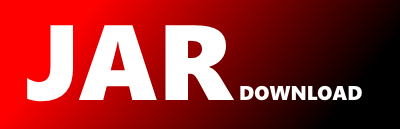
odata.msgraph.client.callrecords.complex.DirectRoutingLogRow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph Show documentation
Show all versions of odata-client-msgraph Show documentation
Java client for use with the Microsoft Graph v1.0 endpoint
package odata.msgraph.client.callrecords.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Optional;
@JsonPropertyOrder({
"@odata.type",
"calleeNumber",
"callEndSubReason",
"callerNumber",
"callType",
"correlationId",
"duration",
"endDateTime",
"failureDateTime",
"finalSipCode",
"finalSipCodePhrase",
"id",
"inviteDateTime",
"mediaBypassEnabled",
"mediaPathLocation",
"signalingLocation",
"startDateTime",
"successfulCall",
"trunkFullyQualifiedDomainName",
"userDisplayName",
"userId",
"userPrincipalName"})
@JsonInclude(Include.NON_NULL)
public class DirectRoutingLogRow implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("calleeNumber")
protected String calleeNumber;
@JsonProperty("callEndSubReason")
protected Integer callEndSubReason;
@JsonProperty("callerNumber")
protected String callerNumber;
@JsonProperty("callType")
protected String callType;
@JsonProperty("correlationId")
protected String correlationId;
@JsonProperty("duration")
protected Integer duration;
@JsonProperty("endDateTime")
protected OffsetDateTime endDateTime;
@JsonProperty("failureDateTime")
protected OffsetDateTime failureDateTime;
@JsonProperty("finalSipCode")
protected Integer finalSipCode;
@JsonProperty("finalSipCodePhrase")
protected String finalSipCodePhrase;
@JsonProperty("id")
protected String id;
@JsonProperty("inviteDateTime")
protected OffsetDateTime inviteDateTime;
@JsonProperty("mediaBypassEnabled")
protected Boolean mediaBypassEnabled;
@JsonProperty("mediaPathLocation")
protected String mediaPathLocation;
@JsonProperty("signalingLocation")
protected String signalingLocation;
@JsonProperty("startDateTime")
protected OffsetDateTime startDateTime;
@JsonProperty("successfulCall")
protected Boolean successfulCall;
@JsonProperty("trunkFullyQualifiedDomainName")
protected String trunkFullyQualifiedDomainName;
@JsonProperty("userDisplayName")
protected String userDisplayName;
@JsonProperty("userId")
protected String userId;
@JsonProperty("userPrincipalName")
protected String userPrincipalName;
protected DirectRoutingLogRow() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.callRecords.directRoutingLogRow";
}
@Property(name="calleeNumber")
@JsonIgnore
public Optional getCalleeNumber() {
return Optional.ofNullable(calleeNumber);
}
public DirectRoutingLogRow withCalleeNumber(String calleeNumber) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.calleeNumber = calleeNumber;
return _x;
}
@Property(name="callEndSubReason")
@JsonIgnore
public Optional getCallEndSubReason() {
return Optional.ofNullable(callEndSubReason);
}
public DirectRoutingLogRow withCallEndSubReason(Integer callEndSubReason) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.callEndSubReason = callEndSubReason;
return _x;
}
@Property(name="callerNumber")
@JsonIgnore
public Optional getCallerNumber() {
return Optional.ofNullable(callerNumber);
}
public DirectRoutingLogRow withCallerNumber(String callerNumber) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.callerNumber = callerNumber;
return _x;
}
@Property(name="callType")
@JsonIgnore
public Optional getCallType() {
return Optional.ofNullable(callType);
}
public DirectRoutingLogRow withCallType(String callType) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.callType = callType;
return _x;
}
@Property(name="correlationId")
@JsonIgnore
public Optional getCorrelationId() {
return Optional.ofNullable(correlationId);
}
public DirectRoutingLogRow withCorrelationId(String correlationId) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.correlationId = correlationId;
return _x;
}
@Property(name="duration")
@JsonIgnore
public Optional getDuration() {
return Optional.ofNullable(duration);
}
public DirectRoutingLogRow withDuration(Integer duration) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.duration = duration;
return _x;
}
@Property(name="endDateTime")
@JsonIgnore
public Optional getEndDateTime() {
return Optional.ofNullable(endDateTime);
}
public DirectRoutingLogRow withEndDateTime(OffsetDateTime endDateTime) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.endDateTime = endDateTime;
return _x;
}
@Property(name="failureDateTime")
@JsonIgnore
public Optional getFailureDateTime() {
return Optional.ofNullable(failureDateTime);
}
public DirectRoutingLogRow withFailureDateTime(OffsetDateTime failureDateTime) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.failureDateTime = failureDateTime;
return _x;
}
@Property(name="finalSipCode")
@JsonIgnore
public Optional getFinalSipCode() {
return Optional.ofNullable(finalSipCode);
}
public DirectRoutingLogRow withFinalSipCode(Integer finalSipCode) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.finalSipCode = finalSipCode;
return _x;
}
@Property(name="finalSipCodePhrase")
@JsonIgnore
public Optional getFinalSipCodePhrase() {
return Optional.ofNullable(finalSipCodePhrase);
}
public DirectRoutingLogRow withFinalSipCodePhrase(String finalSipCodePhrase) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.finalSipCodePhrase = finalSipCodePhrase;
return _x;
}
@Property(name="id")
@JsonIgnore
public Optional getId() {
return Optional.ofNullable(id);
}
public DirectRoutingLogRow withId(String id) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.id = id;
return _x;
}
@Property(name="inviteDateTime")
@JsonIgnore
public Optional getInviteDateTime() {
return Optional.ofNullable(inviteDateTime);
}
public DirectRoutingLogRow withInviteDateTime(OffsetDateTime inviteDateTime) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.inviteDateTime = inviteDateTime;
return _x;
}
@Property(name="mediaBypassEnabled")
@JsonIgnore
public Optional getMediaBypassEnabled() {
return Optional.ofNullable(mediaBypassEnabled);
}
public DirectRoutingLogRow withMediaBypassEnabled(Boolean mediaBypassEnabled) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.mediaBypassEnabled = mediaBypassEnabled;
return _x;
}
@Property(name="mediaPathLocation")
@JsonIgnore
public Optional getMediaPathLocation() {
return Optional.ofNullable(mediaPathLocation);
}
public DirectRoutingLogRow withMediaPathLocation(String mediaPathLocation) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.mediaPathLocation = mediaPathLocation;
return _x;
}
@Property(name="signalingLocation")
@JsonIgnore
public Optional getSignalingLocation() {
return Optional.ofNullable(signalingLocation);
}
public DirectRoutingLogRow withSignalingLocation(String signalingLocation) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.signalingLocation = signalingLocation;
return _x;
}
@Property(name="startDateTime")
@JsonIgnore
public Optional getStartDateTime() {
return Optional.ofNullable(startDateTime);
}
public DirectRoutingLogRow withStartDateTime(OffsetDateTime startDateTime) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.startDateTime = startDateTime;
return _x;
}
@Property(name="successfulCall")
@JsonIgnore
public Optional getSuccessfulCall() {
return Optional.ofNullable(successfulCall);
}
public DirectRoutingLogRow withSuccessfulCall(Boolean successfulCall) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.successfulCall = successfulCall;
return _x;
}
@Property(name="trunkFullyQualifiedDomainName")
@JsonIgnore
public Optional getTrunkFullyQualifiedDomainName() {
return Optional.ofNullable(trunkFullyQualifiedDomainName);
}
public DirectRoutingLogRow withTrunkFullyQualifiedDomainName(String trunkFullyQualifiedDomainName) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.trunkFullyQualifiedDomainName = trunkFullyQualifiedDomainName;
return _x;
}
@Property(name="userDisplayName")
@JsonIgnore
public Optional getUserDisplayName() {
return Optional.ofNullable(userDisplayName);
}
public DirectRoutingLogRow withUserDisplayName(String userDisplayName) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.userDisplayName = userDisplayName;
return _x;
}
@Property(name="userId")
@JsonIgnore
public Optional getUserId() {
return Optional.ofNullable(userId);
}
public DirectRoutingLogRow withUserId(String userId) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.userId = userId;
return _x;
}
@Property(name="userPrincipalName")
@JsonIgnore
public Optional getUserPrincipalName() {
return Optional.ofNullable(userPrincipalName);
}
public DirectRoutingLogRow withUserPrincipalName(String userPrincipalName) {
DirectRoutingLogRow _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.directRoutingLogRow");
_x.userPrincipalName = userPrincipalName;
return _x;
}
public DirectRoutingLogRow withUnmappedField(String name, Object value) {
DirectRoutingLogRow _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String calleeNumber;
private Integer callEndSubReason;
private String callerNumber;
private String callType;
private String correlationId;
private Integer duration;
private OffsetDateTime endDateTime;
private OffsetDateTime failureDateTime;
private Integer finalSipCode;
private String finalSipCodePhrase;
private String id;
private OffsetDateTime inviteDateTime;
private Boolean mediaBypassEnabled;
private String mediaPathLocation;
private String signalingLocation;
private OffsetDateTime startDateTime;
private Boolean successfulCall;
private String trunkFullyQualifiedDomainName;
private String userDisplayName;
private String userId;
private String userPrincipalName;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder calleeNumber(String calleeNumber) {
this.calleeNumber = calleeNumber;
this.changedFields = changedFields.add("calleeNumber");
return this;
}
public Builder callEndSubReason(Integer callEndSubReason) {
this.callEndSubReason = callEndSubReason;
this.changedFields = changedFields.add("callEndSubReason");
return this;
}
public Builder callerNumber(String callerNumber) {
this.callerNumber = callerNumber;
this.changedFields = changedFields.add("callerNumber");
return this;
}
public Builder callType(String callType) {
this.callType = callType;
this.changedFields = changedFields.add("callType");
return this;
}
public Builder correlationId(String correlationId) {
this.correlationId = correlationId;
this.changedFields = changedFields.add("correlationId");
return this;
}
public Builder duration(Integer duration) {
this.duration = duration;
this.changedFields = changedFields.add("duration");
return this;
}
public Builder endDateTime(OffsetDateTime endDateTime) {
this.endDateTime = endDateTime;
this.changedFields = changedFields.add("endDateTime");
return this;
}
public Builder failureDateTime(OffsetDateTime failureDateTime) {
this.failureDateTime = failureDateTime;
this.changedFields = changedFields.add("failureDateTime");
return this;
}
public Builder finalSipCode(Integer finalSipCode) {
this.finalSipCode = finalSipCode;
this.changedFields = changedFields.add("finalSipCode");
return this;
}
public Builder finalSipCodePhrase(String finalSipCodePhrase) {
this.finalSipCodePhrase = finalSipCodePhrase;
this.changedFields = changedFields.add("finalSipCodePhrase");
return this;
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder inviteDateTime(OffsetDateTime inviteDateTime) {
this.inviteDateTime = inviteDateTime;
this.changedFields = changedFields.add("inviteDateTime");
return this;
}
public Builder mediaBypassEnabled(Boolean mediaBypassEnabled) {
this.mediaBypassEnabled = mediaBypassEnabled;
this.changedFields = changedFields.add("mediaBypassEnabled");
return this;
}
public Builder mediaPathLocation(String mediaPathLocation) {
this.mediaPathLocation = mediaPathLocation;
this.changedFields = changedFields.add("mediaPathLocation");
return this;
}
public Builder signalingLocation(String signalingLocation) {
this.signalingLocation = signalingLocation;
this.changedFields = changedFields.add("signalingLocation");
return this;
}
public Builder startDateTime(OffsetDateTime startDateTime) {
this.startDateTime = startDateTime;
this.changedFields = changedFields.add("startDateTime");
return this;
}
public Builder successfulCall(Boolean successfulCall) {
this.successfulCall = successfulCall;
this.changedFields = changedFields.add("successfulCall");
return this;
}
public Builder trunkFullyQualifiedDomainName(String trunkFullyQualifiedDomainName) {
this.trunkFullyQualifiedDomainName = trunkFullyQualifiedDomainName;
this.changedFields = changedFields.add("trunkFullyQualifiedDomainName");
return this;
}
public Builder userDisplayName(String userDisplayName) {
this.userDisplayName = userDisplayName;
this.changedFields = changedFields.add("userDisplayName");
return this;
}
public Builder userId(String userId) {
this.userId = userId;
this.changedFields = changedFields.add("userId");
return this;
}
public Builder userPrincipalName(String userPrincipalName) {
this.userPrincipalName = userPrincipalName;
this.changedFields = changedFields.add("userPrincipalName");
return this;
}
public DirectRoutingLogRow build() {
DirectRoutingLogRow _x = new DirectRoutingLogRow();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.callRecords.directRoutingLogRow";
_x.calleeNumber = calleeNumber;
_x.callEndSubReason = callEndSubReason;
_x.callerNumber = callerNumber;
_x.callType = callType;
_x.correlationId = correlationId;
_x.duration = duration;
_x.endDateTime = endDateTime;
_x.failureDateTime = failureDateTime;
_x.finalSipCode = finalSipCode;
_x.finalSipCodePhrase = finalSipCodePhrase;
_x.id = id;
_x.inviteDateTime = inviteDateTime;
_x.mediaBypassEnabled = mediaBypassEnabled;
_x.mediaPathLocation = mediaPathLocation;
_x.signalingLocation = signalingLocation;
_x.startDateTime = startDateTime;
_x.successfulCall = successfulCall;
_x.trunkFullyQualifiedDomainName = trunkFullyQualifiedDomainName;
_x.userDisplayName = userDisplayName;
_x.userId = userId;
_x.userPrincipalName = userPrincipalName;
return _x;
}
}
private DirectRoutingLogRow _copy() {
DirectRoutingLogRow _x = new DirectRoutingLogRow();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.calleeNumber = calleeNumber;
_x.callEndSubReason = callEndSubReason;
_x.callerNumber = callerNumber;
_x.callType = callType;
_x.correlationId = correlationId;
_x.duration = duration;
_x.endDateTime = endDateTime;
_x.failureDateTime = failureDateTime;
_x.finalSipCode = finalSipCode;
_x.finalSipCodePhrase = finalSipCodePhrase;
_x.id = id;
_x.inviteDateTime = inviteDateTime;
_x.mediaBypassEnabled = mediaBypassEnabled;
_x.mediaPathLocation = mediaPathLocation;
_x.signalingLocation = signalingLocation;
_x.startDateTime = startDateTime;
_x.successfulCall = successfulCall;
_x.trunkFullyQualifiedDomainName = trunkFullyQualifiedDomainName;
_x.userDisplayName = userDisplayName;
_x.userId = userId;
_x.userPrincipalName = userPrincipalName;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("DirectRoutingLogRow[");
b.append("calleeNumber=");
b.append(this.calleeNumber);
b.append(", ");
b.append("callEndSubReason=");
b.append(this.callEndSubReason);
b.append(", ");
b.append("callerNumber=");
b.append(this.callerNumber);
b.append(", ");
b.append("callType=");
b.append(this.callType);
b.append(", ");
b.append("correlationId=");
b.append(this.correlationId);
b.append(", ");
b.append("duration=");
b.append(this.duration);
b.append(", ");
b.append("endDateTime=");
b.append(this.endDateTime);
b.append(", ");
b.append("failureDateTime=");
b.append(this.failureDateTime);
b.append(", ");
b.append("finalSipCode=");
b.append(this.finalSipCode);
b.append(", ");
b.append("finalSipCodePhrase=");
b.append(this.finalSipCodePhrase);
b.append(", ");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("inviteDateTime=");
b.append(this.inviteDateTime);
b.append(", ");
b.append("mediaBypassEnabled=");
b.append(this.mediaBypassEnabled);
b.append(", ");
b.append("mediaPathLocation=");
b.append(this.mediaPathLocation);
b.append(", ");
b.append("signalingLocation=");
b.append(this.signalingLocation);
b.append(", ");
b.append("startDateTime=");
b.append(this.startDateTime);
b.append(", ");
b.append("successfulCall=");
b.append(this.successfulCall);
b.append(", ");
b.append("trunkFullyQualifiedDomainName=");
b.append(this.trunkFullyQualifiedDomainName);
b.append(", ");
b.append("userDisplayName=");
b.append(this.userDisplayName);
b.append(", ");
b.append("userId=");
b.append(this.userId);
b.append(", ");
b.append("userPrincipalName=");
b.append(this.userPrincipalName);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy