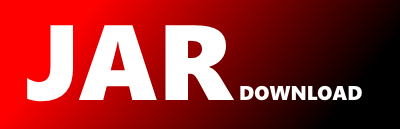
odata.msgraph.client.beta.entity.SalesCreditMemo Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.math.BigDecimal;
import java.time.LocalDate;
import java.time.OffsetDateTime;
import java.util.Optional;
import odata.msgraph.client.beta.complex.PostalAddressType;
import odata.msgraph.client.beta.entity.collection.request.SalesCreditMemoLineCollectionRequest;
import odata.msgraph.client.beta.entity.request.CurrencyRequest;
import odata.msgraph.client.beta.entity.request.CustomerRequest;
import odata.msgraph.client.beta.entity.request.PaymentTermRequest;
/**
*
* Org.OData.Capabilities.V1.DeleteRestrictions
*
* Deletable = false
*
* Org.OData.Capabilities.V1.InsertRestrictions
*
* Insertable = false
*
* Org.OData.Capabilities.V1.UpdateRestrictions
*
* Updatable = true
*/@JsonPropertyOrder({
"@odata.type",
"billingPostalAddress",
"billToCustomerId",
"billToCustomerNumber",
"billToName",
"creditMemoDate",
"currencyCode",
"currencyId",
"customerId",
"customerName",
"customerNumber",
"discountAmount",
"discountAppliedBeforeTax",
"dueDate",
"email",
"externalDocumentNumber",
"invoiceId",
"invoiceNumber",
"lastModifiedDateTime",
"number",
"paymentTermsId",
"phoneNumber",
"pricesIncludeTax",
"salesperson",
"sellingPostalAddress",
"status",
"totalAmountExcludingTax",
"totalAmountIncludingTax",
"totalTaxAmount"})
@JsonInclude(Include.NON_NULL)
public class SalesCreditMemo extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.salesCreditMemo";
}
@JsonProperty("billingPostalAddress")
protected PostalAddressType billingPostalAddress;
@JsonProperty("billToCustomerId")
protected String billToCustomerId;
@JsonProperty("billToCustomerNumber")
protected String billToCustomerNumber;
@JsonProperty("billToName")
protected String billToName;
@JsonProperty("creditMemoDate")
protected LocalDate creditMemoDate;
@JsonProperty("currencyCode")
protected String currencyCode;
@JsonProperty("currencyId")
protected String currencyId;
@JsonProperty("customerId")
protected String customerId;
@JsonProperty("customerName")
protected String customerName;
@JsonProperty("customerNumber")
protected String customerNumber;
@JsonProperty("discountAmount")
protected BigDecimal discountAmount;
@JsonProperty("discountAppliedBeforeTax")
protected Boolean discountAppliedBeforeTax;
@JsonProperty("dueDate")
protected LocalDate dueDate;
@JsonProperty("email")
protected String email;
@JsonProperty("externalDocumentNumber")
protected String externalDocumentNumber;
@JsonProperty("invoiceId")
protected String invoiceId;
@JsonProperty("invoiceNumber")
protected String invoiceNumber;
@JsonProperty("lastModifiedDateTime")
protected OffsetDateTime lastModifiedDateTime;
@JsonProperty("number")
protected String number;
@JsonProperty("paymentTermsId")
protected String paymentTermsId;
@JsonProperty("phoneNumber")
protected String phoneNumber;
@JsonProperty("pricesIncludeTax")
protected Boolean pricesIncludeTax;
@JsonProperty("salesperson")
protected String salesperson;
@JsonProperty("sellingPostalAddress")
protected PostalAddressType sellingPostalAddress;
@JsonProperty("status")
protected String status;
@JsonProperty("totalAmountExcludingTax")
protected BigDecimal totalAmountExcludingTax;
@JsonProperty("totalAmountIncludingTax")
protected BigDecimal totalAmountIncludingTax;
@JsonProperty("totalTaxAmount")
protected BigDecimal totalTaxAmount;
protected SalesCreditMemo() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderSalesCreditMemo() {
return new Builder();
}
public static final class Builder {
private String id;
private PostalAddressType billingPostalAddress;
private String billToCustomerId;
private String billToCustomerNumber;
private String billToName;
private LocalDate creditMemoDate;
private String currencyCode;
private String currencyId;
private String customerId;
private String customerName;
private String customerNumber;
private BigDecimal discountAmount;
private Boolean discountAppliedBeforeTax;
private LocalDate dueDate;
private String email;
private String externalDocumentNumber;
private String invoiceId;
private String invoiceNumber;
private OffsetDateTime lastModifiedDateTime;
private String number;
private String paymentTermsId;
private String phoneNumber;
private Boolean pricesIncludeTax;
private String salesperson;
private PostalAddressType sellingPostalAddress;
private String status;
private BigDecimal totalAmountExcludingTax;
private BigDecimal totalAmountIncludingTax;
private BigDecimal totalTaxAmount;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder billingPostalAddress(PostalAddressType billingPostalAddress) {
this.billingPostalAddress = billingPostalAddress;
this.changedFields = changedFields.add("billingPostalAddress");
return this;
}
public Builder billToCustomerId(String billToCustomerId) {
this.billToCustomerId = billToCustomerId;
this.changedFields = changedFields.add("billToCustomerId");
return this;
}
public Builder billToCustomerNumber(String billToCustomerNumber) {
this.billToCustomerNumber = billToCustomerNumber;
this.changedFields = changedFields.add("billToCustomerNumber");
return this;
}
public Builder billToName(String billToName) {
this.billToName = billToName;
this.changedFields = changedFields.add("billToName");
return this;
}
public Builder creditMemoDate(LocalDate creditMemoDate) {
this.creditMemoDate = creditMemoDate;
this.changedFields = changedFields.add("creditMemoDate");
return this;
}
public Builder currencyCode(String currencyCode) {
this.currencyCode = currencyCode;
this.changedFields = changedFields.add("currencyCode");
return this;
}
public Builder currencyId(String currencyId) {
this.currencyId = currencyId;
this.changedFields = changedFields.add("currencyId");
return this;
}
public Builder customerId(String customerId) {
this.customerId = customerId;
this.changedFields = changedFields.add("customerId");
return this;
}
public Builder customerName(String customerName) {
this.customerName = customerName;
this.changedFields = changedFields.add("customerName");
return this;
}
public Builder customerNumber(String customerNumber) {
this.customerNumber = customerNumber;
this.changedFields = changedFields.add("customerNumber");
return this;
}
public Builder discountAmount(BigDecimal discountAmount) {
this.discountAmount = discountAmount;
this.changedFields = changedFields.add("discountAmount");
return this;
}
public Builder discountAppliedBeforeTax(Boolean discountAppliedBeforeTax) {
this.discountAppliedBeforeTax = discountAppliedBeforeTax;
this.changedFields = changedFields.add("discountAppliedBeforeTax");
return this;
}
public Builder dueDate(LocalDate dueDate) {
this.dueDate = dueDate;
this.changedFields = changedFields.add("dueDate");
return this;
}
public Builder email(String email) {
this.email = email;
this.changedFields = changedFields.add("email");
return this;
}
public Builder externalDocumentNumber(String externalDocumentNumber) {
this.externalDocumentNumber = externalDocumentNumber;
this.changedFields = changedFields.add("externalDocumentNumber");
return this;
}
public Builder invoiceId(String invoiceId) {
this.invoiceId = invoiceId;
this.changedFields = changedFields.add("invoiceId");
return this;
}
public Builder invoiceNumber(String invoiceNumber) {
this.invoiceNumber = invoiceNumber;
this.changedFields = changedFields.add("invoiceNumber");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder number(String number) {
this.number = number;
this.changedFields = changedFields.add("number");
return this;
}
public Builder paymentTermsId(String paymentTermsId) {
this.paymentTermsId = paymentTermsId;
this.changedFields = changedFields.add("paymentTermsId");
return this;
}
public Builder phoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
this.changedFields = changedFields.add("phoneNumber");
return this;
}
public Builder pricesIncludeTax(Boolean pricesIncludeTax) {
this.pricesIncludeTax = pricesIncludeTax;
this.changedFields = changedFields.add("pricesIncludeTax");
return this;
}
public Builder salesperson(String salesperson) {
this.salesperson = salesperson;
this.changedFields = changedFields.add("salesperson");
return this;
}
public Builder sellingPostalAddress(PostalAddressType sellingPostalAddress) {
this.sellingPostalAddress = sellingPostalAddress;
this.changedFields = changedFields.add("sellingPostalAddress");
return this;
}
public Builder status(String status) {
this.status = status;
this.changedFields = changedFields.add("status");
return this;
}
public Builder totalAmountExcludingTax(BigDecimal totalAmountExcludingTax) {
this.totalAmountExcludingTax = totalAmountExcludingTax;
this.changedFields = changedFields.add("totalAmountExcludingTax");
return this;
}
public Builder totalAmountIncludingTax(BigDecimal totalAmountIncludingTax) {
this.totalAmountIncludingTax = totalAmountIncludingTax;
this.changedFields = changedFields.add("totalAmountIncludingTax");
return this;
}
public Builder totalTaxAmount(BigDecimal totalTaxAmount) {
this.totalTaxAmount = totalTaxAmount;
this.changedFields = changedFields.add("totalTaxAmount");
return this;
}
public SalesCreditMemo build() {
SalesCreditMemo _x = new SalesCreditMemo();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.salesCreditMemo";
_x.id = id;
_x.billingPostalAddress = billingPostalAddress;
_x.billToCustomerId = billToCustomerId;
_x.billToCustomerNumber = billToCustomerNumber;
_x.billToName = billToName;
_x.creditMemoDate = creditMemoDate;
_x.currencyCode = currencyCode;
_x.currencyId = currencyId;
_x.customerId = customerId;
_x.customerName = customerName;
_x.customerNumber = customerNumber;
_x.discountAmount = discountAmount;
_x.discountAppliedBeforeTax = discountAppliedBeforeTax;
_x.dueDate = dueDate;
_x.email = email;
_x.externalDocumentNumber = externalDocumentNumber;
_x.invoiceId = invoiceId;
_x.invoiceNumber = invoiceNumber;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.number = number;
_x.paymentTermsId = paymentTermsId;
_x.phoneNumber = phoneNumber;
_x.pricesIncludeTax = pricesIncludeTax;
_x.salesperson = salesperson;
_x.sellingPostalAddress = sellingPostalAddress;
_x.status = status;
_x.totalAmountExcludingTax = totalAmountExcludingTax;
_x.totalAmountIncludingTax = totalAmountIncludingTax;
_x.totalTaxAmount = totalTaxAmount;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
@Property(name="billingPostalAddress")
@JsonIgnore
public Optional getBillingPostalAddress() {
return Optional.ofNullable(billingPostalAddress);
}
public SalesCreditMemo withBillingPostalAddress(PostalAddressType billingPostalAddress) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("billingPostalAddress");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.billingPostalAddress = billingPostalAddress;
return _x;
}
@Property(name="billToCustomerId")
@JsonIgnore
public Optional getBillToCustomerId() {
return Optional.ofNullable(billToCustomerId);
}
public SalesCreditMemo withBillToCustomerId(String billToCustomerId) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("billToCustomerId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.billToCustomerId = billToCustomerId;
return _x;
}
@Property(name="billToCustomerNumber")
@JsonIgnore
public Optional getBillToCustomerNumber() {
return Optional.ofNullable(billToCustomerNumber);
}
public SalesCreditMemo withBillToCustomerNumber(String billToCustomerNumber) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("billToCustomerNumber");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.billToCustomerNumber = billToCustomerNumber;
return _x;
}
@Property(name="billToName")
@JsonIgnore
public Optional getBillToName() {
return Optional.ofNullable(billToName);
}
public SalesCreditMemo withBillToName(String billToName) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("billToName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.billToName = billToName;
return _x;
}
@Property(name="creditMemoDate")
@JsonIgnore
public Optional getCreditMemoDate() {
return Optional.ofNullable(creditMemoDate);
}
public SalesCreditMemo withCreditMemoDate(LocalDate creditMemoDate) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("creditMemoDate");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.creditMemoDate = creditMemoDate;
return _x;
}
@Property(name="currencyCode")
@JsonIgnore
public Optional getCurrencyCode() {
return Optional.ofNullable(currencyCode);
}
public SalesCreditMemo withCurrencyCode(String currencyCode) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("currencyCode");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.currencyCode = currencyCode;
return _x;
}
@Property(name="currencyId")
@JsonIgnore
public Optional getCurrencyId() {
return Optional.ofNullable(currencyId);
}
public SalesCreditMemo withCurrencyId(String currencyId) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("currencyId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.currencyId = currencyId;
return _x;
}
@Property(name="customerId")
@JsonIgnore
public Optional getCustomerId() {
return Optional.ofNullable(customerId);
}
public SalesCreditMemo withCustomerId(String customerId) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("customerId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.customerId = customerId;
return _x;
}
@Property(name="customerName")
@JsonIgnore
public Optional getCustomerName() {
return Optional.ofNullable(customerName);
}
public SalesCreditMemo withCustomerName(String customerName) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("customerName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.customerName = customerName;
return _x;
}
@Property(name="customerNumber")
@JsonIgnore
public Optional getCustomerNumber() {
return Optional.ofNullable(customerNumber);
}
public SalesCreditMemo withCustomerNumber(String customerNumber) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("customerNumber");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.customerNumber = customerNumber;
return _x;
}
@Property(name="discountAmount")
@JsonIgnore
public Optional getDiscountAmount() {
return Optional.ofNullable(discountAmount);
}
public SalesCreditMemo withDiscountAmount(BigDecimal discountAmount) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("discountAmount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.discountAmount = discountAmount;
return _x;
}
@Property(name="discountAppliedBeforeTax")
@JsonIgnore
public Optional getDiscountAppliedBeforeTax() {
return Optional.ofNullable(discountAppliedBeforeTax);
}
public SalesCreditMemo withDiscountAppliedBeforeTax(Boolean discountAppliedBeforeTax) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("discountAppliedBeforeTax");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.discountAppliedBeforeTax = discountAppliedBeforeTax;
return _x;
}
@Property(name="dueDate")
@JsonIgnore
public Optional getDueDate() {
return Optional.ofNullable(dueDate);
}
public SalesCreditMemo withDueDate(LocalDate dueDate) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("dueDate");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.dueDate = dueDate;
return _x;
}
@Property(name="email")
@JsonIgnore
public Optional getEmail() {
return Optional.ofNullable(email);
}
public SalesCreditMemo withEmail(String email) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("email");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.email = email;
return _x;
}
@Property(name="externalDocumentNumber")
@JsonIgnore
public Optional getExternalDocumentNumber() {
return Optional.ofNullable(externalDocumentNumber);
}
public SalesCreditMemo withExternalDocumentNumber(String externalDocumentNumber) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("externalDocumentNumber");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.externalDocumentNumber = externalDocumentNumber;
return _x;
}
@Property(name="invoiceId")
@JsonIgnore
public Optional getInvoiceId() {
return Optional.ofNullable(invoiceId);
}
public SalesCreditMemo withInvoiceId(String invoiceId) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("invoiceId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.invoiceId = invoiceId;
return _x;
}
@Property(name="invoiceNumber")
@JsonIgnore
public Optional getInvoiceNumber() {
return Optional.ofNullable(invoiceNumber);
}
public SalesCreditMemo withInvoiceNumber(String invoiceNumber) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("invoiceNumber");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.invoiceNumber = invoiceNumber;
return _x;
}
@Property(name="lastModifiedDateTime")
@JsonIgnore
public Optional getLastModifiedDateTime() {
return Optional.ofNullable(lastModifiedDateTime);
}
public SalesCreditMemo withLastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("lastModifiedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.lastModifiedDateTime = lastModifiedDateTime;
return _x;
}
@Property(name="number")
@JsonIgnore
public Optional getNumber() {
return Optional.ofNullable(number);
}
public SalesCreditMemo withNumber(String number) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("number");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.number = number;
return _x;
}
@Property(name="paymentTermsId")
@JsonIgnore
public Optional getPaymentTermsId() {
return Optional.ofNullable(paymentTermsId);
}
public SalesCreditMemo withPaymentTermsId(String paymentTermsId) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("paymentTermsId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.paymentTermsId = paymentTermsId;
return _x;
}
@Property(name="phoneNumber")
@JsonIgnore
public Optional getPhoneNumber() {
return Optional.ofNullable(phoneNumber);
}
public SalesCreditMemo withPhoneNumber(String phoneNumber) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("phoneNumber");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.phoneNumber = phoneNumber;
return _x;
}
@Property(name="pricesIncludeTax")
@JsonIgnore
public Optional getPricesIncludeTax() {
return Optional.ofNullable(pricesIncludeTax);
}
public SalesCreditMemo withPricesIncludeTax(Boolean pricesIncludeTax) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("pricesIncludeTax");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.pricesIncludeTax = pricesIncludeTax;
return _x;
}
@Property(name="salesperson")
@JsonIgnore
public Optional getSalesperson() {
return Optional.ofNullable(salesperson);
}
public SalesCreditMemo withSalesperson(String salesperson) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("salesperson");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.salesperson = salesperson;
return _x;
}
@Property(name="sellingPostalAddress")
@JsonIgnore
public Optional getSellingPostalAddress() {
return Optional.ofNullable(sellingPostalAddress);
}
public SalesCreditMemo withSellingPostalAddress(PostalAddressType sellingPostalAddress) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("sellingPostalAddress");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.sellingPostalAddress = sellingPostalAddress;
return _x;
}
@Property(name="status")
@JsonIgnore
public Optional getStatus() {
return Optional.ofNullable(status);
}
public SalesCreditMemo withStatus(String status) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("status");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.status = status;
return _x;
}
@Property(name="totalAmountExcludingTax")
@JsonIgnore
public Optional getTotalAmountExcludingTax() {
return Optional.ofNullable(totalAmountExcludingTax);
}
public SalesCreditMemo withTotalAmountExcludingTax(BigDecimal totalAmountExcludingTax) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("totalAmountExcludingTax");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.totalAmountExcludingTax = totalAmountExcludingTax;
return _x;
}
@Property(name="totalAmountIncludingTax")
@JsonIgnore
public Optional getTotalAmountIncludingTax() {
return Optional.ofNullable(totalAmountIncludingTax);
}
public SalesCreditMemo withTotalAmountIncludingTax(BigDecimal totalAmountIncludingTax) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("totalAmountIncludingTax");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.totalAmountIncludingTax = totalAmountIncludingTax;
return _x;
}
@Property(name="totalTaxAmount")
@JsonIgnore
public Optional getTotalTaxAmount() {
return Optional.ofNullable(totalTaxAmount);
}
public SalesCreditMemo withTotalTaxAmount(BigDecimal totalTaxAmount) {
SalesCreditMemo _x = _copy();
_x.changedFields = changedFields.add("totalTaxAmount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesCreditMemo");
_x.totalTaxAmount = totalTaxAmount;
return _x;
}
public SalesCreditMemo withUnmappedField(String name, String value) {
SalesCreditMemo _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="currency")
@JsonIgnore
public CurrencyRequest getCurrency() {
return new CurrencyRequest(contextPath.addSegment("currency"), RequestHelper.getValue(unmappedFields, "currency"));
}
@NavigationProperty(name="customer")
@JsonIgnore
public CustomerRequest getCustomer() {
return new CustomerRequest(contextPath.addSegment("customer"), RequestHelper.getValue(unmappedFields, "customer"));
}
@NavigationProperty(name="paymentTerm")
@JsonIgnore
public PaymentTermRequest getPaymentTerm() {
return new PaymentTermRequest(contextPath.addSegment("paymentTerm"), RequestHelper.getValue(unmappedFields, "paymentTerm"));
}
@NavigationProperty(name="salesCreditMemoLines")
@JsonIgnore
public SalesCreditMemoLineCollectionRequest getSalesCreditMemoLines() {
return new SalesCreditMemoLineCollectionRequest(
contextPath.addSegment("salesCreditMemoLines"), RequestHelper.getValue(unmappedFields, "salesCreditMemoLines"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public SalesCreditMemo patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
SalesCreditMemo _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public SalesCreditMemo put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
SalesCreditMemo _x = _copy();
_x.changedFields = null;
return _x;
}
private SalesCreditMemo _copy() {
SalesCreditMemo _x = new SalesCreditMemo();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.billingPostalAddress = billingPostalAddress;
_x.billToCustomerId = billToCustomerId;
_x.billToCustomerNumber = billToCustomerNumber;
_x.billToName = billToName;
_x.creditMemoDate = creditMemoDate;
_x.currencyCode = currencyCode;
_x.currencyId = currencyId;
_x.customerId = customerId;
_x.customerName = customerName;
_x.customerNumber = customerNumber;
_x.discountAmount = discountAmount;
_x.discountAppliedBeforeTax = discountAppliedBeforeTax;
_x.dueDate = dueDate;
_x.email = email;
_x.externalDocumentNumber = externalDocumentNumber;
_x.invoiceId = invoiceId;
_x.invoiceNumber = invoiceNumber;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.number = number;
_x.paymentTermsId = paymentTermsId;
_x.phoneNumber = phoneNumber;
_x.pricesIncludeTax = pricesIncludeTax;
_x.salesperson = salesperson;
_x.sellingPostalAddress = sellingPostalAddress;
_x.status = status;
_x.totalAmountExcludingTax = totalAmountExcludingTax;
_x.totalAmountIncludingTax = totalAmountIncludingTax;
_x.totalTaxAmount = totalTaxAmount;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("SalesCreditMemo[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("billingPostalAddress=");
b.append(this.billingPostalAddress);
b.append(", ");
b.append("billToCustomerId=");
b.append(this.billToCustomerId);
b.append(", ");
b.append("billToCustomerNumber=");
b.append(this.billToCustomerNumber);
b.append(", ");
b.append("billToName=");
b.append(this.billToName);
b.append(", ");
b.append("creditMemoDate=");
b.append(this.creditMemoDate);
b.append(", ");
b.append("currencyCode=");
b.append(this.currencyCode);
b.append(", ");
b.append("currencyId=");
b.append(this.currencyId);
b.append(", ");
b.append("customerId=");
b.append(this.customerId);
b.append(", ");
b.append("customerName=");
b.append(this.customerName);
b.append(", ");
b.append("customerNumber=");
b.append(this.customerNumber);
b.append(", ");
b.append("discountAmount=");
b.append(this.discountAmount);
b.append(", ");
b.append("discountAppliedBeforeTax=");
b.append(this.discountAppliedBeforeTax);
b.append(", ");
b.append("dueDate=");
b.append(this.dueDate);
b.append(", ");
b.append("email=");
b.append(this.email);
b.append(", ");
b.append("externalDocumentNumber=");
b.append(this.externalDocumentNumber);
b.append(", ");
b.append("invoiceId=");
b.append(this.invoiceId);
b.append(", ");
b.append("invoiceNumber=");
b.append(this.invoiceNumber);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("number=");
b.append(this.number);
b.append(", ");
b.append("paymentTermsId=");
b.append(this.paymentTermsId);
b.append(", ");
b.append("phoneNumber=");
b.append(this.phoneNumber);
b.append(", ");
b.append("pricesIncludeTax=");
b.append(this.pricesIncludeTax);
b.append(", ");
b.append("salesperson=");
b.append(this.salesperson);
b.append(", ");
b.append("sellingPostalAddress=");
b.append(this.sellingPostalAddress);
b.append(", ");
b.append("status=");
b.append(this.status);
b.append(", ");
b.append("totalAmountExcludingTax=");
b.append(this.totalAmountExcludingTax);
b.append(", ");
b.append("totalAmountIncludingTax=");
b.append(this.totalAmountIncludingTax);
b.append(", ");
b.append("totalTaxAmount=");
b.append(this.totalTaxAmount);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}