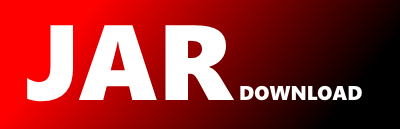
odata.msgraph.client.beta.entity.Post Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.guavamini.Preconditions;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import odata.msgraph.client.beta.complex.ItemBody;
import odata.msgraph.client.beta.complex.Recipient;
import odata.msgraph.client.beta.entity.collection.request.AttachmentCollectionRequest;
import odata.msgraph.client.beta.entity.collection.request.ExtensionCollectionRequest;
import odata.msgraph.client.beta.entity.collection.request.MentionCollectionRequest;
import odata.msgraph.client.beta.entity.collection.request.MultiValueLegacyExtendedPropertyCollectionRequest;
import odata.msgraph.client.beta.entity.collection.request.SingleValueLegacyExtendedPropertyCollectionRequest;
import odata.msgraph.client.beta.entity.request.PostRequest;
import odata.msgraph.client.beta.enums.Importance;
@JsonPropertyOrder({
"@odata.type",
"body",
"conversationId",
"conversationThreadId",
"from",
"hasAttachments",
"importance",
"newParticipants",
"receivedDateTime",
"sender"})
@JsonInclude(Include.NON_NULL)
public class Post extends OutlookItem implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.post";
}
@JsonProperty("body")
protected ItemBody body;
@JsonProperty("conversationId")
protected String conversationId;
@JsonProperty("conversationThreadId")
protected String conversationThreadId;
@JsonProperty("from")
protected Recipient from;
@JsonProperty("hasAttachments")
protected Boolean hasAttachments;
@JsonProperty("importance")
protected Importance importance;
@JsonProperty("newParticipants")
protected List newParticipants;
@JsonProperty("newParticipants@nextLink")
protected String newParticipantsNextLink;
@JsonProperty("receivedDateTime")
protected OffsetDateTime receivedDateTime;
@JsonProperty("sender")
protected Recipient sender;
protected Post() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderPost() {
return new Builder();
}
public static final class Builder {
private String id;
private List categories;
private String categoriesNextLink;
private String changeKey;
private OffsetDateTime createdDateTime;
private OffsetDateTime lastModifiedDateTime;
private ItemBody body;
private String conversationId;
private String conversationThreadId;
private Recipient from;
private Boolean hasAttachments;
private Importance importance;
private List newParticipants;
private String newParticipantsNextLink;
private OffsetDateTime receivedDateTime;
private Recipient sender;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder categories(List categories) {
this.categories = categories;
this.changedFields = changedFields.add("categories");
return this;
}
public Builder categories(String... categories) {
return categories(Arrays.asList(categories));
}
public Builder categoriesNextLink(String categoriesNextLink) {
this.categoriesNextLink = categoriesNextLink;
this.changedFields = changedFields.add("categories");
return this;
}
public Builder changeKey(String changeKey) {
this.changeKey = changeKey;
this.changedFields = changedFields.add("changeKey");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder body(ItemBody body) {
this.body = body;
this.changedFields = changedFields.add("body");
return this;
}
public Builder conversationId(String conversationId) {
this.conversationId = conversationId;
this.changedFields = changedFields.add("conversationId");
return this;
}
public Builder conversationThreadId(String conversationThreadId) {
this.conversationThreadId = conversationThreadId;
this.changedFields = changedFields.add("conversationThreadId");
return this;
}
public Builder from(Recipient from) {
this.from = from;
this.changedFields = changedFields.add("from");
return this;
}
public Builder hasAttachments(Boolean hasAttachments) {
this.hasAttachments = hasAttachments;
this.changedFields = changedFields.add("hasAttachments");
return this;
}
public Builder importance(Importance importance) {
this.importance = importance;
this.changedFields = changedFields.add("importance");
return this;
}
public Builder newParticipants(List newParticipants) {
this.newParticipants = newParticipants;
this.changedFields = changedFields.add("newParticipants");
return this;
}
public Builder newParticipants(Recipient... newParticipants) {
return newParticipants(Arrays.asList(newParticipants));
}
public Builder newParticipantsNextLink(String newParticipantsNextLink) {
this.newParticipantsNextLink = newParticipantsNextLink;
this.changedFields = changedFields.add("newParticipants");
return this;
}
public Builder receivedDateTime(OffsetDateTime receivedDateTime) {
this.receivedDateTime = receivedDateTime;
this.changedFields = changedFields.add("receivedDateTime");
return this;
}
public Builder sender(Recipient sender) {
this.sender = sender;
this.changedFields = changedFields.add("sender");
return this;
}
public Post build() {
Post _x = new Post();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.post";
_x.id = id;
_x.categories = categories;
_x.categoriesNextLink = categoriesNextLink;
_x.changeKey = changeKey;
_x.createdDateTime = createdDateTime;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.body = body;
_x.conversationId = conversationId;
_x.conversationThreadId = conversationThreadId;
_x.from = from;
_x.hasAttachments = hasAttachments;
_x.importance = importance;
_x.newParticipants = newParticipants;
_x.newParticipantsNextLink = newParticipantsNextLink;
_x.receivedDateTime = receivedDateTime;
_x.sender = sender;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
@Property(name="body")
@JsonIgnore
public Optional getBody() {
return Optional.ofNullable(body);
}
public Post withBody(ItemBody body) {
Post _x = _copy();
_x.changedFields = changedFields.add("body");
_x.odataType = Util.nvl(odataType, "microsoft.graph.post");
_x.body = body;
return _x;
}
@Property(name="conversationId")
@JsonIgnore
public Optional getConversationId() {
return Optional.ofNullable(conversationId);
}
public Post withConversationId(String conversationId) {
Post _x = _copy();
_x.changedFields = changedFields.add("conversationId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.post");
_x.conversationId = conversationId;
return _x;
}
@Property(name="conversationThreadId")
@JsonIgnore
public Optional getConversationThreadId() {
return Optional.ofNullable(conversationThreadId);
}
public Post withConversationThreadId(String conversationThreadId) {
Post _x = _copy();
_x.changedFields = changedFields.add("conversationThreadId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.post");
_x.conversationThreadId = conversationThreadId;
return _x;
}
@Property(name="from")
@JsonIgnore
public Optional getFrom() {
return Optional.ofNullable(from);
}
public Post withFrom(Recipient from) {
Post _x = _copy();
_x.changedFields = changedFields.add("from");
_x.odataType = Util.nvl(odataType, "microsoft.graph.post");
_x.from = from;
return _x;
}
@Property(name="hasAttachments")
@JsonIgnore
public Optional getHasAttachments() {
return Optional.ofNullable(hasAttachments);
}
public Post withHasAttachments(Boolean hasAttachments) {
Post _x = _copy();
_x.changedFields = changedFields.add("hasAttachments");
_x.odataType = Util.nvl(odataType, "microsoft.graph.post");
_x.hasAttachments = hasAttachments;
return _x;
}
@Property(name="importance")
@JsonIgnore
public Optional getImportance() {
return Optional.ofNullable(importance);
}
public Post withImportance(Importance importance) {
Post _x = _copy();
_x.changedFields = changedFields.add("importance");
_x.odataType = Util.nvl(odataType, "microsoft.graph.post");
_x.importance = importance;
return _x;
}
@Property(name="newParticipants")
@JsonIgnore
public CollectionPage getNewParticipants() {
return new CollectionPage(contextPath, Recipient.class, this.newParticipants, Optional.ofNullable(newParticipantsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public Post withNewParticipants(List newParticipants) {
Post _x = _copy();
_x.changedFields = changedFields.add("newParticipants");
_x.odataType = Util.nvl(odataType, "microsoft.graph.post");
_x.newParticipants = newParticipants;
return _x;
}
@Property(name="newParticipants")
@JsonIgnore
public CollectionPage getNewParticipants(HttpRequestOptions options) {
return new CollectionPage(contextPath, Recipient.class, this.newParticipants, Optional.ofNullable(newParticipantsNextLink), Collections.emptyList(), options);
}
@Property(name="receivedDateTime")
@JsonIgnore
public Optional getReceivedDateTime() {
return Optional.ofNullable(receivedDateTime);
}
public Post withReceivedDateTime(OffsetDateTime receivedDateTime) {
Post _x = _copy();
_x.changedFields = changedFields.add("receivedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.post");
_x.receivedDateTime = receivedDateTime;
return _x;
}
@Property(name="sender")
@JsonIgnore
public Optional getSender() {
return Optional.ofNullable(sender);
}
public Post withSender(Recipient sender) {
Post _x = _copy();
_x.changedFields = changedFields.add("sender");
_x.odataType = Util.nvl(odataType, "microsoft.graph.post");
_x.sender = sender;
return _x;
}
public Post withUnmappedField(String name, String value) {
Post _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
*
* Org.OData.Capabilities.V1.ChangeTracking
*
* Supported = false
*
* Org.OData.Capabilities.V1.SearchRestrictions
*
* Searchable = false
*
* Org.OData.Capabilities.V1.UpdateRestrictions
*
* Updatable = false
*
* @return navigational property attachments
*/
@NavigationProperty(name="attachments")
@JsonIgnore
public AttachmentCollectionRequest getAttachments() {
return new AttachmentCollectionRequest(
contextPath.addSegment("attachments"), RequestHelper.getValue(unmappedFields, "attachments"));
}
/**
*
* Org.OData.Capabilities.V1.ChangeTracking
*
* Supported = false
*
* Org.OData.Capabilities.V1.SearchRestrictions
*
* Searchable = false
*
* @return navigational property extensions
*/
@NavigationProperty(name="extensions")
@JsonIgnore
public ExtensionCollectionRequest getExtensions() {
return new ExtensionCollectionRequest(
contextPath.addSegment("extensions"), RequestHelper.getValue(unmappedFields, "extensions"));
}
/**
*
* Org.OData.Capabilities.V1.ChangeTracking
*
* Supported = false
*
* Org.OData.Capabilities.V1.DeleteRestrictions
*
* Deletable = false
*
* Org.OData.Capabilities.V1.InsertRestrictions
*
* Insertable = false
*
* Org.OData.Capabilities.V1.NavigationRestrictions
*
* Org.OData.Capabilities.V1.SearchRestrictions
*
* Searchable = false
*
* Org.OData.Capabilities.V1.UpdateRestrictions
*
* Updatable = false
*
* @return navigational property inReplyTo
*/
@NavigationProperty(name="inReplyTo")
@JsonIgnore
public PostRequest getInReplyTo() {
return new PostRequest(contextPath.addSegment("inReplyTo"), RequestHelper.getValue(unmappedFields, "inReplyTo"));
}
/**
*
* Org.OData.Capabilities.V1.ChangeTracking
*
* Supported = false
*
* Org.OData.Capabilities.V1.SearchRestrictions
*
* Searchable = false
*
* Org.OData.Capabilities.V1.UpdateRestrictions
*
* Updatable = false
*
* @return navigational property mentions
*/
@NavigationProperty(name="mentions")
@JsonIgnore
public MentionCollectionRequest getMentions() {
return new MentionCollectionRequest(
contextPath.addSegment("mentions"), RequestHelper.getValue(unmappedFields, "mentions"));
}
@NavigationProperty(name="multiValueExtendedProperties")
@JsonIgnore
public MultiValueLegacyExtendedPropertyCollectionRequest getMultiValueExtendedProperties() {
return new MultiValueLegacyExtendedPropertyCollectionRequest(
contextPath.addSegment("multiValueExtendedProperties"), RequestHelper.getValue(unmappedFields, "multiValueExtendedProperties"));
}
@NavigationProperty(name="singleValueExtendedProperties")
@JsonIgnore
public SingleValueLegacyExtendedPropertyCollectionRequest getSingleValueExtendedProperties() {
return new SingleValueLegacyExtendedPropertyCollectionRequest(
contextPath.addSegment("singleValueExtendedProperties"), RequestHelper.getValue(unmappedFields, "singleValueExtendedProperties"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Post patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Post _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Post put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Post _x = _copy();
_x.changedFields = null;
return _x;
}
private Post _copy() {
Post _x = new Post();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.categories = categories;
_x.changeKey = changeKey;
_x.createdDateTime = createdDateTime;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.body = body;
_x.conversationId = conversationId;
_x.conversationThreadId = conversationThreadId;
_x.from = from;
_x.hasAttachments = hasAttachments;
_x.importance = importance;
_x.newParticipants = newParticipants;
_x.receivedDateTime = receivedDateTime;
_x.sender = sender;
return _x;
}
@Action(name = "forward")
@JsonIgnore
public ActionRequestNoReturn forward(String comment, List toRecipients) {
Preconditions.checkNotNull(toRecipients, "toRecipients cannot be null");
Map _parameters = ParameterMap
.put("Comment", "Edm.String", Checks.checkIsAscii(comment))
.put("ToRecipients", "Collection(microsoft.graph.recipient)", toRecipients)
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("microsoft.graph.forward"), _parameters);
}
@Action(name = "reply")
@JsonIgnore
public ActionRequestNoReturn reply(Post post) {
Preconditions.checkNotNull(post, "post cannot be null");
Map _parameters = ParameterMap
.put("Post", "microsoft.graph.post", post)
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("microsoft.graph.reply"), _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Post[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("categories=");
b.append(this.categories);
b.append(", ");
b.append("changeKey=");
b.append(this.changeKey);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("body=");
b.append(this.body);
b.append(", ");
b.append("conversationId=");
b.append(this.conversationId);
b.append(", ");
b.append("conversationThreadId=");
b.append(this.conversationThreadId);
b.append(", ");
b.append("from=");
b.append(this.from);
b.append(", ");
b.append("hasAttachments=");
b.append(this.hasAttachments);
b.append(", ");
b.append("importance=");
b.append(this.importance);
b.append(", ");
b.append("newParticipants=");
b.append(this.newParticipants);
b.append(", ");
b.append("receivedDateTime=");
b.append(this.receivedDateTime);
b.append(", ");
b.append("sender=");
b.append(this.sender);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}