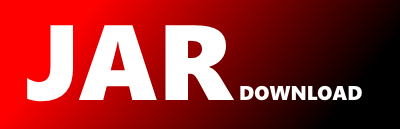
odata.msgraph.client.callrecords.entity.Session Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph Show documentation
Show all versions of odata-client-msgraph Show documentation
Java client for use with the Microsoft Graph v1.0 endpoint
package odata.msgraph.client.callrecords.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.callrecords.complex.Endpoint;
import odata.msgraph.client.callrecords.complex.FailureInfo;
import odata.msgraph.client.callrecords.entity.collection.request.SegmentCollectionRequest;
import odata.msgraph.client.callrecords.enums.Modality;
import odata.msgraph.client.entity.Entity;
@JsonPropertyOrder({
"@odata.type",
"callee",
"caller",
"endDateTime",
"failureInfo",
"isTest",
"modalities",
"startDateTime",
"segments"})
@JsonInclude(Include.NON_NULL)
public class Session extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.callRecords.session";
}
@JsonProperty("callee")
protected Endpoint callee;
@JsonProperty("caller")
protected Endpoint caller;
@JsonProperty("endDateTime")
protected OffsetDateTime endDateTime;
@JsonProperty("failureInfo")
protected FailureInfo failureInfo;
@JsonProperty("isTest")
protected Boolean isTest;
@JsonProperty("modalities")
protected List modalities;
@JsonProperty("modalities@nextLink")
protected String modalitiesNextLink;
@JsonProperty("startDateTime")
protected OffsetDateTime startDateTime;
@JsonProperty("segments")
protected List segments;
protected Session() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderSession() {
return new Builder();
}
public static final class Builder {
private String id;
private Endpoint callee;
private Endpoint caller;
private OffsetDateTime endDateTime;
private FailureInfo failureInfo;
private Boolean isTest;
private List modalities;
private String modalitiesNextLink;
private OffsetDateTime startDateTime;
private List segments;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder callee(Endpoint callee) {
this.callee = callee;
this.changedFields = changedFields.add("callee");
return this;
}
public Builder caller(Endpoint caller) {
this.caller = caller;
this.changedFields = changedFields.add("caller");
return this;
}
public Builder endDateTime(OffsetDateTime endDateTime) {
this.endDateTime = endDateTime;
this.changedFields = changedFields.add("endDateTime");
return this;
}
public Builder failureInfo(FailureInfo failureInfo) {
this.failureInfo = failureInfo;
this.changedFields = changedFields.add("failureInfo");
return this;
}
public Builder isTest(Boolean isTest) {
this.isTest = isTest;
this.changedFields = changedFields.add("isTest");
return this;
}
public Builder modalities(List modalities) {
this.modalities = modalities;
this.changedFields = changedFields.add("modalities");
return this;
}
public Builder modalities(Modality... modalities) {
return modalities(Arrays.asList(modalities));
}
public Builder modalitiesNextLink(String modalitiesNextLink) {
this.modalitiesNextLink = modalitiesNextLink;
this.changedFields = changedFields.add("modalities");
return this;
}
public Builder startDateTime(OffsetDateTime startDateTime) {
this.startDateTime = startDateTime;
this.changedFields = changedFields.add("startDateTime");
return this;
}
public Builder segments(List segments) {
this.segments = segments;
this.changedFields = changedFields.add("segments");
return this;
}
public Builder segments(Segment... segments) {
return segments(Arrays.asList(segments));
}
public Session build() {
Session _x = new Session();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.callRecords.session";
_x.id = id;
_x.callee = callee;
_x.caller = caller;
_x.endDateTime = endDateTime;
_x.failureInfo = failureInfo;
_x.isTest = isTest;
_x.modalities = modalities;
_x.modalitiesNextLink = modalitiesNextLink;
_x.startDateTime = startDateTime;
_x.segments = segments;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
@Property(name="callee")
@JsonIgnore
public Optional getCallee() {
return Optional.ofNullable(callee);
}
public Session withCallee(Endpoint callee) {
Session _x = _copy();
_x.changedFields = changedFields.add("callee");
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.session");
_x.callee = callee;
return _x;
}
@Property(name="caller")
@JsonIgnore
public Optional getCaller() {
return Optional.ofNullable(caller);
}
public Session withCaller(Endpoint caller) {
Session _x = _copy();
_x.changedFields = changedFields.add("caller");
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.session");
_x.caller = caller;
return _x;
}
@Property(name="endDateTime")
@JsonIgnore
public Optional getEndDateTime() {
return Optional.ofNullable(endDateTime);
}
public Session withEndDateTime(OffsetDateTime endDateTime) {
Session _x = _copy();
_x.changedFields = changedFields.add("endDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.session");
_x.endDateTime = endDateTime;
return _x;
}
@Property(name="failureInfo")
@JsonIgnore
public Optional getFailureInfo() {
return Optional.ofNullable(failureInfo);
}
public Session withFailureInfo(FailureInfo failureInfo) {
Session _x = _copy();
_x.changedFields = changedFields.add("failureInfo");
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.session");
_x.failureInfo = failureInfo;
return _x;
}
@Property(name="isTest")
@JsonIgnore
public Optional getIsTest() {
return Optional.ofNullable(isTest);
}
public Session withIsTest(Boolean isTest) {
Session _x = _copy();
_x.changedFields = changedFields.add("isTest");
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.session");
_x.isTest = isTest;
return _x;
}
@Property(name="modalities")
@JsonIgnore
public CollectionPage getModalities() {
return new CollectionPage(contextPath, Modality.class, this.modalities, Optional.ofNullable(modalitiesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public Session withModalities(List modalities) {
Session _x = _copy();
_x.changedFields = changedFields.add("modalities");
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.session");
_x.modalities = modalities;
return _x;
}
@Property(name="modalities")
@JsonIgnore
public CollectionPage getModalities(HttpRequestOptions options) {
return new CollectionPage(contextPath, Modality.class, this.modalities, Optional.ofNullable(modalitiesNextLink), Collections.emptyList(), options);
}
@Property(name="startDateTime")
@JsonIgnore
public Optional getStartDateTime() {
return Optional.ofNullable(startDateTime);
}
public Session withStartDateTime(OffsetDateTime startDateTime) {
Session _x = _copy();
_x.changedFields = changedFields.add("startDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.session");
_x.startDateTime = startDateTime;
return _x;
}
public Session withUnmappedField(String name, Object value) {
Session _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="segments")
@JsonIgnore
public SegmentCollectionRequest getSegments() {
return new SegmentCollectionRequest(
contextPath.addSegment("segments"), Optional.ofNullable(segments));
}
public Session withSegments(List segments) {
Session _x = _copy();
_x.changedFields = changedFields.add("segments");
_x.odataType = Util.nvl(odataType, "microsoft.graph.callRecords.session");
_x.segments = segments;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Session patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
Session _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public Session put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
Session _x = _copy();
_x.changedFields = null;
return _x;
}
private Session _copy() {
Session _x = new Session();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.callee = callee;
_x.caller = caller;
_x.endDateTime = endDateTime;
_x.failureInfo = failureInfo;
_x.isTest = isTest;
_x.modalities = modalities;
_x.startDateTime = startDateTime;
_x.segments = segments;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("Session[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("callee=");
b.append(this.callee);
b.append(", ");
b.append("caller=");
b.append(this.caller);
b.append(", ");
b.append("endDateTime=");
b.append(this.endDateTime);
b.append(", ");
b.append("failureInfo=");
b.append(this.failureInfo);
b.append(", ");
b.append("isTest=");
b.append(this.isTest);
b.append(", ");
b.append("modalities=");
b.append(this.modalities);
b.append(", ");
b.append("startDateTime=");
b.append(this.startDateTime);
b.append(", ");
b.append("segments=");
b.append(this.segments);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy