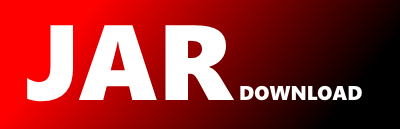
odata.msgraph.client.entity.AdminConsentRequestPolicy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph Show documentation
Show all versions of odata-client-msgraph Show documentation
Java client for use with the Microsoft Graph v1.0 endpoint
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.complex.AccessReviewReviewerScope;
@JsonPropertyOrder({
"@odata.type",
"isEnabled",
"notifyReviewers",
"remindersEnabled",
"requestDurationInDays",
"reviewers",
"version"})
@JsonInclude(Include.NON_NULL)
public class AdminConsentRequestPolicy extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.adminConsentRequestPolicy";
}
@JsonProperty("isEnabled")
protected Boolean isEnabled;
@JsonProperty("notifyReviewers")
protected Boolean notifyReviewers;
@JsonProperty("remindersEnabled")
protected Boolean remindersEnabled;
@JsonProperty("requestDurationInDays")
protected Integer requestDurationInDays;
@JsonProperty("reviewers")
protected List reviewers;
@JsonProperty("reviewers@nextLink")
protected String reviewersNextLink;
@JsonProperty("version")
protected Integer version;
protected AdminConsentRequestPolicy() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderAdminConsentRequestPolicy() {
return new Builder();
}
public static final class Builder {
private String id;
private Boolean isEnabled;
private Boolean notifyReviewers;
private Boolean remindersEnabled;
private Integer requestDurationInDays;
private List reviewers;
private String reviewersNextLink;
private Integer version;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder isEnabled(Boolean isEnabled) {
this.isEnabled = isEnabled;
this.changedFields = changedFields.add("isEnabled");
return this;
}
public Builder notifyReviewers(Boolean notifyReviewers) {
this.notifyReviewers = notifyReviewers;
this.changedFields = changedFields.add("notifyReviewers");
return this;
}
public Builder remindersEnabled(Boolean remindersEnabled) {
this.remindersEnabled = remindersEnabled;
this.changedFields = changedFields.add("remindersEnabled");
return this;
}
public Builder requestDurationInDays(Integer requestDurationInDays) {
this.requestDurationInDays = requestDurationInDays;
this.changedFields = changedFields.add("requestDurationInDays");
return this;
}
public Builder reviewers(List reviewers) {
this.reviewers = reviewers;
this.changedFields = changedFields.add("reviewers");
return this;
}
public Builder reviewers(AccessReviewReviewerScope... reviewers) {
return reviewers(Arrays.asList(reviewers));
}
public Builder reviewersNextLink(String reviewersNextLink) {
this.reviewersNextLink = reviewersNextLink;
this.changedFields = changedFields.add("reviewers");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
public AdminConsentRequestPolicy build() {
AdminConsentRequestPolicy _x = new AdminConsentRequestPolicy();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.adminConsentRequestPolicy";
_x.id = id;
_x.isEnabled = isEnabled;
_x.notifyReviewers = notifyReviewers;
_x.remindersEnabled = remindersEnabled;
_x.requestDurationInDays = requestDurationInDays;
_x.reviewers = reviewers;
_x.reviewersNextLink = reviewersNextLink;
_x.version = version;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
@Property(name="isEnabled")
@JsonIgnore
public Optional getIsEnabled() {
return Optional.ofNullable(isEnabled);
}
public AdminConsentRequestPolicy withIsEnabled(Boolean isEnabled) {
AdminConsentRequestPolicy _x = _copy();
_x.changedFields = changedFields.add("isEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.adminConsentRequestPolicy");
_x.isEnabled = isEnabled;
return _x;
}
@Property(name="notifyReviewers")
@JsonIgnore
public Optional getNotifyReviewers() {
return Optional.ofNullable(notifyReviewers);
}
public AdminConsentRequestPolicy withNotifyReviewers(Boolean notifyReviewers) {
AdminConsentRequestPolicy _x = _copy();
_x.changedFields = changedFields.add("notifyReviewers");
_x.odataType = Util.nvl(odataType, "microsoft.graph.adminConsentRequestPolicy");
_x.notifyReviewers = notifyReviewers;
return _x;
}
@Property(name="remindersEnabled")
@JsonIgnore
public Optional getRemindersEnabled() {
return Optional.ofNullable(remindersEnabled);
}
public AdminConsentRequestPolicy withRemindersEnabled(Boolean remindersEnabled) {
AdminConsentRequestPolicy _x = _copy();
_x.changedFields = changedFields.add("remindersEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.adminConsentRequestPolicy");
_x.remindersEnabled = remindersEnabled;
return _x;
}
@Property(name="requestDurationInDays")
@JsonIgnore
public Optional getRequestDurationInDays() {
return Optional.ofNullable(requestDurationInDays);
}
public AdminConsentRequestPolicy withRequestDurationInDays(Integer requestDurationInDays) {
AdminConsentRequestPolicy _x = _copy();
_x.changedFields = changedFields.add("requestDurationInDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.adminConsentRequestPolicy");
_x.requestDurationInDays = requestDurationInDays;
return _x;
}
@Property(name="reviewers")
@JsonIgnore
public CollectionPage getReviewers() {
return new CollectionPage(contextPath, AccessReviewReviewerScope.class, this.reviewers, Optional.ofNullable(reviewersNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public AdminConsentRequestPolicy withReviewers(List reviewers) {
AdminConsentRequestPolicy _x = _copy();
_x.changedFields = changedFields.add("reviewers");
_x.odataType = Util.nvl(odataType, "microsoft.graph.adminConsentRequestPolicy");
_x.reviewers = reviewers;
return _x;
}
@Property(name="reviewers")
@JsonIgnore
public CollectionPage getReviewers(HttpRequestOptions options) {
return new CollectionPage(contextPath, AccessReviewReviewerScope.class, this.reviewers, Optional.ofNullable(reviewersNextLink), Collections.emptyList(), options);
}
@Property(name="version")
@JsonIgnore
public Optional getVersion() {
return Optional.ofNullable(version);
}
public AdminConsentRequestPolicy withVersion(Integer version) {
AdminConsentRequestPolicy _x = _copy();
_x.changedFields = changedFields.add("version");
_x.odataType = Util.nvl(odataType, "microsoft.graph.adminConsentRequestPolicy");
_x.version = version;
return _x;
}
public AdminConsentRequestPolicy withUnmappedField(String name, Object value) {
AdminConsentRequestPolicy _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public AdminConsentRequestPolicy patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
AdminConsentRequestPolicy _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public AdminConsentRequestPolicy put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
AdminConsentRequestPolicy _x = _copy();
_x.changedFields = null;
return _x;
}
private AdminConsentRequestPolicy _copy() {
AdminConsentRequestPolicy _x = new AdminConsentRequestPolicy();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.isEnabled = isEnabled;
_x.notifyReviewers = notifyReviewers;
_x.remindersEnabled = remindersEnabled;
_x.requestDurationInDays = requestDurationInDays;
_x.reviewers = reviewers;
_x.version = version;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("AdminConsentRequestPolicy[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("isEnabled=");
b.append(this.isEnabled);
b.append(", ");
b.append("notifyReviewers=");
b.append(this.notifyReviewers);
b.append(", ");
b.append("remindersEnabled=");
b.append(this.remindersEnabled);
b.append(", ");
b.append("requestDurationInDays=");
b.append(this.requestDurationInDays);
b.append(", ");
b.append("reviewers=");
b.append(this.reviewers);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy