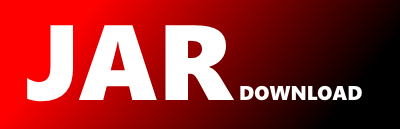
odata.msgraph.client.complex.SynchronizationTaskExecution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph Show documentation
Show all versions of odata-client-msgraph Show documentation
Java client for use with the Microsoft Graph v1.0 endpoint
package odata.msgraph.client.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Optional;
import odata.msgraph.client.enums.SynchronizationTaskExecutionResult;
@JsonPropertyOrder({
"@odata.type",
"activityIdentifier",
"countEntitled",
"countEntitledForProvisioning",
"countEscrowed",
"countEscrowedRaw",
"countExported",
"countExports",
"countImported",
"countImportedDeltas",
"countImportedReferenceDeltas",
"error",
"state",
"timeBegan",
"timeEnded"})
@JsonInclude(Include.NON_NULL)
public class SynchronizationTaskExecution implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("activityIdentifier")
protected String activityIdentifier;
@JsonProperty("countEntitled")
protected Long countEntitled;
@JsonProperty("countEntitledForProvisioning")
protected Long countEntitledForProvisioning;
@JsonProperty("countEscrowed")
protected Long countEscrowed;
@JsonProperty("countEscrowedRaw")
protected Long countEscrowedRaw;
@JsonProperty("countExported")
protected Long countExported;
@JsonProperty("countExports")
protected Long countExports;
@JsonProperty("countImported")
protected Long countImported;
@JsonProperty("countImportedDeltas")
protected Long countImportedDeltas;
@JsonProperty("countImportedReferenceDeltas")
protected Long countImportedReferenceDeltas;
@JsonProperty("error")
protected SynchronizationError error;
@JsonProperty("state")
protected SynchronizationTaskExecutionResult state;
@JsonProperty("timeBegan")
protected OffsetDateTime timeBegan;
@JsonProperty("timeEnded")
protected OffsetDateTime timeEnded;
protected SynchronizationTaskExecution() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.synchronizationTaskExecution";
}
@Property(name="activityIdentifier")
@JsonIgnore
public Optional getActivityIdentifier() {
return Optional.ofNullable(activityIdentifier);
}
public SynchronizationTaskExecution withActivityIdentifier(String activityIdentifier) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.activityIdentifier = activityIdentifier;
return _x;
}
@Property(name="countEntitled")
@JsonIgnore
public Optional getCountEntitled() {
return Optional.ofNullable(countEntitled);
}
public SynchronizationTaskExecution withCountEntitled(Long countEntitled) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.countEntitled = countEntitled;
return _x;
}
@Property(name="countEntitledForProvisioning")
@JsonIgnore
public Optional getCountEntitledForProvisioning() {
return Optional.ofNullable(countEntitledForProvisioning);
}
public SynchronizationTaskExecution withCountEntitledForProvisioning(Long countEntitledForProvisioning) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.countEntitledForProvisioning = countEntitledForProvisioning;
return _x;
}
@Property(name="countEscrowed")
@JsonIgnore
public Optional getCountEscrowed() {
return Optional.ofNullable(countEscrowed);
}
public SynchronizationTaskExecution withCountEscrowed(Long countEscrowed) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.countEscrowed = countEscrowed;
return _x;
}
@Property(name="countEscrowedRaw")
@JsonIgnore
public Optional getCountEscrowedRaw() {
return Optional.ofNullable(countEscrowedRaw);
}
public SynchronizationTaskExecution withCountEscrowedRaw(Long countEscrowedRaw) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.countEscrowedRaw = countEscrowedRaw;
return _x;
}
@Property(name="countExported")
@JsonIgnore
public Optional getCountExported() {
return Optional.ofNullable(countExported);
}
public SynchronizationTaskExecution withCountExported(Long countExported) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.countExported = countExported;
return _x;
}
@Property(name="countExports")
@JsonIgnore
public Optional getCountExports() {
return Optional.ofNullable(countExports);
}
public SynchronizationTaskExecution withCountExports(Long countExports) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.countExports = countExports;
return _x;
}
@Property(name="countImported")
@JsonIgnore
public Optional getCountImported() {
return Optional.ofNullable(countImported);
}
public SynchronizationTaskExecution withCountImported(Long countImported) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.countImported = countImported;
return _x;
}
@Property(name="countImportedDeltas")
@JsonIgnore
public Optional getCountImportedDeltas() {
return Optional.ofNullable(countImportedDeltas);
}
public SynchronizationTaskExecution withCountImportedDeltas(Long countImportedDeltas) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.countImportedDeltas = countImportedDeltas;
return _x;
}
@Property(name="countImportedReferenceDeltas")
@JsonIgnore
public Optional getCountImportedReferenceDeltas() {
return Optional.ofNullable(countImportedReferenceDeltas);
}
public SynchronizationTaskExecution withCountImportedReferenceDeltas(Long countImportedReferenceDeltas) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.countImportedReferenceDeltas = countImportedReferenceDeltas;
return _x;
}
@Property(name="error")
@JsonIgnore
public Optional getError() {
return Optional.ofNullable(error);
}
public SynchronizationTaskExecution withError(SynchronizationError error) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.error = error;
return _x;
}
@Property(name="state")
@JsonIgnore
public Optional getState() {
return Optional.ofNullable(state);
}
public SynchronizationTaskExecution withState(SynchronizationTaskExecutionResult state) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.state = state;
return _x;
}
@Property(name="timeBegan")
@JsonIgnore
public Optional getTimeBegan() {
return Optional.ofNullable(timeBegan);
}
public SynchronizationTaskExecution withTimeBegan(OffsetDateTime timeBegan) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.timeBegan = timeBegan;
return _x;
}
@Property(name="timeEnded")
@JsonIgnore
public Optional getTimeEnded() {
return Optional.ofNullable(timeEnded);
}
public SynchronizationTaskExecution withTimeEnded(OffsetDateTime timeEnded) {
SynchronizationTaskExecution _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.synchronizationTaskExecution");
_x.timeEnded = timeEnded;
return _x;
}
public SynchronizationTaskExecution withUnmappedField(String name, Object value) {
SynchronizationTaskExecution _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private String activityIdentifier;
private Long countEntitled;
private Long countEntitledForProvisioning;
private Long countEscrowed;
private Long countEscrowedRaw;
private Long countExported;
private Long countExports;
private Long countImported;
private Long countImportedDeltas;
private Long countImportedReferenceDeltas;
private SynchronizationError error;
private SynchronizationTaskExecutionResult state;
private OffsetDateTime timeBegan;
private OffsetDateTime timeEnded;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder activityIdentifier(String activityIdentifier) {
this.activityIdentifier = activityIdentifier;
this.changedFields = changedFields.add("activityIdentifier");
return this;
}
public Builder countEntitled(Long countEntitled) {
this.countEntitled = countEntitled;
this.changedFields = changedFields.add("countEntitled");
return this;
}
public Builder countEntitledForProvisioning(Long countEntitledForProvisioning) {
this.countEntitledForProvisioning = countEntitledForProvisioning;
this.changedFields = changedFields.add("countEntitledForProvisioning");
return this;
}
public Builder countEscrowed(Long countEscrowed) {
this.countEscrowed = countEscrowed;
this.changedFields = changedFields.add("countEscrowed");
return this;
}
public Builder countEscrowedRaw(Long countEscrowedRaw) {
this.countEscrowedRaw = countEscrowedRaw;
this.changedFields = changedFields.add("countEscrowedRaw");
return this;
}
public Builder countExported(Long countExported) {
this.countExported = countExported;
this.changedFields = changedFields.add("countExported");
return this;
}
public Builder countExports(Long countExports) {
this.countExports = countExports;
this.changedFields = changedFields.add("countExports");
return this;
}
public Builder countImported(Long countImported) {
this.countImported = countImported;
this.changedFields = changedFields.add("countImported");
return this;
}
public Builder countImportedDeltas(Long countImportedDeltas) {
this.countImportedDeltas = countImportedDeltas;
this.changedFields = changedFields.add("countImportedDeltas");
return this;
}
public Builder countImportedReferenceDeltas(Long countImportedReferenceDeltas) {
this.countImportedReferenceDeltas = countImportedReferenceDeltas;
this.changedFields = changedFields.add("countImportedReferenceDeltas");
return this;
}
public Builder error(SynchronizationError error) {
this.error = error;
this.changedFields = changedFields.add("error");
return this;
}
public Builder state(SynchronizationTaskExecutionResult state) {
this.state = state;
this.changedFields = changedFields.add("state");
return this;
}
public Builder timeBegan(OffsetDateTime timeBegan) {
this.timeBegan = timeBegan;
this.changedFields = changedFields.add("timeBegan");
return this;
}
public Builder timeEnded(OffsetDateTime timeEnded) {
this.timeEnded = timeEnded;
this.changedFields = changedFields.add("timeEnded");
return this;
}
public SynchronizationTaskExecution build() {
SynchronizationTaskExecution _x = new SynchronizationTaskExecution();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.synchronizationTaskExecution";
_x.activityIdentifier = activityIdentifier;
_x.countEntitled = countEntitled;
_x.countEntitledForProvisioning = countEntitledForProvisioning;
_x.countEscrowed = countEscrowed;
_x.countEscrowedRaw = countEscrowedRaw;
_x.countExported = countExported;
_x.countExports = countExports;
_x.countImported = countImported;
_x.countImportedDeltas = countImportedDeltas;
_x.countImportedReferenceDeltas = countImportedReferenceDeltas;
_x.error = error;
_x.state = state;
_x.timeBegan = timeBegan;
_x.timeEnded = timeEnded;
return _x;
}
}
private SynchronizationTaskExecution _copy() {
SynchronizationTaskExecution _x = new SynchronizationTaskExecution();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.activityIdentifier = activityIdentifier;
_x.countEntitled = countEntitled;
_x.countEntitledForProvisioning = countEntitledForProvisioning;
_x.countEscrowed = countEscrowed;
_x.countEscrowedRaw = countEscrowedRaw;
_x.countExported = countExported;
_x.countExports = countExports;
_x.countImported = countImported;
_x.countImportedDeltas = countImportedDeltas;
_x.countImportedReferenceDeltas = countImportedReferenceDeltas;
_x.error = error;
_x.state = state;
_x.timeBegan = timeBegan;
_x.timeEnded = timeEnded;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("SynchronizationTaskExecution[");
b.append("activityIdentifier=");
b.append(this.activityIdentifier);
b.append(", ");
b.append("countEntitled=");
b.append(this.countEntitled);
b.append(", ");
b.append("countEntitledForProvisioning=");
b.append(this.countEntitledForProvisioning);
b.append(", ");
b.append("countEscrowed=");
b.append(this.countEscrowed);
b.append(", ");
b.append("countEscrowedRaw=");
b.append(this.countEscrowedRaw);
b.append(", ");
b.append("countExported=");
b.append(this.countExported);
b.append(", ");
b.append("countExports=");
b.append(this.countExports);
b.append(", ");
b.append("countImported=");
b.append(this.countImported);
b.append(", ");
b.append("countImportedDeltas=");
b.append(this.countImportedDeltas);
b.append(", ");
b.append("countImportedReferenceDeltas=");
b.append(this.countImportedReferenceDeltas);
b.append(", ");
b.append("error=");
b.append(this.error);
b.append(", ");
b.append("state=");
b.append(this.state);
b.append(", ");
b.append("timeBegan=");
b.append(this.timeBegan);
b.append(", ");
b.append("timeEnded=");
b.append(this.timeEnded);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy