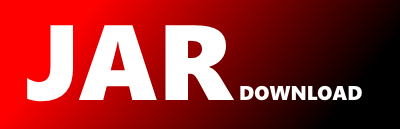
odata.msgraph.client.complex.WindowsFirewallNetworkProfile Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.complex;
import com.fasterxml.jackson.annotation.JacksonInject;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ContextPath;
import com.github.davidmoten.odata.client.ODataType;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
import odata.msgraph.client.enums.StateManagementSetting;
/**
* “Windows Firewall Profile Policies.”
*/@JsonPropertyOrder({
"@odata.type",
"authorizedApplicationRulesFromGroupPolicyMerged",
"connectionSecurityRulesFromGroupPolicyMerged",
"firewallEnabled",
"globalPortRulesFromGroupPolicyMerged",
"inboundConnectionsBlocked",
"inboundNotificationsBlocked",
"incomingTrafficBlocked",
"outboundConnectionsBlocked",
"policyRulesFromGroupPolicyMerged",
"securedPacketExemptionAllowed",
"stealthModeBlocked",
"unicastResponsesToMulticastBroadcastsBlocked"})
@JsonInclude(Include.NON_NULL)
public class WindowsFirewallNetworkProfile implements ODataType {
@JacksonInject
@JsonIgnore
protected ContextPath contextPath;
@JacksonInject
@JsonIgnore
protected UnmappedFieldsImpl unmappedFields;
@JsonProperty("@odata.type")
protected String odataType;
@JsonProperty("authorizedApplicationRulesFromGroupPolicyMerged")
protected Boolean authorizedApplicationRulesFromGroupPolicyMerged;
@JsonProperty("connectionSecurityRulesFromGroupPolicyMerged")
protected Boolean connectionSecurityRulesFromGroupPolicyMerged;
@JsonProperty("firewallEnabled")
protected StateManagementSetting firewallEnabled;
@JsonProperty("globalPortRulesFromGroupPolicyMerged")
protected Boolean globalPortRulesFromGroupPolicyMerged;
@JsonProperty("inboundConnectionsBlocked")
protected Boolean inboundConnectionsBlocked;
@JsonProperty("inboundNotificationsBlocked")
protected Boolean inboundNotificationsBlocked;
@JsonProperty("incomingTrafficBlocked")
protected Boolean incomingTrafficBlocked;
@JsonProperty("outboundConnectionsBlocked")
protected Boolean outboundConnectionsBlocked;
@JsonProperty("policyRulesFromGroupPolicyMerged")
protected Boolean policyRulesFromGroupPolicyMerged;
@JsonProperty("securedPacketExemptionAllowed")
protected Boolean securedPacketExemptionAllowed;
@JsonProperty("stealthModeBlocked")
protected Boolean stealthModeBlocked;
@JsonProperty("unicastResponsesToMulticastBroadcastsBlocked")
protected Boolean unicastResponsesToMulticastBroadcastsBlocked;
protected WindowsFirewallNetworkProfile() {
}
@Override
public String odataTypeName() {
return "microsoft.graph.windowsFirewallNetworkProfile";
}
/**
* “Configures the firewall to merge authorized application rules from group policy
* with those from local store instead of ignoring the local store rules. When
* AuthorizedApplicationRulesFromGroupPolicyNotMerged and
* AuthorizedApplicationRulesFromGroupPolicyMerged are both true,
* AuthorizedApplicationRulesFromGroupPolicyMerged takes priority.”
*
* @return property authorizedApplicationRulesFromGroupPolicyMerged
*/
@Property(name="authorizedApplicationRulesFromGroupPolicyMerged")
@JsonIgnore
public Optional getAuthorizedApplicationRulesFromGroupPolicyMerged() {
return Optional.ofNullable(authorizedApplicationRulesFromGroupPolicyMerged);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* authorizedApplicationRulesFromGroupPolicyMerged} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “Configures the firewall to merge authorized application rules from group policy
* with those from local store instead of ignoring the local store rules. When
* AuthorizedApplicationRulesFromGroupPolicyNotMerged and
* AuthorizedApplicationRulesFromGroupPolicyMerged are both true,
* AuthorizedApplicationRulesFromGroupPolicyMerged takes priority.”
*
* @param authorizedApplicationRulesFromGroupPolicyMerged
* new value of {@code authorizedApplicationRulesFromGroupPolicyMerged} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code authorizedApplicationRulesFromGroupPolicyMerged} field changed
*/
public WindowsFirewallNetworkProfile withAuthorizedApplicationRulesFromGroupPolicyMerged(Boolean authorizedApplicationRulesFromGroupPolicyMerged) {
WindowsFirewallNetworkProfile _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsFirewallNetworkProfile");
_x.authorizedApplicationRulesFromGroupPolicyMerged = authorizedApplicationRulesFromGroupPolicyMerged;
return _x;
}
/**
* “Configures the firewall to merge connection security rules from group policy
* with those from local store instead of ignoring the local store rules. When
* ConnectionSecurityRulesFromGroupPolicyNotMerged and
* ConnectionSecurityRulesFromGroupPolicyMerged are both true,
* ConnectionSecurityRulesFromGroupPolicyMerged takes priority.”
*
* @return property connectionSecurityRulesFromGroupPolicyMerged
*/
@Property(name="connectionSecurityRulesFromGroupPolicyMerged")
@JsonIgnore
public Optional getConnectionSecurityRulesFromGroupPolicyMerged() {
return Optional.ofNullable(connectionSecurityRulesFromGroupPolicyMerged);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* connectionSecurityRulesFromGroupPolicyMerged} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Configures the firewall to merge connection security rules from group policy
* with those from local store instead of ignoring the local store rules. When
* ConnectionSecurityRulesFromGroupPolicyNotMerged and
* ConnectionSecurityRulesFromGroupPolicyMerged are both true,
* ConnectionSecurityRulesFromGroupPolicyMerged takes priority.”
*
* @param connectionSecurityRulesFromGroupPolicyMerged
* new value of {@code connectionSecurityRulesFromGroupPolicyMerged} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code connectionSecurityRulesFromGroupPolicyMerged} field changed
*/
public WindowsFirewallNetworkProfile withConnectionSecurityRulesFromGroupPolicyMerged(Boolean connectionSecurityRulesFromGroupPolicyMerged) {
WindowsFirewallNetworkProfile _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsFirewallNetworkProfile");
_x.connectionSecurityRulesFromGroupPolicyMerged = connectionSecurityRulesFromGroupPolicyMerged;
return _x;
}
/**
* “Configures the host device to allow or block the firewall and advanced security
* enforcement for the network profile.”
*
* @return property firewallEnabled
*/
@Property(name="firewallEnabled")
@JsonIgnore
public Optional getFirewallEnabled() {
return Optional.ofNullable(firewallEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code firewallEnabled}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Configures the host device to allow or block the firewall and advanced security
* enforcement for the network profile.”
*
* @param firewallEnabled
* new value of {@code firewallEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code firewallEnabled} field changed
*/
public WindowsFirewallNetworkProfile withFirewallEnabled(StateManagementSetting firewallEnabled) {
WindowsFirewallNetworkProfile _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsFirewallNetworkProfile");
_x.firewallEnabled = firewallEnabled;
return _x;
}
/**
* “Configures the firewall to merge global port rules from group policy with those
* from local store instead of ignoring the local store rules. When
* GlobalPortRulesFromGroupPolicyNotMerged and GlobalPortRulesFromGroupPolicyMerged
* are both true, GlobalPortRulesFromGroupPolicyMerged takes priority.”
*
* @return property globalPortRulesFromGroupPolicyMerged
*/
@Property(name="globalPortRulesFromGroupPolicyMerged")
@JsonIgnore
public Optional getGlobalPortRulesFromGroupPolicyMerged() {
return Optional.ofNullable(globalPortRulesFromGroupPolicyMerged);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* globalPortRulesFromGroupPolicyMerged} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Configures the firewall to merge global port rules from group policy with those
* from local store instead of ignoring the local store rules. When
* GlobalPortRulesFromGroupPolicyNotMerged and GlobalPortRulesFromGroupPolicyMerged
* are both true, GlobalPortRulesFromGroupPolicyMerged takes priority.”
*
* @param globalPortRulesFromGroupPolicyMerged
* new value of {@code globalPortRulesFromGroupPolicyMerged} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code globalPortRulesFromGroupPolicyMerged} field changed
*/
public WindowsFirewallNetworkProfile withGlobalPortRulesFromGroupPolicyMerged(Boolean globalPortRulesFromGroupPolicyMerged) {
WindowsFirewallNetworkProfile _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsFirewallNetworkProfile");
_x.globalPortRulesFromGroupPolicyMerged = globalPortRulesFromGroupPolicyMerged;
return _x;
}
/**
* “Configures the firewall to block all incoming connections by default. When
* InboundConnectionsRequired and InboundConnectionsBlocked are both true,
* InboundConnectionsBlocked takes priority.”
*
* @return property inboundConnectionsBlocked
*/
@Property(name="inboundConnectionsBlocked")
@JsonIgnore
public Optional getInboundConnectionsBlocked() {
return Optional.ofNullable(inboundConnectionsBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* inboundConnectionsBlocked} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Configures the firewall to block all incoming connections by default. When
* InboundConnectionsRequired and InboundConnectionsBlocked are both true,
* InboundConnectionsBlocked takes priority.”
*
* @param inboundConnectionsBlocked
* new value of {@code inboundConnectionsBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code inboundConnectionsBlocked} field changed
*/
public WindowsFirewallNetworkProfile withInboundConnectionsBlocked(Boolean inboundConnectionsBlocked) {
WindowsFirewallNetworkProfile _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsFirewallNetworkProfile");
_x.inboundConnectionsBlocked = inboundConnectionsBlocked;
return _x;
}
/**
* “Prevents the firewall from displaying notifications when an application is
* blocked from listening on a port. When InboundNotificationsRequired and
* InboundNotificationsBlocked are both true, InboundNotificationsBlocked takes
* priority.”
*
* @return property inboundNotificationsBlocked
*/
@Property(name="inboundNotificationsBlocked")
@JsonIgnore
public Optional getInboundNotificationsBlocked() {
return Optional.ofNullable(inboundNotificationsBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* inboundNotificationsBlocked} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Prevents the firewall from displaying notifications when an application is
* blocked from listening on a port. When InboundNotificationsRequired and
* InboundNotificationsBlocked are both true, InboundNotificationsBlocked takes
* priority.”
*
* @param inboundNotificationsBlocked
* new value of {@code inboundNotificationsBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code inboundNotificationsBlocked} field changed
*/
public WindowsFirewallNetworkProfile withInboundNotificationsBlocked(Boolean inboundNotificationsBlocked) {
WindowsFirewallNetworkProfile _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsFirewallNetworkProfile");
_x.inboundNotificationsBlocked = inboundNotificationsBlocked;
return _x;
}
/**
* “Configures the firewall to block all incoming traffic regardless of other policy
* settings. When IncomingTrafficRequired and IncomingTrafficBlocked are both true,
* IncomingTrafficBlocked takes priority.”
*
* @return property incomingTrafficBlocked
*/
@Property(name="incomingTrafficBlocked")
@JsonIgnore
public Optional getIncomingTrafficBlocked() {
return Optional.ofNullable(incomingTrafficBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* incomingTrafficBlocked} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Configures the firewall to block all incoming traffic regardless of other policy
* settings. When IncomingTrafficRequired and IncomingTrafficBlocked are both true,
* IncomingTrafficBlocked takes priority.”
*
* @param incomingTrafficBlocked
* new value of {@code incomingTrafficBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code incomingTrafficBlocked} field changed
*/
public WindowsFirewallNetworkProfile withIncomingTrafficBlocked(Boolean incomingTrafficBlocked) {
WindowsFirewallNetworkProfile _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsFirewallNetworkProfile");
_x.incomingTrafficBlocked = incomingTrafficBlocked;
return _x;
}
/**
* “Configures the firewall to block all outgoing connections by default. When
* OutboundConnectionsRequired and OutboundConnectionsBlocked are both true,
* OutboundConnectionsBlocked takes priority. This setting will get applied to
* Windows releases version 1809 and above.”
*
* @return property outboundConnectionsBlocked
*/
@Property(name="outboundConnectionsBlocked")
@JsonIgnore
public Optional getOutboundConnectionsBlocked() {
return Optional.ofNullable(outboundConnectionsBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* outboundConnectionsBlocked} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Configures the firewall to block all outgoing connections by default. When
* OutboundConnectionsRequired and OutboundConnectionsBlocked are both true,
* OutboundConnectionsBlocked takes priority. This setting will get applied to
* Windows releases version 1809 and above.”
*
* @param outboundConnectionsBlocked
* new value of {@code outboundConnectionsBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code outboundConnectionsBlocked} field changed
*/
public WindowsFirewallNetworkProfile withOutboundConnectionsBlocked(Boolean outboundConnectionsBlocked) {
WindowsFirewallNetworkProfile _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsFirewallNetworkProfile");
_x.outboundConnectionsBlocked = outboundConnectionsBlocked;
return _x;
}
/**
* “Configures the firewall to merge Firewall Rule policies from group policy with
* those from local store instead of ignoring the local store rules. When
* PolicyRulesFromGroupPolicyNotMerged and PolicyRulesFromGroupPolicyMerged are
* both true, PolicyRulesFromGroupPolicyMerged takes priority.”
*
* @return property policyRulesFromGroupPolicyMerged
*/
@Property(name="policyRulesFromGroupPolicyMerged")
@JsonIgnore
public Optional getPolicyRulesFromGroupPolicyMerged() {
return Optional.ofNullable(policyRulesFromGroupPolicyMerged);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* policyRulesFromGroupPolicyMerged} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Configures the firewall to merge Firewall Rule policies from group policy with
* those from local store instead of ignoring the local store rules. When
* PolicyRulesFromGroupPolicyNotMerged and PolicyRulesFromGroupPolicyMerged are
* both true, PolicyRulesFromGroupPolicyMerged takes priority.”
*
* @param policyRulesFromGroupPolicyMerged
* new value of {@code policyRulesFromGroupPolicyMerged} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code policyRulesFromGroupPolicyMerged} field changed
*/
public WindowsFirewallNetworkProfile withPolicyRulesFromGroupPolicyMerged(Boolean policyRulesFromGroupPolicyMerged) {
WindowsFirewallNetworkProfile _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsFirewallNetworkProfile");
_x.policyRulesFromGroupPolicyMerged = policyRulesFromGroupPolicyMerged;
return _x;
}
/**
* “Configures the firewall to allow the host computer to respond to unsolicited
* network traffic of that traffic is secured by IPSec even when stealthModeBlocked
* is set to true. When SecuredPacketExemptionBlocked and
* SecuredPacketExemptionAllowed are both true, SecuredPacketExemptionAllowed takes
* priority.”
*
* @return property securedPacketExemptionAllowed
*/
@Property(name="securedPacketExemptionAllowed")
@JsonIgnore
public Optional getSecuredPacketExemptionAllowed() {
return Optional.ofNullable(securedPacketExemptionAllowed);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* securedPacketExemptionAllowed} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Configures the firewall to allow the host computer to respond to unsolicited
* network traffic of that traffic is secured by IPSec even when stealthModeBlocked
* is set to true. When SecuredPacketExemptionBlocked and
* SecuredPacketExemptionAllowed are both true, SecuredPacketExemptionAllowed takes
* priority.”
*
* @param securedPacketExemptionAllowed
* new value of {@code securedPacketExemptionAllowed} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code securedPacketExemptionAllowed} field changed
*/
public WindowsFirewallNetworkProfile withSecuredPacketExemptionAllowed(Boolean securedPacketExemptionAllowed) {
WindowsFirewallNetworkProfile _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsFirewallNetworkProfile");
_x.securedPacketExemptionAllowed = securedPacketExemptionAllowed;
return _x;
}
/**
* “Prevent the server from operating in stealth mode. When StealthModeRequired and
* StealthModeBlocked are both true, StealthModeBlocked takes priority.”
*
* @return property stealthModeBlocked
*/
@Property(name="stealthModeBlocked")
@JsonIgnore
public Optional getStealthModeBlocked() {
return Optional.ofNullable(stealthModeBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* stealthModeBlocked} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Prevent the server from operating in stealth mode. When StealthModeRequired and
* StealthModeBlocked are both true, StealthModeBlocked takes priority.”
*
* @param stealthModeBlocked
* new value of {@code stealthModeBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code stealthModeBlocked} field changed
*/
public WindowsFirewallNetworkProfile withStealthModeBlocked(Boolean stealthModeBlocked) {
WindowsFirewallNetworkProfile _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsFirewallNetworkProfile");
_x.stealthModeBlocked = stealthModeBlocked;
return _x;
}
/**
* “Configures the firewall to block unicast responses to multicast broadcast
* traffic. When UnicastResponsesToMulticastBroadcastsRequired and
* UnicastResponsesToMulticastBroadcastsBlocked are both true,
* UnicastResponsesToMulticastBroadcastsBlocked takes priority.”
*
* @return property unicastResponsesToMulticastBroadcastsBlocked
*/
@Property(name="unicastResponsesToMulticastBroadcastsBlocked")
@JsonIgnore
public Optional getUnicastResponsesToMulticastBroadcastsBlocked() {
return Optional.ofNullable(unicastResponsesToMulticastBroadcastsBlocked);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* unicastResponsesToMulticastBroadcastsBlocked} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Configures the firewall to block unicast responses to multicast broadcast
* traffic. When UnicastResponsesToMulticastBroadcastsRequired and
* UnicastResponsesToMulticastBroadcastsBlocked are both true,
* UnicastResponsesToMulticastBroadcastsBlocked takes priority.”
*
* @param unicastResponsesToMulticastBroadcastsBlocked
* new value of {@code unicastResponsesToMulticastBroadcastsBlocked} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code unicastResponsesToMulticastBroadcastsBlocked} field changed
*/
public WindowsFirewallNetworkProfile withUnicastResponsesToMulticastBroadcastsBlocked(Boolean unicastResponsesToMulticastBroadcastsBlocked) {
WindowsFirewallNetworkProfile _x = _copy();
_x.odataType = Util.nvl(odataType, "microsoft.graph.windowsFirewallNetworkProfile");
_x.unicastResponsesToMulticastBroadcastsBlocked = unicastResponsesToMulticastBroadcastsBlocked;
return _x;
}
public WindowsFirewallNetworkProfile withUnmappedField(String name, Object value) {
WindowsFirewallNetworkProfile _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
@Override
public void postInject(boolean addKeysToContextPath) {
// do nothing;
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builder() {
return new Builder();
}
public static final class Builder {
private Boolean authorizedApplicationRulesFromGroupPolicyMerged;
private Boolean connectionSecurityRulesFromGroupPolicyMerged;
private StateManagementSetting firewallEnabled;
private Boolean globalPortRulesFromGroupPolicyMerged;
private Boolean inboundConnectionsBlocked;
private Boolean inboundNotificationsBlocked;
private Boolean incomingTrafficBlocked;
private Boolean outboundConnectionsBlocked;
private Boolean policyRulesFromGroupPolicyMerged;
private Boolean securedPacketExemptionAllowed;
private Boolean stealthModeBlocked;
private Boolean unicastResponsesToMulticastBroadcastsBlocked;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
/**
* “Configures the firewall to merge authorized application rules from group policy
* with those from local store instead of ignoring the local store rules. When
* AuthorizedApplicationRulesFromGroupPolicyNotMerged and
* AuthorizedApplicationRulesFromGroupPolicyMerged are both true,
* AuthorizedApplicationRulesFromGroupPolicyMerged takes priority.”
*
* @param authorizedApplicationRulesFromGroupPolicyMerged
* value of {@code authorizedApplicationRulesFromGroupPolicyMerged} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder authorizedApplicationRulesFromGroupPolicyMerged(Boolean authorizedApplicationRulesFromGroupPolicyMerged) {
this.authorizedApplicationRulesFromGroupPolicyMerged = authorizedApplicationRulesFromGroupPolicyMerged;
this.changedFields = changedFields.add("authorizedApplicationRulesFromGroupPolicyMerged");
return this;
}
/**
* “Configures the firewall to merge connection security rules from group policy
* with those from local store instead of ignoring the local store rules. When
* ConnectionSecurityRulesFromGroupPolicyNotMerged and
* ConnectionSecurityRulesFromGroupPolicyMerged are both true,
* ConnectionSecurityRulesFromGroupPolicyMerged takes priority.”
*
* @param connectionSecurityRulesFromGroupPolicyMerged
* value of {@code connectionSecurityRulesFromGroupPolicyMerged} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder connectionSecurityRulesFromGroupPolicyMerged(Boolean connectionSecurityRulesFromGroupPolicyMerged) {
this.connectionSecurityRulesFromGroupPolicyMerged = connectionSecurityRulesFromGroupPolicyMerged;
this.changedFields = changedFields.add("connectionSecurityRulesFromGroupPolicyMerged");
return this;
}
/**
* “Configures the host device to allow or block the firewall and advanced security
* enforcement for the network profile.”
*
* @param firewallEnabled
* value of {@code firewallEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder firewallEnabled(StateManagementSetting firewallEnabled) {
this.firewallEnabled = firewallEnabled;
this.changedFields = changedFields.add("firewallEnabled");
return this;
}
/**
* “Configures the firewall to merge global port rules from group policy with those
* from local store instead of ignoring the local store rules. When
* GlobalPortRulesFromGroupPolicyNotMerged and GlobalPortRulesFromGroupPolicyMerged
* are both true, GlobalPortRulesFromGroupPolicyMerged takes priority.”
*
* @param globalPortRulesFromGroupPolicyMerged
* value of {@code globalPortRulesFromGroupPolicyMerged} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder globalPortRulesFromGroupPolicyMerged(Boolean globalPortRulesFromGroupPolicyMerged) {
this.globalPortRulesFromGroupPolicyMerged = globalPortRulesFromGroupPolicyMerged;
this.changedFields = changedFields.add("globalPortRulesFromGroupPolicyMerged");
return this;
}
/**
* “Configures the firewall to block all incoming connections by default. When
* InboundConnectionsRequired and InboundConnectionsBlocked are both true,
* InboundConnectionsBlocked takes priority.”
*
* @param inboundConnectionsBlocked
* value of {@code inboundConnectionsBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder inboundConnectionsBlocked(Boolean inboundConnectionsBlocked) {
this.inboundConnectionsBlocked = inboundConnectionsBlocked;
this.changedFields = changedFields.add("inboundConnectionsBlocked");
return this;
}
/**
* “Prevents the firewall from displaying notifications when an application is
* blocked from listening on a port. When InboundNotificationsRequired and
* InboundNotificationsBlocked are both true, InboundNotificationsBlocked takes
* priority.”
*
* @param inboundNotificationsBlocked
* value of {@code inboundNotificationsBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder inboundNotificationsBlocked(Boolean inboundNotificationsBlocked) {
this.inboundNotificationsBlocked = inboundNotificationsBlocked;
this.changedFields = changedFields.add("inboundNotificationsBlocked");
return this;
}
/**
* “Configures the firewall to block all incoming traffic regardless of other policy
* settings. When IncomingTrafficRequired and IncomingTrafficBlocked are both true,
* IncomingTrafficBlocked takes priority.”
*
* @param incomingTrafficBlocked
* value of {@code incomingTrafficBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder incomingTrafficBlocked(Boolean incomingTrafficBlocked) {
this.incomingTrafficBlocked = incomingTrafficBlocked;
this.changedFields = changedFields.add("incomingTrafficBlocked");
return this;
}
/**
* “Configures the firewall to block all outgoing connections by default. When
* OutboundConnectionsRequired and OutboundConnectionsBlocked are both true,
* OutboundConnectionsBlocked takes priority. This setting will get applied to
* Windows releases version 1809 and above.”
*
* @param outboundConnectionsBlocked
* value of {@code outboundConnectionsBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder outboundConnectionsBlocked(Boolean outboundConnectionsBlocked) {
this.outboundConnectionsBlocked = outboundConnectionsBlocked;
this.changedFields = changedFields.add("outboundConnectionsBlocked");
return this;
}
/**
* “Configures the firewall to merge Firewall Rule policies from group policy with
* those from local store instead of ignoring the local store rules. When
* PolicyRulesFromGroupPolicyNotMerged and PolicyRulesFromGroupPolicyMerged are
* both true, PolicyRulesFromGroupPolicyMerged takes priority.”
*
* @param policyRulesFromGroupPolicyMerged
* value of {@code policyRulesFromGroupPolicyMerged} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder policyRulesFromGroupPolicyMerged(Boolean policyRulesFromGroupPolicyMerged) {
this.policyRulesFromGroupPolicyMerged = policyRulesFromGroupPolicyMerged;
this.changedFields = changedFields.add("policyRulesFromGroupPolicyMerged");
return this;
}
/**
* “Configures the firewall to allow the host computer to respond to unsolicited
* network traffic of that traffic is secured by IPSec even when stealthModeBlocked
* is set to true. When SecuredPacketExemptionBlocked and
* SecuredPacketExemptionAllowed are both true, SecuredPacketExemptionAllowed takes
* priority.”
*
* @param securedPacketExemptionAllowed
* value of {@code securedPacketExemptionAllowed} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder securedPacketExemptionAllowed(Boolean securedPacketExemptionAllowed) {
this.securedPacketExemptionAllowed = securedPacketExemptionAllowed;
this.changedFields = changedFields.add("securedPacketExemptionAllowed");
return this;
}
/**
* “Prevent the server from operating in stealth mode. When StealthModeRequired and
* StealthModeBlocked are both true, StealthModeBlocked takes priority.”
*
* @param stealthModeBlocked
* value of {@code stealthModeBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder stealthModeBlocked(Boolean stealthModeBlocked) {
this.stealthModeBlocked = stealthModeBlocked;
this.changedFields = changedFields.add("stealthModeBlocked");
return this;
}
/**
* “Configures the firewall to block unicast responses to multicast broadcast
* traffic. When UnicastResponsesToMulticastBroadcastsRequired and
* UnicastResponsesToMulticastBroadcastsBlocked are both true,
* UnicastResponsesToMulticastBroadcastsBlocked takes priority.”
*
* @param unicastResponsesToMulticastBroadcastsBlocked
* value of {@code unicastResponsesToMulticastBroadcastsBlocked} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder unicastResponsesToMulticastBroadcastsBlocked(Boolean unicastResponsesToMulticastBroadcastsBlocked) {
this.unicastResponsesToMulticastBroadcastsBlocked = unicastResponsesToMulticastBroadcastsBlocked;
this.changedFields = changedFields.add("unicastResponsesToMulticastBroadcastsBlocked");
return this;
}
public WindowsFirewallNetworkProfile build() {
WindowsFirewallNetworkProfile _x = new WindowsFirewallNetworkProfile();
_x.contextPath = null;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.windowsFirewallNetworkProfile";
_x.authorizedApplicationRulesFromGroupPolicyMerged = authorizedApplicationRulesFromGroupPolicyMerged;
_x.connectionSecurityRulesFromGroupPolicyMerged = connectionSecurityRulesFromGroupPolicyMerged;
_x.firewallEnabled = firewallEnabled;
_x.globalPortRulesFromGroupPolicyMerged = globalPortRulesFromGroupPolicyMerged;
_x.inboundConnectionsBlocked = inboundConnectionsBlocked;
_x.inboundNotificationsBlocked = inboundNotificationsBlocked;
_x.incomingTrafficBlocked = incomingTrafficBlocked;
_x.outboundConnectionsBlocked = outboundConnectionsBlocked;
_x.policyRulesFromGroupPolicyMerged = policyRulesFromGroupPolicyMerged;
_x.securedPacketExemptionAllowed = securedPacketExemptionAllowed;
_x.stealthModeBlocked = stealthModeBlocked;
_x.unicastResponsesToMulticastBroadcastsBlocked = unicastResponsesToMulticastBroadcastsBlocked;
return _x;
}
}
private WindowsFirewallNetworkProfile _copy() {
WindowsFirewallNetworkProfile _x = new WindowsFirewallNetworkProfile();
_x.contextPath = contextPath;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.authorizedApplicationRulesFromGroupPolicyMerged = authorizedApplicationRulesFromGroupPolicyMerged;
_x.connectionSecurityRulesFromGroupPolicyMerged = connectionSecurityRulesFromGroupPolicyMerged;
_x.firewallEnabled = firewallEnabled;
_x.globalPortRulesFromGroupPolicyMerged = globalPortRulesFromGroupPolicyMerged;
_x.inboundConnectionsBlocked = inboundConnectionsBlocked;
_x.inboundNotificationsBlocked = inboundNotificationsBlocked;
_x.incomingTrafficBlocked = incomingTrafficBlocked;
_x.outboundConnectionsBlocked = outboundConnectionsBlocked;
_x.policyRulesFromGroupPolicyMerged = policyRulesFromGroupPolicyMerged;
_x.securedPacketExemptionAllowed = securedPacketExemptionAllowed;
_x.stealthModeBlocked = stealthModeBlocked;
_x.unicastResponsesToMulticastBroadcastsBlocked = unicastResponsesToMulticastBroadcastsBlocked;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("WindowsFirewallNetworkProfile[");
b.append("authorizedApplicationRulesFromGroupPolicyMerged=");
b.append(this.authorizedApplicationRulesFromGroupPolicyMerged);
b.append(", ");
b.append("connectionSecurityRulesFromGroupPolicyMerged=");
b.append(this.connectionSecurityRulesFromGroupPolicyMerged);
b.append(", ");
b.append("firewallEnabled=");
b.append(this.firewallEnabled);
b.append(", ");
b.append("globalPortRulesFromGroupPolicyMerged=");
b.append(this.globalPortRulesFromGroupPolicyMerged);
b.append(", ");
b.append("inboundConnectionsBlocked=");
b.append(this.inboundConnectionsBlocked);
b.append(", ");
b.append("inboundNotificationsBlocked=");
b.append(this.inboundNotificationsBlocked);
b.append(", ");
b.append("incomingTrafficBlocked=");
b.append(this.incomingTrafficBlocked);
b.append(", ");
b.append("outboundConnectionsBlocked=");
b.append(this.outboundConnectionsBlocked);
b.append(", ");
b.append("policyRulesFromGroupPolicyMerged=");
b.append(this.policyRulesFromGroupPolicyMerged);
b.append(", ");
b.append("securedPacketExemptionAllowed=");
b.append(this.securedPacketExemptionAllowed);
b.append(", ");
b.append("stealthModeBlocked=");
b.append(this.stealthModeBlocked);
b.append(", ");
b.append("unicastResponsesToMulticastBroadcastsBlocked=");
b.append(this.unicastResponsesToMulticastBroadcastsBlocked);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}