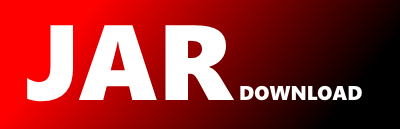
odata.msgraph.client.beta.entity.MacOSDeviceFeaturesConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.complex.AirPrintDestination;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleDeviceMode;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsEdition;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsVersion;
import odata.msgraph.client.beta.complex.IpRange;
import odata.msgraph.client.beta.complex.KeyValuePair;
import odata.msgraph.client.beta.complex.MacOSAssociatedDomainsItem;
import odata.msgraph.client.beta.complex.MacOSLaunchItem;
import odata.msgraph.client.beta.complex.MacOSSingleSignOnExtension;
import odata.msgraph.client.beta.complex.SingleSignOnExtension;
import odata.msgraph.client.beta.entity.request.MacOSCertificateProfileBaseRequest;
import odata.msgraph.client.beta.enums.MacOSContentCachingClientPolicy;
import odata.msgraph.client.beta.enums.MacOSContentCachingParentSelectionPolicy;
import odata.msgraph.client.beta.enums.MacOSContentCachingPeerPolicy;
import odata.msgraph.client.beta.enums.MacOSContentCachingType;
/**
* “MacOS device features configuration profile.”
*/@JsonPropertyOrder({
"@odata.type",
"adminShowHostInfo",
"appAssociatedDomains",
"associatedDomains",
"authorizedUsersListHidden",
"authorizedUsersListHideAdminUsers",
"authorizedUsersListHideLocalUsers",
"authorizedUsersListHideMobileAccounts",
"authorizedUsersListIncludeNetworkUsers",
"authorizedUsersListShowOtherManagedUsers",
"autoLaunchItems",
"consoleAccessDisabled",
"contentCachingBlockDeletion",
"contentCachingClientListenRanges",
"contentCachingClientPolicy",
"contentCachingDataPath",
"contentCachingDisableConnectionSharing",
"contentCachingEnabled",
"contentCachingForceConnectionSharing",
"contentCachingKeepAwake",
"contentCachingLogClientIdentities",
"contentCachingMaxSizeBytes",
"contentCachingParents",
"contentCachingParentSelectionPolicy",
"contentCachingPeerFilterRanges",
"contentCachingPeerListenRanges",
"contentCachingPeerPolicy",
"contentCachingPort",
"contentCachingPublicRanges",
"contentCachingShowAlerts",
"contentCachingType",
"loginWindowText",
"logOutDisabledWhileLoggedIn",
"macOSSingleSignOnExtension",
"powerOffDisabledWhileLoggedIn",
"restartDisabled",
"restartDisabledWhileLoggedIn",
"screenLockDisableImmediate",
"shutDownDisabled",
"shutDownDisabledWhileLoggedIn",
"singleSignOnExtension",
"sleepDisabled"})
@JsonInclude(Include.NON_NULL)
public class MacOSDeviceFeaturesConfiguration extends AppleDeviceFeaturesConfigurationBase implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.macOSDeviceFeaturesConfiguration";
}
@JsonProperty("adminShowHostInfo")
protected Boolean adminShowHostInfo;
@JsonProperty("appAssociatedDomains")
protected List appAssociatedDomains;
@JsonProperty("appAssociatedDomains@nextLink")
protected String appAssociatedDomainsNextLink;
@JsonProperty("associatedDomains")
protected List associatedDomains;
@JsonProperty("associatedDomains@nextLink")
protected String associatedDomainsNextLink;
@JsonProperty("authorizedUsersListHidden")
protected Boolean authorizedUsersListHidden;
@JsonProperty("authorizedUsersListHideAdminUsers")
protected Boolean authorizedUsersListHideAdminUsers;
@JsonProperty("authorizedUsersListHideLocalUsers")
protected Boolean authorizedUsersListHideLocalUsers;
@JsonProperty("authorizedUsersListHideMobileAccounts")
protected Boolean authorizedUsersListHideMobileAccounts;
@JsonProperty("authorizedUsersListIncludeNetworkUsers")
protected Boolean authorizedUsersListIncludeNetworkUsers;
@JsonProperty("authorizedUsersListShowOtherManagedUsers")
protected Boolean authorizedUsersListShowOtherManagedUsers;
@JsonProperty("autoLaunchItems")
protected List autoLaunchItems;
@JsonProperty("autoLaunchItems@nextLink")
protected String autoLaunchItemsNextLink;
@JsonProperty("consoleAccessDisabled")
protected Boolean consoleAccessDisabled;
@JsonProperty("contentCachingBlockDeletion")
protected Boolean contentCachingBlockDeletion;
@JsonProperty("contentCachingClientListenRanges")
protected List contentCachingClientListenRanges;
@JsonProperty("contentCachingClientListenRanges@nextLink")
protected String contentCachingClientListenRangesNextLink;
@JsonProperty("contentCachingClientPolicy")
protected MacOSContentCachingClientPolicy contentCachingClientPolicy;
@JsonProperty("contentCachingDataPath")
protected String contentCachingDataPath;
@JsonProperty("contentCachingDisableConnectionSharing")
protected Boolean contentCachingDisableConnectionSharing;
@JsonProperty("contentCachingEnabled")
protected Boolean contentCachingEnabled;
@JsonProperty("contentCachingForceConnectionSharing")
protected Boolean contentCachingForceConnectionSharing;
@JsonProperty("contentCachingKeepAwake")
protected Boolean contentCachingKeepAwake;
@JsonProperty("contentCachingLogClientIdentities")
protected Boolean contentCachingLogClientIdentities;
@JsonProperty("contentCachingMaxSizeBytes")
protected Integer contentCachingMaxSizeBytes;
@JsonProperty("contentCachingParents")
protected List contentCachingParents;
@JsonProperty("contentCachingParents@nextLink")
protected String contentCachingParentsNextLink;
@JsonProperty("contentCachingParentSelectionPolicy")
protected MacOSContentCachingParentSelectionPolicy contentCachingParentSelectionPolicy;
@JsonProperty("contentCachingPeerFilterRanges")
protected List contentCachingPeerFilterRanges;
@JsonProperty("contentCachingPeerFilterRanges@nextLink")
protected String contentCachingPeerFilterRangesNextLink;
@JsonProperty("contentCachingPeerListenRanges")
protected List contentCachingPeerListenRanges;
@JsonProperty("contentCachingPeerListenRanges@nextLink")
protected String contentCachingPeerListenRangesNextLink;
@JsonProperty("contentCachingPeerPolicy")
protected MacOSContentCachingPeerPolicy contentCachingPeerPolicy;
@JsonProperty("contentCachingPort")
protected Integer contentCachingPort;
@JsonProperty("contentCachingPublicRanges")
protected List contentCachingPublicRanges;
@JsonProperty("contentCachingPublicRanges@nextLink")
protected String contentCachingPublicRangesNextLink;
@JsonProperty("contentCachingShowAlerts")
protected Boolean contentCachingShowAlerts;
@JsonProperty("contentCachingType")
protected MacOSContentCachingType contentCachingType;
@JsonProperty("loginWindowText")
protected String loginWindowText;
@JsonProperty("logOutDisabledWhileLoggedIn")
protected Boolean logOutDisabledWhileLoggedIn;
@JsonProperty("macOSSingleSignOnExtension")
protected MacOSSingleSignOnExtension macOSSingleSignOnExtension;
@JsonProperty("powerOffDisabledWhileLoggedIn")
protected Boolean powerOffDisabledWhileLoggedIn;
@JsonProperty("restartDisabled")
protected Boolean restartDisabled;
@JsonProperty("restartDisabledWhileLoggedIn")
protected Boolean restartDisabledWhileLoggedIn;
@JsonProperty("screenLockDisableImmediate")
protected Boolean screenLockDisableImmediate;
@JsonProperty("shutDownDisabled")
protected Boolean shutDownDisabled;
@JsonProperty("shutDownDisabledWhileLoggedIn")
protected Boolean shutDownDisabledWhileLoggedIn;
@JsonProperty("singleSignOnExtension")
protected SingleSignOnExtension singleSignOnExtension;
@JsonProperty("sleepDisabled")
protected Boolean sleepDisabled;
protected MacOSDeviceFeaturesConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderMacOSDeviceFeaturesConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode;
private DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition;
private DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private Boolean supportsScopeTags;
private Integer version;
private List airPrintDestinations;
private String airPrintDestinationsNextLink;
private Boolean adminShowHostInfo;
private List appAssociatedDomains;
private String appAssociatedDomainsNextLink;
private List associatedDomains;
private String associatedDomainsNextLink;
private Boolean authorizedUsersListHidden;
private Boolean authorizedUsersListHideAdminUsers;
private Boolean authorizedUsersListHideLocalUsers;
private Boolean authorizedUsersListHideMobileAccounts;
private Boolean authorizedUsersListIncludeNetworkUsers;
private Boolean authorizedUsersListShowOtherManagedUsers;
private List autoLaunchItems;
private String autoLaunchItemsNextLink;
private Boolean consoleAccessDisabled;
private Boolean contentCachingBlockDeletion;
private List contentCachingClientListenRanges;
private String contentCachingClientListenRangesNextLink;
private MacOSContentCachingClientPolicy contentCachingClientPolicy;
private String contentCachingDataPath;
private Boolean contentCachingDisableConnectionSharing;
private Boolean contentCachingEnabled;
private Boolean contentCachingForceConnectionSharing;
private Boolean contentCachingKeepAwake;
private Boolean contentCachingLogClientIdentities;
private Integer contentCachingMaxSizeBytes;
private List contentCachingParents;
private String contentCachingParentsNextLink;
private MacOSContentCachingParentSelectionPolicy contentCachingParentSelectionPolicy;
private List contentCachingPeerFilterRanges;
private String contentCachingPeerFilterRangesNextLink;
private List contentCachingPeerListenRanges;
private String contentCachingPeerListenRangesNextLink;
private MacOSContentCachingPeerPolicy contentCachingPeerPolicy;
private Integer contentCachingPort;
private List contentCachingPublicRanges;
private String contentCachingPublicRangesNextLink;
private Boolean contentCachingShowAlerts;
private MacOSContentCachingType contentCachingType;
private String loginWindowText;
private Boolean logOutDisabledWhileLoggedIn;
private MacOSSingleSignOnExtension macOSSingleSignOnExtension;
private Boolean powerOffDisabledWhileLoggedIn;
private Boolean restartDisabled;
private Boolean restartDisabledWhileLoggedIn;
private Boolean screenLockDisableImmediate;
private Boolean shutDownDisabled;
private Boolean shutDownDisabledWhileLoggedIn;
private SingleSignOnExtension singleSignOnExtension;
private Boolean sleepDisabled;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder deviceManagementApplicabilityRuleDeviceMode(DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode) {
this.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleDeviceMode");
return this;
}
public Builder deviceManagementApplicabilityRuleOsEdition(DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition) {
this.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsEdition");
return this;
}
public Builder deviceManagementApplicabilityRuleOsVersion(DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion) {
this.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsVersion");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder supportsScopeTags(Boolean supportsScopeTags) {
this.supportsScopeTags = supportsScopeTags;
this.changedFields = changedFields.add("supportsScopeTags");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
public Builder airPrintDestinations(List airPrintDestinations) {
this.airPrintDestinations = airPrintDestinations;
this.changedFields = changedFields.add("airPrintDestinations");
return this;
}
public Builder airPrintDestinations(AirPrintDestination... airPrintDestinations) {
return airPrintDestinations(Arrays.asList(airPrintDestinations));
}
public Builder airPrintDestinationsNextLink(String airPrintDestinationsNextLink) {
this.airPrintDestinationsNextLink = airPrintDestinationsNextLink;
this.changedFields = changedFields.add("airPrintDestinations");
return this;
}
/**
* “Whether to show admin host information on the login window.”
*
* @param adminShowHostInfo
* value of {@code adminShowHostInfo} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder adminShowHostInfo(Boolean adminShowHostInfo) {
this.adminShowHostInfo = adminShowHostInfo;
this.changedFields = changedFields.add("adminShowHostInfo");
return this;
}
/**
* “Gets or sets a list that maps apps to their associated domains. Application
* identifiers must be unique. This collection can contain a maximum of 500
* elements.”
*
* @param appAssociatedDomains
* value of {@code appAssociatedDomains} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder appAssociatedDomains(List appAssociatedDomains) {
this.appAssociatedDomains = appAssociatedDomains;
this.changedFields = changedFields.add("appAssociatedDomains");
return this;
}
/**
* “Gets or sets a list that maps apps to their associated domains. Application
* identifiers must be unique. This collection can contain a maximum of 500
* elements.”
*
* @param appAssociatedDomains
* value of {@code appAssociatedDomains} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder appAssociatedDomains(MacOSAssociatedDomainsItem... appAssociatedDomains) {
return appAssociatedDomains(Arrays.asList(appAssociatedDomains));
}
/**
* “Gets or sets a list that maps apps to their associated domains. Application
* identifiers must be unique. This collection can contain a maximum of 500
* elements.”
*
* @param appAssociatedDomainsNextLink
* value of {@code appAssociatedDomains@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder appAssociatedDomainsNextLink(String appAssociatedDomainsNextLink) {
this.appAssociatedDomainsNextLink = appAssociatedDomainsNextLink;
this.changedFields = changedFields.add("appAssociatedDomains");
return this;
}
/**
* “DEPRECATED: use appAssociatedDomains instead. Gets or sets a list that maps apps
* to their associated domains. The key should match the app's ID, and the value
* should be a string in the form of "service:domain" where domain is a fully
* qualified hostname (e.g. webcredentials:example.com). This collection can
* contain a maximum of 500 elements.”
*
* @param associatedDomains
* value of {@code associatedDomains} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder associatedDomains(List associatedDomains) {
this.associatedDomains = associatedDomains;
this.changedFields = changedFields.add("associatedDomains");
return this;
}
/**
* “DEPRECATED: use appAssociatedDomains instead. Gets or sets a list that maps apps
* to their associated domains. The key should match the app's ID, and the value
* should be a string in the form of "service:domain" where domain is a fully
* qualified hostname (e.g. webcredentials:example.com). This collection can
* contain a maximum of 500 elements.”
*
* @param associatedDomains
* value of {@code associatedDomains} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder associatedDomains(KeyValuePair... associatedDomains) {
return associatedDomains(Arrays.asList(associatedDomains));
}
/**
* “DEPRECATED: use appAssociatedDomains instead. Gets or sets a list that maps apps
* to their associated domains. The key should match the app's ID, and the value
* should be a string in the form of "service:domain" where domain is a fully
* qualified hostname (e.g. webcredentials:example.com). This collection can
* contain a maximum of 500 elements.”
*
* @param associatedDomainsNextLink
* value of {@code associatedDomains@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder associatedDomainsNextLink(String associatedDomainsNextLink) {
this.associatedDomainsNextLink = associatedDomainsNextLink;
this.changedFields = changedFields.add("associatedDomains");
return this;
}
/**
* “Whether to show the name and password dialog or a list of users on the login
* window.”
*
* @param authorizedUsersListHidden
* value of {@code authorizedUsersListHidden} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder authorizedUsersListHidden(Boolean authorizedUsersListHidden) {
this.authorizedUsersListHidden = authorizedUsersListHidden;
this.changedFields = changedFields.add("authorizedUsersListHidden");
return this;
}
/**
* “Whether to hide admin users in the authorized users list on the login window.”
*
* @param authorizedUsersListHideAdminUsers
* value of {@code authorizedUsersListHideAdminUsers} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder authorizedUsersListHideAdminUsers(Boolean authorizedUsersListHideAdminUsers) {
this.authorizedUsersListHideAdminUsers = authorizedUsersListHideAdminUsers;
this.changedFields = changedFields.add("authorizedUsersListHideAdminUsers");
return this;
}
/**
* “Whether to show only network and system users in the authorized users list on
* the login window.”
*
* @param authorizedUsersListHideLocalUsers
* value of {@code authorizedUsersListHideLocalUsers} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder authorizedUsersListHideLocalUsers(Boolean authorizedUsersListHideLocalUsers) {
this.authorizedUsersListHideLocalUsers = authorizedUsersListHideLocalUsers;
this.changedFields = changedFields.add("authorizedUsersListHideLocalUsers");
return this;
}
/**
* “Whether to hide mobile users in the authorized users list on the login window.”
*
* @param authorizedUsersListHideMobileAccounts
* value of {@code authorizedUsersListHideMobileAccounts} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder authorizedUsersListHideMobileAccounts(Boolean authorizedUsersListHideMobileAccounts) {
this.authorizedUsersListHideMobileAccounts = authorizedUsersListHideMobileAccounts;
this.changedFields = changedFields.add("authorizedUsersListHideMobileAccounts");
return this;
}
/**
* “Whether to show network users in the authorized users list on the login window.”
*
* @param authorizedUsersListIncludeNetworkUsers
* value of {@code authorizedUsersListIncludeNetworkUsers} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder authorizedUsersListIncludeNetworkUsers(Boolean authorizedUsersListIncludeNetworkUsers) {
this.authorizedUsersListIncludeNetworkUsers = authorizedUsersListIncludeNetworkUsers;
this.changedFields = changedFields.add("authorizedUsersListIncludeNetworkUsers");
return this;
}
/**
* “Whether to show other users in the authorized users list on the login window.”
*
* @param authorizedUsersListShowOtherManagedUsers
* value of {@code authorizedUsersListShowOtherManagedUsers} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder authorizedUsersListShowOtherManagedUsers(Boolean authorizedUsersListShowOtherManagedUsers) {
this.authorizedUsersListShowOtherManagedUsers = authorizedUsersListShowOtherManagedUsers;
this.changedFields = changedFields.add("authorizedUsersListShowOtherManagedUsers");
return this;
}
/**
* “List of applications, files, folders, and other items to launch when the user
* logs in. This collection can contain a maximum of 500 elements.”
*
* @param autoLaunchItems
* value of {@code autoLaunchItems} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder autoLaunchItems(List autoLaunchItems) {
this.autoLaunchItems = autoLaunchItems;
this.changedFields = changedFields.add("autoLaunchItems");
return this;
}
/**
* “List of applications, files, folders, and other items to launch when the user
* logs in. This collection can contain a maximum of 500 elements.”
*
* @param autoLaunchItems
* value of {@code autoLaunchItems} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder autoLaunchItems(MacOSLaunchItem... autoLaunchItems) {
return autoLaunchItems(Arrays.asList(autoLaunchItems));
}
/**
* “List of applications, files, folders, and other items to launch when the user
* logs in. This collection can contain a maximum of 500 elements.”
*
* @param autoLaunchItemsNextLink
* value of {@code autoLaunchItems@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder autoLaunchItemsNextLink(String autoLaunchItemsNextLink) {
this.autoLaunchItemsNextLink = autoLaunchItemsNextLink;
this.changedFields = changedFields.add("autoLaunchItems");
return this;
}
/**
* “Whether the Other user will disregard use of the `>console> special user name.”
*
* @param consoleAccessDisabled
* value of {@code consoleAccessDisabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder consoleAccessDisabled(Boolean consoleAccessDisabled) {
this.consoleAccessDisabled = consoleAccessDisabled;
this.changedFields = changedFields.add("consoleAccessDisabled");
return this;
}
/**
* “Prevents content caches from purging content to free up disk space for other
* apps.”
*
* @param contentCachingBlockDeletion
* value of {@code contentCachingBlockDeletion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingBlockDeletion(Boolean contentCachingBlockDeletion) {
this.contentCachingBlockDeletion = contentCachingBlockDeletion;
this.changedFields = changedFields.add("contentCachingBlockDeletion");
return this;
}
/**
* “A list of custom IP ranges content caches will use to listen for clients. This
* collection can contain a maximum of 500 elements.”
*
* @param contentCachingClientListenRanges
* value of {@code contentCachingClientListenRanges} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingClientListenRanges(List contentCachingClientListenRanges) {
this.contentCachingClientListenRanges = contentCachingClientListenRanges;
this.changedFields = changedFields.add("contentCachingClientListenRanges");
return this;
}
/**
* “A list of custom IP ranges content caches will use to listen for clients. This
* collection can contain a maximum of 500 elements.”
*
* @param contentCachingClientListenRanges
* value of {@code contentCachingClientListenRanges} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingClientListenRanges(IpRange... contentCachingClientListenRanges) {
return contentCachingClientListenRanges(Arrays.asList(contentCachingClientListenRanges));
}
/**
* “A list of custom IP ranges content caches will use to listen for clients. This
* collection can contain a maximum of 500 elements.”
*
* @param contentCachingClientListenRangesNextLink
* value of {@code contentCachingClientListenRanges@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingClientListenRangesNextLink(String contentCachingClientListenRangesNextLink) {
this.contentCachingClientListenRangesNextLink = contentCachingClientListenRangesNextLink;
this.changedFields = changedFields.add("contentCachingClientListenRanges");
return this;
}
/**
* “Determines the method in which content caching servers will listen for clients.”
*
* @param contentCachingClientPolicy
* value of {@code contentCachingClientPolicy} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingClientPolicy(MacOSContentCachingClientPolicy contentCachingClientPolicy) {
this.contentCachingClientPolicy = contentCachingClientPolicy;
this.changedFields = changedFields.add("contentCachingClientPolicy");
return this;
}
/**
* “The path to the directory used to store cached content. The value must be (or
* end with) /Library/Application Support/Apple/AssetCache/Data”
*
* @param contentCachingDataPath
* value of {@code contentCachingDataPath} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingDataPath(String contentCachingDataPath) {
this.contentCachingDataPath = contentCachingDataPath;
this.changedFields = changedFields.add("contentCachingDataPath");
return this;
}
/**
* “Disables internet connection sharing.”
*
* @param contentCachingDisableConnectionSharing
* value of {@code contentCachingDisableConnectionSharing} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingDisableConnectionSharing(Boolean contentCachingDisableConnectionSharing) {
this.contentCachingDisableConnectionSharing = contentCachingDisableConnectionSharing;
this.changedFields = changedFields.add("contentCachingDisableConnectionSharing");
return this;
}
/**
* “Enables content caching and prevents it from being disabled by the user.”
*
* @param contentCachingEnabled
* value of {@code contentCachingEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingEnabled(Boolean contentCachingEnabled) {
this.contentCachingEnabled = contentCachingEnabled;
this.changedFields = changedFields.add("contentCachingEnabled");
return this;
}
/**
* “Forces internet connection sharing. contentCachingDisableConnectionSharing
* overrides this setting.”
*
* @param contentCachingForceConnectionSharing
* value of {@code contentCachingForceConnectionSharing} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingForceConnectionSharing(Boolean contentCachingForceConnectionSharing) {
this.contentCachingForceConnectionSharing = contentCachingForceConnectionSharing;
this.changedFields = changedFields.add("contentCachingForceConnectionSharing");
return this;
}
/**
* “Prevent the device from sleeping if content caching is enabled.”
*
* @param contentCachingKeepAwake
* value of {@code contentCachingKeepAwake} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingKeepAwake(Boolean contentCachingKeepAwake) {
this.contentCachingKeepAwake = contentCachingKeepAwake;
this.changedFields = changedFields.add("contentCachingKeepAwake");
return this;
}
/**
* “Enables logging of IP addresses and ports of clients that request cached content
* .”
*
* @param contentCachingLogClientIdentities
* value of {@code contentCachingLogClientIdentities} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingLogClientIdentities(Boolean contentCachingLogClientIdentities) {
this.contentCachingLogClientIdentities = contentCachingLogClientIdentities;
this.changedFields = changedFields.add("contentCachingLogClientIdentities");
return this;
}
/**
* “The maximum number of bytes of disk space that will be used for the content
* cache. A value of 0 (default) indicates unlimited disk space.”
*
* @param contentCachingMaxSizeBytes
* value of {@code contentCachingMaxSizeBytes} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingMaxSizeBytes(Integer contentCachingMaxSizeBytes) {
this.contentCachingMaxSizeBytes = contentCachingMaxSizeBytes;
this.changedFields = changedFields.add("contentCachingMaxSizeBytes");
return this;
}
/**
* “A list of IP addresses representing parent content caches.”
*
* @param contentCachingParents
* value of {@code contentCachingParents} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingParents(List contentCachingParents) {
this.contentCachingParents = contentCachingParents;
this.changedFields = changedFields.add("contentCachingParents");
return this;
}
/**
* “A list of IP addresses representing parent content caches.”
*
* @param contentCachingParents
* value of {@code contentCachingParents} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingParents(String... contentCachingParents) {
return contentCachingParents(Arrays.asList(contentCachingParents));
}
/**
* “A list of IP addresses representing parent content caches.”
*
* @param contentCachingParentsNextLink
* value of {@code contentCachingParents@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingParentsNextLink(String contentCachingParentsNextLink) {
this.contentCachingParentsNextLink = contentCachingParentsNextLink;
this.changedFields = changedFields.add("contentCachingParents");
return this;
}
/**
* “Determines the method in which content caching servers will select parents if
* multiple are present.”
*
* @param contentCachingParentSelectionPolicy
* value of {@code contentCachingParentSelectionPolicy} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingParentSelectionPolicy(MacOSContentCachingParentSelectionPolicy contentCachingParentSelectionPolicy) {
this.contentCachingParentSelectionPolicy = contentCachingParentSelectionPolicy;
this.changedFields = changedFields.add("contentCachingParentSelectionPolicy");
return this;
}
/**
* “A list of custom IP ranges content caches will use to query for content from
* peers caches. This collection can contain a maximum of 500 elements.”
*
* @param contentCachingPeerFilterRanges
* value of {@code contentCachingPeerFilterRanges} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingPeerFilterRanges(List contentCachingPeerFilterRanges) {
this.contentCachingPeerFilterRanges = contentCachingPeerFilterRanges;
this.changedFields = changedFields.add("contentCachingPeerFilterRanges");
return this;
}
/**
* “A list of custom IP ranges content caches will use to query for content from
* peers caches. This collection can contain a maximum of 500 elements.”
*
* @param contentCachingPeerFilterRanges
* value of {@code contentCachingPeerFilterRanges} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingPeerFilterRanges(IpRange... contentCachingPeerFilterRanges) {
return contentCachingPeerFilterRanges(Arrays.asList(contentCachingPeerFilterRanges));
}
/**
* “A list of custom IP ranges content caches will use to query for content from
* peers caches. This collection can contain a maximum of 500 elements.”
*
* @param contentCachingPeerFilterRangesNextLink
* value of {@code contentCachingPeerFilterRanges@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingPeerFilterRangesNextLink(String contentCachingPeerFilterRangesNextLink) {
this.contentCachingPeerFilterRangesNextLink = contentCachingPeerFilterRangesNextLink;
this.changedFields = changedFields.add("contentCachingPeerFilterRanges");
return this;
}
/**
* “A list of custom IP ranges content caches will use to listen for peer caches.
* This collection can contain a maximum of 500 elements.”
*
* @param contentCachingPeerListenRanges
* value of {@code contentCachingPeerListenRanges} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingPeerListenRanges(List contentCachingPeerListenRanges) {
this.contentCachingPeerListenRanges = contentCachingPeerListenRanges;
this.changedFields = changedFields.add("contentCachingPeerListenRanges");
return this;
}
/**
* “A list of custom IP ranges content caches will use to listen for peer caches.
* This collection can contain a maximum of 500 elements.”
*
* @param contentCachingPeerListenRanges
* value of {@code contentCachingPeerListenRanges} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingPeerListenRanges(IpRange... contentCachingPeerListenRanges) {
return contentCachingPeerListenRanges(Arrays.asList(contentCachingPeerListenRanges));
}
/**
* “A list of custom IP ranges content caches will use to listen for peer caches.
* This collection can contain a maximum of 500 elements.”
*
* @param contentCachingPeerListenRangesNextLink
* value of {@code contentCachingPeerListenRanges@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingPeerListenRangesNextLink(String contentCachingPeerListenRangesNextLink) {
this.contentCachingPeerListenRangesNextLink = contentCachingPeerListenRangesNextLink;
this.changedFields = changedFields.add("contentCachingPeerListenRanges");
return this;
}
/**
* “Determines the method in which content caches peer with other caches.”
*
* @param contentCachingPeerPolicy
* value of {@code contentCachingPeerPolicy} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingPeerPolicy(MacOSContentCachingPeerPolicy contentCachingPeerPolicy) {
this.contentCachingPeerPolicy = contentCachingPeerPolicy;
this.changedFields = changedFields.add("contentCachingPeerPolicy");
return this;
}
/**
* “Sets the port used for content caching. If the value is 0, a random available
* port will be selected. Valid values 0 to 65535”
*
* @param contentCachingPort
* value of {@code contentCachingPort} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingPort(Integer contentCachingPort) {
this.contentCachingPort = contentCachingPort;
this.changedFields = changedFields.add("contentCachingPort");
return this;
}
/**
* “A list of custom IP ranges that Apple's content caching service should use to
* match clients to content caches. This collection can contain a maximum of 500
* elements.”
*
* @param contentCachingPublicRanges
* value of {@code contentCachingPublicRanges} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingPublicRanges(List contentCachingPublicRanges) {
this.contentCachingPublicRanges = contentCachingPublicRanges;
this.changedFields = changedFields.add("contentCachingPublicRanges");
return this;
}
/**
* “A list of custom IP ranges that Apple's content caching service should use to
* match clients to content caches. This collection can contain a maximum of 500
* elements.”
*
* @param contentCachingPublicRanges
* value of {@code contentCachingPublicRanges} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingPublicRanges(IpRange... contentCachingPublicRanges) {
return contentCachingPublicRanges(Arrays.asList(contentCachingPublicRanges));
}
/**
* “A list of custom IP ranges that Apple's content caching service should use to
* match clients to content caches. This collection can contain a maximum of 500
* elements.”
*
* @param contentCachingPublicRangesNextLink
* value of {@code contentCachingPublicRanges@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingPublicRangesNextLink(String contentCachingPublicRangesNextLink) {
this.contentCachingPublicRangesNextLink = contentCachingPublicRangesNextLink;
this.changedFields = changedFields.add("contentCachingPublicRanges");
return this;
}
/**
* “Display content caching alerts as system notifications.”
*
* @param contentCachingShowAlerts
* value of {@code contentCachingShowAlerts} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingShowAlerts(Boolean contentCachingShowAlerts) {
this.contentCachingShowAlerts = contentCachingShowAlerts;
this.changedFields = changedFields.add("contentCachingShowAlerts");
return this;
}
/**
* “Determines what type of content is allowed to be cached by Apple's content
* caching service.”
*
* @param contentCachingType
* value of {@code contentCachingType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder contentCachingType(MacOSContentCachingType contentCachingType) {
this.contentCachingType = contentCachingType;
this.changedFields = changedFields.add("contentCachingType");
return this;
}
/**
* “Custom text to be displayed on the login window.”
*
* @param loginWindowText
* value of {@code loginWindowText} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder loginWindowText(String loginWindowText) {
this.loginWindowText = loginWindowText;
this.changedFields = changedFields.add("loginWindowText");
return this;
}
/**
* “Whether the Log Out menu item on the login window will be disabled while the
* user is logged in.”
*
* @param logOutDisabledWhileLoggedIn
* value of {@code logOutDisabledWhileLoggedIn} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder logOutDisabledWhileLoggedIn(Boolean logOutDisabledWhileLoggedIn) {
this.logOutDisabledWhileLoggedIn = logOutDisabledWhileLoggedIn;
this.changedFields = changedFields.add("logOutDisabledWhileLoggedIn");
return this;
}
/**
* “Gets or sets a single sign-on extension profile.”
*
* @param macOSSingleSignOnExtension
* value of {@code macOSSingleSignOnExtension} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder macOSSingleSignOnExtension(MacOSSingleSignOnExtension macOSSingleSignOnExtension) {
this.macOSSingleSignOnExtension = macOSSingleSignOnExtension;
this.changedFields = changedFields.add("macOSSingleSignOnExtension");
return this;
}
/**
* “Whether the Power Off menu item on the login window will be disabled while the
* user is logged in.”
*
* @param powerOffDisabledWhileLoggedIn
* value of {@code powerOffDisabledWhileLoggedIn} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder powerOffDisabledWhileLoggedIn(Boolean powerOffDisabledWhileLoggedIn) {
this.powerOffDisabledWhileLoggedIn = powerOffDisabledWhileLoggedIn;
this.changedFields = changedFields.add("powerOffDisabledWhileLoggedIn");
return this;
}
/**
* “Whether to hide the Restart button item on the login window.”
*
* @param restartDisabled
* value of {@code restartDisabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder restartDisabled(Boolean restartDisabled) {
this.restartDisabled = restartDisabled;
this.changedFields = changedFields.add("restartDisabled");
return this;
}
/**
* “Whether the Restart menu item on the login window will be disabled while the
* user is logged in.”
*
* @param restartDisabledWhileLoggedIn
* value of {@code restartDisabledWhileLoggedIn} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder restartDisabledWhileLoggedIn(Boolean restartDisabledWhileLoggedIn) {
this.restartDisabledWhileLoggedIn = restartDisabledWhileLoggedIn;
this.changedFields = changedFields.add("restartDisabledWhileLoggedIn");
return this;
}
/**
* “Whether to disable the immediate screen lock functions.”
*
* @param screenLockDisableImmediate
* value of {@code screenLockDisableImmediate} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder screenLockDisableImmediate(Boolean screenLockDisableImmediate) {
this.screenLockDisableImmediate = screenLockDisableImmediate;
this.changedFields = changedFields.add("screenLockDisableImmediate");
return this;
}
/**
* “Whether to hide the Shut Down button item on the login window.”
*
* @param shutDownDisabled
* value of {@code shutDownDisabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder shutDownDisabled(Boolean shutDownDisabled) {
this.shutDownDisabled = shutDownDisabled;
this.changedFields = changedFields.add("shutDownDisabled");
return this;
}
/**
* “Whether the Shut Down menu item on the login window will be disabled while the
* user is logged in.”
*
* @param shutDownDisabledWhileLoggedIn
* value of {@code shutDownDisabledWhileLoggedIn} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder shutDownDisabledWhileLoggedIn(Boolean shutDownDisabledWhileLoggedIn) {
this.shutDownDisabledWhileLoggedIn = shutDownDisabledWhileLoggedIn;
this.changedFields = changedFields.add("shutDownDisabledWhileLoggedIn");
return this;
}
/**
* “Gets or sets a single sign-on extension profile. Deprecated: use
* MacOSSingleSignOnExtension instead.”
*
* @param singleSignOnExtension
* value of {@code singleSignOnExtension} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder singleSignOnExtension(SingleSignOnExtension singleSignOnExtension) {
this.singleSignOnExtension = singleSignOnExtension;
this.changedFields = changedFields.add("singleSignOnExtension");
return this;
}
/**
* “Whether to hide the Sleep menu item on the login window.”
*
* @param sleepDisabled
* value of {@code sleepDisabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder sleepDisabled(Boolean sleepDisabled) {
this.sleepDisabled = sleepDisabled;
this.changedFields = changedFields.add("sleepDisabled");
return this;
}
public MacOSDeviceFeaturesConfiguration build() {
MacOSDeviceFeaturesConfiguration _x = new MacOSDeviceFeaturesConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.macOSDeviceFeaturesConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.airPrintDestinations = airPrintDestinations;
_x.airPrintDestinationsNextLink = airPrintDestinationsNextLink;
_x.adminShowHostInfo = adminShowHostInfo;
_x.appAssociatedDomains = appAssociatedDomains;
_x.appAssociatedDomainsNextLink = appAssociatedDomainsNextLink;
_x.associatedDomains = associatedDomains;
_x.associatedDomainsNextLink = associatedDomainsNextLink;
_x.authorizedUsersListHidden = authorizedUsersListHidden;
_x.authorizedUsersListHideAdminUsers = authorizedUsersListHideAdminUsers;
_x.authorizedUsersListHideLocalUsers = authorizedUsersListHideLocalUsers;
_x.authorizedUsersListHideMobileAccounts = authorizedUsersListHideMobileAccounts;
_x.authorizedUsersListIncludeNetworkUsers = authorizedUsersListIncludeNetworkUsers;
_x.authorizedUsersListShowOtherManagedUsers = authorizedUsersListShowOtherManagedUsers;
_x.autoLaunchItems = autoLaunchItems;
_x.autoLaunchItemsNextLink = autoLaunchItemsNextLink;
_x.consoleAccessDisabled = consoleAccessDisabled;
_x.contentCachingBlockDeletion = contentCachingBlockDeletion;
_x.contentCachingClientListenRanges = contentCachingClientListenRanges;
_x.contentCachingClientListenRangesNextLink = contentCachingClientListenRangesNextLink;
_x.contentCachingClientPolicy = contentCachingClientPolicy;
_x.contentCachingDataPath = contentCachingDataPath;
_x.contentCachingDisableConnectionSharing = contentCachingDisableConnectionSharing;
_x.contentCachingEnabled = contentCachingEnabled;
_x.contentCachingForceConnectionSharing = contentCachingForceConnectionSharing;
_x.contentCachingKeepAwake = contentCachingKeepAwake;
_x.contentCachingLogClientIdentities = contentCachingLogClientIdentities;
_x.contentCachingMaxSizeBytes = contentCachingMaxSizeBytes;
_x.contentCachingParents = contentCachingParents;
_x.contentCachingParentsNextLink = contentCachingParentsNextLink;
_x.contentCachingParentSelectionPolicy = contentCachingParentSelectionPolicy;
_x.contentCachingPeerFilterRanges = contentCachingPeerFilterRanges;
_x.contentCachingPeerFilterRangesNextLink = contentCachingPeerFilterRangesNextLink;
_x.contentCachingPeerListenRanges = contentCachingPeerListenRanges;
_x.contentCachingPeerListenRangesNextLink = contentCachingPeerListenRangesNextLink;
_x.contentCachingPeerPolicy = contentCachingPeerPolicy;
_x.contentCachingPort = contentCachingPort;
_x.contentCachingPublicRanges = contentCachingPublicRanges;
_x.contentCachingPublicRangesNextLink = contentCachingPublicRangesNextLink;
_x.contentCachingShowAlerts = contentCachingShowAlerts;
_x.contentCachingType = contentCachingType;
_x.loginWindowText = loginWindowText;
_x.logOutDisabledWhileLoggedIn = logOutDisabledWhileLoggedIn;
_x.macOSSingleSignOnExtension = macOSSingleSignOnExtension;
_x.powerOffDisabledWhileLoggedIn = powerOffDisabledWhileLoggedIn;
_x.restartDisabled = restartDisabled;
_x.restartDisabledWhileLoggedIn = restartDisabledWhileLoggedIn;
_x.screenLockDisableImmediate = screenLockDisableImmediate;
_x.shutDownDisabled = shutDownDisabled;
_x.shutDownDisabledWhileLoggedIn = shutDownDisabledWhileLoggedIn;
_x.singleSignOnExtension = singleSignOnExtension;
_x.sleepDisabled = sleepDisabled;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Whether to show admin host information on the login window.”
*
* @return property adminShowHostInfo
*/
@Property(name="adminShowHostInfo")
@JsonIgnore
public Optional getAdminShowHostInfo() {
return Optional.ofNullable(adminShowHostInfo);
}
/**
* Returns an immutable copy of {@code this} with just the {@code adminShowHostInfo
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Whether to show admin host information on the login window.”
*
* @param adminShowHostInfo
* new value of {@code adminShowHostInfo} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code adminShowHostInfo} field changed
*/
public MacOSDeviceFeaturesConfiguration withAdminShowHostInfo(Boolean adminShowHostInfo) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("adminShowHostInfo");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.adminShowHostInfo = adminShowHostInfo;
return _x;
}
/**
* “Gets or sets a list that maps apps to their associated domains. Application
* identifiers must be unique. This collection can contain a maximum of 500
* elements.”
*
* @return property appAssociatedDomains
*/
@Property(name="appAssociatedDomains")
@JsonIgnore
public CollectionPage getAppAssociatedDomains() {
return new CollectionPage(contextPath, MacOSAssociatedDomainsItem.class, this.appAssociatedDomains, Optional.ofNullable(appAssociatedDomainsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* appAssociatedDomains} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Gets or sets a list that maps apps to their associated domains. Application
* identifiers must be unique. This collection can contain a maximum of 500
* elements.”
*
* @param appAssociatedDomains
* new value of {@code appAssociatedDomains} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code appAssociatedDomains} field changed
*/
public MacOSDeviceFeaturesConfiguration withAppAssociatedDomains(List appAssociatedDomains) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("appAssociatedDomains");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.appAssociatedDomains = appAssociatedDomains;
return _x;
}
/**
* “Gets or sets a list that maps apps to their associated domains. Application
* identifiers must be unique. This collection can contain a maximum of 500
* elements.”
*
* @param options
* specify connect and read timeouts
* @return property appAssociatedDomains
*/
@Property(name="appAssociatedDomains")
@JsonIgnore
public CollectionPage getAppAssociatedDomains(HttpRequestOptions options) {
return new CollectionPage(contextPath, MacOSAssociatedDomainsItem.class, this.appAssociatedDomains, Optional.ofNullable(appAssociatedDomainsNextLink), Collections.emptyList(), options);
}
/**
* “DEPRECATED: use appAssociatedDomains instead. Gets or sets a list that maps apps
* to their associated domains. The key should match the app's ID, and the value
* should be a string in the form of "service:domain" where domain is a fully
* qualified hostname (e.g. webcredentials:example.com). This collection can
* contain a maximum of 500 elements.”
*
* @return property associatedDomains
*/
@Property(name="associatedDomains")
@JsonIgnore
public CollectionPage getAssociatedDomains() {
return new CollectionPage(contextPath, KeyValuePair.class, this.associatedDomains, Optional.ofNullable(associatedDomainsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code associatedDomains
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “DEPRECATED: use appAssociatedDomains instead. Gets or sets a list that maps apps
* to their associated domains. The key should match the app's ID, and the value
* should be a string in the form of "service:domain" where domain is a fully
* qualified hostname (e.g. webcredentials:example.com). This collection can
* contain a maximum of 500 elements.”
*
* @param associatedDomains
* new value of {@code associatedDomains} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code associatedDomains} field changed
*/
public MacOSDeviceFeaturesConfiguration withAssociatedDomains(List associatedDomains) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("associatedDomains");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.associatedDomains = associatedDomains;
return _x;
}
/**
* “DEPRECATED: use appAssociatedDomains instead. Gets or sets a list that maps apps
* to their associated domains. The key should match the app's ID, and the value
* should be a string in the form of "service:domain" where domain is a fully
* qualified hostname (e.g. webcredentials:example.com). This collection can
* contain a maximum of 500 elements.”
*
* @param options
* specify connect and read timeouts
* @return property associatedDomains
*/
@Property(name="associatedDomains")
@JsonIgnore
public CollectionPage getAssociatedDomains(HttpRequestOptions options) {
return new CollectionPage(contextPath, KeyValuePair.class, this.associatedDomains, Optional.ofNullable(associatedDomainsNextLink), Collections.emptyList(), options);
}
/**
* “Whether to show the name and password dialog or a list of users on the login
* window.”
*
* @return property authorizedUsersListHidden
*/
@Property(name="authorizedUsersListHidden")
@JsonIgnore
public Optional getAuthorizedUsersListHidden() {
return Optional.ofNullable(authorizedUsersListHidden);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* authorizedUsersListHidden} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Whether to show the name and password dialog or a list of users on the login
* window.”
*
* @param authorizedUsersListHidden
* new value of {@code authorizedUsersListHidden} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code authorizedUsersListHidden} field changed
*/
public MacOSDeviceFeaturesConfiguration withAuthorizedUsersListHidden(Boolean authorizedUsersListHidden) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("authorizedUsersListHidden");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.authorizedUsersListHidden = authorizedUsersListHidden;
return _x;
}
/**
* “Whether to hide admin users in the authorized users list on the login window.”
*
* @return property authorizedUsersListHideAdminUsers
*/
@Property(name="authorizedUsersListHideAdminUsers")
@JsonIgnore
public Optional getAuthorizedUsersListHideAdminUsers() {
return Optional.ofNullable(authorizedUsersListHideAdminUsers);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* authorizedUsersListHideAdminUsers} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Whether to hide admin users in the authorized users list on the login window.”
*
* @param authorizedUsersListHideAdminUsers
* new value of {@code authorizedUsersListHideAdminUsers} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code authorizedUsersListHideAdminUsers} field changed
*/
public MacOSDeviceFeaturesConfiguration withAuthorizedUsersListHideAdminUsers(Boolean authorizedUsersListHideAdminUsers) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("authorizedUsersListHideAdminUsers");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.authorizedUsersListHideAdminUsers = authorizedUsersListHideAdminUsers;
return _x;
}
/**
* “Whether to show only network and system users in the authorized users list on
* the login window.”
*
* @return property authorizedUsersListHideLocalUsers
*/
@Property(name="authorizedUsersListHideLocalUsers")
@JsonIgnore
public Optional getAuthorizedUsersListHideLocalUsers() {
return Optional.ofNullable(authorizedUsersListHideLocalUsers);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* authorizedUsersListHideLocalUsers} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Whether to show only network and system users in the authorized users list on
* the login window.”
*
* @param authorizedUsersListHideLocalUsers
* new value of {@code authorizedUsersListHideLocalUsers} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code authorizedUsersListHideLocalUsers} field changed
*/
public MacOSDeviceFeaturesConfiguration withAuthorizedUsersListHideLocalUsers(Boolean authorizedUsersListHideLocalUsers) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("authorizedUsersListHideLocalUsers");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.authorizedUsersListHideLocalUsers = authorizedUsersListHideLocalUsers;
return _x;
}
/**
* “Whether to hide mobile users in the authorized users list on the login window.”
*
* @return property authorizedUsersListHideMobileAccounts
*/
@Property(name="authorizedUsersListHideMobileAccounts")
@JsonIgnore
public Optional getAuthorizedUsersListHideMobileAccounts() {
return Optional.ofNullable(authorizedUsersListHideMobileAccounts);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* authorizedUsersListHideMobileAccounts} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Whether to hide mobile users in the authorized users list on the login window.”
*
* @param authorizedUsersListHideMobileAccounts
* new value of {@code authorizedUsersListHideMobileAccounts} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code authorizedUsersListHideMobileAccounts} field changed
*/
public MacOSDeviceFeaturesConfiguration withAuthorizedUsersListHideMobileAccounts(Boolean authorizedUsersListHideMobileAccounts) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("authorizedUsersListHideMobileAccounts");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.authorizedUsersListHideMobileAccounts = authorizedUsersListHideMobileAccounts;
return _x;
}
/**
* “Whether to show network users in the authorized users list on the login window.”
*
* @return property authorizedUsersListIncludeNetworkUsers
*/
@Property(name="authorizedUsersListIncludeNetworkUsers")
@JsonIgnore
public Optional getAuthorizedUsersListIncludeNetworkUsers() {
return Optional.ofNullable(authorizedUsersListIncludeNetworkUsers);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* authorizedUsersListIncludeNetworkUsers} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Whether to show network users in the authorized users list on the login window.”
*
* @param authorizedUsersListIncludeNetworkUsers
* new value of {@code authorizedUsersListIncludeNetworkUsers} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code authorizedUsersListIncludeNetworkUsers} field changed
*/
public MacOSDeviceFeaturesConfiguration withAuthorizedUsersListIncludeNetworkUsers(Boolean authorizedUsersListIncludeNetworkUsers) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("authorizedUsersListIncludeNetworkUsers");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.authorizedUsersListIncludeNetworkUsers = authorizedUsersListIncludeNetworkUsers;
return _x;
}
/**
* “Whether to show other users in the authorized users list on the login window.”
*
* @return property authorizedUsersListShowOtherManagedUsers
*/
@Property(name="authorizedUsersListShowOtherManagedUsers")
@JsonIgnore
public Optional getAuthorizedUsersListShowOtherManagedUsers() {
return Optional.ofNullable(authorizedUsersListShowOtherManagedUsers);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* authorizedUsersListShowOtherManagedUsers} field changed. Field description below
* . The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Whether to show other users in the authorized users list on the login window.”
*
* @param authorizedUsersListShowOtherManagedUsers
* new value of {@code authorizedUsersListShowOtherManagedUsers} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code authorizedUsersListShowOtherManagedUsers} field changed
*/
public MacOSDeviceFeaturesConfiguration withAuthorizedUsersListShowOtherManagedUsers(Boolean authorizedUsersListShowOtherManagedUsers) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("authorizedUsersListShowOtherManagedUsers");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.authorizedUsersListShowOtherManagedUsers = authorizedUsersListShowOtherManagedUsers;
return _x;
}
/**
* “List of applications, files, folders, and other items to launch when the user
* logs in. This collection can contain a maximum of 500 elements.”
*
* @return property autoLaunchItems
*/
@Property(name="autoLaunchItems")
@JsonIgnore
public CollectionPage getAutoLaunchItems() {
return new CollectionPage(contextPath, MacOSLaunchItem.class, this.autoLaunchItems, Optional.ofNullable(autoLaunchItemsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code autoLaunchItems}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “List of applications, files, folders, and other items to launch when the user
* logs in. This collection can contain a maximum of 500 elements.”
*
* @param autoLaunchItems
* new value of {@code autoLaunchItems} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code autoLaunchItems} field changed
*/
public MacOSDeviceFeaturesConfiguration withAutoLaunchItems(List autoLaunchItems) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("autoLaunchItems");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.autoLaunchItems = autoLaunchItems;
return _x;
}
/**
* “List of applications, files, folders, and other items to launch when the user
* logs in. This collection can contain a maximum of 500 elements.”
*
* @param options
* specify connect and read timeouts
* @return property autoLaunchItems
*/
@Property(name="autoLaunchItems")
@JsonIgnore
public CollectionPage getAutoLaunchItems(HttpRequestOptions options) {
return new CollectionPage(contextPath, MacOSLaunchItem.class, this.autoLaunchItems, Optional.ofNullable(autoLaunchItemsNextLink), Collections.emptyList(), options);
}
/**
* “Whether the Other user will disregard use of the `>console> special user name.”
*
* @return property consoleAccessDisabled
*/
@Property(name="consoleAccessDisabled")
@JsonIgnore
public Optional getConsoleAccessDisabled() {
return Optional.ofNullable(consoleAccessDisabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* consoleAccessDisabled} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Whether the Other user will disregard use of the `>console> special user name.”
*
* @param consoleAccessDisabled
* new value of {@code consoleAccessDisabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code consoleAccessDisabled} field changed
*/
public MacOSDeviceFeaturesConfiguration withConsoleAccessDisabled(Boolean consoleAccessDisabled) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("consoleAccessDisabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.consoleAccessDisabled = consoleAccessDisabled;
return _x;
}
/**
* “Prevents content caches from purging content to free up disk space for other
* apps.”
*
* @return property contentCachingBlockDeletion
*/
@Property(name="contentCachingBlockDeletion")
@JsonIgnore
public Optional getContentCachingBlockDeletion() {
return Optional.ofNullable(contentCachingBlockDeletion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingBlockDeletion} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Prevents content caches from purging content to free up disk space for other
* apps.”
*
* @param contentCachingBlockDeletion
* new value of {@code contentCachingBlockDeletion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingBlockDeletion} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingBlockDeletion(Boolean contentCachingBlockDeletion) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingBlockDeletion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingBlockDeletion = contentCachingBlockDeletion;
return _x;
}
/**
* “A list of custom IP ranges content caches will use to listen for clients. This
* collection can contain a maximum of 500 elements.”
*
* @return property contentCachingClientListenRanges
*/
@Property(name="contentCachingClientListenRanges")
@JsonIgnore
public CollectionPage getContentCachingClientListenRanges() {
return new CollectionPage(contextPath, IpRange.class, this.contentCachingClientListenRanges, Optional.ofNullable(contentCachingClientListenRangesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingClientListenRanges} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “A list of custom IP ranges content caches will use to listen for clients. This
* collection can contain a maximum of 500 elements.”
*
* @param contentCachingClientListenRanges
* new value of {@code contentCachingClientListenRanges} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingClientListenRanges} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingClientListenRanges(List contentCachingClientListenRanges) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingClientListenRanges");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingClientListenRanges = contentCachingClientListenRanges;
return _x;
}
/**
* “A list of custom IP ranges content caches will use to listen for clients. This
* collection can contain a maximum of 500 elements.”
*
* @param options
* specify connect and read timeouts
* @return property contentCachingClientListenRanges
*/
@Property(name="contentCachingClientListenRanges")
@JsonIgnore
public CollectionPage getContentCachingClientListenRanges(HttpRequestOptions options) {
return new CollectionPage(contextPath, IpRange.class, this.contentCachingClientListenRanges, Optional.ofNullable(contentCachingClientListenRangesNextLink), Collections.emptyList(), options);
}
/**
* “Determines the method in which content caching servers will listen for clients.”
*
* @return property contentCachingClientPolicy
*/
@Property(name="contentCachingClientPolicy")
@JsonIgnore
public Optional getContentCachingClientPolicy() {
return Optional.ofNullable(contentCachingClientPolicy);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingClientPolicy} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Determines the method in which content caching servers will listen for clients.”
*
* @param contentCachingClientPolicy
* new value of {@code contentCachingClientPolicy} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingClientPolicy} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingClientPolicy(MacOSContentCachingClientPolicy contentCachingClientPolicy) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingClientPolicy");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingClientPolicy = contentCachingClientPolicy;
return _x;
}
/**
* “The path to the directory used to store cached content. The value must be (or
* end with) /Library/Application Support/Apple/AssetCache/Data”
*
* @return property contentCachingDataPath
*/
@Property(name="contentCachingDataPath")
@JsonIgnore
public Optional getContentCachingDataPath() {
return Optional.ofNullable(contentCachingDataPath);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingDataPath} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The path to the directory used to store cached content. The value must be (or
* end with) /Library/Application Support/Apple/AssetCache/Data”
*
* @param contentCachingDataPath
* new value of {@code contentCachingDataPath} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingDataPath} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingDataPath(String contentCachingDataPath) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingDataPath");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingDataPath = contentCachingDataPath;
return _x;
}
/**
* “Disables internet connection sharing.”
*
* @return property contentCachingDisableConnectionSharing
*/
@Property(name="contentCachingDisableConnectionSharing")
@JsonIgnore
public Optional getContentCachingDisableConnectionSharing() {
return Optional.ofNullable(contentCachingDisableConnectionSharing);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingDisableConnectionSharing} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Disables internet connection sharing.”
*
* @param contentCachingDisableConnectionSharing
* new value of {@code contentCachingDisableConnectionSharing} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingDisableConnectionSharing} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingDisableConnectionSharing(Boolean contentCachingDisableConnectionSharing) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingDisableConnectionSharing");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingDisableConnectionSharing = contentCachingDisableConnectionSharing;
return _x;
}
/**
* “Enables content caching and prevents it from being disabled by the user.”
*
* @return property contentCachingEnabled
*/
@Property(name="contentCachingEnabled")
@JsonIgnore
public Optional getContentCachingEnabled() {
return Optional.ofNullable(contentCachingEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingEnabled} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Enables content caching and prevents it from being disabled by the user.”
*
* @param contentCachingEnabled
* new value of {@code contentCachingEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingEnabled} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingEnabled(Boolean contentCachingEnabled) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingEnabled = contentCachingEnabled;
return _x;
}
/**
* “Forces internet connection sharing. contentCachingDisableConnectionSharing
* overrides this setting.”
*
* @return property contentCachingForceConnectionSharing
*/
@Property(name="contentCachingForceConnectionSharing")
@JsonIgnore
public Optional getContentCachingForceConnectionSharing() {
return Optional.ofNullable(contentCachingForceConnectionSharing);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingForceConnectionSharing} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Forces internet connection sharing. contentCachingDisableConnectionSharing
* overrides this setting.”
*
* @param contentCachingForceConnectionSharing
* new value of {@code contentCachingForceConnectionSharing} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingForceConnectionSharing} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingForceConnectionSharing(Boolean contentCachingForceConnectionSharing) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingForceConnectionSharing");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingForceConnectionSharing = contentCachingForceConnectionSharing;
return _x;
}
/**
* “Prevent the device from sleeping if content caching is enabled.”
*
* @return property contentCachingKeepAwake
*/
@Property(name="contentCachingKeepAwake")
@JsonIgnore
public Optional getContentCachingKeepAwake() {
return Optional.ofNullable(contentCachingKeepAwake);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingKeepAwake} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Prevent the device from sleeping if content caching is enabled.”
*
* @param contentCachingKeepAwake
* new value of {@code contentCachingKeepAwake} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingKeepAwake} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingKeepAwake(Boolean contentCachingKeepAwake) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingKeepAwake");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingKeepAwake = contentCachingKeepAwake;
return _x;
}
/**
* “Enables logging of IP addresses and ports of clients that request cached content
* .”
*
* @return property contentCachingLogClientIdentities
*/
@Property(name="contentCachingLogClientIdentities")
@JsonIgnore
public Optional getContentCachingLogClientIdentities() {
return Optional.ofNullable(contentCachingLogClientIdentities);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingLogClientIdentities} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Enables logging of IP addresses and ports of clients that request cached content
* .”
*
* @param contentCachingLogClientIdentities
* new value of {@code contentCachingLogClientIdentities} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingLogClientIdentities} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingLogClientIdentities(Boolean contentCachingLogClientIdentities) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingLogClientIdentities");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingLogClientIdentities = contentCachingLogClientIdentities;
return _x;
}
/**
* “The maximum number of bytes of disk space that will be used for the content
* cache. A value of 0 (default) indicates unlimited disk space.”
*
* @return property contentCachingMaxSizeBytes
*/
@Property(name="contentCachingMaxSizeBytes")
@JsonIgnore
public Optional getContentCachingMaxSizeBytes() {
return Optional.ofNullable(contentCachingMaxSizeBytes);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingMaxSizeBytes} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The maximum number of bytes of disk space that will be used for the content
* cache. A value of 0 (default) indicates unlimited disk space.”
*
* @param contentCachingMaxSizeBytes
* new value of {@code contentCachingMaxSizeBytes} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingMaxSizeBytes} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingMaxSizeBytes(Integer contentCachingMaxSizeBytes) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingMaxSizeBytes");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingMaxSizeBytes = contentCachingMaxSizeBytes;
return _x;
}
/**
* “A list of IP addresses representing parent content caches.”
*
* @return property contentCachingParents
*/
@Property(name="contentCachingParents")
@JsonIgnore
public CollectionPage getContentCachingParents() {
return new CollectionPage(contextPath, String.class, this.contentCachingParents, Optional.ofNullable(contentCachingParentsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingParents} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “A list of IP addresses representing parent content caches.”
*
* @param contentCachingParents
* new value of {@code contentCachingParents} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingParents} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingParents(List contentCachingParents) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingParents");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingParents = contentCachingParents;
return _x;
}
/**
* “A list of IP addresses representing parent content caches.”
*
* @param options
* specify connect and read timeouts
* @return property contentCachingParents
*/
@Property(name="contentCachingParents")
@JsonIgnore
public CollectionPage getContentCachingParents(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.contentCachingParents, Optional.ofNullable(contentCachingParentsNextLink), Collections.emptyList(), options);
}
/**
* “Determines the method in which content caching servers will select parents if
* multiple are present.”
*
* @return property contentCachingParentSelectionPolicy
*/
@Property(name="contentCachingParentSelectionPolicy")
@JsonIgnore
public Optional getContentCachingParentSelectionPolicy() {
return Optional.ofNullable(contentCachingParentSelectionPolicy);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingParentSelectionPolicy} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Determines the method in which content caching servers will select parents if
* multiple are present.”
*
* @param contentCachingParentSelectionPolicy
* new value of {@code contentCachingParentSelectionPolicy} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingParentSelectionPolicy} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingParentSelectionPolicy(MacOSContentCachingParentSelectionPolicy contentCachingParentSelectionPolicy) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingParentSelectionPolicy");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingParentSelectionPolicy = contentCachingParentSelectionPolicy;
return _x;
}
/**
* “A list of custom IP ranges content caches will use to query for content from
* peers caches. This collection can contain a maximum of 500 elements.”
*
* @return property contentCachingPeerFilterRanges
*/
@Property(name="contentCachingPeerFilterRanges")
@JsonIgnore
public CollectionPage getContentCachingPeerFilterRanges() {
return new CollectionPage(contextPath, IpRange.class, this.contentCachingPeerFilterRanges, Optional.ofNullable(contentCachingPeerFilterRangesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingPeerFilterRanges} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “A list of custom IP ranges content caches will use to query for content from
* peers caches. This collection can contain a maximum of 500 elements.”
*
* @param contentCachingPeerFilterRanges
* new value of {@code contentCachingPeerFilterRanges} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingPeerFilterRanges} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingPeerFilterRanges(List contentCachingPeerFilterRanges) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingPeerFilterRanges");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingPeerFilterRanges = contentCachingPeerFilterRanges;
return _x;
}
/**
* “A list of custom IP ranges content caches will use to query for content from
* peers caches. This collection can contain a maximum of 500 elements.”
*
* @param options
* specify connect and read timeouts
* @return property contentCachingPeerFilterRanges
*/
@Property(name="contentCachingPeerFilterRanges")
@JsonIgnore
public CollectionPage getContentCachingPeerFilterRanges(HttpRequestOptions options) {
return new CollectionPage(contextPath, IpRange.class, this.contentCachingPeerFilterRanges, Optional.ofNullable(contentCachingPeerFilterRangesNextLink), Collections.emptyList(), options);
}
/**
* “A list of custom IP ranges content caches will use to listen for peer caches.
* This collection can contain a maximum of 500 elements.”
*
* @return property contentCachingPeerListenRanges
*/
@Property(name="contentCachingPeerListenRanges")
@JsonIgnore
public CollectionPage getContentCachingPeerListenRanges() {
return new CollectionPage(contextPath, IpRange.class, this.contentCachingPeerListenRanges, Optional.ofNullable(contentCachingPeerListenRangesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingPeerListenRanges} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “A list of custom IP ranges content caches will use to listen for peer caches.
* This collection can contain a maximum of 500 elements.”
*
* @param contentCachingPeerListenRanges
* new value of {@code contentCachingPeerListenRanges} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingPeerListenRanges} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingPeerListenRanges(List contentCachingPeerListenRanges) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingPeerListenRanges");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingPeerListenRanges = contentCachingPeerListenRanges;
return _x;
}
/**
* “A list of custom IP ranges content caches will use to listen for peer caches.
* This collection can contain a maximum of 500 elements.”
*
* @param options
* specify connect and read timeouts
* @return property contentCachingPeerListenRanges
*/
@Property(name="contentCachingPeerListenRanges")
@JsonIgnore
public CollectionPage getContentCachingPeerListenRanges(HttpRequestOptions options) {
return new CollectionPage(contextPath, IpRange.class, this.contentCachingPeerListenRanges, Optional.ofNullable(contentCachingPeerListenRangesNextLink), Collections.emptyList(), options);
}
/**
* “Determines the method in which content caches peer with other caches.”
*
* @return property contentCachingPeerPolicy
*/
@Property(name="contentCachingPeerPolicy")
@JsonIgnore
public Optional getContentCachingPeerPolicy() {
return Optional.ofNullable(contentCachingPeerPolicy);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingPeerPolicy} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Determines the method in which content caches peer with other caches.”
*
* @param contentCachingPeerPolicy
* new value of {@code contentCachingPeerPolicy} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingPeerPolicy} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingPeerPolicy(MacOSContentCachingPeerPolicy contentCachingPeerPolicy) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingPeerPolicy");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingPeerPolicy = contentCachingPeerPolicy;
return _x;
}
/**
* “Sets the port used for content caching. If the value is 0, a random available
* port will be selected. Valid values 0 to 65535”
*
* @return property contentCachingPort
*/
@Property(name="contentCachingPort")
@JsonIgnore
public Optional getContentCachingPort() {
return Optional.ofNullable(contentCachingPort);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingPort} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Sets the port used for content caching. If the value is 0, a random available
* port will be selected. Valid values 0 to 65535”
*
* @param contentCachingPort
* new value of {@code contentCachingPort} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingPort} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingPort(Integer contentCachingPort) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingPort");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingPort = contentCachingPort;
return _x;
}
/**
* “A list of custom IP ranges that Apple's content caching service should use to
* match clients to content caches. This collection can contain a maximum of 500
* elements.”
*
* @return property contentCachingPublicRanges
*/
@Property(name="contentCachingPublicRanges")
@JsonIgnore
public CollectionPage getContentCachingPublicRanges() {
return new CollectionPage(contextPath, IpRange.class, this.contentCachingPublicRanges, Optional.ofNullable(contentCachingPublicRangesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingPublicRanges} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “A list of custom IP ranges that Apple's content caching service should use to
* match clients to content caches. This collection can contain a maximum of 500
* elements.”
*
* @param contentCachingPublicRanges
* new value of {@code contentCachingPublicRanges} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingPublicRanges} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingPublicRanges(List contentCachingPublicRanges) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingPublicRanges");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingPublicRanges = contentCachingPublicRanges;
return _x;
}
/**
* “A list of custom IP ranges that Apple's content caching service should use to
* match clients to content caches. This collection can contain a maximum of 500
* elements.”
*
* @param options
* specify connect and read timeouts
* @return property contentCachingPublicRanges
*/
@Property(name="contentCachingPublicRanges")
@JsonIgnore
public CollectionPage getContentCachingPublicRanges(HttpRequestOptions options) {
return new CollectionPage(contextPath, IpRange.class, this.contentCachingPublicRanges, Optional.ofNullable(contentCachingPublicRangesNextLink), Collections.emptyList(), options);
}
/**
* “Display content caching alerts as system notifications.”
*
* @return property contentCachingShowAlerts
*/
@Property(name="contentCachingShowAlerts")
@JsonIgnore
public Optional getContentCachingShowAlerts() {
return Optional.ofNullable(contentCachingShowAlerts);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingShowAlerts} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Display content caching alerts as system notifications.”
*
* @param contentCachingShowAlerts
* new value of {@code contentCachingShowAlerts} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingShowAlerts} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingShowAlerts(Boolean contentCachingShowAlerts) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingShowAlerts");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingShowAlerts = contentCachingShowAlerts;
return _x;
}
/**
* “Determines what type of content is allowed to be cached by Apple's content
* caching service.”
*
* @return property contentCachingType
*/
@Property(name="contentCachingType")
@JsonIgnore
public Optional getContentCachingType() {
return Optional.ofNullable(contentCachingType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contentCachingType} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Determines what type of content is allowed to be cached by Apple's content
* caching service.”
*
* @param contentCachingType
* new value of {@code contentCachingType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentCachingType} field changed
*/
public MacOSDeviceFeaturesConfiguration withContentCachingType(MacOSContentCachingType contentCachingType) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("contentCachingType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.contentCachingType = contentCachingType;
return _x;
}
/**
* “Custom text to be displayed on the login window.”
*
* @return property loginWindowText
*/
@Property(name="loginWindowText")
@JsonIgnore
public Optional getLoginWindowText() {
return Optional.ofNullable(loginWindowText);
}
/**
* Returns an immutable copy of {@code this} with just the {@code loginWindowText}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Custom text to be displayed on the login window.”
*
* @param loginWindowText
* new value of {@code loginWindowText} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code loginWindowText} field changed
*/
public MacOSDeviceFeaturesConfiguration withLoginWindowText(String loginWindowText) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("loginWindowText");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.loginWindowText = loginWindowText;
return _x;
}
/**
* “Whether the Log Out menu item on the login window will be disabled while the
* user is logged in.”
*
* @return property logOutDisabledWhileLoggedIn
*/
@Property(name="logOutDisabledWhileLoggedIn")
@JsonIgnore
public Optional getLogOutDisabledWhileLoggedIn() {
return Optional.ofNullable(logOutDisabledWhileLoggedIn);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* logOutDisabledWhileLoggedIn} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Whether the Log Out menu item on the login window will be disabled while the
* user is logged in.”
*
* @param logOutDisabledWhileLoggedIn
* new value of {@code logOutDisabledWhileLoggedIn} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code logOutDisabledWhileLoggedIn} field changed
*/
public MacOSDeviceFeaturesConfiguration withLogOutDisabledWhileLoggedIn(Boolean logOutDisabledWhileLoggedIn) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("logOutDisabledWhileLoggedIn");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.logOutDisabledWhileLoggedIn = logOutDisabledWhileLoggedIn;
return _x;
}
/**
* “Gets or sets a single sign-on extension profile.”
*
* @return property macOSSingleSignOnExtension
*/
@Property(name="macOSSingleSignOnExtension")
@JsonIgnore
public Optional getMacOSSingleSignOnExtension() {
return Optional.ofNullable(macOSSingleSignOnExtension);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* macOSSingleSignOnExtension} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Gets or sets a single sign-on extension profile.”
*
* @param macOSSingleSignOnExtension
* new value of {@code macOSSingleSignOnExtension} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code macOSSingleSignOnExtension} field changed
*/
public MacOSDeviceFeaturesConfiguration withMacOSSingleSignOnExtension(MacOSSingleSignOnExtension macOSSingleSignOnExtension) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("macOSSingleSignOnExtension");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.macOSSingleSignOnExtension = macOSSingleSignOnExtension;
return _x;
}
/**
* “Whether the Power Off menu item on the login window will be disabled while the
* user is logged in.”
*
* @return property powerOffDisabledWhileLoggedIn
*/
@Property(name="powerOffDisabledWhileLoggedIn")
@JsonIgnore
public Optional getPowerOffDisabledWhileLoggedIn() {
return Optional.ofNullable(powerOffDisabledWhileLoggedIn);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* powerOffDisabledWhileLoggedIn} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Whether the Power Off menu item on the login window will be disabled while the
* user is logged in.”
*
* @param powerOffDisabledWhileLoggedIn
* new value of {@code powerOffDisabledWhileLoggedIn} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code powerOffDisabledWhileLoggedIn} field changed
*/
public MacOSDeviceFeaturesConfiguration withPowerOffDisabledWhileLoggedIn(Boolean powerOffDisabledWhileLoggedIn) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("powerOffDisabledWhileLoggedIn");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.powerOffDisabledWhileLoggedIn = powerOffDisabledWhileLoggedIn;
return _x;
}
/**
* “Whether to hide the Restart button item on the login window.”
*
* @return property restartDisabled
*/
@Property(name="restartDisabled")
@JsonIgnore
public Optional getRestartDisabled() {
return Optional.ofNullable(restartDisabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code restartDisabled}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Whether to hide the Restart button item on the login window.”
*
* @param restartDisabled
* new value of {@code restartDisabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code restartDisabled} field changed
*/
public MacOSDeviceFeaturesConfiguration withRestartDisabled(Boolean restartDisabled) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("restartDisabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.restartDisabled = restartDisabled;
return _x;
}
/**
* “Whether the Restart menu item on the login window will be disabled while the
* user is logged in.”
*
* @return property restartDisabledWhileLoggedIn
*/
@Property(name="restartDisabledWhileLoggedIn")
@JsonIgnore
public Optional getRestartDisabledWhileLoggedIn() {
return Optional.ofNullable(restartDisabledWhileLoggedIn);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* restartDisabledWhileLoggedIn} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Whether the Restart menu item on the login window will be disabled while the
* user is logged in.”
*
* @param restartDisabledWhileLoggedIn
* new value of {@code restartDisabledWhileLoggedIn} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code restartDisabledWhileLoggedIn} field changed
*/
public MacOSDeviceFeaturesConfiguration withRestartDisabledWhileLoggedIn(Boolean restartDisabledWhileLoggedIn) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("restartDisabledWhileLoggedIn");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.restartDisabledWhileLoggedIn = restartDisabledWhileLoggedIn;
return _x;
}
/**
* “Whether to disable the immediate screen lock functions.”
*
* @return property screenLockDisableImmediate
*/
@Property(name="screenLockDisableImmediate")
@JsonIgnore
public Optional getScreenLockDisableImmediate() {
return Optional.ofNullable(screenLockDisableImmediate);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* screenLockDisableImmediate} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Whether to disable the immediate screen lock functions.”
*
* @param screenLockDisableImmediate
* new value of {@code screenLockDisableImmediate} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code screenLockDisableImmediate} field changed
*/
public MacOSDeviceFeaturesConfiguration withScreenLockDisableImmediate(Boolean screenLockDisableImmediate) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("screenLockDisableImmediate");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.screenLockDisableImmediate = screenLockDisableImmediate;
return _x;
}
/**
* “Whether to hide the Shut Down button item on the login window.”
*
* @return property shutDownDisabled
*/
@Property(name="shutDownDisabled")
@JsonIgnore
public Optional getShutDownDisabled() {
return Optional.ofNullable(shutDownDisabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code shutDownDisabled}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Whether to hide the Shut Down button item on the login window.”
*
* @param shutDownDisabled
* new value of {@code shutDownDisabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code shutDownDisabled} field changed
*/
public MacOSDeviceFeaturesConfiguration withShutDownDisabled(Boolean shutDownDisabled) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("shutDownDisabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.shutDownDisabled = shutDownDisabled;
return _x;
}
/**
* “Whether the Shut Down menu item on the login window will be disabled while the
* user is logged in.”
*
* @return property shutDownDisabledWhileLoggedIn
*/
@Property(name="shutDownDisabledWhileLoggedIn")
@JsonIgnore
public Optional getShutDownDisabledWhileLoggedIn() {
return Optional.ofNullable(shutDownDisabledWhileLoggedIn);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* shutDownDisabledWhileLoggedIn} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Whether the Shut Down menu item on the login window will be disabled while the
* user is logged in.”
*
* @param shutDownDisabledWhileLoggedIn
* new value of {@code shutDownDisabledWhileLoggedIn} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code shutDownDisabledWhileLoggedIn} field changed
*/
public MacOSDeviceFeaturesConfiguration withShutDownDisabledWhileLoggedIn(Boolean shutDownDisabledWhileLoggedIn) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("shutDownDisabledWhileLoggedIn");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.shutDownDisabledWhileLoggedIn = shutDownDisabledWhileLoggedIn;
return _x;
}
/**
* “Gets or sets a single sign-on extension profile. Deprecated: use
* MacOSSingleSignOnExtension instead.”
*
* @return property singleSignOnExtension
*/
@Property(name="singleSignOnExtension")
@JsonIgnore
public Optional getSingleSignOnExtension() {
return Optional.ofNullable(singleSignOnExtension);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* singleSignOnExtension} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Gets or sets a single sign-on extension profile. Deprecated: use
* MacOSSingleSignOnExtension instead.”
*
* @param singleSignOnExtension
* new value of {@code singleSignOnExtension} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code singleSignOnExtension} field changed
*/
public MacOSDeviceFeaturesConfiguration withSingleSignOnExtension(SingleSignOnExtension singleSignOnExtension) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("singleSignOnExtension");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.singleSignOnExtension = singleSignOnExtension;
return _x;
}
/**
* “Whether to hide the Sleep menu item on the login window.”
*
* @return property sleepDisabled
*/
@Property(name="sleepDisabled")
@JsonIgnore
public Optional getSleepDisabled() {
return Optional.ofNullable(sleepDisabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code sleepDisabled}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Whether to hide the Sleep menu item on the login window.”
*
* @param sleepDisabled
* new value of {@code sleepDisabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code sleepDisabled} field changed
*/
public MacOSDeviceFeaturesConfiguration withSleepDisabled(Boolean sleepDisabled) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = changedFields.add("sleepDisabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSDeviceFeaturesConfiguration");
_x.sleepDisabled = sleepDisabled;
return _x;
}
public MacOSDeviceFeaturesConfiguration withUnmappedField(String name, String value) {
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “PKINIT Certificate for the authentication with single sign-on extensions.”
*
* @return navigational property singleSignOnExtensionPkinitCertificate
*/
@NavigationProperty(name="singleSignOnExtensionPkinitCertificate")
@JsonIgnore
public MacOSCertificateProfileBaseRequest getSingleSignOnExtensionPkinitCertificate() {
return new MacOSCertificateProfileBaseRequest(contextPath.addSegment("singleSignOnExtensionPkinitCertificate"), RequestHelper.getValue(unmappedFields, "singleSignOnExtensionPkinitCertificate"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public MacOSDeviceFeaturesConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public MacOSDeviceFeaturesConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
MacOSDeviceFeaturesConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private MacOSDeviceFeaturesConfiguration _copy() {
MacOSDeviceFeaturesConfiguration _x = new MacOSDeviceFeaturesConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.airPrintDestinations = airPrintDestinations;
_x.adminShowHostInfo = adminShowHostInfo;
_x.appAssociatedDomains = appAssociatedDomains;
_x.associatedDomains = associatedDomains;
_x.authorizedUsersListHidden = authorizedUsersListHidden;
_x.authorizedUsersListHideAdminUsers = authorizedUsersListHideAdminUsers;
_x.authorizedUsersListHideLocalUsers = authorizedUsersListHideLocalUsers;
_x.authorizedUsersListHideMobileAccounts = authorizedUsersListHideMobileAccounts;
_x.authorizedUsersListIncludeNetworkUsers = authorizedUsersListIncludeNetworkUsers;
_x.authorizedUsersListShowOtherManagedUsers = authorizedUsersListShowOtherManagedUsers;
_x.autoLaunchItems = autoLaunchItems;
_x.consoleAccessDisabled = consoleAccessDisabled;
_x.contentCachingBlockDeletion = contentCachingBlockDeletion;
_x.contentCachingClientListenRanges = contentCachingClientListenRanges;
_x.contentCachingClientPolicy = contentCachingClientPolicy;
_x.contentCachingDataPath = contentCachingDataPath;
_x.contentCachingDisableConnectionSharing = contentCachingDisableConnectionSharing;
_x.contentCachingEnabled = contentCachingEnabled;
_x.contentCachingForceConnectionSharing = contentCachingForceConnectionSharing;
_x.contentCachingKeepAwake = contentCachingKeepAwake;
_x.contentCachingLogClientIdentities = contentCachingLogClientIdentities;
_x.contentCachingMaxSizeBytes = contentCachingMaxSizeBytes;
_x.contentCachingParents = contentCachingParents;
_x.contentCachingParentSelectionPolicy = contentCachingParentSelectionPolicy;
_x.contentCachingPeerFilterRanges = contentCachingPeerFilterRanges;
_x.contentCachingPeerListenRanges = contentCachingPeerListenRanges;
_x.contentCachingPeerPolicy = contentCachingPeerPolicy;
_x.contentCachingPort = contentCachingPort;
_x.contentCachingPublicRanges = contentCachingPublicRanges;
_x.contentCachingShowAlerts = contentCachingShowAlerts;
_x.contentCachingType = contentCachingType;
_x.loginWindowText = loginWindowText;
_x.logOutDisabledWhileLoggedIn = logOutDisabledWhileLoggedIn;
_x.macOSSingleSignOnExtension = macOSSingleSignOnExtension;
_x.powerOffDisabledWhileLoggedIn = powerOffDisabledWhileLoggedIn;
_x.restartDisabled = restartDisabled;
_x.restartDisabledWhileLoggedIn = restartDisabledWhileLoggedIn;
_x.screenLockDisableImmediate = screenLockDisableImmediate;
_x.shutDownDisabled = shutDownDisabled;
_x.shutDownDisabledWhileLoggedIn = shutDownDisabledWhileLoggedIn;
_x.singleSignOnExtension = singleSignOnExtension;
_x.sleepDisabled = sleepDisabled;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("MacOSDeviceFeaturesConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("deviceManagementApplicabilityRuleDeviceMode=");
b.append(this.deviceManagementApplicabilityRuleDeviceMode);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsEdition=");
b.append(this.deviceManagementApplicabilityRuleOsEdition);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsVersion=");
b.append(this.deviceManagementApplicabilityRuleOsVersion);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("supportsScopeTags=");
b.append(this.supportsScopeTags);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("airPrintDestinations=");
b.append(this.airPrintDestinations);
b.append(", ");
b.append("adminShowHostInfo=");
b.append(this.adminShowHostInfo);
b.append(", ");
b.append("appAssociatedDomains=");
b.append(this.appAssociatedDomains);
b.append(", ");
b.append("associatedDomains=");
b.append(this.associatedDomains);
b.append(", ");
b.append("authorizedUsersListHidden=");
b.append(this.authorizedUsersListHidden);
b.append(", ");
b.append("authorizedUsersListHideAdminUsers=");
b.append(this.authorizedUsersListHideAdminUsers);
b.append(", ");
b.append("authorizedUsersListHideLocalUsers=");
b.append(this.authorizedUsersListHideLocalUsers);
b.append(", ");
b.append("authorizedUsersListHideMobileAccounts=");
b.append(this.authorizedUsersListHideMobileAccounts);
b.append(", ");
b.append("authorizedUsersListIncludeNetworkUsers=");
b.append(this.authorizedUsersListIncludeNetworkUsers);
b.append(", ");
b.append("authorizedUsersListShowOtherManagedUsers=");
b.append(this.authorizedUsersListShowOtherManagedUsers);
b.append(", ");
b.append("autoLaunchItems=");
b.append(this.autoLaunchItems);
b.append(", ");
b.append("consoleAccessDisabled=");
b.append(this.consoleAccessDisabled);
b.append(", ");
b.append("contentCachingBlockDeletion=");
b.append(this.contentCachingBlockDeletion);
b.append(", ");
b.append("contentCachingClientListenRanges=");
b.append(this.contentCachingClientListenRanges);
b.append(", ");
b.append("contentCachingClientPolicy=");
b.append(this.contentCachingClientPolicy);
b.append(", ");
b.append("contentCachingDataPath=");
b.append(this.contentCachingDataPath);
b.append(", ");
b.append("contentCachingDisableConnectionSharing=");
b.append(this.contentCachingDisableConnectionSharing);
b.append(", ");
b.append("contentCachingEnabled=");
b.append(this.contentCachingEnabled);
b.append(", ");
b.append("contentCachingForceConnectionSharing=");
b.append(this.contentCachingForceConnectionSharing);
b.append(", ");
b.append("contentCachingKeepAwake=");
b.append(this.contentCachingKeepAwake);
b.append(", ");
b.append("contentCachingLogClientIdentities=");
b.append(this.contentCachingLogClientIdentities);
b.append(", ");
b.append("contentCachingMaxSizeBytes=");
b.append(this.contentCachingMaxSizeBytes);
b.append(", ");
b.append("contentCachingParents=");
b.append(this.contentCachingParents);
b.append(", ");
b.append("contentCachingParentSelectionPolicy=");
b.append(this.contentCachingParentSelectionPolicy);
b.append(", ");
b.append("contentCachingPeerFilterRanges=");
b.append(this.contentCachingPeerFilterRanges);
b.append(", ");
b.append("contentCachingPeerListenRanges=");
b.append(this.contentCachingPeerListenRanges);
b.append(", ");
b.append("contentCachingPeerPolicy=");
b.append(this.contentCachingPeerPolicy);
b.append(", ");
b.append("contentCachingPort=");
b.append(this.contentCachingPort);
b.append(", ");
b.append("contentCachingPublicRanges=");
b.append(this.contentCachingPublicRanges);
b.append(", ");
b.append("contentCachingShowAlerts=");
b.append(this.contentCachingShowAlerts);
b.append(", ");
b.append("contentCachingType=");
b.append(this.contentCachingType);
b.append(", ");
b.append("loginWindowText=");
b.append(this.loginWindowText);
b.append(", ");
b.append("logOutDisabledWhileLoggedIn=");
b.append(this.logOutDisabledWhileLoggedIn);
b.append(", ");
b.append("macOSSingleSignOnExtension=");
b.append(this.macOSSingleSignOnExtension);
b.append(", ");
b.append("powerOffDisabledWhileLoggedIn=");
b.append(this.powerOffDisabledWhileLoggedIn);
b.append(", ");
b.append("restartDisabled=");
b.append(this.restartDisabled);
b.append(", ");
b.append("restartDisabledWhileLoggedIn=");
b.append(this.restartDisabledWhileLoggedIn);
b.append(", ");
b.append("screenLockDisableImmediate=");
b.append(this.screenLockDisableImmediate);
b.append(", ");
b.append("shutDownDisabled=");
b.append(this.shutDownDisabled);
b.append(", ");
b.append("shutDownDisabledWhileLoggedIn=");
b.append(this.shutDownDisabledWhileLoggedIn);
b.append(", ");
b.append("singleSignOnExtension=");
b.append(this.singleSignOnExtension);
b.append(", ");
b.append("sleepDisabled=");
b.append(this.sleepDisabled);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}