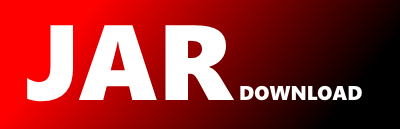
odata.msgraph.client.beta.entity.ManagedDeviceMobileAppConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import odata.msgraph.client.beta.entity.collection.request.ManagedDeviceMobileAppConfigurationAssignmentCollectionRequest;
import odata.msgraph.client.beta.entity.collection.request.ManagedDeviceMobileAppConfigurationDeviceStatusCollectionRequest;
import odata.msgraph.client.beta.entity.collection.request.ManagedDeviceMobileAppConfigurationUserStatusCollectionRequest;
import odata.msgraph.client.beta.entity.request.ManagedDeviceMobileAppConfigurationDeviceSummaryRequest;
import odata.msgraph.client.beta.entity.request.ManagedDeviceMobileAppConfigurationUserSummaryRequest;
/**
* “An abstract class for Mobile app configuration for enrolled devices.”
*/@JsonPropertyOrder({
"@odata.type",
"createdDateTime",
"description",
"displayName",
"lastModifiedDateTime",
"roleScopeTagIds",
"targetedMobileApps",
"version"})
@JsonInclude(Include.NON_NULL)
public class ManagedDeviceMobileAppConfiguration extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.managedDeviceMobileAppConfiguration";
}
@JsonProperty("createdDateTime")
protected OffsetDateTime createdDateTime;
@JsonProperty("description")
protected String description;
@JsonProperty("displayName")
protected String displayName;
@JsonProperty("lastModifiedDateTime")
protected OffsetDateTime lastModifiedDateTime;
@JsonProperty("roleScopeTagIds")
protected List roleScopeTagIds;
@JsonProperty("roleScopeTagIds@nextLink")
protected String roleScopeTagIdsNextLink;
@JsonProperty("targetedMobileApps")
protected List targetedMobileApps;
@JsonProperty("targetedMobileApps@nextLink")
protected String targetedMobileAppsNextLink;
@JsonProperty("version")
protected Integer version;
protected ManagedDeviceMobileAppConfiguration() {
super();
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “DateTime the object was created.”
*
* @return property createdDateTime
*/
@Property(name="createdDateTime")
@JsonIgnore
public Optional getCreatedDateTime() {
return Optional.ofNullable(createdDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code createdDateTime}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “DateTime the object was created.”
*
* @param createdDateTime
* new value of {@code createdDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code createdDateTime} field changed
*/
public ManagedDeviceMobileAppConfiguration withCreatedDateTime(OffsetDateTime createdDateTime) {
ManagedDeviceMobileAppConfiguration _x = _copy();
_x.changedFields = changedFields.add("createdDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfiguration");
_x.createdDateTime = createdDateTime;
return _x;
}
/**
* “Admin provided description of the Device Configuration.”
*
* @return property description
*/
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
/**
* Returns an immutable copy of {@code this} with just the {@code description}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Admin provided description of the Device Configuration.”
*
* @param description
* new value of {@code description} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code description} field changed
*/
public ManagedDeviceMobileAppConfiguration withDescription(String description) {
ManagedDeviceMobileAppConfiguration _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfiguration");
_x.description = description;
return _x;
}
/**
* “Admin provided name of the device configuration.”
*
* @return property displayName
*/
@Property(name="displayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code displayName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Admin provided name of the device configuration.”
*
* @param displayName
* new value of {@code displayName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code displayName} field changed
*/
public ManagedDeviceMobileAppConfiguration withDisplayName(String displayName) {
ManagedDeviceMobileAppConfiguration _x = _copy();
_x.changedFields = changedFields.add("displayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfiguration");
_x.displayName = displayName;
return _x;
}
/**
* “DateTime the object was last modified.”
*
* @return property lastModifiedDateTime
*/
@Property(name="lastModifiedDateTime")
@JsonIgnore
public Optional getLastModifiedDateTime() {
return Optional.ofNullable(lastModifiedDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* lastModifiedDateTime} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “DateTime the object was last modified.”
*
* @param lastModifiedDateTime
* new value of {@code lastModifiedDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code lastModifiedDateTime} field changed
*/
public ManagedDeviceMobileAppConfiguration withLastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
ManagedDeviceMobileAppConfiguration _x = _copy();
_x.changedFields = changedFields.add("lastModifiedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfiguration");
_x.lastModifiedDateTime = lastModifiedDateTime;
return _x;
}
/**
* “List of Scope Tags for this App configuration entity.”
*
* @return property roleScopeTagIds
*/
@Property(name="roleScopeTagIds")
@JsonIgnore
public CollectionPage getRoleScopeTagIds() {
return new CollectionPage(contextPath, String.class, this.roleScopeTagIds, Optional.ofNullable(roleScopeTagIdsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code roleScopeTagIds}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “List of Scope Tags for this App configuration entity.”
*
* @param roleScopeTagIds
* new value of {@code roleScopeTagIds} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code roleScopeTagIds} field changed
*/
public ManagedDeviceMobileAppConfiguration withRoleScopeTagIds(List roleScopeTagIds) {
ManagedDeviceMobileAppConfiguration _x = _copy();
_x.changedFields = changedFields.add("roleScopeTagIds");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfiguration");
_x.roleScopeTagIds = roleScopeTagIds;
return _x;
}
/**
* “List of Scope Tags for this App configuration entity.”
*
* @param options
* specify connect and read timeouts
* @return property roleScopeTagIds
*/
@Property(name="roleScopeTagIds")
@JsonIgnore
public CollectionPage getRoleScopeTagIds(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.roleScopeTagIds, Optional.ofNullable(roleScopeTagIdsNextLink), Collections.emptyList(), options);
}
/**
* “the associated app.”
*
* @return property targetedMobileApps
*/
@Property(name="targetedMobileApps")
@JsonIgnore
public CollectionPage getTargetedMobileApps() {
return new CollectionPage(contextPath, String.class, this.targetedMobileApps, Optional.ofNullable(targetedMobileAppsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* targetedMobileApps} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “the associated app.”
*
* @param targetedMobileApps
* new value of {@code targetedMobileApps} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code targetedMobileApps} field changed
*/
public ManagedDeviceMobileAppConfiguration withTargetedMobileApps(List targetedMobileApps) {
ManagedDeviceMobileAppConfiguration _x = _copy();
_x.changedFields = changedFields.add("targetedMobileApps");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfiguration");
_x.targetedMobileApps = targetedMobileApps;
return _x;
}
/**
* “the associated app.”
*
* @param options
* specify connect and read timeouts
* @return property targetedMobileApps
*/
@Property(name="targetedMobileApps")
@JsonIgnore
public CollectionPage getTargetedMobileApps(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.targetedMobileApps, Optional.ofNullable(targetedMobileAppsNextLink), Collections.emptyList(), options);
}
/**
* “Version of the device configuration.”
*
* @return property version
*/
@Property(name="version")
@JsonIgnore
public Optional getVersion() {
return Optional.ofNullable(version);
}
/**
* Returns an immutable copy of {@code this} with just the {@code version} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Version of the device configuration.”
*
* @param version
* new value of {@code version} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code version} field changed
*/
public ManagedDeviceMobileAppConfiguration withVersion(Integer version) {
ManagedDeviceMobileAppConfiguration _x = _copy();
_x.changedFields = changedFields.add("version");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedDeviceMobileAppConfiguration");
_x.version = version;
return _x;
}
public ManagedDeviceMobileAppConfiguration withUnmappedField(String name, String value) {
ManagedDeviceMobileAppConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “The list of group assignemenets for app configration.”
*
* @return navigational property assignments
*/
@NavigationProperty(name="assignments")
@JsonIgnore
public ManagedDeviceMobileAppConfigurationAssignmentCollectionRequest getAssignments() {
return new ManagedDeviceMobileAppConfigurationAssignmentCollectionRequest(
contextPath.addSegment("assignments"), RequestHelper.getValue(unmappedFields, "assignments"));
}
/**
* “List of ManagedDeviceMobileAppConfigurationDeviceStatus.”
*
* @return navigational property deviceStatuses
*/
@NavigationProperty(name="deviceStatuses")
@JsonIgnore
public ManagedDeviceMobileAppConfigurationDeviceStatusCollectionRequest getDeviceStatuses() {
return new ManagedDeviceMobileAppConfigurationDeviceStatusCollectionRequest(
contextPath.addSegment("deviceStatuses"), RequestHelper.getValue(unmappedFields, "deviceStatuses"));
}
/**
* “App configuration device status summary.”
*
* @return navigational property deviceStatusSummary
*/
@NavigationProperty(name="deviceStatusSummary")
@JsonIgnore
public ManagedDeviceMobileAppConfigurationDeviceSummaryRequest getDeviceStatusSummary() {
return new ManagedDeviceMobileAppConfigurationDeviceSummaryRequest(contextPath.addSegment("deviceStatusSummary"), RequestHelper.getValue(unmappedFields, "deviceStatusSummary"));
}
/**
* “List of ManagedDeviceMobileAppConfigurationUserStatus.”
*
* @return navigational property userStatuses
*/
@NavigationProperty(name="userStatuses")
@JsonIgnore
public ManagedDeviceMobileAppConfigurationUserStatusCollectionRequest getUserStatuses() {
return new ManagedDeviceMobileAppConfigurationUserStatusCollectionRequest(
contextPath.addSegment("userStatuses"), RequestHelper.getValue(unmappedFields, "userStatuses"));
}
/**
* “App configuration user status summary.”
*
* @return navigational property userStatusSummary
*/
@NavigationProperty(name="userStatusSummary")
@JsonIgnore
public ManagedDeviceMobileAppConfigurationUserSummaryRequest getUserStatusSummary() {
return new ManagedDeviceMobileAppConfigurationUserSummaryRequest(contextPath.addSegment("userStatusSummary"), RequestHelper.getValue(unmappedFields, "userStatusSummary"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ManagedDeviceMobileAppConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
ManagedDeviceMobileAppConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ManagedDeviceMobileAppConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
ManagedDeviceMobileAppConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private ManagedDeviceMobileAppConfiguration _copy() {
ManagedDeviceMobileAppConfiguration _x = new ManagedDeviceMobileAppConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.targetedMobileApps = targetedMobileApps;
_x.version = version;
return _x;
}
@Action(name = "assign")
@JsonIgnore
public ActionRequestNoReturn assign(List assignments) {
Map _parameters = ParameterMap
.put("assignments", "Collection(microsoft.graph.managedDeviceMobileAppConfigurationAssignment)", assignments)
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("microsoft.graph.assign"), _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ManagedDeviceMobileAppConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("targetedMobileApps=");
b.append(this.targetedMobileApps);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}