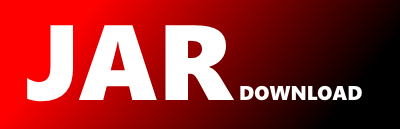
odata.msgraph.client.beta.entity.UserExperienceAnalyticsRemoteConnection Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Double;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
/**
* “The user experience analyte remote connection entity.”
*/@JsonPropertyOrder({
"@odata.type",
"cloudPcFailurePercentage",
"cloudPcRoundTripTime",
"cloudPcSignInTime",
"coreBootTime",
"coreSignInTime",
"deviceCount",
"deviceId",
"deviceName",
"manufacturer",
"model",
"remoteSignInTime",
"userPrincipalName",
"virtualNetwork"})
@JsonInclude(Include.NON_NULL)
public class UserExperienceAnalyticsRemoteConnection extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.userExperienceAnalyticsRemoteConnection";
}
@JsonProperty("cloudPcFailurePercentage")
protected Double cloudPcFailurePercentage;
@JsonProperty("cloudPcRoundTripTime")
protected Double cloudPcRoundTripTime;
@JsonProperty("cloudPcSignInTime")
protected Double cloudPcSignInTime;
@JsonProperty("coreBootTime")
protected Double coreBootTime;
@JsonProperty("coreSignInTime")
protected Double coreSignInTime;
@JsonProperty("deviceCount")
protected Integer deviceCount;
@JsonProperty("deviceId")
protected String deviceId;
@JsonProperty("deviceName")
protected String deviceName;
@JsonProperty("manufacturer")
protected String manufacturer;
@JsonProperty("model")
protected String model;
@JsonProperty("remoteSignInTime")
protected Double remoteSignInTime;
@JsonProperty("userPrincipalName")
protected String userPrincipalName;
@JsonProperty("virtualNetwork")
protected String virtualNetwork;
protected UserExperienceAnalyticsRemoteConnection() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderUserExperienceAnalyticsRemoteConnection() {
return new Builder();
}
public static final class Builder {
private String id;
private Double cloudPcFailurePercentage;
private Double cloudPcRoundTripTime;
private Double cloudPcSignInTime;
private Double coreBootTime;
private Double coreSignInTime;
private Integer deviceCount;
private String deviceId;
private String deviceName;
private String manufacturer;
private String model;
private Double remoteSignInTime;
private String userPrincipalName;
private String virtualNetwork;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
/**
* “The sign in failure percentage of Cloud PC Device. Valid values 0 to 100”
*
* @param cloudPcFailurePercentage
* value of {@code cloudPcFailurePercentage} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder cloudPcFailurePercentage(Double cloudPcFailurePercentage) {
this.cloudPcFailurePercentage = cloudPcFailurePercentage;
this.changedFields = changedFields.add("cloudPcFailurePercentage");
return this;
}
/**
* “The round tip time of Cloud PC Device. Valid values 0 to 1.79769313486232E+308”
*
* @param cloudPcRoundTripTime
* value of {@code cloudPcRoundTripTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder cloudPcRoundTripTime(Double cloudPcRoundTripTime) {
this.cloudPcRoundTripTime = cloudPcRoundTripTime;
this.changedFields = changedFields.add("cloudPcRoundTripTime");
return this;
}
/**
* “The sign in time of Cloud PC Device. Valid values 0 to 1.79769313486232E+308”
*
* @param cloudPcSignInTime
* value of {@code cloudPcSignInTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder cloudPcSignInTime(Double cloudPcSignInTime) {
this.cloudPcSignInTime = cloudPcSignInTime;
this.changedFields = changedFields.add("cloudPcSignInTime");
return this;
}
/**
* “The core boot time of Cloud PC Device. Valid values 0 to 1.79769313486232E+308”
*
* @param coreBootTime
* value of {@code coreBootTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder coreBootTime(Double coreBootTime) {
this.coreBootTime = coreBootTime;
this.changedFields = changedFields.add("coreBootTime");
return this;
}
/**
* “The core sign in time of Cloud PC Device. Valid values 0 to 1.79769313486232E+
* 308”
*
* @param coreSignInTime
* value of {@code coreSignInTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder coreSignInTime(Double coreSignInTime) {
this.coreSignInTime = coreSignInTime;
this.changedFields = changedFields.add("coreSignInTime");
return this;
}
/**
* “The count of remote connection. Valid values 0 to 2147483647”
*
* @param deviceCount
* value of {@code deviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceCount(Integer deviceCount) {
this.deviceCount = deviceCount;
this.changedFields = changedFields.add("deviceCount");
return this;
}
/**
* “The id of the device.”
*
* @param deviceId
* value of {@code deviceId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceId(String deviceId) {
this.deviceId = deviceId;
this.changedFields = changedFields.add("deviceId");
return this;
}
/**
* “The name of the device.”
*
* @param deviceName
* value of {@code deviceName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceName(String deviceName) {
this.deviceName = deviceName;
this.changedFields = changedFields.add("deviceName");
return this;
}
/**
* “The user experience analytics manufacturer.”
*
* @param manufacturer
* value of {@code manufacturer} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder manufacturer(String manufacturer) {
this.manufacturer = manufacturer;
this.changedFields = changedFields.add("manufacturer");
return this;
}
/**
* “The user experience analytics device model.”
*
* @param model
* value of {@code model} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder model(String model) {
this.model = model;
this.changedFields = changedFields.add("model");
return this;
}
/**
* “The remote sign in time of Cloud PC Device. Valid values 0 to 1.79769313486232E+
* 308”
*
* @param remoteSignInTime
* value of {@code remoteSignInTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder remoteSignInTime(Double remoteSignInTime) {
this.remoteSignInTime = remoteSignInTime;
this.changedFields = changedFields.add("remoteSignInTime");
return this;
}
/**
* “The user experience analytics userPrincipalName.”
*
* @param userPrincipalName
* value of {@code userPrincipalName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userPrincipalName(String userPrincipalName) {
this.userPrincipalName = userPrincipalName;
this.changedFields = changedFields.add("userPrincipalName");
return this;
}
/**
* “The user experience analytics virtual network.”
*
* @param virtualNetwork
* value of {@code virtualNetwork} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder virtualNetwork(String virtualNetwork) {
this.virtualNetwork = virtualNetwork;
this.changedFields = changedFields.add("virtualNetwork");
return this;
}
public UserExperienceAnalyticsRemoteConnection build() {
UserExperienceAnalyticsRemoteConnection _x = new UserExperienceAnalyticsRemoteConnection();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.userExperienceAnalyticsRemoteConnection";
_x.id = id;
_x.cloudPcFailurePercentage = cloudPcFailurePercentage;
_x.cloudPcRoundTripTime = cloudPcRoundTripTime;
_x.cloudPcSignInTime = cloudPcSignInTime;
_x.coreBootTime = coreBootTime;
_x.coreSignInTime = coreSignInTime;
_x.deviceCount = deviceCount;
_x.deviceId = deviceId;
_x.deviceName = deviceName;
_x.manufacturer = manufacturer;
_x.model = model;
_x.remoteSignInTime = remoteSignInTime;
_x.userPrincipalName = userPrincipalName;
_x.virtualNetwork = virtualNetwork;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “The sign in failure percentage of Cloud PC Device. Valid values 0 to 100”
*
* @return property cloudPcFailurePercentage
*/
@Property(name="cloudPcFailurePercentage")
@JsonIgnore
public Optional getCloudPcFailurePercentage() {
return Optional.ofNullable(cloudPcFailurePercentage);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* cloudPcFailurePercentage} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The sign in failure percentage of Cloud PC Device. Valid values 0 to 100”
*
* @param cloudPcFailurePercentage
* new value of {@code cloudPcFailurePercentage} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code cloudPcFailurePercentage} field changed
*/
public UserExperienceAnalyticsRemoteConnection withCloudPcFailurePercentage(Double cloudPcFailurePercentage) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("cloudPcFailurePercentage");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.cloudPcFailurePercentage = cloudPcFailurePercentage;
return _x;
}
/**
* “The round tip time of Cloud PC Device. Valid values 0 to 1.79769313486232E+308”
*
* @return property cloudPcRoundTripTime
*/
@Property(name="cloudPcRoundTripTime")
@JsonIgnore
public Optional getCloudPcRoundTripTime() {
return Optional.ofNullable(cloudPcRoundTripTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* cloudPcRoundTripTime} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The round tip time of Cloud PC Device. Valid values 0 to 1.79769313486232E+308”
*
* @param cloudPcRoundTripTime
* new value of {@code cloudPcRoundTripTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code cloudPcRoundTripTime} field changed
*/
public UserExperienceAnalyticsRemoteConnection withCloudPcRoundTripTime(Double cloudPcRoundTripTime) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("cloudPcRoundTripTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.cloudPcRoundTripTime = cloudPcRoundTripTime;
return _x;
}
/**
* “The sign in time of Cloud PC Device. Valid values 0 to 1.79769313486232E+308”
*
* @return property cloudPcSignInTime
*/
@Property(name="cloudPcSignInTime")
@JsonIgnore
public Optional getCloudPcSignInTime() {
return Optional.ofNullable(cloudPcSignInTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code cloudPcSignInTime
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The sign in time of Cloud PC Device. Valid values 0 to 1.79769313486232E+308”
*
* @param cloudPcSignInTime
* new value of {@code cloudPcSignInTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code cloudPcSignInTime} field changed
*/
public UserExperienceAnalyticsRemoteConnection withCloudPcSignInTime(Double cloudPcSignInTime) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("cloudPcSignInTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.cloudPcSignInTime = cloudPcSignInTime;
return _x;
}
/**
* “The core boot time of Cloud PC Device. Valid values 0 to 1.79769313486232E+308”
*
* @return property coreBootTime
*/
@Property(name="coreBootTime")
@JsonIgnore
public Optional getCoreBootTime() {
return Optional.ofNullable(coreBootTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code coreBootTime}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The core boot time of Cloud PC Device. Valid values 0 to 1.79769313486232E+308”
*
* @param coreBootTime
* new value of {@code coreBootTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code coreBootTime} field changed
*/
public UserExperienceAnalyticsRemoteConnection withCoreBootTime(Double coreBootTime) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("coreBootTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.coreBootTime = coreBootTime;
return _x;
}
/**
* “The core sign in time of Cloud PC Device. Valid values 0 to 1.79769313486232E+
* 308”
*
* @return property coreSignInTime
*/
@Property(name="coreSignInTime")
@JsonIgnore
public Optional getCoreSignInTime() {
return Optional.ofNullable(coreSignInTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code coreSignInTime}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The core sign in time of Cloud PC Device. Valid values 0 to 1.79769313486232E+
* 308”
*
* @param coreSignInTime
* new value of {@code coreSignInTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code coreSignInTime} field changed
*/
public UserExperienceAnalyticsRemoteConnection withCoreSignInTime(Double coreSignInTime) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("coreSignInTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.coreSignInTime = coreSignInTime;
return _x;
}
/**
* “The count of remote connection. Valid values 0 to 2147483647”
*
* @return property deviceCount
*/
@Property(name="deviceCount")
@JsonIgnore
public Optional getDeviceCount() {
return Optional.ofNullable(deviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceCount}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The count of remote connection. Valid values 0 to 2147483647”
*
* @param deviceCount
* new value of {@code deviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceCount} field changed
*/
public UserExperienceAnalyticsRemoteConnection withDeviceCount(Integer deviceCount) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("deviceCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.deviceCount = deviceCount;
return _x;
}
/**
* “The id of the device.”
*
* @return property deviceId
*/
@Property(name="deviceId")
@JsonIgnore
public Optional getDeviceId() {
return Optional.ofNullable(deviceId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceId} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The id of the device.”
*
* @param deviceId
* new value of {@code deviceId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceId} field changed
*/
public UserExperienceAnalyticsRemoteConnection withDeviceId(String deviceId) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("deviceId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.deviceId = deviceId;
return _x;
}
/**
* “The name of the device.”
*
* @return property deviceName
*/
@Property(name="deviceName")
@JsonIgnore
public Optional getDeviceName() {
return Optional.ofNullable(deviceName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceName} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The name of the device.”
*
* @param deviceName
* new value of {@code deviceName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceName} field changed
*/
public UserExperienceAnalyticsRemoteConnection withDeviceName(String deviceName) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("deviceName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.deviceName = deviceName;
return _x;
}
/**
* “The user experience analytics manufacturer.”
*
* @return property manufacturer
*/
@Property(name="manufacturer")
@JsonIgnore
public Optional getManufacturer() {
return Optional.ofNullable(manufacturer);
}
/**
* Returns an immutable copy of {@code this} with just the {@code manufacturer}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The user experience analytics manufacturer.”
*
* @param manufacturer
* new value of {@code manufacturer} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code manufacturer} field changed
*/
public UserExperienceAnalyticsRemoteConnection withManufacturer(String manufacturer) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("manufacturer");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.manufacturer = manufacturer;
return _x;
}
/**
* “The user experience analytics device model.”
*
* @return property model
*/
@Property(name="model")
@JsonIgnore
public Optional getModel() {
return Optional.ofNullable(model);
}
/**
* Returns an immutable copy of {@code this} with just the {@code model} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The user experience analytics device model.”
*
* @param model
* new value of {@code model} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code model} field changed
*/
public UserExperienceAnalyticsRemoteConnection withModel(String model) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("model");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.model = model;
return _x;
}
/**
* “The remote sign in time of Cloud PC Device. Valid values 0 to 1.79769313486232E+
* 308”
*
* @return property remoteSignInTime
*/
@Property(name="remoteSignInTime")
@JsonIgnore
public Optional getRemoteSignInTime() {
return Optional.ofNullable(remoteSignInTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code remoteSignInTime}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The remote sign in time of Cloud PC Device. Valid values 0 to 1.79769313486232E+
* 308”
*
* @param remoteSignInTime
* new value of {@code remoteSignInTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code remoteSignInTime} field changed
*/
public UserExperienceAnalyticsRemoteConnection withRemoteSignInTime(Double remoteSignInTime) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("remoteSignInTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.remoteSignInTime = remoteSignInTime;
return _x;
}
/**
* “The user experience analytics userPrincipalName.”
*
* @return property userPrincipalName
*/
@Property(name="userPrincipalName")
@JsonIgnore
public Optional getUserPrincipalName() {
return Optional.ofNullable(userPrincipalName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userPrincipalName
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The user experience analytics userPrincipalName.”
*
* @param userPrincipalName
* new value of {@code userPrincipalName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userPrincipalName} field changed
*/
public UserExperienceAnalyticsRemoteConnection withUserPrincipalName(String userPrincipalName) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("userPrincipalName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.userPrincipalName = userPrincipalName;
return _x;
}
/**
* “The user experience analytics virtual network.”
*
* @return property virtualNetwork
*/
@Property(name="virtualNetwork")
@JsonIgnore
public Optional getVirtualNetwork() {
return Optional.ofNullable(virtualNetwork);
}
/**
* Returns an immutable copy of {@code this} with just the {@code virtualNetwork}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The user experience analytics virtual network.”
*
* @param virtualNetwork
* new value of {@code virtualNetwork} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code virtualNetwork} field changed
*/
public UserExperienceAnalyticsRemoteConnection withVirtualNetwork(String virtualNetwork) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = changedFields.add("virtualNetwork");
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsRemoteConnection");
_x.virtualNetwork = virtualNetwork;
return _x;
}
public UserExperienceAnalyticsRemoteConnection withUnmappedField(String name, String value) {
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public UserExperienceAnalyticsRemoteConnection patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public UserExperienceAnalyticsRemoteConnection put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
UserExperienceAnalyticsRemoteConnection _x = _copy();
_x.changedFields = null;
return _x;
}
private UserExperienceAnalyticsRemoteConnection _copy() {
UserExperienceAnalyticsRemoteConnection _x = new UserExperienceAnalyticsRemoteConnection();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.cloudPcFailurePercentage = cloudPcFailurePercentage;
_x.cloudPcRoundTripTime = cloudPcRoundTripTime;
_x.cloudPcSignInTime = cloudPcSignInTime;
_x.coreBootTime = coreBootTime;
_x.coreSignInTime = coreSignInTime;
_x.deviceCount = deviceCount;
_x.deviceId = deviceId;
_x.deviceName = deviceName;
_x.manufacturer = manufacturer;
_x.model = model;
_x.remoteSignInTime = remoteSignInTime;
_x.userPrincipalName = userPrincipalName;
_x.virtualNetwork = virtualNetwork;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("UserExperienceAnalyticsRemoteConnection[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("cloudPcFailurePercentage=");
b.append(this.cloudPcFailurePercentage);
b.append(", ");
b.append("cloudPcRoundTripTime=");
b.append(this.cloudPcRoundTripTime);
b.append(", ");
b.append("cloudPcSignInTime=");
b.append(this.cloudPcSignInTime);
b.append(", ");
b.append("coreBootTime=");
b.append(this.coreBootTime);
b.append(", ");
b.append("coreSignInTime=");
b.append(this.coreSignInTime);
b.append(", ");
b.append("deviceCount=");
b.append(this.deviceCount);
b.append(", ");
b.append("deviceId=");
b.append(this.deviceId);
b.append(", ");
b.append("deviceName=");
b.append(this.deviceName);
b.append(", ");
b.append("manufacturer=");
b.append(this.manufacturer);
b.append(", ");
b.append("model=");
b.append(this.model);
b.append(", ");
b.append("remoteSignInTime=");
b.append(this.remoteSignInTime);
b.append(", ");
b.append("userPrincipalName=");
b.append(this.userPrincipalName);
b.append(", ");
b.append("virtualNetwork=");
b.append(this.virtualNetwork);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}