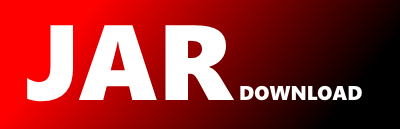
odata.msgraph.client.beta.entity.collection.request.InformationProtectionLabelCollectionRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph-beta Show documentation
Show all versions of odata-client-msgraph-beta Show documentation
Java client for use with Microsoft Graph beta endpoint
package odata.msgraph.client.beta.entity.collection.request;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.github.davidmoten.guavamini.Preconditions;
import com.github.davidmoten.odata.client.ActionRequestReturningNonCollectionUnwrapped;
import com.github.davidmoten.odata.client.CollectionPageEntityRequest;
import com.github.davidmoten.odata.client.CollectionPageNonEntityRequest;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.TypedObject;
import java.lang.Object;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import odata.msgraph.client.beta.complex.ClassificationResult;
import odata.msgraph.client.beta.complex.ContentInfo;
import odata.msgraph.client.beta.complex.DowngradeJustification;
import odata.msgraph.client.beta.complex.InformationProtectionAction;
import odata.msgraph.client.beta.complex.InformationProtectionContentLabel;
import odata.msgraph.client.beta.complex.LabelingOptions;
import odata.msgraph.client.beta.entity.InformationProtectionLabel;
import odata.msgraph.client.beta.entity.request.InformationProtectionLabelRequest;
public class InformationProtectionLabelCollectionRequest extends CollectionPageEntityRequest{
protected ContextPath contextPath;
public InformationProtectionLabelCollectionRequest(ContextPath contextPath, Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy