled() {
return Optional.ofNullable(systemIntegrityProtectionEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* systemIntegrityProtectionEnabled} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Require that devices have enabled system integrity protection.”
*
* @param systemIntegrityProtectionEnabled
* new value of {@code systemIntegrityProtectionEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code systemIntegrityProtectionEnabled} field changed
*/
public MacOSCompliancePolicy withSystemIntegrityProtectionEnabled(Boolean systemIntegrityProtectionEnabled) {
MacOSCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("systemIntegrityProtectionEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSCompliancePolicy");
_x.systemIntegrityProtectionEnabled = systemIntegrityProtectionEnabled;
return _x;
}
public MacOSCompliancePolicy withUnmappedField(String name, String value) {
MacOSCompliancePolicy _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public MacOSCompliancePolicy patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
MacOSCompliancePolicy _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public MacOSCompliancePolicy put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
MacOSCompliancePolicy _x = _copy();
_x.changedFields = null;
return _x;
}
private MacOSCompliancePolicy _copy() {
MacOSCompliancePolicy _x = new MacOSCompliancePolicy();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.version = version;
_x.advancedThreatProtectionRequiredSecurityLevel = advancedThreatProtectionRequiredSecurityLevel;
_x.deviceThreatProtectionEnabled = deviceThreatProtectionEnabled;
_x.deviceThreatProtectionRequiredSecurityLevel = deviceThreatProtectionRequiredSecurityLevel;
_x.firewallBlockAllIncoming = firewallBlockAllIncoming;
_x.firewallEnabled = firewallEnabled;
_x.firewallEnableStealthMode = firewallEnableStealthMode;
_x.gatekeeperAllowedAppSource = gatekeeperAllowedAppSource;
_x.osMaximumBuildVersion = osMaximumBuildVersion;
_x.osMaximumVersion = osMaximumVersion;
_x.osMinimumBuildVersion = osMinimumBuildVersion;
_x.osMinimumVersion = osMinimumVersion;
_x.passwordBlockSimple = passwordBlockSimple;
_x.passwordExpirationDays = passwordExpirationDays;
_x.passwordMinimumCharacterSetCount = passwordMinimumCharacterSetCount;
_x.passwordMinimumLength = passwordMinimumLength;
_x.passwordMinutesOfInactivityBeforeLock = passwordMinutesOfInactivityBeforeLock;
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
_x.passwordRequired = passwordRequired;
_x.passwordRequiredType = passwordRequiredType;
_x.storageRequireEncryption = storageRequireEncryption;
_x.systemIntegrityProtectionEnabled = systemIntegrityProtectionEnabled;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("MacOSCompliancePolicy[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("advancedThreatProtectionRequiredSecurityLevel=");
b.append(this.advancedThreatProtectionRequiredSecurityLevel);
b.append(", ");
b.append("deviceThreatProtectionEnabled=");
b.append(this.deviceThreatProtectionEnabled);
b.append(", ");
b.append("deviceThreatProtectionRequiredSecurityLevel=");
b.append(this.deviceThreatProtectionRequiredSecurityLevel);
b.append(", ");
b.append("firewallBlockAllIncoming=");
b.append(this.firewallBlockAllIncoming);
b.append(", ");
b.append("firewallEnabled=");
b.append(this.firewallEnabled);
b.append(", ");
b.append("firewallEnableStealthMode=");
b.append(this.firewallEnableStealthMode);
b.append(", ");
b.append("gatekeeperAllowedAppSource=");
b.append(this.gatekeeperAllowedAppSource);
b.append(", ");
b.append("osMaximumBuildVersion=");
b.append(this.osMaximumBuildVersion);
b.append(", ");
b.append("osMaximumVersion=");
b.append(this.osMaximumVersion);
b.append(", ");
b.append("osMinimumBuildVersion=");
b.append(this.osMinimumBuildVersion);
b.append(", ");
b.append("osMinimumVersion=");
b.append(this.osMinimumVersion);
b.append(", ");
b.append("passwordBlockSimple=");
b.append(this.passwordBlockSimple);
b.append(", ");
b.append("passwordExpirationDays=");
b.append(this.passwordExpirationDays);
b.append(", ");
b.append("passwordMinimumCharacterSetCount=");
b.append(this.passwordMinimumCharacterSetCount);
b.append(", ");
b.append("passwordMinimumLength=");
b.append(this.passwordMinimumLength);
b.append(", ");
b.append("passwordMinutesOfInactivityBeforeLock=");
b.append(this.passwordMinutesOfInactivityBeforeLock);
b.append(", ");
b.append("passwordPreviousPasswordBlockCount=");
b.append(this.passwordPreviousPasswordBlockCount);
b.append(", ");
b.append("passwordRequired=");
b.append(this.passwordRequired);
b.append(", ");
b.append("passwordRequiredType=");
b.append(this.passwordRequiredType);
b.append(", ");
b.append("storageRequireEncryption=");
b.append(this.storageRequireEncryption);
b.append(", ");
b.append("systemIntegrityProtectionEnabled=");
b.append(this.systemIntegrityProtectionEnabled);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}