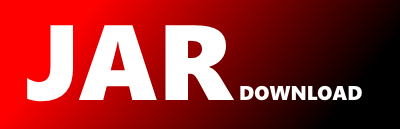
odata.msgraph.client.beta.entity.SalesQuoteLine Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.math.BigDecimal;
import java.util.Optional;
import odata.msgraph.client.beta.entity.request.AccountRequest;
import odata.msgraph.client.beta.entity.request.ItemRequest;
/**
*
* Org.OData.Capabilities.V1.DeleteRestrictions
*
* Deletable = false
*
* Org.OData.Capabilities.V1.InsertRestrictions
*
* Insertable = false
*
* Org.OData.Capabilities.V1.UpdateRestrictions
*
* Updatable = true
*/@JsonPropertyOrder({
"@odata.type",
"accountId",
"amountExcludingTax",
"amountIncludingTax",
"description",
"discountAmount",
"discountAppliedBeforeTax",
"discountPercent",
"documentId",
"itemId",
"lineType",
"netAmount",
"netAmountIncludingTax",
"netTaxAmount",
"quantity",
"sequence",
"taxCode",
"taxPercent",
"totalTaxAmount",
"unitOfMeasureId",
"unitPrice"})
@JsonInclude(Include.NON_NULL)
public class SalesQuoteLine extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.salesQuoteLine";
}
@JsonProperty("accountId")
protected String accountId;
@JsonProperty("amountExcludingTax")
protected BigDecimal amountExcludingTax;
@JsonProperty("amountIncludingTax")
protected BigDecimal amountIncludingTax;
@JsonProperty("description")
protected String description;
@JsonProperty("discountAmount")
protected BigDecimal discountAmount;
@JsonProperty("discountAppliedBeforeTax")
protected Boolean discountAppliedBeforeTax;
@JsonProperty("discountPercent")
protected BigDecimal discountPercent;
@JsonProperty("documentId")
protected String documentId;
@JsonProperty("itemId")
protected String itemId;
@JsonProperty("lineType")
protected String lineType;
@JsonProperty("netAmount")
protected BigDecimal netAmount;
@JsonProperty("netAmountIncludingTax")
protected BigDecimal netAmountIncludingTax;
@JsonProperty("netTaxAmount")
protected BigDecimal netTaxAmount;
@JsonProperty("quantity")
protected BigDecimal quantity;
@JsonProperty("sequence")
protected Integer sequence;
@JsonProperty("taxCode")
protected String taxCode;
@JsonProperty("taxPercent")
protected BigDecimal taxPercent;
@JsonProperty("totalTaxAmount")
protected BigDecimal totalTaxAmount;
@JsonProperty("unitOfMeasureId")
protected String unitOfMeasureId;
@JsonProperty("unitPrice")
protected BigDecimal unitPrice;
protected SalesQuoteLine() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderSalesQuoteLine() {
return new Builder();
}
public static final class Builder {
private String id;
private String accountId;
private BigDecimal amountExcludingTax;
private BigDecimal amountIncludingTax;
private String description;
private BigDecimal discountAmount;
private Boolean discountAppliedBeforeTax;
private BigDecimal discountPercent;
private String documentId;
private String itemId;
private String lineType;
private BigDecimal netAmount;
private BigDecimal netAmountIncludingTax;
private BigDecimal netTaxAmount;
private BigDecimal quantity;
private Integer sequence;
private String taxCode;
private BigDecimal taxPercent;
private BigDecimal totalTaxAmount;
private String unitOfMeasureId;
private BigDecimal unitPrice;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder accountId(String accountId) {
this.accountId = accountId;
this.changedFields = changedFields.add("accountId");
return this;
}
public Builder amountExcludingTax(BigDecimal amountExcludingTax) {
this.amountExcludingTax = amountExcludingTax;
this.changedFields = changedFields.add("amountExcludingTax");
return this;
}
public Builder amountIncludingTax(BigDecimal amountIncludingTax) {
this.amountIncludingTax = amountIncludingTax;
this.changedFields = changedFields.add("amountIncludingTax");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder discountAmount(BigDecimal discountAmount) {
this.discountAmount = discountAmount;
this.changedFields = changedFields.add("discountAmount");
return this;
}
public Builder discountAppliedBeforeTax(Boolean discountAppliedBeforeTax) {
this.discountAppliedBeforeTax = discountAppliedBeforeTax;
this.changedFields = changedFields.add("discountAppliedBeforeTax");
return this;
}
public Builder discountPercent(BigDecimal discountPercent) {
this.discountPercent = discountPercent;
this.changedFields = changedFields.add("discountPercent");
return this;
}
public Builder documentId(String documentId) {
this.documentId = documentId;
this.changedFields = changedFields.add("documentId");
return this;
}
public Builder itemId(String itemId) {
this.itemId = itemId;
this.changedFields = changedFields.add("itemId");
return this;
}
public Builder lineType(String lineType) {
this.lineType = lineType;
this.changedFields = changedFields.add("lineType");
return this;
}
public Builder netAmount(BigDecimal netAmount) {
this.netAmount = netAmount;
this.changedFields = changedFields.add("netAmount");
return this;
}
public Builder netAmountIncludingTax(BigDecimal netAmountIncludingTax) {
this.netAmountIncludingTax = netAmountIncludingTax;
this.changedFields = changedFields.add("netAmountIncludingTax");
return this;
}
public Builder netTaxAmount(BigDecimal netTaxAmount) {
this.netTaxAmount = netTaxAmount;
this.changedFields = changedFields.add("netTaxAmount");
return this;
}
public Builder quantity(BigDecimal quantity) {
this.quantity = quantity;
this.changedFields = changedFields.add("quantity");
return this;
}
public Builder sequence(Integer sequence) {
this.sequence = sequence;
this.changedFields = changedFields.add("sequence");
return this;
}
public Builder taxCode(String taxCode) {
this.taxCode = taxCode;
this.changedFields = changedFields.add("taxCode");
return this;
}
public Builder taxPercent(BigDecimal taxPercent) {
this.taxPercent = taxPercent;
this.changedFields = changedFields.add("taxPercent");
return this;
}
public Builder totalTaxAmount(BigDecimal totalTaxAmount) {
this.totalTaxAmount = totalTaxAmount;
this.changedFields = changedFields.add("totalTaxAmount");
return this;
}
public Builder unitOfMeasureId(String unitOfMeasureId) {
this.unitOfMeasureId = unitOfMeasureId;
this.changedFields = changedFields.add("unitOfMeasureId");
return this;
}
public Builder unitPrice(BigDecimal unitPrice) {
this.unitPrice = unitPrice;
this.changedFields = changedFields.add("unitPrice");
return this;
}
public SalesQuoteLine build() {
SalesQuoteLine _x = new SalesQuoteLine();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.salesQuoteLine";
_x.id = id;
_x.accountId = accountId;
_x.amountExcludingTax = amountExcludingTax;
_x.amountIncludingTax = amountIncludingTax;
_x.description = description;
_x.discountAmount = discountAmount;
_x.discountAppliedBeforeTax = discountAppliedBeforeTax;
_x.discountPercent = discountPercent;
_x.documentId = documentId;
_x.itemId = itemId;
_x.lineType = lineType;
_x.netAmount = netAmount;
_x.netAmountIncludingTax = netAmountIncludingTax;
_x.netTaxAmount = netTaxAmount;
_x.quantity = quantity;
_x.sequence = sequence;
_x.taxCode = taxCode;
_x.taxPercent = taxPercent;
_x.totalTaxAmount = totalTaxAmount;
_x.unitOfMeasureId = unitOfMeasureId;
_x.unitPrice = unitPrice;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
@Property(name="accountId")
@JsonIgnore
public Optional getAccountId() {
return Optional.ofNullable(accountId);
}
public SalesQuoteLine withAccountId(String accountId) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("accountId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.accountId = accountId;
return _x;
}
@Property(name="amountExcludingTax")
@JsonIgnore
public Optional getAmountExcludingTax() {
return Optional.ofNullable(amountExcludingTax);
}
public SalesQuoteLine withAmountExcludingTax(BigDecimal amountExcludingTax) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("amountExcludingTax");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.amountExcludingTax = amountExcludingTax;
return _x;
}
@Property(name="amountIncludingTax")
@JsonIgnore
public Optional getAmountIncludingTax() {
return Optional.ofNullable(amountIncludingTax);
}
public SalesQuoteLine withAmountIncludingTax(BigDecimal amountIncludingTax) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("amountIncludingTax");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.amountIncludingTax = amountIncludingTax;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public SalesQuoteLine withDescription(String description) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.description = description;
return _x;
}
@Property(name="discountAmount")
@JsonIgnore
public Optional getDiscountAmount() {
return Optional.ofNullable(discountAmount);
}
public SalesQuoteLine withDiscountAmount(BigDecimal discountAmount) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("discountAmount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.discountAmount = discountAmount;
return _x;
}
@Property(name="discountAppliedBeforeTax")
@JsonIgnore
public Optional getDiscountAppliedBeforeTax() {
return Optional.ofNullable(discountAppliedBeforeTax);
}
public SalesQuoteLine withDiscountAppliedBeforeTax(Boolean discountAppliedBeforeTax) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("discountAppliedBeforeTax");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.discountAppliedBeforeTax = discountAppliedBeforeTax;
return _x;
}
@Property(name="discountPercent")
@JsonIgnore
public Optional getDiscountPercent() {
return Optional.ofNullable(discountPercent);
}
public SalesQuoteLine withDiscountPercent(BigDecimal discountPercent) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("discountPercent");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.discountPercent = discountPercent;
return _x;
}
@Property(name="documentId")
@JsonIgnore
public Optional getDocumentId() {
return Optional.ofNullable(documentId);
}
public SalesQuoteLine withDocumentId(String documentId) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("documentId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.documentId = documentId;
return _x;
}
@Property(name="itemId")
@JsonIgnore
public Optional getItemId() {
return Optional.ofNullable(itemId);
}
public SalesQuoteLine withItemId(String itemId) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("itemId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.itemId = itemId;
return _x;
}
@Property(name="lineType")
@JsonIgnore
public Optional getLineType() {
return Optional.ofNullable(lineType);
}
public SalesQuoteLine withLineType(String lineType) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("lineType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.lineType = lineType;
return _x;
}
@Property(name="netAmount")
@JsonIgnore
public Optional getNetAmount() {
return Optional.ofNullable(netAmount);
}
public SalesQuoteLine withNetAmount(BigDecimal netAmount) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("netAmount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.netAmount = netAmount;
return _x;
}
@Property(name="netAmountIncludingTax")
@JsonIgnore
public Optional getNetAmountIncludingTax() {
return Optional.ofNullable(netAmountIncludingTax);
}
public SalesQuoteLine withNetAmountIncludingTax(BigDecimal netAmountIncludingTax) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("netAmountIncludingTax");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.netAmountIncludingTax = netAmountIncludingTax;
return _x;
}
@Property(name="netTaxAmount")
@JsonIgnore
public Optional getNetTaxAmount() {
return Optional.ofNullable(netTaxAmount);
}
public SalesQuoteLine withNetTaxAmount(BigDecimal netTaxAmount) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("netTaxAmount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.netTaxAmount = netTaxAmount;
return _x;
}
@Property(name="quantity")
@JsonIgnore
public Optional getQuantity() {
return Optional.ofNullable(quantity);
}
public SalesQuoteLine withQuantity(BigDecimal quantity) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("quantity");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.quantity = quantity;
return _x;
}
@Property(name="sequence")
@JsonIgnore
public Optional getSequence() {
return Optional.ofNullable(sequence);
}
public SalesQuoteLine withSequence(Integer sequence) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("sequence");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.sequence = sequence;
return _x;
}
@Property(name="taxCode")
@JsonIgnore
public Optional getTaxCode() {
return Optional.ofNullable(taxCode);
}
public SalesQuoteLine withTaxCode(String taxCode) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("taxCode");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.taxCode = taxCode;
return _x;
}
@Property(name="taxPercent")
@JsonIgnore
public Optional getTaxPercent() {
return Optional.ofNullable(taxPercent);
}
public SalesQuoteLine withTaxPercent(BigDecimal taxPercent) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("taxPercent");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.taxPercent = taxPercent;
return _x;
}
@Property(name="totalTaxAmount")
@JsonIgnore
public Optional getTotalTaxAmount() {
return Optional.ofNullable(totalTaxAmount);
}
public SalesQuoteLine withTotalTaxAmount(BigDecimal totalTaxAmount) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("totalTaxAmount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.totalTaxAmount = totalTaxAmount;
return _x;
}
@Property(name="unitOfMeasureId")
@JsonIgnore
public Optional getUnitOfMeasureId() {
return Optional.ofNullable(unitOfMeasureId);
}
public SalesQuoteLine withUnitOfMeasureId(String unitOfMeasureId) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("unitOfMeasureId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.unitOfMeasureId = unitOfMeasureId;
return _x;
}
@Property(name="unitPrice")
@JsonIgnore
public Optional getUnitPrice() {
return Optional.ofNullable(unitPrice);
}
public SalesQuoteLine withUnitPrice(BigDecimal unitPrice) {
SalesQuoteLine _x = _copy();
_x.changedFields = changedFields.add("unitPrice");
_x.odataType = Util.nvl(odataType, "microsoft.graph.salesQuoteLine");
_x.unitPrice = unitPrice;
return _x;
}
public SalesQuoteLine withUnmappedField(String name, String value) {
SalesQuoteLine _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="account")
@JsonIgnore
public AccountRequest getAccount() {
return new AccountRequest(contextPath.addSegment("account"), RequestHelper.getValue(unmappedFields, "account"));
}
@NavigationProperty(name="item")
@JsonIgnore
public ItemRequest getItem() {
return new ItemRequest(contextPath.addSegment("item"), RequestHelper.getValue(unmappedFields, "item"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public SalesQuoteLine patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
SalesQuoteLine _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public SalesQuoteLine put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
SalesQuoteLine _x = _copy();
_x.changedFields = null;
return _x;
}
private SalesQuoteLine _copy() {
SalesQuoteLine _x = new SalesQuoteLine();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.accountId = accountId;
_x.amountExcludingTax = amountExcludingTax;
_x.amountIncludingTax = amountIncludingTax;
_x.description = description;
_x.discountAmount = discountAmount;
_x.discountAppliedBeforeTax = discountAppliedBeforeTax;
_x.discountPercent = discountPercent;
_x.documentId = documentId;
_x.itemId = itemId;
_x.lineType = lineType;
_x.netAmount = netAmount;
_x.netAmountIncludingTax = netAmountIncludingTax;
_x.netTaxAmount = netTaxAmount;
_x.quantity = quantity;
_x.sequence = sequence;
_x.taxCode = taxCode;
_x.taxPercent = taxPercent;
_x.totalTaxAmount = totalTaxAmount;
_x.unitOfMeasureId = unitOfMeasureId;
_x.unitPrice = unitPrice;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("SalesQuoteLine[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("accountId=");
b.append(this.accountId);
b.append(", ");
b.append("amountExcludingTax=");
b.append(this.amountExcludingTax);
b.append(", ");
b.append("amountIncludingTax=");
b.append(this.amountIncludingTax);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("discountAmount=");
b.append(this.discountAmount);
b.append(", ");
b.append("discountAppliedBeforeTax=");
b.append(this.discountAppliedBeforeTax);
b.append(", ");
b.append("discountPercent=");
b.append(this.discountPercent);
b.append(", ");
b.append("documentId=");
b.append(this.documentId);
b.append(", ");
b.append("itemId=");
b.append(this.itemId);
b.append(", ");
b.append("lineType=");
b.append(this.lineType);
b.append(", ");
b.append("netAmount=");
b.append(this.netAmount);
b.append(", ");
b.append("netAmountIncludingTax=");
b.append(this.netAmountIncludingTax);
b.append(", ");
b.append("netTaxAmount=");
b.append(this.netTaxAmount);
b.append(", ");
b.append("quantity=");
b.append(this.quantity);
b.append(", ");
b.append("sequence=");
b.append(this.sequence);
b.append(", ");
b.append("taxCode=");
b.append(this.taxCode);
b.append(", ");
b.append("taxPercent=");
b.append(this.taxPercent);
b.append(", ");
b.append("totalTaxAmount=");
b.append(this.totalTaxAmount);
b.append(", ");
b.append("unitOfMeasureId=");
b.append(this.unitOfMeasureId);
b.append(", ");
b.append("unitPrice=");
b.append(this.unitPrice);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}