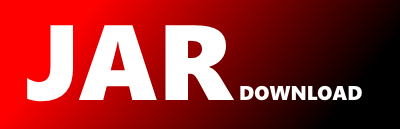
odata.msgraph.client.beta.entity.WindowsUpdateForBusinessConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.LocalDate;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleDeviceMode;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsEdition;
import odata.msgraph.client.beta.complex.DeviceManagementApplicabilityRuleOsVersion;
import odata.msgraph.client.beta.complex.WindowsUpdateInstallScheduleType;
import odata.msgraph.client.beta.entity.collection.request.WindowsUpdateStateCollectionRequest;
import odata.msgraph.client.beta.enums.AutoRestartNotificationDismissalMethod;
import odata.msgraph.client.beta.enums.AutomaticUpdateMode;
import odata.msgraph.client.beta.enums.Enablement;
import odata.msgraph.client.beta.enums.PrereleaseFeatures;
import odata.msgraph.client.beta.enums.WindowsDeliveryOptimizationMode;
import odata.msgraph.client.beta.enums.WindowsUpdateForBusinessUpdateWeeks;
import odata.msgraph.client.beta.enums.WindowsUpdateNotificationDisplayOption;
import odata.msgraph.client.beta.enums.WindowsUpdateType;
/**
* “Windows Update for business configuration.”
*/@JsonPropertyOrder({
"@odata.type",
"automaticUpdateMode",
"autoRestartNotificationDismissal",
"businessReadyUpdatesOnly",
"deadlineForFeatureUpdatesInDays",
"deadlineForQualityUpdatesInDays",
"deadlineGracePeriodInDays",
"deliveryOptimizationMode",
"driversExcluded",
"engagedRestartDeadlineInDays",
"engagedRestartSnoozeScheduleInDays",
"engagedRestartTransitionScheduleInDays",
"featureUpdatesDeferralPeriodInDays",
"featureUpdatesPaused",
"featureUpdatesPauseExpiryDateTime",
"featureUpdatesPauseStartDate",
"featureUpdatesRollbackStartDateTime",
"featureUpdatesRollbackWindowInDays",
"featureUpdatesWillBeRolledBack",
"installationSchedule",
"microsoftUpdateServiceAllowed",
"postponeRebootUntilAfterDeadline",
"prereleaseFeatures",
"qualityUpdatesDeferralPeriodInDays",
"qualityUpdatesPaused",
"qualityUpdatesPauseExpiryDateTime",
"qualityUpdatesPauseStartDate",
"qualityUpdatesRollbackStartDateTime",
"qualityUpdatesWillBeRolledBack",
"scheduleImminentRestartWarningInMinutes",
"scheduleRestartWarningInHours",
"skipChecksBeforeRestart",
"updateNotificationLevel",
"updateWeeks",
"userPauseAccess",
"userWindowsUpdateScanAccess"})
@JsonInclude(Include.NON_NULL)
public class WindowsUpdateForBusinessConfiguration extends DeviceConfiguration implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.windowsUpdateForBusinessConfiguration";
}
@JsonProperty("automaticUpdateMode")
protected AutomaticUpdateMode automaticUpdateMode;
@JsonProperty("autoRestartNotificationDismissal")
protected AutoRestartNotificationDismissalMethod autoRestartNotificationDismissal;
@JsonProperty("businessReadyUpdatesOnly")
protected WindowsUpdateType businessReadyUpdatesOnly;
@JsonProperty("deadlineForFeatureUpdatesInDays")
protected Integer deadlineForFeatureUpdatesInDays;
@JsonProperty("deadlineForQualityUpdatesInDays")
protected Integer deadlineForQualityUpdatesInDays;
@JsonProperty("deadlineGracePeriodInDays")
protected Integer deadlineGracePeriodInDays;
@JsonProperty("deliveryOptimizationMode")
protected WindowsDeliveryOptimizationMode deliveryOptimizationMode;
@JsonProperty("driversExcluded")
protected Boolean driversExcluded;
@JsonProperty("engagedRestartDeadlineInDays")
protected Integer engagedRestartDeadlineInDays;
@JsonProperty("engagedRestartSnoozeScheduleInDays")
protected Integer engagedRestartSnoozeScheduleInDays;
@JsonProperty("engagedRestartTransitionScheduleInDays")
protected Integer engagedRestartTransitionScheduleInDays;
@JsonProperty("featureUpdatesDeferralPeriodInDays")
protected Integer featureUpdatesDeferralPeriodInDays;
@JsonProperty("featureUpdatesPaused")
protected Boolean featureUpdatesPaused;
@JsonProperty("featureUpdatesPauseExpiryDateTime")
protected OffsetDateTime featureUpdatesPauseExpiryDateTime;
@JsonProperty("featureUpdatesPauseStartDate")
protected LocalDate featureUpdatesPauseStartDate;
@JsonProperty("featureUpdatesRollbackStartDateTime")
protected OffsetDateTime featureUpdatesRollbackStartDateTime;
@JsonProperty("featureUpdatesRollbackWindowInDays")
protected Integer featureUpdatesRollbackWindowInDays;
@JsonProperty("featureUpdatesWillBeRolledBack")
protected Boolean featureUpdatesWillBeRolledBack;
@JsonProperty("installationSchedule")
protected WindowsUpdateInstallScheduleType installationSchedule;
@JsonProperty("microsoftUpdateServiceAllowed")
protected Boolean microsoftUpdateServiceAllowed;
@JsonProperty("postponeRebootUntilAfterDeadline")
protected Boolean postponeRebootUntilAfterDeadline;
@JsonProperty("prereleaseFeatures")
protected PrereleaseFeatures prereleaseFeatures;
@JsonProperty("qualityUpdatesDeferralPeriodInDays")
protected Integer qualityUpdatesDeferralPeriodInDays;
@JsonProperty("qualityUpdatesPaused")
protected Boolean qualityUpdatesPaused;
@JsonProperty("qualityUpdatesPauseExpiryDateTime")
protected OffsetDateTime qualityUpdatesPauseExpiryDateTime;
@JsonProperty("qualityUpdatesPauseStartDate")
protected LocalDate qualityUpdatesPauseStartDate;
@JsonProperty("qualityUpdatesRollbackStartDateTime")
protected OffsetDateTime qualityUpdatesRollbackStartDateTime;
@JsonProperty("qualityUpdatesWillBeRolledBack")
protected Boolean qualityUpdatesWillBeRolledBack;
@JsonProperty("scheduleImminentRestartWarningInMinutes")
protected Integer scheduleImminentRestartWarningInMinutes;
@JsonProperty("scheduleRestartWarningInHours")
protected Integer scheduleRestartWarningInHours;
@JsonProperty("skipChecksBeforeRestart")
protected Boolean skipChecksBeforeRestart;
@JsonProperty("updateNotificationLevel")
protected WindowsUpdateNotificationDisplayOption updateNotificationLevel;
@JsonProperty("updateWeeks")
protected WindowsUpdateForBusinessUpdateWeeks updateWeeks;
@JsonProperty("userPauseAccess")
protected Enablement userPauseAccess;
@JsonProperty("userWindowsUpdateScanAccess")
protected Enablement userWindowsUpdateScanAccess;
protected WindowsUpdateForBusinessConfiguration() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderWindowsUpdateForBusinessConfiguration() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode;
private DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition;
private DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private List roleScopeTagIds;
private String roleScopeTagIdsNextLink;
private Boolean supportsScopeTags;
private Integer version;
private AutomaticUpdateMode automaticUpdateMode;
private AutoRestartNotificationDismissalMethod autoRestartNotificationDismissal;
private WindowsUpdateType businessReadyUpdatesOnly;
private Integer deadlineForFeatureUpdatesInDays;
private Integer deadlineForQualityUpdatesInDays;
private Integer deadlineGracePeriodInDays;
private WindowsDeliveryOptimizationMode deliveryOptimizationMode;
private Boolean driversExcluded;
private Integer engagedRestartDeadlineInDays;
private Integer engagedRestartSnoozeScheduleInDays;
private Integer engagedRestartTransitionScheduleInDays;
private Integer featureUpdatesDeferralPeriodInDays;
private Boolean featureUpdatesPaused;
private OffsetDateTime featureUpdatesPauseExpiryDateTime;
private LocalDate featureUpdatesPauseStartDate;
private OffsetDateTime featureUpdatesRollbackStartDateTime;
private Integer featureUpdatesRollbackWindowInDays;
private Boolean featureUpdatesWillBeRolledBack;
private WindowsUpdateInstallScheduleType installationSchedule;
private Boolean microsoftUpdateServiceAllowed;
private Boolean postponeRebootUntilAfterDeadline;
private PrereleaseFeatures prereleaseFeatures;
private Integer qualityUpdatesDeferralPeriodInDays;
private Boolean qualityUpdatesPaused;
private OffsetDateTime qualityUpdatesPauseExpiryDateTime;
private LocalDate qualityUpdatesPauseStartDate;
private OffsetDateTime qualityUpdatesRollbackStartDateTime;
private Boolean qualityUpdatesWillBeRolledBack;
private Integer scheduleImminentRestartWarningInMinutes;
private Integer scheduleRestartWarningInHours;
private Boolean skipChecksBeforeRestart;
private WindowsUpdateNotificationDisplayOption updateNotificationLevel;
private WindowsUpdateForBusinessUpdateWeeks updateWeeks;
private Enablement userPauseAccess;
private Enablement userWindowsUpdateScanAccess;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder deviceManagementApplicabilityRuleDeviceMode(DeviceManagementApplicabilityRuleDeviceMode deviceManagementApplicabilityRuleDeviceMode) {
this.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleDeviceMode");
return this;
}
public Builder deviceManagementApplicabilityRuleOsEdition(DeviceManagementApplicabilityRuleOsEdition deviceManagementApplicabilityRuleOsEdition) {
this.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsEdition");
return this;
}
public Builder deviceManagementApplicabilityRuleOsVersion(DeviceManagementApplicabilityRuleOsVersion deviceManagementApplicabilityRuleOsVersion) {
this.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
this.changedFields = changedFields.add("deviceManagementApplicabilityRuleOsVersion");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder roleScopeTagIds(List roleScopeTagIds) {
this.roleScopeTagIds = roleScopeTagIds;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder roleScopeTagIds(String... roleScopeTagIds) {
return roleScopeTagIds(Arrays.asList(roleScopeTagIds));
}
public Builder roleScopeTagIdsNextLink(String roleScopeTagIdsNextLink) {
this.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
this.changedFields = changedFields.add("roleScopeTagIds");
return this;
}
public Builder supportsScopeTags(Boolean supportsScopeTags) {
this.supportsScopeTags = supportsScopeTags;
this.changedFields = changedFields.add("supportsScopeTags");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
/**
* “Automatic update mode.”
*
* @param automaticUpdateMode
* value of {@code automaticUpdateMode} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder automaticUpdateMode(AutomaticUpdateMode automaticUpdateMode) {
this.automaticUpdateMode = automaticUpdateMode;
this.changedFields = changedFields.add("automaticUpdateMode");
return this;
}
/**
* “Specify the method by which the auto-restart required notification is dismissed”
*
* @param autoRestartNotificationDismissal
* value of {@code autoRestartNotificationDismissal} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder autoRestartNotificationDismissal(AutoRestartNotificationDismissalMethod autoRestartNotificationDismissal) {
this.autoRestartNotificationDismissal = autoRestartNotificationDismissal;
this.changedFields = changedFields.add("autoRestartNotificationDismissal");
return this;
}
/**
* “Determines which branch devices will receive their updates from”
*
* @param businessReadyUpdatesOnly
* value of {@code businessReadyUpdatesOnly} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder businessReadyUpdatesOnly(WindowsUpdateType businessReadyUpdatesOnly) {
this.businessReadyUpdatesOnly = businessReadyUpdatesOnly;
this.changedFields = changedFields.add("businessReadyUpdatesOnly");
return this;
}
/**
* “Number of days before feature updates are installed automatically with valid
* range from 2 to 30 days”
*
* @param deadlineForFeatureUpdatesInDays
* value of {@code deadlineForFeatureUpdatesInDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deadlineForFeatureUpdatesInDays(Integer deadlineForFeatureUpdatesInDays) {
this.deadlineForFeatureUpdatesInDays = deadlineForFeatureUpdatesInDays;
this.changedFields = changedFields.add("deadlineForFeatureUpdatesInDays");
return this;
}
/**
* “Number of days before quality updates are installed automatically with valid
* range from 2 to 30 days”
*
* @param deadlineForQualityUpdatesInDays
* value of {@code deadlineForQualityUpdatesInDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deadlineForQualityUpdatesInDays(Integer deadlineForQualityUpdatesInDays) {
this.deadlineForQualityUpdatesInDays = deadlineForQualityUpdatesInDays;
this.changedFields = changedFields.add("deadlineForQualityUpdatesInDays");
return this;
}
/**
* “Number of days after deadline until restarts occur automatically with valid
* range from 0 to 7 days”
*
* @param deadlineGracePeriodInDays
* value of {@code deadlineGracePeriodInDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deadlineGracePeriodInDays(Integer deadlineGracePeriodInDays) {
this.deadlineGracePeriodInDays = deadlineGracePeriodInDays;
this.changedFields = changedFields.add("deadlineGracePeriodInDays");
return this;
}
/**
* “Delivery Optimization Mode”
*
* @param deliveryOptimizationMode
* value of {@code deliveryOptimizationMode} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deliveryOptimizationMode(WindowsDeliveryOptimizationMode deliveryOptimizationMode) {
this.deliveryOptimizationMode = deliveryOptimizationMode;
this.changedFields = changedFields.add("deliveryOptimizationMode");
return this;
}
/**
* “Exclude Windows update Drivers”
*
* @param driversExcluded
* value of {@code driversExcluded} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder driversExcluded(Boolean driversExcluded) {
this.driversExcluded = driversExcluded;
this.changedFields = changedFields.add("driversExcluded");
return this;
}
/**
* “Deadline in days before automatically scheduling and executing a pending restart
* outside of active hours, with valid range from 2 to 30 days”
*
* @param engagedRestartDeadlineInDays
* value of {@code engagedRestartDeadlineInDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder engagedRestartDeadlineInDays(Integer engagedRestartDeadlineInDays) {
this.engagedRestartDeadlineInDays = engagedRestartDeadlineInDays;
this.changedFields = changedFields.add("engagedRestartDeadlineInDays");
return this;
}
/**
* “Number of days a user can snooze Engaged Restart reminder notifications with
* valid range from 1 to 3 days”
*
* @param engagedRestartSnoozeScheduleInDays
* value of {@code engagedRestartSnoozeScheduleInDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder engagedRestartSnoozeScheduleInDays(Integer engagedRestartSnoozeScheduleInDays) {
this.engagedRestartSnoozeScheduleInDays = engagedRestartSnoozeScheduleInDays;
this.changedFields = changedFields.add("engagedRestartSnoozeScheduleInDays");
return this;
}
/**
* “Number of days before transitioning from Auto Restarts scheduled outside of
* active hours to Engaged Restart, which requires the user to schedule, with valid
* range from 0 to 30 days”
*
* @param engagedRestartTransitionScheduleInDays
* value of {@code engagedRestartTransitionScheduleInDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder engagedRestartTransitionScheduleInDays(Integer engagedRestartTransitionScheduleInDays) {
this.engagedRestartTransitionScheduleInDays = engagedRestartTransitionScheduleInDays;
this.changedFields = changedFields.add("engagedRestartTransitionScheduleInDays");
return this;
}
/**
* “Defer Feature Updates by these many days”
*
* @param featureUpdatesDeferralPeriodInDays
* value of {@code featureUpdatesDeferralPeriodInDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder featureUpdatesDeferralPeriodInDays(Integer featureUpdatesDeferralPeriodInDays) {
this.featureUpdatesDeferralPeriodInDays = featureUpdatesDeferralPeriodInDays;
this.changedFields = changedFields.add("featureUpdatesDeferralPeriodInDays");
return this;
}
/**
* “Pause Feature Updates”
*
* @param featureUpdatesPaused
* value of {@code featureUpdatesPaused} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder featureUpdatesPaused(Boolean featureUpdatesPaused) {
this.featureUpdatesPaused = featureUpdatesPaused;
this.changedFields = changedFields.add("featureUpdatesPaused");
return this;
}
/**
* “Feature Updates Pause Expiry datetime”
*
* @param featureUpdatesPauseExpiryDateTime
* value of {@code featureUpdatesPauseExpiryDateTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder featureUpdatesPauseExpiryDateTime(OffsetDateTime featureUpdatesPauseExpiryDateTime) {
this.featureUpdatesPauseExpiryDateTime = featureUpdatesPauseExpiryDateTime;
this.changedFields = changedFields.add("featureUpdatesPauseExpiryDateTime");
return this;
}
/**
* “Feature Updates Pause start date. This property is read-only.”
*
* Org.OData.Core.V1.Computed
*
* true
*
* Org.OData.Core.V1.Permissions
*
* @param featureUpdatesPauseStartDate
* value of {@code featureUpdatesPauseStartDate} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder featureUpdatesPauseStartDate(LocalDate featureUpdatesPauseStartDate) {
this.featureUpdatesPauseStartDate = featureUpdatesPauseStartDate;
this.changedFields = changedFields.add("featureUpdatesPauseStartDate");
return this;
}
/**
* “Feature Updates Rollback Start datetime”
*
* @param featureUpdatesRollbackStartDateTime
* value of {@code featureUpdatesRollbackStartDateTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder featureUpdatesRollbackStartDateTime(OffsetDateTime featureUpdatesRollbackStartDateTime) {
this.featureUpdatesRollbackStartDateTime = featureUpdatesRollbackStartDateTime;
this.changedFields = changedFields.add("featureUpdatesRollbackStartDateTime");
return this;
}
/**
* “The number of days after a Feature Update for which a rollback is valid”
*
* @param featureUpdatesRollbackWindowInDays
* value of {@code featureUpdatesRollbackWindowInDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder featureUpdatesRollbackWindowInDays(Integer featureUpdatesRollbackWindowInDays) {
this.featureUpdatesRollbackWindowInDays = featureUpdatesRollbackWindowInDays;
this.changedFields = changedFields.add("featureUpdatesRollbackWindowInDays");
return this;
}
/**
* “Specifies whether to rollback Feature Updates on the next device check in”
*
* @param featureUpdatesWillBeRolledBack
* value of {@code featureUpdatesWillBeRolledBack} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder featureUpdatesWillBeRolledBack(Boolean featureUpdatesWillBeRolledBack) {
this.featureUpdatesWillBeRolledBack = featureUpdatesWillBeRolledBack;
this.changedFields = changedFields.add("featureUpdatesWillBeRolledBack");
return this;
}
/**
* “Installation schedule”
*
* @param installationSchedule
* value of {@code installationSchedule} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder installationSchedule(WindowsUpdateInstallScheduleType installationSchedule) {
this.installationSchedule = installationSchedule;
this.changedFields = changedFields.add("installationSchedule");
return this;
}
/**
* “Allow Microsoft Update Service”
*
* @param microsoftUpdateServiceAllowed
* value of {@code microsoftUpdateServiceAllowed} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder microsoftUpdateServiceAllowed(Boolean microsoftUpdateServiceAllowed) {
this.microsoftUpdateServiceAllowed = microsoftUpdateServiceAllowed;
this.changedFields = changedFields.add("microsoftUpdateServiceAllowed");
return this;
}
/**
* “Specifies if the device should wait until deadline for rebooting outside of
* active hours”
*
* @param postponeRebootUntilAfterDeadline
* value of {@code postponeRebootUntilAfterDeadline} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder postponeRebootUntilAfterDeadline(Boolean postponeRebootUntilAfterDeadline) {
this.postponeRebootUntilAfterDeadline = postponeRebootUntilAfterDeadline;
this.changedFields = changedFields.add("postponeRebootUntilAfterDeadline");
return this;
}
/**
* “The pre-release features.”
*
* @param prereleaseFeatures
* value of {@code prereleaseFeatures} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder prereleaseFeatures(PrereleaseFeatures prereleaseFeatures) {
this.prereleaseFeatures = prereleaseFeatures;
this.changedFields = changedFields.add("prereleaseFeatures");
return this;
}
/**
* “Defer Quality Updates by these many days”
*
* @param qualityUpdatesDeferralPeriodInDays
* value of {@code qualityUpdatesDeferralPeriodInDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder qualityUpdatesDeferralPeriodInDays(Integer qualityUpdatesDeferralPeriodInDays) {
this.qualityUpdatesDeferralPeriodInDays = qualityUpdatesDeferralPeriodInDays;
this.changedFields = changedFields.add("qualityUpdatesDeferralPeriodInDays");
return this;
}
/**
* “Pause Quality Updates”
*
* @param qualityUpdatesPaused
* value of {@code qualityUpdatesPaused} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder qualityUpdatesPaused(Boolean qualityUpdatesPaused) {
this.qualityUpdatesPaused = qualityUpdatesPaused;
this.changedFields = changedFields.add("qualityUpdatesPaused");
return this;
}
/**
* “Quality Updates Pause Expiry datetime”
*
* @param qualityUpdatesPauseExpiryDateTime
* value of {@code qualityUpdatesPauseExpiryDateTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder qualityUpdatesPauseExpiryDateTime(OffsetDateTime qualityUpdatesPauseExpiryDateTime) {
this.qualityUpdatesPauseExpiryDateTime = qualityUpdatesPauseExpiryDateTime;
this.changedFields = changedFields.add("qualityUpdatesPauseExpiryDateTime");
return this;
}
/**
* “Quality Updates Pause start date. This property is read-only.”
*
* Org.OData.Core.V1.Computed
*
* true
*
* Org.OData.Core.V1.Permissions
*
* @param qualityUpdatesPauseStartDate
* value of {@code qualityUpdatesPauseStartDate} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder qualityUpdatesPauseStartDate(LocalDate qualityUpdatesPauseStartDate) {
this.qualityUpdatesPauseStartDate = qualityUpdatesPauseStartDate;
this.changedFields = changedFields.add("qualityUpdatesPauseStartDate");
return this;
}
/**
* “Quality Updates Rollback Start datetime”
*
* @param qualityUpdatesRollbackStartDateTime
* value of {@code qualityUpdatesRollbackStartDateTime} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder qualityUpdatesRollbackStartDateTime(OffsetDateTime qualityUpdatesRollbackStartDateTime) {
this.qualityUpdatesRollbackStartDateTime = qualityUpdatesRollbackStartDateTime;
this.changedFields = changedFields.add("qualityUpdatesRollbackStartDateTime");
return this;
}
/**
* “Specifies whether to rollback Quality Updates on the next device check in”
*
* @param qualityUpdatesWillBeRolledBack
* value of {@code qualityUpdatesWillBeRolledBack} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder qualityUpdatesWillBeRolledBack(Boolean qualityUpdatesWillBeRolledBack) {
this.qualityUpdatesWillBeRolledBack = qualityUpdatesWillBeRolledBack;
this.changedFields = changedFields.add("qualityUpdatesWillBeRolledBack");
return this;
}
/**
* “Specify the period for auto-restart imminent warning notifications. Supported
* values: 15, 30 or 60 (minutes).”
*
* @param scheduleImminentRestartWarningInMinutes
* value of {@code scheduleImminentRestartWarningInMinutes} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder scheduleImminentRestartWarningInMinutes(Integer scheduleImminentRestartWarningInMinutes) {
this.scheduleImminentRestartWarningInMinutes = scheduleImminentRestartWarningInMinutes;
this.changedFields = changedFields.add("scheduleImminentRestartWarningInMinutes");
return this;
}
/**
* “Specify the period for auto-restart warning reminder notifications. Supported
* values: 2, 4, 8, 12 or 24 (hours).”
*
* @param scheduleRestartWarningInHours
* value of {@code scheduleRestartWarningInHours} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder scheduleRestartWarningInHours(Integer scheduleRestartWarningInHours) {
this.scheduleRestartWarningInHours = scheduleRestartWarningInHours;
this.changedFields = changedFields.add("scheduleRestartWarningInHours");
return this;
}
/**
* “Set to skip all check before restart: Battery level = 40%, User presence,
* Display Needed, Presentation mode, Full screen mode, phone call state, game mode
* etc.”
*
* @param skipChecksBeforeRestart
* value of {@code skipChecksBeforeRestart} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder skipChecksBeforeRestart(Boolean skipChecksBeforeRestart) {
this.skipChecksBeforeRestart = skipChecksBeforeRestart;
this.changedFields = changedFields.add("skipChecksBeforeRestart");
return this;
}
/**
* “Specifies what Windows Update notifications users see.”
*
* @param updateNotificationLevel
* value of {@code updateNotificationLevel} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder updateNotificationLevel(WindowsUpdateNotificationDisplayOption updateNotificationLevel) {
this.updateNotificationLevel = updateNotificationLevel;
this.changedFields = changedFields.add("updateNotificationLevel");
return this;
}
/**
* “Scheduled the update installation on the weeks of the month”
*
* @param updateWeeks
* value of {@code updateWeeks} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder updateWeeks(WindowsUpdateForBusinessUpdateWeeks updateWeeks) {
this.updateWeeks = updateWeeks;
this.changedFields = changedFields.add("updateWeeks");
return this;
}
/**
* “Specifies whether to enable end user’s access to pause software updates.”
*
* @param userPauseAccess
* value of {@code userPauseAccess} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userPauseAccess(Enablement userPauseAccess) {
this.userPauseAccess = userPauseAccess;
this.changedFields = changedFields.add("userPauseAccess");
return this;
}
/**
* “Specifies whether to disable user’s access to scan Windows Update.”
*
* @param userWindowsUpdateScanAccess
* value of {@code userWindowsUpdateScanAccess} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userWindowsUpdateScanAccess(Enablement userWindowsUpdateScanAccess) {
this.userWindowsUpdateScanAccess = userWindowsUpdateScanAccess;
this.changedFields = changedFields.add("userWindowsUpdateScanAccess");
return this;
}
public WindowsUpdateForBusinessConfiguration build() {
WindowsUpdateForBusinessConfiguration _x = new WindowsUpdateForBusinessConfiguration();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.windowsUpdateForBusinessConfiguration";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.roleScopeTagIdsNextLink = roleScopeTagIdsNextLink;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.automaticUpdateMode = automaticUpdateMode;
_x.autoRestartNotificationDismissal = autoRestartNotificationDismissal;
_x.businessReadyUpdatesOnly = businessReadyUpdatesOnly;
_x.deadlineForFeatureUpdatesInDays = deadlineForFeatureUpdatesInDays;
_x.deadlineForQualityUpdatesInDays = deadlineForQualityUpdatesInDays;
_x.deadlineGracePeriodInDays = deadlineGracePeriodInDays;
_x.deliveryOptimizationMode = deliveryOptimizationMode;
_x.driversExcluded = driversExcluded;
_x.engagedRestartDeadlineInDays = engagedRestartDeadlineInDays;
_x.engagedRestartSnoozeScheduleInDays = engagedRestartSnoozeScheduleInDays;
_x.engagedRestartTransitionScheduleInDays = engagedRestartTransitionScheduleInDays;
_x.featureUpdatesDeferralPeriodInDays = featureUpdatesDeferralPeriodInDays;
_x.featureUpdatesPaused = featureUpdatesPaused;
_x.featureUpdatesPauseExpiryDateTime = featureUpdatesPauseExpiryDateTime;
_x.featureUpdatesPauseStartDate = featureUpdatesPauseStartDate;
_x.featureUpdatesRollbackStartDateTime = featureUpdatesRollbackStartDateTime;
_x.featureUpdatesRollbackWindowInDays = featureUpdatesRollbackWindowInDays;
_x.featureUpdatesWillBeRolledBack = featureUpdatesWillBeRolledBack;
_x.installationSchedule = installationSchedule;
_x.microsoftUpdateServiceAllowed = microsoftUpdateServiceAllowed;
_x.postponeRebootUntilAfterDeadline = postponeRebootUntilAfterDeadline;
_x.prereleaseFeatures = prereleaseFeatures;
_x.qualityUpdatesDeferralPeriodInDays = qualityUpdatesDeferralPeriodInDays;
_x.qualityUpdatesPaused = qualityUpdatesPaused;
_x.qualityUpdatesPauseExpiryDateTime = qualityUpdatesPauseExpiryDateTime;
_x.qualityUpdatesPauseStartDate = qualityUpdatesPauseStartDate;
_x.qualityUpdatesRollbackStartDateTime = qualityUpdatesRollbackStartDateTime;
_x.qualityUpdatesWillBeRolledBack = qualityUpdatesWillBeRolledBack;
_x.scheduleImminentRestartWarningInMinutes = scheduleImminentRestartWarningInMinutes;
_x.scheduleRestartWarningInHours = scheduleRestartWarningInHours;
_x.skipChecksBeforeRestart = skipChecksBeforeRestart;
_x.updateNotificationLevel = updateNotificationLevel;
_x.updateWeeks = updateWeeks;
_x.userPauseAccess = userPauseAccess;
_x.userWindowsUpdateScanAccess = userWindowsUpdateScanAccess;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Automatic update mode.”
*
* @return property automaticUpdateMode
*/
@Property(name="automaticUpdateMode")
@JsonIgnore
public Optional getAutomaticUpdateMode() {
return Optional.ofNullable(automaticUpdateMode);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* automaticUpdateMode} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Automatic update mode.”
*
* @param automaticUpdateMode
* new value of {@code automaticUpdateMode} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code automaticUpdateMode} field changed
*/
public WindowsUpdateForBusinessConfiguration withAutomaticUpdateMode(AutomaticUpdateMode automaticUpdateMode) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("automaticUpdateMode");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.automaticUpdateMode = automaticUpdateMode;
return _x;
}
/**
* “Specify the method by which the auto-restart required notification is dismissed”
*
* @return property autoRestartNotificationDismissal
*/
@Property(name="autoRestartNotificationDismissal")
@JsonIgnore
public Optional getAutoRestartNotificationDismissal() {
return Optional.ofNullable(autoRestartNotificationDismissal);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* autoRestartNotificationDismissal} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Specify the method by which the auto-restart required notification is dismissed”
*
* @param autoRestartNotificationDismissal
* new value of {@code autoRestartNotificationDismissal} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code autoRestartNotificationDismissal} field changed
*/
public WindowsUpdateForBusinessConfiguration withAutoRestartNotificationDismissal(AutoRestartNotificationDismissalMethod autoRestartNotificationDismissal) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("autoRestartNotificationDismissal");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.autoRestartNotificationDismissal = autoRestartNotificationDismissal;
return _x;
}
/**
* “Determines which branch devices will receive their updates from”
*
* @return property businessReadyUpdatesOnly
*/
@Property(name="businessReadyUpdatesOnly")
@JsonIgnore
public Optional getBusinessReadyUpdatesOnly() {
return Optional.ofNullable(businessReadyUpdatesOnly);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* businessReadyUpdatesOnly} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Determines which branch devices will receive their updates from”
*
* @param businessReadyUpdatesOnly
* new value of {@code businessReadyUpdatesOnly} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code businessReadyUpdatesOnly} field changed
*/
public WindowsUpdateForBusinessConfiguration withBusinessReadyUpdatesOnly(WindowsUpdateType businessReadyUpdatesOnly) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("businessReadyUpdatesOnly");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.businessReadyUpdatesOnly = businessReadyUpdatesOnly;
return _x;
}
/**
* “Number of days before feature updates are installed automatically with valid
* range from 2 to 30 days”
*
* @return property deadlineForFeatureUpdatesInDays
*/
@Property(name="deadlineForFeatureUpdatesInDays")
@JsonIgnore
public Optional getDeadlineForFeatureUpdatesInDays() {
return Optional.ofNullable(deadlineForFeatureUpdatesInDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deadlineForFeatureUpdatesInDays} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Number of days before feature updates are installed automatically with valid
* range from 2 to 30 days”
*
* @param deadlineForFeatureUpdatesInDays
* new value of {@code deadlineForFeatureUpdatesInDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deadlineForFeatureUpdatesInDays} field changed
*/
public WindowsUpdateForBusinessConfiguration withDeadlineForFeatureUpdatesInDays(Integer deadlineForFeatureUpdatesInDays) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("deadlineForFeatureUpdatesInDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.deadlineForFeatureUpdatesInDays = deadlineForFeatureUpdatesInDays;
return _x;
}
/**
* “Number of days before quality updates are installed automatically with valid
* range from 2 to 30 days”
*
* @return property deadlineForQualityUpdatesInDays
*/
@Property(name="deadlineForQualityUpdatesInDays")
@JsonIgnore
public Optional getDeadlineForQualityUpdatesInDays() {
return Optional.ofNullable(deadlineForQualityUpdatesInDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deadlineForQualityUpdatesInDays} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Number of days before quality updates are installed automatically with valid
* range from 2 to 30 days”
*
* @param deadlineForQualityUpdatesInDays
* new value of {@code deadlineForQualityUpdatesInDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deadlineForQualityUpdatesInDays} field changed
*/
public WindowsUpdateForBusinessConfiguration withDeadlineForQualityUpdatesInDays(Integer deadlineForQualityUpdatesInDays) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("deadlineForQualityUpdatesInDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.deadlineForQualityUpdatesInDays = deadlineForQualityUpdatesInDays;
return _x;
}
/**
* “Number of days after deadline until restarts occur automatically with valid
* range from 0 to 7 days”
*
* @return property deadlineGracePeriodInDays
*/
@Property(name="deadlineGracePeriodInDays")
@JsonIgnore
public Optional getDeadlineGracePeriodInDays() {
return Optional.ofNullable(deadlineGracePeriodInDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deadlineGracePeriodInDays} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Number of days after deadline until restarts occur automatically with valid
* range from 0 to 7 days”
*
* @param deadlineGracePeriodInDays
* new value of {@code deadlineGracePeriodInDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deadlineGracePeriodInDays} field changed
*/
public WindowsUpdateForBusinessConfiguration withDeadlineGracePeriodInDays(Integer deadlineGracePeriodInDays) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("deadlineGracePeriodInDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.deadlineGracePeriodInDays = deadlineGracePeriodInDays;
return _x;
}
/**
* “Delivery Optimization Mode”
*
* @return property deliveryOptimizationMode
*/
@Property(name="deliveryOptimizationMode")
@JsonIgnore
public Optional getDeliveryOptimizationMode() {
return Optional.ofNullable(deliveryOptimizationMode);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deliveryOptimizationMode} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Delivery Optimization Mode”
*
* @param deliveryOptimizationMode
* new value of {@code deliveryOptimizationMode} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deliveryOptimizationMode} field changed
*/
public WindowsUpdateForBusinessConfiguration withDeliveryOptimizationMode(WindowsDeliveryOptimizationMode deliveryOptimizationMode) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("deliveryOptimizationMode");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.deliveryOptimizationMode = deliveryOptimizationMode;
return _x;
}
/**
* “Exclude Windows update Drivers”
*
* @return property driversExcluded
*/
@Property(name="driversExcluded")
@JsonIgnore
public Optional getDriversExcluded() {
return Optional.ofNullable(driversExcluded);
}
/**
* Returns an immutable copy of {@code this} with just the {@code driversExcluded}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Exclude Windows update Drivers”
*
* @param driversExcluded
* new value of {@code driversExcluded} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code driversExcluded} field changed
*/
public WindowsUpdateForBusinessConfiguration withDriversExcluded(Boolean driversExcluded) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("driversExcluded");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.driversExcluded = driversExcluded;
return _x;
}
/**
* “Deadline in days before automatically scheduling and executing a pending restart
* outside of active hours, with valid range from 2 to 30 days”
*
* @return property engagedRestartDeadlineInDays
*/
@Property(name="engagedRestartDeadlineInDays")
@JsonIgnore
public Optional getEngagedRestartDeadlineInDays() {
return Optional.ofNullable(engagedRestartDeadlineInDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* engagedRestartDeadlineInDays} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Deadline in days before automatically scheduling and executing a pending restart
* outside of active hours, with valid range from 2 to 30 days”
*
* @param engagedRestartDeadlineInDays
* new value of {@code engagedRestartDeadlineInDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code engagedRestartDeadlineInDays} field changed
*/
public WindowsUpdateForBusinessConfiguration withEngagedRestartDeadlineInDays(Integer engagedRestartDeadlineInDays) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("engagedRestartDeadlineInDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.engagedRestartDeadlineInDays = engagedRestartDeadlineInDays;
return _x;
}
/**
* “Number of days a user can snooze Engaged Restart reminder notifications with
* valid range from 1 to 3 days”
*
* @return property engagedRestartSnoozeScheduleInDays
*/
@Property(name="engagedRestartSnoozeScheduleInDays")
@JsonIgnore
public Optional getEngagedRestartSnoozeScheduleInDays() {
return Optional.ofNullable(engagedRestartSnoozeScheduleInDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* engagedRestartSnoozeScheduleInDays} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Number of days a user can snooze Engaged Restart reminder notifications with
* valid range from 1 to 3 days”
*
* @param engagedRestartSnoozeScheduleInDays
* new value of {@code engagedRestartSnoozeScheduleInDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code engagedRestartSnoozeScheduleInDays} field changed
*/
public WindowsUpdateForBusinessConfiguration withEngagedRestartSnoozeScheduleInDays(Integer engagedRestartSnoozeScheduleInDays) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("engagedRestartSnoozeScheduleInDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.engagedRestartSnoozeScheduleInDays = engagedRestartSnoozeScheduleInDays;
return _x;
}
/**
* “Number of days before transitioning from Auto Restarts scheduled outside of
* active hours to Engaged Restart, which requires the user to schedule, with valid
* range from 0 to 30 days”
*
* @return property engagedRestartTransitionScheduleInDays
*/
@Property(name="engagedRestartTransitionScheduleInDays")
@JsonIgnore
public Optional getEngagedRestartTransitionScheduleInDays() {
return Optional.ofNullable(engagedRestartTransitionScheduleInDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* engagedRestartTransitionScheduleInDays} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Number of days before transitioning from Auto Restarts scheduled outside of
* active hours to Engaged Restart, which requires the user to schedule, with valid
* range from 0 to 30 days”
*
* @param engagedRestartTransitionScheduleInDays
* new value of {@code engagedRestartTransitionScheduleInDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code engagedRestartTransitionScheduleInDays} field changed
*/
public WindowsUpdateForBusinessConfiguration withEngagedRestartTransitionScheduleInDays(Integer engagedRestartTransitionScheduleInDays) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("engagedRestartTransitionScheduleInDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.engagedRestartTransitionScheduleInDays = engagedRestartTransitionScheduleInDays;
return _x;
}
/**
* “Defer Feature Updates by these many days”
*
* @return property featureUpdatesDeferralPeriodInDays
*/
@Property(name="featureUpdatesDeferralPeriodInDays")
@JsonIgnore
public Optional getFeatureUpdatesDeferralPeriodInDays() {
return Optional.ofNullable(featureUpdatesDeferralPeriodInDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* featureUpdatesDeferralPeriodInDays} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Defer Feature Updates by these many days”
*
* @param featureUpdatesDeferralPeriodInDays
* new value of {@code featureUpdatesDeferralPeriodInDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code featureUpdatesDeferralPeriodInDays} field changed
*/
public WindowsUpdateForBusinessConfiguration withFeatureUpdatesDeferralPeriodInDays(Integer featureUpdatesDeferralPeriodInDays) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("featureUpdatesDeferralPeriodInDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.featureUpdatesDeferralPeriodInDays = featureUpdatesDeferralPeriodInDays;
return _x;
}
/**
* “Pause Feature Updates”
*
* @return property featureUpdatesPaused
*/
@Property(name="featureUpdatesPaused")
@JsonIgnore
public Optional getFeatureUpdatesPaused() {
return Optional.ofNullable(featureUpdatesPaused);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* featureUpdatesPaused} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Pause Feature Updates”
*
* @param featureUpdatesPaused
* new value of {@code featureUpdatesPaused} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code featureUpdatesPaused} field changed
*/
public WindowsUpdateForBusinessConfiguration withFeatureUpdatesPaused(Boolean featureUpdatesPaused) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("featureUpdatesPaused");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.featureUpdatesPaused = featureUpdatesPaused;
return _x;
}
/**
* “Feature Updates Pause Expiry datetime”
*
* @return property featureUpdatesPauseExpiryDateTime
*/
@Property(name="featureUpdatesPauseExpiryDateTime")
@JsonIgnore
public Optional getFeatureUpdatesPauseExpiryDateTime() {
return Optional.ofNullable(featureUpdatesPauseExpiryDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* featureUpdatesPauseExpiryDateTime} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Feature Updates Pause Expiry datetime”
*
* @param featureUpdatesPauseExpiryDateTime
* new value of {@code featureUpdatesPauseExpiryDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code featureUpdatesPauseExpiryDateTime} field changed
*/
public WindowsUpdateForBusinessConfiguration withFeatureUpdatesPauseExpiryDateTime(OffsetDateTime featureUpdatesPauseExpiryDateTime) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("featureUpdatesPauseExpiryDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.featureUpdatesPauseExpiryDateTime = featureUpdatesPauseExpiryDateTime;
return _x;
}
/**
* “Feature Updates Pause start date. This property is read-only.”
*
* Org.OData.Core.V1.Computed
*
* true
*
* Org.OData.Core.V1.Permissions
*
* @return property featureUpdatesPauseStartDate
*/
@Property(name="featureUpdatesPauseStartDate")
@JsonIgnore
public Optional getFeatureUpdatesPauseStartDate() {
return Optional.ofNullable(featureUpdatesPauseStartDate);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* featureUpdatesPauseStartDate} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Feature Updates Pause start date. This property is read-only.”
*
* Org.OData.Core.V1.Computed
*
* true
*
* Org.OData.Core.V1.Permissions
*
* @param featureUpdatesPauseStartDate
* new value of {@code featureUpdatesPauseStartDate} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code featureUpdatesPauseStartDate} field changed
*/
public WindowsUpdateForBusinessConfiguration withFeatureUpdatesPauseStartDate(LocalDate featureUpdatesPauseStartDate) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("featureUpdatesPauseStartDate");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.featureUpdatesPauseStartDate = featureUpdatesPauseStartDate;
return _x;
}
/**
* “Feature Updates Rollback Start datetime”
*
* @return property featureUpdatesRollbackStartDateTime
*/
@Property(name="featureUpdatesRollbackStartDateTime")
@JsonIgnore
public Optional getFeatureUpdatesRollbackStartDateTime() {
return Optional.ofNullable(featureUpdatesRollbackStartDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* featureUpdatesRollbackStartDateTime} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Feature Updates Rollback Start datetime”
*
* @param featureUpdatesRollbackStartDateTime
* new value of {@code featureUpdatesRollbackStartDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code featureUpdatesRollbackStartDateTime} field changed
*/
public WindowsUpdateForBusinessConfiguration withFeatureUpdatesRollbackStartDateTime(OffsetDateTime featureUpdatesRollbackStartDateTime) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("featureUpdatesRollbackStartDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.featureUpdatesRollbackStartDateTime = featureUpdatesRollbackStartDateTime;
return _x;
}
/**
* “The number of days after a Feature Update for which a rollback is valid”
*
* @return property featureUpdatesRollbackWindowInDays
*/
@Property(name="featureUpdatesRollbackWindowInDays")
@JsonIgnore
public Optional getFeatureUpdatesRollbackWindowInDays() {
return Optional.ofNullable(featureUpdatesRollbackWindowInDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* featureUpdatesRollbackWindowInDays} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The number of days after a Feature Update for which a rollback is valid”
*
* @param featureUpdatesRollbackWindowInDays
* new value of {@code featureUpdatesRollbackWindowInDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code featureUpdatesRollbackWindowInDays} field changed
*/
public WindowsUpdateForBusinessConfiguration withFeatureUpdatesRollbackWindowInDays(Integer featureUpdatesRollbackWindowInDays) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("featureUpdatesRollbackWindowInDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.featureUpdatesRollbackWindowInDays = featureUpdatesRollbackWindowInDays;
return _x;
}
/**
* “Specifies whether to rollback Feature Updates on the next device check in”
*
* @return property featureUpdatesWillBeRolledBack
*/
@Property(name="featureUpdatesWillBeRolledBack")
@JsonIgnore
public Optional getFeatureUpdatesWillBeRolledBack() {
return Optional.ofNullable(featureUpdatesWillBeRolledBack);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* featureUpdatesWillBeRolledBack} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Specifies whether to rollback Feature Updates on the next device check in”
*
* @param featureUpdatesWillBeRolledBack
* new value of {@code featureUpdatesWillBeRolledBack} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code featureUpdatesWillBeRolledBack} field changed
*/
public WindowsUpdateForBusinessConfiguration withFeatureUpdatesWillBeRolledBack(Boolean featureUpdatesWillBeRolledBack) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("featureUpdatesWillBeRolledBack");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.featureUpdatesWillBeRolledBack = featureUpdatesWillBeRolledBack;
return _x;
}
/**
* “Installation schedule”
*
* @return property installationSchedule
*/
@Property(name="installationSchedule")
@JsonIgnore
public Optional getInstallationSchedule() {
return Optional.ofNullable(installationSchedule);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* installationSchedule} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Installation schedule”
*
* @param installationSchedule
* new value of {@code installationSchedule} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code installationSchedule} field changed
*/
public WindowsUpdateForBusinessConfiguration withInstallationSchedule(WindowsUpdateInstallScheduleType installationSchedule) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("installationSchedule");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.installationSchedule = installationSchedule;
return _x;
}
/**
* “Allow Microsoft Update Service”
*
* @return property microsoftUpdateServiceAllowed
*/
@Property(name="microsoftUpdateServiceAllowed")
@JsonIgnore
public Optional getMicrosoftUpdateServiceAllowed() {
return Optional.ofNullable(microsoftUpdateServiceAllowed);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* microsoftUpdateServiceAllowed} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Allow Microsoft Update Service”
*
* @param microsoftUpdateServiceAllowed
* new value of {@code microsoftUpdateServiceAllowed} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code microsoftUpdateServiceAllowed} field changed
*/
public WindowsUpdateForBusinessConfiguration withMicrosoftUpdateServiceAllowed(Boolean microsoftUpdateServiceAllowed) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("microsoftUpdateServiceAllowed");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.microsoftUpdateServiceAllowed = microsoftUpdateServiceAllowed;
return _x;
}
/**
* “Specifies if the device should wait until deadline for rebooting outside of
* active hours”
*
* @return property postponeRebootUntilAfterDeadline
*/
@Property(name="postponeRebootUntilAfterDeadline")
@JsonIgnore
public Optional getPostponeRebootUntilAfterDeadline() {
return Optional.ofNullable(postponeRebootUntilAfterDeadline);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* postponeRebootUntilAfterDeadline} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Specifies if the device should wait until deadline for rebooting outside of
* active hours”
*
* @param postponeRebootUntilAfterDeadline
* new value of {@code postponeRebootUntilAfterDeadline} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code postponeRebootUntilAfterDeadline} field changed
*/
public WindowsUpdateForBusinessConfiguration withPostponeRebootUntilAfterDeadline(Boolean postponeRebootUntilAfterDeadline) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("postponeRebootUntilAfterDeadline");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.postponeRebootUntilAfterDeadline = postponeRebootUntilAfterDeadline;
return _x;
}
/**
* “The pre-release features.”
*
* @return property prereleaseFeatures
*/
@Property(name="prereleaseFeatures")
@JsonIgnore
public Optional getPrereleaseFeatures() {
return Optional.ofNullable(prereleaseFeatures);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* prereleaseFeatures} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The pre-release features.”
*
* @param prereleaseFeatures
* new value of {@code prereleaseFeatures} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code prereleaseFeatures} field changed
*/
public WindowsUpdateForBusinessConfiguration withPrereleaseFeatures(PrereleaseFeatures prereleaseFeatures) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("prereleaseFeatures");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.prereleaseFeatures = prereleaseFeatures;
return _x;
}
/**
* “Defer Quality Updates by these many days”
*
* @return property qualityUpdatesDeferralPeriodInDays
*/
@Property(name="qualityUpdatesDeferralPeriodInDays")
@JsonIgnore
public Optional getQualityUpdatesDeferralPeriodInDays() {
return Optional.ofNullable(qualityUpdatesDeferralPeriodInDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* qualityUpdatesDeferralPeriodInDays} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Defer Quality Updates by these many days”
*
* @param qualityUpdatesDeferralPeriodInDays
* new value of {@code qualityUpdatesDeferralPeriodInDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code qualityUpdatesDeferralPeriodInDays} field changed
*/
public WindowsUpdateForBusinessConfiguration withQualityUpdatesDeferralPeriodInDays(Integer qualityUpdatesDeferralPeriodInDays) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("qualityUpdatesDeferralPeriodInDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.qualityUpdatesDeferralPeriodInDays = qualityUpdatesDeferralPeriodInDays;
return _x;
}
/**
* “Pause Quality Updates”
*
* @return property qualityUpdatesPaused
*/
@Property(name="qualityUpdatesPaused")
@JsonIgnore
public Optional getQualityUpdatesPaused() {
return Optional.ofNullable(qualityUpdatesPaused);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* qualityUpdatesPaused} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Pause Quality Updates”
*
* @param qualityUpdatesPaused
* new value of {@code qualityUpdatesPaused} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code qualityUpdatesPaused} field changed
*/
public WindowsUpdateForBusinessConfiguration withQualityUpdatesPaused(Boolean qualityUpdatesPaused) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("qualityUpdatesPaused");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.qualityUpdatesPaused = qualityUpdatesPaused;
return _x;
}
/**
* “Quality Updates Pause Expiry datetime”
*
* @return property qualityUpdatesPauseExpiryDateTime
*/
@Property(name="qualityUpdatesPauseExpiryDateTime")
@JsonIgnore
public Optional getQualityUpdatesPauseExpiryDateTime() {
return Optional.ofNullable(qualityUpdatesPauseExpiryDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* qualityUpdatesPauseExpiryDateTime} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Quality Updates Pause Expiry datetime”
*
* @param qualityUpdatesPauseExpiryDateTime
* new value of {@code qualityUpdatesPauseExpiryDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code qualityUpdatesPauseExpiryDateTime} field changed
*/
public WindowsUpdateForBusinessConfiguration withQualityUpdatesPauseExpiryDateTime(OffsetDateTime qualityUpdatesPauseExpiryDateTime) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("qualityUpdatesPauseExpiryDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.qualityUpdatesPauseExpiryDateTime = qualityUpdatesPauseExpiryDateTime;
return _x;
}
/**
* “Quality Updates Pause start date. This property is read-only.”
*
* Org.OData.Core.V1.Computed
*
* true
*
* Org.OData.Core.V1.Permissions
*
* @return property qualityUpdatesPauseStartDate
*/
@Property(name="qualityUpdatesPauseStartDate")
@JsonIgnore
public Optional getQualityUpdatesPauseStartDate() {
return Optional.ofNullable(qualityUpdatesPauseStartDate);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* qualityUpdatesPauseStartDate} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Quality Updates Pause start date. This property is read-only.”
*
* Org.OData.Core.V1.Computed
*
* true
*
* Org.OData.Core.V1.Permissions
*
* @param qualityUpdatesPauseStartDate
* new value of {@code qualityUpdatesPauseStartDate} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code qualityUpdatesPauseStartDate} field changed
*/
public WindowsUpdateForBusinessConfiguration withQualityUpdatesPauseStartDate(LocalDate qualityUpdatesPauseStartDate) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("qualityUpdatesPauseStartDate");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.qualityUpdatesPauseStartDate = qualityUpdatesPauseStartDate;
return _x;
}
/**
* “Quality Updates Rollback Start datetime”
*
* @return property qualityUpdatesRollbackStartDateTime
*/
@Property(name="qualityUpdatesRollbackStartDateTime")
@JsonIgnore
public Optional getQualityUpdatesRollbackStartDateTime() {
return Optional.ofNullable(qualityUpdatesRollbackStartDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* qualityUpdatesRollbackStartDateTime} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Quality Updates Rollback Start datetime”
*
* @param qualityUpdatesRollbackStartDateTime
* new value of {@code qualityUpdatesRollbackStartDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code qualityUpdatesRollbackStartDateTime} field changed
*/
public WindowsUpdateForBusinessConfiguration withQualityUpdatesRollbackStartDateTime(OffsetDateTime qualityUpdatesRollbackStartDateTime) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("qualityUpdatesRollbackStartDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.qualityUpdatesRollbackStartDateTime = qualityUpdatesRollbackStartDateTime;
return _x;
}
/**
* “Specifies whether to rollback Quality Updates on the next device check in”
*
* @return property qualityUpdatesWillBeRolledBack
*/
@Property(name="qualityUpdatesWillBeRolledBack")
@JsonIgnore
public Optional getQualityUpdatesWillBeRolledBack() {
return Optional.ofNullable(qualityUpdatesWillBeRolledBack);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* qualityUpdatesWillBeRolledBack} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Specifies whether to rollback Quality Updates on the next device check in”
*
* @param qualityUpdatesWillBeRolledBack
* new value of {@code qualityUpdatesWillBeRolledBack} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code qualityUpdatesWillBeRolledBack} field changed
*/
public WindowsUpdateForBusinessConfiguration withQualityUpdatesWillBeRolledBack(Boolean qualityUpdatesWillBeRolledBack) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("qualityUpdatesWillBeRolledBack");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.qualityUpdatesWillBeRolledBack = qualityUpdatesWillBeRolledBack;
return _x;
}
/**
* “Specify the period for auto-restart imminent warning notifications. Supported
* values: 15, 30 or 60 (minutes).”
*
* @return property scheduleImminentRestartWarningInMinutes
*/
@Property(name="scheduleImminentRestartWarningInMinutes")
@JsonIgnore
public Optional getScheduleImminentRestartWarningInMinutes() {
return Optional.ofNullable(scheduleImminentRestartWarningInMinutes);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* scheduleImminentRestartWarningInMinutes} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Specify the period for auto-restart imminent warning notifications. Supported
* values: 15, 30 or 60 (minutes).”
*
* @param scheduleImminentRestartWarningInMinutes
* new value of {@code scheduleImminentRestartWarningInMinutes} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code scheduleImminentRestartWarningInMinutes} field changed
*/
public WindowsUpdateForBusinessConfiguration withScheduleImminentRestartWarningInMinutes(Integer scheduleImminentRestartWarningInMinutes) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("scheduleImminentRestartWarningInMinutes");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.scheduleImminentRestartWarningInMinutes = scheduleImminentRestartWarningInMinutes;
return _x;
}
/**
* “Specify the period for auto-restart warning reminder notifications. Supported
* values: 2, 4, 8, 12 or 24 (hours).”
*
* @return property scheduleRestartWarningInHours
*/
@Property(name="scheduleRestartWarningInHours")
@JsonIgnore
public Optional getScheduleRestartWarningInHours() {
return Optional.ofNullable(scheduleRestartWarningInHours);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* scheduleRestartWarningInHours} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Specify the period for auto-restart warning reminder notifications. Supported
* values: 2, 4, 8, 12 or 24 (hours).”
*
* @param scheduleRestartWarningInHours
* new value of {@code scheduleRestartWarningInHours} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code scheduleRestartWarningInHours} field changed
*/
public WindowsUpdateForBusinessConfiguration withScheduleRestartWarningInHours(Integer scheduleRestartWarningInHours) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("scheduleRestartWarningInHours");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.scheduleRestartWarningInHours = scheduleRestartWarningInHours;
return _x;
}
/**
* “Set to skip all check before restart: Battery level = 40%, User presence,
* Display Needed, Presentation mode, Full screen mode, phone call state, game mode
* etc.”
*
* @return property skipChecksBeforeRestart
*/
@Property(name="skipChecksBeforeRestart")
@JsonIgnore
public Optional getSkipChecksBeforeRestart() {
return Optional.ofNullable(skipChecksBeforeRestart);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* skipChecksBeforeRestart} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Set to skip all check before restart: Battery level = 40%, User presence,
* Display Needed, Presentation mode, Full screen mode, phone call state, game mode
* etc.”
*
* @param skipChecksBeforeRestart
* new value of {@code skipChecksBeforeRestart} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code skipChecksBeforeRestart} field changed
*/
public WindowsUpdateForBusinessConfiguration withSkipChecksBeforeRestart(Boolean skipChecksBeforeRestart) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("skipChecksBeforeRestart");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.skipChecksBeforeRestart = skipChecksBeforeRestart;
return _x;
}
/**
* “Specifies what Windows Update notifications users see.”
*
* @return property updateNotificationLevel
*/
@Property(name="updateNotificationLevel")
@JsonIgnore
public Optional getUpdateNotificationLevel() {
return Optional.ofNullable(updateNotificationLevel);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* updateNotificationLevel} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Specifies what Windows Update notifications users see.”
*
* @param updateNotificationLevel
* new value of {@code updateNotificationLevel} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code updateNotificationLevel} field changed
*/
public WindowsUpdateForBusinessConfiguration withUpdateNotificationLevel(WindowsUpdateNotificationDisplayOption updateNotificationLevel) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("updateNotificationLevel");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.updateNotificationLevel = updateNotificationLevel;
return _x;
}
/**
* “Scheduled the update installation on the weeks of the month”
*
* @return property updateWeeks
*/
@Property(name="updateWeeks")
@JsonIgnore
public Optional getUpdateWeeks() {
return Optional.ofNullable(updateWeeks);
}
/**
* Returns an immutable copy of {@code this} with just the {@code updateWeeks}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Scheduled the update installation on the weeks of the month”
*
* @param updateWeeks
* new value of {@code updateWeeks} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code updateWeeks} field changed
*/
public WindowsUpdateForBusinessConfiguration withUpdateWeeks(WindowsUpdateForBusinessUpdateWeeks updateWeeks) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("updateWeeks");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.updateWeeks = updateWeeks;
return _x;
}
/**
* “Specifies whether to enable end user’s access to pause software updates.”
*
* @return property userPauseAccess
*/
@Property(name="userPauseAccess")
@JsonIgnore
public Optional getUserPauseAccess() {
return Optional.ofNullable(userPauseAccess);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userPauseAccess}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Specifies whether to enable end user’s access to pause software updates.”
*
* @param userPauseAccess
* new value of {@code userPauseAccess} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userPauseAccess} field changed
*/
public WindowsUpdateForBusinessConfiguration withUserPauseAccess(Enablement userPauseAccess) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("userPauseAccess");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.userPauseAccess = userPauseAccess;
return _x;
}
/**
* “Specifies whether to disable user’s access to scan Windows Update.”
*
* @return property userWindowsUpdateScanAccess
*/
@Property(name="userWindowsUpdateScanAccess")
@JsonIgnore
public Optional getUserWindowsUpdateScanAccess() {
return Optional.ofNullable(userWindowsUpdateScanAccess);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userWindowsUpdateScanAccess} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Specifies whether to disable user’s access to scan Windows Update.”
*
* @param userWindowsUpdateScanAccess
* new value of {@code userWindowsUpdateScanAccess} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userWindowsUpdateScanAccess} field changed
*/
public WindowsUpdateForBusinessConfiguration withUserWindowsUpdateScanAccess(Enablement userWindowsUpdateScanAccess) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = changedFields.add("userWindowsUpdateScanAccess");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsUpdateForBusinessConfiguration");
_x.userWindowsUpdateScanAccess = userWindowsUpdateScanAccess;
return _x;
}
public WindowsUpdateForBusinessConfiguration withUnmappedField(String name, String value) {
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “Windows update for business configuration device states.”
*
* @return navigational property deviceUpdateStates
*/
@NavigationProperty(name="deviceUpdateStates")
@JsonIgnore
public WindowsUpdateStateCollectionRequest getDeviceUpdateStates() {
return new WindowsUpdateStateCollectionRequest(
contextPath.addSegment("deviceUpdateStates"), RequestHelper.getValue(unmappedFields, "deviceUpdateStates"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WindowsUpdateForBusinessConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WindowsUpdateForBusinessConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
WindowsUpdateForBusinessConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private WindowsUpdateForBusinessConfiguration _copy() {
WindowsUpdateForBusinessConfiguration _x = new WindowsUpdateForBusinessConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.automaticUpdateMode = automaticUpdateMode;
_x.autoRestartNotificationDismissal = autoRestartNotificationDismissal;
_x.businessReadyUpdatesOnly = businessReadyUpdatesOnly;
_x.deadlineForFeatureUpdatesInDays = deadlineForFeatureUpdatesInDays;
_x.deadlineForQualityUpdatesInDays = deadlineForQualityUpdatesInDays;
_x.deadlineGracePeriodInDays = deadlineGracePeriodInDays;
_x.deliveryOptimizationMode = deliveryOptimizationMode;
_x.driversExcluded = driversExcluded;
_x.engagedRestartDeadlineInDays = engagedRestartDeadlineInDays;
_x.engagedRestartSnoozeScheduleInDays = engagedRestartSnoozeScheduleInDays;
_x.engagedRestartTransitionScheduleInDays = engagedRestartTransitionScheduleInDays;
_x.featureUpdatesDeferralPeriodInDays = featureUpdatesDeferralPeriodInDays;
_x.featureUpdatesPaused = featureUpdatesPaused;
_x.featureUpdatesPauseExpiryDateTime = featureUpdatesPauseExpiryDateTime;
_x.featureUpdatesPauseStartDate = featureUpdatesPauseStartDate;
_x.featureUpdatesRollbackStartDateTime = featureUpdatesRollbackStartDateTime;
_x.featureUpdatesRollbackWindowInDays = featureUpdatesRollbackWindowInDays;
_x.featureUpdatesWillBeRolledBack = featureUpdatesWillBeRolledBack;
_x.installationSchedule = installationSchedule;
_x.microsoftUpdateServiceAllowed = microsoftUpdateServiceAllowed;
_x.postponeRebootUntilAfterDeadline = postponeRebootUntilAfterDeadline;
_x.prereleaseFeatures = prereleaseFeatures;
_x.qualityUpdatesDeferralPeriodInDays = qualityUpdatesDeferralPeriodInDays;
_x.qualityUpdatesPaused = qualityUpdatesPaused;
_x.qualityUpdatesPauseExpiryDateTime = qualityUpdatesPauseExpiryDateTime;
_x.qualityUpdatesPauseStartDate = qualityUpdatesPauseStartDate;
_x.qualityUpdatesRollbackStartDateTime = qualityUpdatesRollbackStartDateTime;
_x.qualityUpdatesWillBeRolledBack = qualityUpdatesWillBeRolledBack;
_x.scheduleImminentRestartWarningInMinutes = scheduleImminentRestartWarningInMinutes;
_x.scheduleRestartWarningInHours = scheduleRestartWarningInHours;
_x.skipChecksBeforeRestart = skipChecksBeforeRestart;
_x.updateNotificationLevel = updateNotificationLevel;
_x.updateWeeks = updateWeeks;
_x.userPauseAccess = userPauseAccess;
_x.userWindowsUpdateScanAccess = userWindowsUpdateScanAccess;
return _x;
}
@Action(name = "extendFeatureUpdatesPause")
@JsonIgnore
public ActionRequestNoReturn extendFeatureUpdatesPause() {
Map _parameters = ParameterMap.empty();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("microsoft.graph.extendFeatureUpdatesPause"), _parameters);
}
@Action(name = "extendQualityUpdatesPause")
@JsonIgnore
public ActionRequestNoReturn extendQualityUpdatesPause() {
Map _parameters = ParameterMap.empty();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("microsoft.graph.extendQualityUpdatesPause"), _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("WindowsUpdateForBusinessConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("deviceManagementApplicabilityRuleDeviceMode=");
b.append(this.deviceManagementApplicabilityRuleDeviceMode);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsEdition=");
b.append(this.deviceManagementApplicabilityRuleOsEdition);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsVersion=");
b.append(this.deviceManagementApplicabilityRuleOsVersion);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("supportsScopeTags=");
b.append(this.supportsScopeTags);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("automaticUpdateMode=");
b.append(this.automaticUpdateMode);
b.append(", ");
b.append("autoRestartNotificationDismissal=");
b.append(this.autoRestartNotificationDismissal);
b.append(", ");
b.append("businessReadyUpdatesOnly=");
b.append(this.businessReadyUpdatesOnly);
b.append(", ");
b.append("deadlineForFeatureUpdatesInDays=");
b.append(this.deadlineForFeatureUpdatesInDays);
b.append(", ");
b.append("deadlineForQualityUpdatesInDays=");
b.append(this.deadlineForQualityUpdatesInDays);
b.append(", ");
b.append("deadlineGracePeriodInDays=");
b.append(this.deadlineGracePeriodInDays);
b.append(", ");
b.append("deliveryOptimizationMode=");
b.append(this.deliveryOptimizationMode);
b.append(", ");
b.append("driversExcluded=");
b.append(this.driversExcluded);
b.append(", ");
b.append("engagedRestartDeadlineInDays=");
b.append(this.engagedRestartDeadlineInDays);
b.append(", ");
b.append("engagedRestartSnoozeScheduleInDays=");
b.append(this.engagedRestartSnoozeScheduleInDays);
b.append(", ");
b.append("engagedRestartTransitionScheduleInDays=");
b.append(this.engagedRestartTransitionScheduleInDays);
b.append(", ");
b.append("featureUpdatesDeferralPeriodInDays=");
b.append(this.featureUpdatesDeferralPeriodInDays);
b.append(", ");
b.append("featureUpdatesPaused=");
b.append(this.featureUpdatesPaused);
b.append(", ");
b.append("featureUpdatesPauseExpiryDateTime=");
b.append(this.featureUpdatesPauseExpiryDateTime);
b.append(", ");
b.append("featureUpdatesPauseStartDate=");
b.append(this.featureUpdatesPauseStartDate);
b.append(", ");
b.append("featureUpdatesRollbackStartDateTime=");
b.append(this.featureUpdatesRollbackStartDateTime);
b.append(", ");
b.append("featureUpdatesRollbackWindowInDays=");
b.append(this.featureUpdatesRollbackWindowInDays);
b.append(", ");
b.append("featureUpdatesWillBeRolledBack=");
b.append(this.featureUpdatesWillBeRolledBack);
b.append(", ");
b.append("installationSchedule=");
b.append(this.installationSchedule);
b.append(", ");
b.append("microsoftUpdateServiceAllowed=");
b.append(this.microsoftUpdateServiceAllowed);
b.append(", ");
b.append("postponeRebootUntilAfterDeadline=");
b.append(this.postponeRebootUntilAfterDeadline);
b.append(", ");
b.append("prereleaseFeatures=");
b.append(this.prereleaseFeatures);
b.append(", ");
b.append("qualityUpdatesDeferralPeriodInDays=");
b.append(this.qualityUpdatesDeferralPeriodInDays);
b.append(", ");
b.append("qualityUpdatesPaused=");
b.append(this.qualityUpdatesPaused);
b.append(", ");
b.append("qualityUpdatesPauseExpiryDateTime=");
b.append(this.qualityUpdatesPauseExpiryDateTime);
b.append(", ");
b.append("qualityUpdatesPauseStartDate=");
b.append(this.qualityUpdatesPauseStartDate);
b.append(", ");
b.append("qualityUpdatesRollbackStartDateTime=");
b.append(this.qualityUpdatesRollbackStartDateTime);
b.append(", ");
b.append("qualityUpdatesWillBeRolledBack=");
b.append(this.qualityUpdatesWillBeRolledBack);
b.append(", ");
b.append("scheduleImminentRestartWarningInMinutes=");
b.append(this.scheduleImminentRestartWarningInMinutes);
b.append(", ");
b.append("scheduleRestartWarningInHours=");
b.append(this.scheduleRestartWarningInHours);
b.append(", ");
b.append("skipChecksBeforeRestart=");
b.append(this.skipChecksBeforeRestart);
b.append(", ");
b.append("updateNotificationLevel=");
b.append(this.updateNotificationLevel);
b.append(", ");
b.append("updateWeeks=");
b.append(this.updateWeeks);
b.append(", ");
b.append("userPauseAccess=");
b.append(this.userPauseAccess);
b.append(", ");
b.append("userWindowsUpdateScanAccess=");
b.append(this.userWindowsUpdateScanAccess);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}