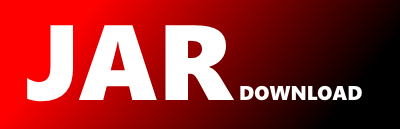
odata.msgraph.client.beta.entity.PrivilegedOperationEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph-beta Show documentation
Show all versions of odata-client-msgraph-beta Show documentation
Java client for use with Microsoft Graph beta endpoint
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Optional;
@JsonPropertyOrder({
"@odata.type",
"additionalInformation",
"creationDateTime",
"expirationDateTime",
"referenceKey",
"referenceSystem",
"requestorId",
"requestorName",
"requestType",
"roleId",
"roleName",
"tenantId",
"userId",
"userMail",
"userName"})
@JsonInclude(Include.NON_NULL)
public class PrivilegedOperationEvent extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.privilegedOperationEvent";
}
@JsonProperty("additionalInformation")
protected String additionalInformation;
@JsonProperty("creationDateTime")
protected OffsetDateTime creationDateTime;
@JsonProperty("expirationDateTime")
protected OffsetDateTime expirationDateTime;
@JsonProperty("referenceKey")
protected String referenceKey;
@JsonProperty("referenceSystem")
protected String referenceSystem;
@JsonProperty("requestorId")
protected String requestorId;
@JsonProperty("requestorName")
protected String requestorName;
@JsonProperty("requestType")
protected String requestType;
@JsonProperty("roleId")
protected String roleId;
@JsonProperty("roleName")
protected String roleName;
@JsonProperty("tenantId")
protected String tenantId;
@JsonProperty("userId")
protected String userId;
@JsonProperty("userMail")
protected String userMail;
@JsonProperty("userName")
protected String userName;
protected PrivilegedOperationEvent() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderPrivilegedOperationEvent() {
return new Builder();
}
public static final class Builder {
private String id;
private String additionalInformation;
private OffsetDateTime creationDateTime;
private OffsetDateTime expirationDateTime;
private String referenceKey;
private String referenceSystem;
private String requestorId;
private String requestorName;
private String requestType;
private String roleId;
private String roleName;
private String tenantId;
private String userId;
private String userMail;
private String userName;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder additionalInformation(String additionalInformation) {
this.additionalInformation = additionalInformation;
this.changedFields = changedFields.add("additionalInformation");
return this;
}
public Builder creationDateTime(OffsetDateTime creationDateTime) {
this.creationDateTime = creationDateTime;
this.changedFields = changedFields.add("creationDateTime");
return this;
}
public Builder expirationDateTime(OffsetDateTime expirationDateTime) {
this.expirationDateTime = expirationDateTime;
this.changedFields = changedFields.add("expirationDateTime");
return this;
}
public Builder referenceKey(String referenceKey) {
this.referenceKey = referenceKey;
this.changedFields = changedFields.add("referenceKey");
return this;
}
public Builder referenceSystem(String referenceSystem) {
this.referenceSystem = referenceSystem;
this.changedFields = changedFields.add("referenceSystem");
return this;
}
public Builder requestorId(String requestorId) {
this.requestorId = requestorId;
this.changedFields = changedFields.add("requestorId");
return this;
}
public Builder requestorName(String requestorName) {
this.requestorName = requestorName;
this.changedFields = changedFields.add("requestorName");
return this;
}
public Builder requestType(String requestType) {
this.requestType = requestType;
this.changedFields = changedFields.add("requestType");
return this;
}
public Builder roleId(String roleId) {
this.roleId = roleId;
this.changedFields = changedFields.add("roleId");
return this;
}
public Builder roleName(String roleName) {
this.roleName = roleName;
this.changedFields = changedFields.add("roleName");
return this;
}
public Builder tenantId(String tenantId) {
this.tenantId = tenantId;
this.changedFields = changedFields.add("tenantId");
return this;
}
public Builder userId(String userId) {
this.userId = userId;
this.changedFields = changedFields.add("userId");
return this;
}
public Builder userMail(String userMail) {
this.userMail = userMail;
this.changedFields = changedFields.add("userMail");
return this;
}
public Builder userName(String userName) {
this.userName = userName;
this.changedFields = changedFields.add("userName");
return this;
}
public PrivilegedOperationEvent build() {
PrivilegedOperationEvent _x = new PrivilegedOperationEvent();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.privilegedOperationEvent";
_x.id = id;
_x.additionalInformation = additionalInformation;
_x.creationDateTime = creationDateTime;
_x.expirationDateTime = expirationDateTime;
_x.referenceKey = referenceKey;
_x.referenceSystem = referenceSystem;
_x.requestorId = requestorId;
_x.requestorName = requestorName;
_x.requestType = requestType;
_x.roleId = roleId;
_x.roleName = roleName;
_x.tenantId = tenantId;
_x.userId = userId;
_x.userMail = userMail;
_x.userName = userName;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
@Property(name="additionalInformation")
@JsonIgnore
public Optional getAdditionalInformation() {
return Optional.ofNullable(additionalInformation);
}
public PrivilegedOperationEvent withAdditionalInformation(String additionalInformation) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("additionalInformation");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.additionalInformation = additionalInformation;
return _x;
}
@Property(name="creationDateTime")
@JsonIgnore
public Optional getCreationDateTime() {
return Optional.ofNullable(creationDateTime);
}
public PrivilegedOperationEvent withCreationDateTime(OffsetDateTime creationDateTime) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("creationDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.creationDateTime = creationDateTime;
return _x;
}
@Property(name="expirationDateTime")
@JsonIgnore
public Optional getExpirationDateTime() {
return Optional.ofNullable(expirationDateTime);
}
public PrivilegedOperationEvent withExpirationDateTime(OffsetDateTime expirationDateTime) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("expirationDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.expirationDateTime = expirationDateTime;
return _x;
}
@Property(name="referenceKey")
@JsonIgnore
public Optional getReferenceKey() {
return Optional.ofNullable(referenceKey);
}
public PrivilegedOperationEvent withReferenceKey(String referenceKey) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("referenceKey");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.referenceKey = referenceKey;
return _x;
}
@Property(name="referenceSystem")
@JsonIgnore
public Optional getReferenceSystem() {
return Optional.ofNullable(referenceSystem);
}
public PrivilegedOperationEvent withReferenceSystem(String referenceSystem) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("referenceSystem");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.referenceSystem = referenceSystem;
return _x;
}
@Property(name="requestorId")
@JsonIgnore
public Optional getRequestorId() {
return Optional.ofNullable(requestorId);
}
public PrivilegedOperationEvent withRequestorId(String requestorId) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("requestorId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.requestorId = requestorId;
return _x;
}
@Property(name="requestorName")
@JsonIgnore
public Optional getRequestorName() {
return Optional.ofNullable(requestorName);
}
public PrivilegedOperationEvent withRequestorName(String requestorName) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("requestorName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.requestorName = requestorName;
return _x;
}
@Property(name="requestType")
@JsonIgnore
public Optional getRequestType() {
return Optional.ofNullable(requestType);
}
public PrivilegedOperationEvent withRequestType(String requestType) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("requestType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.requestType = requestType;
return _x;
}
@Property(name="roleId")
@JsonIgnore
public Optional getRoleId() {
return Optional.ofNullable(roleId);
}
public PrivilegedOperationEvent withRoleId(String roleId) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("roleId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.roleId = roleId;
return _x;
}
@Property(name="roleName")
@JsonIgnore
public Optional getRoleName() {
return Optional.ofNullable(roleName);
}
public PrivilegedOperationEvent withRoleName(String roleName) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("roleName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.roleName = roleName;
return _x;
}
@Property(name="tenantId")
@JsonIgnore
public Optional getTenantId() {
return Optional.ofNullable(tenantId);
}
public PrivilegedOperationEvent withTenantId(String tenantId) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("tenantId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.tenantId = tenantId;
return _x;
}
@Property(name="userId")
@JsonIgnore
public Optional getUserId() {
return Optional.ofNullable(userId);
}
public PrivilegedOperationEvent withUserId(String userId) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("userId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.userId = userId;
return _x;
}
@Property(name="userMail")
@JsonIgnore
public Optional getUserMail() {
return Optional.ofNullable(userMail);
}
public PrivilegedOperationEvent withUserMail(String userMail) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("userMail");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.userMail = userMail;
return _x;
}
@Property(name="userName")
@JsonIgnore
public Optional getUserName() {
return Optional.ofNullable(userName);
}
public PrivilegedOperationEvent withUserName(String userName) {
PrivilegedOperationEvent _x = _copy();
_x.changedFields = changedFields.add("userName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.privilegedOperationEvent");
_x.userName = userName;
return _x;
}
public PrivilegedOperationEvent withUnmappedField(String name, String value) {
PrivilegedOperationEvent _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public PrivilegedOperationEvent patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
PrivilegedOperationEvent _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public PrivilegedOperationEvent put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
PrivilegedOperationEvent _x = _copy();
_x.changedFields = null;
return _x;
}
private PrivilegedOperationEvent _copy() {
PrivilegedOperationEvent _x = new PrivilegedOperationEvent();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.additionalInformation = additionalInformation;
_x.creationDateTime = creationDateTime;
_x.expirationDateTime = expirationDateTime;
_x.referenceKey = referenceKey;
_x.referenceSystem = referenceSystem;
_x.requestorId = requestorId;
_x.requestorName = requestorName;
_x.requestType = requestType;
_x.roleId = roleId;
_x.roleName = roleName;
_x.tenantId = tenantId;
_x.userId = userId;
_x.userMail = userMail;
_x.userName = userName;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("PrivilegedOperationEvent[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("additionalInformation=");
b.append(this.additionalInformation);
b.append(", ");
b.append("creationDateTime=");
b.append(this.creationDateTime);
b.append(", ");
b.append("expirationDateTime=");
b.append(this.expirationDateTime);
b.append(", ");
b.append("referenceKey=");
b.append(this.referenceKey);
b.append(", ");
b.append("referenceSystem=");
b.append(this.referenceSystem);
b.append(", ");
b.append("requestorId=");
b.append(this.requestorId);
b.append(", ");
b.append("requestorName=");
b.append(this.requestorName);
b.append(", ");
b.append("requestType=");
b.append(this.requestType);
b.append(", ");
b.append("roleId=");
b.append(this.roleId);
b.append(", ");
b.append("roleName=");
b.append(this.roleName);
b.append(", ");
b.append("tenantId=");
b.append(this.tenantId);
b.append(", ");
b.append("userId=");
b.append(this.userId);
b.append(", ");
b.append("userMail=");
b.append(this.userMail);
b.append(", ");
b.append("userName=");
b.append(this.userName);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy