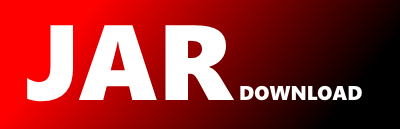
odata.msgraph.client.beta.entity.SubscribedSku Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.complex.LicenseUnitsDetail;
import odata.msgraph.client.beta.complex.ServicePlanInfo;
/**
*
* Org.OData.Capabilities.V1.CountRestrictions
*
* Countable = false
*
* Org.OData.Capabilities.V1.ExpandRestrictions
*
* Expandable = false
*
* Org.OData.Capabilities.V1.FilterRestrictions
*
* Filterable = false
*
* Org.OData.Capabilities.V1.NavigationRestrictions
*
* Referenceable = false
*
* Org.OData.Capabilities.V1.SkipSupported
*
* false
*
* Org.OData.Capabilities.V1.TopSupported
*
* false
*/@JsonPropertyOrder({
"@odata.type",
"appliesTo",
"capabilityStatus",
"consumedUnits",
"prepaidUnits",
"servicePlans",
"skuId",
"skuPartNumber"})
@JsonInclude(Include.NON_NULL)
public class SubscribedSku extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.subscribedSku";
}
@JsonProperty("appliesTo")
protected String appliesTo;
@JsonProperty("capabilityStatus")
protected String capabilityStatus;
@JsonProperty("consumedUnits")
protected Integer consumedUnits;
@JsonProperty("prepaidUnits")
protected LicenseUnitsDetail prepaidUnits;
@JsonProperty("servicePlans")
protected List servicePlans;
@JsonProperty("servicePlans@nextLink")
protected String servicePlansNextLink;
@JsonProperty("skuId")
protected String skuId;
@JsonProperty("skuPartNumber")
protected String skuPartNumber;
protected SubscribedSku() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderSubscribedSku() {
return new Builder();
}
public static final class Builder {
private String id;
private String appliesTo;
private String capabilityStatus;
private Integer consumedUnits;
private LicenseUnitsDetail prepaidUnits;
private List servicePlans;
private String servicePlansNextLink;
private String skuId;
private String skuPartNumber;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder appliesTo(String appliesTo) {
this.appliesTo = appliesTo;
this.changedFields = changedFields.add("appliesTo");
return this;
}
public Builder capabilityStatus(String capabilityStatus) {
this.capabilityStatus = capabilityStatus;
this.changedFields = changedFields.add("capabilityStatus");
return this;
}
public Builder consumedUnits(Integer consumedUnits) {
this.consumedUnits = consumedUnits;
this.changedFields = changedFields.add("consumedUnits");
return this;
}
public Builder prepaidUnits(LicenseUnitsDetail prepaidUnits) {
this.prepaidUnits = prepaidUnits;
this.changedFields = changedFields.add("prepaidUnits");
return this;
}
public Builder servicePlans(List servicePlans) {
this.servicePlans = servicePlans;
this.changedFields = changedFields.add("servicePlans");
return this;
}
public Builder servicePlans(ServicePlanInfo... servicePlans) {
return servicePlans(Arrays.asList(servicePlans));
}
public Builder servicePlansNextLink(String servicePlansNextLink) {
this.servicePlansNextLink = servicePlansNextLink;
this.changedFields = changedFields.add("servicePlans");
return this;
}
public Builder skuId(String skuId) {
this.skuId = skuId;
this.changedFields = changedFields.add("skuId");
return this;
}
public Builder skuPartNumber(String skuPartNumber) {
this.skuPartNumber = skuPartNumber;
this.changedFields = changedFields.add("skuPartNumber");
return this;
}
public SubscribedSku build() {
SubscribedSku _x = new SubscribedSku();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.subscribedSku";
_x.id = id;
_x.appliesTo = appliesTo;
_x.capabilityStatus = capabilityStatus;
_x.consumedUnits = consumedUnits;
_x.prepaidUnits = prepaidUnits;
_x.servicePlans = servicePlans;
_x.servicePlansNextLink = servicePlansNextLink;
_x.skuId = skuId;
_x.skuPartNumber = skuPartNumber;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
@Property(name="appliesTo")
@JsonIgnore
public Optional getAppliesTo() {
return Optional.ofNullable(appliesTo);
}
public SubscribedSku withAppliesTo(String appliesTo) {
SubscribedSku _x = _copy();
_x.changedFields = changedFields.add("appliesTo");
_x.odataType = Util.nvl(odataType, "microsoft.graph.subscribedSku");
_x.appliesTo = appliesTo;
return _x;
}
@Property(name="capabilityStatus")
@JsonIgnore
public Optional getCapabilityStatus() {
return Optional.ofNullable(capabilityStatus);
}
public SubscribedSku withCapabilityStatus(String capabilityStatus) {
SubscribedSku _x = _copy();
_x.changedFields = changedFields.add("capabilityStatus");
_x.odataType = Util.nvl(odataType, "microsoft.graph.subscribedSku");
_x.capabilityStatus = capabilityStatus;
return _x;
}
@Property(name="consumedUnits")
@JsonIgnore
public Optional getConsumedUnits() {
return Optional.ofNullable(consumedUnits);
}
public SubscribedSku withConsumedUnits(Integer consumedUnits) {
SubscribedSku _x = _copy();
_x.changedFields = changedFields.add("consumedUnits");
_x.odataType = Util.nvl(odataType, "microsoft.graph.subscribedSku");
_x.consumedUnits = consumedUnits;
return _x;
}
@Property(name="prepaidUnits")
@JsonIgnore
public Optional getPrepaidUnits() {
return Optional.ofNullable(prepaidUnits);
}
public SubscribedSku withPrepaidUnits(LicenseUnitsDetail prepaidUnits) {
SubscribedSku _x = _copy();
_x.changedFields = changedFields.add("prepaidUnits");
_x.odataType = Util.nvl(odataType, "microsoft.graph.subscribedSku");
_x.prepaidUnits = prepaidUnits;
return _x;
}
@Property(name="servicePlans")
@JsonIgnore
public CollectionPage getServicePlans() {
return new CollectionPage(contextPath, ServicePlanInfo.class, this.servicePlans, Optional.ofNullable(servicePlansNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public SubscribedSku withServicePlans(List servicePlans) {
SubscribedSku _x = _copy();
_x.changedFields = changedFields.add("servicePlans");
_x.odataType = Util.nvl(odataType, "microsoft.graph.subscribedSku");
_x.servicePlans = servicePlans;
return _x;
}
@Property(name="servicePlans")
@JsonIgnore
public CollectionPage getServicePlans(HttpRequestOptions options) {
return new CollectionPage(contextPath, ServicePlanInfo.class, this.servicePlans, Optional.ofNullable(servicePlansNextLink), Collections.emptyList(), options);
}
@Property(name="skuId")
@JsonIgnore
public Optional getSkuId() {
return Optional.ofNullable(skuId);
}
public SubscribedSku withSkuId(String skuId) {
SubscribedSku _x = _copy();
_x.changedFields = changedFields.add("skuId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.subscribedSku");
_x.skuId = skuId;
return _x;
}
@Property(name="skuPartNumber")
@JsonIgnore
public Optional getSkuPartNumber() {
return Optional.ofNullable(skuPartNumber);
}
public SubscribedSku withSkuPartNumber(String skuPartNumber) {
SubscribedSku _x = _copy();
_x.changedFields = changedFields.add("skuPartNumber");
_x.odataType = Util.nvl(odataType, "microsoft.graph.subscribedSku");
_x.skuPartNumber = skuPartNumber;
return _x;
}
public SubscribedSku withUnmappedField(String name, String value) {
SubscribedSku _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public SubscribedSku patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
SubscribedSku _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public SubscribedSku put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
SubscribedSku _x = _copy();
_x.changedFields = null;
return _x;
}
private SubscribedSku _copy() {
SubscribedSku _x = new SubscribedSku();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.appliesTo = appliesTo;
_x.capabilityStatus = capabilityStatus;
_x.consumedUnits = consumedUnits;
_x.prepaidUnits = prepaidUnits;
_x.servicePlans = servicePlans;
_x.skuId = skuId;
_x.skuPartNumber = skuPartNumber;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("SubscribedSku[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("appliesTo=");
b.append(this.appliesTo);
b.append(", ");
b.append("capabilityStatus=");
b.append(this.capabilityStatus);
b.append(", ");
b.append("consumedUnits=");
b.append(this.consumedUnits);
b.append(", ");
b.append("prepaidUnits=");
b.append(this.prepaidUnits);
b.append(", ");
b.append("servicePlans=");
b.append(this.servicePlans);
b.append(", ");
b.append("skuId=");
b.append(this.skuId);
b.append(", ");
b.append("skuPartNumber=");
b.append(this.skuPartNumber);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}