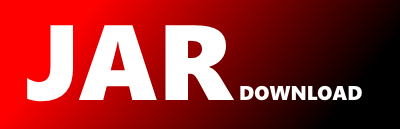
odata.msgraph.client.beta.entity.MacOSSoftwareUpdateConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.complex.CustomUpdateTimeWindow;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleDeviceMode;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsEdition;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsVersion;
import odata.msgraph.client.beta.enums.MacOSSoftwareUpdateBehavior;
import odata.msgraph.client.beta.enums.MacOSSoftwareUpdateScheduleType;
/**
* “MacOS Software Update Configuration”
*/@JsonPropertyOrder({
"@odata.type",
"allOtherUpdateBehavior",
"configDataUpdateBehavior",
"criticalUpdateBehavior",
"customUpdateTimeWindows",
"firmwareUpdateBehavior",
"updateScheduleType",
"updateTimeWindowUtcOffsetInMinutes"})
@JsonInclude(Include.NON_NULL)
public class MacOSSoftwareUpdateConfiguration extends DeviceConfiguration implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.macOSSoftwareUpdateConfiguration";
}
@JsonProperty("allOtherUpdateBehavior")
protected MacOSSoftwareUpdateBehavior allOtherUpdateBehavior;
@JsonProperty("configDataUpdateBehavior")
protected MacOSSoftwareUpdateBehavior configDataUpdateBehavior;
@JsonProperty("criticalUpdateBehavior")
protected MacOSSoftwareUpdateBehavior criticalUpdateBehavior;
@JsonProperty("customUpdateTimeWindows")
protected List customUpdateTimeWindows;
@JsonProperty("customUpdateTimeWindows@nextLink")
protected String customUpdateTimeWindowsNextLink;
@JsonProperty("firmwareUpdateBehavior")
protected MacOSSoftwareUpdateBehavior firmwareUpdateBehavior;
@JsonProperty("updateScheduleType")
protected MacOSSoftwareUpdateScheduleType updateScheduleType;
@JsonProperty("updateTimeWindowUtcOffsetInMinutes")
protected Integer updateTimeWindowUtcOffsetInMinutes;
protected MacOSSoftwareUpdateConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderMacOSSoftwareUpdateConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode;
private DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition;
private DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private Boolean supportsScopeTags;
private Integer version;
private MacOSSoftwareUpdateBehavior allOtherUpdateBehavior;
private MacOSSoftwareUpdateBehavior configDataUpdateBehavior;
private MacOSSoftwareUpdateBehavior criticalUpdateBehavior;
private List customUpdateTimeWindows;
private String customUpdateTimeWindowsNextLink;
private MacOSSoftwareUpdateBehavior firmwareUpdateBehavior;
private MacOSSoftwareUpdateScheduleType updateScheduleType;
private Integer updateTimeWindowUtcOffsetInMinutes;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder deviceManagementApplicabilityRuleDeviceMode(DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode) {
this.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleDeviceMode");
return this;
}
public Builder deviceManagementApplicabilityRuleOsEdition(DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition) {
this.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsEdition");
return this;
}
public Builder deviceManagementApplicabilityRuleOsVersion(DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion) {
this.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsVersion");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder supportsScopeTags(Boolean supportsScopeTags) {
this.supportsScopeTags = supportsScopeTags;
this.changedFields = changedFields.add("supportsScopeTags");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
/**
* “Update behavior for all other updates.”
*
* @param allOtherUpdateBehavior
* value of {@code allOtherUpdateBehavior} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder allOtherUpdateBehavior(MacOSSoftwareUpdateBehavior allOtherUpdateBehavior) {
this.allOtherUpdateBehavior = allOtherUpdateBehavior;
this.changedFields = changedFields.add("allOtherUpdateBehavior");
return this;
}
/**
* “Update behavior for configuration data file updates.”
*
* @param configDataUpdateBehavior
* value of {@code configDataUpdateBehavior} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder configDataUpdateBehavior(MacOSSoftwareUpdateBehavior configDataUpdateBehavior) {
this.configDataUpdateBehavior = configDataUpdateBehavior;
this.changedFields = changedFields.add("configDataUpdateBehavior");
return this;
}
/**
* “Update behavior for critical updates.”
*
* @param criticalUpdateBehavior
* value of {@code criticalUpdateBehavior} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder criticalUpdateBehavior(MacOSSoftwareUpdateBehavior criticalUpdateBehavior) {
this.criticalUpdateBehavior = criticalUpdateBehavior;
this.changedFields = changedFields.add("criticalUpdateBehavior");
return this;
}
/**
* “Custom Time windows when updates will be allowed or blocked. This collection can
* contain a maximum of 20 elements.”
*
* @param customUpdateTimeWindows
* value of {@code customUpdateTimeWindows} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder customUpdateTimeWindows(List customUpdateTimeWindows) {
this.customUpdateTimeWindows = customUpdateTimeWindows;
this.changedFields = changedFields.add("customUpdateTimeWindows");
return this;
}
/**
* “Custom Time windows when updates will be allowed or blocked. This collection can
* contain a maximum of 20 elements.”
*
* @param customUpdateTimeWindows
* value of {@code customUpdateTimeWindows} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder customUpdateTimeWindows(CustomUpdateTimeWindow... customUpdateTimeWindows) {
return customUpdateTimeWindows(Arrays.asList(customUpdateTimeWindows));
}
/**
* “Custom Time windows when updates will be allowed or blocked. This collection can
* contain a maximum of 20 elements.”
*
* @param customUpdateTimeWindowsNextLink
* value of {@code customUpdateTimeWindows@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder customUpdateTimeWindowsNextLink(String customUpdateTimeWindowsNextLink) {
this.customUpdateTimeWindowsNextLink = customUpdateTimeWindowsNextLink;
this.changedFields = changedFields.add("customUpdateTimeWindows");
return this;
}
/**
* “Update behavior for firmware updates.”
*
* @param firmwareUpdateBehavior
* value of {@code firmwareUpdateBehavior} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder firmwareUpdateBehavior(MacOSSoftwareUpdateBehavior firmwareUpdateBehavior) {
this.firmwareUpdateBehavior = firmwareUpdateBehavior;
this.changedFields = changedFields.add("firmwareUpdateBehavior");
return this;
}
/**
* “Update schedule type”
*
* @param updateScheduleType
* value of {@code updateScheduleType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder updateScheduleType(MacOSSoftwareUpdateScheduleType updateScheduleType) {
this.updateScheduleType = updateScheduleType;
this.changedFields = changedFields.add("updateScheduleType");
return this;
}
/**
* “Minutes indicating UTC offset for each update time window”
*
* @param updateTimeWindowUtcOffsetInMinutes
* value of {@code updateTimeWindowUtcOffsetInMinutes} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder updateTimeWindowUtcOffsetInMinutes(Integer updateTimeWindowUtcOffsetInMinutes) {
this.updateTimeWindowUtcOffsetInMinutes = updateTimeWindowUtcOffsetInMinutes;
this.changedFields = changedFields.add("updateTimeWindowUtcOffsetInMinutes");
return this;
}
public MacOSSoftwareUpdateConfiguration build() {
MacOSSoftwareUpdateConfiguration _x = new MacOSSoftwareUpdateConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.macOSSoftwareUpdateConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.allOtherUpdateBehavior = allOtherUpdateBehavior;
_x.configDataUpdateBehavior = configDataUpdateBehavior;
_x.criticalUpdateBehavior = criticalUpdateBehavior;
_x.customUpdateTimeWindows = customUpdateTimeWindows;
_x.customUpdateTimeWindowsNextLink = customUpdateTimeWindowsNextLink;
_x.firmwareUpdateBehavior = firmwareUpdateBehavior;
_x.updateScheduleType = updateScheduleType;
_x.updateTimeWindowUtcOffsetInMinutes = updateTimeWindowUtcOffsetInMinutes;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Update behavior for all other updates.”
*
* @return property allOtherUpdateBehavior
*/
@Property(name="allOtherUpdateBehavior")
@JsonIgnore
public Optional getAllOtherUpdateBehavior() {
return Optional.ofNullable(allOtherUpdateBehavior);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* allOtherUpdateBehavior} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Update behavior for all other updates.”
*
* @param allOtherUpdateBehavior
* new value of {@code allOtherUpdateBehavior} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code allOtherUpdateBehavior} field changed
*/
public MacOSSoftwareUpdateConfiguration withAllOtherUpdateBehavior(MacOSSoftwareUpdateBehavior allOtherUpdateBehavior) {
MacOSSoftwareUpdateConfiguration _x = _copy();
_x.changedFields = changedFields.add("allOtherUpdateBehavior");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSSoftwareUpdateConfiguration");
_x.allOtherUpdateBehavior = allOtherUpdateBehavior;
return _x;
}
/**
* “Update behavior for configuration data file updates.”
*
* @return property configDataUpdateBehavior
*/
@Property(name="configDataUpdateBehavior")
@JsonIgnore
public Optional getConfigDataUpdateBehavior() {
return Optional.ofNullable(configDataUpdateBehavior);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* configDataUpdateBehavior} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Update behavior for configuration data file updates.”
*
* @param configDataUpdateBehavior
* new value of {@code configDataUpdateBehavior} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code configDataUpdateBehavior} field changed
*/
public MacOSSoftwareUpdateConfiguration withConfigDataUpdateBehavior(MacOSSoftwareUpdateBehavior configDataUpdateBehavior) {
MacOSSoftwareUpdateConfiguration _x = _copy();
_x.changedFields = changedFields.add("configDataUpdateBehavior");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSSoftwareUpdateConfiguration");
_x.configDataUpdateBehavior = configDataUpdateBehavior;
return _x;
}
/**
* “Update behavior for critical updates.”
*
* @return property criticalUpdateBehavior
*/
@Property(name="criticalUpdateBehavior")
@JsonIgnore
public Optional getCriticalUpdateBehavior() {
return Optional.ofNullable(criticalUpdateBehavior);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* criticalUpdateBehavior} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Update behavior for critical updates.”
*
* @param criticalUpdateBehavior
* new value of {@code criticalUpdateBehavior} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code criticalUpdateBehavior} field changed
*/
public MacOSSoftwareUpdateConfiguration withCriticalUpdateBehavior(MacOSSoftwareUpdateBehavior criticalUpdateBehavior) {
MacOSSoftwareUpdateConfiguration _x = _copy();
_x.changedFields = changedFields.add("criticalUpdateBehavior");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSSoftwareUpdateConfiguration");
_x.criticalUpdateBehavior = criticalUpdateBehavior;
return _x;
}
/**
* “Custom Time windows when updates will be allowed or blocked. This collection can
* contain a maximum of 20 elements.”
*
* @return property customUpdateTimeWindows
*/
@Property(name="customUpdateTimeWindows")
@JsonIgnore
public CollectionPage getCustomUpdateTimeWindows() {
return new CollectionPage(contextPath, CustomUpdateTimeWindow.class, this.customUpdateTimeWindows, Optional.ofNullable(customUpdateTimeWindowsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* customUpdateTimeWindows} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Custom Time windows when updates will be allowed or blocked. This collection can
* contain a maximum of 20 elements.”
*
* @param customUpdateTimeWindows
* new value of {@code customUpdateTimeWindows} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code customUpdateTimeWindows} field changed
*/
public MacOSSoftwareUpdateConfiguration withCustomUpdateTimeWindows(List customUpdateTimeWindows) {
MacOSSoftwareUpdateConfiguration _x = _copy();
_x.changedFields = changedFields.add("customUpdateTimeWindows");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSSoftwareUpdateConfiguration");
_x.customUpdateTimeWindows = customUpdateTimeWindows;
return _x;
}
/**
* “Custom Time windows when updates will be allowed or blocked. This collection can
* contain a maximum of 20 elements.”
*
* @param options
* specify connect and read timeouts
* @return property customUpdateTimeWindows
*/
@Property(name="customUpdateTimeWindows")
@JsonIgnore
public CollectionPage getCustomUpdateTimeWindows(HttpRequestOptions options) {
return new CollectionPage(contextPath, CustomUpdateTimeWindow.class, this.customUpdateTimeWindows, Optional.ofNullable(customUpdateTimeWindowsNextLink), Collections.emptyList(), options);
}
/**
* “Update behavior for firmware updates.”
*
* @return property firmwareUpdateBehavior
*/
@Property(name="firmwareUpdateBehavior")
@JsonIgnore
public Optional getFirmwareUpdateBehavior() {
return Optional.ofNullable(firmwareUpdateBehavior);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* firmwareUpdateBehavior} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Update behavior for firmware updates.”
*
* @param firmwareUpdateBehavior
* new value of {@code firmwareUpdateBehavior} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code firmwareUpdateBehavior} field changed
*/
public MacOSSoftwareUpdateConfiguration withFirmwareUpdateBehavior(MacOSSoftwareUpdateBehavior firmwareUpdateBehavior) {
MacOSSoftwareUpdateConfiguration _x = _copy();
_x.changedFields = changedFields.add("firmwareUpdateBehavior");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSSoftwareUpdateConfiguration");
_x.firmwareUpdateBehavior = firmwareUpdateBehavior;
return _x;
}
/**
* “Update schedule type”
*
* @return property updateScheduleType
*/
@Property(name="updateScheduleType")
@JsonIgnore
public Optional getUpdateScheduleType() {
return Optional.ofNullable(updateScheduleType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* updateScheduleType} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Update schedule type”
*
* @param updateScheduleType
* new value of {@code updateScheduleType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code updateScheduleType} field changed
*/
public MacOSSoftwareUpdateConfiguration withUpdateScheduleType(MacOSSoftwareUpdateScheduleType updateScheduleType) {
MacOSSoftwareUpdateConfiguration _x = _copy();
_x.changedFields = changedFields.add("updateScheduleType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSSoftwareUpdateConfiguration");
_x.updateScheduleType = updateScheduleType;
return _x;
}
/**
* “Minutes indicating UTC offset for each update time window”
*
* @return property updateTimeWindowUtcOffsetInMinutes
*/
@Property(name="updateTimeWindowUtcOffsetInMinutes")
@JsonIgnore
public Optional getUpdateTimeWindowUtcOffsetInMinutes() {
return Optional.ofNullable(updateTimeWindowUtcOffsetInMinutes);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* updateTimeWindowUtcOffsetInMinutes} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Minutes indicating UTC offset for each update time window”
*
* @param updateTimeWindowUtcOffsetInMinutes
* new value of {@code updateTimeWindowUtcOffsetInMinutes} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code updateTimeWindowUtcOffsetInMinutes} field changed
*/
public MacOSSoftwareUpdateConfiguration withUpdateTimeWindowUtcOffsetInMinutes(Integer updateTimeWindowUtcOffsetInMinutes) {
MacOSSoftwareUpdateConfiguration _x = _copy();
_x.changedFields = changedFields.add("updateTimeWindowUtcOffsetInMinutes");
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSSoftwareUpdateConfiguration");
_x.updateTimeWindowUtcOffsetInMinutes = updateTimeWindowUtcOffsetInMinutes;
return _x;
}
public MacOSSoftwareUpdateConfiguration withUnmappedField(String name, String value) {
MacOSSoftwareUpdateConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public MacOSSoftwareUpdateConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
MacOSSoftwareUpdateConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public MacOSSoftwareUpdateConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
MacOSSoftwareUpdateConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private MacOSSoftwareUpdateConfiguration _copy() {
MacOSSoftwareUpdateConfiguration _x = new MacOSSoftwareUpdateConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.allOtherUpdateBehavior = allOtherUpdateBehavior;
_x.configDataUpdateBehavior = configDataUpdateBehavior;
_x.criticalUpdateBehavior = criticalUpdateBehavior;
_x.customUpdateTimeWindows = customUpdateTimeWindows;
_x.firmwareUpdateBehavior = firmwareUpdateBehavior;
_x.updateScheduleType = updateScheduleType;
_x.updateTimeWindowUtcOffsetInMinutes = updateTimeWindowUtcOffsetInMinutes;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("MacOSSoftwareUpdateConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("deviceManagementApplicabilityRuleDeviceMode=");
b.append(this.deviceManagementApplicabilityRuleDeviceMode);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsEdition=");
b.append(this.deviceManagementApplicabilityRuleOsEdition);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsVersion=");
b.append(this.deviceManagementApplicabilityRuleOsVersion);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("supportsScopeTags=");
b.append(this.supportsScopeTags);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("allOtherUpdateBehavior=");
b.append(this.allOtherUpdateBehavior);
b.append(", ");
b.append("configDataUpdateBehavior=");
b.append(this.configDataUpdateBehavior);
b.append(", ");
b.append("criticalUpdateBehavior=");
b.append(this.criticalUpdateBehavior);
b.append(", ");
b.append("customUpdateTimeWindows=");
b.append(this.customUpdateTimeWindows);
b.append(", ");
b.append("firmwareUpdateBehavior=");
b.append(this.firmwareUpdateBehavior);
b.append(", ");
b.append("updateScheduleType=");
b.append(this.updateScheduleType);
b.append(", ");
b.append("updateTimeWindowUtcOffsetInMinutes=");
b.append(this.updateTimeWindowUtcOffsetInMinutes);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}