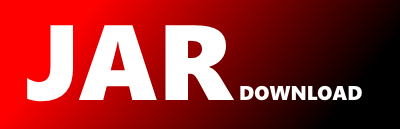
odata.msgraph.client.entity.ListItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-client-msgraph Show documentation
Show all versions of odata-client-msgraph Show documentation
Java client for use with the Microsoft Graph v1.0 endpoint
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.guavamini.Preconditions;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPageNonEntityRequest;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Function;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.Checks;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import odata.msgraph.client.complex.ContentTypeInfo;
import odata.msgraph.client.complex.IdentitySet;
import odata.msgraph.client.complex.ItemReference;
import odata.msgraph.client.complex.SharepointIds;
import odata.msgraph.client.entity.collection.request.DocumentSetVersionCollectionRequest;
import odata.msgraph.client.entity.collection.request.ListItemVersionCollectionRequest;
import odata.msgraph.client.entity.request.DriveItemRequest;
import odata.msgraph.client.entity.request.FieldValueSetRequest;
import odata.msgraph.client.entity.request.ItemAnalyticsRequest;
@JsonPropertyOrder({
"@odata.type",
"contentType",
"sharepointIds",
"documentSetVersions",
"driveItem",
"fields",
"versions"})
@JsonInclude(Include.NON_NULL)
public class ListItem extends BaseItem implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.listItem";
}
@JsonProperty("contentType")
protected ContentTypeInfo contentType;
@JsonProperty("sharepointIds")
protected SharepointIds sharepointIds;
@JsonProperty("documentSetVersions")
protected List documentSetVersions;
@JsonProperty("driveItem")
protected DriveItem driveItem;
@JsonProperty("fields")
protected FieldValueSet fields;
@JsonProperty("versions")
protected List versions;
protected ListItem() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderListItem() {
return new Builder();
}
public static final class Builder {
private String id;
private IdentitySet createdBy;
private OffsetDateTime createdDateTime;
private String description;
private String eTag;
private IdentitySet lastModifiedBy;
private OffsetDateTime lastModifiedDateTime;
private String name;
private ItemReference parentReference;
private String webUrl;
private ContentTypeInfo contentType;
private SharepointIds sharepointIds;
private List documentSetVersions;
private DriveItem driveItem;
private FieldValueSet fields;
private List versions;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdBy(IdentitySet createdBy) {
this.createdBy = createdBy;
this.changedFields = changedFields.add("createdBy");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder eTag(String eTag) {
this.eTag = eTag;
this.changedFields = changedFields.add("eTag");
return this;
}
public Builder lastModifiedBy(IdentitySet lastModifiedBy) {
this.lastModifiedBy = lastModifiedBy;
this.changedFields = changedFields.add("lastModifiedBy");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder name(String name) {
this.name = name;
this.changedFields = changedFields.add("name");
return this;
}
public Builder parentReference(ItemReference parentReference) {
this.parentReference = parentReference;
this.changedFields = changedFields.add("parentReference");
return this;
}
public Builder webUrl(String webUrl) {
this.webUrl = webUrl;
this.changedFields = changedFields.add("webUrl");
return this;
}
public Builder contentType(ContentTypeInfo contentType) {
this.contentType = contentType;
this.changedFields = changedFields.add("contentType");
return this;
}
public Builder sharepointIds(SharepointIds sharepointIds) {
this.sharepointIds = sharepointIds;
this.changedFields = changedFields.add("sharepointIds");
return this;
}
public Builder documentSetVersions(List documentSetVersions) {
this.documentSetVersions = documentSetVersions;
this.changedFields = changedFields.add("documentSetVersions");
return this;
}
public Builder documentSetVersions(DocumentSetVersion... documentSetVersions) {
return documentSetVersions(Arrays.asList(documentSetVersions));
}
public Builder driveItem(DriveItem driveItem) {
this.driveItem = driveItem;
this.changedFields = changedFields.add("driveItem");
return this;
}
public Builder fields(FieldValueSet fields) {
this.fields = fields;
this.changedFields = changedFields.add("fields");
return this;
}
public Builder versions(List versions) {
this.versions = versions;
this.changedFields = changedFields.add("versions");
return this;
}
public Builder versions(ListItemVersion... versions) {
return versions(Arrays.asList(versions));
}
public ListItem build() {
ListItem _x = new ListItem();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.listItem";
_x.id = id;
_x.createdBy = createdBy;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.eTag = eTag;
_x.lastModifiedBy = lastModifiedBy;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.name = name;
_x.parentReference = parentReference;
_x.webUrl = webUrl;
_x.contentType = contentType;
_x.sharepointIds = sharepointIds;
_x.documentSetVersions = documentSetVersions;
_x.driveItem = driveItem;
_x.fields = fields;
_x.versions = versions;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
@Property(name="contentType")
@JsonIgnore
public Optional getContentType() {
return Optional.ofNullable(contentType);
}
public ListItem withContentType(ContentTypeInfo contentType) {
ListItem _x = _copy();
_x.changedFields = changedFields.add("contentType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.listItem");
_x.contentType = contentType;
return _x;
}
@Property(name="sharepointIds")
@JsonIgnore
public Optional getSharepointIds() {
return Optional.ofNullable(sharepointIds);
}
public ListItem withSharepointIds(SharepointIds sharepointIds) {
ListItem _x = _copy();
_x.changedFields = changedFields.add("sharepointIds");
_x.odataType = Util.nvl(odataType, "microsoft.graph.listItem");
_x.sharepointIds = sharepointIds;
return _x;
}
public ListItem withUnmappedField(String name, Object value) {
ListItem _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="analytics")
@JsonIgnore
public ItemAnalyticsRequest getAnalytics() {
return new ItemAnalyticsRequest(contextPath.addSegment("analytics"), RequestHelper.getValue(unmappedFields, "analytics"));
}
@NavigationProperty(name="documentSetVersions")
@JsonIgnore
public DocumentSetVersionCollectionRequest getDocumentSetVersions() {
return new DocumentSetVersionCollectionRequest(
contextPath.addSegment("documentSetVersions"), Optional.ofNullable(documentSetVersions));
}
@NavigationProperty(name="driveItem")
@JsonIgnore
public DriveItemRequest getDriveItem() {
return new DriveItemRequest(contextPath.addSegment("driveItem"), Optional.ofNullable(driveItem));
}
@NavigationProperty(name="fields")
@JsonIgnore
public FieldValueSetRequest getFields() {
return new FieldValueSetRequest(contextPath.addSegment("fields"), Optional.ofNullable(fields));
}
@NavigationProperty(name="versions")
@JsonIgnore
public ListItemVersionCollectionRequest getVersions() {
return new ListItemVersionCollectionRequest(
contextPath.addSegment("versions"), Optional.ofNullable(versions));
}
public ListItem withDocumentSetVersions(List documentSetVersions) {
ListItem _x = _copy();
_x.changedFields = changedFields.add("documentSetVersions");
_x.odataType = Util.nvl(odataType, "microsoft.graph.listItem");
_x.documentSetVersions = documentSetVersions;
return _x;
}
public ListItem withDriveItem(DriveItem driveItem) {
ListItem _x = _copy();
_x.changedFields = changedFields.add("driveItem");
_x.odataType = Util.nvl(odataType, "microsoft.graph.listItem");
_x.driveItem = driveItem;
return _x;
}
public ListItem withFields(FieldValueSet fields) {
ListItem _x = _copy();
_x.changedFields = changedFields.add("fields");
_x.odataType = Util.nvl(odataType, "microsoft.graph.listItem");
_x.fields = fields;
return _x;
}
public ListItem withVersions(List versions) {
ListItem _x = _copy();
_x.changedFields = changedFields.add("versions");
_x.odataType = Util.nvl(odataType, "microsoft.graph.listItem");
_x.versions = versions;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ListItem patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
ListItem _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ListItem put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
ListItem _x = _copy();
_x.changedFields = null;
return _x;
}
private ListItem _copy() {
ListItem _x = new ListItem();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdBy = createdBy;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.eTag = eTag;
_x.lastModifiedBy = lastModifiedBy;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.name = name;
_x.parentReference = parentReference;
_x.webUrl = webUrl;
_x.contentType = contentType;
_x.sharepointIds = sharepointIds;
_x.documentSetVersions = documentSetVersions;
_x.driveItem = driveItem;
_x.fields = fields;
_x.versions = versions;
return _x;
}
@Function(name = "getActivitiesByInterval")
@JsonIgnore
public CollectionPageNonEntityRequest getActivitiesByInterval() {
Map _parameters = ParameterMap.empty();
return CollectionPageNonEntityRequest.forFunction(this.contextPath.addActionOrFunctionSegment("microsoft.graph.getActivitiesByInterval"), ItemActivityStat.class, _parameters);
}
@Function(name = "getActivitiesByInterval")
@JsonIgnore
public CollectionPageNonEntityRequest getActivitiesByInterval_Function(String startDateTime, String endDateTime, String interval) {
Preconditions.checkNotNull(startDateTime, "startDateTime cannot be null");
Preconditions.checkNotNull(endDateTime, "endDateTime cannot be null");
Preconditions.checkNotNull(interval, "interval cannot be null");
Map _parameters = ParameterMap
.put("startDateTime", "Edm.String", Checks.checkIsAscii(startDateTime))
.put("endDateTime", "Edm.String", Checks.checkIsAscii(endDateTime))
.put("interval", "Edm.String", Checks.checkIsAscii(interval))
.build();
return CollectionPageNonEntityRequest.forFunction(this.contextPath.addActionOrFunctionSegment("microsoft.graph.getActivitiesByInterval"), ItemActivityStat.class, _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ListItem[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdBy=");
b.append(this.createdBy);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("eTag=");
b.append(this.eTag);
b.append(", ");
b.append("lastModifiedBy=");
b.append(this.lastModifiedBy);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("name=");
b.append(this.name);
b.append(", ");
b.append("parentReference=");
b.append(this.parentReference);
b.append(", ");
b.append("webUrl=");
b.append(this.webUrl);
b.append(", ");
b.append("contentType=");
b.append(this.contentType);
b.append(", ");
b.append("sharepointIds=");
b.append(this.sharepointIds);
b.append(", ");
b.append("documentSetVersions=");
b.append(this.documentSetVersions);
b.append(", ");
b.append("driveItem=");
b.append(this.driveItem);
b.append(", ");
b.append("fields=");
b.append(this.fields);
b.append(", ");
b.append("versions=");
b.append(this.versions);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy