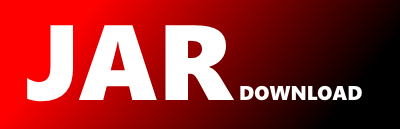
odata.msgraph.client.complex.DeviceProtectionOverview Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
/**
* “Hardware information of a given device.”
*/@JsonPropertyOrder({
"@odata.type",
"cleanDeviceCount",
"criticalFailuresDeviceCount",
"inactiveThreatAgentDeviceCount",
"pendingFullScanDeviceCount",
"pendingManualStepsDeviceCount",
"pendingOfflineScanDeviceCount",
"pendingQuickScanDeviceCount",
"pendingRestartDeviceCount",
"pendingSignatureUpdateDeviceCount",
"totalReportedDeviceCount",
"unknownStateThreatAgentDeviceCount"})
@JsonInclude(Include.NON_NULL)
public class DeviceProtectionOverview implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("cleanDeviceCount")
protected Integer cleanDeviceCount;
@JsonProperty("criticalFailuresDeviceCount")
protected Integer criticalFailuresDeviceCount;
@JsonProperty("inactiveThreatAgentDeviceCount")
protected Integer inactiveThreatAgentDeviceCount;
@JsonProperty("pendingFullScanDeviceCount")
protected Integer pendingFullScanDeviceCount;
@JsonProperty("pendingManualStepsDeviceCount")
protected Integer pendingManualStepsDeviceCount;
@JsonProperty("pendingOfflineScanDeviceCount")
protected Integer pendingOfflineScanDeviceCount;
@JsonProperty("pendingQuickScanDeviceCount")
protected Integer pendingQuickScanDeviceCount;
@JsonProperty("pendingRestartDeviceCount")
protected Integer pendingRestartDeviceCount;
@JsonProperty("pendingSignatureUpdateDeviceCount")
protected Integer pendingSignatureUpdateDeviceCount;
@JsonProperty("totalReportedDeviceCount")
protected Integer totalReportedDeviceCount;
@JsonProperty("unknownStateThreatAgentDeviceCount")
protected Integer unknownStateThreatAgentDeviceCount;
protected DeviceProtectionOverview() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.deviceProtectionOverview";
}
/**
* “Indicates number of devices reporting as clean”
*
* @return property cleanDeviceCount
*/
@Property(name="cleanDeviceCount")
@JsonIgnore
public Optional getCleanDeviceCount() {
return Optional.ofNullable(cleanDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code cleanDeviceCount}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Indicates number of devices reporting as clean”
*
* @param cleanDeviceCount
* new value of {@code cleanDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code cleanDeviceCount} field changed
*/
public DeviceProtectionOverview withCleanDeviceCount(Integer cleanDeviceCount) {
DeviceProtectionOverview _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceProtectionOverview");
_x.cleanDeviceCount = cleanDeviceCount;
return _x;
}
/**
* “Indicates number of devices with critical failures”
*
* @return property criticalFailuresDeviceCount
*/
@Property(name="criticalFailuresDeviceCount")
@JsonIgnore
public Optional getCriticalFailuresDeviceCount() {
return Optional.ofNullable(criticalFailuresDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* criticalFailuresDeviceCount} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates number of devices with critical failures”
*
* @param criticalFailuresDeviceCount
* new value of {@code criticalFailuresDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code criticalFailuresDeviceCount} field changed
*/
public DeviceProtectionOverview withCriticalFailuresDeviceCount(Integer criticalFailuresDeviceCount) {
DeviceProtectionOverview _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceProtectionOverview");
_x.criticalFailuresDeviceCount = criticalFailuresDeviceCount;
return _x;
}
/**
* “Indicates number of devices with inactive threat agent”
*
* @return property inactiveThreatAgentDeviceCount
*/
@Property(name="inactiveThreatAgentDeviceCount")
@JsonIgnore
public Optional getInactiveThreatAgentDeviceCount() {
return Optional.ofNullable(inactiveThreatAgentDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* inactiveThreatAgentDeviceCount} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Indicates number of devices with inactive threat agent”
*
* @param inactiveThreatAgentDeviceCount
* new value of {@code inactiveThreatAgentDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code inactiveThreatAgentDeviceCount} field changed
*/
public DeviceProtectionOverview withInactiveThreatAgentDeviceCount(Integer inactiveThreatAgentDeviceCount) {
DeviceProtectionOverview _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceProtectionOverview");
_x.inactiveThreatAgentDeviceCount = inactiveThreatAgentDeviceCount;
return _x;
}
/**
* “Indicates number of devices pending full scan”
*
* @return property pendingFullScanDeviceCount
*/
@Property(name="pendingFullScanDeviceCount")
@JsonIgnore
public Optional getPendingFullScanDeviceCount() {
return Optional.ofNullable(pendingFullScanDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* pendingFullScanDeviceCount} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates number of devices pending full scan”
*
* @param pendingFullScanDeviceCount
* new value of {@code pendingFullScanDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code pendingFullScanDeviceCount} field changed
*/
public DeviceProtectionOverview withPendingFullScanDeviceCount(Integer pendingFullScanDeviceCount) {
DeviceProtectionOverview _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceProtectionOverview");
_x.pendingFullScanDeviceCount = pendingFullScanDeviceCount;
return _x;
}
/**
* “Indicates number of devices with pending manual steps”
*
* @return property pendingManualStepsDeviceCount
*/
@Property(name="pendingManualStepsDeviceCount")
@JsonIgnore
public Optional getPendingManualStepsDeviceCount() {
return Optional.ofNullable(pendingManualStepsDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* pendingManualStepsDeviceCount} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates number of devices with pending manual steps”
*
* @param pendingManualStepsDeviceCount
* new value of {@code pendingManualStepsDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code pendingManualStepsDeviceCount} field changed
*/
public DeviceProtectionOverview withPendingManualStepsDeviceCount(Integer pendingManualStepsDeviceCount) {
DeviceProtectionOverview _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceProtectionOverview");
_x.pendingManualStepsDeviceCount = pendingManualStepsDeviceCount;
return _x;
}
/**
* “Indicates number of pending offline scan devices”
*
* @return property pendingOfflineScanDeviceCount
*/
@Property(name="pendingOfflineScanDeviceCount")
@JsonIgnore
public Optional getPendingOfflineScanDeviceCount() {
return Optional.ofNullable(pendingOfflineScanDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* pendingOfflineScanDeviceCount} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates number of pending offline scan devices”
*
* @param pendingOfflineScanDeviceCount
* new value of {@code pendingOfflineScanDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code pendingOfflineScanDeviceCount} field changed
*/
public DeviceProtectionOverview withPendingOfflineScanDeviceCount(Integer pendingOfflineScanDeviceCount) {
DeviceProtectionOverview _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceProtectionOverview");
_x.pendingOfflineScanDeviceCount = pendingOfflineScanDeviceCount;
return _x;
}
/**
* “Indicates the number of devices that have a pending full scan. Valid values -
* 2147483648 to 2147483647”
*
* @return property pendingQuickScanDeviceCount
*/
@Property(name="pendingQuickScanDeviceCount")
@JsonIgnore
public Optional getPendingQuickScanDeviceCount() {
return Optional.ofNullable(pendingQuickScanDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* pendingQuickScanDeviceCount} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates the number of devices that have a pending full scan. Valid values -
* 2147483648 to 2147483647”
*
* @param pendingQuickScanDeviceCount
* new value of {@code pendingQuickScanDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code pendingQuickScanDeviceCount} field changed
*/
public DeviceProtectionOverview withPendingQuickScanDeviceCount(Integer pendingQuickScanDeviceCount) {
DeviceProtectionOverview _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceProtectionOverview");
_x.pendingQuickScanDeviceCount = pendingQuickScanDeviceCount;
return _x;
}
/**
* “Indicates number of devices pending restart”
*
* @return property pendingRestartDeviceCount
*/
@Property(name="pendingRestartDeviceCount")
@JsonIgnore
public Optional getPendingRestartDeviceCount() {
return Optional.ofNullable(pendingRestartDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* pendingRestartDeviceCount} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Indicates number of devices pending restart”
*
* @param pendingRestartDeviceCount
* new value of {@code pendingRestartDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code pendingRestartDeviceCount} field changed
*/
public DeviceProtectionOverview withPendingRestartDeviceCount(Integer pendingRestartDeviceCount) {
DeviceProtectionOverview _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceProtectionOverview");
_x.pendingRestartDeviceCount = pendingRestartDeviceCount;
return _x;
}
/**
* “Indicates number of devices with an old signature”
*
* @return property pendingSignatureUpdateDeviceCount
*/
@Property(name="pendingSignatureUpdateDeviceCount")
@JsonIgnore
public Optional getPendingSignatureUpdateDeviceCount() {
return Optional.ofNullable(pendingSignatureUpdateDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* pendingSignatureUpdateDeviceCount} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Indicates number of devices with an old signature”
*
* @param pendingSignatureUpdateDeviceCount
* new value of {@code pendingSignatureUpdateDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code pendingSignatureUpdateDeviceCount} field changed
*/
public DeviceProtectionOverview withPendingSignatureUpdateDeviceCount(Integer pendingSignatureUpdateDeviceCount) {
DeviceProtectionOverview _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceProtectionOverview");
_x.pendingSignatureUpdateDeviceCount = pendingSignatureUpdateDeviceCount;
return _x;
}
/**
* “Total device count.”
*
* @return property totalReportedDeviceCount
*/
@Property(name="totalReportedDeviceCount")
@JsonIgnore
public Optional getTotalReportedDeviceCount() {
return Optional.ofNullable(totalReportedDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* totalReportedDeviceCount} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Total device count.”
*
* @param totalReportedDeviceCount
* new value of {@code totalReportedDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code totalReportedDeviceCount} field changed
*/
public DeviceProtectionOverview withTotalReportedDeviceCount(Integer totalReportedDeviceCount) {
DeviceProtectionOverview _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceProtectionOverview");
_x.totalReportedDeviceCount = totalReportedDeviceCount;
return _x;
}
/**
* “Indicates number of devices with threat agent state as unknown”
*
* @return property unknownStateThreatAgentDeviceCount
*/
@Property(name="unknownStateThreatAgentDeviceCount")
@JsonIgnore
public Optional getUnknownStateThreatAgentDeviceCount() {
return Optional.ofNullable(unknownStateThreatAgentDeviceCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* unknownStateThreatAgentDeviceCount} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Indicates number of devices with threat agent state as unknown”
*
* @param unknownStateThreatAgentDeviceCount
* new value of {@code unknownStateThreatAgentDeviceCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code unknownStateThreatAgentDeviceCount} field changed
*/
public DeviceProtectionOverview withUnknownStateThreatAgentDeviceCount(Integer unknownStateThreatAgentDeviceCount) {
DeviceProtectionOverview _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceProtectionOverview");
_x.unknownStateThreatAgentDeviceCount = unknownStateThreatAgentDeviceCount;
return _x;
}
public DeviceProtectionOverview withUnmappedField(String name, Object value) {
DeviceProtectionOverview _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private Integer cleanDeviceCount;
private Integer criticalFailuresDeviceCount;
private Integer inactiveThreatAgentDeviceCount;
private Integer pendingFullScanDeviceCount;
private Integer pendingManualStepsDeviceCount;
private Integer pendingOfflineScanDeviceCount;
private Integer pendingQuickScanDeviceCount;
private Integer pendingRestartDeviceCount;
private Integer pendingSignatureUpdateDeviceCount;
private Integer totalReportedDeviceCount;
private Integer unknownStateThreatAgentDeviceCount;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
/**
* “Indicates number of devices reporting as clean”
*
* @param cleanDeviceCount
* value of {@code cleanDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder cleanDeviceCount(Integer cleanDeviceCount) {
this.cleanDeviceCount = cleanDeviceCount;
this.changedFields = changedFields.add("cleanDeviceCount");
return this;
}
/**
* “Indicates number of devices with critical failures”
*
* @param criticalFailuresDeviceCount
* value of {@code criticalFailuresDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder criticalFailuresDeviceCount(Integer criticalFailuresDeviceCount) {
this.criticalFailuresDeviceCount = criticalFailuresDeviceCount;
this.changedFields = changedFields.add("criticalFailuresDeviceCount");
return this;
}
/**
* “Indicates number of devices with inactive threat agent”
*
* @param inactiveThreatAgentDeviceCount
* value of {@code inactiveThreatAgentDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder inactiveThreatAgentDeviceCount(Integer inactiveThreatAgentDeviceCount) {
this.inactiveThreatAgentDeviceCount = inactiveThreatAgentDeviceCount;
this.changedFields = changedFields.add("inactiveThreatAgentDeviceCount");
return this;
}
/**
* “Indicates number of devices pending full scan”
*
* @param pendingFullScanDeviceCount
* value of {@code pendingFullScanDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder pendingFullScanDeviceCount(Integer pendingFullScanDeviceCount) {
this.pendingFullScanDeviceCount = pendingFullScanDeviceCount;
this.changedFields = changedFields.add("pendingFullScanDeviceCount");
return this;
}
/**
* “Indicates number of devices with pending manual steps”
*
* @param pendingManualStepsDeviceCount
* value of {@code pendingManualStepsDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder pendingManualStepsDeviceCount(Integer pendingManualStepsDeviceCount) {
this.pendingManualStepsDeviceCount = pendingManualStepsDeviceCount;
this.changedFields = changedFields.add("pendingManualStepsDeviceCount");
return this;
}
/**
* “Indicates number of pending offline scan devices”
*
* @param pendingOfflineScanDeviceCount
* value of {@code pendingOfflineScanDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder pendingOfflineScanDeviceCount(Integer pendingOfflineScanDeviceCount) {
this.pendingOfflineScanDeviceCount = pendingOfflineScanDeviceCount;
this.changedFields = changedFields.add("pendingOfflineScanDeviceCount");
return this;
}
/**
* “Indicates the number of devices that have a pending full scan. Valid values -
* 2147483648 to 2147483647”
*
* @param pendingQuickScanDeviceCount
* value of {@code pendingQuickScanDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder pendingQuickScanDeviceCount(Integer pendingQuickScanDeviceCount) {
this.pendingQuickScanDeviceCount = pendingQuickScanDeviceCount;
this.changedFields = changedFields.add("pendingQuickScanDeviceCount");
return this;
}
/**
* “Indicates number of devices pending restart”
*
* @param pendingRestartDeviceCount
* value of {@code pendingRestartDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder pendingRestartDeviceCount(Integer pendingRestartDeviceCount) {
this.pendingRestartDeviceCount = pendingRestartDeviceCount;
this.changedFields = changedFields.add("pendingRestartDeviceCount");
return this;
}
/**
* “Indicates number of devices with an old signature”
*
* @param pendingSignatureUpdateDeviceCount
* value of {@code pendingSignatureUpdateDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder pendingSignatureUpdateDeviceCount(Integer pendingSignatureUpdateDeviceCount) {
this.pendingSignatureUpdateDeviceCount = pendingSignatureUpdateDeviceCount;
this.changedFields = changedFields.add("pendingSignatureUpdateDeviceCount");
return this;
}
/**
* “Total device count.”
*
* @param totalReportedDeviceCount
* value of {@code totalReportedDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder totalReportedDeviceCount(Integer totalReportedDeviceCount) {
this.totalReportedDeviceCount = totalReportedDeviceCount;
this.changedFields = changedFields.add("totalReportedDeviceCount");
return this;
}
/**
* “Indicates number of devices with threat agent state as unknown”
*
* @param unknownStateThreatAgentDeviceCount
* value of {@code unknownStateThreatAgentDeviceCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder unknownStateThreatAgentDeviceCount(Integer unknownStateThreatAgentDeviceCount) {
this.unknownStateThreatAgentDeviceCount = unknownStateThreatAgentDeviceCount;
this.changedFields = changedFields.add("unknownStateThreatAgentDeviceCount");
return this;
}
public DeviceProtectionOverview build() {
DeviceProtectionOverview _x = new DeviceProtectionOverview();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.deviceProtectionOverview";
_x.cleanDeviceCount = cleanDeviceCount;
_x.criticalFailuresDeviceCount = criticalFailuresDeviceCount;
_x.inactiveThreatAgentDeviceCount = inactiveThreatAgentDeviceCount;
_x.pendingFullScanDeviceCount = pendingFullScanDeviceCount;
_x.pendingManualStepsDeviceCount = pendingManualStepsDeviceCount;
_x.pendingOfflineScanDeviceCount = pendingOfflineScanDeviceCount;
_x.pendingQuickScanDeviceCount = pendingQuickScanDeviceCount;
_x.pendingRestartDeviceCount = pendingRestartDeviceCount;
_x.pendingSignatureUpdateDeviceCount = pendingSignatureUpdateDeviceCount;
_x.totalReportedDeviceCount = totalReportedDeviceCount;
_x.unknownStateThreatAgentDeviceCount = unknownStateThreatAgentDeviceCount;
return _x;
}
}
private DeviceProtectionOverview _copy() {
DeviceProtectionOverview _x = new DeviceProtectionOverview();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.cleanDeviceCount = cleanDeviceCount;
_x.criticalFailuresDeviceCount = criticalFailuresDeviceCount;
_x.inactiveThreatAgentDeviceCount = inactiveThreatAgentDeviceCount;
_x.pendingFullScanDeviceCount = pendingFullScanDeviceCount;
_x.pendingManualStepsDeviceCount = pendingManualStepsDeviceCount;
_x.pendingOfflineScanDeviceCount = pendingOfflineScanDeviceCount;
_x.pendingQuickScanDeviceCount = pendingQuickScanDeviceCount;
_x.pendingRestartDeviceCount = pendingRestartDeviceCount;
_x.pendingSignatureUpdateDeviceCount = pendingSignatureUpdateDeviceCount;
_x.totalReportedDeviceCount = totalReportedDeviceCount;
_x.unknownStateThreatAgentDeviceCount = unknownStateThreatAgentDeviceCount;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("DeviceProtectionOverview[");
b.append("cleanDeviceCount=");
b.append(this.cleanDeviceCount);
b.append(", ");
b.append("criticalFailuresDeviceCount=");
b.append(this.criticalFailuresDeviceCount);
b.append(", ");
b.append("inactiveThreatAgentDeviceCount=");
b.append(this.inactiveThreatAgentDeviceCount);
b.append(", ");
b.append("pendingFullScanDeviceCount=");
b.append(this.pendingFullScanDeviceCount);
b.append(", ");
b.append("pendingManualStepsDeviceCount=");
b.append(this.pendingManualStepsDeviceCount);
b.append(", ");
b.append("pendingOfflineScanDeviceCount=");
b.append(this.pendingOfflineScanDeviceCount);
b.append(", ");
b.append("pendingQuickScanDeviceCount=");
b.append(this.pendingQuickScanDeviceCount);
b.append(", ");
b.append("pendingRestartDeviceCount=");
b.append(this.pendingRestartDeviceCount);
b.append(", ");
b.append("pendingSignatureUpdateDeviceCount=");
b.append(this.pendingSignatureUpdateDeviceCount);
b.append(", ");
b.append("totalReportedDeviceCount=");
b.append(this.totalReportedDeviceCount);
b.append(", ");
b.append("unknownStateThreatAgentDeviceCount=");
b.append(this.unknownStateThreatAgentDeviceCount);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}