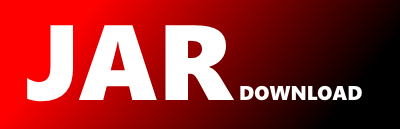
odata.msgraph.client.beta.entity.TeamsDeviceUsageDistributionUserCounts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph-beta Show documentation
Show all versions of odata-client-msgraph-beta Show documentation
Java client for use with Microsoft Graph beta endpoint
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.LocalDate;
import java.util.Optional;
@JsonPropertyOrder({
"@odata.type",
"androidPhone",
"chromeOS",
"ios",
"linux",
"mac",
"reportPeriod",
"reportRefreshDate",
"web",
"windows",
"windowsPhone"})
@JsonInclude(Include.NON_NULL)
public class TeamsDeviceUsageDistributionUserCounts extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.teamsDeviceUsageDistributionUserCounts";
}
@JsonProperty("androidPhone")
protected Long androidPhone;
@JsonProperty("chromeOS")
protected Long chromeOS;
@JsonProperty("ios")
protected Long ios;
@JsonProperty("linux")
protected Long linux;
@JsonProperty("mac")
protected Long mac;
@JsonProperty("reportPeriod")
protected String reportPeriod;
@JsonProperty("reportRefreshDate")
protected LocalDate reportRefreshDate;
@JsonProperty("web")
protected Long web;
@JsonProperty("windows")
protected Long windows;
@JsonProperty("windowsPhone")
protected Long windowsPhone;
protected TeamsDeviceUsageDistributionUserCounts() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderTeamsDeviceUsageDistributionUserCounts() {
return new Builder();
}
public static final class Builder {
private String id;
private Long androidPhone;
private Long chromeOS;
private Long ios;
private Long linux;
private Long mac;
private String reportPeriod;
private LocalDate reportRefreshDate;
private Long web;
private Long windows;
private Long windowsPhone;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder androidPhone(Long androidPhone) {
this.androidPhone = androidPhone;
this.changedFields = changedFields.add("androidPhone");
return this;
}
public Builder chromeOS(Long chromeOS) {
this.chromeOS = chromeOS;
this.changedFields = changedFields.add("chromeOS");
return this;
}
public Builder ios(Long ios) {
this.ios = ios;
this.changedFields = changedFields.add("ios");
return this;
}
public Builder linux(Long linux) {
this.linux = linux;
this.changedFields = changedFields.add("linux");
return this;
}
public Builder mac(Long mac) {
this.mac = mac;
this.changedFields = changedFields.add("mac");
return this;
}
public Builder reportPeriod(String reportPeriod) {
this.reportPeriod = reportPeriod;
this.changedFields = changedFields.add("reportPeriod");
return this;
}
public Builder reportRefreshDate(LocalDate reportRefreshDate) {
this.reportRefreshDate = reportRefreshDate;
this.changedFields = changedFields.add("reportRefreshDate");
return this;
}
public Builder web(Long web) {
this.web = web;
this.changedFields = changedFields.add("web");
return this;
}
public Builder windows(Long windows) {
this.windows = windows;
this.changedFields = changedFields.add("windows");
return this;
}
public Builder windowsPhone(Long windowsPhone) {
this.windowsPhone = windowsPhone;
this.changedFields = changedFields.add("windowsPhone");
return this;
}
public TeamsDeviceUsageDistributionUserCounts build() {
TeamsDeviceUsageDistributionUserCounts _x = new TeamsDeviceUsageDistributionUserCounts();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.teamsDeviceUsageDistributionUserCounts";
_x.id = id;
_x.androidPhone = androidPhone;
_x.chromeOS = chromeOS;
_x.ios = ios;
_x.linux = linux;
_x.mac = mac;
_x.reportPeriod = reportPeriod;
_x.reportRefreshDate = reportRefreshDate;
_x.web = web;
_x.windows = windows;
_x.windowsPhone = windowsPhone;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
@Property(name="androidPhone")
@JsonIgnore
public Optional getAndroidPhone() {
return Optional.ofNullable(androidPhone);
}
public TeamsDeviceUsageDistributionUserCounts withAndroidPhone(Long androidPhone) {
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.changedFields = changedFields.add("androidPhone");
_x.odataType = Util.nvl(odataType, "microsoft.graph.teamsDeviceUsageDistributionUserCounts");
_x.androidPhone = androidPhone;
return _x;
}
@Property(name="chromeOS")
@JsonIgnore
public Optional getChromeOS() {
return Optional.ofNullable(chromeOS);
}
public TeamsDeviceUsageDistributionUserCounts withChromeOS(Long chromeOS) {
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.changedFields = changedFields.add("chromeOS");
_x.odataType = Util.nvl(odataType, "microsoft.graph.teamsDeviceUsageDistributionUserCounts");
_x.chromeOS = chromeOS;
return _x;
}
@Property(name="ios")
@JsonIgnore
public Optional getIos() {
return Optional.ofNullable(ios);
}
public TeamsDeviceUsageDistributionUserCounts withIos(Long ios) {
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.changedFields = changedFields.add("ios");
_x.odataType = Util.nvl(odataType, "microsoft.graph.teamsDeviceUsageDistributionUserCounts");
_x.ios = ios;
return _x;
}
@Property(name="linux")
@JsonIgnore
public Optional getLinux() {
return Optional.ofNullable(linux);
}
public TeamsDeviceUsageDistributionUserCounts withLinux(Long linux) {
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.changedFields = changedFields.add("linux");
_x.odataType = Util.nvl(odataType, "microsoft.graph.teamsDeviceUsageDistributionUserCounts");
_x.linux = linux;
return _x;
}
@Property(name="mac")
@JsonIgnore
public Optional getMac() {
return Optional.ofNullable(mac);
}
public TeamsDeviceUsageDistributionUserCounts withMac(Long mac) {
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.changedFields = changedFields.add("mac");
_x.odataType = Util.nvl(odataType, "microsoft.graph.teamsDeviceUsageDistributionUserCounts");
_x.mac = mac;
return _x;
}
@Property(name="reportPeriod")
@JsonIgnore
public Optional getReportPeriod() {
return Optional.ofNullable(reportPeriod);
}
public TeamsDeviceUsageDistributionUserCounts withReportPeriod(String reportPeriod) {
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.changedFields = changedFields.add("reportPeriod");
_x.odataType = Util.nvl(odataType, "microsoft.graph.teamsDeviceUsageDistributionUserCounts");
_x.reportPeriod = reportPeriod;
return _x;
}
@Property(name="reportRefreshDate")
@JsonIgnore
public Optional getReportRefreshDate() {
return Optional.ofNullable(reportRefreshDate);
}
public TeamsDeviceUsageDistributionUserCounts withReportRefreshDate(LocalDate reportRefreshDate) {
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.changedFields = changedFields.add("reportRefreshDate");
_x.odataType = Util.nvl(odataType, "microsoft.graph.teamsDeviceUsageDistributionUserCounts");
_x.reportRefreshDate = reportRefreshDate;
return _x;
}
@Property(name="web")
@JsonIgnore
public Optional getWeb() {
return Optional.ofNullable(web);
}
public TeamsDeviceUsageDistributionUserCounts withWeb(Long web) {
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.changedFields = changedFields.add("web");
_x.odataType = Util.nvl(odataType, "microsoft.graph.teamsDeviceUsageDistributionUserCounts");
_x.web = web;
return _x;
}
@Property(name="windows")
@JsonIgnore
public Optional getWindows() {
return Optional.ofNullable(windows);
}
public TeamsDeviceUsageDistributionUserCounts withWindows(Long windows) {
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.changedFields = changedFields.add("windows");
_x.odataType = Util.nvl(odataType, "microsoft.graph.teamsDeviceUsageDistributionUserCounts");
_x.windows = windows;
return _x;
}
@Property(name="windowsPhone")
@JsonIgnore
public Optional getWindowsPhone() {
return Optional.ofNullable(windowsPhone);
}
public TeamsDeviceUsageDistributionUserCounts withWindowsPhone(Long windowsPhone) {
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.changedFields = changedFields.add("windowsPhone");
_x.odataType = Util.nvl(odataType, "microsoft.graph.teamsDeviceUsageDistributionUserCounts");
_x.windowsPhone = windowsPhone;
return _x;
}
public TeamsDeviceUsageDistributionUserCounts withUnmappedField(String name, String value) {
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public TeamsDeviceUsageDistributionUserCounts patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public TeamsDeviceUsageDistributionUserCounts put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
TeamsDeviceUsageDistributionUserCounts _x = _copy();
_x.changedFields = null;
return _x;
}
private TeamsDeviceUsageDistributionUserCounts _copy() {
TeamsDeviceUsageDistributionUserCounts _x = new TeamsDeviceUsageDistributionUserCounts();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.androidPhone = androidPhone;
_x.chromeOS = chromeOS;
_x.ios = ios;
_x.linux = linux;
_x.mac = mac;
_x.reportPeriod = reportPeriod;
_x.reportRefreshDate = reportRefreshDate;
_x.web = web;
_x.windows = windows;
_x.windowsPhone = windowsPhone;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("TeamsDeviceUsageDistributionUserCounts[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("androidPhone=");
b.append(this.androidPhone);
b.append(", ");
b.append("chromeOS=");
b.append(this.chromeOS);
b.append(", ");
b.append("ios=");
b.append(this.ios);
b.append(", ");
b.append("linux=");
b.append(this.linux);
b.append(", ");
b.append("mac=");
b.append(this.mac);
b.append(", ");
b.append("reportPeriod=");
b.append(this.reportPeriod);
b.append(", ");
b.append("reportRefreshDate=");
b.append(this.reportRefreshDate);
b.append(", ");
b.append("web=");
b.append(this.web);
b.append(", ");
b.append("windows=");
b.append(this.windows);
b.append(", ");
b.append("windowsPhone=");
b.append(this.windowsPhone);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy