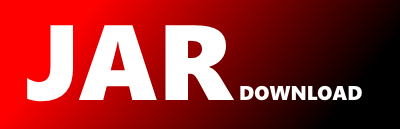
odata.msgraph.client.complex.UserExperienceAnalyticsAutopilotDevicesSummary Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
/**
* “The user experience analytics summary of Devices not windows autopilot ready.”
*/@JsonPropertyOrder({
"@odata.type",
"devicesNotAutopilotRegistered",
"devicesWithoutAutopilotProfileAssigned",
"totalWindows10DevicesWithoutTenantAttached"})
@JsonInclude(Include.NON_NULL)
public class UserExperienceAnalyticsAutopilotDevicesSummary implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("devicesNotAutopilotRegistered")
protected Integer devicesNotAutopilotRegistered;
@JsonProperty("devicesWithoutAutopilotProfileAssigned")
protected Integer devicesWithoutAutopilotProfileAssigned;
@JsonProperty("totalWindows10DevicesWithoutTenantAttached")
protected Integer totalWindows10DevicesWithoutTenantAttached;
protected UserExperienceAnalyticsAutopilotDevicesSummary() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.userExperienceAnalyticsAutopilotDevicesSummary";
}
/**
* “The count of intune devices that are not autopilot registerd. Read-only.”
*
* @return property devicesNotAutopilotRegistered
*/
@Property(name="devicesNotAutopilotRegistered")
@JsonIgnore
public Optional getDevicesNotAutopilotRegistered() {
return Optional.ofNullable(devicesNotAutopilotRegistered);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* devicesNotAutopilotRegistered} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The count of intune devices that are not autopilot registerd. Read-only.”
*
* @param devicesNotAutopilotRegistered
* new value of {@code devicesNotAutopilotRegistered} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code devicesNotAutopilotRegistered} field changed
*/
public UserExperienceAnalyticsAutopilotDevicesSummary withDevicesNotAutopilotRegistered(Integer devicesNotAutopilotRegistered) {
UserExperienceAnalyticsAutopilotDevicesSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsAutopilotDevicesSummary");
_x.devicesNotAutopilotRegistered = devicesNotAutopilotRegistered;
return _x;
}
/**
* “The count of intune devices not autopilot profile assigned. Read-only.”
*
* @return property devicesWithoutAutopilotProfileAssigned
*/
@Property(name="devicesWithoutAutopilotProfileAssigned")
@JsonIgnore
public Optional getDevicesWithoutAutopilotProfileAssigned() {
return Optional.ofNullable(devicesWithoutAutopilotProfileAssigned);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* devicesWithoutAutopilotProfileAssigned} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “The count of intune devices not autopilot profile assigned. Read-only.”
*
* @param devicesWithoutAutopilotProfileAssigned
* new value of {@code devicesWithoutAutopilotProfileAssigned} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code devicesWithoutAutopilotProfileAssigned} field changed
*/
public UserExperienceAnalyticsAutopilotDevicesSummary withDevicesWithoutAutopilotProfileAssigned(Integer devicesWithoutAutopilotProfileAssigned) {
UserExperienceAnalyticsAutopilotDevicesSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsAutopilotDevicesSummary");
_x.devicesWithoutAutopilotProfileAssigned = devicesWithoutAutopilotProfileAssigned;
return _x;
}
/**
* “The count of windows 10 devices that are Intune and co-managed. Read-only.”
*
* @return property totalWindows10DevicesWithoutTenantAttached
*/
@Property(name="totalWindows10DevicesWithoutTenantAttached")
@JsonIgnore
public Optional getTotalWindows10DevicesWithoutTenantAttached() {
return Optional.ofNullable(totalWindows10DevicesWithoutTenantAttached);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* totalWindows10DevicesWithoutTenantAttached} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “The count of windows 10 devices that are Intune and co-managed. Read-only.”
*
* @param totalWindows10DevicesWithoutTenantAttached
* new value of {@code totalWindows10DevicesWithoutTenantAttached} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code totalWindows10DevicesWithoutTenantAttached} field changed
*/
public UserExperienceAnalyticsAutopilotDevicesSummary withTotalWindows10DevicesWithoutTenantAttached(Integer totalWindows10DevicesWithoutTenantAttached) {
UserExperienceAnalyticsAutopilotDevicesSummary _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.userExperienceAnalyticsAutopilotDevicesSummary");
_x.totalWindows10DevicesWithoutTenantAttached = totalWindows10DevicesWithoutTenantAttached;
return _x;
}
public UserExperienceAnalyticsAutopilotDevicesSummary withUnmappedField(String name, Object value) {
UserExperienceAnalyticsAutopilotDevicesSummary _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private Integer devicesNotAutopilotRegistered;
private Integer devicesWithoutAutopilotProfileAssigned;
private Integer totalWindows10DevicesWithoutTenantAttached;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
/**
* “The count of intune devices that are not autopilot registerd. Read-only.”
*
* @param devicesNotAutopilotRegistered
* value of {@code devicesNotAutopilotRegistered} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder devicesNotAutopilotRegistered(Integer devicesNotAutopilotRegistered) {
this.devicesNotAutopilotRegistered = devicesNotAutopilotRegistered;
this.changedFields = changedFields.add("devicesNotAutopilotRegistered");
return this;
}
/**
* “The count of intune devices not autopilot profile assigned. Read-only.”
*
* @param devicesWithoutAutopilotProfileAssigned
* value of {@code devicesWithoutAutopilotProfileAssigned} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder devicesWithoutAutopilotProfileAssigned(Integer devicesWithoutAutopilotProfileAssigned) {
this.devicesWithoutAutopilotProfileAssigned = devicesWithoutAutopilotProfileAssigned;
this.changedFields = changedFields.add("devicesWithoutAutopilotProfileAssigned");
return this;
}
/**
* “The count of windows 10 devices that are Intune and co-managed. Read-only.”
*
* @param totalWindows10DevicesWithoutTenantAttached
* value of {@code totalWindows10DevicesWithoutTenantAttached} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder totalWindows10DevicesWithoutTenantAttached(Integer totalWindows10DevicesWithoutTenantAttached) {
this.totalWindows10DevicesWithoutTenantAttached = totalWindows10DevicesWithoutTenantAttached;
this.changedFields = changedFields.add("totalWindows10DevicesWithoutTenantAttached");
return this;
}
public UserExperienceAnalyticsAutopilotDevicesSummary build() {
UserExperienceAnalyticsAutopilotDevicesSummary _x = new UserExperienceAnalyticsAutopilotDevicesSummary();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.userExperienceAnalyticsAutopilotDevicesSummary";
_x.devicesNotAutopilotRegistered = devicesNotAutopilotRegistered;
_x.devicesWithoutAutopilotProfileAssigned = devicesWithoutAutopilotProfileAssigned;
_x.totalWindows10DevicesWithoutTenantAttached = totalWindows10DevicesWithoutTenantAttached;
return _x;
}
}
private UserExperienceAnalyticsAutopilotDevicesSummary _copy() {
UserExperienceAnalyticsAutopilotDevicesSummary _x = new UserExperienceAnalyticsAutopilotDevicesSummary();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.devicesNotAutopilotRegistered = devicesNotAutopilotRegistered;
_x.devicesWithoutAutopilotProfileAssigned = devicesWithoutAutopilotProfileAssigned;
_x.totalWindows10DevicesWithoutTenantAttached = totalWindows10DevicesWithoutTenantAttached;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("UserExperienceAnalyticsAutopilotDevicesSummary[");
b.append("devicesNotAutopilotRegistered=");
b.append(this.devicesNotAutopilotRegistered);
b.append(", ");
b.append("devicesWithoutAutopilotProfileAssigned=");
b.append(this.devicesWithoutAutopilotProfileAssigned);
b.append(", ");
b.append("totalWindows10DevicesWithoutTenantAttached=");
b.append(this.totalWindows10DevicesWithoutTenantAttached);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}