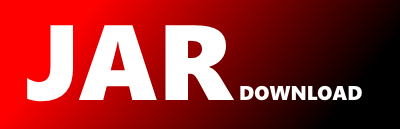
odata.msgraph.client.entity.EducationUser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph Show documentation
Show all versions of odata-client-msgraph Show documentation
Java client for use with the Microsoft Graph v1.0 endpoint
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.complex.AssignedLicense;
import odata.msgraph.client.complex.AssignedPlan;
import odata.msgraph.client.complex.EducationOnPremisesInfo;
import odata.msgraph.client.complex.EducationStudent;
import odata.msgraph.client.complex.EducationTeacher;
import odata.msgraph.client.complex.IdentitySet;
import odata.msgraph.client.complex.PasswordProfile;
import odata.msgraph.client.complex.PhysicalAddress;
import odata.msgraph.client.complex.ProvisionedPlan;
import odata.msgraph.client.complex.RelatedContact;
import odata.msgraph.client.entity.collection.request.EducationAssignmentCollectionRequest;
import odata.msgraph.client.entity.collection.request.EducationClassCollectionRequest;
import odata.msgraph.client.entity.collection.request.EducationRubricCollectionRequest;
import odata.msgraph.client.entity.collection.request.EducationSchoolCollectionRequest;
import odata.msgraph.client.entity.request.UserRequest;
import odata.msgraph.client.enums.EducationExternalSource;
import odata.msgraph.client.enums.EducationUserRole;
@JsonPropertyOrder({
"@odata.type",
"relatedContacts",
"accountEnabled",
"assignedLicenses",
"assignedPlans",
"businessPhones",
"createdBy",
"department",
"displayName",
"externalSource",
"externalSourceDetail",
"givenName",
"mail",
"mailingAddress",
"mailNickname",
"middleName",
"mobilePhone",
"officeLocation",
"onPremisesInfo",
"passwordPolicies",
"passwordProfile",
"preferredLanguage",
"primaryRole",
"provisionedPlans",
"refreshTokensValidFromDateTime",
"residenceAddress",
"showInAddressList",
"student",
"surname",
"teacher",
"usageLocation",
"userPrincipalName",
"userType",
"assignments",
"rubrics"})
@JsonInclude(Include.NON_NULL)
public class EducationUser extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.educationUser";
}
@JsonProperty("relatedContacts")
protected List relatedContacts;
@JsonProperty("relatedContacts@nextLink")
protected String relatedContactsNextLink;
@JsonProperty("accountEnabled")
protected Boolean accountEnabled;
@JsonProperty("assignedLicenses")
protected List assignedLicenses;
@JsonProperty("assignedLicenses@nextLink")
protected String assignedLicensesNextLink;
@JsonProperty("assignedPlans")
protected List assignedPlans;
@JsonProperty("assignedPlans@nextLink")
protected String assignedPlansNextLink;
@JsonProperty("businessPhones")
protected List businessPhones;
@JsonProperty("businessPhones@nextLink")
protected String businessPhonesNextLink;
@JsonProperty("createdBy")
protected IdentitySet createdBy;
@JsonProperty("department")
protected String department;
@JsonProperty("displayName")
protected String displayName;
@JsonProperty("externalSource")
protected EducationExternalSource externalSource;
@JsonProperty("externalSourceDetail")
protected String externalSourceDetail;
@JsonProperty("givenName")
protected String givenName;
@JsonProperty("mail")
protected String mail;
@JsonProperty("mailingAddress")
protected PhysicalAddress mailingAddress;
@JsonProperty("mailNickname")
protected String mailNickname;
@JsonProperty("middleName")
protected String middleName;
@JsonProperty("mobilePhone")
protected String mobilePhone;
@JsonProperty("officeLocation")
protected String officeLocation;
@JsonProperty("onPremisesInfo")
protected EducationOnPremisesInfo onPremisesInfo;
@JsonProperty("passwordPolicies")
protected String passwordPolicies;
@JsonProperty("passwordProfile")
protected PasswordProfile passwordProfile;
@JsonProperty("preferredLanguage")
protected String preferredLanguage;
@JsonProperty("primaryRole")
protected EducationUserRole primaryRole;
@JsonProperty("provisionedPlans")
protected List provisionedPlans;
@JsonProperty("provisionedPlans@nextLink")
protected String provisionedPlansNextLink;
@JsonProperty("refreshTokensValidFromDateTime")
protected OffsetDateTime refreshTokensValidFromDateTime;
@JsonProperty("residenceAddress")
protected PhysicalAddress residenceAddress;
@JsonProperty("showInAddressList")
protected Boolean showInAddressList;
@JsonProperty("student")
protected EducationStudent student;
@JsonProperty("surname")
protected String surname;
@JsonProperty("teacher")
protected EducationTeacher teacher;
@JsonProperty("usageLocation")
protected String usageLocation;
@JsonProperty("userPrincipalName")
protected String userPrincipalName;
@JsonProperty("userType")
protected String userType;
@JsonProperty("assignments")
protected List assignments;
@JsonProperty("rubrics")
protected List rubrics;
protected EducationUser() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderEducationUser() {
return new Builder();
}
public static final class Builder {
private String id;
private List relatedContacts;
private String relatedContactsNextLink;
private Boolean accountEnabled;
private List assignedLicenses;
private String assignedLicensesNextLink;
private List assignedPlans;
private String assignedPlansNextLink;
private List businessPhones;
private String businessPhonesNextLink;
private IdentitySet createdBy;
private String department;
private String displayName;
private EducationExternalSource externalSource;
private String externalSourceDetail;
private String givenName;
private String mail;
private PhysicalAddress mailingAddress;
private String mailNickname;
private String middleName;
private String mobilePhone;
private String officeLocation;
private EducationOnPremisesInfo onPremisesInfo;
private String passwordPolicies;
private PasswordProfile passwordProfile;
private String preferredLanguage;
private EducationUserRole primaryRole;
private List provisionedPlans;
private String provisionedPlansNextLink;
private OffsetDateTime refreshTokensValidFromDateTime;
private PhysicalAddress residenceAddress;
private Boolean showInAddressList;
private EducationStudent student;
private String surname;
private EducationTeacher teacher;
private String usageLocation;
private String userPrincipalName;
private String userType;
private List assignments;
private List rubrics;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder relatedContacts(List relatedContacts) {
this.relatedContacts = relatedContacts;
this.changedFields = changedFields.add("relatedContacts");
return this;
}
public Builder relatedContacts(RelatedContact... relatedContacts) {
return relatedContacts(Arrays.asList(relatedContacts));
}
public Builder relatedContactsNextLink(String relatedContactsNextLink) {
this.relatedContactsNextLink = relatedContactsNextLink;
this.changedFields = changedFields.add("relatedContacts");
return this;
}
public Builder accountEnabled(Boolean accountEnabled) {
this.accountEnabled = accountEnabled;
this.changedFields = changedFields.add("accountEnabled");
return this;
}
public Builder assignedLicenses(List assignedLicenses) {
this.assignedLicenses = assignedLicenses;
this.changedFields = changedFields.add("assignedLicenses");
return this;
}
public Builder assignedLicenses(AssignedLicense... assignedLicenses) {
return assignedLicenses(Arrays.asList(assignedLicenses));
}
public Builder assignedLicensesNextLink(String assignedLicensesNextLink) {
this.assignedLicensesNextLink = assignedLicensesNextLink;
this.changedFields = changedFields.add("assignedLicenses");
return this;
}
public Builder assignedPlans(List assignedPlans) {
this.assignedPlans = assignedPlans;
this.changedFields = changedFields.add("assignedPlans");
return this;
}
public Builder assignedPlans(AssignedPlan... assignedPlans) {
return assignedPlans(Arrays.asList(assignedPlans));
}
public Builder assignedPlansNextLink(String assignedPlansNextLink) {
this.assignedPlansNextLink = assignedPlansNextLink;
this.changedFields = changedFields.add("assignedPlans");
return this;
}
public Builder businessPhones(List businessPhones) {
this.businessPhones = businessPhones;
this.changedFields = changedFields.add("businessPhones");
return this;
}
public Builder businessPhones(String... businessPhones) {
return businessPhones(Arrays.asList(businessPhones));
}
public Builder businessPhonesNextLink(String businessPhonesNextLink) {
this.businessPhonesNextLink = businessPhonesNextLink;
this.changedFields = changedFields.add("businessPhones");
return this;
}
public Builder createdBy(IdentitySet createdBy) {
this.createdBy = createdBy;
this.changedFields = changedFields.add("createdBy");
return this;
}
public Builder department(String department) {
this.department = department;
this.changedFields = changedFields.add("department");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder externalSource(EducationExternalSource externalSource) {
this.externalSource = externalSource;
this.changedFields = changedFields.add("externalSource");
return this;
}
public Builder externalSourceDetail(String externalSourceDetail) {
this.externalSourceDetail = externalSourceDetail;
this.changedFields = changedFields.add("externalSourceDetail");
return this;
}
public Builder givenName(String givenName) {
this.givenName = givenName;
this.changedFields = changedFields.add("givenName");
return this;
}
public Builder mail(String mail) {
this.mail = mail;
this.changedFields = changedFields.add("mail");
return this;
}
public Builder mailingAddress(PhysicalAddress mailingAddress) {
this.mailingAddress = mailingAddress;
this.changedFields = changedFields.add("mailingAddress");
return this;
}
public Builder mailNickname(String mailNickname) {
this.mailNickname = mailNickname;
this.changedFields = changedFields.add("mailNickname");
return this;
}
public Builder middleName(String middleName) {
this.middleName = middleName;
this.changedFields = changedFields.add("middleName");
return this;
}
public Builder mobilePhone(String mobilePhone) {
this.mobilePhone = mobilePhone;
this.changedFields = changedFields.add("mobilePhone");
return this;
}
public Builder officeLocation(String officeLocation) {
this.officeLocation = officeLocation;
this.changedFields = changedFields.add("officeLocation");
return this;
}
public Builder onPremisesInfo(EducationOnPremisesInfo onPremisesInfo) {
this.onPremisesInfo = onPremisesInfo;
this.changedFields = changedFields.add("onPremisesInfo");
return this;
}
public Builder passwordPolicies(String passwordPolicies) {
this.passwordPolicies = passwordPolicies;
this.changedFields = changedFields.add("passwordPolicies");
return this;
}
public Builder passwordProfile(PasswordProfile passwordProfile) {
this.passwordProfile = passwordProfile;
this.changedFields = changedFields.add("passwordProfile");
return this;
}
public Builder preferredLanguage(String preferredLanguage) {
this.preferredLanguage = preferredLanguage;
this.changedFields = changedFields.add("preferredLanguage");
return this;
}
public Builder primaryRole(EducationUserRole primaryRole) {
this.primaryRole = primaryRole;
this.changedFields = changedFields.add("primaryRole");
return this;
}
public Builder provisionedPlans(List provisionedPlans) {
this.provisionedPlans = provisionedPlans;
this.changedFields = changedFields.add("provisionedPlans");
return this;
}
public Builder provisionedPlans(ProvisionedPlan... provisionedPlans) {
return provisionedPlans(Arrays.asList(provisionedPlans));
}
public Builder provisionedPlansNextLink(String provisionedPlansNextLink) {
this.provisionedPlansNextLink = provisionedPlansNextLink;
this.changedFields = changedFields.add("provisionedPlans");
return this;
}
public Builder refreshTokensValidFromDateTime(OffsetDateTime refreshTokensValidFromDateTime) {
this.refreshTokensValidFromDateTime = refreshTokensValidFromDateTime;
this.changedFields = changedFields.add("refreshTokensValidFromDateTime");
return this;
}
public Builder residenceAddress(PhysicalAddress residenceAddress) {
this.residenceAddress = residenceAddress;
this.changedFields = changedFields.add("residenceAddress");
return this;
}
public Builder showInAddressList(Boolean showInAddressList) {
this.showInAddressList = showInAddressList;
this.changedFields = changedFields.add("showInAddressList");
return this;
}
public Builder student(EducationStudent student) {
this.student = student;
this.changedFields = changedFields.add("student");
return this;
}
public Builder surname(String surname) {
this.surname = surname;
this.changedFields = changedFields.add("surname");
return this;
}
public Builder teacher(EducationTeacher teacher) {
this.teacher = teacher;
this.changedFields = changedFields.add("teacher");
return this;
}
public Builder usageLocation(String usageLocation) {
this.usageLocation = usageLocation;
this.changedFields = changedFields.add("usageLocation");
return this;
}
public Builder userPrincipalName(String userPrincipalName) {
this.userPrincipalName = userPrincipalName;
this.changedFields = changedFields.add("userPrincipalName");
return this;
}
public Builder userType(String userType) {
this.userType = userType;
this.changedFields = changedFields.add("userType");
return this;
}
public Builder assignments(List assignments) {
this.assignments = assignments;
this.changedFields = changedFields.add("assignments");
return this;
}
public Builder assignments(EducationAssignment... assignments) {
return assignments(Arrays.asList(assignments));
}
public Builder rubrics(List rubrics) {
this.rubrics = rubrics;
this.changedFields = changedFields.add("rubrics");
return this;
}
public Builder rubrics(EducationRubric... rubrics) {
return rubrics(Arrays.asList(rubrics));
}
public EducationUser build() {
EducationUser _x = new EducationUser();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.educationUser";
_x.id = id;
_x.relatedContacts = relatedContacts;
_x.relatedContactsNextLink = relatedContactsNextLink;
_x.accountEnabled = accountEnabled;
_x.assignedLicenses = assignedLicenses;
_x.assignedLicensesNextLink = assignedLicensesNextLink;
_x.assignedPlans = assignedPlans;
_x.assignedPlansNextLink = assignedPlansNextLink;
_x.businessPhones = businessPhones;
_x.businessPhonesNextLink = businessPhonesNextLink;
_x.createdBy = createdBy;
_x.department = department;
_x.displayName = displayName;
_x.externalSource = externalSource;
_x.externalSourceDetail = externalSourceDetail;
_x.givenName = givenName;
_x.mail = mail;
_x.mailingAddress = mailingAddress;
_x.mailNickname = mailNickname;
_x.middleName = middleName;
_x.mobilePhone = mobilePhone;
_x.officeLocation = officeLocation;
_x.onPremisesInfo = onPremisesInfo;
_x.passwordPolicies = passwordPolicies;
_x.passwordProfile = passwordProfile;
_x.preferredLanguage = preferredLanguage;
_x.primaryRole = primaryRole;
_x.provisionedPlans = provisionedPlans;
_x.provisionedPlansNextLink = provisionedPlansNextLink;
_x.refreshTokensValidFromDateTime = refreshTokensValidFromDateTime;
_x.residenceAddress = residenceAddress;
_x.showInAddressList = showInAddressList;
_x.student = student;
_x.surname = surname;
_x.teacher = teacher;
_x.usageLocation = usageLocation;
_x.userPrincipalName = userPrincipalName;
_x.userType = userType;
_x.assignments = assignments;
_x.rubrics = rubrics;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
@Property(name="relatedContacts")
@JsonIgnore
public CollectionPage getRelatedContacts() {
return new CollectionPage(contextPath, RelatedContact.class, this.relatedContacts, Optional.ofNullable(relatedContactsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public EducationUser withRelatedContacts(List relatedContacts) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("relatedContacts");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.relatedContacts = relatedContacts;
return _x;
}
@Property(name="relatedContacts")
@JsonIgnore
public CollectionPage getRelatedContacts(HttpRequestOptions options) {
return new CollectionPage(contextPath, RelatedContact.class, this.relatedContacts, Optional.ofNullable(relatedContactsNextLink), Collections.emptyList(), options);
}
@Property(name="accountEnabled")
@JsonIgnore
public Optional getAccountEnabled() {
return Optional.ofNullable(accountEnabled);
}
public EducationUser withAccountEnabled(Boolean accountEnabled) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("accountEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.accountEnabled = accountEnabled;
return _x;
}
@Property(name="assignedLicenses")
@JsonIgnore
public CollectionPage getAssignedLicenses() {
return new CollectionPage(contextPath, AssignedLicense.class, this.assignedLicenses, Optional.ofNullable(assignedLicensesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public EducationUser withAssignedLicenses(List assignedLicenses) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("assignedLicenses");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.assignedLicenses = assignedLicenses;
return _x;
}
@Property(name="assignedLicenses")
@JsonIgnore
public CollectionPage getAssignedLicenses(HttpRequestOptions options) {
return new CollectionPage(contextPath, AssignedLicense.class, this.assignedLicenses, Optional.ofNullable(assignedLicensesNextLink), Collections.emptyList(), options);
}
@Property(name="assignedPlans")
@JsonIgnore
public CollectionPage getAssignedPlans() {
return new CollectionPage(contextPath, AssignedPlan.class, this.assignedPlans, Optional.ofNullable(assignedPlansNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public EducationUser withAssignedPlans(List assignedPlans) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("assignedPlans");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.assignedPlans = assignedPlans;
return _x;
}
@Property(name="assignedPlans")
@JsonIgnore
public CollectionPage getAssignedPlans(HttpRequestOptions options) {
return new CollectionPage(contextPath, AssignedPlan.class, this.assignedPlans, Optional.ofNullable(assignedPlansNextLink), Collections.emptyList(), options);
}
@Property(name="businessPhones")
@JsonIgnore
public CollectionPage getBusinessPhones() {
return new CollectionPage(contextPath, String.class, this.businessPhones, Optional.ofNullable(businessPhonesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public EducationUser withBusinessPhones(List businessPhones) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("businessPhones");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.businessPhones = businessPhones;
return _x;
}
@Property(name="businessPhones")
@JsonIgnore
public CollectionPage getBusinessPhones(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.businessPhones, Optional.ofNullable(businessPhonesNextLink), Collections.emptyList(), options);
}
@Property(name="createdBy")
@JsonIgnore
public Optional getCreatedBy() {
return Optional.ofNullable(createdBy);
}
public EducationUser withCreatedBy(IdentitySet createdBy) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("createdBy");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.createdBy = createdBy;
return _x;
}
@Property(name="department")
@JsonIgnore
public Optional getDepartment() {
return Optional.ofNullable(department);
}
public EducationUser withDepartment(String department) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("department");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.department = department;
return _x;
}
@Property(name="displayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
public EducationUser withDisplayName(String displayName) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("displayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.displayName = displayName;
return _x;
}
@Property(name="externalSource")
@JsonIgnore
public Optional getExternalSource() {
return Optional.ofNullable(externalSource);
}
public EducationUser withExternalSource(EducationExternalSource externalSource) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("externalSource");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.externalSource = externalSource;
return _x;
}
@Property(name="externalSourceDetail")
@JsonIgnore
public Optional getExternalSourceDetail() {
return Optional.ofNullable(externalSourceDetail);
}
public EducationUser withExternalSourceDetail(String externalSourceDetail) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("externalSourceDetail");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.externalSourceDetail = externalSourceDetail;
return _x;
}
@Property(name="givenName")
@JsonIgnore
public Optional getGivenName() {
return Optional.ofNullable(givenName);
}
public EducationUser withGivenName(String givenName) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("givenName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.givenName = givenName;
return _x;
}
@Property(name="mail")
@JsonIgnore
public Optional getMail() {
return Optional.ofNullable(mail);
}
public EducationUser withMail(String mail) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("mail");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.mail = mail;
return _x;
}
@Property(name="mailingAddress")
@JsonIgnore
public Optional getMailingAddress() {
return Optional.ofNullable(mailingAddress);
}
public EducationUser withMailingAddress(PhysicalAddress mailingAddress) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("mailingAddress");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.mailingAddress = mailingAddress;
return _x;
}
@Property(name="mailNickname")
@JsonIgnore
public Optional getMailNickname() {
return Optional.ofNullable(mailNickname);
}
public EducationUser withMailNickname(String mailNickname) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("mailNickname");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.mailNickname = mailNickname;
return _x;
}
@Property(name="middleName")
@JsonIgnore
public Optional getMiddleName() {
return Optional.ofNullable(middleName);
}
public EducationUser withMiddleName(String middleName) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("middleName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.middleName = middleName;
return _x;
}
@Property(name="mobilePhone")
@JsonIgnore
public Optional getMobilePhone() {
return Optional.ofNullable(mobilePhone);
}
public EducationUser withMobilePhone(String mobilePhone) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("mobilePhone");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.mobilePhone = mobilePhone;
return _x;
}
@Property(name="officeLocation")
@JsonIgnore
public Optional getOfficeLocation() {
return Optional.ofNullable(officeLocation);
}
public EducationUser withOfficeLocation(String officeLocation) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("officeLocation");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.officeLocation = officeLocation;
return _x;
}
@Property(name="onPremisesInfo")
@JsonIgnore
public Optional getOnPremisesInfo() {
return Optional.ofNullable(onPremisesInfo);
}
public EducationUser withOnPremisesInfo(EducationOnPremisesInfo onPremisesInfo) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("onPremisesInfo");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.onPremisesInfo = onPremisesInfo;
return _x;
}
@Property(name="passwordPolicies")
@JsonIgnore
public Optional getPasswordPolicies() {
return Optional.ofNullable(passwordPolicies);
}
public EducationUser withPasswordPolicies(String passwordPolicies) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("passwordPolicies");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.passwordPolicies = passwordPolicies;
return _x;
}
@Property(name="passwordProfile")
@JsonIgnore
public Optional getPasswordProfile() {
return Optional.ofNullable(passwordProfile);
}
public EducationUser withPasswordProfile(PasswordProfile passwordProfile) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("passwordProfile");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.passwordProfile = passwordProfile;
return _x;
}
@Property(name="preferredLanguage")
@JsonIgnore
public Optional getPreferredLanguage() {
return Optional.ofNullable(preferredLanguage);
}
public EducationUser withPreferredLanguage(String preferredLanguage) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("preferredLanguage");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.preferredLanguage = preferredLanguage;
return _x;
}
@Property(name="primaryRole")
@JsonIgnore
public Optional getPrimaryRole() {
return Optional.ofNullable(primaryRole);
}
public EducationUser withPrimaryRole(EducationUserRole primaryRole) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("primaryRole");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.primaryRole = primaryRole;
return _x;
}
@Property(name="provisionedPlans")
@JsonIgnore
public CollectionPage getProvisionedPlans() {
return new CollectionPage(contextPath, ProvisionedPlan.class, this.provisionedPlans, Optional.ofNullable(provisionedPlansNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public EducationUser withProvisionedPlans(List provisionedPlans) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("provisionedPlans");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.provisionedPlans = provisionedPlans;
return _x;
}
@Property(name="provisionedPlans")
@JsonIgnore
public CollectionPage getProvisionedPlans(HttpRequestOptions options) {
return new CollectionPage(contextPath, ProvisionedPlan.class, this.provisionedPlans, Optional.ofNullable(provisionedPlansNextLink), Collections.emptyList(), options);
}
@Property(name="refreshTokensValidFromDateTime")
@JsonIgnore
public Optional getRefreshTokensValidFromDateTime() {
return Optional.ofNullable(refreshTokensValidFromDateTime);
}
public EducationUser withRefreshTokensValidFromDateTime(OffsetDateTime refreshTokensValidFromDateTime) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("refreshTokensValidFromDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.refreshTokensValidFromDateTime = refreshTokensValidFromDateTime;
return _x;
}
@Property(name="residenceAddress")
@JsonIgnore
public Optional getResidenceAddress() {
return Optional.ofNullable(residenceAddress);
}
public EducationUser withResidenceAddress(PhysicalAddress residenceAddress) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("residenceAddress");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.residenceAddress = residenceAddress;
return _x;
}
@Property(name="showInAddressList")
@JsonIgnore
public Optional getShowInAddressList() {
return Optional.ofNullable(showInAddressList);
}
public EducationUser withShowInAddressList(Boolean showInAddressList) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("showInAddressList");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.showInAddressList = showInAddressList;
return _x;
}
@Property(name="student")
@JsonIgnore
public Optional getStudent() {
return Optional.ofNullable(student);
}
public EducationUser withStudent(EducationStudent student) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("student");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.student = student;
return _x;
}
@Property(name="surname")
@JsonIgnore
public Optional getSurname() {
return Optional.ofNullable(surname);
}
public EducationUser withSurname(String surname) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("surname");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.surname = surname;
return _x;
}
@Property(name="teacher")
@JsonIgnore
public Optional getTeacher() {
return Optional.ofNullable(teacher);
}
public EducationUser withTeacher(EducationTeacher teacher) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("teacher");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.teacher = teacher;
return _x;
}
@Property(name="usageLocation")
@JsonIgnore
public Optional getUsageLocation() {
return Optional.ofNullable(usageLocation);
}
public EducationUser withUsageLocation(String usageLocation) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("usageLocation");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.usageLocation = usageLocation;
return _x;
}
@Property(name="userPrincipalName")
@JsonIgnore
public Optional getUserPrincipalName() {
return Optional.ofNullable(userPrincipalName);
}
public EducationUser withUserPrincipalName(String userPrincipalName) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("userPrincipalName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.userPrincipalName = userPrincipalName;
return _x;
}
@Property(name="userType")
@JsonIgnore
public Optional getUserType() {
return Optional.ofNullable(userType);
}
public EducationUser withUserType(String userType) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("userType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.userType = userType;
return _x;
}
public EducationUser withUnmappedField(String name, Object value) {
EducationUser _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="assignments")
@JsonIgnore
public EducationAssignmentCollectionRequest getAssignments() {
return new EducationAssignmentCollectionRequest(
contextPath.addSegment("assignments"), Optional.ofNullable(assignments));
}
@NavigationProperty(name="rubrics")
@JsonIgnore
public EducationRubricCollectionRequest getRubrics() {
return new EducationRubricCollectionRequest(
contextPath.addSegment("rubrics"), Optional.ofNullable(rubrics));
}
@NavigationProperty(name="classes")
@JsonIgnore
public EducationClassCollectionRequest getClasses() {
return new EducationClassCollectionRequest(
contextPath.addSegment("classes"), RequestHelper.getValue(unmappedFields, "classes"));
}
@NavigationProperty(name="schools")
@JsonIgnore
public EducationSchoolCollectionRequest getSchools() {
return new EducationSchoolCollectionRequest(
contextPath.addSegment("schools"), RequestHelper.getValue(unmappedFields, "schools"));
}
@NavigationProperty(name="taughtClasses")
@JsonIgnore
public EducationClassCollectionRequest getTaughtClasses() {
return new EducationClassCollectionRequest(
contextPath.addSegment("taughtClasses"), RequestHelper.getValue(unmappedFields, "taughtClasses"));
}
@NavigationProperty(name="user")
@JsonIgnore
public UserRequest getUser() {
return new UserRequest(contextPath.addSegment("user"), RequestHelper.getValue(unmappedFields, "user"));
}
public EducationUser withAssignments(List assignments) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("assignments");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.assignments = assignments;
return _x;
}
public EducationUser withRubrics(List rubrics) {
EducationUser _x = _copy();
_x.changedFields = changedFields.add("rubrics");
_x.odataType = Util.nvl(odataType, "microsoft.graph.educationUser");
_x.rubrics = rubrics;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public EducationUser patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
EducationUser _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public EducationUser put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
EducationUser _x = _copy();
_x.changedFields = null;
return _x;
}
private EducationUser _copy() {
EducationUser _x = new EducationUser();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.relatedContacts = relatedContacts;
_x.accountEnabled = accountEnabled;
_x.assignedLicenses = assignedLicenses;
_x.assignedPlans = assignedPlans;
_x.businessPhones = businessPhones;
_x.createdBy = createdBy;
_x.department = department;
_x.displayName = displayName;
_x.externalSource = externalSource;
_x.externalSourceDetail = externalSourceDetail;
_x.givenName = givenName;
_x.mail = mail;
_x.mailingAddress = mailingAddress;
_x.mailNickname = mailNickname;
_x.middleName = middleName;
_x.mobilePhone = mobilePhone;
_x.officeLocation = officeLocation;
_x.onPremisesInfo = onPremisesInfo;
_x.passwordPolicies = passwordPolicies;
_x.passwordProfile = passwordProfile;
_x.preferredLanguage = preferredLanguage;
_x.primaryRole = primaryRole;
_x.provisionedPlans = provisionedPlans;
_x.refreshTokensValidFromDateTime = refreshTokensValidFromDateTime;
_x.residenceAddress = residenceAddress;
_x.showInAddressList = showInAddressList;
_x.student = student;
_x.surname = surname;
_x.teacher = teacher;
_x.usageLocation = usageLocation;
_x.userPrincipalName = userPrincipalName;
_x.userType = userType;
_x.assignments = assignments;
_x.rubrics = rubrics;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("EducationUser[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("relatedContacts=");
b.append(this.relatedContacts);
b.append(", ");
b.append("accountEnabled=");
b.append(this.accountEnabled);
b.append(", ");
b.append("assignedLicenses=");
b.append(this.assignedLicenses);
b.append(", ");
b.append("assignedPlans=");
b.append(this.assignedPlans);
b.append(", ");
b.append("businessPhones=");
b.append(this.businessPhones);
b.append(", ");
b.append("createdBy=");
b.append(this.createdBy);
b.append(", ");
b.append("department=");
b.append(this.department);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("externalSource=");
b.append(this.externalSource);
b.append(", ");
b.append("externalSourceDetail=");
b.append(this.externalSourceDetail);
b.append(", ");
b.append("givenName=");
b.append(this.givenName);
b.append(", ");
b.append("mail=");
b.append(this.mail);
b.append(", ");
b.append("mailingAddress=");
b.append(this.mailingAddress);
b.append(", ");
b.append("mailNickname=");
b.append(this.mailNickname);
b.append(", ");
b.append("middleName=");
b.append(this.middleName);
b.append(", ");
b.append("mobilePhone=");
b.append(this.mobilePhone);
b.append(", ");
b.append("officeLocation=");
b.append(this.officeLocation);
b.append(", ");
b.append("onPremisesInfo=");
b.append(this.onPremisesInfo);
b.append(", ");
b.append("passwordPolicies=");
b.append(this.passwordPolicies);
b.append(", ");
b.append("passwordProfile=");
b.append(this.passwordProfile);
b.append(", ");
b.append("preferredLanguage=");
b.append(this.preferredLanguage);
b.append(", ");
b.append("primaryRole=");
b.append(this.primaryRole);
b.append(", ");
b.append("provisionedPlans=");
b.append(this.provisionedPlans);
b.append(", ");
b.append("refreshTokensValidFromDateTime=");
b.append(this.refreshTokensValidFromDateTime);
b.append(", ");
b.append("residenceAddress=");
b.append(this.residenceAddress);
b.append(", ");
b.append("showInAddressList=");
b.append(this.showInAddressList);
b.append(", ");
b.append("student=");
b.append(this.student);
b.append(", ");
b.append("surname=");
b.append(this.surname);
b.append(", ");
b.append("teacher=");
b.append(this.teacher);
b.append(", ");
b.append("usageLocation=");
b.append(this.usageLocation);
b.append(", ");
b.append("userPrincipalName=");
b.append(this.userPrincipalName);
b.append(", ");
b.append("userType=");
b.append(this.userType);
b.append(", ");
b.append("assignments=");
b.append(this.assignments);
b.append(", ");
b.append("rubrics=");
b.append(this.rubrics);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy