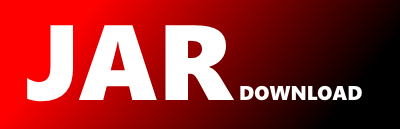
odata.msgraph.client.entity.ColumnDefinition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph Show documentation
Show all versions of odata-client-msgraph Show documentation
Java client for use with the Microsoft Graph v1.0 endpoint
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Optional;
import odata.msgraph.client.complex.BooleanColumn;
import odata.msgraph.client.complex.CalculatedColumn;
import odata.msgraph.client.complex.ChoiceColumn;
import odata.msgraph.client.complex.ColumnValidation;
import odata.msgraph.client.complex.ContentApprovalStatusColumn;
import odata.msgraph.client.complex.ContentTypeInfo;
import odata.msgraph.client.complex.CurrencyColumn;
import odata.msgraph.client.complex.DateTimeColumn;
import odata.msgraph.client.complex.DefaultColumnValue;
import odata.msgraph.client.complex.GeolocationColumn;
import odata.msgraph.client.complex.HyperlinkOrPictureColumn;
import odata.msgraph.client.complex.LookupColumn;
import odata.msgraph.client.complex.NumberColumn;
import odata.msgraph.client.complex.PersonOrGroupColumn;
import odata.msgraph.client.complex.TermColumn;
import odata.msgraph.client.complex.TextColumn;
import odata.msgraph.client.complex.ThumbnailColumn;
import odata.msgraph.client.entity.request.ColumnDefinitionRequest;
import odata.msgraph.client.enums.ColumnTypes;
@JsonPropertyOrder({
"@odata.type",
"boolean",
"calculated",
"choice",
"columnGroup",
"contentApprovalStatus",
"currency",
"dateTime",
"defaultValue",
"description",
"displayName",
"enforceUniqueValues",
"geolocation",
"hidden",
"hyperlinkOrPicture",
"indexed",
"isDeletable",
"isReorderable",
"isSealed",
"lookup",
"name",
"number",
"personOrGroup",
"propagateChanges",
"readOnly",
"required",
"sourceContentType",
"term",
"text",
"thumbnail",
"type",
"validation"})
@JsonInclude(Include.NON_NULL)
public class ColumnDefinition extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.columnDefinition";
}
@JsonProperty("boolean")
protected BooleanColumn boolean_;
@JsonProperty("calculated")
protected CalculatedColumn calculated;
@JsonProperty("choice")
protected ChoiceColumn choice;
@JsonProperty("columnGroup")
protected String columnGroup;
@JsonProperty("contentApprovalStatus")
protected ContentApprovalStatusColumn contentApprovalStatus;
@JsonProperty("currency")
protected CurrencyColumn currency;
@JsonProperty("dateTime")
protected DateTimeColumn dateTime;
@JsonProperty("defaultValue")
protected DefaultColumnValue defaultValue;
@JsonProperty("description")
protected String description;
@JsonProperty("displayName")
protected String displayName;
@JsonProperty("enforceUniqueValues")
protected Boolean enforceUniqueValues;
@JsonProperty("geolocation")
protected GeolocationColumn geolocation;
@JsonProperty("hidden")
protected Boolean hidden;
@JsonProperty("hyperlinkOrPicture")
protected HyperlinkOrPictureColumn hyperlinkOrPicture;
@JsonProperty("indexed")
protected Boolean indexed;
@JsonProperty("isDeletable")
protected Boolean isDeletable;
@JsonProperty("isReorderable")
protected Boolean isReorderable;
@JsonProperty("isSealed")
protected Boolean isSealed;
@JsonProperty("lookup")
protected LookupColumn lookup;
@JsonProperty("name")
protected String name;
@JsonProperty("number")
protected NumberColumn number;
@JsonProperty("personOrGroup")
protected PersonOrGroupColumn personOrGroup;
@JsonProperty("propagateChanges")
protected Boolean propagateChanges;
@JsonProperty("readOnly")
protected Boolean readOnly;
@JsonProperty("required")
protected Boolean required;
@JsonProperty("sourceContentType")
protected ContentTypeInfo sourceContentType;
@JsonProperty("term")
protected TermColumn term;
@JsonProperty("text")
protected TextColumn text;
@JsonProperty("thumbnail")
protected ThumbnailColumn thumbnail;
@JsonProperty("type")
protected ColumnTypes type;
@JsonProperty("validation")
protected ColumnValidation validation;
protected ColumnDefinition() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderColumnDefinition() {
return new Builder();
}
public static final class Builder {
private String id;
private BooleanColumn boolean_;
private CalculatedColumn calculated;
private ChoiceColumn choice;
private String columnGroup;
private ContentApprovalStatusColumn contentApprovalStatus;
private CurrencyColumn currency;
private DateTimeColumn dateTime;
private DefaultColumnValue defaultValue;
private String description;
private String displayName;
private Boolean enforceUniqueValues;
private GeolocationColumn geolocation;
private Boolean hidden;
private HyperlinkOrPictureColumn hyperlinkOrPicture;
private Boolean indexed;
private Boolean isDeletable;
private Boolean isReorderable;
private Boolean isSealed;
private LookupColumn lookup;
private String name;
private NumberColumn number;
private PersonOrGroupColumn personOrGroup;
private Boolean propagateChanges;
private Boolean readOnly;
private Boolean required;
private ContentTypeInfo sourceContentType;
private TermColumn term;
private TextColumn text;
private ThumbnailColumn thumbnail;
private ColumnTypes type;
private ColumnValidation validation;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder boolean_(BooleanColumn boolean_) {
this.boolean_ = boolean_;
this.changedFields = changedFields.add("boolean");
return this;
}
public Builder calculated(CalculatedColumn calculated) {
this.calculated = calculated;
this.changedFields = changedFields.add("calculated");
return this;
}
public Builder choice(ChoiceColumn choice) {
this.choice = choice;
this.changedFields = changedFields.add("choice");
return this;
}
public Builder columnGroup(String columnGroup) {
this.columnGroup = columnGroup;
this.changedFields = changedFields.add("columnGroup");
return this;
}
public Builder contentApprovalStatus(ContentApprovalStatusColumn contentApprovalStatus) {
this.contentApprovalStatus = contentApprovalStatus;
this.changedFields = changedFields.add("contentApprovalStatus");
return this;
}
public Builder currency(CurrencyColumn currency) {
this.currency = currency;
this.changedFields = changedFields.add("currency");
return this;
}
public Builder dateTime(DateTimeColumn dateTime) {
this.dateTime = dateTime;
this.changedFields = changedFields.add("dateTime");
return this;
}
public Builder defaultValue(DefaultColumnValue defaultValue) {
this.defaultValue = defaultValue;
this.changedFields = changedFields.add("defaultValue");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder enforceUniqueValues(Boolean enforceUniqueValues) {
this.enforceUniqueValues = enforceUniqueValues;
this.changedFields = changedFields.add("enforceUniqueValues");
return this;
}
public Builder geolocation(GeolocationColumn geolocation) {
this.geolocation = geolocation;
this.changedFields = changedFields.add("geolocation");
return this;
}
public Builder hidden(Boolean hidden) {
this.hidden = hidden;
this.changedFields = changedFields.add("hidden");
return this;
}
public Builder hyperlinkOrPicture(HyperlinkOrPictureColumn hyperlinkOrPicture) {
this.hyperlinkOrPicture = hyperlinkOrPicture;
this.changedFields = changedFields.add("hyperlinkOrPicture");
return this;
}
public Builder indexed(Boolean indexed) {
this.indexed = indexed;
this.changedFields = changedFields.add("indexed");
return this;
}
public Builder isDeletable(Boolean isDeletable) {
this.isDeletable = isDeletable;
this.changedFields = changedFields.add("isDeletable");
return this;
}
public Builder isReorderable(Boolean isReorderable) {
this.isReorderable = isReorderable;
this.changedFields = changedFields.add("isReorderable");
return this;
}
public Builder isSealed(Boolean isSealed) {
this.isSealed = isSealed;
this.changedFields = changedFields.add("isSealed");
return this;
}
public Builder lookup(LookupColumn lookup) {
this.lookup = lookup;
this.changedFields = changedFields.add("lookup");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Builder number(NumberColumn number) {
this.number = number;
this.changedFields = changedFields.add("number");
return this;
}
public Builder personOrGroup(PersonOrGroupColumn personOrGroup) {
this.personOrGroup = personOrGroup;
this.changedFields = changedFields.add("personOrGroup");
return this;
}
public Builder propagateChanges(Boolean propagateChanges) {
this.propagateChanges = propagateChanges;
this.changedFields = changedFields.add("propagateChanges");
return this;
}
public Builder readOnly(Boolean readOnly) {
this.readOnly = readOnly;
this.changedFields = changedFields.add("readOnly");
return this;
}
public Builder required(Boolean required) {
this.required = required;
this.changedFields = changedFields.add("required");
return this;
}
public Builder sourceContentType(ContentTypeInfo sourceContentType) {
this.sourceContentType = sourceContentType;
this.changedFields = changedFields.add("sourceContentType");
return this;
}
public Builder term(TermColumn term) {
this.term = term;
this.changedFields = changedFields.add("term");
return this;
}
public Builder text(TextColumn text) {
this.text = text;
this.changedFields = changedFields.add("text");
return this;
}
public Builder thumbnail(ThumbnailColumn thumbnail) {
this.thumbnail = thumbnail;
this.changedFields = changedFields.add("thumbnail");
return this;
}
public Builder type(ColumnTypes type) {
this.type = type;
this.changedFields = changedFields.add("type");
return this;
}
public Builder validation(ColumnValidation validation) {
this.validation = validation;
this.changedFields = changedFields.add("validation");
return this;
}
public ColumnDefinition build() {
ColumnDefinition _x = new ColumnDefinition();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.columnDefinition";
_x.id = id;
_x.boolean_ = boolean_;
_x.calculated = calculated;
_x.choice = choice;
_x.columnGroup = columnGroup;
_x.contentApprovalStatus = contentApprovalStatus;
_x.currency = currency;
_x.dateTime = dateTime;
_x.defaultValue = defaultValue;
_x.description = description;
_x.displayName = displayName;
_x.enforceUniqueValues = enforceUniqueValues;
_x.geolocation = geolocation;
_x.hidden = hidden;
_x.hyperlinkOrPicture = hyperlinkOrPicture;
_x.indexed = indexed;
_x.isDeletable = isDeletable;
_x.isReorderable = isReorderable;
_x.isSealed = isSealed;
_x.lookup = lookup;
_x.name = name;
_x.number = number;
_x.personOrGroup = personOrGroup;
_x.propagateChanges = propagateChanges;
_x.readOnly = readOnly;
_x.required = required;
_x.sourceContentType = sourceContentType;
_x.term = term;
_x.text = text;
_x.thumbnail = thumbnail;
_x.type = type;
_x.validation = validation;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
@Property(name="boolean")
@JsonIgnore
public Optional getBoolean() {
return Optional.ofNullable(boolean_);
}
public ColumnDefinition withBoolean(BooleanColumn boolean_) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("boolean");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.boolean_ = boolean_;
return _x;
}
@Property(name="calculated")
@JsonIgnore
public Optional getCalculated() {
return Optional.ofNullable(calculated);
}
public ColumnDefinition withCalculated(CalculatedColumn calculated) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("calculated");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.calculated = calculated;
return _x;
}
@Property(name="choice")
@JsonIgnore
public Optional getChoice() {
return Optional.ofNullable(choice);
}
public ColumnDefinition withChoice(ChoiceColumn choice) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("choice");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.choice = choice;
return _x;
}
@Property(name="columnGroup")
@JsonIgnore
public Optional getColumnGroup() {
return Optional.ofNullable(columnGroup);
}
public ColumnDefinition withColumnGroup(String columnGroup) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("columnGroup");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.columnGroup = columnGroup;
return _x;
}
@Property(name="contentApprovalStatus")
@JsonIgnore
public Optional getContentApprovalStatus() {
return Optional.ofNullable(contentApprovalStatus);
}
public ColumnDefinition withContentApprovalStatus(ContentApprovalStatusColumn contentApprovalStatus) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("contentApprovalStatus");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.contentApprovalStatus = contentApprovalStatus;
return _x;
}
@Property(name="currency")
@JsonIgnore
public Optional getCurrency() {
return Optional.ofNullable(currency);
}
public ColumnDefinition withCurrency(CurrencyColumn currency) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("currency");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.currency = currency;
return _x;
}
@Property(name="dateTime")
@JsonIgnore
public Optional getDateTime() {
return Optional.ofNullable(dateTime);
}
public ColumnDefinition withDateTime(DateTimeColumn dateTime) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("dateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.dateTime = dateTime;
return _x;
}
@Property(name="defaultValue")
@JsonIgnore
public Optional getDefaultValue() {
return Optional.ofNullable(defaultValue);
}
public ColumnDefinition withDefaultValue(DefaultColumnValue defaultValue) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("defaultValue");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.defaultValue = defaultValue;
return _x;
}
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
public ColumnDefinition withDescription(String description) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.description = description;
return _x;
}
@Property(name="displayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
public ColumnDefinition withDisplayName(String displayName) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("displayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.displayName = displayName;
return _x;
}
@Property(name="enforceUniqueValues")
@JsonIgnore
public Optional getEnforceUniqueValues() {
return Optional.ofNullable(enforceUniqueValues);
}
public ColumnDefinition withEnforceUniqueValues(Boolean enforceUniqueValues) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("enforceUniqueValues");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.enforceUniqueValues = enforceUniqueValues;
return _x;
}
@Property(name="geolocation")
@JsonIgnore
public Optional getGeolocation() {
return Optional.ofNullable(geolocation);
}
public ColumnDefinition withGeolocation(GeolocationColumn geolocation) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("geolocation");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.geolocation = geolocation;
return _x;
}
@Property(name="hidden")
@JsonIgnore
public Optional getHidden() {
return Optional.ofNullable(hidden);
}
public ColumnDefinition withHidden(Boolean hidden) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("hidden");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.hidden = hidden;
return _x;
}
@Property(name="hyperlinkOrPicture")
@JsonIgnore
public Optional getHyperlinkOrPicture() {
return Optional.ofNullable(hyperlinkOrPicture);
}
public ColumnDefinition withHyperlinkOrPicture(HyperlinkOrPictureColumn hyperlinkOrPicture) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("hyperlinkOrPicture");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.hyperlinkOrPicture = hyperlinkOrPicture;
return _x;
}
@Property(name="indexed")
@JsonIgnore
public Optional getIndexed() {
return Optional.ofNullable(indexed);
}
public ColumnDefinition withIndexed(Boolean indexed) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("indexed");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.indexed = indexed;
return _x;
}
@Property(name="isDeletable")
@JsonIgnore
public Optional getIsDeletable() {
return Optional.ofNullable(isDeletable);
}
public ColumnDefinition withIsDeletable(Boolean isDeletable) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("isDeletable");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.isDeletable = isDeletable;
return _x;
}
@Property(name="isReorderable")
@JsonIgnore
public Optional getIsReorderable() {
return Optional.ofNullable(isReorderable);
}
public ColumnDefinition withIsReorderable(Boolean isReorderable) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("isReorderable");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.isReorderable = isReorderable;
return _x;
}
@Property(name="isSealed")
@JsonIgnore
public Optional getIsSealed() {
return Optional.ofNullable(isSealed);
}
public ColumnDefinition withIsSealed(Boolean isSealed) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("isSealed");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.isSealed = isSealed;
return _x;
}
@Property(name="lookup")
@JsonIgnore
public Optional getLookup() {
return Optional.ofNullable(lookup);
}
public ColumnDefinition withLookup(LookupColumn lookup) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("lookup");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.lookup = lookup;
return _x;
}
@Property(name="name")
@JsonIgnore
public Optional getName() {
return Optional.ofNullable(name);
}
public ColumnDefinition withName(String name) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("name");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.name = name;
return _x;
}
@Property(name="number")
@JsonIgnore
public Optional getNumber() {
return Optional.ofNullable(number);
}
public ColumnDefinition withNumber(NumberColumn number) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("number");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.number = number;
return _x;
}
@Property(name="personOrGroup")
@JsonIgnore
public Optional getPersonOrGroup() {
return Optional.ofNullable(personOrGroup);
}
public ColumnDefinition withPersonOrGroup(PersonOrGroupColumn personOrGroup) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("personOrGroup");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.personOrGroup = personOrGroup;
return _x;
}
@Property(name="propagateChanges")
@JsonIgnore
public Optional getPropagateChanges() {
return Optional.ofNullable(propagateChanges);
}
public ColumnDefinition withPropagateChanges(Boolean propagateChanges) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("propagateChanges");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.propagateChanges = propagateChanges;
return _x;
}
@Property(name="readOnly")
@JsonIgnore
public Optional getReadOnly() {
return Optional.ofNullable(readOnly);
}
public ColumnDefinition withReadOnly(Boolean readOnly) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("readOnly");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.readOnly = readOnly;
return _x;
}
@Property(name="required")
@JsonIgnore
public Optional getRequired() {
return Optional.ofNullable(required);
}
public ColumnDefinition withRequired(Boolean required) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("required");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.required = required;
return _x;
}
@Property(name="sourceContentType")
@JsonIgnore
public Optional getSourceContentType() {
return Optional.ofNullable(sourceContentType);
}
public ColumnDefinition withSourceContentType(ContentTypeInfo sourceContentType) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("sourceContentType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.sourceContentType = sourceContentType;
return _x;
}
@Property(name="term")
@JsonIgnore
public Optional getTerm() {
return Optional.ofNullable(term);
}
public ColumnDefinition withTerm(TermColumn term) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("term");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.term = term;
return _x;
}
@Property(name="text")
@JsonIgnore
public Optional getText() {
return Optional.ofNullable(text);
}
public ColumnDefinition withText(TextColumn text) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("text");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.text = text;
return _x;
}
@Property(name="thumbnail")
@JsonIgnore
public Optional getThumbnail() {
return Optional.ofNullable(thumbnail);
}
public ColumnDefinition withThumbnail(ThumbnailColumn thumbnail) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("thumbnail");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.thumbnail = thumbnail;
return _x;
}
@Property(name="type")
@JsonIgnore
public Optional getType() {
return Optional.ofNullable(type);
}
public ColumnDefinition withType(ColumnTypes type) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("type");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.type = type;
return _x;
}
@Property(name="validation")
@JsonIgnore
public Optional getValidation() {
return Optional.ofNullable(validation);
}
public ColumnDefinition withValidation(ColumnValidation validation) {
ColumnDefinition _x = _copy();
_x.changedFields = changedFields.add("validation");
_x.odataType = Util.nvl(odataType, "microsoft.graph.columnDefinition");
_x.validation = validation;
return _x;
}
public ColumnDefinition withUnmappedField(String name, Object value) {
ColumnDefinition _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="sourceColumn")
@JsonIgnore
public ColumnDefinitionRequest getSourceColumn() {
return new ColumnDefinitionRequest(contextPath.addSegment("sourceColumn"), RequestHelper.getValue(unmappedFields, "sourceColumn"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ColumnDefinition patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
ColumnDefinition _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ColumnDefinition put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
ColumnDefinition _x = _copy();
_x.changedFields = null;
return _x;
}
private ColumnDefinition _copy() {
ColumnDefinition _x = new ColumnDefinition();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.boolean_ = boolean_;
_x.calculated = calculated;
_x.choice = choice;
_x.columnGroup = columnGroup;
_x.contentApprovalStatus = contentApprovalStatus;
_x.currency = currency;
_x.dateTime = dateTime;
_x.defaultValue = defaultValue;
_x.description = description;
_x.displayName = displayName;
_x.enforceUniqueValues = enforceUniqueValues;
_x.geolocation = geolocation;
_x.hidden = hidden;
_x.hyperlinkOrPicture = hyperlinkOrPicture;
_x.indexed = indexed;
_x.isDeletable = isDeletable;
_x.isReorderable = isReorderable;
_x.isSealed = isSealed;
_x.lookup = lookup;
_x.name = name;
_x.number = number;
_x.personOrGroup = personOrGroup;
_x.propagateChanges = propagateChanges;
_x.readOnly = readOnly;
_x.required = required;
_x.sourceContentType = sourceContentType;
_x.term = term;
_x.text = text;
_x.thumbnail = thumbnail;
_x.type = type;
_x.validation = validation;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ColumnDefinition[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("boolean=");
b.append(this.boolean_);
b.append(", ");
b.append("calculated=");
b.append(this.calculated);
b.append(", ");
b.append("choice=");
b.append(this.choice);
b.append(", ");
b.append("columnGroup=");
b.append(this.columnGroup);
b.append(", ");
b.append("contentApprovalStatus=");
b.append(this.contentApprovalStatus);
b.append(", ");
b.append("currency=");
b.append(this.currency);
b.append(", ");
b.append("dateTime=");
b.append(this.dateTime);
b.append(", ");
b.append("defaultValue=");
b.append(this.defaultValue);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("enforceUniqueValues=");
b.append(this.enforceUniqueValues);
b.append(", ");
b.append("geolocation=");
b.append(this.geolocation);
b.append(", ");
b.append("hidden=");
b.append(this.hidden);
b.append(", ");
b.append("hyperlinkOrPicture=");
b.append(this.hyperlinkOrPicture);
b.append(", ");
b.append("indexed=");
b.append(this.indexed);
b.append(", ");
b.append("isDeletable=");
b.append(this.isDeletable);
b.append(", ");
b.append("isReorderable=");
b.append(this.isReorderable);
b.append(", ");
b.append("isSealed=");
b.append(this.isSealed);
b.append(", ");
b.append("lookup=");
b.append(this.lookup);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append(", ");
b.append("number=");
b.append(this.number);
b.append(", ");
b.append("personOrGroup=");
b.append(this.personOrGroup);
b.append(", ");
b.append("propagateChanges=");
b.append(this.propagateChanges);
b.append(", ");
b.append("readOnly=");
b.append(this.readOnly);
b.append(", ");
b.append("required=");
b.append(this.required);
b.append(", ");
b.append("sourceContentType=");
b.append(this.sourceContentType);
b.append(", ");
b.append("term=");
b.append(this.term);
b.append(", ");
b.append("text=");
b.append(this.text);
b.append(", ");
b.append("thumbnail=");
b.append(this.thumbnail);
b.append(", ");
b.append("type=");
b.append(this.type);
b.append(", ");
b.append("validation=");
b.append(this.validation);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy