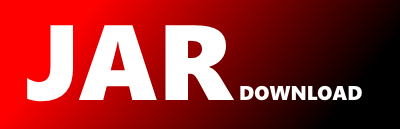
odata.msgraph.client.entity.EnrollmentTroubleshootingEvent Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Optional;
import odata.msgraph.client.enums.DeviceEnrollmentFailureReason;
import odata.msgraph.client.enums.DeviceEnrollmentType;
/**
* “Event representing an enrollment failure.”
*/@JsonPropertyOrder({
"@odata.type",
"deviceId",
"enrollmentType",
"failureCategory",
"failureReason",
"managedDeviceIdentifier",
"operatingSystem",
"osVersion",
"userId"})
@JsonInclude(Include.NON_NULL)
public class EnrollmentTroubleshootingEvent extends DeviceManagementTroubleshootingEvent implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.enrollmentTroubleshootingEvent";
}
@JsonProperty("deviceId")
protected String deviceId;
@JsonProperty("enrollmentType")
protected DeviceEnrollmentType enrollmentType;
@JsonProperty("failureCategory")
protected DeviceEnrollmentFailureReason failureCategory;
@JsonProperty("failureReason")
protected String failureReason;
@JsonProperty("managedDeviceIdentifier")
protected String managedDeviceIdentifier;
@JsonProperty("operatingSystem")
protected String operatingSystem;
@JsonProperty("osVersion")
protected String osVersion;
@JsonProperty("userId")
protected String userId;
protected EnrollmentTroubleshootingEvent() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderEnrollmentTroubleshootingEvent() {
return new Builder();
}
public static final class Builder {
private String id;
private String correlationId;
private OffsetDateTime eventDateTime;
private String deviceId;
private DeviceEnrollmentType enrollmentType;
private DeviceEnrollmentFailureReason failureCategory;
private String failureReason;
private String managedDeviceIdentifier;
private String operatingSystem;
private String osVersion;
private String userId;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder correlationId(String correlationId) {
this.correlationId = correlationId;
this.changedFields = changedFields.add("correlationId");
return this;
}
public Builder eventDateTime(OffsetDateTime eventDateTime) {
this.eventDateTime = eventDateTime;
this.changedFields = changedFields.add("eventDateTime");
return this;
}
/**
* “Azure AD device identifier.”
*
* @param deviceId
* value of {@code deviceId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder deviceId(String deviceId) {
this.deviceId = deviceId;
this.changedFields = changedFields.add("deviceId");
return this;
}
/**
* “Type of the enrollment.”
*
* @param enrollmentType
* value of {@code enrollmentType} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder enrollmentType(DeviceEnrollmentType enrollmentType) {
this.enrollmentType = enrollmentType;
this.changedFields = changedFields.add("enrollmentType");
return this;
}
/**
* “Highlevel failure category.”
*
* @param failureCategory
* value of {@code failureCategory} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder failureCategory(DeviceEnrollmentFailureReason failureCategory) {
this.failureCategory = failureCategory;
this.changedFields = changedFields.add("failureCategory");
return this;
}
/**
* “Detailed failure reason.”
*
* @param failureReason
* value of {@code failureReason} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder failureReason(String failureReason) {
this.failureReason = failureReason;
this.changedFields = changedFields.add("failureReason");
return this;
}
/**
* “Device identifier created or collected by Intune.”
*
* @param managedDeviceIdentifier
* value of {@code managedDeviceIdentifier} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder managedDeviceIdentifier(String managedDeviceIdentifier) {
this.managedDeviceIdentifier = managedDeviceIdentifier;
this.changedFields = changedFields.add("managedDeviceIdentifier");
return this;
}
/**
* “Operating System.”
*
* @param operatingSystem
* value of {@code operatingSystem} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder operatingSystem(String operatingSystem) {
this.operatingSystem = operatingSystem;
this.changedFields = changedFields.add("operatingSystem");
return this;
}
/**
* “OS Version.”
*
* @param osVersion
* value of {@code osVersion} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder osVersion(String osVersion) {
this.osVersion = osVersion;
this.changedFields = changedFields.add("osVersion");
return this;
}
/**
* “Identifier for the user that tried to enroll the device.”
*
* @param userId
* value of {@code userId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder userId(String userId) {
this.userId = userId;
this.changedFields = changedFields.add("userId");
return this;
}
public EnrollmentTroubleshootingEvent build() {
EnrollmentTroubleshootingEvent _x = new EnrollmentTroubleshootingEvent();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.enrollmentTroubleshootingEvent";
_x.id = id;
_x.correlationId = correlationId;
_x.eventDateTime = eventDateTime;
_x.deviceId = deviceId;
_x.enrollmentType = enrollmentType;
_x.failureCategory = failureCategory;
_x.failureReason = failureReason;
_x.managedDeviceIdentifier = managedDeviceIdentifier;
_x.operatingSystem = operatingSystem;
_x.osVersion = osVersion;
_x.userId = userId;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “Azure AD device identifier.”
*
* @return property deviceId
*/
@Property(name="deviceId")
@JsonIgnore
public Optional getDeviceId() {
return Optional.ofNullable(deviceId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceId} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Azure AD device identifier.”
*
* @param deviceId
* new value of {@code deviceId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceId} field changed
*/
public EnrollmentTroubleshootingEvent withDeviceId(String deviceId) {
EnrollmentTroubleshootingEvent _x = _copy();
_x.changedFields = changedFields.add("deviceId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.enrollmentTroubleshootingEvent");
_x.deviceId = deviceId;
return _x;
}
/**
* “Type of the enrollment.”
*
* @return property enrollmentType
*/
@Property(name="enrollmentType")
@JsonIgnore
public Optional getEnrollmentType() {
return Optional.ofNullable(enrollmentType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code enrollmentType}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Type of the enrollment.”
*
* @param enrollmentType
* new value of {@code enrollmentType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code enrollmentType} field changed
*/
public EnrollmentTroubleshootingEvent withEnrollmentType(DeviceEnrollmentType enrollmentType) {
EnrollmentTroubleshootingEvent _x = _copy();
_x.changedFields = changedFields.add("enrollmentType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.enrollmentTroubleshootingEvent");
_x.enrollmentType = enrollmentType;
return _x;
}
/**
* “Highlevel failure category.”
*
* @return property failureCategory
*/
@Property(name="failureCategory")
@JsonIgnore
public Optional getFailureCategory() {
return Optional.ofNullable(failureCategory);
}
/**
* Returns an immutable copy of {@code this} with just the {@code failureCategory}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Highlevel failure category.”
*
* @param failureCategory
* new value of {@code failureCategory} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code failureCategory} field changed
*/
public EnrollmentTroubleshootingEvent withFailureCategory(DeviceEnrollmentFailureReason failureCategory) {
EnrollmentTroubleshootingEvent _x = _copy();
_x.changedFields = changedFields.add("failureCategory");
_x.odataType = Util.nvl(odataType, "microsoft.graph.enrollmentTroubleshootingEvent");
_x.failureCategory = failureCategory;
return _x;
}
/**
* “Detailed failure reason.”
*
* @return property failureReason
*/
@Property(name="failureReason")
@JsonIgnore
public Optional getFailureReason() {
return Optional.ofNullable(failureReason);
}
/**
* Returns an immutable copy of {@code this} with just the {@code failureReason}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Detailed failure reason.”
*
* @param failureReason
* new value of {@code failureReason} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code failureReason} field changed
*/
public EnrollmentTroubleshootingEvent withFailureReason(String failureReason) {
EnrollmentTroubleshootingEvent _x = _copy();
_x.changedFields = changedFields.add("failureReason");
_x.odataType = Util.nvl(odataType, "microsoft.graph.enrollmentTroubleshootingEvent");
_x.failureReason = failureReason;
return _x;
}
/**
* “Device identifier created or collected by Intune.”
*
* @return property managedDeviceIdentifier
*/
@Property(name="managedDeviceIdentifier")
@JsonIgnore
public Optional getManagedDeviceIdentifier() {
return Optional.ofNullable(managedDeviceIdentifier);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* managedDeviceIdentifier} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “Device identifier created or collected by Intune.”
*
* @param managedDeviceIdentifier
* new value of {@code managedDeviceIdentifier} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code managedDeviceIdentifier} field changed
*/
public EnrollmentTroubleshootingEvent withManagedDeviceIdentifier(String managedDeviceIdentifier) {
EnrollmentTroubleshootingEvent _x = _copy();
_x.changedFields = changedFields.add("managedDeviceIdentifier");
_x.odataType = Util.nvl(odataType, "microsoft.graph.enrollmentTroubleshootingEvent");
_x.managedDeviceIdentifier = managedDeviceIdentifier;
return _x;
}
/**
* “Operating System.”
*
* @return property operatingSystem
*/
@Property(name="operatingSystem")
@JsonIgnore
public Optional getOperatingSystem() {
return Optional.ofNullable(operatingSystem);
}
/**
* Returns an immutable copy of {@code this} with just the {@code operatingSystem}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Operating System.”
*
* @param operatingSystem
* new value of {@code operatingSystem} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code operatingSystem} field changed
*/
public EnrollmentTroubleshootingEvent withOperatingSystem(String operatingSystem) {
EnrollmentTroubleshootingEvent _x = _copy();
_x.changedFields = changedFields.add("operatingSystem");
_x.odataType = Util.nvl(odataType, "microsoft.graph.enrollmentTroubleshootingEvent");
_x.operatingSystem = operatingSystem;
return _x;
}
/**
* “OS Version.”
*
* @return property osVersion
*/
@Property(name="osVersion")
@JsonIgnore
public Optional getOsVersion() {
return Optional.ofNullable(osVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code osVersion} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “OS Version.”
*
* @param osVersion
* new value of {@code osVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code osVersion} field changed
*/
public EnrollmentTroubleshootingEvent withOsVersion(String osVersion) {
EnrollmentTroubleshootingEvent _x = _copy();
_x.changedFields = changedFields.add("osVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.enrollmentTroubleshootingEvent");
_x.osVersion = osVersion;
return _x;
}
/**
* “Identifier for the user that tried to enroll the device.”
*
* @return property userId
*/
@Property(name="userId")
@JsonIgnore
public Optional getUserId() {
return Optional.ofNullable(userId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userId} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Identifier for the user that tried to enroll the device.”
*
* @param userId
* new value of {@code userId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userId} field changed
*/
public EnrollmentTroubleshootingEvent withUserId(String userId) {
EnrollmentTroubleshootingEvent _x = _copy();
_x.changedFields = changedFields.add("userId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.enrollmentTroubleshootingEvent");
_x.userId = userId;
return _x;
}
public EnrollmentTroubleshootingEvent withUnmappedField(String name, Object value) {
EnrollmentTroubleshootingEvent _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public EnrollmentTroubleshootingEvent patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
EnrollmentTroubleshootingEvent _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public EnrollmentTroubleshootingEvent put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
EnrollmentTroubleshootingEvent _x = _copy();
_x.changedFields = null;
return _x;
}
private EnrollmentTroubleshootingEvent _copy() {
EnrollmentTroubleshootingEvent _x = new EnrollmentTroubleshootingEvent();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.correlationId = correlationId;
_x.eventDateTime = eventDateTime;
_x.deviceId = deviceId;
_x.enrollmentType = enrollmentType;
_x.failureCategory = failureCategory;
_x.failureReason = failureReason;
_x.managedDeviceIdentifier = managedDeviceIdentifier;
_x.operatingSystem = operatingSystem;
_x.osVersion = osVersion;
_x.userId = userId;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("EnrollmentTroubleshootingEvent[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("correlationId=");
b.append(this.correlationId);
b.append(", ");
b.append("eventDateTime=");
b.append(this.eventDateTime);
b.append(", ");
b.append("deviceId=");
b.append(this.deviceId);
b.append(", ");
b.append("enrollmentType=");
b.append(this.enrollmentType);
b.append(", ");
b.append("failureCategory=");
b.append(this.failureCategory);
b.append(", ");
b.append("failureReason=");
b.append(this.failureReason);
b.append(", ");
b.append("managedDeviceIdentifier=");
b.append(this.managedDeviceIdentifier);
b.append(", ");
b.append("operatingSystem=");
b.append(this.operatingSystem);
b.append(", ");
b.append("osVersion=");
b.append(this.osVersion);
b.append(", ");
b.append("userId=");
b.append(this.userId);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}