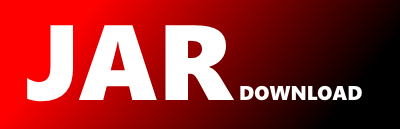
odata.msgraph.client.beta.entity.WindowsVpnConfiguration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph-beta Show documentation
package odata.msgraph.client.beta.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.beta.complex.VpnServer;
/**
* “Windows VPN configuration profile.”
*/@JsonPropertyOrder({
"@odata.type",
"connectionName",
"customXml",
"servers"})
@JsonInclude(Include.NON_NULL)
public class WindowsVpnConfiguration extends DeviceConfiguration implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.windowsVpnConfiguration";
}
@JsonProperty("connectionName")
protected String connectionName;
@JsonProperty("customXml")
protected byte[] customXml;
@JsonProperty("servers")
protected List servers;
@JsonProperty("servers@nextLink")
protected String serversNextLink;
protected WindowsVpnConfiguration() {
super();
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id.toString()));
}
}
/**
* “Connection name displayed to the user.”
*
* @return property connectionName
*/
@Property(name="connectionName")
@JsonIgnore
public Optional getConnectionName() {
return Optional.ofNullable(connectionName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code connectionName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Connection name displayed to the user.”
*
* @param connectionName
* new value of {@code connectionName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code connectionName} field changed
*/
public WindowsVpnConfiguration withConnectionName(String connectionName) {
WindowsVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("connectionName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsVpnConfiguration");
_x.connectionName = connectionName;
return _x;
}
/**
* “Custom XML commands that configures the VPN connection. (UTF8 encoded byte array
* )”
*
* @return property customXml
*/
@Property(name="customXml")
@JsonIgnore
public Optional getCustomXml() {
return Optional.ofNullable(customXml);
}
/**
* Returns an immutable copy of {@code this} with just the {@code customXml} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Custom XML commands that configures the VPN connection. (UTF8 encoded byte array
* )”
*
* @param customXml
* new value of {@code customXml} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code customXml} field changed
*/
public WindowsVpnConfiguration withCustomXml(byte[] customXml) {
WindowsVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("customXml");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsVpnConfiguration");
_x.customXml = customXml;
return _x;
}
/**
* “List of VPN Servers on the network. Make sure end users can access these network
* locations. This collection can contain a maximum of 500 elements.”
*
* @return property servers
*/
@Property(name="servers")
@JsonIgnore
public CollectionPage getServers() {
return new CollectionPage(contextPath, VpnServer.class, this.servers, Optional.ofNullable(serversNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code servers} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “List of VPN Servers on the network. Make sure end users can access these network
* locations. This collection can contain a maximum of 500 elements.”
*
* @param servers
* new value of {@code servers} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code servers} field changed
*/
public WindowsVpnConfiguration withServers(List servers) {
WindowsVpnConfiguration _x = _copy();
_x.changedFields = changedFields.add("servers");
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsVpnConfiguration");
_x.servers = servers;
return _x;
}
/**
* “List of VPN Servers on the network. Make sure end users can access these network
* locations. This collection can contain a maximum of 500 elements.”
*
* @param options
* specify connect and read timeouts
* @return property servers
*/
@Property(name="servers")
@JsonIgnore
public CollectionPage getServers(HttpRequestOptions options) {
return new CollectionPage(contextPath, VpnServer.class, this.servers, Optional.ofNullable(serversNextLink), Collections.emptyList(), options);
}
public WindowsVpnConfiguration withUnmappedField(String name, String value) {
WindowsVpnConfiguration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WindowsVpnConfiguration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
WindowsVpnConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public WindowsVpnConfiguration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
WindowsVpnConfiguration _x = _copy();
_x.changedFields = null;
return _x;
}
private WindowsVpnConfiguration _copy() {
WindowsVpnConfiguration _x = new WindowsVpnConfiguration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.deviceManagementApplicabilityRuleDeviceMode = deviceManagementApplicabilityRuleDeviceMode;
_x.deviceManagementApplicabilityRuleOsEdition = deviceManagementApplicabilityRuleOsEdition;
_x.deviceManagementApplicabilityRuleOsVersion = deviceManagementApplicabilityRuleOsVersion;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.roleScopeTagIds = roleScopeTagIds;
_x.supportsScopeTags = supportsScopeTags;
_x.version = version;
_x.connectionName = connectionName;
_x.customXml = customXml;
_x.servers = servers;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("WindowsVpnConfiguration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("deviceManagementApplicabilityRuleDeviceMode=");
b.append(this.deviceManagementApplicabilityRuleDeviceMode);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsEdition=");
b.append(this.deviceManagementApplicabilityRuleOsEdition);
b.append(", ");
b.append("deviceManagementApplicabilityRuleOsVersion=");
b.append(this.deviceManagementApplicabilityRuleOsVersion);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("roleScopeTagIds=");
b.append(this.roleScopeTagIds);
b.append(", ");
b.append("supportsScopeTags=");
b.append(this.supportsScopeTags);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("connectionName=");
b.append(this.connectionName);
b.append(", ");
b.append("customXml=");
b.append(this.customXml);
b.append(", ");
b.append("servers=");
b.append(this.servers);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}