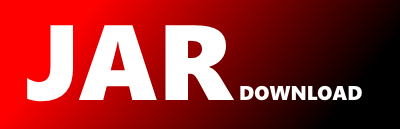
odata.msgraph.client.entity.AndroidCompliancePolicy Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.enums.AndroidRequiredPasswordType;
import odata.msgraph.client.enums.DeviceThreatProtectionLevel;
/**
* “This class contains compliance settings for Android.”
*/@JsonPropertyOrder({
"@odata.type",
"deviceThreatProtectionEnabled",
"deviceThreatProtectionRequiredSecurityLevel",
"minAndroidSecurityPatchLevel",
"osMaximumVersion",
"osMinimumVersion",
"passwordExpirationDays",
"passwordMinimumLength",
"passwordMinutesOfInactivityBeforeLock",
"passwordPreviousPasswordBlockCount",
"passwordRequired",
"passwordRequiredType",
"securityBlockJailbrokenDevices",
"securityDisableUsbDebugging",
"securityPreventInstallAppsFromUnknownSources",
"securityRequireCompanyPortalAppIntegrity",
"securityRequireGooglePlayServices",
"securityRequireSafetyNetAttestationBasicIntegrity",
"securityRequireSafetyNetAttestationCertifiedDevice",
"securityRequireUpToDateSecurityProviders",
"securityRequireVerifyApps",
"storageRequireEncryption"})
@JsonInclude(Include.NON_NULL)
public class AndroidCompliancePolicy extends DeviceCompliancePolicy implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.androidCompliancePolicy";
}
@JsonProperty("deviceThreatProtectionEnabled")
protected Boolean deviceThreatProtectionEnabled;
@JsonProperty("deviceThreatProtectionRequiredSecurityLevel")
protected DeviceThreatProtectionLevel deviceThreatProtectionRequiredSecurityLevel;
@JsonProperty("minAndroidSecurityPatchLevel")
protected String minAndroidSecurityPatchLevel;
@JsonProperty("osMaximumVersion")
protected String osMaximumVersion;
@JsonProperty("osMinimumVersion")
protected String osMinimumVersion;
@JsonProperty("passwordExpirationDays")
protected Integer passwordExpirationDays;
@JsonProperty("passwordMinimumLength")
protected Integer passwordMinimumLength;
@JsonProperty("passwordMinutesOfInactivityBeforeLock")
protected Integer passwordMinutesOfInactivityBeforeLock;
@JsonProperty("passwordPreviousPasswordBlockCount")
protected Integer passwordPreviousPasswordBlockCount;
@JsonProperty("passwordRequired")
protected Boolean passwordRequired;
@JsonProperty("passwordRequiredType")
protected AndroidRequiredPasswordType passwordRequiredType;
@JsonProperty("securityBlockJailbrokenDevices")
protected Boolean securityBlockJailbrokenDevices;
@JsonProperty("securityDisableUsbDebugging")
protected Boolean securityDisableUsbDebugging;
@JsonProperty("securityPreventInstallAppsFromUnknownSources")
protected Boolean securityPreventInstallAppsFromUnknownSources;
@JsonProperty("securityRequireCompanyPortalAppIntegrity")
protected Boolean securityRequireCompanyPortalAppIntegrity;
@JsonProperty("securityRequireGooglePlayServices")
protected Boolean securityRequireGooglePlayServices;
@JsonProperty("securityRequireSafetyNetAttestationBasicIntegrity")
protected Boolean securityRequireSafetyNetAttestationBasicIntegrity;
@JsonProperty("securityRequireSafetyNetAttestationCertifiedDevice")
protected Boolean securityRequireSafetyNetAttestationCertifiedDevice;
@JsonProperty("securityRequireUpToDateSecurityProviders")
protected Boolean securityRequireUpToDateSecurityProviders;
@JsonProperty("securityRequireVerifyApps")
protected Boolean securityRequireVerifyApps;
@JsonProperty("storageRequireEncryption")
protected Boolean storageRequireEncryption;
protected AndroidCompliancePolicy() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderAndroidCompliancePolicy() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private String displayName;
private OffsetDateTime lastModifiedDateTime;
private Integer version;
private List assignments;
private List deviceSettingStateSummaries;
private List deviceStatuses;
private DeviceComplianceDeviceOverview deviceStatusOverview;
private List scheduledActionsForRule;
private List userStatuses;
private DeviceComplianceUserOverview userStatusOverview;
private Boolean deviceThreatProtectionEnabled;
private DeviceThreatProtectionLevel deviceThreatProtectionRequiredSecurityLevel;
private String minAndroidSecurityPatchLevel;
private String osMaximumVersion;
private String osMinimumVersion;
private Integer passwordExpirationDays;
private Integer passwordMinimumLength;
private Integer passwordMinutesOfInactivityBeforeLock;
private Integer passwordPreviousPasswordBlockCount;
private Boolean passwordRequired;
private AndroidRequiredPasswordType passwordRequiredType;
private Boolean securityBlockJailbrokenDevices;
private Boolean securityDisableUsbDebugging;
private Boolean securityPreventInstallAppsFromUnknownSources;
private Boolean securityRequireCompanyPortalAppIntegrity;
private Boolean securityRequireGooglePlayServices;
private Boolean securityRequireSafetyNetAttestationBasicIntegrity;
private Boolean securityRequireSafetyNetAttestationCertifiedDevice;
private Boolean securityRequireUpToDateSecurityProviders;
private Boolean securityRequireVerifyApps;
private Boolean storageRequireEncryption;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder version(Integer version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
public Builder assignments(List assignments) {
this.assignments = assignments;
this.changedFields = changedFields.add("assignments");
return this;
}
public Builder assignments(DeviceCompliancePolicyAssignment... assignments) {
return assignments(Arrays.asList(assignments));
}
public Builder deviceSettingStateSummaries(List deviceSettingStateSummaries) {
this.deviceSettingStateSummaries = deviceSettingStateSummaries;
this.changedFields = changedFields.add("deviceSettingStateSummaries");
return this;
}
public Builder deviceSettingStateSummaries(SettingStateDeviceSummary... deviceSettingStateSummaries) {
return deviceSettingStateSummaries(Arrays.asList(deviceSettingStateSummaries));
}
public Builder deviceStatuses(List deviceStatuses) {
this.deviceStatuses = deviceStatuses;
this.changedFields = changedFields.add("deviceStatuses");
return this;
}
public Builder deviceStatuses(DeviceComplianceDeviceStatus... deviceStatuses) {
return deviceStatuses(Arrays.asList(deviceStatuses));
}
public Builder deviceStatusOverview(DeviceComplianceDeviceOverview deviceStatusOverview) {
this.deviceStatusOverview = deviceStatusOverview;
this.changedFields = changedFields.add("deviceStatusOverview");
return this;
}
public Builder scheduledActionsForRule(List scheduledActionsForRule) {
this.scheduledActionsForRule = scheduledActionsForRule;
this.changedFields = changedFields.add("scheduledActionsForRule");
return this;
}
public Builder scheduledActionsForRule(DeviceComplianceScheduledActionForRule... scheduledActionsForRule) {
return scheduledActionsForRule(Arrays.asList(scheduledActionsForRule));
}
public Builder userStatuses(List userStatuses) {
this.userStatuses = userStatuses;
this.changedFields = changedFields.add("userStatuses");
return this;
}
public Builder userStatuses(DeviceComplianceUserStatus... userStatuses) {
return userStatuses(Arrays.asList(userStatuses));
}
public Builder userStatusOverview(DeviceComplianceUserOverview userStatusOverview) {
this.userStatusOverview = userStatusOverview;
this.changedFields = changedFields.add("userStatusOverview");
return this;
}
/**
* “Require that devices have enabled device threat protection.”
*
* @param deviceThreatProtectionEnabled
* value of {@code deviceThreatProtectionEnabled} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceThreatProtectionEnabled(Boolean deviceThreatProtectionEnabled) {
this.deviceThreatProtectionEnabled = deviceThreatProtectionEnabled;
this.changedFields = changedFields.add("deviceThreatProtectionEnabled");
return this;
}
/**
* “Require Mobile Threat Protection minimum risk level to report noncompliance.”
*
* @param deviceThreatProtectionRequiredSecurityLevel
* value of {@code deviceThreatProtectionRequiredSecurityLevel} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceThreatProtectionRequiredSecurityLevel(DeviceThreatProtectionLevel deviceThreatProtectionRequiredSecurityLevel) {
this.deviceThreatProtectionRequiredSecurityLevel = deviceThreatProtectionRequiredSecurityLevel;
this.changedFields = changedFields.add("deviceThreatProtectionRequiredSecurityLevel");
return this;
}
/**
* “Minimum Android security patch level.”
*
* @param minAndroidSecurityPatchLevel
* value of {@code minAndroidSecurityPatchLevel} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder minAndroidSecurityPatchLevel(String minAndroidSecurityPatchLevel) {
this.minAndroidSecurityPatchLevel = minAndroidSecurityPatchLevel;
this.changedFields = changedFields.add("minAndroidSecurityPatchLevel");
return this;
}
/**
* “Maximum Android version.”
*
* @param osMaximumVersion
* value of {@code osMaximumVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder osMaximumVersion(String osMaximumVersion) {
this.osMaximumVersion = osMaximumVersion;
this.changedFields = changedFields.add("osMaximumVersion");
return this;
}
/**
* “Minimum Android version.”
*
* @param osMinimumVersion
* value of {@code osMinimumVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder osMinimumVersion(String osMinimumVersion) {
this.osMinimumVersion = osMinimumVersion;
this.changedFields = changedFields.add("osMinimumVersion");
return this;
}
/**
* “Number of days before the password expires. Valid values 1 to 365”
*
* @param passwordExpirationDays
* value of {@code passwordExpirationDays} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordExpirationDays(Integer passwordExpirationDays) {
this.passwordExpirationDays = passwordExpirationDays;
this.changedFields = changedFields.add("passwordExpirationDays");
return this;
}
/**
* “Minimum password length. Valid values 4 to 16”
*
* @param passwordMinimumLength
* value of {@code passwordMinimumLength} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordMinimumLength(Integer passwordMinimumLength) {
this.passwordMinimumLength = passwordMinimumLength;
this.changedFields = changedFields.add("passwordMinimumLength");
return this;
}
/**
* “Minutes of inactivity before a password is required.”
*
* @param passwordMinutesOfInactivityBeforeLock
* value of {@code passwordMinutesOfInactivityBeforeLock} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordMinutesOfInactivityBeforeLock(Integer passwordMinutesOfInactivityBeforeLock) {
this.passwordMinutesOfInactivityBeforeLock = passwordMinutesOfInactivityBeforeLock;
this.changedFields = changedFields.add("passwordMinutesOfInactivityBeforeLock");
return this;
}
/**
* “Number of previous passwords to block. Valid values 1 to 24”
*
* @param passwordPreviousPasswordBlockCount
* value of {@code passwordPreviousPasswordBlockCount} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordPreviousPasswordBlockCount(Integer passwordPreviousPasswordBlockCount) {
this.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
this.changedFields = changedFields.add("passwordPreviousPasswordBlockCount");
return this;
}
/**
* “Require a password to unlock device.”
*
* @param passwordRequired
* value of {@code passwordRequired} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordRequired(Boolean passwordRequired) {
this.passwordRequired = passwordRequired;
this.changedFields = changedFields.add("passwordRequired");
return this;
}
/**
* “Type of characters in password”
*
* @param passwordRequiredType
* value of {@code passwordRequiredType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder passwordRequiredType(AndroidRequiredPasswordType passwordRequiredType) {
this.passwordRequiredType = passwordRequiredType;
this.changedFields = changedFields.add("passwordRequiredType");
return this;
}
/**
* “Devices must not be jailbroken or rooted.”
*
* @param securityBlockJailbrokenDevices
* value of {@code securityBlockJailbrokenDevices} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder securityBlockJailbrokenDevices(Boolean securityBlockJailbrokenDevices) {
this.securityBlockJailbrokenDevices = securityBlockJailbrokenDevices;
this.changedFields = changedFields.add("securityBlockJailbrokenDevices");
return this;
}
/**
* “Disable USB debugging on Android devices.”
*
* @param securityDisableUsbDebugging
* value of {@code securityDisableUsbDebugging} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder securityDisableUsbDebugging(Boolean securityDisableUsbDebugging) {
this.securityDisableUsbDebugging = securityDisableUsbDebugging;
this.changedFields = changedFields.add("securityDisableUsbDebugging");
return this;
}
/**
* “Require that devices disallow installation of apps from unknown sources.”
*
* @param securityPreventInstallAppsFromUnknownSources
* value of {@code securityPreventInstallAppsFromUnknownSources} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder securityPreventInstallAppsFromUnknownSources(Boolean securityPreventInstallAppsFromUnknownSources) {
this.securityPreventInstallAppsFromUnknownSources = securityPreventInstallAppsFromUnknownSources;
this.changedFields = changedFields.add("securityPreventInstallAppsFromUnknownSources");
return this;
}
/**
* “Require the device to pass the Company Portal client app runtime integrity check
* .”
*
* @param securityRequireCompanyPortalAppIntegrity
* value of {@code securityRequireCompanyPortalAppIntegrity} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder securityRequireCompanyPortalAppIntegrity(Boolean securityRequireCompanyPortalAppIntegrity) {
this.securityRequireCompanyPortalAppIntegrity = securityRequireCompanyPortalAppIntegrity;
this.changedFields = changedFields.add("securityRequireCompanyPortalAppIntegrity");
return this;
}
/**
* “Require Google Play Services to be installed and enabled on the device.”
*
* @param securityRequireGooglePlayServices
* value of {@code securityRequireGooglePlayServices} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder securityRequireGooglePlayServices(Boolean securityRequireGooglePlayServices) {
this.securityRequireGooglePlayServices = securityRequireGooglePlayServices;
this.changedFields = changedFields.add("securityRequireGooglePlayServices");
return this;
}
/**
* “Require the device to pass the SafetyNet basic integrity check.”
*
* @param securityRequireSafetyNetAttestationBasicIntegrity
* value of {@code securityRequireSafetyNetAttestationBasicIntegrity} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder securityRequireSafetyNetAttestationBasicIntegrity(Boolean securityRequireSafetyNetAttestationBasicIntegrity) {
this.securityRequireSafetyNetAttestationBasicIntegrity = securityRequireSafetyNetAttestationBasicIntegrity;
this.changedFields = changedFields.add("securityRequireSafetyNetAttestationBasicIntegrity");
return this;
}
/**
* “Require the device to pass the SafetyNet certified device check.”
*
* @param securityRequireSafetyNetAttestationCertifiedDevice
* value of {@code securityRequireSafetyNetAttestationCertifiedDevice} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder securityRequireSafetyNetAttestationCertifiedDevice(Boolean securityRequireSafetyNetAttestationCertifiedDevice) {
this.securityRequireSafetyNetAttestationCertifiedDevice = securityRequireSafetyNetAttestationCertifiedDevice;
this.changedFields = changedFields.add("securityRequireSafetyNetAttestationCertifiedDevice");
return this;
}
/**
* “Require the device to have up to date security providers. The device will
* require Google Play Services to be enabled and up to date.”
*
* @param securityRequireUpToDateSecurityProviders
* value of {@code securityRequireUpToDateSecurityProviders} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder securityRequireUpToDateSecurityProviders(Boolean securityRequireUpToDateSecurityProviders) {
this.securityRequireUpToDateSecurityProviders = securityRequireUpToDateSecurityProviders;
this.changedFields = changedFields.add("securityRequireUpToDateSecurityProviders");
return this;
}
/**
* “Require the Android Verify apps feature is turned on.”
*
* @param securityRequireVerifyApps
* value of {@code securityRequireVerifyApps} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder securityRequireVerifyApps(Boolean securityRequireVerifyApps) {
this.securityRequireVerifyApps = securityRequireVerifyApps;
this.changedFields = changedFields.add("securityRequireVerifyApps");
return this;
}
/**
* “Require encryption on Android devices.”
*
* @param storageRequireEncryption
* value of {@code storageRequireEncryption} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder storageRequireEncryption(Boolean storageRequireEncryption) {
this.storageRequireEncryption = storageRequireEncryption;
this.changedFields = changedFields.add("storageRequireEncryption");
return this;
}
public AndroidCompliancePolicy build() {
AndroidCompliancePolicy _x = new AndroidCompliancePolicy();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.androidCompliancePolicy";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.version = version;
_x.assignments = assignments;
_x.deviceSettingStateSummaries = deviceSettingStateSummaries;
_x.deviceStatuses = deviceStatuses;
_x.deviceStatusOverview = deviceStatusOverview;
_x.scheduledActionsForRule = scheduledActionsForRule;
_x.userStatuses = userStatuses;
_x.userStatusOverview = userStatusOverview;
_x.deviceThreatProtectionEnabled = deviceThreatProtectionEnabled;
_x.deviceThreatProtectionRequiredSecurityLevel = deviceThreatProtectionRequiredSecurityLevel;
_x.minAndroidSecurityPatchLevel = minAndroidSecurityPatchLevel;
_x.osMaximumVersion = osMaximumVersion;
_x.osMinimumVersion = osMinimumVersion;
_x.passwordExpirationDays = passwordExpirationDays;
_x.passwordMinimumLength = passwordMinimumLength;
_x.passwordMinutesOfInactivityBeforeLock = passwordMinutesOfInactivityBeforeLock;
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
_x.passwordRequired = passwordRequired;
_x.passwordRequiredType = passwordRequiredType;
_x.securityBlockJailbrokenDevices = securityBlockJailbrokenDevices;
_x.securityDisableUsbDebugging = securityDisableUsbDebugging;
_x.securityPreventInstallAppsFromUnknownSources = securityPreventInstallAppsFromUnknownSources;
_x.securityRequireCompanyPortalAppIntegrity = securityRequireCompanyPortalAppIntegrity;
_x.securityRequireGooglePlayServices = securityRequireGooglePlayServices;
_x.securityRequireSafetyNetAttestationBasicIntegrity = securityRequireSafetyNetAttestationBasicIntegrity;
_x.securityRequireSafetyNetAttestationCertifiedDevice = securityRequireSafetyNetAttestationCertifiedDevice;
_x.securityRequireUpToDateSecurityProviders = securityRequireUpToDateSecurityProviders;
_x.securityRequireVerifyApps = securityRequireVerifyApps;
_x.storageRequireEncryption = storageRequireEncryption;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “Require that devices have enabled device threat protection.”
*
* @return property deviceThreatProtectionEnabled
*/
@Property(name="deviceThreatProtectionEnabled")
@JsonIgnore
public Optional getDeviceThreatProtectionEnabled() {
return Optional.ofNullable(deviceThreatProtectionEnabled);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceThreatProtectionEnabled} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Require that devices have enabled device threat protection.”
*
* @param deviceThreatProtectionEnabled
* new value of {@code deviceThreatProtectionEnabled} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceThreatProtectionEnabled} field changed
*/
public AndroidCompliancePolicy withDeviceThreatProtectionEnabled(Boolean deviceThreatProtectionEnabled) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("deviceThreatProtectionEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.deviceThreatProtectionEnabled = deviceThreatProtectionEnabled;
return _x;
}
/**
* “Require Mobile Threat Protection minimum risk level to report noncompliance.”
*
* @return property deviceThreatProtectionRequiredSecurityLevel
*/
@Property(name="deviceThreatProtectionRequiredSecurityLevel")
@JsonIgnore
public Optional getDeviceThreatProtectionRequiredSecurityLevel() {
return Optional.ofNullable(deviceThreatProtectionRequiredSecurityLevel);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceThreatProtectionRequiredSecurityLevel} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Require Mobile Threat Protection minimum risk level to report noncompliance.”
*
* @param deviceThreatProtectionRequiredSecurityLevel
* new value of {@code deviceThreatProtectionRequiredSecurityLevel} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceThreatProtectionRequiredSecurityLevel} field changed
*/
public AndroidCompliancePolicy withDeviceThreatProtectionRequiredSecurityLevel(DeviceThreatProtectionLevel deviceThreatProtectionRequiredSecurityLevel) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("deviceThreatProtectionRequiredSecurityLevel");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.deviceThreatProtectionRequiredSecurityLevel = deviceThreatProtectionRequiredSecurityLevel;
return _x;
}
/**
* “Minimum Android security patch level.”
*
* @return property minAndroidSecurityPatchLevel
*/
@Property(name="minAndroidSecurityPatchLevel")
@JsonIgnore
public Optional getMinAndroidSecurityPatchLevel() {
return Optional.ofNullable(minAndroidSecurityPatchLevel);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* minAndroidSecurityPatchLevel} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Minimum Android security patch level.”
*
* @param minAndroidSecurityPatchLevel
* new value of {@code minAndroidSecurityPatchLevel} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code minAndroidSecurityPatchLevel} field changed
*/
public AndroidCompliancePolicy withMinAndroidSecurityPatchLevel(String minAndroidSecurityPatchLevel) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("minAndroidSecurityPatchLevel");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.minAndroidSecurityPatchLevel = minAndroidSecurityPatchLevel;
return _x;
}
/**
* “Maximum Android version.”
*
* @return property osMaximumVersion
*/
@Property(name="osMaximumVersion")
@JsonIgnore
public Optional getOsMaximumVersion() {
return Optional.ofNullable(osMaximumVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code osMaximumVersion}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Maximum Android version.”
*
* @param osMaximumVersion
* new value of {@code osMaximumVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code osMaximumVersion} field changed
*/
public AndroidCompliancePolicy withOsMaximumVersion(String osMaximumVersion) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("osMaximumVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.osMaximumVersion = osMaximumVersion;
return _x;
}
/**
* “Minimum Android version.”
*
* @return property osMinimumVersion
*/
@Property(name="osMinimumVersion")
@JsonIgnore
public Optional getOsMinimumVersion() {
return Optional.ofNullable(osMinimumVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code osMinimumVersion}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Minimum Android version.”
*
* @param osMinimumVersion
* new value of {@code osMinimumVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code osMinimumVersion} field changed
*/
public AndroidCompliancePolicy withOsMinimumVersion(String osMinimumVersion) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("osMinimumVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.osMinimumVersion = osMinimumVersion;
return _x;
}
/**
* “Number of days before the password expires. Valid values 1 to 365”
*
* @return property passwordExpirationDays
*/
@Property(name="passwordExpirationDays")
@JsonIgnore
public Optional getPasswordExpirationDays() {
return Optional.ofNullable(passwordExpirationDays);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordExpirationDays} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Number of days before the password expires. Valid values 1 to 365”
*
* @param passwordExpirationDays
* new value of {@code passwordExpirationDays} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordExpirationDays} field changed
*/
public AndroidCompliancePolicy withPasswordExpirationDays(Integer passwordExpirationDays) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordExpirationDays");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.passwordExpirationDays = passwordExpirationDays;
return _x;
}
/**
* “Minimum password length. Valid values 4 to 16”
*
* @return property passwordMinimumLength
*/
@Property(name="passwordMinimumLength")
@JsonIgnore
public Optional getPasswordMinimumLength() {
return Optional.ofNullable(passwordMinimumLength);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordMinimumLength} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Minimum password length. Valid values 4 to 16”
*
* @param passwordMinimumLength
* new value of {@code passwordMinimumLength} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordMinimumLength} field changed
*/
public AndroidCompliancePolicy withPasswordMinimumLength(Integer passwordMinimumLength) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordMinimumLength");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.passwordMinimumLength = passwordMinimumLength;
return _x;
}
/**
* “Minutes of inactivity before a password is required.”
*
* @return property passwordMinutesOfInactivityBeforeLock
*/
@Property(name="passwordMinutesOfInactivityBeforeLock")
@JsonIgnore
public Optional getPasswordMinutesOfInactivityBeforeLock() {
return Optional.ofNullable(passwordMinutesOfInactivityBeforeLock);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordMinutesOfInactivityBeforeLock} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Minutes of inactivity before a password is required.”
*
* @param passwordMinutesOfInactivityBeforeLock
* new value of {@code passwordMinutesOfInactivityBeforeLock} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordMinutesOfInactivityBeforeLock} field changed
*/
public AndroidCompliancePolicy withPasswordMinutesOfInactivityBeforeLock(Integer passwordMinutesOfInactivityBeforeLock) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordMinutesOfInactivityBeforeLock");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.passwordMinutesOfInactivityBeforeLock = passwordMinutesOfInactivityBeforeLock;
return _x;
}
/**
* “Number of previous passwords to block. Valid values 1 to 24”
*
* @return property passwordPreviousPasswordBlockCount
*/
@Property(name="passwordPreviousPasswordBlockCount")
@JsonIgnore
public Optional getPasswordPreviousPasswordBlockCount() {
return Optional.ofNullable(passwordPreviousPasswordBlockCount);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordPreviousPasswordBlockCount} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Number of previous passwords to block. Valid values 1 to 24”
*
* @param passwordPreviousPasswordBlockCount
* new value of {@code passwordPreviousPasswordBlockCount} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordPreviousPasswordBlockCount} field changed
*/
public AndroidCompliancePolicy withPasswordPreviousPasswordBlockCount(Integer passwordPreviousPasswordBlockCount) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordPreviousPasswordBlockCount");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
return _x;
}
/**
* “Require a password to unlock device.”
*
* @return property passwordRequired
*/
@Property(name="passwordRequired")
@JsonIgnore
public Optional getPasswordRequired() {
return Optional.ofNullable(passwordRequired);
}
/**
* Returns an immutable copy of {@code this} with just the {@code passwordRequired}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Require a password to unlock device.”
*
* @param passwordRequired
* new value of {@code passwordRequired} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordRequired} field changed
*/
public AndroidCompliancePolicy withPasswordRequired(Boolean passwordRequired) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordRequired");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.passwordRequired = passwordRequired;
return _x;
}
/**
* “Type of characters in password”
*
* @return property passwordRequiredType
*/
@Property(name="passwordRequiredType")
@JsonIgnore
public Optional getPasswordRequiredType() {
return Optional.ofNullable(passwordRequiredType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* passwordRequiredType} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “Type of characters in password”
*
* @param passwordRequiredType
* new value of {@code passwordRequiredType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code passwordRequiredType} field changed
*/
public AndroidCompliancePolicy withPasswordRequiredType(AndroidRequiredPasswordType passwordRequiredType) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("passwordRequiredType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.passwordRequiredType = passwordRequiredType;
return _x;
}
/**
* “Devices must not be jailbroken or rooted.”
*
* @return property securityBlockJailbrokenDevices
*/
@Property(name="securityBlockJailbrokenDevices")
@JsonIgnore
public Optional getSecurityBlockJailbrokenDevices() {
return Optional.ofNullable(securityBlockJailbrokenDevices);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* securityBlockJailbrokenDevices} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Devices must not be jailbroken or rooted.”
*
* @param securityBlockJailbrokenDevices
* new value of {@code securityBlockJailbrokenDevices} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code securityBlockJailbrokenDevices} field changed
*/
public AndroidCompliancePolicy withSecurityBlockJailbrokenDevices(Boolean securityBlockJailbrokenDevices) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("securityBlockJailbrokenDevices");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.securityBlockJailbrokenDevices = securityBlockJailbrokenDevices;
return _x;
}
/**
* “Disable USB debugging on Android devices.”
*
* @return property securityDisableUsbDebugging
*/
@Property(name="securityDisableUsbDebugging")
@JsonIgnore
public Optional getSecurityDisableUsbDebugging() {
return Optional.ofNullable(securityDisableUsbDebugging);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* securityDisableUsbDebugging} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Disable USB debugging on Android devices.”
*
* @param securityDisableUsbDebugging
* new value of {@code securityDisableUsbDebugging} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code securityDisableUsbDebugging} field changed
*/
public AndroidCompliancePolicy withSecurityDisableUsbDebugging(Boolean securityDisableUsbDebugging) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("securityDisableUsbDebugging");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.securityDisableUsbDebugging = securityDisableUsbDebugging;
return _x;
}
/**
* “Require that devices disallow installation of apps from unknown sources.”
*
* @return property securityPreventInstallAppsFromUnknownSources
*/
@Property(name="securityPreventInstallAppsFromUnknownSources")
@JsonIgnore
public Optional getSecurityPreventInstallAppsFromUnknownSources() {
return Optional.ofNullable(securityPreventInstallAppsFromUnknownSources);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* securityPreventInstallAppsFromUnknownSources} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Require that devices disallow installation of apps from unknown sources.”
*
* @param securityPreventInstallAppsFromUnknownSources
* new value of {@code securityPreventInstallAppsFromUnknownSources} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code securityPreventInstallAppsFromUnknownSources} field changed
*/
public AndroidCompliancePolicy withSecurityPreventInstallAppsFromUnknownSources(Boolean securityPreventInstallAppsFromUnknownSources) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("securityPreventInstallAppsFromUnknownSources");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.securityPreventInstallAppsFromUnknownSources = securityPreventInstallAppsFromUnknownSources;
return _x;
}
/**
* “Require the device to pass the Company Portal client app runtime integrity check
* .”
*
* @return property securityRequireCompanyPortalAppIntegrity
*/
@Property(name="securityRequireCompanyPortalAppIntegrity")
@JsonIgnore
public Optional getSecurityRequireCompanyPortalAppIntegrity() {
return Optional.ofNullable(securityRequireCompanyPortalAppIntegrity);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* securityRequireCompanyPortalAppIntegrity} field changed. Field description below
* . The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Require the device to pass the Company Portal client app runtime integrity check
* .”
*
* @param securityRequireCompanyPortalAppIntegrity
* new value of {@code securityRequireCompanyPortalAppIntegrity} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code securityRequireCompanyPortalAppIntegrity} field changed
*/
public AndroidCompliancePolicy withSecurityRequireCompanyPortalAppIntegrity(Boolean securityRequireCompanyPortalAppIntegrity) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("securityRequireCompanyPortalAppIntegrity");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.securityRequireCompanyPortalAppIntegrity = securityRequireCompanyPortalAppIntegrity;
return _x;
}
/**
* “Require Google Play Services to be installed and enabled on the device.”
*
* @return property securityRequireGooglePlayServices
*/
@Property(name="securityRequireGooglePlayServices")
@JsonIgnore
public Optional getSecurityRequireGooglePlayServices() {
return Optional.ofNullable(securityRequireGooglePlayServices);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* securityRequireGooglePlayServices} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Require Google Play Services to be installed and enabled on the device.”
*
* @param securityRequireGooglePlayServices
* new value of {@code securityRequireGooglePlayServices} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code securityRequireGooglePlayServices} field changed
*/
public AndroidCompliancePolicy withSecurityRequireGooglePlayServices(Boolean securityRequireGooglePlayServices) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("securityRequireGooglePlayServices");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.securityRequireGooglePlayServices = securityRequireGooglePlayServices;
return _x;
}
/**
* “Require the device to pass the SafetyNet basic integrity check.”
*
* @return property securityRequireSafetyNetAttestationBasicIntegrity
*/
@Property(name="securityRequireSafetyNetAttestationBasicIntegrity")
@JsonIgnore
public Optional getSecurityRequireSafetyNetAttestationBasicIntegrity() {
return Optional.ofNullable(securityRequireSafetyNetAttestationBasicIntegrity);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* securityRequireSafetyNetAttestationBasicIntegrity} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “Require the device to pass the SafetyNet basic integrity check.”
*
* @param securityRequireSafetyNetAttestationBasicIntegrity
* new value of {@code securityRequireSafetyNetAttestationBasicIntegrity} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code securityRequireSafetyNetAttestationBasicIntegrity} field changed
*/
public AndroidCompliancePolicy withSecurityRequireSafetyNetAttestationBasicIntegrity(Boolean securityRequireSafetyNetAttestationBasicIntegrity) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("securityRequireSafetyNetAttestationBasicIntegrity");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.securityRequireSafetyNetAttestationBasicIntegrity = securityRequireSafetyNetAttestationBasicIntegrity;
return _x;
}
/**
* “Require the device to pass the SafetyNet certified device check.”
*
* @return property securityRequireSafetyNetAttestationCertifiedDevice
*/
@Property(name="securityRequireSafetyNetAttestationCertifiedDevice")
@JsonIgnore
public Optional getSecurityRequireSafetyNetAttestationCertifiedDevice() {
return Optional.ofNullable(securityRequireSafetyNetAttestationCertifiedDevice);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* securityRequireSafetyNetAttestationCertifiedDevice} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “Require the device to pass the SafetyNet certified device check.”
*
* @param securityRequireSafetyNetAttestationCertifiedDevice
* new value of {@code securityRequireSafetyNetAttestationCertifiedDevice} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code securityRequireSafetyNetAttestationCertifiedDevice} field changed
*/
public AndroidCompliancePolicy withSecurityRequireSafetyNetAttestationCertifiedDevice(Boolean securityRequireSafetyNetAttestationCertifiedDevice) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("securityRequireSafetyNetAttestationCertifiedDevice");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.securityRequireSafetyNetAttestationCertifiedDevice = securityRequireSafetyNetAttestationCertifiedDevice;
return _x;
}
/**
* “Require the device to have up to date security providers. The device will
* require Google Play Services to be enabled and up to date.”
*
* @return property securityRequireUpToDateSecurityProviders
*/
@Property(name="securityRequireUpToDateSecurityProviders")
@JsonIgnore
public Optional getSecurityRequireUpToDateSecurityProviders() {
return Optional.ofNullable(securityRequireUpToDateSecurityProviders);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* securityRequireUpToDateSecurityProviders} field changed. Field description below
* . The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Require the device to have up to date security providers. The device will
* require Google Play Services to be enabled and up to date.”
*
* @param securityRequireUpToDateSecurityProviders
* new value of {@code securityRequireUpToDateSecurityProviders} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code securityRequireUpToDateSecurityProviders} field changed
*/
public AndroidCompliancePolicy withSecurityRequireUpToDateSecurityProviders(Boolean securityRequireUpToDateSecurityProviders) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("securityRequireUpToDateSecurityProviders");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.securityRequireUpToDateSecurityProviders = securityRequireUpToDateSecurityProviders;
return _x;
}
/**
* “Require the Android Verify apps feature is turned on.”
*
* @return property securityRequireVerifyApps
*/
@Property(name="securityRequireVerifyApps")
@JsonIgnore
public Optional getSecurityRequireVerifyApps() {
return Optional.ofNullable(securityRequireVerifyApps);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* securityRequireVerifyApps} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Require the Android Verify apps feature is turned on.”
*
* @param securityRequireVerifyApps
* new value of {@code securityRequireVerifyApps} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code securityRequireVerifyApps} field changed
*/
public AndroidCompliancePolicy withSecurityRequireVerifyApps(Boolean securityRequireVerifyApps) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("securityRequireVerifyApps");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.securityRequireVerifyApps = securityRequireVerifyApps;
return _x;
}
/**
* “Require encryption on Android devices.”
*
* @return property storageRequireEncryption
*/
@Property(name="storageRequireEncryption")
@JsonIgnore
public Optional getStorageRequireEncryption() {
return Optional.ofNullable(storageRequireEncryption);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* storageRequireEncryption} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Require encryption on Android devices.”
*
* @param storageRequireEncryption
* new value of {@code storageRequireEncryption} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code storageRequireEncryption} field changed
*/
public AndroidCompliancePolicy withStorageRequireEncryption(Boolean storageRequireEncryption) {
AndroidCompliancePolicy _x = _copy();
_x.changedFields = changedFields.add("storageRequireEncryption");
_x.odataType = Util.nvl(odataType, "microsoft.graph.androidCompliancePolicy");
_x.storageRequireEncryption = storageRequireEncryption;
return _x;
}
public AndroidCompliancePolicy withUnmappedField(String name, Object value) {
AndroidCompliancePolicy _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public AndroidCompliancePolicy patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
AndroidCompliancePolicy _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public AndroidCompliancePolicy put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
AndroidCompliancePolicy _x = _copy();
_x.changedFields = null;
return _x;
}
private AndroidCompliancePolicy _copy() {
AndroidCompliancePolicy _x = new AndroidCompliancePolicy();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.version = version;
_x.assignments = assignments;
_x.deviceSettingStateSummaries = deviceSettingStateSummaries;
_x.deviceStatuses = deviceStatuses;
_x.deviceStatusOverview = deviceStatusOverview;
_x.scheduledActionsForRule = scheduledActionsForRule;
_x.userStatuses = userStatuses;
_x.userStatusOverview = userStatusOverview;
_x.deviceThreatProtectionEnabled = deviceThreatProtectionEnabled;
_x.deviceThreatProtectionRequiredSecurityLevel = deviceThreatProtectionRequiredSecurityLevel;
_x.minAndroidSecurityPatchLevel = minAndroidSecurityPatchLevel;
_x.osMaximumVersion = osMaximumVersion;
_x.osMinimumVersion = osMinimumVersion;
_x.passwordExpirationDays = passwordExpirationDays;
_x.passwordMinimumLength = passwordMinimumLength;
_x.passwordMinutesOfInactivityBeforeLock = passwordMinutesOfInactivityBeforeLock;
_x.passwordPreviousPasswordBlockCount = passwordPreviousPasswordBlockCount;
_x.passwordRequired = passwordRequired;
_x.passwordRequiredType = passwordRequiredType;
_x.securityBlockJailbrokenDevices = securityBlockJailbrokenDevices;
_x.securityDisableUsbDebugging = securityDisableUsbDebugging;
_x.securityPreventInstallAppsFromUnknownSources = securityPreventInstallAppsFromUnknownSources;
_x.securityRequireCompanyPortalAppIntegrity = securityRequireCompanyPortalAppIntegrity;
_x.securityRequireGooglePlayServices = securityRequireGooglePlayServices;
_x.securityRequireSafetyNetAttestationBasicIntegrity = securityRequireSafetyNetAttestationBasicIntegrity;
_x.securityRequireSafetyNetAttestationCertifiedDevice = securityRequireSafetyNetAttestationCertifiedDevice;
_x.securityRequireUpToDateSecurityProviders = securityRequireUpToDateSecurityProviders;
_x.securityRequireVerifyApps = securityRequireVerifyApps;
_x.storageRequireEncryption = storageRequireEncryption;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("AndroidCompliancePolicy[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("assignments=");
b.append(this.assignments);
b.append(", ");
b.append("deviceSettingStateSummaries=");
b.append(this.deviceSettingStateSummaries);
b.append(", ");
b.append("deviceStatuses=");
b.append(this.deviceStatuses);
b.append(", ");
b.append("deviceStatusOverview=");
b.append(this.deviceStatusOverview);
b.append(", ");
b.append("scheduledActionsForRule=");
b.append(this.scheduledActionsForRule);
b.append(", ");
b.append("userStatuses=");
b.append(this.userStatuses);
b.append(", ");
b.append("userStatusOverview=");
b.append(this.userStatusOverview);
b.append(", ");
b.append("deviceThreatProtectionEnabled=");
b.append(this.deviceThreatProtectionEnabled);
b.append(", ");
b.append("deviceThreatProtectionRequiredSecurityLevel=");
b.append(this.deviceThreatProtectionRequiredSecurityLevel);
b.append(", ");
b.append("minAndroidSecurityPatchLevel=");
b.append(this.minAndroidSecurityPatchLevel);
b.append(", ");
b.append("osMaximumVersion=");
b.append(this.osMaximumVersion);
b.append(", ");
b.append("osMinimumVersion=");
b.append(this.osMinimumVersion);
b.append(", ");
b.append("passwordExpirationDays=");
b.append(this.passwordExpirationDays);
b.append(", ");
b.append("passwordMinimumLength=");
b.append(this.passwordMinimumLength);
b.append(", ");
b.append("passwordMinutesOfInactivityBeforeLock=");
b.append(this.passwordMinutesOfInactivityBeforeLock);
b.append(", ");
b.append("passwordPreviousPasswordBlockCount=");
b.append(this.passwordPreviousPasswordBlockCount);
b.append(", ");
b.append("passwordRequired=");
b.append(this.passwordRequired);
b.append(", ");
b.append("passwordRequiredType=");
b.append(this.passwordRequiredType);
b.append(", ");
b.append("securityBlockJailbrokenDevices=");
b.append(this.securityBlockJailbrokenDevices);
b.append(", ");
b.append("securityDisableUsbDebugging=");
b.append(this.securityDisableUsbDebugging);
b.append(", ");
b.append("securityPreventInstallAppsFromUnknownSources=");
b.append(this.securityPreventInstallAppsFromUnknownSources);
b.append(", ");
b.append("securityRequireCompanyPortalAppIntegrity=");
b.append(this.securityRequireCompanyPortalAppIntegrity);
b.append(", ");
b.append("securityRequireGooglePlayServices=");
b.append(this.securityRequireGooglePlayServices);
b.append(", ");
b.append("securityRequireSafetyNetAttestationBasicIntegrity=");
b.append(this.securityRequireSafetyNetAttestationBasicIntegrity);
b.append(", ");
b.append("securityRequireSafetyNetAttestationCertifiedDevice=");
b.append(this.securityRequireSafetyNetAttestationCertifiedDevice);
b.append(", ");
b.append("securityRequireUpToDateSecurityProviders=");
b.append(this.securityRequireUpToDateSecurityProviders);
b.append(", ");
b.append("securityRequireVerifyApps=");
b.append(this.securityRequireVerifyApps);
b.append(", ");
b.append("storageRequireEncryption=");
b.append(this.storageRequireEncryption);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}