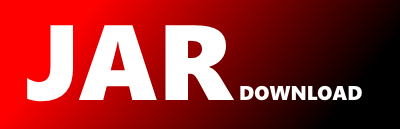
odata.msgraph.client.entity.DeviceManagement Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.guavamini.Preconditions;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPageNonEntityRequest;
import com.github.davidmoten.odata.client.FunctionRequestReturningNonCollection;
import com.github.davidmoten.odata.client.FunctionRequestReturningNonCollectionUnwrapped;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Function;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.UUID;
import odata.msgraph.client.complex.DeviceManagementSettings;
import odata.msgraph.client.complex.DeviceProtectionOverview;
import odata.msgraph.client.complex.IntuneBrand;
import odata.msgraph.client.complex.RolePermission;
import odata.msgraph.client.complex.UserExperienceAnalyticsSettings;
import odata.msgraph.client.complex.UserExperienceAnalyticsWorkFromAnywhereDevicesSummary;
import odata.msgraph.client.complex.WindowsMalwareOverview;
import odata.msgraph.client.entity.collection.request.AuditEventCollectionRequest;
import odata.msgraph.client.entity.collection.request.ComplianceManagementPartnerCollectionRequest;
import odata.msgraph.client.entity.collection.request.DetectedAppCollectionRequest;
import odata.msgraph.client.entity.collection.request.DeviceAndAppManagementRoleAssignmentCollectionRequest;
import odata.msgraph.client.entity.collection.request.DeviceCategoryCollectionRequest;
import odata.msgraph.client.entity.collection.request.DeviceCompliancePolicyCollectionRequest;
import odata.msgraph.client.entity.collection.request.DeviceCompliancePolicySettingStateSummaryCollectionRequest;
import odata.msgraph.client.entity.collection.request.DeviceConfigurationCollectionRequest;
import odata.msgraph.client.entity.collection.request.DeviceEnrollmentConfigurationCollectionRequest;
import odata.msgraph.client.entity.collection.request.DeviceManagementExchangeConnectorCollectionRequest;
import odata.msgraph.client.entity.collection.request.DeviceManagementPartnerCollectionRequest;
import odata.msgraph.client.entity.collection.request.DeviceManagementTroubleshootingEventCollectionRequest;
import odata.msgraph.client.entity.collection.request.ImportedWindowsAutopilotDeviceIdentityCollectionRequest;
import odata.msgraph.client.entity.collection.request.IosUpdateDeviceStatusCollectionRequest;
import odata.msgraph.client.entity.collection.request.ManagedDeviceCollectionRequest;
import odata.msgraph.client.entity.collection.request.MobileAppTroubleshootingEventCollectionRequest;
import odata.msgraph.client.entity.collection.request.MobileThreatDefenseConnectorCollectionRequest;
import odata.msgraph.client.entity.collection.request.NotificationMessageTemplateCollectionRequest;
import odata.msgraph.client.entity.collection.request.RemoteAssistancePartnerCollectionRequest;
import odata.msgraph.client.entity.collection.request.ResourceOperationCollectionRequest;
import odata.msgraph.client.entity.collection.request.RoleDefinitionCollectionRequest;
import odata.msgraph.client.entity.collection.request.TelecomExpenseManagementPartnerCollectionRequest;
import odata.msgraph.client.entity.collection.request.TermsAndConditionsCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsAppHealthAppPerformanceByAppVersionDetailsCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsAppHealthAppPerformanceByAppVersionDeviceIdCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsAppHealthAppPerformanceByOSVersionCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsAppHealthApplicationPerformanceCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsAppHealthDeviceModelPerformanceCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsAppHealthDevicePerformanceCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsAppHealthDevicePerformanceDetailsCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsAppHealthOSVersionPerformanceCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsBaselineCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsCategoryCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsDevicePerformanceCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsDeviceScoresCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsDeviceStartupHistoryCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsDeviceStartupProcessCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsDeviceStartupProcessPerformanceCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsMetricHistoryCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsModelScoresCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsScoreHistoryCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsWorkFromAnywhereMetricCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserExperienceAnalyticsWorkFromAnywhereModelPerformanceCollectionRequest;
import odata.msgraph.client.entity.collection.request.WindowsAutopilotDeviceIdentityCollectionRequest;
import odata.msgraph.client.entity.collection.request.WindowsInformationProtectionAppLearningSummaryCollectionRequest;
import odata.msgraph.client.entity.collection.request.WindowsInformationProtectionNetworkLearningSummaryCollectionRequest;
import odata.msgraph.client.entity.collection.request.WindowsMalwareInformationCollectionRequest;
import odata.msgraph.client.entity.request.ApplePushNotificationCertificateRequest;
import odata.msgraph.client.entity.request.DeviceCompliancePolicyDeviceStateSummaryRequest;
import odata.msgraph.client.entity.request.DeviceConfigurationDeviceStateSummaryRequest;
import odata.msgraph.client.entity.request.DeviceManagementReportsRequest;
import odata.msgraph.client.entity.request.ManagedDeviceOverviewRequest;
import odata.msgraph.client.entity.request.OnPremisesConditionalAccessSettingsRequest;
import odata.msgraph.client.entity.request.SoftwareUpdateStatusSummaryRequest;
import odata.msgraph.client.entity.request.UserExperienceAnalyticsCategoryRequest;
import odata.msgraph.client.entity.request.UserExperienceAnalyticsOverviewRequest;
import odata.msgraph.client.entity.request.UserExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetricRequest;
import odata.msgraph.client.enums.DeviceManagementSubscriptionState;
/**
* “Singleton entity that acts as a container for all device management
* functionality.”
*/@JsonPropertyOrder({
"@odata.type",
"intuneAccountId",
"settings",
"intuneBrand",
"deviceProtectionOverview",
"subscriptionState",
"userExperienceAnalyticsSettings",
"windowsMalwareOverview",
"termsAndConditions",
"auditEvents",
"deviceCompliancePolicies",
"deviceCompliancePolicyDeviceStateSummary",
"deviceCompliancePolicySettingStateSummaries",
"deviceConfigurationDeviceStateSummaries",
"deviceConfigurations",
"iosUpdateStatuses",
"complianceManagementPartners",
"conditionalAccessSettings",
"deviceCategories",
"deviceEnrollmentConfigurations",
"deviceManagementPartners",
"exchangeConnectors",
"mobileThreatDefenseConnectors",
"applePushNotificationCertificate",
"detectedApps",
"managedDevices",
"mobileAppTroubleshootingEvents",
"userExperienceAnalyticsAppHealthApplicationPerformance",
"userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails",
"userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId",
"userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion",
"userExperienceAnalyticsAppHealthDeviceModelPerformance",
"userExperienceAnalyticsAppHealthDevicePerformance",
"userExperienceAnalyticsAppHealthDevicePerformanceDetails",
"userExperienceAnalyticsAppHealthOSVersionPerformance",
"userExperienceAnalyticsAppHealthOverview",
"userExperienceAnalyticsBaselines",
"userExperienceAnalyticsCategories",
"userExperienceAnalyticsDevicePerformance",
"userExperienceAnalyticsDeviceScores",
"userExperienceAnalyticsDeviceStartupHistory",
"userExperienceAnalyticsDeviceStartupProcesses",
"userExperienceAnalyticsDeviceStartupProcessPerformance",
"userExperienceAnalyticsMetricHistory",
"userExperienceAnalyticsModelScores",
"userExperienceAnalyticsOverview",
"userExperienceAnalyticsScoreHistory",
"userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric",
"userExperienceAnalyticsWorkFromAnywhereMetrics",
"userExperienceAnalyticsWorkFromAnywhereModelPerformance",
"windowsMalwareInformation",
"importedWindowsAutopilotDeviceIdentities",
"windowsAutopilotDeviceIdentities",
"notificationMessageTemplates",
"resourceOperations",
"roleAssignments",
"roleDefinitions",
"remoteAssistancePartners",
"reports",
"telecomExpenseManagementPartners",
"troubleshootingEvents",
"windowsInformationProtectionAppLearningSummaries",
"windowsInformationProtectionNetworkLearningSummaries"})
@JsonInclude(Include.NON_NULL)
public class DeviceManagement extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.deviceManagement";
}
@JsonProperty("intuneAccountId")
protected UUID intuneAccountId;
@JsonProperty("settings")
protected DeviceManagementSettings settings;
@JsonProperty("intuneBrand")
protected IntuneBrand intuneBrand;
@JsonProperty("deviceProtectionOverview")
protected DeviceProtectionOverview deviceProtectionOverview;
@JsonProperty("subscriptionState")
protected DeviceManagementSubscriptionState subscriptionState;
@JsonProperty("userExperienceAnalyticsSettings")
protected UserExperienceAnalyticsSettings userExperienceAnalyticsSettings;
@JsonProperty("windowsMalwareOverview")
protected WindowsMalwareOverview windowsMalwareOverview;
@JsonProperty("termsAndConditions")
protected List termsAndConditions;
@JsonProperty("auditEvents")
protected List auditEvents;
@JsonProperty("deviceCompliancePolicies")
protected List deviceCompliancePolicies;
@JsonProperty("deviceCompliancePolicyDeviceStateSummary")
protected DeviceCompliancePolicyDeviceStateSummary deviceCompliancePolicyDeviceStateSummary;
@JsonProperty("deviceCompliancePolicySettingStateSummaries")
protected List deviceCompliancePolicySettingStateSummaries;
@JsonProperty("deviceConfigurationDeviceStateSummaries")
protected DeviceConfigurationDeviceStateSummary deviceConfigurationDeviceStateSummaries;
@JsonProperty("deviceConfigurations")
protected List deviceConfigurations;
@JsonProperty("iosUpdateStatuses")
protected List iosUpdateStatuses;
@JsonProperty("complianceManagementPartners")
protected List complianceManagementPartners;
@JsonProperty("conditionalAccessSettings")
protected OnPremisesConditionalAccessSettings conditionalAccessSettings;
@JsonProperty("deviceCategories")
protected List deviceCategories;
@JsonProperty("deviceEnrollmentConfigurations")
protected List deviceEnrollmentConfigurations;
@JsonProperty("deviceManagementPartners")
protected List deviceManagementPartners;
@JsonProperty("exchangeConnectors")
protected List exchangeConnectors;
@JsonProperty("mobileThreatDefenseConnectors")
protected List mobileThreatDefenseConnectors;
@JsonProperty("applePushNotificationCertificate")
protected ApplePushNotificationCertificate applePushNotificationCertificate;
@JsonProperty("detectedApps")
protected List detectedApps;
@JsonProperty("managedDevices")
protected List managedDevices;
@JsonProperty("mobileAppTroubleshootingEvents")
protected List mobileAppTroubleshootingEvents;
@JsonProperty("userExperienceAnalyticsAppHealthApplicationPerformance")
protected List userExperienceAnalyticsAppHealthApplicationPerformance;
@JsonProperty("userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails")
protected List userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails;
@JsonProperty("userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId")
protected List userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId;
@JsonProperty("userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion")
protected List userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion;
@JsonProperty("userExperienceAnalyticsAppHealthDeviceModelPerformance")
protected List userExperienceAnalyticsAppHealthDeviceModelPerformance;
@JsonProperty("userExperienceAnalyticsAppHealthDevicePerformance")
protected List userExperienceAnalyticsAppHealthDevicePerformance;
@JsonProperty("userExperienceAnalyticsAppHealthDevicePerformanceDetails")
protected List userExperienceAnalyticsAppHealthDevicePerformanceDetails;
@JsonProperty("userExperienceAnalyticsAppHealthOSVersionPerformance")
protected List userExperienceAnalyticsAppHealthOSVersionPerformance;
@JsonProperty("userExperienceAnalyticsAppHealthOverview")
protected UserExperienceAnalyticsCategory userExperienceAnalyticsAppHealthOverview;
@JsonProperty("userExperienceAnalyticsBaselines")
protected List userExperienceAnalyticsBaselines;
@JsonProperty("userExperienceAnalyticsCategories")
protected List userExperienceAnalyticsCategories;
@JsonProperty("userExperienceAnalyticsDevicePerformance")
protected List userExperienceAnalyticsDevicePerformance;
@JsonProperty("userExperienceAnalyticsDeviceScores")
protected List userExperienceAnalyticsDeviceScores;
@JsonProperty("userExperienceAnalyticsDeviceStartupHistory")
protected List userExperienceAnalyticsDeviceStartupHistory;
@JsonProperty("userExperienceAnalyticsDeviceStartupProcesses")
protected List userExperienceAnalyticsDeviceStartupProcesses;
@JsonProperty("userExperienceAnalyticsDeviceStartupProcessPerformance")
protected List userExperienceAnalyticsDeviceStartupProcessPerformance;
@JsonProperty("userExperienceAnalyticsMetricHistory")
protected List userExperienceAnalyticsMetricHistory;
@JsonProperty("userExperienceAnalyticsModelScores")
protected List userExperienceAnalyticsModelScores;
@JsonProperty("userExperienceAnalyticsOverview")
protected UserExperienceAnalyticsOverview userExperienceAnalyticsOverview;
@JsonProperty("userExperienceAnalyticsScoreHistory")
protected List userExperienceAnalyticsScoreHistory;
@JsonProperty("userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric")
protected UserExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric;
@JsonProperty("userExperienceAnalyticsWorkFromAnywhereMetrics")
protected List userExperienceAnalyticsWorkFromAnywhereMetrics;
@JsonProperty("userExperienceAnalyticsWorkFromAnywhereModelPerformance")
protected List userExperienceAnalyticsWorkFromAnywhereModelPerformance;
@JsonProperty("windowsMalwareInformation")
protected List windowsMalwareInformation;
@JsonProperty("importedWindowsAutopilotDeviceIdentities")
protected List importedWindowsAutopilotDeviceIdentities;
@JsonProperty("windowsAutopilotDeviceIdentities")
protected List windowsAutopilotDeviceIdentities;
@JsonProperty("notificationMessageTemplates")
protected List notificationMessageTemplates;
@JsonProperty("resourceOperations")
protected List resourceOperations;
@JsonProperty("roleAssignments")
protected List roleAssignments;
@JsonProperty("roleDefinitions")
protected List roleDefinitions;
@JsonProperty("remoteAssistancePartners")
protected List remoteAssistancePartners;
@JsonProperty("reports")
protected DeviceManagementReports reports;
@JsonProperty("telecomExpenseManagementPartners")
protected List telecomExpenseManagementPartners;
@JsonProperty("troubleshootingEvents")
protected List troubleshootingEvents;
@JsonProperty("windowsInformationProtectionAppLearningSummaries")
protected List windowsInformationProtectionAppLearningSummaries;
@JsonProperty("windowsInformationProtectionNetworkLearningSummaries")
protected List windowsInformationProtectionNetworkLearningSummaries;
protected DeviceManagement() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderDeviceManagement() {
return new Builder();
}
public static final class Builder {
private String id;
private UUID intuneAccountId;
private DeviceManagementSettings settings;
private IntuneBrand intuneBrand;
private DeviceProtectionOverview deviceProtectionOverview;
private DeviceManagementSubscriptionState subscriptionState;
private UserExperienceAnalyticsSettings userExperienceAnalyticsSettings;
private WindowsMalwareOverview windowsMalwareOverview;
private List termsAndConditions;
private List auditEvents;
private List deviceCompliancePolicies;
private DeviceCompliancePolicyDeviceStateSummary deviceCompliancePolicyDeviceStateSummary;
private List deviceCompliancePolicySettingStateSummaries;
private DeviceConfigurationDeviceStateSummary deviceConfigurationDeviceStateSummaries;
private List deviceConfigurations;
private List iosUpdateStatuses;
private List complianceManagementPartners;
private OnPremisesConditionalAccessSettings conditionalAccessSettings;
private List deviceCategories;
private List deviceEnrollmentConfigurations;
private List deviceManagementPartners;
private List exchangeConnectors;
private List mobileThreatDefenseConnectors;
private ApplePushNotificationCertificate applePushNotificationCertificate;
private List detectedApps;
private List managedDevices;
private List mobileAppTroubleshootingEvents;
private List userExperienceAnalyticsAppHealthApplicationPerformance;
private List userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails;
private List userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId;
private List userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion;
private List userExperienceAnalyticsAppHealthDeviceModelPerformance;
private List userExperienceAnalyticsAppHealthDevicePerformance;
private List userExperienceAnalyticsAppHealthDevicePerformanceDetails;
private List userExperienceAnalyticsAppHealthOSVersionPerformance;
private UserExperienceAnalyticsCategory userExperienceAnalyticsAppHealthOverview;
private List userExperienceAnalyticsBaselines;
private List userExperienceAnalyticsCategories;
private List userExperienceAnalyticsDevicePerformance;
private List userExperienceAnalyticsDeviceScores;
private List userExperienceAnalyticsDeviceStartupHistory;
private List userExperienceAnalyticsDeviceStartupProcesses;
private List userExperienceAnalyticsDeviceStartupProcessPerformance;
private List userExperienceAnalyticsMetricHistory;
private List userExperienceAnalyticsModelScores;
private UserExperienceAnalyticsOverview userExperienceAnalyticsOverview;
private List userExperienceAnalyticsScoreHistory;
private UserExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric;
private List userExperienceAnalyticsWorkFromAnywhereMetrics;
private List userExperienceAnalyticsWorkFromAnywhereModelPerformance;
private List windowsMalwareInformation;
private List importedWindowsAutopilotDeviceIdentities;
private List windowsAutopilotDeviceIdentities;
private List notificationMessageTemplates;
private List resourceOperations;
private List roleAssignments;
private List roleDefinitions;
private List remoteAssistancePartners;
private DeviceManagementReports reports;
private List telecomExpenseManagementPartners;
private List troubleshootingEvents;
private List windowsInformationProtectionAppLearningSummaries;
private List windowsInformationProtectionNetworkLearningSummaries;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
/**
* “Intune Account Id for given tenant”
*
* @param intuneAccountId
* value of {@code intuneAccountId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder intuneAccountId(UUID intuneAccountId) {
this.intuneAccountId = intuneAccountId;
this.changedFields = changedFields.add("intuneAccountId");
return this;
}
/**
* “Account level settings.”
*
* @param settings
* value of {@code settings} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder settings(DeviceManagementSettings settings) {
this.settings = settings;
this.changedFields = changedFields.add("settings");
return this;
}
/**
* “intuneBrand contains data which is used in customizing the appearance of the
* Company Portal applications as well as the end user web portal.”
*
* @param intuneBrand
* value of {@code intuneBrand} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder intuneBrand(IntuneBrand intuneBrand) {
this.intuneBrand = intuneBrand;
this.changedFields = changedFields.add("intuneBrand");
return this;
}
/**
* “Device protection overview.”
*
* @param deviceProtectionOverview
* value of {@code deviceProtectionOverview} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceProtectionOverview(DeviceProtectionOverview deviceProtectionOverview) {
this.deviceProtectionOverview = deviceProtectionOverview;
this.changedFields = changedFields.add("deviceProtectionOverview");
return this;
}
/**
* “Tenant mobile device management subscription state.”
*
* @param subscriptionState
* value of {@code subscriptionState} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder subscriptionState(DeviceManagementSubscriptionState subscriptionState) {
this.subscriptionState = subscriptionState;
this.changedFields = changedFields.add("subscriptionState");
return this;
}
/**
* “User experience analytics device settings”
*
* @param userExperienceAnalyticsSettings
* value of {@code userExperienceAnalyticsSettings} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsSettings(UserExperienceAnalyticsSettings userExperienceAnalyticsSettings) {
this.userExperienceAnalyticsSettings = userExperienceAnalyticsSettings;
this.changedFields = changedFields.add("userExperienceAnalyticsSettings");
return this;
}
/**
* “Malware overview for windows devices.”
*
* @param windowsMalwareOverview
* value of {@code windowsMalwareOverview} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder windowsMalwareOverview(WindowsMalwareOverview windowsMalwareOverview) {
this.windowsMalwareOverview = windowsMalwareOverview;
this.changedFields = changedFields.add("windowsMalwareOverview");
return this;
}
/**
* “The terms and conditions associated with device management of the company.”
*
* @param termsAndConditions
* value of {@code termsAndConditions} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder termsAndConditions(List termsAndConditions) {
this.termsAndConditions = termsAndConditions;
this.changedFields = changedFields.add("termsAndConditions");
return this;
}
/**
* “The terms and conditions associated with device management of the company.”
*
* @param termsAndConditions
* value of {@code termsAndConditions} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder termsAndConditions(TermsAndConditions... termsAndConditions) {
return termsAndConditions(Arrays.asList(termsAndConditions));
}
/**
* “The Audit Events”
*
* @param auditEvents
* value of {@code auditEvents} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder auditEvents(List auditEvents) {
this.auditEvents = auditEvents;
this.changedFields = changedFields.add("auditEvents");
return this;
}
/**
* “The Audit Events”
*
* @param auditEvents
* value of {@code auditEvents} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder auditEvents(AuditEvent... auditEvents) {
return auditEvents(Arrays.asList(auditEvents));
}
/**
* “The device compliance policies.”
*
* @param deviceCompliancePolicies
* value of {@code deviceCompliancePolicies} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceCompliancePolicies(List deviceCompliancePolicies) {
this.deviceCompliancePolicies = deviceCompliancePolicies;
this.changedFields = changedFields.add("deviceCompliancePolicies");
return this;
}
/**
* “The device compliance policies.”
*
* @param deviceCompliancePolicies
* value of {@code deviceCompliancePolicies} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceCompliancePolicies(DeviceCompliancePolicy... deviceCompliancePolicies) {
return deviceCompliancePolicies(Arrays.asList(deviceCompliancePolicies));
}
/**
* “The device compliance state summary for this account.”
*
* @param deviceCompliancePolicyDeviceStateSummary
* value of {@code deviceCompliancePolicyDeviceStateSummary} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceCompliancePolicyDeviceStateSummary(DeviceCompliancePolicyDeviceStateSummary deviceCompliancePolicyDeviceStateSummary) {
this.deviceCompliancePolicyDeviceStateSummary = deviceCompliancePolicyDeviceStateSummary;
this.changedFields = changedFields.add("deviceCompliancePolicyDeviceStateSummary");
return this;
}
/**
* “The summary states of compliance policy settings for this account.”
*
* @param deviceCompliancePolicySettingStateSummaries
* value of {@code deviceCompliancePolicySettingStateSummaries} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceCompliancePolicySettingStateSummaries(List deviceCompliancePolicySettingStateSummaries) {
this.deviceCompliancePolicySettingStateSummaries = deviceCompliancePolicySettingStateSummaries;
this.changedFields = changedFields.add("deviceCompliancePolicySettingStateSummaries");
return this;
}
/**
* “The summary states of compliance policy settings for this account.”
*
* @param deviceCompliancePolicySettingStateSummaries
* value of {@code deviceCompliancePolicySettingStateSummaries} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceCompliancePolicySettingStateSummaries(DeviceCompliancePolicySettingStateSummary... deviceCompliancePolicySettingStateSummaries) {
return deviceCompliancePolicySettingStateSummaries(Arrays.asList(deviceCompliancePolicySettingStateSummaries));
}
/**
* “The device configuration device state summary for this account.”
*
* @param deviceConfigurationDeviceStateSummaries
* value of {@code deviceConfigurationDeviceStateSummaries} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceConfigurationDeviceStateSummaries(DeviceConfigurationDeviceStateSummary deviceConfigurationDeviceStateSummaries) {
this.deviceConfigurationDeviceStateSummaries = deviceConfigurationDeviceStateSummaries;
this.changedFields = changedFields.add("deviceConfigurationDeviceStateSummaries");
return this;
}
/**
* “The device configurations.”
*
* @param deviceConfigurations
* value of {@code deviceConfigurations} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceConfigurations(List deviceConfigurations) {
this.deviceConfigurations = deviceConfigurations;
this.changedFields = changedFields.add("deviceConfigurations");
return this;
}
/**
* “The device configurations.”
*
* @param deviceConfigurations
* value of {@code deviceConfigurations} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceConfigurations(DeviceConfiguration... deviceConfigurations) {
return deviceConfigurations(Arrays.asList(deviceConfigurations));
}
/**
* “The IOS software update installation statuses for this account.”
*
* @param iosUpdateStatuses
* value of {@code iosUpdateStatuses} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder iosUpdateStatuses(List iosUpdateStatuses) {
this.iosUpdateStatuses = iosUpdateStatuses;
this.changedFields = changedFields.add("iosUpdateStatuses");
return this;
}
/**
* “The IOS software update installation statuses for this account.”
*
* @param iosUpdateStatuses
* value of {@code iosUpdateStatuses} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder iosUpdateStatuses(IosUpdateDeviceStatus... iosUpdateStatuses) {
return iosUpdateStatuses(Arrays.asList(iosUpdateStatuses));
}
/**
* “The list of Compliance Management Partners configured by the tenant.”
*
* @param complianceManagementPartners
* value of {@code complianceManagementPartners} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder complianceManagementPartners(List complianceManagementPartners) {
this.complianceManagementPartners = complianceManagementPartners;
this.changedFields = changedFields.add("complianceManagementPartners");
return this;
}
/**
* “The list of Compliance Management Partners configured by the tenant.”
*
* @param complianceManagementPartners
* value of {@code complianceManagementPartners} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder complianceManagementPartners(ComplianceManagementPartner... complianceManagementPartners) {
return complianceManagementPartners(Arrays.asList(complianceManagementPartners));
}
/**
* “The Exchange on premises conditional access settings. On premises conditional
* access will require devices to be both enrolled and compliant for mail access”
*
* @param conditionalAccessSettings
* value of {@code conditionalAccessSettings} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder conditionalAccessSettings(OnPremisesConditionalAccessSettings conditionalAccessSettings) {
this.conditionalAccessSettings = conditionalAccessSettings;
this.changedFields = changedFields.add("conditionalAccessSettings");
return this;
}
/**
* “The list of device categories with the tenant.”
*
* @param deviceCategories
* value of {@code deviceCategories} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceCategories(List deviceCategories) {
this.deviceCategories = deviceCategories;
this.changedFields = changedFields.add("deviceCategories");
return this;
}
/**
* “The list of device categories with the tenant.”
*
* @param deviceCategories
* value of {@code deviceCategories} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceCategories(DeviceCategory... deviceCategories) {
return deviceCategories(Arrays.asList(deviceCategories));
}
/**
* “The list of device enrollment configurations”
*
* @param deviceEnrollmentConfigurations
* value of {@code deviceEnrollmentConfigurations} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceEnrollmentConfigurations(List deviceEnrollmentConfigurations) {
this.deviceEnrollmentConfigurations = deviceEnrollmentConfigurations;
this.changedFields = changedFields.add("deviceEnrollmentConfigurations");
return this;
}
/**
* “The list of device enrollment configurations”
*
* @param deviceEnrollmentConfigurations
* value of {@code deviceEnrollmentConfigurations} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceEnrollmentConfigurations(DeviceEnrollmentConfiguration... deviceEnrollmentConfigurations) {
return deviceEnrollmentConfigurations(Arrays.asList(deviceEnrollmentConfigurations));
}
/**
* “The list of Device Management Partners configured by the tenant.”
*
* @param deviceManagementPartners
* value of {@code deviceManagementPartners} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceManagementPartners(List deviceManagementPartners) {
this.deviceManagementPartners = deviceManagementPartners;
this.changedFields = changedFields.add("deviceManagementPartners");
return this;
}
/**
* “The list of Device Management Partners configured by the tenant.”
*
* @param deviceManagementPartners
* value of {@code deviceManagementPartners} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceManagementPartners(DeviceManagementPartner... deviceManagementPartners) {
return deviceManagementPartners(Arrays.asList(deviceManagementPartners));
}
/**
* “The list of Exchange Connectors configured by the tenant.”
*
* @param exchangeConnectors
* value of {@code exchangeConnectors} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder exchangeConnectors(List exchangeConnectors) {
this.exchangeConnectors = exchangeConnectors;
this.changedFields = changedFields.add("exchangeConnectors");
return this;
}
/**
* “The list of Exchange Connectors configured by the tenant.”
*
* @param exchangeConnectors
* value of {@code exchangeConnectors} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder exchangeConnectors(DeviceManagementExchangeConnector... exchangeConnectors) {
return exchangeConnectors(Arrays.asList(exchangeConnectors));
}
/**
* “The list of Mobile threat Defense connectors configured by the tenant.”
*
* @param mobileThreatDefenseConnectors
* value of {@code mobileThreatDefenseConnectors} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder mobileThreatDefenseConnectors(List mobileThreatDefenseConnectors) {
this.mobileThreatDefenseConnectors = mobileThreatDefenseConnectors;
this.changedFields = changedFields.add("mobileThreatDefenseConnectors");
return this;
}
/**
* “The list of Mobile threat Defense connectors configured by the tenant.”
*
* @param mobileThreatDefenseConnectors
* value of {@code mobileThreatDefenseConnectors} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder mobileThreatDefenseConnectors(MobileThreatDefenseConnector... mobileThreatDefenseConnectors) {
return mobileThreatDefenseConnectors(Arrays.asList(mobileThreatDefenseConnectors));
}
/**
* “Apple push notification certificate.”
*
* @param applePushNotificationCertificate
* value of {@code applePushNotificationCertificate} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder applePushNotificationCertificate(ApplePushNotificationCertificate applePushNotificationCertificate) {
this.applePushNotificationCertificate = applePushNotificationCertificate;
this.changedFields = changedFields.add("applePushNotificationCertificate");
return this;
}
/**
* “The list of detected apps associated with a device.”
*
* @param detectedApps
* value of {@code detectedApps} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder detectedApps(List detectedApps) {
this.detectedApps = detectedApps;
this.changedFields = changedFields.add("detectedApps");
return this;
}
/**
* “The list of detected apps associated with a device.”
*
* @param detectedApps
* value of {@code detectedApps} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder detectedApps(DetectedApp... detectedApps) {
return detectedApps(Arrays.asList(detectedApps));
}
/**
* “The list of managed devices.”
*
* @param managedDevices
* value of {@code managedDevices} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder managedDevices(List managedDevices) {
this.managedDevices = managedDevices;
this.changedFields = changedFields.add("managedDevices");
return this;
}
/**
* “The list of managed devices.”
*
* @param managedDevices
* value of {@code managedDevices} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder managedDevices(ManagedDevice... managedDevices) {
return managedDevices(Arrays.asList(managedDevices));
}
/**
* “The collection property of MobileAppTroubleshootingEvent.”
*
* @param mobileAppTroubleshootingEvents
* value of {@code mobileAppTroubleshootingEvents} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder mobileAppTroubleshootingEvents(List mobileAppTroubleshootingEvents) {
this.mobileAppTroubleshootingEvents = mobileAppTroubleshootingEvents;
this.changedFields = changedFields.add("mobileAppTroubleshootingEvents");
return this;
}
/**
* “The collection property of MobileAppTroubleshootingEvent.”
*
* @param mobileAppTroubleshootingEvents
* value of {@code mobileAppTroubleshootingEvents} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder mobileAppTroubleshootingEvents(MobileAppTroubleshootingEvent... mobileAppTroubleshootingEvents) {
return mobileAppTroubleshootingEvents(Arrays.asList(mobileAppTroubleshootingEvents));
}
/**
* “User experience analytics appHealth Application Performance”
*
* @param userExperienceAnalyticsAppHealthApplicationPerformance
* value of {@code userExperienceAnalyticsAppHealthApplicationPerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthApplicationPerformance(List userExperienceAnalyticsAppHealthApplicationPerformance) {
this.userExperienceAnalyticsAppHealthApplicationPerformance = userExperienceAnalyticsAppHealthApplicationPerformance;
this.changedFields = changedFields.add("userExperienceAnalyticsAppHealthApplicationPerformance");
return this;
}
/**
* “User experience analytics appHealth Application Performance”
*
* @param userExperienceAnalyticsAppHealthApplicationPerformance
* value of {@code userExperienceAnalyticsAppHealthApplicationPerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthApplicationPerformance(UserExperienceAnalyticsAppHealthApplicationPerformance... userExperienceAnalyticsAppHealthApplicationPerformance) {
return userExperienceAnalyticsAppHealthApplicationPerformance(Arrays.asList(userExperienceAnalyticsAppHealthApplicationPerformance));
}
/**
* “User experience analytics appHealth Application Performance by App Version
* details”
*
* @param userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails
* value of {@code userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails(List userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails) {
this.userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails = userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails;
this.changedFields = changedFields.add("userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails");
return this;
}
/**
* “User experience analytics appHealth Application Performance by App Version
* details”
*
* @param userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails
* value of {@code userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails(UserExperienceAnalyticsAppHealthAppPerformanceByAppVersionDetails... userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails) {
return userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails(Arrays.asList(userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails));
}
/**
* “User experience analytics appHealth Application Performance by App Version
* Device Id”
*
* @param userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId
* value of {@code userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId(List userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId) {
this.userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId = userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId;
this.changedFields = changedFields.add("userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId");
return this;
}
/**
* “User experience analytics appHealth Application Performance by App Version
* Device Id”
*
* @param userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId
* value of {@code userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId(UserExperienceAnalyticsAppHealthAppPerformanceByAppVersionDeviceId... userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId) {
return userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId(Arrays.asList(userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId));
}
/**
* “User experience analytics appHealth Application Performance by OS Version”
*
* @param userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion
* value of {@code userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion(List userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion) {
this.userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion = userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion;
this.changedFields = changedFields.add("userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion");
return this;
}
/**
* “User experience analytics appHealth Application Performance by OS Version”
*
* @param userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion
* value of {@code userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion(UserExperienceAnalyticsAppHealthAppPerformanceByOSVersion... userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion) {
return userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion(Arrays.asList(userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion));
}
/**
* “User experience analytics appHealth Model Performance”
*
* @param userExperienceAnalyticsAppHealthDeviceModelPerformance
* value of {@code userExperienceAnalyticsAppHealthDeviceModelPerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthDeviceModelPerformance(List userExperienceAnalyticsAppHealthDeviceModelPerformance) {
this.userExperienceAnalyticsAppHealthDeviceModelPerformance = userExperienceAnalyticsAppHealthDeviceModelPerformance;
this.changedFields = changedFields.add("userExperienceAnalyticsAppHealthDeviceModelPerformance");
return this;
}
/**
* “User experience analytics appHealth Model Performance”
*
* @param userExperienceAnalyticsAppHealthDeviceModelPerformance
* value of {@code userExperienceAnalyticsAppHealthDeviceModelPerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthDeviceModelPerformance(UserExperienceAnalyticsAppHealthDeviceModelPerformance... userExperienceAnalyticsAppHealthDeviceModelPerformance) {
return userExperienceAnalyticsAppHealthDeviceModelPerformance(Arrays.asList(userExperienceAnalyticsAppHealthDeviceModelPerformance));
}
/**
* “User experience analytics appHealth Device Performance”
*
* @param userExperienceAnalyticsAppHealthDevicePerformance
* value of {@code userExperienceAnalyticsAppHealthDevicePerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthDevicePerformance(List userExperienceAnalyticsAppHealthDevicePerformance) {
this.userExperienceAnalyticsAppHealthDevicePerformance = userExperienceAnalyticsAppHealthDevicePerformance;
this.changedFields = changedFields.add("userExperienceAnalyticsAppHealthDevicePerformance");
return this;
}
/**
* “User experience analytics appHealth Device Performance”
*
* @param userExperienceAnalyticsAppHealthDevicePerformance
* value of {@code userExperienceAnalyticsAppHealthDevicePerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthDevicePerformance(UserExperienceAnalyticsAppHealthDevicePerformance... userExperienceAnalyticsAppHealthDevicePerformance) {
return userExperienceAnalyticsAppHealthDevicePerformance(Arrays.asList(userExperienceAnalyticsAppHealthDevicePerformance));
}
/**
* “User experience analytics device performance details”
*
* @param userExperienceAnalyticsAppHealthDevicePerformanceDetails
* value of {@code userExperienceAnalyticsAppHealthDevicePerformanceDetails} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthDevicePerformanceDetails(List userExperienceAnalyticsAppHealthDevicePerformanceDetails) {
this.userExperienceAnalyticsAppHealthDevicePerformanceDetails = userExperienceAnalyticsAppHealthDevicePerformanceDetails;
this.changedFields = changedFields.add("userExperienceAnalyticsAppHealthDevicePerformanceDetails");
return this;
}
/**
* “User experience analytics device performance details”
*
* @param userExperienceAnalyticsAppHealthDevicePerformanceDetails
* value of {@code userExperienceAnalyticsAppHealthDevicePerformanceDetails} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthDevicePerformanceDetails(UserExperienceAnalyticsAppHealthDevicePerformanceDetails... userExperienceAnalyticsAppHealthDevicePerformanceDetails) {
return userExperienceAnalyticsAppHealthDevicePerformanceDetails(Arrays.asList(userExperienceAnalyticsAppHealthDevicePerformanceDetails));
}
/**
* “User experience analytics appHealth OS version Performance”
*
* @param userExperienceAnalyticsAppHealthOSVersionPerformance
* value of {@code userExperienceAnalyticsAppHealthOSVersionPerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthOSVersionPerformance(List userExperienceAnalyticsAppHealthOSVersionPerformance) {
this.userExperienceAnalyticsAppHealthOSVersionPerformance = userExperienceAnalyticsAppHealthOSVersionPerformance;
this.changedFields = changedFields.add("userExperienceAnalyticsAppHealthOSVersionPerformance");
return this;
}
/**
* “User experience analytics appHealth OS version Performance”
*
* @param userExperienceAnalyticsAppHealthOSVersionPerformance
* value of {@code userExperienceAnalyticsAppHealthOSVersionPerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthOSVersionPerformance(UserExperienceAnalyticsAppHealthOSVersionPerformance... userExperienceAnalyticsAppHealthOSVersionPerformance) {
return userExperienceAnalyticsAppHealthOSVersionPerformance(Arrays.asList(userExperienceAnalyticsAppHealthOSVersionPerformance));
}
/**
* “User experience analytics appHealth overview”
*
* @param userExperienceAnalyticsAppHealthOverview
* value of {@code userExperienceAnalyticsAppHealthOverview} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsAppHealthOverview(UserExperienceAnalyticsCategory userExperienceAnalyticsAppHealthOverview) {
this.userExperienceAnalyticsAppHealthOverview = userExperienceAnalyticsAppHealthOverview;
this.changedFields = changedFields.add("userExperienceAnalyticsAppHealthOverview");
return this;
}
/**
* “User experience analytics baselines”
*
* @param userExperienceAnalyticsBaselines
* value of {@code userExperienceAnalyticsBaselines} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsBaselines(List userExperienceAnalyticsBaselines) {
this.userExperienceAnalyticsBaselines = userExperienceAnalyticsBaselines;
this.changedFields = changedFields.add("userExperienceAnalyticsBaselines");
return this;
}
/**
* “User experience analytics baselines”
*
* @param userExperienceAnalyticsBaselines
* value of {@code userExperienceAnalyticsBaselines} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsBaselines(UserExperienceAnalyticsBaseline... userExperienceAnalyticsBaselines) {
return userExperienceAnalyticsBaselines(Arrays.asList(userExperienceAnalyticsBaselines));
}
/**
* “User experience analytics categories”
*
* @param userExperienceAnalyticsCategories
* value of {@code userExperienceAnalyticsCategories} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsCategories(List userExperienceAnalyticsCategories) {
this.userExperienceAnalyticsCategories = userExperienceAnalyticsCategories;
this.changedFields = changedFields.add("userExperienceAnalyticsCategories");
return this;
}
/**
* “User experience analytics categories”
*
* @param userExperienceAnalyticsCategories
* value of {@code userExperienceAnalyticsCategories} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsCategories(UserExperienceAnalyticsCategory... userExperienceAnalyticsCategories) {
return userExperienceAnalyticsCategories(Arrays.asList(userExperienceAnalyticsCategories));
}
/**
* “User experience analytics device performance”
*
* @param userExperienceAnalyticsDevicePerformance
* value of {@code userExperienceAnalyticsDevicePerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsDevicePerformance(List userExperienceAnalyticsDevicePerformance) {
this.userExperienceAnalyticsDevicePerformance = userExperienceAnalyticsDevicePerformance;
this.changedFields = changedFields.add("userExperienceAnalyticsDevicePerformance");
return this;
}
/**
* “User experience analytics device performance”
*
* @param userExperienceAnalyticsDevicePerformance
* value of {@code userExperienceAnalyticsDevicePerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsDevicePerformance(UserExperienceAnalyticsDevicePerformance... userExperienceAnalyticsDevicePerformance) {
return userExperienceAnalyticsDevicePerformance(Arrays.asList(userExperienceAnalyticsDevicePerformance));
}
/**
* “User experience analytics device scores”
*
* @param userExperienceAnalyticsDeviceScores
* value of {@code userExperienceAnalyticsDeviceScores} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsDeviceScores(List userExperienceAnalyticsDeviceScores) {
this.userExperienceAnalyticsDeviceScores = userExperienceAnalyticsDeviceScores;
this.changedFields = changedFields.add("userExperienceAnalyticsDeviceScores");
return this;
}
/**
* “User experience analytics device scores”
*
* @param userExperienceAnalyticsDeviceScores
* value of {@code userExperienceAnalyticsDeviceScores} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsDeviceScores(UserExperienceAnalyticsDeviceScores... userExperienceAnalyticsDeviceScores) {
return userExperienceAnalyticsDeviceScores(Arrays.asList(userExperienceAnalyticsDeviceScores));
}
/**
* “User experience analytics device Startup History”
*
* @param userExperienceAnalyticsDeviceStartupHistory
* value of {@code userExperienceAnalyticsDeviceStartupHistory} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsDeviceStartupHistory(List userExperienceAnalyticsDeviceStartupHistory) {
this.userExperienceAnalyticsDeviceStartupHistory = userExperienceAnalyticsDeviceStartupHistory;
this.changedFields = changedFields.add("userExperienceAnalyticsDeviceStartupHistory");
return this;
}
/**
* “User experience analytics device Startup History”
*
* @param userExperienceAnalyticsDeviceStartupHistory
* value of {@code userExperienceAnalyticsDeviceStartupHistory} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsDeviceStartupHistory(UserExperienceAnalyticsDeviceStartupHistory... userExperienceAnalyticsDeviceStartupHistory) {
return userExperienceAnalyticsDeviceStartupHistory(Arrays.asList(userExperienceAnalyticsDeviceStartupHistory));
}
/**
* “User experience analytics device Startup Processes”
*
* @param userExperienceAnalyticsDeviceStartupProcesses
* value of {@code userExperienceAnalyticsDeviceStartupProcesses} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsDeviceStartupProcesses(List userExperienceAnalyticsDeviceStartupProcesses) {
this.userExperienceAnalyticsDeviceStartupProcesses = userExperienceAnalyticsDeviceStartupProcesses;
this.changedFields = changedFields.add("userExperienceAnalyticsDeviceStartupProcesses");
return this;
}
/**
* “User experience analytics device Startup Processes”
*
* @param userExperienceAnalyticsDeviceStartupProcesses
* value of {@code userExperienceAnalyticsDeviceStartupProcesses} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsDeviceStartupProcesses(UserExperienceAnalyticsDeviceStartupProcess... userExperienceAnalyticsDeviceStartupProcesses) {
return userExperienceAnalyticsDeviceStartupProcesses(Arrays.asList(userExperienceAnalyticsDeviceStartupProcesses));
}
/**
* “User experience analytics device Startup Process Performance”
*
* @param userExperienceAnalyticsDeviceStartupProcessPerformance
* value of {@code userExperienceAnalyticsDeviceStartupProcessPerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsDeviceStartupProcessPerformance(List userExperienceAnalyticsDeviceStartupProcessPerformance) {
this.userExperienceAnalyticsDeviceStartupProcessPerformance = userExperienceAnalyticsDeviceStartupProcessPerformance;
this.changedFields = changedFields.add("userExperienceAnalyticsDeviceStartupProcessPerformance");
return this;
}
/**
* “User experience analytics device Startup Process Performance”
*
* @param userExperienceAnalyticsDeviceStartupProcessPerformance
* value of {@code userExperienceAnalyticsDeviceStartupProcessPerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsDeviceStartupProcessPerformance(UserExperienceAnalyticsDeviceStartupProcessPerformance... userExperienceAnalyticsDeviceStartupProcessPerformance) {
return userExperienceAnalyticsDeviceStartupProcessPerformance(Arrays.asList(userExperienceAnalyticsDeviceStartupProcessPerformance));
}
/**
* “User experience analytics metric history”
*
* @param userExperienceAnalyticsMetricHistory
* value of {@code userExperienceAnalyticsMetricHistory} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsMetricHistory(List userExperienceAnalyticsMetricHistory) {
this.userExperienceAnalyticsMetricHistory = userExperienceAnalyticsMetricHistory;
this.changedFields = changedFields.add("userExperienceAnalyticsMetricHistory");
return this;
}
/**
* “User experience analytics metric history”
*
* @param userExperienceAnalyticsMetricHistory
* value of {@code userExperienceAnalyticsMetricHistory} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsMetricHistory(UserExperienceAnalyticsMetricHistory... userExperienceAnalyticsMetricHistory) {
return userExperienceAnalyticsMetricHistory(Arrays.asList(userExperienceAnalyticsMetricHistory));
}
/**
* “User experience analytics model scores”
*
* @param userExperienceAnalyticsModelScores
* value of {@code userExperienceAnalyticsModelScores} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsModelScores(List userExperienceAnalyticsModelScores) {
this.userExperienceAnalyticsModelScores = userExperienceAnalyticsModelScores;
this.changedFields = changedFields.add("userExperienceAnalyticsModelScores");
return this;
}
/**
* “User experience analytics model scores”
*
* @param userExperienceAnalyticsModelScores
* value of {@code userExperienceAnalyticsModelScores} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsModelScores(UserExperienceAnalyticsModelScores... userExperienceAnalyticsModelScores) {
return userExperienceAnalyticsModelScores(Arrays.asList(userExperienceAnalyticsModelScores));
}
/**
* “User experience analytics overview”
*
* @param userExperienceAnalyticsOverview
* value of {@code userExperienceAnalyticsOverview} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsOverview(UserExperienceAnalyticsOverview userExperienceAnalyticsOverview) {
this.userExperienceAnalyticsOverview = userExperienceAnalyticsOverview;
this.changedFields = changedFields.add("userExperienceAnalyticsOverview");
return this;
}
/**
* “User experience analytics device Startup Score History”
*
* @param userExperienceAnalyticsScoreHistory
* value of {@code userExperienceAnalyticsScoreHistory} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsScoreHistory(List userExperienceAnalyticsScoreHistory) {
this.userExperienceAnalyticsScoreHistory = userExperienceAnalyticsScoreHistory;
this.changedFields = changedFields.add("userExperienceAnalyticsScoreHistory");
return this;
}
/**
* “User experience analytics device Startup Score History”
*
* @param userExperienceAnalyticsScoreHistory
* value of {@code userExperienceAnalyticsScoreHistory} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsScoreHistory(UserExperienceAnalyticsScoreHistory... userExperienceAnalyticsScoreHistory) {
return userExperienceAnalyticsScoreHistory(Arrays.asList(userExperienceAnalyticsScoreHistory));
}
/**
* “User experience analytics work from anywhere hardware readiness metrics.”
*
* @param userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric
* value of {@code userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric(UserExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric) {
this.userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric = userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric;
this.changedFields = changedFields.add("userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric");
return this;
}
/**
* “User experience analytics work from anywhere metrics.”
*
* @param userExperienceAnalyticsWorkFromAnywhereMetrics
* value of {@code userExperienceAnalyticsWorkFromAnywhereMetrics} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsWorkFromAnywhereMetrics(List userExperienceAnalyticsWorkFromAnywhereMetrics) {
this.userExperienceAnalyticsWorkFromAnywhereMetrics = userExperienceAnalyticsWorkFromAnywhereMetrics;
this.changedFields = changedFields.add("userExperienceAnalyticsWorkFromAnywhereMetrics");
return this;
}
/**
* “User experience analytics work from anywhere metrics.”
*
* @param userExperienceAnalyticsWorkFromAnywhereMetrics
* value of {@code userExperienceAnalyticsWorkFromAnywhereMetrics} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsWorkFromAnywhereMetrics(UserExperienceAnalyticsWorkFromAnywhereMetric... userExperienceAnalyticsWorkFromAnywhereMetrics) {
return userExperienceAnalyticsWorkFromAnywhereMetrics(Arrays.asList(userExperienceAnalyticsWorkFromAnywhereMetrics));
}
/**
* “The user experience analytics work from anywhere model performance”
*
* @param userExperienceAnalyticsWorkFromAnywhereModelPerformance
* value of {@code userExperienceAnalyticsWorkFromAnywhereModelPerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsWorkFromAnywhereModelPerformance(List userExperienceAnalyticsWorkFromAnywhereModelPerformance) {
this.userExperienceAnalyticsWorkFromAnywhereModelPerformance = userExperienceAnalyticsWorkFromAnywhereModelPerformance;
this.changedFields = changedFields.add("userExperienceAnalyticsWorkFromAnywhereModelPerformance");
return this;
}
/**
* “The user experience analytics work from anywhere model performance”
*
* @param userExperienceAnalyticsWorkFromAnywhereModelPerformance
* value of {@code userExperienceAnalyticsWorkFromAnywhereModelPerformance} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userExperienceAnalyticsWorkFromAnywhereModelPerformance(UserExperienceAnalyticsWorkFromAnywhereModelPerformance... userExperienceAnalyticsWorkFromAnywhereModelPerformance) {
return userExperienceAnalyticsWorkFromAnywhereModelPerformance(Arrays.asList(userExperienceAnalyticsWorkFromAnywhereModelPerformance));
}
/**
* “The list of affected malware in the tenant.”
*
* @param windowsMalwareInformation
* value of {@code windowsMalwareInformation} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder windowsMalwareInformation(List windowsMalwareInformation) {
this.windowsMalwareInformation = windowsMalwareInformation;
this.changedFields = changedFields.add("windowsMalwareInformation");
return this;
}
/**
* “The list of affected malware in the tenant.”
*
* @param windowsMalwareInformation
* value of {@code windowsMalwareInformation} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder windowsMalwareInformation(WindowsMalwareInformation... windowsMalwareInformation) {
return windowsMalwareInformation(Arrays.asList(windowsMalwareInformation));
}
/**
* “Collection of imported Windows autopilot devices.”
*
* @param importedWindowsAutopilotDeviceIdentities
* value of {@code importedWindowsAutopilotDeviceIdentities} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder importedWindowsAutopilotDeviceIdentities(List importedWindowsAutopilotDeviceIdentities) {
this.importedWindowsAutopilotDeviceIdentities = importedWindowsAutopilotDeviceIdentities;
this.changedFields = changedFields.add("importedWindowsAutopilotDeviceIdentities");
return this;
}
/**
* “Collection of imported Windows autopilot devices.”
*
* @param importedWindowsAutopilotDeviceIdentities
* value of {@code importedWindowsAutopilotDeviceIdentities} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder importedWindowsAutopilotDeviceIdentities(ImportedWindowsAutopilotDeviceIdentity... importedWindowsAutopilotDeviceIdentities) {
return importedWindowsAutopilotDeviceIdentities(Arrays.asList(importedWindowsAutopilotDeviceIdentities));
}
/**
* “The Windows autopilot device identities contained collection.”
*
* @param windowsAutopilotDeviceIdentities
* value of {@code windowsAutopilotDeviceIdentities} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder windowsAutopilotDeviceIdentities(List windowsAutopilotDeviceIdentities) {
this.windowsAutopilotDeviceIdentities = windowsAutopilotDeviceIdentities;
this.changedFields = changedFields.add("windowsAutopilotDeviceIdentities");
return this;
}
/**
* “The Windows autopilot device identities contained collection.”
*
* @param windowsAutopilotDeviceIdentities
* value of {@code windowsAutopilotDeviceIdentities} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder windowsAutopilotDeviceIdentities(WindowsAutopilotDeviceIdentity... windowsAutopilotDeviceIdentities) {
return windowsAutopilotDeviceIdentities(Arrays.asList(windowsAutopilotDeviceIdentities));
}
/**
* “The Notification Message Templates.”
*
* @param notificationMessageTemplates
* value of {@code notificationMessageTemplates} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder notificationMessageTemplates(List notificationMessageTemplates) {
this.notificationMessageTemplates = notificationMessageTemplates;
this.changedFields = changedFields.add("notificationMessageTemplates");
return this;
}
/**
* “The Notification Message Templates.”
*
* @param notificationMessageTemplates
* value of {@code notificationMessageTemplates} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder notificationMessageTemplates(NotificationMessageTemplate... notificationMessageTemplates) {
return notificationMessageTemplates(Arrays.asList(notificationMessageTemplates));
}
/**
* “The Resource Operations.”
*
* @param resourceOperations
* value of {@code resourceOperations} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder resourceOperations(List resourceOperations) {
this.resourceOperations = resourceOperations;
this.changedFields = changedFields.add("resourceOperations");
return this;
}
/**
* “The Resource Operations.”
*
* @param resourceOperations
* value of {@code resourceOperations} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder resourceOperations(ResourceOperation... resourceOperations) {
return resourceOperations(Arrays.asList(resourceOperations));
}
/**
* “The Role Assignments.”
*
* @param roleAssignments
* value of {@code roleAssignments} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder roleAssignments(List roleAssignments) {
this.roleAssignments = roleAssignments;
this.changedFields = changedFields.add("roleAssignments");
return this;
}
/**
* “The Role Assignments.”
*
* @param roleAssignments
* value of {@code roleAssignments} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder roleAssignments(DeviceAndAppManagementRoleAssignment... roleAssignments) {
return roleAssignments(Arrays.asList(roleAssignments));
}
/**
* “The Role Definitions.”
*
* @param roleDefinitions
* value of {@code roleDefinitions} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder roleDefinitions(List roleDefinitions) {
this.roleDefinitions = roleDefinitions;
this.changedFields = changedFields.add("roleDefinitions");
return this;
}
/**
* “The Role Definitions.”
*
* @param roleDefinitions
* value of {@code roleDefinitions} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder roleDefinitions(RoleDefinition... roleDefinitions) {
return roleDefinitions(Arrays.asList(roleDefinitions));
}
/**
* “The remote assist partners.”
*
* @param remoteAssistancePartners
* value of {@code remoteAssistancePartners} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder remoteAssistancePartners(List remoteAssistancePartners) {
this.remoteAssistancePartners = remoteAssistancePartners;
this.changedFields = changedFields.add("remoteAssistancePartners");
return this;
}
/**
* “The remote assist partners.”
*
* @param remoteAssistancePartners
* value of {@code remoteAssistancePartners} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder remoteAssistancePartners(RemoteAssistancePartner... remoteAssistancePartners) {
return remoteAssistancePartners(Arrays.asList(remoteAssistancePartners));
}
/**
* “Reports singleton”
*
* @param reports
* value of {@code reports} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder reports(DeviceManagementReports reports) {
this.reports = reports;
this.changedFields = changedFields.add("reports");
return this;
}
/**
* “The telecom expense management partners.”
*
* @param telecomExpenseManagementPartners
* value of {@code telecomExpenseManagementPartners} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder telecomExpenseManagementPartners(List telecomExpenseManagementPartners) {
this.telecomExpenseManagementPartners = telecomExpenseManagementPartners;
this.changedFields = changedFields.add("telecomExpenseManagementPartners");
return this;
}
/**
* “The telecom expense management partners.”
*
* @param telecomExpenseManagementPartners
* value of {@code telecomExpenseManagementPartners} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder telecomExpenseManagementPartners(TelecomExpenseManagementPartner... telecomExpenseManagementPartners) {
return telecomExpenseManagementPartners(Arrays.asList(telecomExpenseManagementPartners));
}
/**
* “The list of troubleshooting events for the tenant.”
*
* @param troubleshootingEvents
* value of {@code troubleshootingEvents} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder troubleshootingEvents(List troubleshootingEvents) {
this.troubleshootingEvents = troubleshootingEvents;
this.changedFields = changedFields.add("troubleshootingEvents");
return this;
}
/**
* “The list of troubleshooting events for the tenant.”
*
* @param troubleshootingEvents
* value of {@code troubleshootingEvents} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder troubleshootingEvents(DeviceManagementTroubleshootingEvent... troubleshootingEvents) {
return troubleshootingEvents(Arrays.asList(troubleshootingEvents));
}
/**
* “The windows information protection app learning summaries.”
*
* @param windowsInformationProtectionAppLearningSummaries
* value of {@code windowsInformationProtectionAppLearningSummaries} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder windowsInformationProtectionAppLearningSummaries(List windowsInformationProtectionAppLearningSummaries) {
this.windowsInformationProtectionAppLearningSummaries = windowsInformationProtectionAppLearningSummaries;
this.changedFields = changedFields.add("windowsInformationProtectionAppLearningSummaries");
return this;
}
/**
* “The windows information protection app learning summaries.”
*
* @param windowsInformationProtectionAppLearningSummaries
* value of {@code windowsInformationProtectionAppLearningSummaries} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder windowsInformationProtectionAppLearningSummaries(WindowsInformationProtectionAppLearningSummary... windowsInformationProtectionAppLearningSummaries) {
return windowsInformationProtectionAppLearningSummaries(Arrays.asList(windowsInformationProtectionAppLearningSummaries));
}
/**
* “The windows information protection network learning summaries.”
*
* @param windowsInformationProtectionNetworkLearningSummaries
* value of {@code windowsInformationProtectionNetworkLearningSummaries} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder windowsInformationProtectionNetworkLearningSummaries(List windowsInformationProtectionNetworkLearningSummaries) {
this.windowsInformationProtectionNetworkLearningSummaries = windowsInformationProtectionNetworkLearningSummaries;
this.changedFields = changedFields.add("windowsInformationProtectionNetworkLearningSummaries");
return this;
}
/**
* “The windows information protection network learning summaries.”
*
* @param windowsInformationProtectionNetworkLearningSummaries
* value of {@code windowsInformationProtectionNetworkLearningSummaries} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder windowsInformationProtectionNetworkLearningSummaries(WindowsInformationProtectionNetworkLearningSummary... windowsInformationProtectionNetworkLearningSummaries) {
return windowsInformationProtectionNetworkLearningSummaries(Arrays.asList(windowsInformationProtectionNetworkLearningSummaries));
}
public DeviceManagement build() {
DeviceManagement _x = new DeviceManagement();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.deviceManagement";
_x.id = id;
_x.intuneAccountId = intuneAccountId;
_x.settings = settings;
_x.intuneBrand = intuneBrand;
_x.deviceProtectionOverview = deviceProtectionOverview;
_x.subscriptionState = subscriptionState;
_x.userExperienceAnalyticsSettings = userExperienceAnalyticsSettings;
_x.windowsMalwareOverview = windowsMalwareOverview;
_x.termsAndConditions = termsAndConditions;
_x.auditEvents = auditEvents;
_x.deviceCompliancePolicies = deviceCompliancePolicies;
_x.deviceCompliancePolicyDeviceStateSummary = deviceCompliancePolicyDeviceStateSummary;
_x.deviceCompliancePolicySettingStateSummaries = deviceCompliancePolicySettingStateSummaries;
_x.deviceConfigurationDeviceStateSummaries = deviceConfigurationDeviceStateSummaries;
_x.deviceConfigurations = deviceConfigurations;
_x.iosUpdateStatuses = iosUpdateStatuses;
_x.complianceManagementPartners = complianceManagementPartners;
_x.conditionalAccessSettings = conditionalAccessSettings;
_x.deviceCategories = deviceCategories;
_x.deviceEnrollmentConfigurations = deviceEnrollmentConfigurations;
_x.deviceManagementPartners = deviceManagementPartners;
_x.exchangeConnectors = exchangeConnectors;
_x.mobileThreatDefenseConnectors = mobileThreatDefenseConnectors;
_x.applePushNotificationCertificate = applePushNotificationCertificate;
_x.detectedApps = detectedApps;
_x.managedDevices = managedDevices;
_x.mobileAppTroubleshootingEvents = mobileAppTroubleshootingEvents;
_x.userExperienceAnalyticsAppHealthApplicationPerformance = userExperienceAnalyticsAppHealthApplicationPerformance;
_x.userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails = userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails;
_x.userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId = userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId;
_x.userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion = userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion;
_x.userExperienceAnalyticsAppHealthDeviceModelPerformance = userExperienceAnalyticsAppHealthDeviceModelPerformance;
_x.userExperienceAnalyticsAppHealthDevicePerformance = userExperienceAnalyticsAppHealthDevicePerformance;
_x.userExperienceAnalyticsAppHealthDevicePerformanceDetails = userExperienceAnalyticsAppHealthDevicePerformanceDetails;
_x.userExperienceAnalyticsAppHealthOSVersionPerformance = userExperienceAnalyticsAppHealthOSVersionPerformance;
_x.userExperienceAnalyticsAppHealthOverview = userExperienceAnalyticsAppHealthOverview;
_x.userExperienceAnalyticsBaselines = userExperienceAnalyticsBaselines;
_x.userExperienceAnalyticsCategories = userExperienceAnalyticsCategories;
_x.userExperienceAnalyticsDevicePerformance = userExperienceAnalyticsDevicePerformance;
_x.userExperienceAnalyticsDeviceScores = userExperienceAnalyticsDeviceScores;
_x.userExperienceAnalyticsDeviceStartupHistory = userExperienceAnalyticsDeviceStartupHistory;
_x.userExperienceAnalyticsDeviceStartupProcesses = userExperienceAnalyticsDeviceStartupProcesses;
_x.userExperienceAnalyticsDeviceStartupProcessPerformance = userExperienceAnalyticsDeviceStartupProcessPerformance;
_x.userExperienceAnalyticsMetricHistory = userExperienceAnalyticsMetricHistory;
_x.userExperienceAnalyticsModelScores = userExperienceAnalyticsModelScores;
_x.userExperienceAnalyticsOverview = userExperienceAnalyticsOverview;
_x.userExperienceAnalyticsScoreHistory = userExperienceAnalyticsScoreHistory;
_x.userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric = userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric;
_x.userExperienceAnalyticsWorkFromAnywhereMetrics = userExperienceAnalyticsWorkFromAnywhereMetrics;
_x.userExperienceAnalyticsWorkFromAnywhereModelPerformance = userExperienceAnalyticsWorkFromAnywhereModelPerformance;
_x.windowsMalwareInformation = windowsMalwareInformation;
_x.importedWindowsAutopilotDeviceIdentities = importedWindowsAutopilotDeviceIdentities;
_x.windowsAutopilotDeviceIdentities = windowsAutopilotDeviceIdentities;
_x.notificationMessageTemplates = notificationMessageTemplates;
_x.resourceOperations = resourceOperations;
_x.roleAssignments = roleAssignments;
_x.roleDefinitions = roleDefinitions;
_x.remoteAssistancePartners = remoteAssistancePartners;
_x.reports = reports;
_x.telecomExpenseManagementPartners = telecomExpenseManagementPartners;
_x.troubleshootingEvents = troubleshootingEvents;
_x.windowsInformationProtectionAppLearningSummaries = windowsInformationProtectionAppLearningSummaries;
_x.windowsInformationProtectionNetworkLearningSummaries = windowsInformationProtectionNetworkLearningSummaries;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “Intune Account Id for given tenant”
*
* @return property intuneAccountId
*/
@Property(name="intuneAccountId")
@JsonIgnore
public Optional getIntuneAccountId() {
return Optional.ofNullable(intuneAccountId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code intuneAccountId}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Intune Account Id for given tenant”
*
* @param intuneAccountId
* new value of {@code intuneAccountId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code intuneAccountId} field changed
*/
public DeviceManagement withIntuneAccountId(UUID intuneAccountId) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("intuneAccountId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.intuneAccountId = intuneAccountId;
return _x;
}
/**
* “Account level settings.”
*
* @return property settings
*/
@Property(name="settings")
@JsonIgnore
public Optional getSettings() {
return Optional.ofNullable(settings);
}
/**
* Returns an immutable copy of {@code this} with just the {@code settings} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Account level settings.”
*
* @param settings
* new value of {@code settings} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code settings} field changed
*/
public DeviceManagement withSettings(DeviceManagementSettings settings) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("settings");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.settings = settings;
return _x;
}
/**
* “intuneBrand contains data which is used in customizing the appearance of the
* Company Portal applications as well as the end user web portal.”
*
* @return property intuneBrand
*/
@Property(name="intuneBrand")
@JsonIgnore
public Optional getIntuneBrand() {
return Optional.ofNullable(intuneBrand);
}
/**
* Returns an immutable copy of {@code this} with just the {@code intuneBrand}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “intuneBrand contains data which is used in customizing the appearance of the
* Company Portal applications as well as the end user web portal.”
*
* @param intuneBrand
* new value of {@code intuneBrand} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code intuneBrand} field changed
*/
public DeviceManagement withIntuneBrand(IntuneBrand intuneBrand) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("intuneBrand");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.intuneBrand = intuneBrand;
return _x;
}
/**
* “Device protection overview.”
*
* @return property deviceProtectionOverview
*/
@Property(name="deviceProtectionOverview")
@JsonIgnore
public Optional getDeviceProtectionOverview() {
return Optional.ofNullable(deviceProtectionOverview);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceProtectionOverview} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Device protection overview.”
*
* @param deviceProtectionOverview
* new value of {@code deviceProtectionOverview} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceProtectionOverview} field changed
*/
public DeviceManagement withDeviceProtectionOverview(DeviceProtectionOverview deviceProtectionOverview) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("deviceProtectionOverview");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.deviceProtectionOverview = deviceProtectionOverview;
return _x;
}
/**
* “Tenant mobile device management subscription state.”
*
* @return property subscriptionState
*/
@Property(name="subscriptionState")
@JsonIgnore
public Optional getSubscriptionState() {
return Optional.ofNullable(subscriptionState);
}
/**
* Returns an immutable copy of {@code this} with just the {@code subscriptionState
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Tenant mobile device management subscription state.”
*
* @param subscriptionState
* new value of {@code subscriptionState} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code subscriptionState} field changed
*/
public DeviceManagement withSubscriptionState(DeviceManagementSubscriptionState subscriptionState) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("subscriptionState");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.subscriptionState = subscriptionState;
return _x;
}
/**
* “User experience analytics device settings”
*
* @return property userExperienceAnalyticsSettings
*/
@Property(name="userExperienceAnalyticsSettings")
@JsonIgnore
public Optional getUserExperienceAnalyticsSettings() {
return Optional.ofNullable(userExperienceAnalyticsSettings);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsSettings} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “User experience analytics device settings”
*
* @param userExperienceAnalyticsSettings
* new value of {@code userExperienceAnalyticsSettings} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsSettings} field changed
*/
public DeviceManagement withUserExperienceAnalyticsSettings(UserExperienceAnalyticsSettings userExperienceAnalyticsSettings) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsSettings");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsSettings = userExperienceAnalyticsSettings;
return _x;
}
/**
* “Malware overview for windows devices.”
*
* @return property windowsMalwareOverview
*/
@Property(name="windowsMalwareOverview")
@JsonIgnore
public Optional getWindowsMalwareOverview() {
return Optional.ofNullable(windowsMalwareOverview);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* windowsMalwareOverview} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Malware overview for windows devices.”
*
* @param windowsMalwareOverview
* new value of {@code windowsMalwareOverview} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code windowsMalwareOverview} field changed
*/
public DeviceManagement withWindowsMalwareOverview(WindowsMalwareOverview windowsMalwareOverview) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("windowsMalwareOverview");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.windowsMalwareOverview = windowsMalwareOverview;
return _x;
}
public DeviceManagement withUnmappedField(String name, Object value) {
DeviceManagement _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “The terms and conditions associated with device management of the company.”
*
* @return navigational property termsAndConditions
*/
@NavigationProperty(name="termsAndConditions")
@JsonIgnore
public TermsAndConditionsCollectionRequest getTermsAndConditions() {
return new TermsAndConditionsCollectionRequest(
contextPath.addSegment("termsAndConditions"), Optional.ofNullable(termsAndConditions));
}
/**
* “The Audit Events”
*
* @return navigational property auditEvents
*/
@NavigationProperty(name="auditEvents")
@JsonIgnore
public AuditEventCollectionRequest getAuditEvents() {
return new AuditEventCollectionRequest(
contextPath.addSegment("auditEvents"), Optional.ofNullable(auditEvents));
}
/**
* “The device compliance policies.”
*
* @return navigational property deviceCompliancePolicies
*/
@NavigationProperty(name="deviceCompliancePolicies")
@JsonIgnore
public DeviceCompliancePolicyCollectionRequest getDeviceCompliancePolicies() {
return new DeviceCompliancePolicyCollectionRequest(
contextPath.addSegment("deviceCompliancePolicies"), Optional.ofNullable(deviceCompliancePolicies));
}
/**
* “The device compliance state summary for this account.”
*
* @return navigational property deviceCompliancePolicyDeviceStateSummary
*/
@NavigationProperty(name="deviceCompliancePolicyDeviceStateSummary")
@JsonIgnore
public DeviceCompliancePolicyDeviceStateSummaryRequest getDeviceCompliancePolicyDeviceStateSummary() {
return new DeviceCompliancePolicyDeviceStateSummaryRequest(contextPath.addSegment("deviceCompliancePolicyDeviceStateSummary"), Optional.ofNullable(deviceCompliancePolicyDeviceStateSummary));
}
/**
* “The summary states of compliance policy settings for this account.”
*
* @return navigational property deviceCompliancePolicySettingStateSummaries
*/
@NavigationProperty(name="deviceCompliancePolicySettingStateSummaries")
@JsonIgnore
public DeviceCompliancePolicySettingStateSummaryCollectionRequest getDeviceCompliancePolicySettingStateSummaries() {
return new DeviceCompliancePolicySettingStateSummaryCollectionRequest(
contextPath.addSegment("deviceCompliancePolicySettingStateSummaries"), Optional.ofNullable(deviceCompliancePolicySettingStateSummaries));
}
/**
* “The device configuration device state summary for this account.”
*
* @return navigational property deviceConfigurationDeviceStateSummaries
*/
@NavigationProperty(name="deviceConfigurationDeviceStateSummaries")
@JsonIgnore
public DeviceConfigurationDeviceStateSummaryRequest getDeviceConfigurationDeviceStateSummaries() {
return new DeviceConfigurationDeviceStateSummaryRequest(contextPath.addSegment("deviceConfigurationDeviceStateSummaries"), Optional.ofNullable(deviceConfigurationDeviceStateSummaries));
}
/**
* “The device configurations.”
*
* @return navigational property deviceConfigurations
*/
@NavigationProperty(name="deviceConfigurations")
@JsonIgnore
public DeviceConfigurationCollectionRequest getDeviceConfigurations() {
return new DeviceConfigurationCollectionRequest(
contextPath.addSegment("deviceConfigurations"), Optional.ofNullable(deviceConfigurations));
}
/**
* “The IOS software update installation statuses for this account.”
*
* @return navigational property iosUpdateStatuses
*/
@NavigationProperty(name="iosUpdateStatuses")
@JsonIgnore
public IosUpdateDeviceStatusCollectionRequest getIosUpdateStatuses() {
return new IosUpdateDeviceStatusCollectionRequest(
contextPath.addSegment("iosUpdateStatuses"), Optional.ofNullable(iosUpdateStatuses));
}
/**
* “The software update status summary.”
*
* @return navigational property softwareUpdateStatusSummary
*/
@NavigationProperty(name="softwareUpdateStatusSummary")
@JsonIgnore
public SoftwareUpdateStatusSummaryRequest getSoftwareUpdateStatusSummary() {
return new SoftwareUpdateStatusSummaryRequest(contextPath.addSegment("softwareUpdateStatusSummary"), RequestHelper.getValue(unmappedFields, "softwareUpdateStatusSummary"));
}
/**
* “The list of Compliance Management Partners configured by the tenant.”
*
* @return navigational property complianceManagementPartners
*/
@NavigationProperty(name="complianceManagementPartners")
@JsonIgnore
public ComplianceManagementPartnerCollectionRequest getComplianceManagementPartners() {
return new ComplianceManagementPartnerCollectionRequest(
contextPath.addSegment("complianceManagementPartners"), Optional.ofNullable(complianceManagementPartners));
}
/**
* “The Exchange on premises conditional access settings. On premises conditional
* access will require devices to be both enrolled and compliant for mail access”
*
* @return navigational property conditionalAccessSettings
*/
@NavigationProperty(name="conditionalAccessSettings")
@JsonIgnore
public OnPremisesConditionalAccessSettingsRequest getConditionalAccessSettings() {
return new OnPremisesConditionalAccessSettingsRequest(contextPath.addSegment("conditionalAccessSettings"), Optional.ofNullable(conditionalAccessSettings));
}
/**
* “The list of device categories with the tenant.”
*
* @return navigational property deviceCategories
*/
@NavigationProperty(name="deviceCategories")
@JsonIgnore
public DeviceCategoryCollectionRequest getDeviceCategories() {
return new DeviceCategoryCollectionRequest(
contextPath.addSegment("deviceCategories"), Optional.ofNullable(deviceCategories));
}
/**
* “The list of device enrollment configurations”
*
* @return navigational property deviceEnrollmentConfigurations
*/
@NavigationProperty(name="deviceEnrollmentConfigurations")
@JsonIgnore
public DeviceEnrollmentConfigurationCollectionRequest getDeviceEnrollmentConfigurations() {
return new DeviceEnrollmentConfigurationCollectionRequest(
contextPath.addSegment("deviceEnrollmentConfigurations"), Optional.ofNullable(deviceEnrollmentConfigurations));
}
/**
* “The list of Device Management Partners configured by the tenant.”
*
* @return navigational property deviceManagementPartners
*/
@NavigationProperty(name="deviceManagementPartners")
@JsonIgnore
public DeviceManagementPartnerCollectionRequest getDeviceManagementPartners() {
return new DeviceManagementPartnerCollectionRequest(
contextPath.addSegment("deviceManagementPartners"), Optional.ofNullable(deviceManagementPartners));
}
/**
* “The list of Exchange Connectors configured by the tenant.”
*
* @return navigational property exchangeConnectors
*/
@NavigationProperty(name="exchangeConnectors")
@JsonIgnore
public DeviceManagementExchangeConnectorCollectionRequest getExchangeConnectors() {
return new DeviceManagementExchangeConnectorCollectionRequest(
contextPath.addSegment("exchangeConnectors"), Optional.ofNullable(exchangeConnectors));
}
/**
* “The list of Mobile threat Defense connectors configured by the tenant.”
*
* @return navigational property mobileThreatDefenseConnectors
*/
@NavigationProperty(name="mobileThreatDefenseConnectors")
@JsonIgnore
public MobileThreatDefenseConnectorCollectionRequest getMobileThreatDefenseConnectors() {
return new MobileThreatDefenseConnectorCollectionRequest(
contextPath.addSegment("mobileThreatDefenseConnectors"), Optional.ofNullable(mobileThreatDefenseConnectors));
}
/**
* “Apple push notification certificate.”
*
* @return navigational property applePushNotificationCertificate
*/
@NavigationProperty(name="applePushNotificationCertificate")
@JsonIgnore
public ApplePushNotificationCertificateRequest getApplePushNotificationCertificate() {
return new ApplePushNotificationCertificateRequest(contextPath.addSegment("applePushNotificationCertificate"), Optional.ofNullable(applePushNotificationCertificate));
}
/**
* “The list of detected apps associated with a device.”
*
* @return navigational property detectedApps
*/
@NavigationProperty(name="detectedApps")
@JsonIgnore
public DetectedAppCollectionRequest getDetectedApps() {
return new DetectedAppCollectionRequest(
contextPath.addSegment("detectedApps"), Optional.ofNullable(detectedApps));
}
/**
* “Device overview”
*
* @return navigational property managedDeviceOverview
*/
@NavigationProperty(name="managedDeviceOverview")
@JsonIgnore
public ManagedDeviceOverviewRequest getManagedDeviceOverview() {
return new ManagedDeviceOverviewRequest(contextPath.addSegment("managedDeviceOverview"), RequestHelper.getValue(unmappedFields, "managedDeviceOverview"));
}
/**
* “The list of managed devices.”
*
* @return navigational property managedDevices
*/
@NavigationProperty(name="managedDevices")
@JsonIgnore
public ManagedDeviceCollectionRequest getManagedDevices() {
return new ManagedDeviceCollectionRequest(
contextPath.addSegment("managedDevices"), Optional.ofNullable(managedDevices));
}
/**
* “The collection property of MobileAppTroubleshootingEvent.”
*
* @return navigational property mobileAppTroubleshootingEvents
*/
@NavigationProperty(name="mobileAppTroubleshootingEvents")
@JsonIgnore
public MobileAppTroubleshootingEventCollectionRequest getMobileAppTroubleshootingEvents() {
return new MobileAppTroubleshootingEventCollectionRequest(
contextPath.addSegment("mobileAppTroubleshootingEvents"), Optional.ofNullable(mobileAppTroubleshootingEvents));
}
/**
* “User experience analytics appHealth Application Performance”
*
* @return navigational property userExperienceAnalyticsAppHealthApplicationPerformance
*/
@NavigationProperty(name="userExperienceAnalyticsAppHealthApplicationPerformance")
@JsonIgnore
public UserExperienceAnalyticsAppHealthApplicationPerformanceCollectionRequest getUserExperienceAnalyticsAppHealthApplicationPerformance() {
return new UserExperienceAnalyticsAppHealthApplicationPerformanceCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsAppHealthApplicationPerformance"), Optional.ofNullable(userExperienceAnalyticsAppHealthApplicationPerformance));
}
/**
* “User experience analytics appHealth Application Performance by App Version
* details”
*
* @return navigational property userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails
*/
@NavigationProperty(name="userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails")
@JsonIgnore
public UserExperienceAnalyticsAppHealthAppPerformanceByAppVersionDetailsCollectionRequest getUserExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails() {
return new UserExperienceAnalyticsAppHealthAppPerformanceByAppVersionDetailsCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails"), Optional.ofNullable(userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails));
}
/**
* “User experience analytics appHealth Application Performance by App Version
* Device Id”
*
* @return navigational property userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId
*/
@NavigationProperty(name="userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId")
@JsonIgnore
public UserExperienceAnalyticsAppHealthAppPerformanceByAppVersionDeviceIdCollectionRequest getUserExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId() {
return new UserExperienceAnalyticsAppHealthAppPerformanceByAppVersionDeviceIdCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId"), Optional.ofNullable(userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId));
}
/**
* “User experience analytics appHealth Application Performance by OS Version”
*
* @return navigational property userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion
*/
@NavigationProperty(name="userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion")
@JsonIgnore
public UserExperienceAnalyticsAppHealthAppPerformanceByOSVersionCollectionRequest getUserExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion() {
return new UserExperienceAnalyticsAppHealthAppPerformanceByOSVersionCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion"), Optional.ofNullable(userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion));
}
/**
* “User experience analytics appHealth Model Performance”
*
* @return navigational property userExperienceAnalyticsAppHealthDeviceModelPerformance
*/
@NavigationProperty(name="userExperienceAnalyticsAppHealthDeviceModelPerformance")
@JsonIgnore
public UserExperienceAnalyticsAppHealthDeviceModelPerformanceCollectionRequest getUserExperienceAnalyticsAppHealthDeviceModelPerformance() {
return new UserExperienceAnalyticsAppHealthDeviceModelPerformanceCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsAppHealthDeviceModelPerformance"), Optional.ofNullable(userExperienceAnalyticsAppHealthDeviceModelPerformance));
}
/**
* “User experience analytics appHealth Device Performance”
*
* @return navigational property userExperienceAnalyticsAppHealthDevicePerformance
*/
@NavigationProperty(name="userExperienceAnalyticsAppHealthDevicePerformance")
@JsonIgnore
public UserExperienceAnalyticsAppHealthDevicePerformanceCollectionRequest getUserExperienceAnalyticsAppHealthDevicePerformance() {
return new UserExperienceAnalyticsAppHealthDevicePerformanceCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsAppHealthDevicePerformance"), Optional.ofNullable(userExperienceAnalyticsAppHealthDevicePerformance));
}
/**
* “User experience analytics device performance details”
*
* @return navigational property userExperienceAnalyticsAppHealthDevicePerformanceDetails
*/
@NavigationProperty(name="userExperienceAnalyticsAppHealthDevicePerformanceDetails")
@JsonIgnore
public UserExperienceAnalyticsAppHealthDevicePerformanceDetailsCollectionRequest getUserExperienceAnalyticsAppHealthDevicePerformanceDetails() {
return new UserExperienceAnalyticsAppHealthDevicePerformanceDetailsCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsAppHealthDevicePerformanceDetails"), Optional.ofNullable(userExperienceAnalyticsAppHealthDevicePerformanceDetails));
}
/**
* “User experience analytics appHealth OS version Performance”
*
* @return navigational property userExperienceAnalyticsAppHealthOSVersionPerformance
*/
@NavigationProperty(name="userExperienceAnalyticsAppHealthOSVersionPerformance")
@JsonIgnore
public UserExperienceAnalyticsAppHealthOSVersionPerformanceCollectionRequest getUserExperienceAnalyticsAppHealthOSVersionPerformance() {
return new UserExperienceAnalyticsAppHealthOSVersionPerformanceCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsAppHealthOSVersionPerformance"), Optional.ofNullable(userExperienceAnalyticsAppHealthOSVersionPerformance));
}
/**
* “User experience analytics appHealth overview”
*
* @return navigational property userExperienceAnalyticsAppHealthOverview
*/
@NavigationProperty(name="userExperienceAnalyticsAppHealthOverview")
@JsonIgnore
public UserExperienceAnalyticsCategoryRequest getUserExperienceAnalyticsAppHealthOverview() {
return new UserExperienceAnalyticsCategoryRequest(contextPath.addSegment("userExperienceAnalyticsAppHealthOverview"), Optional.ofNullable(userExperienceAnalyticsAppHealthOverview));
}
/**
* “User experience analytics baselines”
*
* @return navigational property userExperienceAnalyticsBaselines
*/
@NavigationProperty(name="userExperienceAnalyticsBaselines")
@JsonIgnore
public UserExperienceAnalyticsBaselineCollectionRequest getUserExperienceAnalyticsBaselines() {
return new UserExperienceAnalyticsBaselineCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsBaselines"), Optional.ofNullable(userExperienceAnalyticsBaselines));
}
/**
* “User experience analytics categories”
*
* @return navigational property userExperienceAnalyticsCategories
*/
@NavigationProperty(name="userExperienceAnalyticsCategories")
@JsonIgnore
public UserExperienceAnalyticsCategoryCollectionRequest getUserExperienceAnalyticsCategories() {
return new UserExperienceAnalyticsCategoryCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsCategories"), Optional.ofNullable(userExperienceAnalyticsCategories));
}
/**
* “User experience analytics device performance”
*
* @return navigational property userExperienceAnalyticsDevicePerformance
*/
@NavigationProperty(name="userExperienceAnalyticsDevicePerformance")
@JsonIgnore
public UserExperienceAnalyticsDevicePerformanceCollectionRequest getUserExperienceAnalyticsDevicePerformance() {
return new UserExperienceAnalyticsDevicePerformanceCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsDevicePerformance"), Optional.ofNullable(userExperienceAnalyticsDevicePerformance));
}
/**
* “User experience analytics device scores”
*
* @return navigational property userExperienceAnalyticsDeviceScores
*/
@NavigationProperty(name="userExperienceAnalyticsDeviceScores")
@JsonIgnore
public UserExperienceAnalyticsDeviceScoresCollectionRequest getUserExperienceAnalyticsDeviceScores() {
return new UserExperienceAnalyticsDeviceScoresCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsDeviceScores"), Optional.ofNullable(userExperienceAnalyticsDeviceScores));
}
/**
* “User experience analytics device Startup History”
*
* @return navigational property userExperienceAnalyticsDeviceStartupHistory
*/
@NavigationProperty(name="userExperienceAnalyticsDeviceStartupHistory")
@JsonIgnore
public UserExperienceAnalyticsDeviceStartupHistoryCollectionRequest getUserExperienceAnalyticsDeviceStartupHistory() {
return new UserExperienceAnalyticsDeviceStartupHistoryCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsDeviceStartupHistory"), Optional.ofNullable(userExperienceAnalyticsDeviceStartupHistory));
}
/**
* “User experience analytics device Startup Processes”
*
* @return navigational property userExperienceAnalyticsDeviceStartupProcesses
*/
@NavigationProperty(name="userExperienceAnalyticsDeviceStartupProcesses")
@JsonIgnore
public UserExperienceAnalyticsDeviceStartupProcessCollectionRequest getUserExperienceAnalyticsDeviceStartupProcesses() {
return new UserExperienceAnalyticsDeviceStartupProcessCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsDeviceStartupProcesses"), Optional.ofNullable(userExperienceAnalyticsDeviceStartupProcesses));
}
/**
* “User experience analytics device Startup Process Performance”
*
* @return navigational property userExperienceAnalyticsDeviceStartupProcessPerformance
*/
@NavigationProperty(name="userExperienceAnalyticsDeviceStartupProcessPerformance")
@JsonIgnore
public UserExperienceAnalyticsDeviceStartupProcessPerformanceCollectionRequest getUserExperienceAnalyticsDeviceStartupProcessPerformance() {
return new UserExperienceAnalyticsDeviceStartupProcessPerformanceCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsDeviceStartupProcessPerformance"), Optional.ofNullable(userExperienceAnalyticsDeviceStartupProcessPerformance));
}
/**
* “User experience analytics metric history”
*
* @return navigational property userExperienceAnalyticsMetricHistory
*/
@NavigationProperty(name="userExperienceAnalyticsMetricHistory")
@JsonIgnore
public UserExperienceAnalyticsMetricHistoryCollectionRequest getUserExperienceAnalyticsMetricHistory() {
return new UserExperienceAnalyticsMetricHistoryCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsMetricHistory"), Optional.ofNullable(userExperienceAnalyticsMetricHistory));
}
/**
* “User experience analytics model scores”
*
* @return navigational property userExperienceAnalyticsModelScores
*/
@NavigationProperty(name="userExperienceAnalyticsModelScores")
@JsonIgnore
public UserExperienceAnalyticsModelScoresCollectionRequest getUserExperienceAnalyticsModelScores() {
return new UserExperienceAnalyticsModelScoresCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsModelScores"), Optional.ofNullable(userExperienceAnalyticsModelScores));
}
/**
* “User experience analytics overview”
*
* @return navigational property userExperienceAnalyticsOverview
*/
@NavigationProperty(name="userExperienceAnalyticsOverview")
@JsonIgnore
public UserExperienceAnalyticsOverviewRequest getUserExperienceAnalyticsOverview() {
return new UserExperienceAnalyticsOverviewRequest(contextPath.addSegment("userExperienceAnalyticsOverview"), Optional.ofNullable(userExperienceAnalyticsOverview));
}
/**
* “User experience analytics device Startup Score History”
*
* @return navigational property userExperienceAnalyticsScoreHistory
*/
@NavigationProperty(name="userExperienceAnalyticsScoreHistory")
@JsonIgnore
public UserExperienceAnalyticsScoreHistoryCollectionRequest getUserExperienceAnalyticsScoreHistory() {
return new UserExperienceAnalyticsScoreHistoryCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsScoreHistory"), Optional.ofNullable(userExperienceAnalyticsScoreHistory));
}
/**
* “User experience analytics work from anywhere hardware readiness metrics.”
*
* @return navigational property userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric
*/
@NavigationProperty(name="userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric")
@JsonIgnore
public UserExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetricRequest getUserExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric() {
return new UserExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetricRequest(contextPath.addSegment("userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric"), Optional.ofNullable(userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric));
}
/**
* “User experience analytics work from anywhere metrics.”
*
* @return navigational property userExperienceAnalyticsWorkFromAnywhereMetrics
*/
@NavigationProperty(name="userExperienceAnalyticsWorkFromAnywhereMetrics")
@JsonIgnore
public UserExperienceAnalyticsWorkFromAnywhereMetricCollectionRequest getUserExperienceAnalyticsWorkFromAnywhereMetrics() {
return new UserExperienceAnalyticsWorkFromAnywhereMetricCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsWorkFromAnywhereMetrics"), Optional.ofNullable(userExperienceAnalyticsWorkFromAnywhereMetrics));
}
/**
* “The user experience analytics work from anywhere model performance”
*
* @return navigational property userExperienceAnalyticsWorkFromAnywhereModelPerformance
*/
@NavigationProperty(name="userExperienceAnalyticsWorkFromAnywhereModelPerformance")
@JsonIgnore
public UserExperienceAnalyticsWorkFromAnywhereModelPerformanceCollectionRequest getUserExperienceAnalyticsWorkFromAnywhereModelPerformance() {
return new UserExperienceAnalyticsWorkFromAnywhereModelPerformanceCollectionRequest(
contextPath.addSegment("userExperienceAnalyticsWorkFromAnywhereModelPerformance"), Optional.ofNullable(userExperienceAnalyticsWorkFromAnywhereModelPerformance));
}
/**
* “The list of affected malware in the tenant.”
*
* @return navigational property windowsMalwareInformation
*/
@NavigationProperty(name="windowsMalwareInformation")
@JsonIgnore
public WindowsMalwareInformationCollectionRequest getWindowsMalwareInformation() {
return new WindowsMalwareInformationCollectionRequest(
contextPath.addSegment("windowsMalwareInformation"), Optional.ofNullable(windowsMalwareInformation));
}
/**
* “Collection of imported Windows autopilot devices.”
*
* @return navigational property importedWindowsAutopilotDeviceIdentities
*/
@NavigationProperty(name="importedWindowsAutopilotDeviceIdentities")
@JsonIgnore
public ImportedWindowsAutopilotDeviceIdentityCollectionRequest getImportedWindowsAutopilotDeviceIdentities() {
return new ImportedWindowsAutopilotDeviceIdentityCollectionRequest(
contextPath.addSegment("importedWindowsAutopilotDeviceIdentities"), Optional.ofNullable(importedWindowsAutopilotDeviceIdentities));
}
/**
* “The Windows autopilot device identities contained collection.”
*
* @return navigational property windowsAutopilotDeviceIdentities
*/
@NavigationProperty(name="windowsAutopilotDeviceIdentities")
@JsonIgnore
public WindowsAutopilotDeviceIdentityCollectionRequest getWindowsAutopilotDeviceIdentities() {
return new WindowsAutopilotDeviceIdentityCollectionRequest(
contextPath.addSegment("windowsAutopilotDeviceIdentities"), Optional.ofNullable(windowsAutopilotDeviceIdentities));
}
/**
* “The Notification Message Templates.”
*
* @return navigational property notificationMessageTemplates
*/
@NavigationProperty(name="notificationMessageTemplates")
@JsonIgnore
public NotificationMessageTemplateCollectionRequest getNotificationMessageTemplates() {
return new NotificationMessageTemplateCollectionRequest(
contextPath.addSegment("notificationMessageTemplates"), Optional.ofNullable(notificationMessageTemplates));
}
/**
* “The Resource Operations.”
*
* @return navigational property resourceOperations
*/
@NavigationProperty(name="resourceOperations")
@JsonIgnore
public ResourceOperationCollectionRequest getResourceOperations() {
return new ResourceOperationCollectionRequest(
contextPath.addSegment("resourceOperations"), Optional.ofNullable(resourceOperations));
}
/**
* “The Role Assignments.”
*
* @return navigational property roleAssignments
*/
@NavigationProperty(name="roleAssignments")
@JsonIgnore
public DeviceAndAppManagementRoleAssignmentCollectionRequest getRoleAssignments() {
return new DeviceAndAppManagementRoleAssignmentCollectionRequest(
contextPath.addSegment("roleAssignments"), Optional.ofNullable(roleAssignments));
}
/**
* “The Role Definitions.”
*
* @return navigational property roleDefinitions
*/
@NavigationProperty(name="roleDefinitions")
@JsonIgnore
public RoleDefinitionCollectionRequest getRoleDefinitions() {
return new RoleDefinitionCollectionRequest(
contextPath.addSegment("roleDefinitions"), Optional.ofNullable(roleDefinitions));
}
/**
* “The remote assist partners.”
*
* @return navigational property remoteAssistancePartners
*/
@NavigationProperty(name="remoteAssistancePartners")
@JsonIgnore
public RemoteAssistancePartnerCollectionRequest getRemoteAssistancePartners() {
return new RemoteAssistancePartnerCollectionRequest(
contextPath.addSegment("remoteAssistancePartners"), Optional.ofNullable(remoteAssistancePartners));
}
/**
* “Reports singleton”
*
* @return navigational property reports
*/
@NavigationProperty(name="reports")
@JsonIgnore
public DeviceManagementReportsRequest getReports() {
return new DeviceManagementReportsRequest(contextPath.addSegment("reports"), Optional.ofNullable(reports));
}
/**
* “The telecom expense management partners.”
*
* @return navigational property telecomExpenseManagementPartners
*/
@NavigationProperty(name="telecomExpenseManagementPartners")
@JsonIgnore
public TelecomExpenseManagementPartnerCollectionRequest getTelecomExpenseManagementPartners() {
return new TelecomExpenseManagementPartnerCollectionRequest(
contextPath.addSegment("telecomExpenseManagementPartners"), Optional.ofNullable(telecomExpenseManagementPartners));
}
/**
* “The list of troubleshooting events for the tenant.”
*
* @return navigational property troubleshootingEvents
*/
@NavigationProperty(name="troubleshootingEvents")
@JsonIgnore
public DeviceManagementTroubleshootingEventCollectionRequest getTroubleshootingEvents() {
return new DeviceManagementTroubleshootingEventCollectionRequest(
contextPath.addSegment("troubleshootingEvents"), Optional.ofNullable(troubleshootingEvents));
}
/**
* “The windows information protection app learning summaries.”
*
* @return navigational property windowsInformationProtectionAppLearningSummaries
*/
@NavigationProperty(name="windowsInformationProtectionAppLearningSummaries")
@JsonIgnore
public WindowsInformationProtectionAppLearningSummaryCollectionRequest getWindowsInformationProtectionAppLearningSummaries() {
return new WindowsInformationProtectionAppLearningSummaryCollectionRequest(
contextPath.addSegment("windowsInformationProtectionAppLearningSummaries"), Optional.ofNullable(windowsInformationProtectionAppLearningSummaries));
}
/**
* “The windows information protection network learning summaries.”
*
* @return navigational property windowsInformationProtectionNetworkLearningSummaries
*/
@NavigationProperty(name="windowsInformationProtectionNetworkLearningSummaries")
@JsonIgnore
public WindowsInformationProtectionNetworkLearningSummaryCollectionRequest getWindowsInformationProtectionNetworkLearningSummaries() {
return new WindowsInformationProtectionNetworkLearningSummaryCollectionRequest(
contextPath.addSegment("windowsInformationProtectionNetworkLearningSummaries"), Optional.ofNullable(windowsInformationProtectionNetworkLearningSummaries));
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* termsAndConditions} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The terms and conditions associated with device management of the company.”
*
* @param termsAndConditions
* new value of {@code termsAndConditions} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code termsAndConditions} field changed
*/
public DeviceManagement withTermsAndConditions(List termsAndConditions) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("termsAndConditions");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.termsAndConditions = termsAndConditions;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code auditEvents}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The Audit Events”
*
* @param auditEvents
* new value of {@code auditEvents} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code auditEvents} field changed
*/
public DeviceManagement withAuditEvents(List auditEvents) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("auditEvents");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.auditEvents = auditEvents;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceCompliancePolicies} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The device compliance policies.”
*
* @param deviceCompliancePolicies
* new value of {@code deviceCompliancePolicies} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceCompliancePolicies} field changed
*/
public DeviceManagement withDeviceCompliancePolicies(List deviceCompliancePolicies) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("deviceCompliancePolicies");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.deviceCompliancePolicies = deviceCompliancePolicies;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceCompliancePolicyDeviceStateSummary} field changed. Field description below
* . The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “The device compliance state summary for this account.”
*
* @param deviceCompliancePolicyDeviceStateSummary
* new value of {@code deviceCompliancePolicyDeviceStateSummary} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceCompliancePolicyDeviceStateSummary} field changed
*/
public DeviceManagement withDeviceCompliancePolicyDeviceStateSummary(DeviceCompliancePolicyDeviceStateSummary deviceCompliancePolicyDeviceStateSummary) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("deviceCompliancePolicyDeviceStateSummary");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.deviceCompliancePolicyDeviceStateSummary = deviceCompliancePolicyDeviceStateSummary;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceCompliancePolicySettingStateSummaries} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “The summary states of compliance policy settings for this account.”
*
* @param deviceCompliancePolicySettingStateSummaries
* new value of {@code deviceCompliancePolicySettingStateSummaries} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceCompliancePolicySettingStateSummaries} field changed
*/
public DeviceManagement withDeviceCompliancePolicySettingStateSummaries(List deviceCompliancePolicySettingStateSummaries) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("deviceCompliancePolicySettingStateSummaries");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.deviceCompliancePolicySettingStateSummaries = deviceCompliancePolicySettingStateSummaries;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceConfigurationDeviceStateSummaries} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “The device configuration device state summary for this account.”
*
* @param deviceConfigurationDeviceStateSummaries
* new value of {@code deviceConfigurationDeviceStateSummaries} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceConfigurationDeviceStateSummaries} field changed
*/
public DeviceManagement withDeviceConfigurationDeviceStateSummaries(DeviceConfigurationDeviceStateSummary deviceConfigurationDeviceStateSummaries) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("deviceConfigurationDeviceStateSummaries");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.deviceConfigurationDeviceStateSummaries = deviceConfigurationDeviceStateSummaries;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceConfigurations} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The device configurations.”
*
* @param deviceConfigurations
* new value of {@code deviceConfigurations} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceConfigurations} field changed
*/
public DeviceManagement withDeviceConfigurations(List deviceConfigurations) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("deviceConfigurations");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.deviceConfigurations = deviceConfigurations;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code iosUpdateStatuses
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The IOS software update installation statuses for this account.”
*
* @param iosUpdateStatuses
* new value of {@code iosUpdateStatuses} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code iosUpdateStatuses} field changed
*/
public DeviceManagement withIosUpdateStatuses(List iosUpdateStatuses) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("iosUpdateStatuses");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.iosUpdateStatuses = iosUpdateStatuses;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* complianceManagementPartners} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The list of Compliance Management Partners configured by the tenant.”
*
* @param complianceManagementPartners
* new value of {@code complianceManagementPartners} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code complianceManagementPartners} field changed
*/
public DeviceManagement withComplianceManagementPartners(List complianceManagementPartners) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("complianceManagementPartners");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.complianceManagementPartners = complianceManagementPartners;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* conditionalAccessSettings} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The Exchange on premises conditional access settings. On premises conditional
* access will require devices to be both enrolled and compliant for mail access”
*
* @param conditionalAccessSettings
* new value of {@code conditionalAccessSettings} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code conditionalAccessSettings} field changed
*/
public DeviceManagement withConditionalAccessSettings(OnPremisesConditionalAccessSettings conditionalAccessSettings) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("conditionalAccessSettings");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.conditionalAccessSettings = conditionalAccessSettings;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceCategories}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The list of device categories with the tenant.”
*
* @param deviceCategories
* new value of {@code deviceCategories} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceCategories} field changed
*/
public DeviceManagement withDeviceCategories(List deviceCategories) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("deviceCategories");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.deviceCategories = deviceCategories;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceEnrollmentConfigurations} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The list of device enrollment configurations”
*
* @param deviceEnrollmentConfigurations
* new value of {@code deviceEnrollmentConfigurations} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceEnrollmentConfigurations} field changed
*/
public DeviceManagement withDeviceEnrollmentConfigurations(List deviceEnrollmentConfigurations) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("deviceEnrollmentConfigurations");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.deviceEnrollmentConfigurations = deviceEnrollmentConfigurations;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* deviceManagementPartners} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The list of Device Management Partners configured by the tenant.”
*
* @param deviceManagementPartners
* new value of {@code deviceManagementPartners} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceManagementPartners} field changed
*/
public DeviceManagement withDeviceManagementPartners(List deviceManagementPartners) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("deviceManagementPartners");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.deviceManagementPartners = deviceManagementPartners;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* exchangeConnectors} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The list of Exchange Connectors configured by the tenant.”
*
* @param exchangeConnectors
* new value of {@code exchangeConnectors} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code exchangeConnectors} field changed
*/
public DeviceManagement withExchangeConnectors(List exchangeConnectors) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("exchangeConnectors");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.exchangeConnectors = exchangeConnectors;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* mobileThreatDefenseConnectors} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The list of Mobile threat Defense connectors configured by the tenant.”
*
* @param mobileThreatDefenseConnectors
* new value of {@code mobileThreatDefenseConnectors} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code mobileThreatDefenseConnectors} field changed
*/
public DeviceManagement withMobileThreatDefenseConnectors(List mobileThreatDefenseConnectors) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("mobileThreatDefenseConnectors");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.mobileThreatDefenseConnectors = mobileThreatDefenseConnectors;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* applePushNotificationCertificate} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “Apple push notification certificate.”
*
* @param applePushNotificationCertificate
* new value of {@code applePushNotificationCertificate} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code applePushNotificationCertificate} field changed
*/
public DeviceManagement withApplePushNotificationCertificate(ApplePushNotificationCertificate applePushNotificationCertificate) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("applePushNotificationCertificate");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.applePushNotificationCertificate = applePushNotificationCertificate;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code detectedApps}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The list of detected apps associated with a device.”
*
* @param detectedApps
* new value of {@code detectedApps} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code detectedApps} field changed
*/
public DeviceManagement withDetectedApps(List detectedApps) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("detectedApps");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.detectedApps = detectedApps;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code managedDevices}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The list of managed devices.”
*
* @param managedDevices
* new value of {@code managedDevices} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code managedDevices} field changed
*/
public DeviceManagement withManagedDevices(List managedDevices) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("managedDevices");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.managedDevices = managedDevices;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* mobileAppTroubleshootingEvents} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The collection property of MobileAppTroubleshootingEvent.”
*
* @param mobileAppTroubleshootingEvents
* new value of {@code mobileAppTroubleshootingEvents} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code mobileAppTroubleshootingEvents} field changed
*/
public DeviceManagement withMobileAppTroubleshootingEvents(List mobileAppTroubleshootingEvents) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("mobileAppTroubleshootingEvents");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.mobileAppTroubleshootingEvents = mobileAppTroubleshootingEvents;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsAppHealthApplicationPerformance} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “User experience analytics appHealth Application Performance”
*
* @param userExperienceAnalyticsAppHealthApplicationPerformance
* new value of {@code userExperienceAnalyticsAppHealthApplicationPerformance} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsAppHealthApplicationPerformance} field changed
*/
public DeviceManagement withUserExperienceAnalyticsAppHealthApplicationPerformance(List userExperienceAnalyticsAppHealthApplicationPerformance) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsAppHealthApplicationPerformance");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsAppHealthApplicationPerformance = userExperienceAnalyticsAppHealthApplicationPerformance;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “User experience analytics appHealth Application Performance by App Version
* details”
*
* @param userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails
* new value of {@code userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails} field changed
*/
public DeviceManagement withUserExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails(List userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails = userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “User experience analytics appHealth Application Performance by App Version
* Device Id”
*
* @param userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId
* new value of {@code userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId} field changed
*/
public DeviceManagement withUserExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId(List userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId = userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion} field changed
* . Field description below. The field name is also added to an internal map of
* changed fields in the returned object so that when {@code this.patch()} is
* called (if available)on the returned object only the changed fields are
* submitted.
*
* “User experience analytics appHealth Application Performance by OS Version”
*
* @param userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion
* new value of {@code userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion} field changed
*/
public DeviceManagement withUserExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion(List userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion = userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsAppHealthDeviceModelPerformance} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “User experience analytics appHealth Model Performance”
*
* @param userExperienceAnalyticsAppHealthDeviceModelPerformance
* new value of {@code userExperienceAnalyticsAppHealthDeviceModelPerformance} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsAppHealthDeviceModelPerformance} field changed
*/
public DeviceManagement withUserExperienceAnalyticsAppHealthDeviceModelPerformance(List userExperienceAnalyticsAppHealthDeviceModelPerformance) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsAppHealthDeviceModelPerformance");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsAppHealthDeviceModelPerformance = userExperienceAnalyticsAppHealthDeviceModelPerformance;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsAppHealthDevicePerformance} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “User experience analytics appHealth Device Performance”
*
* @param userExperienceAnalyticsAppHealthDevicePerformance
* new value of {@code userExperienceAnalyticsAppHealthDevicePerformance} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsAppHealthDevicePerformance} field changed
*/
public DeviceManagement withUserExperienceAnalyticsAppHealthDevicePerformance(List userExperienceAnalyticsAppHealthDevicePerformance) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsAppHealthDevicePerformance");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsAppHealthDevicePerformance = userExperienceAnalyticsAppHealthDevicePerformance;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsAppHealthDevicePerformanceDetails} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “User experience analytics device performance details”
*
* @param userExperienceAnalyticsAppHealthDevicePerformanceDetails
* new value of {@code userExperienceAnalyticsAppHealthDevicePerformanceDetails} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsAppHealthDevicePerformanceDetails} field changed
*/
public DeviceManagement withUserExperienceAnalyticsAppHealthDevicePerformanceDetails(List userExperienceAnalyticsAppHealthDevicePerformanceDetails) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsAppHealthDevicePerformanceDetails");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsAppHealthDevicePerformanceDetails = userExperienceAnalyticsAppHealthDevicePerformanceDetails;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsAppHealthOSVersionPerformance} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “User experience analytics appHealth OS version Performance”
*
* @param userExperienceAnalyticsAppHealthOSVersionPerformance
* new value of {@code userExperienceAnalyticsAppHealthOSVersionPerformance} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsAppHealthOSVersionPerformance} field changed
*/
public DeviceManagement withUserExperienceAnalyticsAppHealthOSVersionPerformance(List userExperienceAnalyticsAppHealthOSVersionPerformance) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsAppHealthOSVersionPerformance");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsAppHealthOSVersionPerformance = userExperienceAnalyticsAppHealthOSVersionPerformance;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsAppHealthOverview} field changed. Field description below
* . The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “User experience analytics appHealth overview”
*
* @param userExperienceAnalyticsAppHealthOverview
* new value of {@code userExperienceAnalyticsAppHealthOverview} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsAppHealthOverview} field changed
*/
public DeviceManagement withUserExperienceAnalyticsAppHealthOverview(UserExperienceAnalyticsCategory userExperienceAnalyticsAppHealthOverview) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsAppHealthOverview");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsAppHealthOverview = userExperienceAnalyticsAppHealthOverview;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsBaselines} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “User experience analytics baselines”
*
* @param userExperienceAnalyticsBaselines
* new value of {@code userExperienceAnalyticsBaselines} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsBaselines} field changed
*/
public DeviceManagement withUserExperienceAnalyticsBaselines(List userExperienceAnalyticsBaselines) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsBaselines");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsBaselines = userExperienceAnalyticsBaselines;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsCategories} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “User experience analytics categories”
*
* @param userExperienceAnalyticsCategories
* new value of {@code userExperienceAnalyticsCategories} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsCategories} field changed
*/
public DeviceManagement withUserExperienceAnalyticsCategories(List userExperienceAnalyticsCategories) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsCategories");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsCategories = userExperienceAnalyticsCategories;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsDevicePerformance} field changed. Field description below
* . The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “User experience analytics device performance”
*
* @param userExperienceAnalyticsDevicePerformance
* new value of {@code userExperienceAnalyticsDevicePerformance} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsDevicePerformance} field changed
*/
public DeviceManagement withUserExperienceAnalyticsDevicePerformance(List userExperienceAnalyticsDevicePerformance) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsDevicePerformance");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsDevicePerformance = userExperienceAnalyticsDevicePerformance;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsDeviceScores} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “User experience analytics device scores”
*
* @param userExperienceAnalyticsDeviceScores
* new value of {@code userExperienceAnalyticsDeviceScores} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsDeviceScores} field changed
*/
public DeviceManagement withUserExperienceAnalyticsDeviceScores(List userExperienceAnalyticsDeviceScores) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsDeviceScores");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsDeviceScores = userExperienceAnalyticsDeviceScores;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsDeviceStartupHistory} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “User experience analytics device Startup History”
*
* @param userExperienceAnalyticsDeviceStartupHistory
* new value of {@code userExperienceAnalyticsDeviceStartupHistory} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsDeviceStartupHistory} field changed
*/
public DeviceManagement withUserExperienceAnalyticsDeviceStartupHistory(List userExperienceAnalyticsDeviceStartupHistory) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsDeviceStartupHistory");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsDeviceStartupHistory = userExperienceAnalyticsDeviceStartupHistory;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsDeviceStartupProcesses} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “User experience analytics device Startup Processes”
*
* @param userExperienceAnalyticsDeviceStartupProcesses
* new value of {@code userExperienceAnalyticsDeviceStartupProcesses} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsDeviceStartupProcesses} field changed
*/
public DeviceManagement withUserExperienceAnalyticsDeviceStartupProcesses(List userExperienceAnalyticsDeviceStartupProcesses) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsDeviceStartupProcesses");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsDeviceStartupProcesses = userExperienceAnalyticsDeviceStartupProcesses;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsDeviceStartupProcessPerformance} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “User experience analytics device Startup Process Performance”
*
* @param userExperienceAnalyticsDeviceStartupProcessPerformance
* new value of {@code userExperienceAnalyticsDeviceStartupProcessPerformance} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsDeviceStartupProcessPerformance} field changed
*/
public DeviceManagement withUserExperienceAnalyticsDeviceStartupProcessPerformance(List userExperienceAnalyticsDeviceStartupProcessPerformance) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsDeviceStartupProcessPerformance");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsDeviceStartupProcessPerformance = userExperienceAnalyticsDeviceStartupProcessPerformance;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsMetricHistory} field changed. Field description below.
* The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “User experience analytics metric history”
*
* @param userExperienceAnalyticsMetricHistory
* new value of {@code userExperienceAnalyticsMetricHistory} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsMetricHistory} field changed
*/
public DeviceManagement withUserExperienceAnalyticsMetricHistory(List userExperienceAnalyticsMetricHistory) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsMetricHistory");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsMetricHistory = userExperienceAnalyticsMetricHistory;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsModelScores} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “User experience analytics model scores”
*
* @param userExperienceAnalyticsModelScores
* new value of {@code userExperienceAnalyticsModelScores} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsModelScores} field changed
*/
public DeviceManagement withUserExperienceAnalyticsModelScores(List userExperienceAnalyticsModelScores) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsModelScores");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsModelScores = userExperienceAnalyticsModelScores;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsOverview} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “User experience analytics overview”
*
* @param userExperienceAnalyticsOverview
* new value of {@code userExperienceAnalyticsOverview} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsOverview} field changed
*/
public DeviceManagement withUserExperienceAnalyticsOverview(UserExperienceAnalyticsOverview userExperienceAnalyticsOverview) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsOverview");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsOverview = userExperienceAnalyticsOverview;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsScoreHistory} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “User experience analytics device Startup Score History”
*
* @param userExperienceAnalyticsScoreHistory
* new value of {@code userExperienceAnalyticsScoreHistory} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsScoreHistory} field changed
*/
public DeviceManagement withUserExperienceAnalyticsScoreHistory(List userExperienceAnalyticsScoreHistory) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsScoreHistory");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsScoreHistory = userExperienceAnalyticsScoreHistory;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric} field changed.
* Field description below. The field name is also added to an internal map of
* changed fields in the returned object so that when {@code this.patch()} is
* called (if available)on the returned object only the changed fields are
* submitted.
*
* “User experience analytics work from anywhere hardware readiness metrics.”
*
* @param userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric
* new value of {@code userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric} field changed
*/
public DeviceManagement withUserExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric(UserExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric = userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsWorkFromAnywhereMetrics} field changed. Field description
* below. The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “User experience analytics work from anywhere metrics.”
*
* @param userExperienceAnalyticsWorkFromAnywhereMetrics
* new value of {@code userExperienceAnalyticsWorkFromAnywhereMetrics} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsWorkFromAnywhereMetrics} field changed
*/
public DeviceManagement withUserExperienceAnalyticsWorkFromAnywhereMetrics(List userExperienceAnalyticsWorkFromAnywhereMetrics) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsWorkFromAnywhereMetrics");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsWorkFromAnywhereMetrics = userExperienceAnalyticsWorkFromAnywhereMetrics;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* userExperienceAnalyticsWorkFromAnywhereModelPerformance} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “The user experience analytics work from anywhere model performance”
*
* @param userExperienceAnalyticsWorkFromAnywhereModelPerformance
* new value of {@code userExperienceAnalyticsWorkFromAnywhereModelPerformance} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userExperienceAnalyticsWorkFromAnywhereModelPerformance} field changed
*/
public DeviceManagement withUserExperienceAnalyticsWorkFromAnywhereModelPerformance(List userExperienceAnalyticsWorkFromAnywhereModelPerformance) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("userExperienceAnalyticsWorkFromAnywhereModelPerformance");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.userExperienceAnalyticsWorkFromAnywhereModelPerformance = userExperienceAnalyticsWorkFromAnywhereModelPerformance;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* windowsMalwareInformation} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The list of affected malware in the tenant.”
*
* @param windowsMalwareInformation
* new value of {@code windowsMalwareInformation} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code windowsMalwareInformation} field changed
*/
public DeviceManagement withWindowsMalwareInformation(List windowsMalwareInformation) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("windowsMalwareInformation");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.windowsMalwareInformation = windowsMalwareInformation;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* importedWindowsAutopilotDeviceIdentities} field changed. Field description below
* . The field name is also added to an internal map of changed fields in the
* returned object so that when {@code this.patch()} is called (if available)on the
* returned object only the changed fields are submitted.
*
* “Collection of imported Windows autopilot devices.”
*
* @param importedWindowsAutopilotDeviceIdentities
* new value of {@code importedWindowsAutopilotDeviceIdentities} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code importedWindowsAutopilotDeviceIdentities} field changed
*/
public DeviceManagement withImportedWindowsAutopilotDeviceIdentities(List importedWindowsAutopilotDeviceIdentities) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("importedWindowsAutopilotDeviceIdentities");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.importedWindowsAutopilotDeviceIdentities = importedWindowsAutopilotDeviceIdentities;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* windowsAutopilotDeviceIdentities} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The Windows autopilot device identities contained collection.”
*
* @param windowsAutopilotDeviceIdentities
* new value of {@code windowsAutopilotDeviceIdentities} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code windowsAutopilotDeviceIdentities} field changed
*/
public DeviceManagement withWindowsAutopilotDeviceIdentities(List windowsAutopilotDeviceIdentities) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("windowsAutopilotDeviceIdentities");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.windowsAutopilotDeviceIdentities = windowsAutopilotDeviceIdentities;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* notificationMessageTemplates} field changed. Field description below. The field
* name is also added to an internal map of changed fields in the returned object
* so that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The Notification Message Templates.”
*
* @param notificationMessageTemplates
* new value of {@code notificationMessageTemplates} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code notificationMessageTemplates} field changed
*/
public DeviceManagement withNotificationMessageTemplates(List notificationMessageTemplates) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("notificationMessageTemplates");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.notificationMessageTemplates = notificationMessageTemplates;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* resourceOperations} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The Resource Operations.”
*
* @param resourceOperations
* new value of {@code resourceOperations} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code resourceOperations} field changed
*/
public DeviceManagement withResourceOperations(List resourceOperations) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("resourceOperations");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.resourceOperations = resourceOperations;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code roleAssignments}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The Role Assignments.”
*
* @param roleAssignments
* new value of {@code roleAssignments} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code roleAssignments} field changed
*/
public DeviceManagement withRoleAssignments(List roleAssignments) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("roleAssignments");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.roleAssignments = roleAssignments;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code roleDefinitions}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The Role Definitions.”
*
* @param roleDefinitions
* new value of {@code roleDefinitions} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code roleDefinitions} field changed
*/
public DeviceManagement withRoleDefinitions(List roleDefinitions) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("roleDefinitions");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.roleDefinitions = roleDefinitions;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* remoteAssistancePartners} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The remote assist partners.”
*
* @param remoteAssistancePartners
* new value of {@code remoteAssistancePartners} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code remoteAssistancePartners} field changed
*/
public DeviceManagement withRemoteAssistancePartners(List remoteAssistancePartners) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("remoteAssistancePartners");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.remoteAssistancePartners = remoteAssistancePartners;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code reports} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Reports singleton”
*
* @param reports
* new value of {@code reports} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code reports} field changed
*/
public DeviceManagement withReports(DeviceManagementReports reports) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("reports");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.reports = reports;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* telecomExpenseManagementPartners} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The telecom expense management partners.”
*
* @param telecomExpenseManagementPartners
* new value of {@code telecomExpenseManagementPartners} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code telecomExpenseManagementPartners} field changed
*/
public DeviceManagement withTelecomExpenseManagementPartners(List telecomExpenseManagementPartners) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("telecomExpenseManagementPartners");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.telecomExpenseManagementPartners = telecomExpenseManagementPartners;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* troubleshootingEvents} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The list of troubleshooting events for the tenant.”
*
* @param troubleshootingEvents
* new value of {@code troubleshootingEvents} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code troubleshootingEvents} field changed
*/
public DeviceManagement withTroubleshootingEvents(List troubleshootingEvents) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("troubleshootingEvents");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.troubleshootingEvents = troubleshootingEvents;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* windowsInformationProtectionAppLearningSummaries} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “The windows information protection app learning summaries.”
*
* @param windowsInformationProtectionAppLearningSummaries
* new value of {@code windowsInformationProtectionAppLearningSummaries} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code windowsInformationProtectionAppLearningSummaries} field changed
*/
public DeviceManagement withWindowsInformationProtectionAppLearningSummaries(List windowsInformationProtectionAppLearningSummaries) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("windowsInformationProtectionAppLearningSummaries");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.windowsInformationProtectionAppLearningSummaries = windowsInformationProtectionAppLearningSummaries;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* windowsInformationProtectionNetworkLearningSummaries} field changed. Field
* description below. The field name is also added to an internal map of changed
* fields in the returned object so that when {@code this.patch()} is called (if
* available)on the returned object only the changed fields are submitted.
*
* “The windows information protection network learning summaries.”
*
* @param windowsInformationProtectionNetworkLearningSummaries
* new value of {@code windowsInformationProtectionNetworkLearningSummaries} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code windowsInformationProtectionNetworkLearningSummaries} field changed
*/
public DeviceManagement withWindowsInformationProtectionNetworkLearningSummaries(List windowsInformationProtectionNetworkLearningSummaries) {
DeviceManagement _x = _copy();
_x.changedFields = changedFields.add("windowsInformationProtectionNetworkLearningSummaries");
_x.odataType = Util.nvl(odataType, "microsoft.graph.deviceManagement");
_x.windowsInformationProtectionNetworkLearningSummaries = windowsInformationProtectionNetworkLearningSummaries;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public DeviceManagement patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
DeviceManagement _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public DeviceManagement put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
DeviceManagement _x = _copy();
_x.changedFields = null;
return _x;
}
private DeviceManagement _copy() {
DeviceManagement _x = new DeviceManagement();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.intuneAccountId = intuneAccountId;
_x.settings = settings;
_x.intuneBrand = intuneBrand;
_x.deviceProtectionOverview = deviceProtectionOverview;
_x.subscriptionState = subscriptionState;
_x.userExperienceAnalyticsSettings = userExperienceAnalyticsSettings;
_x.windowsMalwareOverview = windowsMalwareOverview;
_x.termsAndConditions = termsAndConditions;
_x.auditEvents = auditEvents;
_x.deviceCompliancePolicies = deviceCompliancePolicies;
_x.deviceCompliancePolicyDeviceStateSummary = deviceCompliancePolicyDeviceStateSummary;
_x.deviceCompliancePolicySettingStateSummaries = deviceCompliancePolicySettingStateSummaries;
_x.deviceConfigurationDeviceStateSummaries = deviceConfigurationDeviceStateSummaries;
_x.deviceConfigurations = deviceConfigurations;
_x.iosUpdateStatuses = iosUpdateStatuses;
_x.complianceManagementPartners = complianceManagementPartners;
_x.conditionalAccessSettings = conditionalAccessSettings;
_x.deviceCategories = deviceCategories;
_x.deviceEnrollmentConfigurations = deviceEnrollmentConfigurations;
_x.deviceManagementPartners = deviceManagementPartners;
_x.exchangeConnectors = exchangeConnectors;
_x.mobileThreatDefenseConnectors = mobileThreatDefenseConnectors;
_x.applePushNotificationCertificate = applePushNotificationCertificate;
_x.detectedApps = detectedApps;
_x.managedDevices = managedDevices;
_x.mobileAppTroubleshootingEvents = mobileAppTroubleshootingEvents;
_x.userExperienceAnalyticsAppHealthApplicationPerformance = userExperienceAnalyticsAppHealthApplicationPerformance;
_x.userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails = userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDetails;
_x.userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId = userExperienceAnalyticsAppHealthApplicationPerformanceByAppVersionDeviceId;
_x.userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion = userExperienceAnalyticsAppHealthApplicationPerformanceByOSVersion;
_x.userExperienceAnalyticsAppHealthDeviceModelPerformance = userExperienceAnalyticsAppHealthDeviceModelPerformance;
_x.userExperienceAnalyticsAppHealthDevicePerformance = userExperienceAnalyticsAppHealthDevicePerformance;
_x.userExperienceAnalyticsAppHealthDevicePerformanceDetails = userExperienceAnalyticsAppHealthDevicePerformanceDetails;
_x.userExperienceAnalyticsAppHealthOSVersionPerformance = userExperienceAnalyticsAppHealthOSVersionPerformance;
_x.userExperienceAnalyticsAppHealthOverview = userExperienceAnalyticsAppHealthOverview;
_x.userExperienceAnalyticsBaselines = userExperienceAnalyticsBaselines;
_x.userExperienceAnalyticsCategories = userExperienceAnalyticsCategories;
_x.userExperienceAnalyticsDevicePerformance = userExperienceAnalyticsDevicePerformance;
_x.userExperienceAnalyticsDeviceScores = userExperienceAnalyticsDeviceScores;
_x.userExperienceAnalyticsDeviceStartupHistory = userExperienceAnalyticsDeviceStartupHistory;
_x.userExperienceAnalyticsDeviceStartupProcesses = userExperienceAnalyticsDeviceStartupProcesses;
_x.userExperienceAnalyticsDeviceStartupProcessPerformance = userExperienceAnalyticsDeviceStartupProcessPerformance;
_x.userExperienceAnalyticsMetricHistory = userExperienceAnalyticsMetricHistory;
_x.userExperienceAnalyticsModelScores = userExperienceAnalyticsModelScores;
_x.userExperienceAnalyticsOverview = userExperienceAnalyticsOverview;
_x.userExperienceAnalyticsScoreHistory = userExperienceAnalyticsScoreHistory;
_x.userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric = userExperienceAnalyticsWorkFromAnywhereHardwareReadinessMetric;
_x.userExperienceAnalyticsWorkFromAnywhereMetrics = userExperienceAnalyticsWorkFromAnywhereMetrics;
_x.userExperienceAnalyticsWorkFromAnywhereModelPerformance = userExperienceAnalyticsWorkFromAnywhereModelPerformance;
_x.windowsMalwareInformation = windowsMalwareInformation;
_x.importedWindowsAutopilotDeviceIdentities = importedWindowsAutopilotDeviceIdentities;
_x.windowsAutopilotDeviceIdentities = windowsAutopilotDeviceIdentities;
_x.notificationMessageTemplates = notificationMessageTemplates;
_x.resourceOperations = resourceOperations;
_x.roleAssignments = roleAssignments;
_x.roleDefinitions = roleDefinitions;
_x.remoteAssistancePartners = remoteAssistancePartners;
_x.reports = reports;
_x.telecomExpenseManagementPartners = telecomExpenseManagementPartners;
_x.troubleshootingEvents = troubleshootingEvents;
_x.windowsInformationProtectionAppLearningSummaries = windowsInformationProtectionAppLearningSummaries;
_x.windowsInformationProtectionNetworkLearningSummaries = windowsInformationProtectionNetworkLearningSummaries;
return _x;
}
@Function(name = "verifyWindowsEnrollmentAutoDiscovery")
@JsonIgnore
public FunctionRequestReturningNonCollection verifyWindowsEnrollmentAutoDiscovery(String domainName) {
Preconditions.checkNotNull(domainName, "domainName cannot be null");
Map _parameters = ParameterMap
.put("domainName", "Edm.String", Checks.checkIsAscii(domainName))
.build();
return new FunctionRequestReturningNonCollection