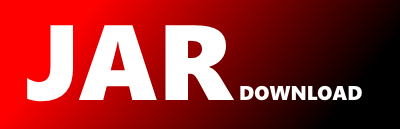
odata.msgraph.client.complex.CommunicationsPhoneIdentity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph Show documentation
Show all versions of odata-client-msgraph Show documentation
Java client for use with the Microsoft Graph v1.0 endpoint
package odata.msgraph.client.complex;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
@JsonPropertyOrder({
"@odata.type"})
@JsonInclude(Include.NON_NULL)
public class CommunicationsPhoneIdentity extends Identity implements ODataType {
protected CommunicationsPhoneIdentity() {
super();
}
@Override
public String odataTypeName() {
return "microsoft.graph.communicationsPhoneIdentity";
}
public CommunicationsPhoneIdentity withUnmappedField(String name, Object value) {
CommunicationsPhoneIdentity _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderCommunicationsPhoneIdentity() {
return new Builder();
}
public static final class Builder {
private String displayName;
private String id;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public CommunicationsPhoneIdentity build() {
CommunicationsPhoneIdentity _x = new CommunicationsPhoneIdentity();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.communicationsPhoneIdentity";
_x.displayName = displayName;
_x.id = id;
return _x;
}
}
private CommunicationsPhoneIdentity _copy() {
CommunicationsPhoneIdentity _x = new CommunicationsPhoneIdentity();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.displayName = displayName;
_x.id = id;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("CommunicationsPhoneIdentity[");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("id=");
b.append(this.id);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy