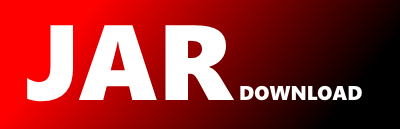
odata.msgraph.client.complex.MacOSMinimumOperatingSystem Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
/**
* “The minimum operating system required for a macOS app.”
*/@JsonPropertyOrder({
"@odata.type",
"v10_10",
"v10_11",
"v10_12",
"v10_13",
"v10_14",
"v10_15",
"v10_7",
"v10_8",
"v10_9",
"v11_0",
"v12_0",
"v13_0"})
@JsonInclude(Include.NON_NULL)
public class MacOSMinimumOperatingSystem implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("v10_10")
protected Boolean v10_10;
@JsonProperty("v10_11")
protected Boolean v10_11;
@JsonProperty("v10_12")
protected Boolean v10_12;
@JsonProperty("v10_13")
protected Boolean v10_13;
@JsonProperty("v10_14")
protected Boolean v10_14;
@JsonProperty("v10_15")
protected Boolean v10_15;
@JsonProperty("v10_7")
protected Boolean v10_7;
@JsonProperty("v10_8")
protected Boolean v10_8;
@JsonProperty("v10_9")
protected Boolean v10_9;
@JsonProperty("v11_0")
protected Boolean v11_0;
@JsonProperty("v12_0")
protected Boolean v12_0;
@JsonProperty("v13_0")
protected Boolean v13_0;
protected MacOSMinimumOperatingSystem() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.macOSMinimumOperatingSystem";
}
/**
* “When TRUE, indicates OS X 10.10 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @return property v10_10
*/
@Property(name="v10_10")
@JsonIgnore
public Optional getV10_10() {
return Optional.ofNullable(v10_10);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_10} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates OS X 10.10 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_10
* new value of {@code v10_10} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_10} field changed
*/
public MacOSMinimumOperatingSystem withV10_10(Boolean v10_10) {
MacOSMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSMinimumOperatingSystem");
_x.v10_10 = v10_10;
return _x;
}
/**
* “When TRUE, indicates OS X 10.11 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @return property v10_11
*/
@Property(name="v10_11")
@JsonIgnore
public Optional getV10_11() {
return Optional.ofNullable(v10_11);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_11} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates OS X 10.11 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_11
* new value of {@code v10_11} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_11} field changed
*/
public MacOSMinimumOperatingSystem withV10_11(Boolean v10_11) {
MacOSMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSMinimumOperatingSystem");
_x.v10_11 = v10_11;
return _x;
}
/**
* “When TRUE, indicates macOS 10.12 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @return property v10_12
*/
@Property(name="v10_12")
@JsonIgnore
public Optional getV10_12() {
return Optional.ofNullable(v10_12);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_12} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates macOS 10.12 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_12
* new value of {@code v10_12} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_12} field changed
*/
public MacOSMinimumOperatingSystem withV10_12(Boolean v10_12) {
MacOSMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSMinimumOperatingSystem");
_x.v10_12 = v10_12;
return _x;
}
/**
* “When TRUE, indicates macOS 10.13 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @return property v10_13
*/
@Property(name="v10_13")
@JsonIgnore
public Optional getV10_13() {
return Optional.ofNullable(v10_13);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_13} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates macOS 10.13 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_13
* new value of {@code v10_13} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_13} field changed
*/
public MacOSMinimumOperatingSystem withV10_13(Boolean v10_13) {
MacOSMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSMinimumOperatingSystem");
_x.v10_13 = v10_13;
return _x;
}
/**
* “When TRUE, indicates macOS 10.14 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @return property v10_14
*/
@Property(name="v10_14")
@JsonIgnore
public Optional getV10_14() {
return Optional.ofNullable(v10_14);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_14} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates macOS 10.14 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_14
* new value of {@code v10_14} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_14} field changed
*/
public MacOSMinimumOperatingSystem withV10_14(Boolean v10_14) {
MacOSMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSMinimumOperatingSystem");
_x.v10_14 = v10_14;
return _x;
}
/**
* “When TRUE, indicates macOS 10.15 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @return property v10_15
*/
@Property(name="v10_15")
@JsonIgnore
public Optional getV10_15() {
return Optional.ofNullable(v10_15);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_15} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates macOS 10.15 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_15
* new value of {@code v10_15} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_15} field changed
*/
public MacOSMinimumOperatingSystem withV10_15(Boolean v10_15) {
MacOSMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSMinimumOperatingSystem");
_x.v10_15 = v10_15;
return _x;
}
/**
* “When TRUE, indicates Mac OS X 10.7 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @return property v10_7
*/
@Property(name="v10_7")
@JsonIgnore
public Optional getV10_7() {
return Optional.ofNullable(v10_7);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_7} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates Mac OS X 10.7 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_7
* new value of {@code v10_7} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_7} field changed
*/
public MacOSMinimumOperatingSystem withV10_7(Boolean v10_7) {
MacOSMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSMinimumOperatingSystem");
_x.v10_7 = v10_7;
return _x;
}
/**
* “When TRUE, indicates OS X 10.8 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @return property v10_8
*/
@Property(name="v10_8")
@JsonIgnore
public Optional getV10_8() {
return Optional.ofNullable(v10_8);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_8} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates OS X 10.8 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_8
* new value of {@code v10_8} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_8} field changed
*/
public MacOSMinimumOperatingSystem withV10_8(Boolean v10_8) {
MacOSMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSMinimumOperatingSystem");
_x.v10_8 = v10_8;
return _x;
}
/**
* “When TRUE, indicates OS X 10.9 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @return property v10_9
*/
@Property(name="v10_9")
@JsonIgnore
public Optional getV10_9() {
return Optional.ofNullable(v10_9);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v10_9} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates OS X 10.9 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_9
* new value of {@code v10_9} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v10_9} field changed
*/
public MacOSMinimumOperatingSystem withV10_9(Boolean v10_9) {
MacOSMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSMinimumOperatingSystem");
_x.v10_9 = v10_9;
return _x;
}
/**
* “When TRUE, indicates macOS 11.0 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @return property v11_0
*/
@Property(name="v11_0")
@JsonIgnore
public Optional getV11_0() {
return Optional.ofNullable(v11_0);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v11_0} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates macOS 11.0 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v11_0
* new value of {@code v11_0} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v11_0} field changed
*/
public MacOSMinimumOperatingSystem withV11_0(Boolean v11_0) {
MacOSMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSMinimumOperatingSystem");
_x.v11_0 = v11_0;
return _x;
}
/**
* “When TRUE, indicates macOS 12.0 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @return property v12_0
*/
@Property(name="v12_0")
@JsonIgnore
public Optional getV12_0() {
return Optional.ofNullable(v12_0);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v12_0} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates macOS 12.0 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v12_0
* new value of {@code v12_0} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v12_0} field changed
*/
public MacOSMinimumOperatingSystem withV12_0(Boolean v12_0) {
MacOSMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSMinimumOperatingSystem");
_x.v12_0 = v12_0;
return _x;
}
/**
* “When TRUE, indicates macOS 13.0 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @return property v13_0
*/
@Property(name="v13_0")
@JsonIgnore
public Optional getV13_0() {
return Optional.ofNullable(v13_0);
}
/**
* Returns an immutable copy of {@code this} with just the {@code v13_0} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “When TRUE, indicates macOS 13.0 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v13_0
* new value of {@code v13_0} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code v13_0} field changed
*/
public MacOSMinimumOperatingSystem withV13_0(Boolean v13_0) {
MacOSMinimumOperatingSystem _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.macOSMinimumOperatingSystem");
_x.v13_0 = v13_0;
return _x;
}
public MacOSMinimumOperatingSystem withUnmappedField(String name, Object value) {
MacOSMinimumOperatingSystem _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private Boolean v10_10;
private Boolean v10_11;
private Boolean v10_12;
private Boolean v10_13;
private Boolean v10_14;
private Boolean v10_15;
private Boolean v10_7;
private Boolean v10_8;
private Boolean v10_9;
private Boolean v11_0;
private Boolean v12_0;
private Boolean v13_0;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
/**
* “When TRUE, indicates OS X 10.10 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_10
* value of {@code v10_10} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_10(Boolean v10_10) {
this.v10_10 = v10_10;
this.changedFields = changedFields.add("v10_10");
return this;
}
/**
* “When TRUE, indicates OS X 10.11 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_11
* value of {@code v10_11} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_11(Boolean v10_11) {
this.v10_11 = v10_11;
this.changedFields = changedFields.add("v10_11");
return this;
}
/**
* “When TRUE, indicates macOS 10.12 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_12
* value of {@code v10_12} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_12(Boolean v10_12) {
this.v10_12 = v10_12;
this.changedFields = changedFields.add("v10_12");
return this;
}
/**
* “When TRUE, indicates macOS 10.13 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_13
* value of {@code v10_13} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_13(Boolean v10_13) {
this.v10_13 = v10_13;
this.changedFields = changedFields.add("v10_13");
return this;
}
/**
* “When TRUE, indicates macOS 10.14 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_14
* value of {@code v10_14} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_14(Boolean v10_14) {
this.v10_14 = v10_14;
this.changedFields = changedFields.add("v10_14");
return this;
}
/**
* “When TRUE, indicates macOS 10.15 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_15
* value of {@code v10_15} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_15(Boolean v10_15) {
this.v10_15 = v10_15;
this.changedFields = changedFields.add("v10_15");
return this;
}
/**
* “When TRUE, indicates Mac OS X 10.7 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_7
* value of {@code v10_7} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_7(Boolean v10_7) {
this.v10_7 = v10_7;
this.changedFields = changedFields.add("v10_7");
return this;
}
/**
* “When TRUE, indicates OS X 10.8 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_8
* value of {@code v10_8} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_8(Boolean v10_8) {
this.v10_8 = v10_8;
this.changedFields = changedFields.add("v10_8");
return this;
}
/**
* “When TRUE, indicates OS X 10.9 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v10_9
* value of {@code v10_9} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v10_9(Boolean v10_9) {
this.v10_9 = v10_9;
this.changedFields = changedFields.add("v10_9");
return this;
}
/**
* “When TRUE, indicates macOS 11.0 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v11_0
* value of {@code v11_0} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v11_0(Boolean v11_0) {
this.v11_0 = v11_0;
this.changedFields = changedFields.add("v11_0");
return this;
}
/**
* “When TRUE, indicates macOS 12.0 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v12_0
* value of {@code v12_0} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v12_0(Boolean v12_0) {
this.v12_0 = v12_0;
this.changedFields = changedFields.add("v12_0");
return this;
}
/**
* “When TRUE, indicates macOS 13.0 or later is required to install the app. When
* FALSE, indicates some other OS version is the minimum OS to install the app.
* Default value is FALSE.”
*
* @param v13_0
* value of {@code v13_0} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder v13_0(Boolean v13_0) {
this.v13_0 = v13_0;
this.changedFields = changedFields.add("v13_0");
return this;
}
public MacOSMinimumOperatingSystem build() {
MacOSMinimumOperatingSystem _x = new MacOSMinimumOperatingSystem();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.macOSMinimumOperatingSystem";
_x.v10_10 = v10_10;
_x.v10_11 = v10_11;
_x.v10_12 = v10_12;
_x.v10_13 = v10_13;
_x.v10_14 = v10_14;
_x.v10_15 = v10_15;
_x.v10_7 = v10_7;
_x.v10_8 = v10_8;
_x.v10_9 = v10_9;
_x.v11_0 = v11_0;
_x.v12_0 = v12_0;
_x.v13_0 = v13_0;
return _x;
}
}
private MacOSMinimumOperatingSystem _copy() {
MacOSMinimumOperatingSystem _x = new MacOSMinimumOperatingSystem();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.v10_10 = v10_10;
_x.v10_11 = v10_11;
_x.v10_12 = v10_12;
_x.v10_13 = v10_13;
_x.v10_14 = v10_14;
_x.v10_15 = v10_15;
_x.v10_7 = v10_7;
_x.v10_8 = v10_8;
_x.v10_9 = v10_9;
_x.v11_0 = v11_0;
_x.v12_0 = v12_0;
_x.v13_0 = v13_0;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("MacOSMinimumOperatingSystem[");
b.append("v10_10=");
b.append(this.v10_10);
b.append(", ");
b.append("v10_11=");
b.append(this.v10_11);
b.append(", ");
b.append("v10_12=");
b.append(this.v10_12);
b.append(", ");
b.append("v10_13=");
b.append(this.v10_13);
b.append(", ");
b.append("v10_14=");
b.append(this.v10_14);
b.append(", ");
b.append("v10_15=");
b.append(this.v10_15);
b.append(", ");
b.append("v10_7=");
b.append(this.v10_7);
b.append(", ");
b.append("v10_8=");
b.append(this.v10_8);
b.append(", ");
b.append("v10_9=");
b.append(this.v10_9);
b.append(", ");
b.append("v11_0=");
b.append(this.v11_0);
b.append(", ");
b.append("v12_0=");
b.append(this.v12_0);
b.append(", ");
b.append("v13_0=");
b.append(this.v13_0);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}