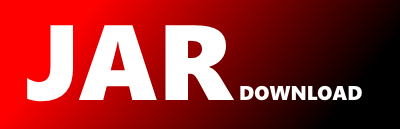
odata.msgraph.client.entity.ManagedAndroidLobApp Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.complex.AndroidMinimumOperatingSystem;
import odata.msgraph.client.complex.MimeContent;
import odata.msgraph.client.enums.ManagedAppAvailability;
import odata.msgraph.client.enums.MobileAppPublishingState;
/**
* “Contains properties and inherited properties for Managed Android Line Of
* Business apps.”
*/@JsonPropertyOrder({
"@odata.type",
"minimumSupportedOperatingSystem",
"packageId",
"versionCode",
"versionName"})
@JsonInclude(Include.NON_NULL)
public class ManagedAndroidLobApp extends ManagedMobileLobApp implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.managedAndroidLobApp";
}
@JsonProperty("minimumSupportedOperatingSystem")
protected AndroidMinimumOperatingSystem minimumSupportedOperatingSystem;
@JsonProperty("packageId")
protected String packageId;
@JsonProperty("versionCode")
protected String versionCode;
@JsonProperty("versionName")
protected String versionName;
protected ManagedAndroidLobApp() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderManagedAndroidLobApp() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime createdDateTime;
private String description;
private String developer;
private String displayName;
private String informationUrl;
private Boolean isFeatured;
private MimeContent largeIcon;
private OffsetDateTime lastModifiedDateTime;
private String notes;
private String owner;
private String privacyInformationUrl;
private String publisher;
private MobileAppPublishingState publishingState;
private List assignments;
private ManagedAppAvailability appAvailability;
private String version;
private String committedContentVersion;
private String fileName;
private Long size;
private List contentVersions;
private AndroidMinimumOperatingSystem minimumSupportedOperatingSystem;
private String packageId;
private String versionCode;
private String versionName;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder createdDateTime(OffsetDateTime createdDateTime) {
this.createdDateTime = createdDateTime;
this.changedFields = changedFields.add("createdDateTime");
return this;
}
public Builder description(String description) {
this.description = description;
this.changedFields = changedFields.add("description");
return this;
}
public Builder developer(String developer) {
this.developer = developer;
this.changedFields = changedFields.add("developer");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder informationUrl(String informationUrl) {
this.informationUrl = informationUrl;
this.changedFields = changedFields.add("informationUrl");
return this;
}
public Builder isFeatured(Boolean isFeatured) {
this.isFeatured = isFeatured;
this.changedFields = changedFields.add("isFeatured");
return this;
}
public Builder largeIcon(MimeContent largeIcon) {
this.largeIcon = largeIcon;
this.changedFields = changedFields.add("largeIcon");
return this;
}
public Builder lastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
this.lastModifiedDateTime = lastModifiedDateTime;
this.changedFields = changedFields.add("lastModifiedDateTime");
return this;
}
public Builder notes(String notes) {
this.notes = notes;
this.changedFields = changedFields.add("notes");
return this;
}
public Builder owner(String owner) {
this.owner = owner;
this.changedFields = changedFields.add("owner");
return this;
}
public Builder privacyInformationUrl(String privacyInformationUrl) {
this.privacyInformationUrl = privacyInformationUrl;
this.changedFields = changedFields.add("privacyInformationUrl");
return this;
}
public Builder publisher(String publisher) {
this.publisher = publisher;
this.changedFields = changedFields.add("publisher");
return this;
}
public Builder publishingState(MobileAppPublishingState publishingState) {
this.publishingState = publishingState;
this.changedFields = changedFields.add("publishingState");
return this;
}
public Builder assignments(List assignments) {
this.assignments = assignments;
this.changedFields = changedFields.add("assignments");
return this;
}
public Builder assignments(MobileAppAssignment... assignments) {
return assignments(Arrays.asList(assignments));
}
public Builder appAvailability(ManagedAppAvailability appAvailability) {
this.appAvailability = appAvailability;
this.changedFields = changedFields.add("appAvailability");
return this;
}
public Builder version(String version) {
this.version = version;
this.changedFields = changedFields.add("version");
return this;
}
public Builder committedContentVersion(String committedContentVersion) {
this.committedContentVersion = committedContentVersion;
this.changedFields = changedFields.add("committedContentVersion");
return this;
}
public Builder fileName(String fileName) {
this.fileName = fileName;
this.changedFields = changedFields.add("fileName");
return this;
}
public Builder size(Long size) {
this.size = size;
this.changedFields = changedFields.add("size");
return this;
}
public Builder contentVersions(List contentVersions) {
this.contentVersions = contentVersions;
this.changedFields = changedFields.add("contentVersions");
return this;
}
public Builder contentVersions(MobileAppContent... contentVersions) {
return contentVersions(Arrays.asList(contentVersions));
}
/**
* “The value for the minimum applicable operating system.”
*
* @param minimumSupportedOperatingSystem
* value of {@code minimumSupportedOperatingSystem} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder minimumSupportedOperatingSystem(AndroidMinimumOperatingSystem minimumSupportedOperatingSystem) {
this.minimumSupportedOperatingSystem = minimumSupportedOperatingSystem;
this.changedFields = changedFields.add("minimumSupportedOperatingSystem");
return this;
}
/**
* “The package identifier.”
*
* @param packageId
* value of {@code packageId} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder packageId(String packageId) {
this.packageId = packageId;
this.changedFields = changedFields.add("packageId");
return this;
}
/**
* “The version code of managed Android Line of Business (LoB) app.”
*
* @param versionCode
* value of {@code versionCode} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder versionCode(String versionCode) {
this.versionCode = versionCode;
this.changedFields = changedFields.add("versionCode");
return this;
}
/**
* “The version name of managed Android Line of Business (LoB) app.”
*
* @param versionName
* value of {@code versionName} property (as defined in service metadata)
* @return {@code this} (for method chaining)
*/
public Builder versionName(String versionName) {
this.versionName = versionName;
this.changedFields = changedFields.add("versionName");
return this;
}
public ManagedAndroidLobApp build() {
ManagedAndroidLobApp _x = new ManagedAndroidLobApp();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.managedAndroidLobApp";
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.developer = developer;
_x.displayName = displayName;
_x.informationUrl = informationUrl;
_x.isFeatured = isFeatured;
_x.largeIcon = largeIcon;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.notes = notes;
_x.owner = owner;
_x.privacyInformationUrl = privacyInformationUrl;
_x.publisher = publisher;
_x.publishingState = publishingState;
_x.assignments = assignments;
_x.appAvailability = appAvailability;
_x.version = version;
_x.committedContentVersion = committedContentVersion;
_x.fileName = fileName;
_x.size = size;
_x.contentVersions = contentVersions;
_x.minimumSupportedOperatingSystem = minimumSupportedOperatingSystem;
_x.packageId = packageId;
_x.versionCode = versionCode;
_x.versionName = versionName;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “The value for the minimum applicable operating system.”
*
* @return property minimumSupportedOperatingSystem
*/
@Property(name="minimumSupportedOperatingSystem")
@JsonIgnore
public Optional getMinimumSupportedOperatingSystem() {
return Optional.ofNullable(minimumSupportedOperatingSystem);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* minimumSupportedOperatingSystem} field changed. Field description below. The
* field name is also added to an internal map of changed fields in the returned
* object so that when {@code this.patch()} is called (if available)on the returned
* object only the changed fields are submitted.
*
* “The value for the minimum applicable operating system.”
*
* @param minimumSupportedOperatingSystem
* new value of {@code minimumSupportedOperatingSystem} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code minimumSupportedOperatingSystem} field changed
*/
public ManagedAndroidLobApp withMinimumSupportedOperatingSystem(AndroidMinimumOperatingSystem minimumSupportedOperatingSystem) {
ManagedAndroidLobApp _x = _copy();
_x.changedFields = changedFields.add("minimumSupportedOperatingSystem");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAndroidLobApp");
_x.minimumSupportedOperatingSystem = minimumSupportedOperatingSystem;
return _x;
}
/**
* “The package identifier.”
*
* @return property packageId
*/
@Property(name="packageId")
@JsonIgnore
public Optional getPackageId() {
return Optional.ofNullable(packageId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code packageId} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The package identifier.”
*
* @param packageId
* new value of {@code packageId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code packageId} field changed
*/
public ManagedAndroidLobApp withPackageId(String packageId) {
ManagedAndroidLobApp _x = _copy();
_x.changedFields = changedFields.add("packageId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAndroidLobApp");
_x.packageId = packageId;
return _x;
}
/**
* “The version code of managed Android Line of Business (LoB) app.”
*
* @return property versionCode
*/
@Property(name="versionCode")
@JsonIgnore
public Optional getVersionCode() {
return Optional.ofNullable(versionCode);
}
/**
* Returns an immutable copy of {@code this} with just the {@code versionCode}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The version code of managed Android Line of Business (LoB) app.”
*
* @param versionCode
* new value of {@code versionCode} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code versionCode} field changed
*/
public ManagedAndroidLobApp withVersionCode(String versionCode) {
ManagedAndroidLobApp _x = _copy();
_x.changedFields = changedFields.add("versionCode");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAndroidLobApp");
_x.versionCode = versionCode;
return _x;
}
/**
* “The version name of managed Android Line of Business (LoB) app.”
*
* @return property versionName
*/
@Property(name="versionName")
@JsonIgnore
public Optional getVersionName() {
return Optional.ofNullable(versionName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code versionName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The version name of managed Android Line of Business (LoB) app.”
*
* @param versionName
* new value of {@code versionName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code versionName} field changed
*/
public ManagedAndroidLobApp withVersionName(String versionName) {
ManagedAndroidLobApp _x = _copy();
_x.changedFields = changedFields.add("versionName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAndroidLobApp");
_x.versionName = versionName;
return _x;
}
public ManagedAndroidLobApp withUnmappedField(String name, Object value) {
ManagedAndroidLobApp _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ManagedAndroidLobApp patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
ManagedAndroidLobApp _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ManagedAndroidLobApp put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
ManagedAndroidLobApp _x = _copy();
_x.changedFields = null;
return _x;
}
private ManagedAndroidLobApp _copy() {
ManagedAndroidLobApp _x = new ManagedAndroidLobApp();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.developer = developer;
_x.displayName = displayName;
_x.informationUrl = informationUrl;
_x.isFeatured = isFeatured;
_x.largeIcon = largeIcon;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.notes = notes;
_x.owner = owner;
_x.privacyInformationUrl = privacyInformationUrl;
_x.publisher = publisher;
_x.publishingState = publishingState;
_x.assignments = assignments;
_x.appAvailability = appAvailability;
_x.version = version;
_x.committedContentVersion = committedContentVersion;
_x.fileName = fileName;
_x.size = size;
_x.contentVersions = contentVersions;
_x.minimumSupportedOperatingSystem = minimumSupportedOperatingSystem;
_x.packageId = packageId;
_x.versionCode = versionCode;
_x.versionName = versionName;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ManagedAndroidLobApp[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("developer=");
b.append(this.developer);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("informationUrl=");
b.append(this.informationUrl);
b.append(", ");
b.append("isFeatured=");
b.append(this.isFeatured);
b.append(", ");
b.append("largeIcon=");
b.append(this.largeIcon);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("notes=");
b.append(this.notes);
b.append(", ");
b.append("owner=");
b.append(this.owner);
b.append(", ");
b.append("privacyInformationUrl=");
b.append(this.privacyInformationUrl);
b.append(", ");
b.append("publisher=");
b.append(this.publisher);
b.append(", ");
b.append("publishingState=");
b.append(this.publishingState);
b.append(", ");
b.append("assignments=");
b.append(this.assignments);
b.append(", ");
b.append("appAvailability=");
b.append(this.appAvailability);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("committedContentVersion=");
b.append(this.committedContentVersion);
b.append(", ");
b.append("fileName=");
b.append(this.fileName);
b.append(", ");
b.append("size=");
b.append(this.size);
b.append(", ");
b.append("contentVersions=");
b.append(this.contentVersions);
b.append(", ");
b.append("minimumSupportedOperatingSystem=");
b.append(this.minimumSupportedOperatingSystem);
b.append(", ");
b.append("packageId=");
b.append(this.packageId);
b.append(", ");
b.append("versionCode=");
b.append(this.versionCode);
b.append(", ");
b.append("versionName=");
b.append(this.versionName);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}