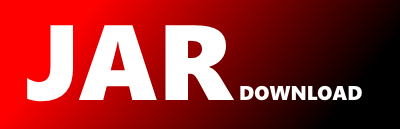
odata.msgraph.client.beta.entity.WindowsKioskConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleDeviceMode;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsEdition;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsVersion;
import odata.msgraph.client.beta.complex.WindowsKioskForceUpdateSchedule;
import odata.msgraph.client.beta.complex.WindowsKioskProfile;
/**
* “This entity provides descriptions of the declared methods, properties and
* relationships exposed by the kiosk resource.”
*/@JsonPropertyOrder({
"@odata.type",
"edgeKioskEnablePublicBrowsing",
"kioskBrowserBlockedUrlExceptions",
"kioskBrowserBlockedURLs",
"kioskBrowserDefaultUrl",
"kioskBrowserEnableEndSessionButton",
"kioskBrowserEnableHomeButton",
"kioskBrowserEnableNavigationButtons",
"kioskBrowserRestartOnIdleTimeInMinutes",
"kioskProfiles",
"windowsKioskForceUpdateSchedule"})
@JsonInclude(Include.NON_NULL)
public class WindowsKioskConfiguration extends DeviceConfiguration implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.windowsKioskConfiguration";
}
@JsonProperty("edgeKioskEnablePublicBrowsing")
protected Boolean edgeKioskEnablePublicBrowsing;
@JsonProperty("kioskBrowserBlockedUrlExceptions")
protected List kioskBrowserBlockedUrlExceptions;
@JsonProperty("kioskBrowserBlockedUrlExceptions@nextLink")
protected String kioskBrowserBlockedUrlExceptionsNextLink;
@JsonProperty("kioskBrowserBlockedURLs")
protected List kioskBrowserBlockedURLs;
@JsonProperty("kioskBrowserBlockedURLs@nextLink")
protected String kioskBrowserBlockedURLsNextLink;
@JsonProperty("kioskBrowserDefaultUrl")
protected String kioskBrowserDefaultUrl;
@JsonProperty("kioskBrowserEnableEndSessionButton")
protected Boolean kioskBrowserEnableEndSessionButton;
@JsonProperty("kioskBrowserEnableHomeButton")
protected Boolean kioskBrowserEnableHomeButton;
@JsonProperty("kioskBrowserEnableNavigationButtons")
protected Boolean kioskBrowserEnableNavigationButtons;
@JsonProperty("kioskBrowserRestartOnIdleTimeInMinutes")
protected Integer kioskBrowserRestartOnIdleTimeInMinutes;
@JsonProperty("kioskProfiles")
protected List kioskProfiles;
@JsonProperty("kioskProfiles@nextLink")
protected String kioskProfilesNextLink;
@JsonProperty("windowsKioskForceUpdateSchedule")
protected WindowsKioskForceUpdateSchedule windowsKioskForceUpdateSchedule;
protected WindowsKioskConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderWindowsKioskConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode;
private DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition;
private DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private Boolean supportsScopeTags;
private Integer version;
private Boolean edgeKioskEnablePublicBrowsing;
private List kioskBrowserBlockedUrlExceptions;
private String kioskBrowserBlockedUrlExceptionsNextLink;
private List kioskBrowserBlockedURLs;
private String kioskBrowserBlockedURLsNextLink;
private String kioskBrowserDefaultUrl;
private Boolean kioskBrowserEnableEndSessionButton;
private Boolean kioskBrowserEnableHomeButton;
private Boolean kioskBrowserEnableNavigationButtons;
private Integer kioskBrowserRestartOnIdleTimeInMinutes;
private List kioskProfiles;
private String kioskProfilesNextLink;
private WindowsKioskForceUpdateSchedule windowsKioskForceUpdateSchedule;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder deviceManagementApplicabilityRuleDeviceMode(DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode) {
this.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleDeviceMode");
return this;
}
public Builder deviceManagementApplicabilityRuleOsEdition(DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition) {
this.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsEdition");
return this;
}
public Builder deviceManagementApplicabilityRuleOsVersion(DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion) {
this.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsVersion");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder supportsScopeTags(Boolean supportsScopeTags) {
this.supportsScopeTags = supportsScopeTags;
this.changedFields = changedFields.add("supportsScopeTags");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
/**
* “Enable public browsing kiosk mode for the Microsoft Edge browser. The Default is
* false.”
*
* @param edgeKioskEnablePublicBrowsing
* value of {@code edgeKioskEnablePublicBrowsing} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder edgeKioskEnablePublicBrowsing(Boolean edgeKioskEnablePublicBrowsing) {
this.edgeKioskEnablePublicBrowsing = edgeKioskEnablePublicBrowsing;
this.changedFields = changedFields.add("edgeKioskEnablePublicBrowsing");
return this;
}
/**
* “Specify URLs that the kiosk browser is allowed to navigate to”
*
* @param kioskBrowserBlockedUrlExceptions
* value of {@code kioskBrowserBlockedUrlExceptions} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskBrowserBlockedUrlExceptions(List kioskBrowserBlockedUrlExceptions) {
this.kioskBrowserBlockedUrlExceptions = kioskBrowserBlockedUrlExceptions;
this.changedFields = changedFields.add("kioskBrowserBlockedUrlExceptions");
return this;
}
/**
* “Specify URLs that the kiosk browser is allowed to navigate to”
*
* @param kioskBrowserBlockedUrlExceptions
* value of {@code kioskBrowserBlockedUrlExceptions} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskBrowserBlockedUrlExceptions(String... kioskBrowserBlockedUrlExceptions) {
return kioskBrowserBlockedUrlExceptions(Arrays.asList(kioskBrowserBlockedUrlExceptions));
}
/**
* “Specify URLs that the kiosk browser is allowed to navigate to”
*
* @param kioskBrowserBlockedUrlExceptionsNextLink
* value of {@code kioskBrowserBlockedUrlExceptions@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskBrowserBlockedUrlExceptionsNextLink(String kioskBrowserBlockedUrlExceptionsNextLink) {
this.kioskBrowserBlockedUrlExceptionsNextLink = kioskBrowserBlockedUrlExceptionsNextLink;
this.changedFields = changedFields.add("kioskBrowserBlockedUrlExceptions");
return this;
}
/**
* “Specify URLs that the kiosk browsers should not navigate to”
*
* @param kioskBrowserBlockedURLs
* value of {@code kioskBrowserBlockedURLs} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskBrowserBlockedURLs(List kioskBrowserBlockedURLs) {
this.kioskBrowserBlockedURLs = kioskBrowserBlockedURLs;
this.changedFields = changedFields.add("kioskBrowserBlockedURLs");
return this;
}
/**
* “Specify URLs that the kiosk browsers should not navigate to”
*
* @param kioskBrowserBlockedURLs
* value of {@code kioskBrowserBlockedURLs} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskBrowserBlockedURLs(String... kioskBrowserBlockedURLs) {
return kioskBrowserBlockedURLs(Arrays.asList(kioskBrowserBlockedURLs));
}
/**
* “Specify URLs that the kiosk browsers should not navigate to”
*
* @param kioskBrowserBlockedURLsNextLink
* value of {@code kioskBrowserBlockedURLs@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskBrowserBlockedURLsNextLink(String kioskBrowserBlockedURLsNextLink) {
this.kioskBrowserBlockedURLsNextLink = kioskBrowserBlockedURLsNextLink;
this.changedFields = changedFields.add("kioskBrowserBlockedURLs");
return this;
}
/**
* “Specify the default URL the browser should navigate to on launch.”
*
* @param kioskBrowserDefaultUrl
* value of {@code kioskBrowserDefaultUrl} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskBrowserDefaultUrl(String kioskBrowserDefaultUrl) {
this.kioskBrowserDefaultUrl = kioskBrowserDefaultUrl;
this.changedFields = changedFields.add("kioskBrowserDefaultUrl");
return this;
}
/**
* “Enable the kiosk browser's end session button. By default, the end session
* button is disabled.”
*
* @param kioskBrowserEnableEndSessionButton
* value of {@code kioskBrowserEnableEndSessionButton} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskBrowserEnableEndSessionButton(Boolean kioskBrowserEnableEndSessionButton) {
this.kioskBrowserEnableEndSessionButton = kioskBrowserEnableEndSessionButton;
this.changedFields = changedFields.add("kioskBrowserEnableEndSessionButton");
return this;
}
/**
* “Enable the kiosk browser's home button. By default, the home button is disabled.”
*
* @param kioskBrowserEnableHomeButton
* value of {@code kioskBrowserEnableHomeButton} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskBrowserEnableHomeButton(Boolean kioskBrowserEnableHomeButton) {
this.kioskBrowserEnableHomeButton = kioskBrowserEnableHomeButton;
this.changedFields = changedFields.add("kioskBrowserEnableHomeButton");
return this;
}
/**
* “Enable the kiosk browser's navigation buttons(forward/back). By default, the
* navigation buttons are disabled.”
*
* @param kioskBrowserEnableNavigationButtons
* value of {@code kioskBrowserEnableNavigationButtons} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskBrowserEnableNavigationButtons(Boolean kioskBrowserEnableNavigationButtons) {
this.kioskBrowserEnableNavigationButtons = kioskBrowserEnableNavigationButtons;
this.changedFields = changedFields.add("kioskBrowserEnableNavigationButtons");
return this;
}
/**
* “Specify the number of minutes the session is idle until the kiosk browser
* restarts in a fresh state. Valid values are 1-1440. Valid values 1 to 1440”
*
* @param kioskBrowserRestartOnIdleTimeInMinutes
* value of {@code kioskBrowserRestartOnIdleTimeInMinutes} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskBrowserRestartOnIdleTimeInMinutes(Integer kioskBrowserRestartOnIdleTimeInMinutes) {
this.kioskBrowserRestartOnIdleTimeInMinutes = kioskBrowserRestartOnIdleTimeInMinutes;
this.changedFields = changedFields.add("kioskBrowserRestartOnIdleTimeInMinutes");
return this;
}
/**
* “This policy setting allows to define a list of Kiosk profiles for a Kiosk
* configuration. This collection can contain a maximum of 3 elements.”
*
* @param kioskProfiles
* value of {@code kioskProfiles} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskProfiles(List kioskProfiles) {
this.kioskProfiles = kioskProfiles;
this.changedFields = changedFields.add("kioskProfiles");
return this;
}
/**
* “This policy setting allows to define a list of Kiosk profiles for a Kiosk
* configuration. This collection can contain a maximum of 3 elements.”
*
* @param kioskProfiles
* value of {@code kioskProfiles} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskProfiles(WindowsKioskProfile... kioskProfiles) {
return kioskProfiles(Arrays.asList(kioskProfiles));
}
/**
* “This policy setting allows to define a list of Kiosk profiles for a Kiosk
* configuration. This collection can contain a maximum of 3 elements.”
*
* @param kioskProfilesNextLink
* value of {@code kioskProfiles@nextLink} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder kioskProfilesNextLink(String kioskProfilesNextLink) {
this.kioskProfilesNextLink = kioskProfilesNextLink;
this.changedFields = changedFields.add("kioskProfiles");
return this;
}
/**
* “force update schedule for Kiosk devices.”
*
* @param windowsKioskForceUpdateSchedule
* value of {@code windowsKioskForceUpdateSchedule} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder windowsKioskForceUpdateSchedule(WindowsKioskForceUpdateSchedule windowsKioskForceUpdateSchedule) {
this.windowsKioskForceUpdateSchedule = windowsKioskForceUpdateSchedule;
this.changedFields = changedFields.add("windowsKioskForceUpdateSchedule");
return this;
}
public WindowsKioskConfiguration build() {
WindowsKioskConfiguration _x = new WindowsKioskConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.windowsKioskConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.edgeKioskEnablePublicBrowsing = edgeKioskEnablePublicBrowsing;
_x.kioskBrowserBlockedUrlExceptions = kioskBrowserBlockedUrlExceptions;
_x.kioskBrowserBlockedUrlExceptionsNextLink = kioskBrowserBlockedUrlExceptionsNextLink;
_x.kioskBrowserBlockedURLs = kioskBrowserBlockedURLs;
_x.kioskBrowserBlockedURLsNextLink = kioskBrowserBlockedURLsNextLink;
_x.kioskBrowserDefaultUrl = kioskBrowserDefaultUrl;
_x.kioskBrowserEnableEndSessionButton = kioskBrowserEnableEndSessionButton;
_x.kioskBrowserEnableHomeButton = kioskBrowserEnableHomeButton;
_x.kioskBrowserEnableNavigationButtons = kioskBrowserEnableNavigationButtons;
_x.kioskBrowserRestartOnIdleTimeInMinutes = kioskBrowserRestartOnIdleTimeInMinutes;
_x.kioskProfiles = kioskProfiles;
_x.kioskProfilesNextLink = kioskProfilesNextLink;
_x.windowsKioskForceUpdateSchedule = windowsKioskForceUpdateSchedule;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Enable public browsing kiosk mode for the Microsoft Edge browser. The Default is
* false.”
*
* @return property edgeKioskEnablePublicBrowsing
*/
@Property(name="edgeKioskEnablePublicBrowsing")
@JsonIgnore
public Optional getEdgeKioskEnablePublicBrowsing() {
return Optional.ofNullable(edgeKioskEnablePublicBrowsing);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* edgeKioskEnablePublicBrowsing} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Enable public browsing kiosk mode for the Microsoft Edge browser. The Default is
* false.”
*
* @param edgeKioskEnablePublicBrowsing
* new value of {@code edgeKioskEnablePublicBrowsing} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code edgeKioskEnablePublicBrowsing} field changed
*/
public WindowsKioskConfiguration withEdgeKioskEnablePublicBrowsing(Boolean edgeKioskEnablePublicBrowsing) {
WindowsKioskConfiguration _x = _copy();
_x.changedFields = changedFields.add("edgeKioskEnablePublicBrowsing");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsKioskConfiguration");
_x.edgeKioskEnablePublicBrowsing = edgeKioskEnablePublicBrowsing;
return _x;
}
/**
* “Specify URLs that the kiosk browser is allowed to navigate to”
*
* @return property kioskBrowserBlockedUrlExceptions
*/
@Property(name="kioskBrowserBlockedUrlExceptions")
@JsonIgnore
public CollectionPage getKioskBrowserBlockedUrlExceptions() {
return new CollectionPage(contextPath, String.class, this.kioskBrowserBlockedUrlExceptions, Optional.ofNullable(kioskBrowserBlockedUrlExceptionsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* kioskBrowserBlockedUrlExceptions} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Specify URLs that the kiosk browser is allowed to navigate to”
*
* @param kioskBrowserBlockedUrlExceptions
* new value of {@code kioskBrowserBlockedUrlExceptions} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code kioskBrowserBlockedUrlExceptions} field changed
*/
public WindowsKioskConfiguration withKioskBrowserBlockedUrlExceptions(List kioskBrowserBlockedUrlExceptions) {
WindowsKioskConfiguration _x = _copy();
_x.changedFields = changedFields.add("kioskBrowserBlockedUrlExceptions");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsKioskConfiguration");
_x.kioskBrowserBlockedUrlExceptions = kioskBrowserBlockedUrlExceptions;
return _x;
}
/**
* “Specify URLs that the kiosk browser is allowed to navigate to”
*
* @param options
* specify connect and read timeouts
* @return property kioskBrowserBlockedUrlExceptions
*/
@Property(name="kioskBrowserBlockedUrlExceptions")
@JsonIgnore
public CollectionPage getKioskBrowserBlockedUrlExceptions(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.kioskBrowserBlockedUrlExceptions, Optional.ofNullable(kioskBrowserBlockedUrlExceptionsNextLink), Collections.emptyList(), options);
}
/**
* “Specify URLs that the kiosk browsers should not navigate to”
*
* @return property kioskBrowserBlockedURLs
*/
@Property(name="kioskBrowserBlockedURLs")
@JsonIgnore
public CollectionPage getKioskBrowserBlockedURLs() {
return new CollectionPage(contextPath, String.class, this.kioskBrowserBlockedURLs, Optional.ofNullable(kioskBrowserBlockedURLsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* kioskBrowserBlockedURLs} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Specify URLs that the kiosk browsers should not navigate to”
*
* @param kioskBrowserBlockedURLs
* new value of {@code kioskBrowserBlockedURLs} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code kioskBrowserBlockedURLs} field changed
*/
public WindowsKioskConfiguration withKioskBrowserBlockedURLs(List kioskBrowserBlockedURLs) {
WindowsKioskConfiguration _x = _copy();
_x.changedFields = changedFields.add("kioskBrowserBlockedURLs");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsKioskConfiguration");
_x.kioskBrowserBlockedURLs = kioskBrowserBlockedURLs;
return _x;
}
/**
* “Specify URLs that the kiosk browsers should not navigate to”
*
* @param options
* specify connect and read timeouts
* @return property kioskBrowserBlockedURLs
*/
@Property(name="kioskBrowserBlockedURLs")
@JsonIgnore
public CollectionPage getKioskBrowserBlockedURLs(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.kioskBrowserBlockedURLs, Optional.ofNullable(kioskBrowserBlockedURLsNextLink), Collections.emptyList(), options);
}
/**
* “Specify the default URL the browser should navigate to on launch.”
*
* @return property kioskBrowserDefaultUrl
*/
@Property(name="kioskBrowserDefaultUrl")
@JsonIgnore
public Optional getKioskBrowserDefaultUrl() {
return Optional.ofNullable(kioskBrowserDefaultUrl);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* kioskBrowserDefaultUrl} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Specify the default URL the browser should navigate to on launch.”
*
* @param kioskBrowserDefaultUrl
* new value of {@code kioskBrowserDefaultUrl} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code kioskBrowserDefaultUrl} field changed
*/
public WindowsKioskConfiguration withKioskBrowserDefaultUrl(String kioskBrowserDefaultUrl) {
WindowsKioskConfiguration _x = _copy();
_x.changedFields = changedFields.add("kioskBrowserDefaultUrl");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsKioskConfiguration");
_x.kioskBrowserDefaultUrl = kioskBrowserDefaultUrl;
return _x;
}
/**
* “Enable the kiosk browser's end session button. By default, the end session
* button is disabled.”
*
* @return property kioskBrowserEnableEndSessionButton
*/
@Property(name="kioskBrowserEnableEndSessionButton")
@JsonIgnore
public Optional getKioskBrowserEnableEndSessionButton() {
return Optional.ofNullable(kioskBrowserEnableEndSessionButton);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* kioskBrowserEnableEndSessionButton} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Enable the kiosk browser's end session button. By default, the end session
* button is disabled.”
*
* @param kioskBrowserEnableEndSessionButton
* new value of {@code kioskBrowserEnableEndSessionButton} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code kioskBrowserEnableEndSessionButton} field changed
*/
public WindowsKioskConfiguration withKioskBrowserEnableEndSessionButton(Boolean kioskBrowserEnableEndSessionButton) {
WindowsKioskConfiguration _x = _copy();
_x.changedFields = changedFields.add("kioskBrowserEnableEndSessionButton");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsKioskConfiguration");
_x.kioskBrowserEnableEndSessionButton = kioskBrowserEnableEndSessionButton;
return _x;
}
/**
* “Enable the kiosk browser's home button. By default, the home button is disabled.”
*
* @return property kioskBrowserEnableHomeButton
*/
@Property(name="kioskBrowserEnableHomeButton")
@JsonIgnore
public Optional getKioskBrowserEnableHomeButton() {
return Optional.ofNullable(kioskBrowserEnableHomeButton);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* kioskBrowserEnableHomeButton} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Enable the kiosk browser's home button. By default, the home button is disabled.”
*
* @param kioskBrowserEnableHomeButton
* new value of {@code kioskBrowserEnableHomeButton} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code kioskBrowserEnableHomeButton} field changed
*/
public WindowsKioskConfiguration withKioskBrowserEnableHomeButton(Boolean kioskBrowserEnableHomeButton) {
WindowsKioskConfiguration _x = _copy();
_x.changedFields = changedFields.add("kioskBrowserEnableHomeButton");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsKioskConfiguration");
_x.kioskBrowserEnableHomeButton = kioskBrowserEnableHomeButton;
return _x;
}
/**
* “Enable the kiosk browser's navigation buttons(forward/back). By default, the
* navigation buttons are disabled.”
*
* @return property kioskBrowserEnableNavigationButtons
*/
@Property(name="kioskBrowserEnableNavigationButtons")
@JsonIgnore
public Optional getKioskBrowserEnableNavigationButtons() {
return Optional.ofNullable(kioskBrowserEnableNavigationButtons);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* kioskBrowserEnableNavigationButtons} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Enable the kiosk browser's navigation buttons(forward/back). By default, the
* navigation buttons are disabled.”
*
* @param kioskBrowserEnableNavigationButtons
* new value of {@code kioskBrowserEnableNavigationButtons} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code kioskBrowserEnableNavigationButtons} field changed
*/
public WindowsKioskConfiguration withKioskBrowserEnableNavigationButtons(Boolean kioskBrowserEnableNavigationButtons) {
WindowsKioskConfiguration _x = _copy();
_x.changedFields = changedFields.add("kioskBrowserEnableNavigationButtons");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsKioskConfiguration");
_x.kioskBrowserEnableNavigationButtons = kioskBrowserEnableNavigationButtons;
return _x;
}
/**
* “Specify the number of minutes the session is idle until the kiosk browser
* restarts in a fresh state. Valid values are 1-1440. Valid values 1 to 1440”
*
* @return property kioskBrowserRestartOnIdleTimeInMinutes
*/
@Property(name="kioskBrowserRestartOnIdleTimeInMinutes")
@JsonIgnore
public Optional getKioskBrowserRestartOnIdleTimeInMinutes() {
return Optional.ofNullable(kioskBrowserRestartOnIdleTimeInMinutes);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* kioskBrowserRestartOnIdleTimeInMinutes} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Specify the number of minutes the session is idle until the kiosk browser
* restarts in a fresh state. Valid values are 1-1440. Valid values 1 to 1440”
*
* @param kioskBrowserRestartOnIdleTimeInMinutes
* new value of {@code kioskBrowserRestartOnIdleTimeInMinutes} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code kioskBrowserRestartOnIdleTimeInMinutes} field changed
*/
public WindowsKioskConfiguration withKioskBrowserRestartOnIdleTimeInMinutes(Integer kioskBrowserRestartOnIdleTimeInMinutes) {
WindowsKioskConfiguration _x = _copy();
_x.changedFields = changedFields.add("kioskBrowserRestartOnIdleTimeInMinutes");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsKioskConfiguration");
_x.kioskBrowserRestartOnIdleTimeInMinutes = kioskBrowserRestartOnIdleTimeInMinutes;
return _x;
}
/**
* “This policy setting allows to define a list of Kiosk profiles for a Kiosk
* configuration. This collection can contain a maximum of 3 elements.”
*
* @return property kioskProfiles
*/
@Property(name="kioskProfiles")
@JsonIgnore
public CollectionPage getKioskProfiles() {
return new CollectionPage(contextPath, WindowsKioskProfile.class, this.kioskProfiles, Optional.ofNullable(kioskProfilesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code kioskProfiles}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “This policy setting allows to define a list of Kiosk profiles for a Kiosk
* configuration. This collection can contain a maximum of 3 elements.”
*
* @param kioskProfiles
* new value of {@code kioskProfiles} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code kioskProfiles} field changed
*/
public WindowsKioskConfiguration withKioskProfiles(List kioskProfiles) {
WindowsKioskConfiguration _x = _copy();
_x.changedFields = changedFields.add("kioskProfiles");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsKioskConfiguration");
_x.kioskProfiles = kioskProfiles;
return _x;
}
/**
* “This policy setting allows to define a list of Kiosk profiles for a Kiosk
* configuration. This collection can contain a maximum of 3 elements.”
*
* @param options
* specify connect and read timeouts
* @return property kioskProfiles
*/
@Property(name="kioskProfiles")
@JsonIgnore
public CollectionPage getKioskProfiles(HttpRequestOptions options) {
return new CollectionPage(contextPath, WindowsKioskProfile.class, this.kioskProfiles, Optional.ofNullable(kioskProfilesNextLink), Collections.emptyList(), options);
}
/**
* “force update schedule for Kiosk devices.”
*
* @return property windowsKioskForceUpdateSchedule
*/
@Property(name="windowsKioskForceUpdateSchedule")
@JsonIgnore
public Optional getWindowsKioskForceUpdateSchedule() {
return Optional.ofNullable(windowsKioskForceUpdateSchedule);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* windowsKioskForceUpdateSchedule} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “force update schedule for Kiosk devices.”
*
* @param windowsKioskForceUpdateSchedule
* new value of {@code windowsKioskForceUpdateSchedule} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code windowsKioskForceUpdateSchedule} field changed
*/
public WindowsKioskConfiguration withWindowsKioskForceUpdateSchedule(WindowsKioskForceUpdateSchedule windowsKioskForceUpdateSchedule) {
WindowsKioskConfiguration _x = _copy();
_x.changedFields = changedFields.add("windowsKioskForceUpdateSchedule");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsKioskConfiguration");
_x.windowsKioskForceUpdateSchedule = windowsKioskForceUpdateSchedule;
return _x;
}
public WindowsKioskConfiguration withUnmappedField(String name, String value) {
WindowsKioskConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WindowsKioskConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
WindowsKioskConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WindowsKioskConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
WindowsKioskConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private WindowsKioskConfiguration _copy() {
WindowsKioskConfiguration _x = new WindowsKioskConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.edgeKioskEnablePublicBrowsing = edgeKioskEnablePublicBrowsing;
_x.kioskBrowserBlockedUrlExceptions = kioskBrowserBlockedUrlExceptions;
_x.kioskBrowserBlockedURLs = kioskBrowserBlockedURLs;
_x.kioskBrowserDefaultUrl = kioskBrowserDefaultUrl;
_x.kioskBrowserEnableEndSessionButton = kioskBrowserEnableEndSessionButton;
_x.kioskBrowserEnableHomeButton = kioskBrowserEnableHomeButton;
_x.kioskBrowserEnableNavigationButtons = kioskBrowserEnableNavigationButtons;
_x.kioskBrowserRestartOnIdleTimeInMinutes = kioskBrowserRestartOnIdleTimeInMinutes;
_x.kioskProfiles = kioskProfiles;
_x.windowsKioskForceUpdateSchedule = windowsKioskForceUpdateSchedule;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("WindowsKioskConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("deviceManagementApplicabilityRuleDeviceMode=");
b.append(this.deviceManagementApplicabilityRuleDeviceMode);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsEdition=");
b.append(this.deviceManagementApplicabilityRuleOsEdition);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsVersion=");
b.append(this.deviceManagementApplicabilityRuleOsVersion);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("supportsScopeTags=");
b.append(this.supportsScopeTags);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("edgeKioskEnablePublicBrowsing=");
b.append(this.edgeKioskEnablePublicBrowsing);
b.append(", ");
b.append("kioskBrowserBlockedUrlExceptions=");
b.append(this.kioskBrowserBlockedUrlExceptions);
b.append(", ");
b.append("kioskBrowserBlockedURLs=");
b.append(this.kioskBrowserBlockedURLs);
b.append(", ");
b.append("kioskBrowserDefaultUrl=");
b.append(this.kioskBrowserDefaultUrl);
b.append(", ");
b.append("kioskBrowserEnableEndSessionButton=");
b.append(this.kioskBrowserEnableEndSessionButton);
b.append(", ");
b.append("kioskBrowserEnableHomeButton=");
b.append(this.kioskBrowserEnableHomeButton);
b.append(", ");
b.append("kioskBrowserEnableNavigationButtons=");
b.append(this.kioskBrowserEnableNavigationButtons);
b.append(", ");
b.append("kioskBrowserRestartOnIdleTimeInMinutes=");
b.append(this.kioskBrowserRestartOnIdleTimeInMinutes);
b.append(", ");
b.append("kioskProfiles=");
b.append(this.kioskProfiles);
b.append(", ");
b.append("windowsKioskForceUpdateSchedule=");
b.append(this.windowsKioskForceUpdateSchedule);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}