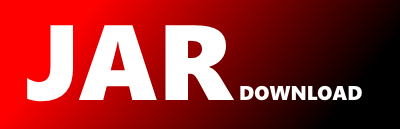
odata.msgraph.client.complex.SubjectRightsRequestDetail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph Show documentation
Show all versions of odata-client-msgraph Show documentation
Java client for use with the Microsoft Graph v1.0 endpoint
package odata.msgraph.client.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
@JsonPropertyOrder({
"@odata.type",
"excludedItemCount",
"insightCounts",
"itemCount",
"itemNeedReview",
"productItemCounts",
"signedOffItemCount",
"totalItemSize"})
@JsonInclude(Include.NON_NULL)
public class SubjectRightsRequestDetail implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("excludedItemCount")
protected Long excludedItemCount;
@JsonProperty("insightCounts")
protected List insightCounts;
@JsonProperty("insightCounts@nextLink")
protected String insightCountsNextLink;
@JsonProperty("itemCount")
protected Long itemCount;
@JsonProperty("itemNeedReview")
protected Long itemNeedReview;
@JsonProperty("productItemCounts")
protected List productItemCounts;
@JsonProperty("productItemCounts@nextLink")
protected String productItemCountsNextLink;
@JsonProperty("signedOffItemCount")
protected Long signedOffItemCount;
@JsonProperty("totalItemSize")
protected Long totalItemSize;
protected SubjectRightsRequestDetail() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.subjectRightsRequestDetail";
}
@Property(name="excludedItemCount")
@JsonIgnore
public Optional getExcludedItemCount() {
return Optional.ofNullable(excludedItemCount);
}
public SubjectRightsRequestDetail withExcludedItemCount(Long excludedItemCount) {
SubjectRightsRequestDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.subjectRightsRequestDetail");
_x.excludedItemCount = excludedItemCount;
return _x;
}
@Property(name="insightCounts")
@JsonIgnore
public CollectionPage getInsightCounts() {
return new CollectionPage(contextPath, KeyValuePair.class, this.insightCounts, Optional.ofNullable(insightCountsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="insightCounts")
@JsonIgnore
public CollectionPage getInsightCounts(HttpRequestOptions options) {
return new CollectionPage(contextPath, KeyValuePair.class, this.insightCounts, Optional.ofNullable(insightCountsNextLink), Collections.emptyList(), options);
}
@Property(name="itemCount")
@JsonIgnore
public Optional getItemCount() {
return Optional.ofNullable(itemCount);
}
public SubjectRightsRequestDetail withItemCount(Long itemCount) {
SubjectRightsRequestDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.subjectRightsRequestDetail");
_x.itemCount = itemCount;
return _x;
}
@Property(name="itemNeedReview")
@JsonIgnore
public Optional getItemNeedReview() {
return Optional.ofNullable(itemNeedReview);
}
public SubjectRightsRequestDetail withItemNeedReview(Long itemNeedReview) {
SubjectRightsRequestDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.subjectRightsRequestDetail");
_x.itemNeedReview = itemNeedReview;
return _x;
}
@Property(name="productItemCounts")
@JsonIgnore
public CollectionPage getProductItemCounts() {
return new CollectionPage(contextPath, KeyValuePair.class, this.productItemCounts, Optional.ofNullable(productItemCountsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
@Property(name="productItemCounts")
@JsonIgnore
public CollectionPage getProductItemCounts(HttpRequestOptions options) {
return new CollectionPage(contextPath, KeyValuePair.class, this.productItemCounts, Optional.ofNullable(productItemCountsNextLink), Collections.emptyList(), options);
}
@Property(name="signedOffItemCount")
@JsonIgnore
public Optional getSignedOffItemCount() {
return Optional.ofNullable(signedOffItemCount);
}
public SubjectRightsRequestDetail withSignedOffItemCount(Long signedOffItemCount) {
SubjectRightsRequestDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.subjectRightsRequestDetail");
_x.signedOffItemCount = signedOffItemCount;
return _x;
}
@Property(name="totalItemSize")
@JsonIgnore
public Optional getTotalItemSize() {
return Optional.ofNullable(totalItemSize);
}
public SubjectRightsRequestDetail withTotalItemSize(Long totalItemSize) {
SubjectRightsRequestDetail _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.subjectRightsRequestDetail");
_x.totalItemSize = totalItemSize;
return _x;
}
public SubjectRightsRequestDetail withUnmappedField(String name, Object value) {
SubjectRightsRequestDetail _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private Long excludedItemCount;
private List insightCounts;
private String insightCountsNextLink;
private Long itemCount;
private Long itemNeedReview;
private List productItemCounts;
private String productItemCountsNextLink;
private Long signedOffItemCount;
private Long totalItemSize;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder excludedItemCount(Long excludedItemCount) {
this.excludedItemCount = excludedItemCount;
this.changedFields = changedFields.add("excludedItemCount");
return this;
}
public Builder insightCounts(List insightCounts) {
this.insightCounts = insightCounts;
this.changedFields = changedFields.add("insightCounts");
return this;
}
public Builder insightCounts(KeyValuePair... insightCounts) {
return insightCounts(Arrays.asList(insightCounts));
}
public Builder insightCountsNextLink(String insightCountsNextLink) {
this.insightCountsNextLink = insightCountsNextLink;
this.changedFields = changedFields.add("insightCounts");
return this;
}
public Builder itemCount(Long itemCount) {
this.itemCount = itemCount;
this.changedFields = changedFields.add("itemCount");
return this;
}
public Builder itemNeedReview(Long itemNeedReview) {
this.itemNeedReview = itemNeedReview;
this.changedFields = changedFields.add("itemNeedReview");
return this;
}
public Builder productItemCounts(List productItemCounts) {
this.productItemCounts = productItemCounts;
this.changedFields = changedFields.add("productItemCounts");
return this;
}
public Builder productItemCounts(KeyValuePair... productItemCounts) {
return productItemCounts(Arrays.asList(productItemCounts));
}
public Builder productItemCountsNextLink(String productItemCountsNextLink) {
this.productItemCountsNextLink = productItemCountsNextLink;
this.changedFields = changedFields.add("productItemCounts");
return this;
}
public Builder signedOffItemCount(Long signedOffItemCount) {
this.signedOffItemCount = signedOffItemCount;
this.changedFields = changedFields.add("signedOffItemCount");
return this;
}
public Builder totalItemSize(Long totalItemSize) {
this.totalItemSize = totalItemSize;
this.changedFields = changedFields.add("totalItemSize");
return this;
}
public SubjectRightsRequestDetail build() {
SubjectRightsRequestDetail _x = new SubjectRightsRequestDetail();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.subjectRightsRequestDetail";
_x.excludedItemCount = excludedItemCount;
_x.insightCounts = insightCounts;
_x.insightCountsNextLink = insightCountsNextLink;
_x.itemCount = itemCount;
_x.itemNeedReview = itemNeedReview;
_x.productItemCounts = productItemCounts;
_x.productItemCountsNextLink = productItemCountsNextLink;
_x.signedOffItemCount = signedOffItemCount;
_x.totalItemSize = totalItemSize;
return _x;
}
}
private SubjectRightsRequestDetail _copy() {
SubjectRightsRequestDetail _x = new SubjectRightsRequestDetail();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.excludedItemCount = excludedItemCount;
_x.insightCounts = insightCounts;
_x.itemCount = itemCount;
_x.itemNeedReview = itemNeedReview;
_x.productItemCounts = productItemCounts;
_x.signedOffItemCount = signedOffItemCount;
_x.totalItemSize = totalItemSize;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("SubjectRightsRequestDetail[");
b.append("excludedItemCount=");
b.append(this.excludedItemCount);
b.append(", ");
b.append("insightCounts=");
b.append(this.insightCounts);
b.append(", ");
b.append("itemCount=");
b.append(this.itemCount);
b.append(", ");
b.append("itemNeedReview=");
b.append(this.itemNeedReview);
b.append(", ");
b.append("productItemCounts=");
b.append(this.productItemCounts);
b.append(", ");
b.append("signedOffItemCount=");
b.append(this.signedOffItemCount);
b.append(", ");
b.append("totalItemSize=");
b.append(this.totalItemSize);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy