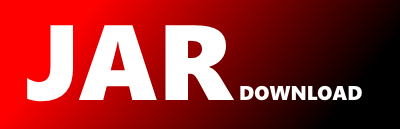
odata.msgraph.client.beta.entity.MacOSEndpointProtectionConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleDeviceMode;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsEdition;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsVersion;
import odata.msgraph.client.beta.complex.MacOSFirewallApplication;
import odata.msgraph.client.beta.enums.Enablement;
import odata.msgraph.client.beta.enums.MacOSFileVaultRecoveryKeyTypes;
import odata.msgraph.client.beta.enums.MacOSGatekeeperAppSources;
/**
* “MacOS endpoint protection configuration profile.”
*/@JsonPropertyOrder({
"@odata.type",
"advancedThreatProtectionAutomaticSampleSubmission",
"advancedThreatProtectionCloudDelivered",
"advancedThreatProtectionDiagnosticDataCollection",
"advancedThreatProtectionExcludedExtensions",
"advancedThreatProtectionExcludedFiles",
"advancedThreatProtectionExcludedFolders",
"advancedThreatProtectionExcludedProcesses",
"advancedThreatProtectionRealTime",
"fileVaultAllowDeferralUntilSignOut",
"fileVaultDisablePromptAtSignOut",
"fileVaultEnabled",
"fileVaultHidePersonalRecoveryKey",
"fileVaultInstitutionalRecoveryKeyCertificate",
"fileVaultInstitutionalRecoveryKeyCertificateFileName",
"fileVaultNumberOfTimesUserCanIgnore",
"fileVaultPersonalRecoveryKeyHelpMessage",
"fileVaultPersonalRecoveryKeyRotationInMonths",
"fileVaultSelectedRecoveryKeyTypes",
"firewallApplications",
"firewallBlockAllIncoming",
"firewallEnabled",
"firewallEnableStealthMode",
"gatekeeperAllowedAppSource",
"gatekeeperBlockOverride"})
@JsonInclude(Include.NON_NULL)
public class MacOSEndpointProtectionConfiguration extends DeviceConfiguration implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.macOSEndpointProtectionConfiguration";
}
@JsonProperty("advancedThreatProtectionAutomaticSampleSubmission")
protected Enablement advancedThreatProtectionAutomaticSampleSubmission;
@JsonProperty("advancedThreatProtectionCloudDelivered")
protected Enablement advancedThreatProtectionCloudDelivered;
@JsonProperty("advancedThreatProtectionDiagnosticDataCollection")
protected Enablement advancedThreatProtectionDiagnosticDataCollection;
@JsonProperty("advancedThreatProtectionExcludedExtensions")
protected List advancedThreatProtectionExcludedExtensions;
@JsonProperty("advancedThreatProtectionExcludedExtensions@nextLink")
protected String advancedThreatProtectionExcludedExtensionsNextLink;
@JsonProperty("advancedThreatProtectionExcludedFiles")
protected List advancedThreatProtectionExcludedFiles;
@JsonProperty("advancedThreatProtectionExcludedFiles@nextLink")
protected String advancedThreatProtectionExcludedFilesNextLink;
@JsonProperty("advancedThreatProtectionExcludedFolders")
protected List advancedThreatProtectionExcludedFolders;
@JsonProperty("advancedThreatProtectionExcludedFolders@nextLink")
protected String advancedThreatProtectionExcludedFoldersNextLink;
@JsonProperty("advancedThreatProtectionExcludedProcesses")
protected List advancedThreatProtectionExcludedProcesses;
@JsonProperty("advancedThreatProtectionExcludedProcesses@nextLink")
protected String advancedThreatProtectionExcludedProcessesNextLink;
@JsonProperty("advancedThreatProtectionRealTime")
protected Enablement advancedThreatProtectionRealTime;
@JsonProperty("fileVaultAllowDeferralUntilSignOut")
protected Boolean fileVaultAllowDeferralUntilSignOut;
@JsonProperty("fileVaultDisablePromptAtSignOut")
protected Boolean fileVaultDisablePromptAtSignOut;
@JsonProperty("fileVaultEnabled")
protected Boolean fileVaultEnabled;
@JsonProperty("fileVaultHidePersonalRecoveryKey")
protected Boolean fileVaultHidePersonalRecoveryKey;
@JsonProperty("fileVaultInstitutionalRecoveryKeyCertificate")
protected byte[] fileVaultInstitutionalRecoveryKeyCertificate;
@JsonProperty("fileVaultInstitutionalRecoveryKeyCertificateFileName")
protected String fileVaultInstitutionalRecoveryKeyCertificateFileName;
@JsonProperty("fileVaultNumberOfTimesUserCanIgnore")
protected Integer fileVaultNumberOfTimesUserCanIgnore;
@JsonProperty("fileVaultPersonalRecoveryKeyHelpMessage")
protected String fileVaultPersonalRecoveryKeyHelpMessage;
@JsonProperty("fileVaultPersonalRecoveryKeyRotationInMonths")
protected Integer fileVaultPersonalRecoveryKeyRotationInMonths;
@JsonProperty("fileVaultSelectedRecoveryKeyTypes")
protected MacOSFileVaultRecoveryKeyTypes fileVaultSelectedRecoveryKeyTypes;
@JsonProperty("firewallApplications")
protected List firewallApplications;
@JsonProperty("firewallApplications@nextLink")
protected String firewallApplicationsNextLink;
@JsonProperty("firewallBlockAllIncoming")
protected Boolean firewallBlockAllIncoming;
@JsonProperty("firewallEnabled")
protected Boolean firewallEnabled;
@JsonProperty("firewallEnableStealthMode")
protected Boolean firewallEnableStealthMode;
@JsonProperty("gatekeeperAllowedAppSource")
protected MacOSGatekeeperAppSources gatekeeperAllowedAppSource;
@JsonProperty("gatekeeperBlockOverride")
protected Boolean gatekeeperBlockOverride;
protected MacOSEndpointProtectionConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderMacOSEndpointProtectionConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode;
private DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition;
private DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private Boolean supportsScopeTags;
private Integer version;
private Enablement advancedThreatProtectionAutomaticSampleSubmission;
private Enablement advancedThreatProtectionCloudDelivered;
private Enablement advancedThreatProtectionDiagnosticDataCollection;
private List advancedThreatProtectionExcludedExtensions;
private String advancedThreatProtectionExcludedExtensionsNextLink;
private List advancedThreatProtectionExcludedFiles;
private String advancedThreatProtectionExcludedFilesNextLink;
private List advancedThreatProtectionExcludedFolders;
private String advancedThreatProtectionExcludedFoldersNextLink;
private List advancedThreatProtectionExcludedProcesses;
private String advancedThreatProtectionExcludedProcessesNextLink;
private Enablement advancedThreatProtectionRealTime;
private Boolean fileVaultAllowDeferralUntilSignOut;
private Boolean fileVaultDisablePromptAtSignOut;
private Boolean fileVaultEnabled;
private Boolean fileVaultHidePersonalRecoveryKey;
private byte[] fileVaultInstitutionalRecoveryKeyCertificate;
private String fileVaultInstitutionalRecoveryKeyCertificateFileName;
private Integer fileVaultNumberOfTimesUserCanIgnore;
private String fileVaultPersonalRecoveryKeyHelpMessage;
private Integer fileVaultPersonalRecoveryKeyRotationInMonths;
private MacOSFileVaultRecoveryKeyTypes fileVaultSelectedRecoveryKeyTypes;
private List firewallApplications;
private String firewallApplicationsNextLink;
private Boolean firewallBlockAllIncoming;
private Boolean firewallEnabled;
private Boolean firewallEnableStealthMode;
private MacOSGatekeeperAppSources gatekeeperAllowedAppSource;
private Boolean gatekeeperBlockOverride;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder deviceManagementApplicabilityRuleDeviceMode(DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode) {
this.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleDeviceMode");
return this;
}
public Builder deviceManagementApplicabilityRuleOsEdition(DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition) {
this.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsEdition");
return this;
}
public Builder deviceManagementApplicabilityRuleOsVersion(DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion) {
this.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsVersion");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder supportsScopeTags(Boolean supportsScopeTags) {
this.supportsScopeTags = supportsScopeTags;
this.changedFields = changedFields.add("supportsScopeTags");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
/**
* “Determines whether or not to enable automatic file sample submission for
* Microsoft Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionAutomaticSampleSubmission
* value of {@code advancedThreatProtectionAutomaticSampleSubmission} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionAutomaticSampleSubmission(Enablement advancedThreatProtectionAutomaticSampleSubmission) {
this.advancedThreatProtectionAutomaticSampleSubmission = advancedThreatProtectionAutomaticSampleSubmission;
this.changedFields = changedFields.add("advancedThreatProtectionAutomaticSampleSubmission");
return this;
}
/**
* “Determines whether or not to enable cloud-delivered protection for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionCloudDelivered
* value of {@code advancedThreatProtectionCloudDelivered} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionCloudDelivered(Enablement advancedThreatProtectionCloudDelivered) {
this.advancedThreatProtectionCloudDelivered = advancedThreatProtectionCloudDelivered;
this.changedFields = changedFields.add("advancedThreatProtectionCloudDelivered");
return this;
}
/**
* “Determines whether or not to enable diagnostic and usage data collection for
* Microsoft Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionDiagnosticDataCollection
* value of {@code advancedThreatProtectionDiagnosticDataCollection} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionDiagnosticDataCollection(Enablement advancedThreatProtectionDiagnosticDataCollection) {
this.advancedThreatProtectionDiagnosticDataCollection = advancedThreatProtectionDiagnosticDataCollection;
this.changedFields = changedFields.add("advancedThreatProtectionDiagnosticDataCollection");
return this;
}
/**
* “A list of file extensions to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedExtensions
* value of {@code advancedThreatProtectionExcludedExtensions} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionExcludedExtensions(List advancedThreatProtectionExcludedExtensions) {
this.advancedThreatProtectionExcludedExtensions = advancedThreatProtectionExcludedExtensions;
this.changedFields = changedFields.add("advancedThreatProtectionExcludedExtensions");
return this;
}
/**
* “A list of file extensions to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedExtensions
* value of {@code advancedThreatProtectionExcludedExtensions} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionExcludedExtensions(String... advancedThreatProtectionExcludedExtensions) {
return advancedThreatProtectionExcludedExtensions(Arrays.asList(advancedThreatProtectionExcludedExtensions));
}
/**
* “A list of file extensions to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedExtensionsNextLink
* value of {@code advancedThreatProtectionExcludedExtensions@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionExcludedExtensionsNextLink(String advancedThreatProtectionExcludedExtensionsNextLink) {
this.advancedThreatProtectionExcludedExtensionsNextLink = advancedThreatProtectionExcludedExtensionsNextLink;
this.changedFields = changedFields.add("advancedThreatProtectionExcludedExtensions");
return this;
}
/**
* “A list of paths to files to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedFiles
* value of {@code advancedThreatProtectionExcludedFiles} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionExcludedFiles(List advancedThreatProtectionExcludedFiles) {
this.advancedThreatProtectionExcludedFiles = advancedThreatProtectionExcludedFiles;
this.changedFields = changedFields.add("advancedThreatProtectionExcludedFiles");
return this;
}
/**
* “A list of paths to files to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedFiles
* value of {@code advancedThreatProtectionExcludedFiles} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionExcludedFiles(String... advancedThreatProtectionExcludedFiles) {
return advancedThreatProtectionExcludedFiles(Arrays.asList(advancedThreatProtectionExcludedFiles));
}
/**
* “A list of paths to files to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedFilesNextLink
* value of {@code advancedThreatProtectionExcludedFiles@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionExcludedFilesNextLink(String advancedThreatProtectionExcludedFilesNextLink) {
this.advancedThreatProtectionExcludedFilesNextLink = advancedThreatProtectionExcludedFilesNextLink;
this.changedFields = changedFields.add("advancedThreatProtectionExcludedFiles");
return this;
}
/**
* “A list of paths to folders to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedFolders
* value of {@code advancedThreatProtectionExcludedFolders} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionExcludedFolders(List advancedThreatProtectionExcludedFolders) {
this.advancedThreatProtectionExcludedFolders = advancedThreatProtectionExcludedFolders;
this.changedFields = changedFields.add("advancedThreatProtectionExcludedFolders");
return this;
}
/**
* “A list of paths to folders to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedFolders
* value of {@code advancedThreatProtectionExcludedFolders} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionExcludedFolders(String... advancedThreatProtectionExcludedFolders) {
return advancedThreatProtectionExcludedFolders(Arrays.asList(advancedThreatProtectionExcludedFolders));
}
/**
* “A list of paths to folders to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedFoldersNextLink
* value of {@code advancedThreatProtectionExcludedFolders@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionExcludedFoldersNextLink(String advancedThreatProtectionExcludedFoldersNextLink) {
this.advancedThreatProtectionExcludedFoldersNextLink = advancedThreatProtectionExcludedFoldersNextLink;
this.changedFields = changedFields.add("advancedThreatProtectionExcludedFolders");
return this;
}
/**
* “A list of process names to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedProcesses
* value of {@code advancedThreatProtectionExcludedProcesses} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionExcludedProcesses(List advancedThreatProtectionExcludedProcesses) {
this.advancedThreatProtectionExcludedProcesses = advancedThreatProtectionExcludedProcesses;
this.changedFields = changedFields.add("advancedThreatProtectionExcludedProcesses");
return this;
}
/**
* “A list of process names to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedProcesses
* value of {@code advancedThreatProtectionExcludedProcesses} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionExcludedProcesses(String... advancedThreatProtectionExcludedProcesses) {
return advancedThreatProtectionExcludedProcesses(Arrays.asList(advancedThreatProtectionExcludedProcesses));
}
/**
* “A list of process names to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedProcessesNextLink
* value of {@code advancedThreatProtectionExcludedProcesses@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionExcludedProcessesNextLink(String advancedThreatProtectionExcludedProcessesNextLink) {
this.advancedThreatProtectionExcludedProcessesNextLink = advancedThreatProtectionExcludedProcessesNextLink;
this.changedFields = changedFields.add("advancedThreatProtectionExcludedProcesses");
return this;
}
/**
* “Determines whether or not to enable real-time protection for Microsoft Defender
* Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionRealTime
* value of {@code advancedThreatProtectionRealTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder advancedThreatProtectionRealTime(Enablement advancedThreatProtectionRealTime) {
this.advancedThreatProtectionRealTime = advancedThreatProtectionRealTime;
this.changedFields = changedFields.add("advancedThreatProtectionRealTime");
return this;
}
/**
* “Optional. If set to true, the user can defer the enabling of FileVault until
* they sign out.”
*
* @param fileVaultAllowDeferralUntilSignOut
* value of {@code fileVaultAllowDeferralUntilSignOut} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder fileVaultAllowDeferralUntilSignOut(Boolean fileVaultAllowDeferralUntilSignOut) {
this.fileVaultAllowDeferralUntilSignOut = fileVaultAllowDeferralUntilSignOut;
this.changedFields = changedFields.add("fileVaultAllowDeferralUntilSignOut");
return this;
}
/**
* “Optional. When using the Defer option, if set to true, the user is not prompted
* to enable FileVault at sign-out.”
*
* @param fileVaultDisablePromptAtSignOut
* value of {@code fileVaultDisablePromptAtSignOut} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder fileVaultDisablePromptAtSignOut(Boolean fileVaultDisablePromptAtSignOut) {
this.fileVaultDisablePromptAtSignOut = fileVaultDisablePromptAtSignOut;
this.changedFields = changedFields.add("fileVaultDisablePromptAtSignOut");
return this;
}
/**
* “Whether FileVault should be enabled or not.”
*
* @param fileVaultEnabled
* value of {@code fileVaultEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder fileVaultEnabled(Boolean fileVaultEnabled) {
this.fileVaultEnabled = fileVaultEnabled;
this.changedFields = changedFields.add("fileVaultEnabled");
return this;
}
/**
* “Optional. A hidden personal recovery key does not appear on the user's screen
* during FileVault encryption, reducing the risk of it ending up in the wrong
* hands.”
*
* @param fileVaultHidePersonalRecoveryKey
* value of {@code fileVaultHidePersonalRecoveryKey} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder fileVaultHidePersonalRecoveryKey(Boolean fileVaultHidePersonalRecoveryKey) {
this.fileVaultHidePersonalRecoveryKey = fileVaultHidePersonalRecoveryKey;
this.changedFields = changedFields.add("fileVaultHidePersonalRecoveryKey");
return this;
}
/**
* “Required if selected recovery key type(s) include InstitutionalRecoveryKey. The
* DER Encoded certificate file used to set an institutional recovery key.”
*
* @param fileVaultInstitutionalRecoveryKeyCertificate
* value of {@code fileVaultInstitutionalRecoveryKeyCertificate} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder fileVaultInstitutionalRecoveryKeyCertificate(byte[] fileVaultInstitutionalRecoveryKeyCertificate) {
this.fileVaultInstitutionalRecoveryKeyCertificate = fileVaultInstitutionalRecoveryKeyCertificate;
this.changedFields = changedFields.add("fileVaultInstitutionalRecoveryKeyCertificate");
return this;
}
/**
* “File name of the institutional recovery key certificate to display in UI. (*.der
* ).”
*
* @param fileVaultInstitutionalRecoveryKeyCertificateFileName
* value of {@code fileVaultInstitutionalRecoveryKeyCertificateFileName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder fileVaultInstitutionalRecoveryKeyCertificateFileName(String fileVaultInstitutionalRecoveryKeyCertificateFileName) {
this.fileVaultInstitutionalRecoveryKeyCertificateFileName = fileVaultInstitutionalRecoveryKeyCertificateFileName;
this.changedFields = changedFields.add("fileVaultInstitutionalRecoveryKeyCertificateFileName");
return this;
}
/**
* “Optional. When using the Defer option, this is the maximum number of times the
* user can ignore prompts to enable FileVault before FileVault will be required
* for the user to sign in. If set to -1, it will always prompt to enable FileVault
* until FileVault is enabled, though it will allow the user to bypass enabling
* FileVault. Setting this to 0 will disable the feature.”
*
* @param fileVaultNumberOfTimesUserCanIgnore
* value of {@code fileVaultNumberOfTimesUserCanIgnore} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder fileVaultNumberOfTimesUserCanIgnore(Integer fileVaultNumberOfTimesUserCanIgnore) {
this.fileVaultNumberOfTimesUserCanIgnore = fileVaultNumberOfTimesUserCanIgnore;
this.changedFields = changedFields.add("fileVaultNumberOfTimesUserCanIgnore");
return this;
}
/**
* “Required if selected recovery key type(s) include PersonalRecoveryKey. A short
* message displayed to the user that explains how they can retrieve their personal
* recovery key.”
*
* @param fileVaultPersonalRecoveryKeyHelpMessage
* value of {@code fileVaultPersonalRecoveryKeyHelpMessage} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder fileVaultPersonalRecoveryKeyHelpMessage(String fileVaultPersonalRecoveryKeyHelpMessage) {
this.fileVaultPersonalRecoveryKeyHelpMessage = fileVaultPersonalRecoveryKeyHelpMessage;
this.changedFields = changedFields.add("fileVaultPersonalRecoveryKeyHelpMessage");
return this;
}
/**
* “Optional. If selected recovery key type(s) include PersonalRecoveryKey, the
* frequency to rotate that key, in months.”
*
* @param fileVaultPersonalRecoveryKeyRotationInMonths
* value of {@code fileVaultPersonalRecoveryKeyRotationInMonths} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder fileVaultPersonalRecoveryKeyRotationInMonths(Integer fileVaultPersonalRecoveryKeyRotationInMonths) {
this.fileVaultPersonalRecoveryKeyRotationInMonths = fileVaultPersonalRecoveryKeyRotationInMonths;
this.changedFields = changedFields.add("fileVaultPersonalRecoveryKeyRotationInMonths");
return this;
}
/**
* “Required if FileVault is enabled, determines the type(s) of recovery key to use.”
*
* @param fileVaultSelectedRecoveryKeyTypes
* value of {@code fileVaultSelectedRecoveryKeyTypes} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder fileVaultSelectedRecoveryKeyTypes(MacOSFileVaultRecoveryKeyTypes fileVaultSelectedRecoveryKeyTypes) {
this.fileVaultSelectedRecoveryKeyTypes = fileVaultSelectedRecoveryKeyTypes;
this.changedFields = changedFields.add("fileVaultSelectedRecoveryKeyTypes");
return this;
}
/**
* “List of applications with firewall settings. Firewall settings for applications
* not on this list are determined by the user. This collection can contain a
* maximum of 500 elements.”
*
* @param firewallApplications
* value of {@code firewallApplications} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder firewallApplications(List firewallApplications) {
this.firewallApplications = firewallApplications;
this.changedFields = changedFields.add("firewallApplications");
return this;
}
/**
* “List of applications with firewall settings. Firewall settings for applications
* not on this list are determined by the user. This collection can contain a
* maximum of 500 elements.”
*
* @param firewallApplications
* value of {@code firewallApplications} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder firewallApplications(MacOSFirewallApplication... firewallApplications) {
return firewallApplications(Arrays.asList(firewallApplications));
}
/**
* “List of applications with firewall settings. Firewall settings for applications
* not on this list are determined by the user. This collection can contain a
* maximum of 500 elements.”
*
* @param firewallApplicationsNextLink
* value of {@code firewallApplications@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder firewallApplicationsNextLink(String firewallApplicationsNextLink) {
this.firewallApplicationsNextLink = firewallApplicationsNextLink;
this.changedFields = changedFields.add("firewallApplications");
return this;
}
/**
* “Corresponds to the “Block all incoming connections” option.”
*
* @param firewallBlockAllIncoming
* value of {@code firewallBlockAllIncoming} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder firewallBlockAllIncoming(Boolean firewallBlockAllIncoming) {
this.firewallBlockAllIncoming = firewallBlockAllIncoming;
this.changedFields = changedFields.add("firewallBlockAllIncoming");
return this;
}
/**
* “Whether the firewall should be enabled or not.”
*
* @param firewallEnabled
* value of {@code firewallEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder firewallEnabled(Boolean firewallEnabled) {
this.firewallEnabled = firewallEnabled;
this.changedFields = changedFields.add("firewallEnabled");
return this;
}
/**
* “Corresponds to “Enable stealth mode.””
*
* @param firewallEnableStealthMode
* value of {@code firewallEnableStealthMode} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder firewallEnableStealthMode(Boolean firewallEnableStealthMode) {
this.firewallEnableStealthMode = firewallEnableStealthMode;
this.changedFields = changedFields.add("firewallEnableStealthMode");
return this;
}
/**
* “System and Privacy setting that determines which download locations apps can be
* run from on a macOS device.”
*
* @param gatekeeperAllowedAppSource
* value of {@code gatekeeperAllowedAppSource} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder gatekeeperAllowedAppSource(MacOSGatekeeperAppSources gatekeeperAllowedAppSource) {
this.gatekeeperAllowedAppSource = gatekeeperAllowedAppSource;
this.changedFields = changedFields.add("gatekeeperAllowedAppSource");
return this;
}
/**
* “If set to true, the user override for Gatekeeper will be disabled.”
*
* @param gatekeeperBlockOverride
* value of {@code gatekeeperBlockOverride} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder gatekeeperBlockOverride(Boolean gatekeeperBlockOverride) {
this.gatekeeperBlockOverride = gatekeeperBlockOverride;
this.changedFields = changedFields.add("gatekeeperBlockOverride");
return this;
}
public MacOSEndpointProtectionConfiguration build() {
MacOSEndpointProtectionConfiguration _x = new MacOSEndpointProtectionConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.macOSEndpointProtectionConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.advancedThreatProtectionAutomaticSampleSubmission = advancedThreatProtectionAutomaticSampleSubmission;
_x.advancedThreatProtectionCloudDelivered = advancedThreatProtectionCloudDelivered;
_x.advancedThreatProtectionDiagnosticDataCollection = advancedThreatProtectionDiagnosticDataCollection;
_x.advancedThreatProtectionExcludedExtensions = advancedThreatProtectionExcludedExtensions;
_x.advancedThreatProtectionExcludedExtensionsNextLink = advancedThreatProtectionExcludedExtensionsNextLink;
_x.advancedThreatProtectionExcludedFiles = advancedThreatProtectionExcludedFiles;
_x.advancedThreatProtectionExcludedFilesNextLink = advancedThreatProtectionExcludedFilesNextLink;
_x.advancedThreatProtectionExcludedFolders = advancedThreatProtectionExcludedFolders;
_x.advancedThreatProtectionExcludedFoldersNextLink = advancedThreatProtectionExcludedFoldersNextLink;
_x.advancedThreatProtectionExcludedProcesses = advancedThreatProtectionExcludedProcesses;
_x.advancedThreatProtectionExcludedProcessesNextLink = advancedThreatProtectionExcludedProcessesNextLink;
_x.advancedThreatProtectionRealTime = advancedThreatProtectionRealTime;
_x.fileVaultAllowDeferralUntilSignOut = fileVaultAllowDeferralUntilSignOut;
_x.fileVaultDisablePromptAtSignOut = fileVaultDisablePromptAtSignOut;
_x.fileVaultEnabled = fileVaultEnabled;
_x.fileVaultHidePersonalRecoveryKey = fileVaultHidePersonalRecoveryKey;
_x.fileVaultInstitutionalRecoveryKeyCertificate = fileVaultInstitutionalRecoveryKeyCertificate;
_x.fileVaultInstitutionalRecoveryKeyCertificateFileName = fileVaultInstitutionalRecoveryKeyCertificateFileName;
_x.fileVaultNumberOfTimesUserCanIgnore = fileVaultNumberOfTimesUserCanIgnore;
_x.fileVaultPersonalRecoveryKeyHelpMessage = fileVaultPersonalRecoveryKeyHelpMessage;
_x.fileVaultPersonalRecoveryKeyRotationInMonths = fileVaultPersonalRecoveryKeyRotationInMonths;
_x.fileVaultSelectedRecoveryKeyTypes = fileVaultSelectedRecoveryKeyTypes;
_x.firewallApplications = firewallApplications;
_x.firewallApplicationsNextLink = firewallApplicationsNextLink;
_x.firewallBlockAllIncoming = firewallBlockAllIncoming;
_x.firewallEnabled = firewallEnabled;
_x.firewallEnableStealthMode = firewallEnableStealthMode;
_x.gatekeeperAllowedAppSource = gatekeeperAllowedAppSource;
_x.gatekeeperBlockOverride = gatekeeperBlockOverride;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Determines whether or not to enable automatic file sample submission for
* Microsoft Defender Advanced Threat Protection on macOS.”
*
* @return property advancedThreatProtectionAutomaticSampleSubmission
*/
@Property(name="advancedThreatProtectionAutomaticSampleSubmission")
@JsonIgnore
public Optional getAdvancedThreatProtectionAutomaticSampleSubmission() {
return Optional.ofNullable(advancedThreatProtectionAutomaticSampleSubmission);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* advancedThreatProtectionAutomaticSampleSubmission} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “Determines whether or not to enable automatic file sample submission for
* Microsoft Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionAutomaticSampleSubmission
* new value of {@code advancedThreatProtectionAutomaticSampleSubmission} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code advancedThreatProtectionAutomaticSampleSubmission} field changed
*/
public MacOSEndpointProtectionConfiguration withAdvancedThreatProtectionAutomaticSampleSubmission(Enablement advancedThreatProtectionAutomaticSampleSubmission) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("advancedThreatProtectionAutomaticSampleSubmission");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.advancedThreatProtectionAutomaticSampleSubmission = advancedThreatProtectionAutomaticSampleSubmission;
return _x;
}
/**
* “Determines whether or not to enable cloud-delivered protection for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @return property advancedThreatProtectionCloudDelivered
*/
@Property(name="advancedThreatProtectionCloudDelivered")
@JsonIgnore
public Optional getAdvancedThreatProtectionCloudDelivered() {
return Optional.ofNullable(advancedThreatProtectionCloudDelivered);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* advancedThreatProtectionCloudDelivered} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Determines whether or not to enable cloud-delivered protection for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionCloudDelivered
* new value of {@code advancedThreatProtectionCloudDelivered} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code advancedThreatProtectionCloudDelivered} field changed
*/
public MacOSEndpointProtectionConfiguration withAdvancedThreatProtectionCloudDelivered(Enablement advancedThreatProtectionCloudDelivered) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("advancedThreatProtectionCloudDelivered");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.advancedThreatProtectionCloudDelivered = advancedThreatProtectionCloudDelivered;
return _x;
}
/**
* “Determines whether or not to enable diagnostic and usage data collection for
* Microsoft Defender Advanced Threat Protection on macOS.”
*
* @return property advancedThreatProtectionDiagnosticDataCollection
*/
@Property(name="advancedThreatProtectionDiagnosticDataCollection")
@JsonIgnore
public Optional getAdvancedThreatProtectionDiagnosticDataCollection() {
return Optional.ofNullable(advancedThreatProtectionDiagnosticDataCollection);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* advancedThreatProtectionDiagnosticDataCollection} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “Determines whether or not to enable diagnostic and usage data collection for
* Microsoft Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionDiagnosticDataCollection
* new value of {@code advancedThreatProtectionDiagnosticDataCollection} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code advancedThreatProtectionDiagnosticDataCollection} field changed
*/
public MacOSEndpointProtectionConfiguration withAdvancedThreatProtectionDiagnosticDataCollection(Enablement advancedThreatProtectionDiagnosticDataCollection) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("advancedThreatProtectionDiagnosticDataCollection");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.advancedThreatProtectionDiagnosticDataCollection = advancedThreatProtectionDiagnosticDataCollection;
return _x;
}
/**
* “A list of file extensions to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @return property advancedThreatProtectionExcludedExtensions
*/
@Property(name="advancedThreatProtectionExcludedExtensions")
@JsonIgnore
public CollectionPage getAdvancedThreatProtectionExcludedExtensions() {
return new CollectionPage(contextPath, String.class, this.advancedThreatProtectionExcludedExtensions, Optional.ofNullable(advancedThreatProtectionExcludedExtensionsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* advancedThreatProtectionExcludedExtensions} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “A list of file extensions to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedExtensions
* new value of {@code advancedThreatProtectionExcludedExtensions} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code advancedThreatProtectionExcludedExtensions} field changed
*/
public MacOSEndpointProtectionConfiguration withAdvancedThreatProtectionExcludedExtensions(List advancedThreatProtectionExcludedExtensions) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("advancedThreatProtectionExcludedExtensions");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.advancedThreatProtectionExcludedExtensions = advancedThreatProtectionExcludedExtensions;
return _x;
}
/**
* “A list of file extensions to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param options
* specify connect and read timeouts
* @return property advancedThreatProtectionExcludedExtensions
*/
@Property(name="advancedThreatProtectionExcludedExtensions")
@JsonIgnore
public CollectionPage getAdvancedThreatProtectionExcludedExtensions(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.advancedThreatProtectionExcludedExtensions, Optional.ofNullable(advancedThreatProtectionExcludedExtensionsNextLink), Collections.emptyList(), options);
}
/**
* “A list of paths to files to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @return property advancedThreatProtectionExcludedFiles
*/
@Property(name="advancedThreatProtectionExcludedFiles")
@JsonIgnore
public CollectionPage getAdvancedThreatProtectionExcludedFiles() {
return new CollectionPage(contextPath, String.class, this.advancedThreatProtectionExcludedFiles, Optional.ofNullable(advancedThreatProtectionExcludedFilesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* advancedThreatProtectionExcludedFiles} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “A list of paths to files to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedFiles
* new value of {@code advancedThreatProtectionExcludedFiles} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code advancedThreatProtectionExcludedFiles} field changed
*/
public MacOSEndpointProtectionConfiguration withAdvancedThreatProtectionExcludedFiles(List advancedThreatProtectionExcludedFiles) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("advancedThreatProtectionExcludedFiles");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.advancedThreatProtectionExcludedFiles = advancedThreatProtectionExcludedFiles;
return _x;
}
/**
* “A list of paths to files to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param options
* specify connect and read timeouts
* @return property advancedThreatProtectionExcludedFiles
*/
@Property(name="advancedThreatProtectionExcludedFiles")
@JsonIgnore
public CollectionPage getAdvancedThreatProtectionExcludedFiles(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.advancedThreatProtectionExcludedFiles, Optional.ofNullable(advancedThreatProtectionExcludedFilesNextLink), Collections.emptyList(), options);
}
/**
* “A list of paths to folders to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @return property advancedThreatProtectionExcludedFolders
*/
@Property(name="advancedThreatProtectionExcludedFolders")
@JsonIgnore
public CollectionPage getAdvancedThreatProtectionExcludedFolders() {
return new CollectionPage(contextPath, String.class, this.advancedThreatProtectionExcludedFolders, Optional.ofNullable(advancedThreatProtectionExcludedFoldersNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* advancedThreatProtectionExcludedFolders} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “A list of paths to folders to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedFolders
* new value of {@code advancedThreatProtectionExcludedFolders} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code advancedThreatProtectionExcludedFolders} field changed
*/
public MacOSEndpointProtectionConfiguration withAdvancedThreatProtectionExcludedFolders(List advancedThreatProtectionExcludedFolders) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("advancedThreatProtectionExcludedFolders");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.advancedThreatProtectionExcludedFolders = advancedThreatProtectionExcludedFolders;
return _x;
}
/**
* “A list of paths to folders to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param options
* specify connect and read timeouts
* @return property advancedThreatProtectionExcludedFolders
*/
@Property(name="advancedThreatProtectionExcludedFolders")
@JsonIgnore
public CollectionPage getAdvancedThreatProtectionExcludedFolders(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.advancedThreatProtectionExcludedFolders, Optional.ofNullable(advancedThreatProtectionExcludedFoldersNextLink), Collections.emptyList(), options);
}
/**
* “A list of process names to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @return property advancedThreatProtectionExcludedProcesses
*/
@Property(name="advancedThreatProtectionExcludedProcesses")
@JsonIgnore
public CollectionPage getAdvancedThreatProtectionExcludedProcesses() {
return new CollectionPage(contextPath, String.class, this.advancedThreatProtectionExcludedProcesses, Optional.ofNullable(advancedThreatProtectionExcludedProcessesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* advancedThreatProtectionExcludedProcesses} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “A list of process names to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionExcludedProcesses
* new value of {@code advancedThreatProtectionExcludedProcesses} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code advancedThreatProtectionExcludedProcesses} field changed
*/
public MacOSEndpointProtectionConfiguration withAdvancedThreatProtectionExcludedProcesses(List advancedThreatProtectionExcludedProcesses) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("advancedThreatProtectionExcludedProcesses");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.advancedThreatProtectionExcludedProcesses = advancedThreatProtectionExcludedProcesses;
return _x;
}
/**
* “A list of process names to exclude from antivirus scanning for Microsoft
* Defender Advanced Threat Protection on macOS.”
*
* @param options
* specify connect and read timeouts
* @return property advancedThreatProtectionExcludedProcesses
*/
@Property(name="advancedThreatProtectionExcludedProcesses")
@JsonIgnore
public CollectionPage getAdvancedThreatProtectionExcludedProcesses(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.advancedThreatProtectionExcludedProcesses, Optional.ofNullable(advancedThreatProtectionExcludedProcessesNextLink), Collections.emptyList(), options);
}
/**
* “Determines whether or not to enable real-time protection for Microsoft Defender
* Advanced Threat Protection on macOS.”
*
* @return property advancedThreatProtectionRealTime
*/
@Property(name="advancedThreatProtectionRealTime")
@JsonIgnore
public Optional getAdvancedThreatProtectionRealTime() {
return Optional.ofNullable(advancedThreatProtectionRealTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* advancedThreatProtectionRealTime} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Determines whether or not to enable real-time protection for Microsoft Defender
* Advanced Threat Protection on macOS.”
*
* @param advancedThreatProtectionRealTime
* new value of {@code advancedThreatProtectionRealTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code advancedThreatProtectionRealTime} field changed
*/
public MacOSEndpointProtectionConfiguration withAdvancedThreatProtectionRealTime(Enablement advancedThreatProtectionRealTime) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("advancedThreatProtectionRealTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.advancedThreatProtectionRealTime = advancedThreatProtectionRealTime;
return _x;
}
/**
* “Optional. If set to true, the user can defer the enabling of FileVault until
* they sign out.”
*
* @return property fileVaultAllowDeferralUntilSignOut
*/
@Property(name="fileVaultAllowDeferralUntilSignOut")
@JsonIgnore
public Optional getFileVaultAllowDeferralUntilSignOut() {
return Optional.ofNullable(fileVaultAllowDeferralUntilSignOut);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* fileVaultAllowDeferralUntilSignOut} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Optional. If set to true, the user can defer the enabling of FileVault until
* they sign out.”
*
* @param fileVaultAllowDeferralUntilSignOut
* new value of {@code fileVaultAllowDeferralUntilSignOut} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code fileVaultAllowDeferralUntilSignOut} field changed
*/
public MacOSEndpointProtectionConfiguration withFileVaultAllowDeferralUntilSignOut(Boolean fileVaultAllowDeferralUntilSignOut) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("fileVaultAllowDeferralUntilSignOut");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.fileVaultAllowDeferralUntilSignOut = fileVaultAllowDeferralUntilSignOut;
return _x;
}
/**
* “Optional. When using the Defer option, if set to true, the user is not prompted
* to enable FileVault at sign-out.”
*
* @return property fileVaultDisablePromptAtSignOut
*/
@Property(name="fileVaultDisablePromptAtSignOut")
@JsonIgnore
public Optional getFileVaultDisablePromptAtSignOut() {
return Optional.ofNullable(fileVaultDisablePromptAtSignOut);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* fileVaultDisablePromptAtSignOut} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Optional. When using the Defer option, if set to true, the user is not prompted
* to enable FileVault at sign-out.”
*
* @param fileVaultDisablePromptAtSignOut
* new value of {@code fileVaultDisablePromptAtSignOut} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code fileVaultDisablePromptAtSignOut} field changed
*/
public MacOSEndpointProtectionConfiguration withFileVaultDisablePromptAtSignOut(Boolean fileVaultDisablePromptAtSignOut) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("fileVaultDisablePromptAtSignOut");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.fileVaultDisablePromptAtSignOut = fileVaultDisablePromptAtSignOut;
return _x;
}
/**
* “Whether FileVault should be enabled or not.”
*
* @return property fileVaultEnabled
*/
@Property(name="fileVaultEnabled")
@JsonIgnore
public Optional getFileVaultEnabled() {
return Optional.ofNullable(fileVaultEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code fileVaultEnabled}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Whether FileVault should be enabled or not.”
*
* @param fileVaultEnabled
* new value of {@code fileVaultEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code fileVaultEnabled} field changed
*/
public MacOSEndpointProtectionConfiguration withFileVaultEnabled(Boolean fileVaultEnabled) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("fileVaultEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.fileVaultEnabled = fileVaultEnabled;
return _x;
}
/**
* “Optional. A hidden personal recovery key does not appear on the user's screen
* during FileVault encryption, reducing the risk of it ending up in the wrong
* hands.”
*
* @return property fileVaultHidePersonalRecoveryKey
*/
@Property(name="fileVaultHidePersonalRecoveryKey")
@JsonIgnore
public Optional getFileVaultHidePersonalRecoveryKey() {
return Optional.ofNullable(fileVaultHidePersonalRecoveryKey);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* fileVaultHidePersonalRecoveryKey} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Optional. A hidden personal recovery key does not appear on the user's screen
* during FileVault encryption, reducing the risk of it ending up in the wrong
* hands.”
*
* @param fileVaultHidePersonalRecoveryKey
* new value of {@code fileVaultHidePersonalRecoveryKey} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code fileVaultHidePersonalRecoveryKey} field changed
*/
public MacOSEndpointProtectionConfiguration withFileVaultHidePersonalRecoveryKey(Boolean fileVaultHidePersonalRecoveryKey) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("fileVaultHidePersonalRecoveryKey");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.fileVaultHidePersonalRecoveryKey = fileVaultHidePersonalRecoveryKey;
return _x;
}
/**
* “Required if selected recovery key type(s) include InstitutionalRecoveryKey. The
* DER Encoded certificate file used to set an institutional recovery key.”
*
* @return property fileVaultInstitutionalRecoveryKeyCertificate
*/
@Property(name="fileVaultInstitutionalRecoveryKeyCertificate")
@JsonIgnore
public Optional getFileVaultInstitutionalRecoveryKeyCertificate() {
return Optional.ofNullable(fileVaultInstitutionalRecoveryKeyCertificate);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* fileVaultInstitutionalRecoveryKeyCertificate} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Required if selected recovery key type(s) include InstitutionalRecoveryKey. The
* DER Encoded certificate file used to set an institutional recovery key.”
*
* @param fileVaultInstitutionalRecoveryKeyCertificate
* new value of {@code fileVaultInstitutionalRecoveryKeyCertificate} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code fileVaultInstitutionalRecoveryKeyCertificate} field changed
*/
public MacOSEndpointProtectionConfiguration withFileVaultInstitutionalRecoveryKeyCertificate(byte[] fileVaultInstitutionalRecoveryKeyCertificate) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("fileVaultInstitutionalRecoveryKeyCertificate");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.fileVaultInstitutionalRecoveryKeyCertificate = fileVaultInstitutionalRecoveryKeyCertificate;
return _x;
}
/**
* “File name of the institutional recovery key certificate to display in UI. (*.der
* ).”
*
* @return property fileVaultInstitutionalRecoveryKeyCertificateFileName
*/
@Property(name="fileVaultInstitutionalRecoveryKeyCertificateFileName")
@JsonIgnore
public Optional getFileVaultInstitutionalRecoveryKeyCertificateFileName() {
return Optional.ofNullable(fileVaultInstitutionalRecoveryKeyCertificateFileName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* fileVaultInstitutionalRecoveryKeyCertificateFileName} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “File name of the institutional recovery key certificate to display in UI. (*.der
* ).”
*
* @param fileVaultInstitutionalRecoveryKeyCertificateFileName
* new value of {@code fileVaultInstitutionalRecoveryKeyCertificateFileName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code fileVaultInstitutionalRecoveryKeyCertificateFileName} field changed
*/
public MacOSEndpointProtectionConfiguration withFileVaultInstitutionalRecoveryKeyCertificateFileName(String fileVaultInstitutionalRecoveryKeyCertificateFileName) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("fileVaultInstitutionalRecoveryKeyCertificateFileName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.fileVaultInstitutionalRecoveryKeyCertificateFileName = fileVaultInstitutionalRecoveryKeyCertificateFileName;
return _x;
}
/**
* “Optional. When using the Defer option, this is the maximum number of times the
* user can ignore prompts to enable FileVault before FileVault will be required
* for the user to sign in. If set to -1, it will always prompt to enable FileVault
* until FileVault is enabled, though it will allow the user to bypass enabling
* FileVault. Setting this to 0 will disable the feature.”
*
* @return property fileVaultNumberOfTimesUserCanIgnore
*/
@Property(name="fileVaultNumberOfTimesUserCanIgnore")
@JsonIgnore
public Optional getFileVaultNumberOfTimesUserCanIgnore() {
return Optional.ofNullable(fileVaultNumberOfTimesUserCanIgnore);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* fileVaultNumberOfTimesUserCanIgnore} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Optional. When using the Defer option, this is the maximum number of times the
* user can ignore prompts to enable FileVault before FileVault will be required
* for the user to sign in. If set to -1, it will always prompt to enable FileVault
* until FileVault is enabled, though it will allow the user to bypass enabling
* FileVault. Setting this to 0 will disable the feature.”
*
* @param fileVaultNumberOfTimesUserCanIgnore
* new value of {@code fileVaultNumberOfTimesUserCanIgnore} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code fileVaultNumberOfTimesUserCanIgnore} field changed
*/
public MacOSEndpointProtectionConfiguration withFileVaultNumberOfTimesUserCanIgnore(Integer fileVaultNumberOfTimesUserCanIgnore) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("fileVaultNumberOfTimesUserCanIgnore");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.fileVaultNumberOfTimesUserCanIgnore = fileVaultNumberOfTimesUserCanIgnore;
return _x;
}
/**
* “Required if selected recovery key type(s) include PersonalRecoveryKey. A short
* message displayed to the user that explains how they can retrieve their personal
* recovery key.”
*
* @return property fileVaultPersonalRecoveryKeyHelpMessage
*/
@Property(name="fileVaultPersonalRecoveryKeyHelpMessage")
@JsonIgnore
public Optional getFileVaultPersonalRecoveryKeyHelpMessage() {
return Optional.ofNullable(fileVaultPersonalRecoveryKeyHelpMessage);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* fileVaultPersonalRecoveryKeyHelpMessage} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Required if selected recovery key type(s) include PersonalRecoveryKey. A short
* message displayed to the user that explains how they can retrieve their personal
* recovery key.”
*
* @param fileVaultPersonalRecoveryKeyHelpMessage
* new value of {@code fileVaultPersonalRecoveryKeyHelpMessage} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code fileVaultPersonalRecoveryKeyHelpMessage} field changed
*/
public MacOSEndpointProtectionConfiguration withFileVaultPersonalRecoveryKeyHelpMessage(String fileVaultPersonalRecoveryKeyHelpMessage) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("fileVaultPersonalRecoveryKeyHelpMessage");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.fileVaultPersonalRecoveryKeyHelpMessage = fileVaultPersonalRecoveryKeyHelpMessage;
return _x;
}
/**
* “Optional. If selected recovery key type(s) include PersonalRecoveryKey, the
* frequency to rotate that key, in months.”
*
* @return property fileVaultPersonalRecoveryKeyRotationInMonths
*/
@Property(name="fileVaultPersonalRecoveryKeyRotationInMonths")
@JsonIgnore
public Optional getFileVaultPersonalRecoveryKeyRotationInMonths() {
return Optional.ofNullable(fileVaultPersonalRecoveryKeyRotationInMonths);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* fileVaultPersonalRecoveryKeyRotationInMonths} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Optional. If selected recovery key type(s) include PersonalRecoveryKey, the
* frequency to rotate that key, in months.”
*
* @param fileVaultPersonalRecoveryKeyRotationInMonths
* new value of {@code fileVaultPersonalRecoveryKeyRotationInMonths} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code fileVaultPersonalRecoveryKeyRotationInMonths} field changed
*/
public MacOSEndpointProtectionConfiguration withFileVaultPersonalRecoveryKeyRotationInMonths(Integer fileVaultPersonalRecoveryKeyRotationInMonths) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("fileVaultPersonalRecoveryKeyRotationInMonths");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.fileVaultPersonalRecoveryKeyRotationInMonths = fileVaultPersonalRecoveryKeyRotationInMonths;
return _x;
}
/**
* “Required if FileVault is enabled, determines the type(s) of recovery key to use.”
*
* @return property fileVaultSelectedRecoveryKeyTypes
*/
@Property(name="fileVaultSelectedRecoveryKeyTypes")
@JsonIgnore
public Optional getFileVaultSelectedRecoveryKeyTypes() {
return Optional.ofNullable(fileVaultSelectedRecoveryKeyTypes);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* fileVaultSelectedRecoveryKeyTypes} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Required if FileVault is enabled, determines the type(s) of recovery key to use.”
*
* @param fileVaultSelectedRecoveryKeyTypes
* new value of {@code fileVaultSelectedRecoveryKeyTypes} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code fileVaultSelectedRecoveryKeyTypes} field changed
*/
public MacOSEndpointProtectionConfiguration withFileVaultSelectedRecoveryKeyTypes(MacOSFileVaultRecoveryKeyTypes fileVaultSelectedRecoveryKeyTypes) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("fileVaultSelectedRecoveryKeyTypes");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.fileVaultSelectedRecoveryKeyTypes = fileVaultSelectedRecoveryKeyTypes;
return _x;
}
/**
* “List of applications with firewall settings. Firewall settings for applications
* not on this list are determined by the user. This collection can contain a
* maximum of 500 elements.”
*
* @return property firewallApplications
*/
@Property(name="firewallApplications")
@JsonIgnore
public CollectionPage getFirewallApplications() {
return new CollectionPage(contextPath, MacOSFirewallApplication.class, this.firewallApplications, Optional.ofNullable(firewallApplicationsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* firewallApplications} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “List of applications with firewall settings. Firewall settings for applications
* not on this list are determined by the user. This collection can contain a
* maximum of 500 elements.”
*
* @param firewallApplications
* new value of {@code firewallApplications} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code firewallApplications} field changed
*/
public MacOSEndpointProtectionConfiguration withFirewallApplications(List firewallApplications) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("firewallApplications");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.firewallApplications = firewallApplications;
return _x;
}
/**
* “List of applications with firewall settings. Firewall settings for applications
* not on this list are determined by the user. This collection can contain a
* maximum of 500 elements.”
*
* @param options
* specify connect and read timeouts
* @return property firewallApplications
*/
@Property(name="firewallApplications")
@JsonIgnore
public CollectionPage getFirewallApplications(HttpRequestOptions options) {
return new CollectionPage(contextPath, MacOSFirewallApplication.class, this.firewallApplications, Optional.ofNullable(firewallApplicationsNextLink), Collections.emptyList(), options);
}
/**
* “Corresponds to the “Block all incoming connections” option.”
*
* @return property firewallBlockAllIncoming
*/
@Property(name="firewallBlockAllIncoming")
@JsonIgnore
public Optional getFirewallBlockAllIncoming() {
return Optional.ofNullable(firewallBlockAllIncoming);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* firewallBlockAllIncoming} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Corresponds to the “Block all incoming connections” option.”
*
* @param firewallBlockAllIncoming
* new value of {@code firewallBlockAllIncoming} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code firewallBlockAllIncoming} field changed
*/
public MacOSEndpointProtectionConfiguration withFirewallBlockAllIncoming(Boolean firewallBlockAllIncoming) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("firewallBlockAllIncoming");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.firewallBlockAllIncoming = firewallBlockAllIncoming;
return _x;
}
/**
* “Whether the firewall should be enabled or not.”
*
* @return property firewallEnabled
*/
@Property(name="firewallEnabled")
@JsonIgnore
public Optional getFirewallEnabled() {
return Optional.ofNullable(firewallEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code firewallEnabled}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Whether the firewall should be enabled or not.”
*
* @param firewallEnabled
* new value of {@code firewallEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code firewallEnabled} field changed
*/
public MacOSEndpointProtectionConfiguration withFirewallEnabled(Boolean firewallEnabled) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("firewallEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.firewallEnabled = firewallEnabled;
return _x;
}
/**
* “Corresponds to “Enable stealth mode.””
*
* @return property firewallEnableStealthMode
*/
@Property(name="firewallEnableStealthMode")
@JsonIgnore
public Optional getFirewallEnableStealthMode() {
return Optional.ofNullable(firewallEnableStealthMode);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* firewallEnableStealthMode} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Corresponds to “Enable stealth mode.””
*
* @param firewallEnableStealthMode
* new value of {@code firewallEnableStealthMode} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code firewallEnableStealthMode} field changed
*/
public MacOSEndpointProtectionConfiguration withFirewallEnableStealthMode(Boolean firewallEnableStealthMode) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("firewallEnableStealthMode");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.firewallEnableStealthMode = firewallEnableStealthMode;
return _x;
}
/**
* “System and Privacy setting that determines which download locations apps can be
* run from on a macOS device.”
*
* @return property gatekeeperAllowedAppSource
*/
@Property(name="gatekeeperAllowedAppSource")
@JsonIgnore
public Optional getGatekeeperAllowedAppSource() {
return Optional.ofNullable(gatekeeperAllowedAppSource);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* gatekeeperAllowedAppSource} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “System and Privacy setting that determines which download locations apps can be
* run from on a macOS device.”
*
* @param gatekeeperAllowedAppSource
* new value of {@code gatekeeperAllowedAppSource} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code gatekeeperAllowedAppSource} field changed
*/
public MacOSEndpointProtectionConfiguration withGatekeeperAllowedAppSource(MacOSGatekeeperAppSources gatekeeperAllowedAppSource) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("gatekeeperAllowedAppSource");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.gatekeeperAllowedAppSource = gatekeeperAllowedAppSource;
return _x;
}
/**
* “If set to true, the user override for Gatekeeper will be disabled.”
*
* @return property gatekeeperBlockOverride
*/
@Property(name="gatekeeperBlockOverride")
@JsonIgnore
public Optional getGatekeeperBlockOverride() {
return Optional.ofNullable(gatekeeperBlockOverride);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* gatekeeperBlockOverride} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “If set to true, the user override for Gatekeeper will be disabled.”
*
* @param gatekeeperBlockOverride
* new value of {@code gatekeeperBlockOverride} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code gatekeeperBlockOverride} field changed
*/
public MacOSEndpointProtectionConfiguration withGatekeeperBlockOverride(Boolean gatekeeperBlockOverride) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = changedFields.add("gatekeeperBlockOverride");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSEndpointProtectionConfiguration");
_x.gatekeeperBlockOverride = gatekeeperBlockOverride;
return _x;
}
public MacOSEndpointProtectionConfiguration withUnmappedField(String name, String value) {
MacOSEndpointProtectionConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public MacOSEndpointProtectionConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public MacOSEndpointProtectionConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
MacOSEndpointProtectionConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private MacOSEndpointProtectionConfiguration _copy() {
MacOSEndpointProtectionConfiguration _x = new MacOSEndpointProtectionConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.advancedThreatProtectionAutomaticSampleSubmission = advancedThreatProtectionAutomaticSampleSubmission;
_x.advancedThreatProtectionCloudDelivered = advancedThreatProtectionCloudDelivered;
_x.advancedThreatProtectionDiagnosticDataCollection = advancedThreatProtectionDiagnosticDataCollection;
_x.advancedThreatProtectionExcludedExtensions = advancedThreatProtectionExcludedExtensions;
_x.advancedThreatProtectionExcludedFiles = advancedThreatProtectionExcludedFiles;
_x.advancedThreatProtectionExcludedFolders = advancedThreatProtectionExcludedFolders;
_x.advancedThreatProtectionExcludedProcesses = advancedThreatProtectionExcludedProcesses;
_x.advancedThreatProtectionRealTime = advancedThreatProtectionRealTime;
_x.fileVaultAllowDeferralUntilSignOut = fileVaultAllowDeferralUntilSignOut;
_x.fileVaultDisablePromptAtSignOut = fileVaultDisablePromptAtSignOut;
_x.fileVaultEnabled = fileVaultEnabled;
_x.fileVaultHidePersonalRecoveryKey = fileVaultHidePersonalRecoveryKey;
_x.fileVaultInstitutionalRecoveryKeyCertificate = fileVaultInstitutionalRecoveryKeyCertificate;
_x.fileVaultInstitutionalRecoveryKeyCertificateFileName = fileVaultInstitutionalRecoveryKeyCertificateFileName;
_x.fileVaultNumberOfTimesUserCanIgnore = fileVaultNumberOfTimesUserCanIgnore;
_x.fileVaultPersonalRecoveryKeyHelpMessage = fileVaultPersonalRecoveryKeyHelpMessage;
_x.fileVaultPersonalRecoveryKeyRotationInMonths = fileVaultPersonalRecoveryKeyRotationInMonths;
_x.fileVaultSelectedRecoveryKeyTypes = fileVaultSelectedRecoveryKeyTypes;
_x.firewallApplications = firewallApplications;
_x.firewallBlockAllIncoming = firewallBlockAllIncoming;
_x.firewallEnabled = firewallEnabled;
_x.firewallEnableStealthMode = firewallEnableStealthMode;
_x.gatekeeperAllowedAppSource = gatekeeperAllowedAppSource;
_x.gatekeeperBlockOverride = gatekeeperBlockOverride;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("MacOSEndpointProtectionConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("deviceManagementApplicabilityRuleDeviceMode=");
b.append(this.deviceManagementApplicabilityRuleDeviceMode);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsEdition=");
b.append(this.deviceManagementApplicabilityRuleOsEdition);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsVersion=");
b.append(this.deviceManagementApplicabilityRuleOsVersion);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("supportsScopeTags=");
b.append(this.supportsScopeTags);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("advancedThreatProtectionAutomaticSampleSubmission=");
b.append(this.advancedThreatProtectionAutomaticSampleSubmission);
b.append(", ");
b.append("advancedThreatProtectionCloudDelivered=");
b.append(this.advancedThreatProtectionCloudDelivered);
b.append(", ");
b.append("advancedThreatProtectionDiagnosticDataCollection=");
b.append(this.advancedThreatProtectionDiagnosticDataCollection);
b.append(", ");
b.append("advancedThreatProtectionExcludedExtensions=");
b.append(this.advancedThreatProtectionExcludedExtensions);
b.append(", ");
b.append("advancedThreatProtectionExcludedFiles=");
b.append(this.advancedThreatProtectionExcludedFiles);
b.append(", ");
b.append("advancedThreatProtectionExcludedFolders=");
b.append(this.advancedThreatProtectionExcludedFolders);
b.append(", ");
b.append("advancedThreatProtectionExcludedProcesses=");
b.append(this.advancedThreatProtectionExcludedProcesses);
b.append(", ");
b.append("advancedThreatProtectionRealTime=");
b.append(this.advancedThreatProtectionRealTime);
b.append(", ");
b.append("fileVaultAllowDeferralUntilSignOut=");
b.append(this.fileVaultAllowDeferralUntilSignOut);
b.append(", ");
b.append("fileVaultDisablePromptAtSignOut=");
b.append(this.fileVaultDisablePromptAtSignOut);
b.append(", ");
b.append("fileVaultEnabled=");
b.append(this.fileVaultEnabled);
b.append(", ");
b.append("fileVaultHidePersonalRecoveryKey=");
b.append(this.fileVaultHidePersonalRecoveryKey);
b.append(", ");
b.append("fileVaultInstitutionalRecoveryKeyCertificate=");
b.append(this.fileVaultInstitutionalRecoveryKeyCertificate);
b.append(", ");
b.append("fileVaultInstitutionalRecoveryKeyCertificateFileName=");
b.append(this.fileVaultInstitutionalRecoveryKeyCertificateFileName);
b.append(", ");
b.append("fileVaultNumberOfTimesUserCanIgnore=");
b.append(this.fileVaultNumberOfTimesUserCanIgnore);
b.append(", ");
b.append("fileVaultPersonalRecoveryKeyHelpMessage=");
b.append(this.fileVaultPersonalRecoveryKeyHelpMessage);
b.append(", ");
b.append("fileVaultPersonalRecoveryKeyRotationInMonths=");
b.append(this.fileVaultPersonalRecoveryKeyRotationInMonths);
b.append(", ");
b.append("fileVaultSelectedRecoveryKeyTypes=");
b.append(this.fileVaultSelectedRecoveryKeyTypes);
b.append(", ");
b.append("firewallApplications=");
b.append(this.firewallApplications);
b.append(", ");
b.append("firewallBlockAllIncoming=");
b.append(this.firewallBlockAllIncoming);
b.append(", ");
b.append("firewallEnabled=");
b.append(this.firewallEnabled);
b.append(", ");
b.append("firewallEnableStealthMode=");
b.append(this.firewallEnableStealthMode);
b.append(", ");
b.append("gatekeeperAllowedAppSource=");
b.append(this.gatekeeperAllowedAppSource);
b.append(", ");
b.append("gatekeeperBlockOverride=");
b.append(this.gatekeeperBlockOverride);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}