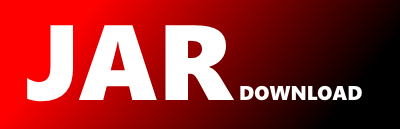
odata.msgraph.client.beta.entity.ManagedAppProtection Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.Duration;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import odata.msgraph.client.beta.enums.ManagedAppClipboardSharingLevel;
import odata.msgraph.client.beta.enums.ManagedAppDataIngestionLocation;
import odata.msgraph.client.beta.enums.ManagedAppDataStorageLocation;
import odata.msgraph.client.beta.enums.ManagedAppDataTransferLevel;
import odata.msgraph.client.beta.enums.ManagedAppDeviceThreatLevel;
import odata.msgraph.client.beta.enums.ManagedAppNotificationRestriction;
import odata.msgraph.client.beta.enums.ManagedAppPhoneNumberRedirectLevel;
import odata.msgraph.client.beta.enums.ManagedAppPinCharacterSet;
import odata.msgraph.client.beta.enums.ManagedAppRemediationAction;
import odata.msgraph.client.beta.enums.ManagedBrowserType;
/**
* “Policy used to configure detailed management settings for a specified set of
* apps”
*/@JsonPropertyOrder({
"@odata.type",
"allowedDataIngestionLocations",
"allowedDataStorageLocations",
"allowedInboundDataTransferSources",
"allowedOutboundClipboardSharingExceptionLength",
"allowedOutboundClipboardSharingLevel",
"allowedOutboundDataTransferDestinations",
"appActionIfDeviceComplianceRequired",
"appActionIfMaximumPinRetriesExceeded",
"appActionIfUnableToAuthenticateUser",
"blockDataIngestionIntoOrganizationDocuments",
"contactSyncBlocked",
"dataBackupBlocked",
"deviceComplianceRequired",
"dialerRestrictionLevel",
"disableAppPinIfDevicePinIsSet",
"fingerprintBlocked",
"managedBrowser",
"managedBrowserToOpenLinksRequired",
"maximumAllowedDeviceThreatLevel",
"maximumPinRetries",
"maximumRequiredOsVersion",
"maximumWarningOsVersion",
"maximumWipeOsVersion",
"minimumPinLength",
"minimumRequiredAppVersion",
"minimumRequiredOsVersion",
"minimumWarningAppVersion",
"minimumWarningOsVersion",
"minimumWipeAppVersion",
"minimumWipeOsVersion",
"mobileThreatDefenseRemediationAction",
"notificationRestriction",
"organizationalCredentialsRequired",
"periodBeforePinReset",
"periodOfflineBeforeAccessCheck",
"periodOfflineBeforeWipeIsEnforced",
"periodOnlineBeforeAccessCheck",
"pinCharacterSet",
"pinRequired",
"pinRequiredInsteadOfBiometricTimeout",
"previousPinBlockCount",
"printBlocked",
"saveAsBlocked",
"simplePinBlocked"})
@JsonInclude(Include.NON_NULL)
public class ManagedAppProtection extends ManagedAppPolicy implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.managedAppProtection";
}
@JsonProperty("allowedDataIngestionLocations")
protected List allowedDataIngestionLocations;
@JsonProperty("allowedDataIngestionLocations@nextLink")
protected String allowedDataIngestionLocationsNextLink;
@JsonProperty("allowedDataStorageLocations")
protected List allowedDataStorageLocations;
@JsonProperty("allowedDataStorageLocations@nextLink")
protected String allowedDataStorageLocationsNextLink;
@JsonProperty("allowedInboundDataTransferSources")
protected ManagedAppDataTransferLevel allowedInboundDataTransferSources;
@JsonProperty("allowedOutboundClipboardSharingExceptionLength")
protected Integer allowedOutboundClipboardSharingExceptionLength;
@JsonProperty("allowedOutboundClipboardSharingLevel")
protected ManagedAppClipboardSharingLevel allowedOutboundClipboardSharingLevel;
@JsonProperty("allowedOutboundDataTransferDestinations")
protected ManagedAppDataTransferLevel allowedOutboundDataTransferDestinations;
@JsonProperty("appActionIfDeviceComplianceRequired")
protected ManagedAppRemediationAction appActionIfDeviceComplianceRequired;
@JsonProperty("appActionIfMaximumPinRetriesExceeded")
protected ManagedAppRemediationAction appActionIfMaximumPinRetriesExceeded;
@JsonProperty("appActionIfUnableToAuthenticateUser")
protected ManagedAppRemediationAction appActionIfUnableToAuthenticateUser;
@JsonProperty("blockDataIngestionIntoOrganizationDocuments")
protected Boolean blockDataIngestionIntoOrganizationDocuments;
@JsonProperty("contactSyncBlocked")
protected Boolean contactSyncBlocked;
@JsonProperty("dataBackupBlocked")
protected Boolean dataBackupBlocked;
@JsonProperty("deviceComplianceRequired")
protected Boolean deviceComplianceRequired;
@JsonProperty("dialerRestrictionLevel")
protected ManagedAppPhoneNumberRedirectLevel dialerRestrictionLevel;
@JsonProperty("disableAppPinIfDevicePinIsSet")
protected Boolean disableAppPinIfDevicePinIsSet;
@JsonProperty("fingerprintBlocked")
protected Boolean fingerprintBlocked;
@JsonProperty("managedBrowser")
protected ManagedBrowserType managedBrowser;
@JsonProperty("managedBrowserToOpenLinksRequired")
protected Boolean managedBrowserToOpenLinksRequired;
@JsonProperty("maximumAllowedDeviceThreatLevel")
protected ManagedAppDeviceThreatLevel maximumAllowedDeviceThreatLevel;
@JsonProperty("maximumPinRetries")
protected Integer maximumPinRetries;
@JsonProperty("maximumRequiredOsVersion")
protected String maximumRequiredOsVersion;
@JsonProperty("maximumWarningOsVersion")
protected String maximumWarningOsVersion;
@JsonProperty("maximumWipeOsVersion")
protected String maximumWipeOsVersion;
@JsonProperty("minimumPinLength")
protected Integer minimumPinLength;
@JsonProperty("minimumRequiredAppVersion")
protected String minimumRequiredAppVersion;
@JsonProperty("minimumRequiredOsVersion")
protected String minimumRequiredOsVersion;
@JsonProperty("minimumWarningAppVersion")
protected String minimumWarningAppVersion;
@JsonProperty("minimumWarningOsVersion")
protected String minimumWarningOsVersion;
@JsonProperty("minimumWipeAppVersion")
protected String minimumWipeAppVersion;
@JsonProperty("minimumWipeOsVersion")
protected String minimumWipeOsVersion;
@JsonProperty("mobileThreatDefenseRemediationAction")
protected ManagedAppRemediationAction mobileThreatDefenseRemediationAction;
@JsonProperty("notificationRestriction")
protected ManagedAppNotificationRestriction notificationRestriction;
@JsonProperty("organizationalCredentialsRequired")
protected Boolean organizationalCredentialsRequired;
@JsonProperty("periodBeforePinReset")
protected Duration periodBeforePinReset;
@JsonProperty("periodOfflineBeforeAccessCheck")
protected Duration periodOfflineBeforeAccessCheck;
@JsonProperty("periodOfflineBeforeWipeIsEnforced")
protected Duration periodOfflineBeforeWipeIsEnforced;
@JsonProperty("periodOnlineBeforeAccessCheck")
protected Duration periodOnlineBeforeAccessCheck;
@JsonProperty("pinCharacterSet")
protected ManagedAppPinCharacterSet pinCharacterSet;
@JsonProperty("pinRequired")
protected Boolean pinRequired;
@JsonProperty("pinRequiredInsteadOfBiometricTimeout")
protected Duration pinRequiredInsteadOfBiometricTimeout;
@JsonProperty("previousPinBlockCount")
protected Integer previousPinBlockCount;
@JsonProperty("printBlocked")
protected Boolean printBlocked;
@JsonProperty("saveAsBlocked")
protected Boolean saveAsBlocked;
@JsonProperty("simplePinBlocked")
protected Boolean simplePinBlocked;
protected ManagedAppProtection() {
super();
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Data storage locations where a user may store managed data.”
*
* @return property allowedDataIngestionLocations
*/
@Property(name="allowedDataIngestionLocations")
@JsonIgnore
public CollectionPage getAllowedDataIngestionLocations() {
return new CollectionPage(contextPath, ManagedAppDataIngestionLocation.class, this.allowedDataIngestionLocations, Optional.ofNullable(allowedDataIngestionLocationsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* allowedDataIngestionLocations} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Data storage locations where a user may store managed data.”
*
* @param allowedDataIngestionLocations
* new value of {@code allowedDataIngestionLocations} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code allowedDataIngestionLocations} field changed
*/
public ManagedAppProtection withAllowedDataIngestionLocations(List allowedDataIngestionLocations) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("allowedDataIngestionLocations");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.allowedDataIngestionLocations = allowedDataIngestionLocations;
return _x;
}
/**
* “Data storage locations where a user may store managed data.”
*
* @param options
* specify connect and read timeouts
* @return property allowedDataIngestionLocations
*/
@Property(name="allowedDataIngestionLocations")
@JsonIgnore
public CollectionPage getAllowedDataIngestionLocations(HttpRequestOptions options) {
return new CollectionPage(contextPath, ManagedAppDataIngestionLocation.class, this.allowedDataIngestionLocations, Optional.ofNullable(allowedDataIngestionLocationsNextLink), Collections.emptyList(), options);
}
/**
* “Data storage locations where a user may store managed data.”
*
* @return property allowedDataStorageLocations
*/
@Property(name="allowedDataStorageLocations")
@JsonIgnore
public CollectionPage getAllowedDataStorageLocations() {
return new CollectionPage(contextPath, ManagedAppDataStorageLocation.class, this.allowedDataStorageLocations, Optional.ofNullable(allowedDataStorageLocationsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* allowedDataStorageLocations} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Data storage locations where a user may store managed data.”
*
* @param allowedDataStorageLocations
* new value of {@code allowedDataStorageLocations} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code allowedDataStorageLocations} field changed
*/
public ManagedAppProtection withAllowedDataStorageLocations(List allowedDataStorageLocations) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("allowedDataStorageLocations");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.allowedDataStorageLocations = allowedDataStorageLocations;
return _x;
}
/**
* “Data storage locations where a user may store managed data.”
*
* @param options
* specify connect and read timeouts
* @return property allowedDataStorageLocations
*/
@Property(name="allowedDataStorageLocations")
@JsonIgnore
public CollectionPage getAllowedDataStorageLocations(HttpRequestOptions options) {
return new CollectionPage(contextPath, ManagedAppDataStorageLocation.class, this.allowedDataStorageLocations, Optional.ofNullable(allowedDataStorageLocationsNextLink), Collections.emptyList(), options);
}
/**
* “Sources from which data is allowed to be transferred.”
*
* @return property allowedInboundDataTransferSources
*/
@Property(name="allowedInboundDataTransferSources")
@JsonIgnore
public Optional getAllowedInboundDataTransferSources() {
return Optional.ofNullable(allowedInboundDataTransferSources);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* allowedInboundDataTransferSources} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Sources from which data is allowed to be transferred.”
*
* @param allowedInboundDataTransferSources
* new value of {@code allowedInboundDataTransferSources} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code allowedInboundDataTransferSources} field changed
*/
public ManagedAppProtection withAllowedInboundDataTransferSources(ManagedAppDataTransferLevel allowedInboundDataTransferSources) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("allowedInboundDataTransferSources");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.allowedInboundDataTransferSources = allowedInboundDataTransferSources;
return _x;
}
/**
* “Specify the number of characters that may be cut or copied from Org data and
* accounts to any application. This setting overrides the
* AllowedOutboundClipboardSharingLevel restriction. Default value of '0' means no
* exception is allowed.”
*
* @return property allowedOutboundClipboardSharingExceptionLength
*/
@Property(name="allowedOutboundClipboardSharingExceptionLength")
@JsonIgnore
public Optional getAllowedOutboundClipboardSharingExceptionLength() {
return Optional.ofNullable(allowedOutboundClipboardSharingExceptionLength);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* allowedOutboundClipboardSharingExceptionLength} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Specify the number of characters that may be cut or copied from Org data and
* accounts to any application. This setting overrides the
* AllowedOutboundClipboardSharingLevel restriction. Default value of '0' means no
* exception is allowed.”
*
* @param allowedOutboundClipboardSharingExceptionLength
* new value of {@code allowedOutboundClipboardSharingExceptionLength} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code allowedOutboundClipboardSharingExceptionLength} field changed
*/
public ManagedAppProtection withAllowedOutboundClipboardSharingExceptionLength(Integer allowedOutboundClipboardSharingExceptionLength) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("allowedOutboundClipboardSharingExceptionLength");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.allowedOutboundClipboardSharingExceptionLength = allowedOutboundClipboardSharingExceptionLength;
return _x;
}
/**
* “The level to which the clipboard may be shared between apps on the managed
* device.”
*
* @return property allowedOutboundClipboardSharingLevel
*/
@Property(name="allowedOutboundClipboardSharingLevel")
@JsonIgnore
public Optional getAllowedOutboundClipboardSharingLevel() {
return Optional.ofNullable(allowedOutboundClipboardSharingLevel);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* allowedOutboundClipboardSharingLevel} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “The level to which the clipboard may be shared between apps on the managed
* device.”
*
* @param allowedOutboundClipboardSharingLevel
* new value of {@code allowedOutboundClipboardSharingLevel} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code allowedOutboundClipboardSharingLevel} field changed
*/
public ManagedAppProtection withAllowedOutboundClipboardSharingLevel(ManagedAppClipboardSharingLevel allowedOutboundClipboardSharingLevel) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("allowedOutboundClipboardSharingLevel");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.allowedOutboundClipboardSharingLevel = allowedOutboundClipboardSharingLevel;
return _x;
}
/**
* “Destinations to which data is allowed to be transferred.”
*
* @return property allowedOutboundDataTransferDestinations
*/
@Property(name="allowedOutboundDataTransferDestinations")
@JsonIgnore
public Optional getAllowedOutboundDataTransferDestinations() {
return Optional.ofNullable(allowedOutboundDataTransferDestinations);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* allowedOutboundDataTransferDestinations} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Destinations to which data is allowed to be transferred.”
*
* @param allowedOutboundDataTransferDestinations
* new value of {@code allowedOutboundDataTransferDestinations} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code allowedOutboundDataTransferDestinations} field changed
*/
public ManagedAppProtection withAllowedOutboundDataTransferDestinations(ManagedAppDataTransferLevel allowedOutboundDataTransferDestinations) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("allowedOutboundDataTransferDestinations");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.allowedOutboundDataTransferDestinations = allowedOutboundDataTransferDestinations;
return _x;
}
/**
* “Defines a managed app behavior, either block or wipe, when the device is either
* rooted or jailbroken, if DeviceComplianceRequired is set to true.”
*
* @return property appActionIfDeviceComplianceRequired
*/
@Property(name="appActionIfDeviceComplianceRequired")
@JsonIgnore
public Optional getAppActionIfDeviceComplianceRequired() {
return Optional.ofNullable(appActionIfDeviceComplianceRequired);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* appActionIfDeviceComplianceRequired} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Defines a managed app behavior, either block or wipe, when the device is either
* rooted or jailbroken, if DeviceComplianceRequired is set to true.”
*
* @param appActionIfDeviceComplianceRequired
* new value of {@code appActionIfDeviceComplianceRequired} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code appActionIfDeviceComplianceRequired} field changed
*/
public ManagedAppProtection withAppActionIfDeviceComplianceRequired(ManagedAppRemediationAction appActionIfDeviceComplianceRequired) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("appActionIfDeviceComplianceRequired");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.appActionIfDeviceComplianceRequired = appActionIfDeviceComplianceRequired;
return _x;
}
/**
* “Defines a managed app behavior, either block or wipe, based on maximum number of
* incorrect pin retry attempts.”
*
* @return property appActionIfMaximumPinRetriesExceeded
*/
@Property(name="appActionIfMaximumPinRetriesExceeded")
@JsonIgnore
public Optional getAppActionIfMaximumPinRetriesExceeded() {
return Optional.ofNullable(appActionIfMaximumPinRetriesExceeded);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* appActionIfMaximumPinRetriesExceeded} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Defines a managed app behavior, either block or wipe, based on maximum number of
* incorrect pin retry attempts.”
*
* @param appActionIfMaximumPinRetriesExceeded
* new value of {@code appActionIfMaximumPinRetriesExceeded} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code appActionIfMaximumPinRetriesExceeded} field changed
*/
public ManagedAppProtection withAppActionIfMaximumPinRetriesExceeded(ManagedAppRemediationAction appActionIfMaximumPinRetriesExceeded) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("appActionIfMaximumPinRetriesExceeded");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.appActionIfMaximumPinRetriesExceeded = appActionIfMaximumPinRetriesExceeded;
return _x;
}
/**
* “If set, it will specify what action to take in the case where the user is unable
* to checkin because their authentication token is invalid. This happens when the
* user is deleted or disabled in AAD.”
*
* @return property appActionIfUnableToAuthenticateUser
*/
@Property(name="appActionIfUnableToAuthenticateUser")
@JsonIgnore
public Optional getAppActionIfUnableToAuthenticateUser() {
return Optional.ofNullable(appActionIfUnableToAuthenticateUser);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* appActionIfUnableToAuthenticateUser} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “If set, it will specify what action to take in the case where the user is unable
* to checkin because their authentication token is invalid. This happens when the
* user is deleted or disabled in AAD.”
*
* @param appActionIfUnableToAuthenticateUser
* new value of {@code appActionIfUnableToAuthenticateUser} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code appActionIfUnableToAuthenticateUser} field changed
*/
public ManagedAppProtection withAppActionIfUnableToAuthenticateUser(ManagedAppRemediationAction appActionIfUnableToAuthenticateUser) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("appActionIfUnableToAuthenticateUser");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.appActionIfUnableToAuthenticateUser = appActionIfUnableToAuthenticateUser;
return _x;
}
/**
* “Indicates whether a user can bring data into org documents.”
*
* @return property blockDataIngestionIntoOrganizationDocuments
*/
@Property(name="blockDataIngestionIntoOrganizationDocuments")
@JsonIgnore
public Optional getBlockDataIngestionIntoOrganizationDocuments() {
return Optional.ofNullable(blockDataIngestionIntoOrganizationDocuments);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* blockDataIngestionIntoOrganizationDocuments} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Indicates whether a user can bring data into org documents.”
*
* @param blockDataIngestionIntoOrganizationDocuments
* new value of {@code blockDataIngestionIntoOrganizationDocuments} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code blockDataIngestionIntoOrganizationDocuments} field changed
*/
public ManagedAppProtection withBlockDataIngestionIntoOrganizationDocuments(Boolean blockDataIngestionIntoOrganizationDocuments) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("blockDataIngestionIntoOrganizationDocuments");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.blockDataIngestionIntoOrganizationDocuments = blockDataIngestionIntoOrganizationDocuments;
return _x;
}
/**
* “Indicates whether contacts can be synced to the user's device.”
*
* @return property contactSyncBlocked
*/
@Property(name="contactSyncBlocked")
@JsonIgnore
public Optional getContactSyncBlocked() {
return Optional.ofNullable(contactSyncBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* contactSyncBlocked} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Indicates whether contacts can be synced to the user's device.”
*
* @param contactSyncBlocked
* new value of {@code contactSyncBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contactSyncBlocked} field changed
*/
public ManagedAppProtection withContactSyncBlocked(Boolean contactSyncBlocked) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("contactSyncBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.contactSyncBlocked = contactSyncBlocked;
return _x;
}
/**
* “Indicates whether the backup of a managed app's data is blocked.”
*
* @return property dataBackupBlocked
*/
@Property(name="dataBackupBlocked")
@JsonIgnore
public Optional getDataBackupBlocked() {
return Optional.ofNullable(dataBackupBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code dataBackupBlocked
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates whether the backup of a managed app's data is blocked.”
*
* @param dataBackupBlocked
* new value of {@code dataBackupBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code dataBackupBlocked} field changed
*/
public ManagedAppProtection withDataBackupBlocked(Boolean dataBackupBlocked) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("dataBackupBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.dataBackupBlocked = dataBackupBlocked;
return _x;
}
/**
* “Indicates whether device compliance is required.”
*
* @return property deviceComplianceRequired
*/
@Property(name="deviceComplianceRequired")
@JsonIgnore
public Optional getDeviceComplianceRequired() {
return Optional.ofNullable(deviceComplianceRequired);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceComplianceRequired} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether device compliance is required.”
*
* @param deviceComplianceRequired
* new value of {@code deviceComplianceRequired} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceComplianceRequired} field changed
*/
public ManagedAppProtection withDeviceComplianceRequired(Boolean deviceComplianceRequired) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("deviceComplianceRequired");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.deviceComplianceRequired = deviceComplianceRequired;
return _x;
}
/**
* “The classes of dialer apps that are allowed to click-to-open a phone number.”
*
* @return property dialerRestrictionLevel
*/
@Property(name="dialerRestrictionLevel")
@JsonIgnore
public Optional getDialerRestrictionLevel() {
return Optional.ofNullable(dialerRestrictionLevel);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* dialerRestrictionLevel} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The classes of dialer apps that are allowed to click-to-open a phone number.”
*
* @param dialerRestrictionLevel
* new value of {@code dialerRestrictionLevel} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code dialerRestrictionLevel} field changed
*/
public ManagedAppProtection withDialerRestrictionLevel(ManagedAppPhoneNumberRedirectLevel dialerRestrictionLevel) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("dialerRestrictionLevel");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.dialerRestrictionLevel = dialerRestrictionLevel;
return _x;
}
/**
* “Indicates whether use of the app pin is required if the device pin is set.”
*
* @return property disableAppPinIfDevicePinIsSet
*/
@Property(name="disableAppPinIfDevicePinIsSet")
@JsonIgnore
public Optional getDisableAppPinIfDevicePinIsSet() {
return Optional.ofNullable(disableAppPinIfDevicePinIsSet);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* disableAppPinIfDevicePinIsSet} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates whether use of the app pin is required if the device pin is set.”
*
* @param disableAppPinIfDevicePinIsSet
* new value of {@code disableAppPinIfDevicePinIsSet} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code disableAppPinIfDevicePinIsSet} field changed
*/
public ManagedAppProtection withDisableAppPinIfDevicePinIsSet(Boolean disableAppPinIfDevicePinIsSet) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("disableAppPinIfDevicePinIsSet");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.disableAppPinIfDevicePinIsSet = disableAppPinIfDevicePinIsSet;
return _x;
}
/**
* “Indicates whether use of the fingerprint reader is allowed in place of a pin if
* PinRequired is set to True.”
*
* @return property fingerprintBlocked
*/
@Property(name="fingerprintBlocked")
@JsonIgnore
public Optional getFingerprintBlocked() {
return Optional.ofNullable(fingerprintBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* fingerprintBlocked} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Indicates whether use of the fingerprint reader is allowed in place of a pin if
* PinRequired is set to True.”
*
* @param fingerprintBlocked
* new value of {@code fingerprintBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code fingerprintBlocked} field changed
*/
public ManagedAppProtection withFingerprintBlocked(Boolean fingerprintBlocked) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("fingerprintBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.fingerprintBlocked = fingerprintBlocked;
return _x;
}
/**
* “Indicates in which managed browser(s) that internet links should be opened. When
* this property is configured, ManagedBrowserToOpenLinksRequired should be true.”
*
* @return property managedBrowser
*/
@Property(name="managedBrowser")
@JsonIgnore
public Optional getManagedBrowser() {
return Optional.ofNullable(managedBrowser);
}
/**
* Returns an immutable copy of {@code this} with just the {@code managedBrowser}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates in which managed browser(s) that internet links should be opened. When
* this property is configured, ManagedBrowserToOpenLinksRequired should be true.”
*
* @param managedBrowser
* new value of {@code managedBrowser} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code managedBrowser} field changed
*/
public ManagedAppProtection withManagedBrowser(ManagedBrowserType managedBrowser) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("managedBrowser");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.managedBrowser = managedBrowser;
return _x;
}
/**
* “Indicates whether internet links should be opened in the managed browser app, or
* any custom browser specified by CustomBrowserProtocol (for iOS) or
* CustomBrowserPackageId/CustomBrowserDisplayName (for Android)”
*
* @return property managedBrowserToOpenLinksRequired
*/
@Property(name="managedBrowserToOpenLinksRequired")
@JsonIgnore
public Optional getManagedBrowserToOpenLinksRequired() {
return Optional.ofNullable(managedBrowserToOpenLinksRequired);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* managedBrowserToOpenLinksRequired} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Indicates whether internet links should be opened in the managed browser app, or
* any custom browser specified by CustomBrowserProtocol (for iOS) or
* CustomBrowserPackageId/CustomBrowserDisplayName (for Android)”
*
* @param managedBrowserToOpenLinksRequired
* new value of {@code managedBrowserToOpenLinksRequired} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code managedBrowserToOpenLinksRequired} field changed
*/
public ManagedAppProtection withManagedBrowserToOpenLinksRequired(Boolean managedBrowserToOpenLinksRequired) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("managedBrowserToOpenLinksRequired");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.managedBrowserToOpenLinksRequired = managedBrowserToOpenLinksRequired;
return _x;
}
/**
* “Maximum allowed device threat level, as reported by the MTD app”
*
* @return property maximumAllowedDeviceThreatLevel
*/
@Property(name="maximumAllowedDeviceThreatLevel")
@JsonIgnore
public Optional getMaximumAllowedDeviceThreatLevel() {
return Optional.ofNullable(maximumAllowedDeviceThreatLevel);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* maximumAllowedDeviceThreatLevel} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Maximum allowed device threat level, as reported by the MTD app”
*
* @param maximumAllowedDeviceThreatLevel
* new value of {@code maximumAllowedDeviceThreatLevel} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code maximumAllowedDeviceThreatLevel} field changed
*/
public ManagedAppProtection withMaximumAllowedDeviceThreatLevel(ManagedAppDeviceThreatLevel maximumAllowedDeviceThreatLevel) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("maximumAllowedDeviceThreatLevel");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.maximumAllowedDeviceThreatLevel = maximumAllowedDeviceThreatLevel;
return _x;
}
/**
* “Maximum number of incorrect pin retry attempts before the managed app is either
* blocked or wiped.”
*
* @return property maximumPinRetries
*/
@Property(name="maximumPinRetries")
@JsonIgnore
public Optional getMaximumPinRetries() {
return Optional.ofNullable(maximumPinRetries);
}
/**
* Returns an immutable copy of {@code this} with just the {@code maximumPinRetries
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Maximum number of incorrect pin retry attempts before the managed app is either
* blocked or wiped.”
*
* @param maximumPinRetries
* new value of {@code maximumPinRetries} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code maximumPinRetries} field changed
*/
public ManagedAppProtection withMaximumPinRetries(Integer maximumPinRetries) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("maximumPinRetries");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.maximumPinRetries = maximumPinRetries;
return _x;
}
/**
* “Versions bigger than the specified version will block the managed app from
* accessing company data.”
*
* @return property maximumRequiredOsVersion
*/
@Property(name="maximumRequiredOsVersion")
@JsonIgnore
public Optional getMaximumRequiredOsVersion() {
return Optional.ofNullable(maximumRequiredOsVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* maximumRequiredOsVersion} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Versions bigger than the specified version will block the managed app from
* accessing company data.”
*
* @param maximumRequiredOsVersion
* new value of {@code maximumRequiredOsVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code maximumRequiredOsVersion} field changed
*/
public ManagedAppProtection withMaximumRequiredOsVersion(String maximumRequiredOsVersion) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("maximumRequiredOsVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.maximumRequiredOsVersion = maximumRequiredOsVersion;
return _x;
}
/**
* “Versions bigger than the specified version will block the managed app from
* accessing company data.”
*
* @return property maximumWarningOsVersion
*/
@Property(name="maximumWarningOsVersion")
@JsonIgnore
public Optional getMaximumWarningOsVersion() {
return Optional.ofNullable(maximumWarningOsVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* maximumWarningOsVersion} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Versions bigger than the specified version will block the managed app from
* accessing company data.”
*
* @param maximumWarningOsVersion
* new value of {@code maximumWarningOsVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code maximumWarningOsVersion} field changed
*/
public ManagedAppProtection withMaximumWarningOsVersion(String maximumWarningOsVersion) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("maximumWarningOsVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.maximumWarningOsVersion = maximumWarningOsVersion;
return _x;
}
/**
* “Versions bigger than the specified version will block the managed app from
* accessing company data.”
*
* @return property maximumWipeOsVersion
*/
@Property(name="maximumWipeOsVersion")
@JsonIgnore
public Optional getMaximumWipeOsVersion() {
return Optional.ofNullable(maximumWipeOsVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* maximumWipeOsVersion} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Versions bigger than the specified version will block the managed app from
* accessing company data.”
*
* @param maximumWipeOsVersion
* new value of {@code maximumWipeOsVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code maximumWipeOsVersion} field changed
*/
public ManagedAppProtection withMaximumWipeOsVersion(String maximumWipeOsVersion) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("maximumWipeOsVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.maximumWipeOsVersion = maximumWipeOsVersion;
return _x;
}
/**
* “Minimum pin length required for an app-level pin if PinRequired is set to True”
*
* @return property minimumPinLength
*/
@Property(name="minimumPinLength")
@JsonIgnore
public Optional getMinimumPinLength() {
return Optional.ofNullable(minimumPinLength);
}
/**
* Returns an immutable copy of {@code this} with just the {@code minimumPinLength}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Minimum pin length required for an app-level pin if PinRequired is set to True”
*
* @param minimumPinLength
* new value of {@code minimumPinLength} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code minimumPinLength} field changed
*/
public ManagedAppProtection withMinimumPinLength(Integer minimumPinLength) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("minimumPinLength");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.minimumPinLength = minimumPinLength;
return _x;
}
/**
* “Versions less than the specified version will block the managed app from
* accessing company data.”
*
* @return property minimumRequiredAppVersion
*/
@Property(name="minimumRequiredAppVersion")
@JsonIgnore
public Optional getMinimumRequiredAppVersion() {
return Optional.ofNullable(minimumRequiredAppVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* minimumRequiredAppVersion} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Versions less than the specified version will block the managed app from
* accessing company data.”
*
* @param minimumRequiredAppVersion
* new value of {@code minimumRequiredAppVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code minimumRequiredAppVersion} field changed
*/
public ManagedAppProtection withMinimumRequiredAppVersion(String minimumRequiredAppVersion) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("minimumRequiredAppVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.minimumRequiredAppVersion = minimumRequiredAppVersion;
return _x;
}
/**
* “Versions less than the specified version will block the managed app from
* accessing company data.”
*
* @return property minimumRequiredOsVersion
*/
@Property(name="minimumRequiredOsVersion")
@JsonIgnore
public Optional getMinimumRequiredOsVersion() {
return Optional.ofNullable(minimumRequiredOsVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* minimumRequiredOsVersion} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Versions less than the specified version will block the managed app from
* accessing company data.”
*
* @param minimumRequiredOsVersion
* new value of {@code minimumRequiredOsVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code minimumRequiredOsVersion} field changed
*/
public ManagedAppProtection withMinimumRequiredOsVersion(String minimumRequiredOsVersion) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("minimumRequiredOsVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.minimumRequiredOsVersion = minimumRequiredOsVersion;
return _x;
}
/**
* “Versions less than the specified version will result in warning message on the
* managed app.”
*
* @return property minimumWarningAppVersion
*/
@Property(name="minimumWarningAppVersion")
@JsonIgnore
public Optional getMinimumWarningAppVersion() {
return Optional.ofNullable(minimumWarningAppVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* minimumWarningAppVersion} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Versions less than the specified version will result in warning message on the
* managed app.”
*
* @param minimumWarningAppVersion
* new value of {@code minimumWarningAppVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code minimumWarningAppVersion} field changed
*/
public ManagedAppProtection withMinimumWarningAppVersion(String minimumWarningAppVersion) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("minimumWarningAppVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.minimumWarningAppVersion = minimumWarningAppVersion;
return _x;
}
/**
* “Versions less than the specified version will result in warning message on the
* managed app from accessing company data.”
*
* @return property minimumWarningOsVersion
*/
@Property(name="minimumWarningOsVersion")
@JsonIgnore
public Optional getMinimumWarningOsVersion() {
return Optional.ofNullable(minimumWarningOsVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* minimumWarningOsVersion} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Versions less than the specified version will result in warning message on the
* managed app from accessing company data.”
*
* @param minimumWarningOsVersion
* new value of {@code minimumWarningOsVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code minimumWarningOsVersion} field changed
*/
public ManagedAppProtection withMinimumWarningOsVersion(String minimumWarningOsVersion) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("minimumWarningOsVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.minimumWarningOsVersion = minimumWarningOsVersion;
return _x;
}
/**
* “Versions less than or equal to the specified version will wipe the managed app
* and the associated company data.”
*
* @return property minimumWipeAppVersion
*/
@Property(name="minimumWipeAppVersion")
@JsonIgnore
public Optional getMinimumWipeAppVersion() {
return Optional.ofNullable(minimumWipeAppVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* minimumWipeAppVersion} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Versions less than or equal to the specified version will wipe the managed app
* and the associated company data.”
*
* @param minimumWipeAppVersion
* new value of {@code minimumWipeAppVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code minimumWipeAppVersion} field changed
*/
public ManagedAppProtection withMinimumWipeAppVersion(String minimumWipeAppVersion) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("minimumWipeAppVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.minimumWipeAppVersion = minimumWipeAppVersion;
return _x;
}
/**
* “Versions less than or equal to the specified version will wipe the managed app
* and the associated company data.”
*
* @return property minimumWipeOsVersion
*/
@Property(name="minimumWipeOsVersion")
@JsonIgnore
public Optional getMinimumWipeOsVersion() {
return Optional.ofNullable(minimumWipeOsVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* minimumWipeOsVersion} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Versions less than or equal to the specified version will wipe the managed app
* and the associated company data.”
*
* @param minimumWipeOsVersion
* new value of {@code minimumWipeOsVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code minimumWipeOsVersion} field changed
*/
public ManagedAppProtection withMinimumWipeOsVersion(String minimumWipeOsVersion) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("minimumWipeOsVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.minimumWipeOsVersion = minimumWipeOsVersion;
return _x;
}
/**
* “Determines what action to take if the mobile threat defense threat threshold isn
* 't met. Warn isn't a supported value for this property”
*
* @return property mobileThreatDefenseRemediationAction
*/
@Property(name="mobileThreatDefenseRemediationAction")
@JsonIgnore
public Optional getMobileThreatDefenseRemediationAction() {
return Optional.ofNullable(mobileThreatDefenseRemediationAction);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* mobileThreatDefenseRemediationAction} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Determines what action to take if the mobile threat defense threat threshold isn
* 't met. Warn isn't a supported value for this property”
*
* @param mobileThreatDefenseRemediationAction
* new value of {@code mobileThreatDefenseRemediationAction} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code mobileThreatDefenseRemediationAction} field changed
*/
public ManagedAppProtection withMobileThreatDefenseRemediationAction(ManagedAppRemediationAction mobileThreatDefenseRemediationAction) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("mobileThreatDefenseRemediationAction");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.mobileThreatDefenseRemediationAction = mobileThreatDefenseRemediationAction;
return _x;
}
/**
* “Specify app notification restriction”
*
* @return property notificationRestriction
*/
@Property(name="notificationRestriction")
@JsonIgnore
public Optional getNotificationRestriction() {
return Optional.ofNullable(notificationRestriction);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* notificationRestriction} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Specify app notification restriction”
*
* @param notificationRestriction
* new value of {@code notificationRestriction} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code notificationRestriction} field changed
*/
public ManagedAppProtection withNotificationRestriction(ManagedAppNotificationRestriction notificationRestriction) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("notificationRestriction");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.notificationRestriction = notificationRestriction;
return _x;
}
/**
* “Indicates whether organizational credentials are required for app use.”
*
* @return property organizationalCredentialsRequired
*/
@Property(name="organizationalCredentialsRequired")
@JsonIgnore
public Optional getOrganizationalCredentialsRequired() {
return Optional.ofNullable(organizationalCredentialsRequired);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* organizationalCredentialsRequired} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Indicates whether organizational credentials are required for app use.”
*
* @param organizationalCredentialsRequired
* new value of {@code organizationalCredentialsRequired} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code organizationalCredentialsRequired} field changed
*/
public ManagedAppProtection withOrganizationalCredentialsRequired(Boolean organizationalCredentialsRequired) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("organizationalCredentialsRequired");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.organizationalCredentialsRequired = organizationalCredentialsRequired;
return _x;
}
/**
* “TimePeriod before the all-level pin must be reset if PinRequired is set to True.”
*
* @return property periodBeforePinReset
*/
@Property(name="periodBeforePinReset")
@JsonIgnore
public Optional getPeriodBeforePinReset() {
return Optional.ofNullable(periodBeforePinReset);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* periodBeforePinReset} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “TimePeriod before the all-level pin must be reset if PinRequired is set to True.”
*
* @param periodBeforePinReset
* new value of {@code periodBeforePinReset} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code periodBeforePinReset} field changed
*/
public ManagedAppProtection withPeriodBeforePinReset(Duration periodBeforePinReset) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("periodBeforePinReset");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.periodBeforePinReset = periodBeforePinReset;
return _x;
}
/**
* “The period after which access is checked when the device is not connected to the
* internet.”
*
* @return property periodOfflineBeforeAccessCheck
*/
@Property(name="periodOfflineBeforeAccessCheck")
@JsonIgnore
public Optional getPeriodOfflineBeforeAccessCheck() {
return Optional.ofNullable(periodOfflineBeforeAccessCheck);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* periodOfflineBeforeAccessCheck} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The period after which access is checked when the device is not connected to the
* internet.”
*
* @param periodOfflineBeforeAccessCheck
* new value of {@code periodOfflineBeforeAccessCheck} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code periodOfflineBeforeAccessCheck} field changed
*/
public ManagedAppProtection withPeriodOfflineBeforeAccessCheck(Duration periodOfflineBeforeAccessCheck) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("periodOfflineBeforeAccessCheck");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.periodOfflineBeforeAccessCheck = periodOfflineBeforeAccessCheck;
return _x;
}
/**
* “The amount of time an app is allowed to remain disconnected from the internet
* before all managed data it is wiped.”
*
* @return property periodOfflineBeforeWipeIsEnforced
*/
@Property(name="periodOfflineBeforeWipeIsEnforced")
@JsonIgnore
public Optional getPeriodOfflineBeforeWipeIsEnforced() {
return Optional.ofNullable(periodOfflineBeforeWipeIsEnforced);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* periodOfflineBeforeWipeIsEnforced} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The amount of time an app is allowed to remain disconnected from the internet
* before all managed data it is wiped.”
*
* @param periodOfflineBeforeWipeIsEnforced
* new value of {@code periodOfflineBeforeWipeIsEnforced} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code periodOfflineBeforeWipeIsEnforced} field changed
*/
public ManagedAppProtection withPeriodOfflineBeforeWipeIsEnforced(Duration periodOfflineBeforeWipeIsEnforced) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("periodOfflineBeforeWipeIsEnforced");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.periodOfflineBeforeWipeIsEnforced = periodOfflineBeforeWipeIsEnforced;
return _x;
}
/**
* “The period after which access is checked when the device is connected to the
* internet.”
*
* @return property periodOnlineBeforeAccessCheck
*/
@Property(name="periodOnlineBeforeAccessCheck")
@JsonIgnore
public Optional getPeriodOnlineBeforeAccessCheck() {
return Optional.ofNullable(periodOnlineBeforeAccessCheck);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* periodOnlineBeforeAccessCheck} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The period after which access is checked when the device is connected to the
* internet.”
*
* @param periodOnlineBeforeAccessCheck
* new value of {@code periodOnlineBeforeAccessCheck} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code periodOnlineBeforeAccessCheck} field changed
*/
public ManagedAppProtection withPeriodOnlineBeforeAccessCheck(Duration periodOnlineBeforeAccessCheck) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("periodOnlineBeforeAccessCheck");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.periodOnlineBeforeAccessCheck = periodOnlineBeforeAccessCheck;
return _x;
}
/**
* “Character set which may be used for an app-level pin if PinRequired is set to
* True.”
*
* @return property pinCharacterSet
*/
@Property(name="pinCharacterSet")
@JsonIgnore
public Optional getPinCharacterSet() {
return Optional.ofNullable(pinCharacterSet);
}
/**
* Returns an immutable copy of {@code this} with just the {@code pinCharacterSet}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Character set which may be used for an app-level pin if PinRequired is set to
* True.”
*
* @param pinCharacterSet
* new value of {@code pinCharacterSet} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code pinCharacterSet} field changed
*/
public ManagedAppProtection withPinCharacterSet(ManagedAppPinCharacterSet pinCharacterSet) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("pinCharacterSet");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.pinCharacterSet = pinCharacterSet;
return _x;
}
/**
* “Indicates whether an app-level pin is required.”
*
* @return property pinRequired
*/
@Property(name="pinRequired")
@JsonIgnore
public Optional getPinRequired() {
return Optional.ofNullable(pinRequired);
}
/**
* Returns an immutable copy of {@code this} with just the {@code pinRequired}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates whether an app-level pin is required.”
*
* @param pinRequired
* new value of {@code pinRequired} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code pinRequired} field changed
*/
public ManagedAppProtection withPinRequired(Boolean pinRequired) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("pinRequired");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.pinRequired = pinRequired;
return _x;
}
/**
* “Timeout in minutes for an app pin instead of non biometrics passcode”
*
* @return property pinRequiredInsteadOfBiometricTimeout
*/
@Property(name="pinRequiredInsteadOfBiometricTimeout")
@JsonIgnore
public Optional getPinRequiredInsteadOfBiometricTimeout() {
return Optional.ofNullable(pinRequiredInsteadOfBiometricTimeout);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* pinRequiredInsteadOfBiometricTimeout} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Timeout in minutes for an app pin instead of non biometrics passcode”
*
* @param pinRequiredInsteadOfBiometricTimeout
* new value of {@code pinRequiredInsteadOfBiometricTimeout} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code pinRequiredInsteadOfBiometricTimeout} field changed
*/
public ManagedAppProtection withPinRequiredInsteadOfBiometricTimeout(Duration pinRequiredInsteadOfBiometricTimeout) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("pinRequiredInsteadOfBiometricTimeout");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.pinRequiredInsteadOfBiometricTimeout = pinRequiredInsteadOfBiometricTimeout;
return _x;
}
/**
* “Requires a pin to be unique from the number specified in this property.”
*
* @return property previousPinBlockCount
*/
@Property(name="previousPinBlockCount")
@JsonIgnore
public Optional getPreviousPinBlockCount() {
return Optional.ofNullable(previousPinBlockCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* previousPinBlockCount} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Requires a pin to be unique from the number specified in this property.”
*
* @param previousPinBlockCount
* new value of {@code previousPinBlockCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code previousPinBlockCount} field changed
*/
public ManagedAppProtection withPreviousPinBlockCount(Integer previousPinBlockCount) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("previousPinBlockCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.previousPinBlockCount = previousPinBlockCount;
return _x;
}
/**
* “Indicates whether printing is allowed from managed apps.”
*
* @return property printBlocked
*/
@Property(name="printBlocked")
@JsonIgnore
public Optional getPrintBlocked() {
return Optional.ofNullable(printBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code printBlocked}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates whether printing is allowed from managed apps.”
*
* @param printBlocked
* new value of {@code printBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code printBlocked} field changed
*/
public ManagedAppProtection withPrintBlocked(Boolean printBlocked) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("printBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.printBlocked = printBlocked;
return _x;
}
/**
* “Indicates whether users may use the "Save As" menu item to save a copy of
* protected files.”
*
* @return property saveAsBlocked
*/
@Property(name="saveAsBlocked")
@JsonIgnore
public Optional getSaveAsBlocked() {
return Optional.ofNullable(saveAsBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code saveAsBlocked}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates whether users may use the "Save As" menu item to save a copy of
* protected files.”
*
* @param saveAsBlocked
* new value of {@code saveAsBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code saveAsBlocked} field changed
*/
public ManagedAppProtection withSaveAsBlocked(Boolean saveAsBlocked) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("saveAsBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.saveAsBlocked = saveAsBlocked;
return _x;
}
/**
* “Indicates whether simplePin is blocked.”
*
* @return property simplePinBlocked
*/
@Property(name="simplePinBlocked")
@JsonIgnore
public Optional getSimplePinBlocked() {
return Optional.ofNullable(simplePinBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code simplePinBlocked}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates whether simplePin is blocked.”
*
* @param simplePinBlocked
* new value of {@code simplePinBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code simplePinBlocked} field changed
*/
public ManagedAppProtection withSimplePinBlocked(Boolean simplePinBlocked) {
ManagedAppProtection _x = _copy();
_x.changedFields = changedFields.add("simplePinBlocked");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppProtection");
_x.simplePinBlocked = simplePinBlocked;
return _x;
}
public ManagedAppProtection withUnmappedField(String name, String value) {
ManagedAppProtection _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ManagedAppProtection patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
ManagedAppProtection _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ManagedAppProtection put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
ManagedAppProtection _x = _copy();
_x.changedFields = null;
return _x;
}
private ManagedAppProtection _copy() {
ManagedAppProtection _x = new ManagedAppProtection();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.version = version;
_x.allowedDataIngestionLocations = allowedDataIngestionLocations;
_x.allowedDataStorageLocations = allowedDataStorageLocations;
_x.allowedInboundDataTransferSources = allowedInboundDataTransferSources;
_x.allowedOutboundClipboardSharingExceptionLength = allowedOutboundClipboardSharingExceptionLength;
_x.allowedOutboundClipboardSharingLevel = allowedOutboundClipboardSharingLevel;
_x.allowedOutboundDataTransferDestinations = allowedOutboundDataTransferDestinations;
_x.appActionIfDeviceComplianceRequired = appActionIfDeviceComplianceRequired;
_x.appActionIfMaximumPinRetriesExceeded = appActionIfMaximumPinRetriesExceeded;
_x.appActionIfUnableToAuthenticateUser = appActionIfUnableToAuthenticateUser;
_x.blockDataIngestionIntoOrganizationDocuments = blockDataIngestionIntoOrganizationDocuments;
_x.contactSyncBlocked = contactSyncBlocked;
_x.dataBackupBlocked = dataBackupBlocked;
_x.deviceComplianceRequired = deviceComplianceRequired;
_x.dialerRestrictionLevel = dialerRestrictionLevel;
_x.disableAppPinIfDevicePinIsSet = disableAppPinIfDevicePinIsSet;
_x.fingerprintBlocked = fingerprintBlocked;
_x.managedBrowser = managedBrowser;
_x.managedBrowserToOpenLinksRequired = managedBrowserToOpenLinksRequired;
_x.maximumAllowedDeviceThreatLevel = maximumAllowedDeviceThreatLevel;
_x.maximumPinRetries = maximumPinRetries;
_x.maximumRequiredOsVersion = maximumRequiredOsVersion;
_x.maximumWarningOsVersion = maximumWarningOsVersion;
_x.maximumWipeOsVersion = maximumWipeOsVersion;
_x.minimumPinLength = minimumPinLength;
_x.minimumRequiredAppVersion = minimumRequiredAppVersion;
_x.minimumRequiredOsVersion = minimumRequiredOsVersion;
_x.minimumWarningAppVersion = minimumWarningAppVersion;
_x.minimumWarningOsVersion = minimumWarningOsVersion;
_x.minimumWipeAppVersion = minimumWipeAppVersion;
_x.minimumWipeOsVersion = minimumWipeOsVersion;
_x.mobileThreatDefenseRemediationAction = mobileThreatDefenseRemediationAction;
_x.notificationRestriction = notificationRestriction;
_x.organizationalCredentialsRequired = organizationalCredentialsRequired;
_x.periodBeforePinReset = periodBeforePinReset;
_x.periodOfflineBeforeAccessCheck = periodOfflineBeforeAccessCheck;
_x.periodOfflineBeforeWipeIsEnforced = periodOfflineBeforeWipeIsEnforced;
_x.periodOnlineBeforeAccessCheck = periodOnlineBeforeAccessCheck;
_x.pinCharacterSet = pinCharacterSet;
_x.pinRequired = pinRequired;
_x.pinRequiredInsteadOfBiometricTimeout = pinRequiredInsteadOfBiometricTimeout;
_x.previousPinBlockCount = previousPinBlockCount;
_x.printBlocked = printBlocked;
_x.saveAsBlocked = saveAsBlocked;
_x.simplePinBlocked = simplePinBlocked;
return _x;
}
@Action(name = "targetApps")
@JsonIgnore
public ActionRequestNoReturn targetApps(List apps) {
Map _parameters = ParameterMap
.put("apps", "Collection(microsoft.graph.managedMobileApp)", apps)
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("microsoft.graph.targetApps"), _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ManagedAppProtection[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("allowedDataIngestionLocations=");
b.append(this.allowedDataIngestionLocations);
b.append(", ");
b.append("allowedDataStorageLocations=");
b.append(this.allowedDataStorageLocations);
b.append(", ");
b.append("allowedInboundDataTransferSources=");
b.append(this.allowedInboundDataTransferSources);
b.append(", ");
b.append("allowedOutboundClipboardSharingExceptionLength=");
b.append(this.allowedOutboundClipboardSharingExceptionLength);
b.append(", ");
b.append("allowedOutboundClipboardSharingLevel=");
b.append(this.allowedOutboundClipboardSharingLevel);
b.append(", ");
b.append("allowedOutboundDataTransferDestinations=");
b.append(this.allowedOutboundDataTransferDestinations);
b.append(", ");
b.append("appActionIfDeviceComplianceRequired=");
b.append(this.appActionIfDeviceComplianceRequired);
b.append(", ");
b.append("appActionIfMaximumPinRetriesExceeded=");
b.append(this.appActionIfMaximumPinRetriesExceeded);
b.append(", ");
b.append("appActionIfUnableToAuthenticateUser=");
b.append(this.appActionIfUnableToAuthenticateUser);
b.append(", ");
b.append("blockDataIngestionIntoOrganizationDocuments=");
b.append(this.blockDataIngestionIntoOrganizationDocuments);
b.append(", ");
b.append("contactSyncBlocked=");
b.append(this.contactSyncBlocked);
b.append(", ");
b.append("dataBackupBlocked=");
b.append(this.dataBackupBlocked);
b.append(", ");
b.append("deviceComplianceRequired=");
b.append(this.deviceComplianceRequired);
b.append(", ");
b.append("dialerRestrictionLevel=");
b.append(this.dialerRestrictionLevel);
b.append(", ");
b.append("disableAppPinIfDevicePinIsSet=");
b.append(this.disableAppPinIfDevicePinIsSet);
b.append(", ");
b.append("fingerprintBlocked=");
b.append(this.fingerprintBlocked);
b.append(", ");
b.append("managedBrowser=");
b.append(this.managedBrowser);
b.append(", ");
b.append("managedBrowserToOpenLinksRequired=");
b.append(this.managedBrowserToOpenLinksRequired);
b.append(", ");
b.append("maximumAllowedDeviceThreatLevel=");
b.append(this.maximumAllowedDeviceThreatLevel);
b.append(", ");
b.append("maximumPinRetries=");
b.append(this.maximumPinRetries);
b.append(", ");
b.append("maximumRequiredOsVersion=");
b.append(this.maximumRequiredOsVersion);
b.append(", ");
b.append("maximumWarningOsVersion=");
b.append(this.maximumWarningOsVersion);
b.append(", ");
b.append("maximumWipeOsVersion=");
b.append(this.maximumWipeOsVersion);
b.append(", ");
b.append("minimumPinLength=");
b.append(this.minimumPinLength);
b.append(", ");
b.append("minimumRequiredAppVersion=");
b.append(this.minimumRequiredAppVersion);
b.append(", ");
b.append("minimumRequiredOsVersion=");
b.append(this.minimumRequiredOsVersion);
b.append(", ");
b.append("minimumWarningAppVersion=");
b.append(this.minimumWarningAppVersion);
b.append(", ");
b.append("minimumWarningOsVersion=");
b.append(this.minimumWarningOsVersion);
b.append(", ");
b.append("minimumWipeAppVersion=");
b.append(this.minimumWipeAppVersion);
b.append(", ");
b.append("minimumWipeOsVersion=");
b.append(this.minimumWipeOsVersion);
b.append(", ");
b.append("mobileThreatDefenseRemediationAction=");
b.append(this.mobileThreatDefenseRemediationAction);
b.append(", ");
b.append("notificationRestriction=");
b.append(this.notificationRestriction);
b.append(", ");
b.append("organizationalCredentialsRequired=");
b.append(this.organizationalCredentialsRequired);
b.append(", ");
b.append("periodBeforePinReset=");
b.append(this.periodBeforePinReset);
b.append(", ");
b.append("periodOfflineBeforeAccessCheck=");
b.append(this.periodOfflineBeforeAccessCheck);
b.append(", ");
b.append("periodOfflineBeforeWipeIsEnforced=");
b.append(this.periodOfflineBeforeWipeIsEnforced);
b.append(", ");
b.append("periodOnlineBeforeAccessCheck=");
b.append(this.periodOnlineBeforeAccessCheck);
b.append(", ");
b.append("pinCharacterSet=");
b.append(this.pinCharacterSet);
b.append(", ");
b.append("pinRequired=");
b.append(this.pinRequired);
b.append(", ");
b.append("pinRequiredInsteadOfBiometricTimeout=");
b.append(this.pinRequiredInsteadOfBiometricTimeout);
b.append(", ");
b.append("previousPinBlockCount=");
b.append(this.previousPinBlockCount);
b.append(", ");
b.append("printBlocked=");
b.append(this.printBlocked);
b.append(", ");
b.append("saveAsBlocked=");
b.append(this.saveAsBlocked);
b.append(", ");
b.append("simplePinBlocked=");
b.append(this.simplePinBlocked);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}