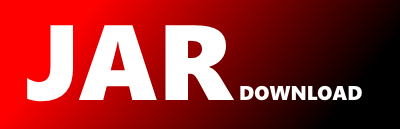
odata.msgraph.client.entity.ManagedAppRegistration Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.complex.MobileAppIdentifier;
import odata.msgraph.client.entity.collection.request.ManagedAppOperationCollectionRequest;
import odata.msgraph.client.entity.collection.request.ManagedAppPolicyCollectionRequest;
import odata.msgraph.client.enums.ManagedAppFlaggedReason;
/**
* “The ManagedAppRegistration resource represents the details of an app, with
* management capability, used by a member of the organization.”
*/@JsonPropertyOrder({
"@odata.type",
"appIdentifier",
"applicationVersion",
"createdDateTime",
"deviceName",
"deviceTag",
"deviceType",
"flaggedReasons",
"lastSyncDateTime",
"managementSdkVersion",
"platformVersion",
"userId",
"version",
"appliedPolicies",
"intendedPolicies",
"operations"})
@JsonInclude(Include.NON_NULL)
public class ManagedAppRegistration extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.managedAppRegistration";
}
@JsonProperty("appIdentifier")
protected MobileAppIdentifier appIdentifier;
@JsonProperty("applicationVersion")
protected String applicationVersion;
@JsonProperty("createdDateTime")
protected OffsetDateTime createdDateTime;
@JsonProperty("deviceName")
protected String deviceName;
@JsonProperty("deviceTag")
protected String deviceTag;
@JsonProperty("deviceType")
protected String deviceType;
@JsonProperty("flaggedReasons")
protected List flaggedReasons;
@JsonProperty("flaggedReasons@nextLink")
protected String flaggedReasonsNextLink;
@JsonProperty("lastSyncDateTime")
protected OffsetDateTime lastSyncDateTime;
@JsonProperty("managementSdkVersion")
protected String managementSdkVersion;
@JsonProperty("platformVersion")
protected String platformVersion;
@JsonProperty("userId")
protected String userId;
@JsonProperty("version")
protected String version;
@JsonProperty("appliedPolicies")
protected List appliedPolicies;
@JsonProperty("intendedPolicies")
protected List intendedPolicies;
@JsonProperty("operations")
protected List operations;
protected ManagedAppRegistration() {
super();
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “The app package Identifier”
*
* @return property appIdentifier
*/
@Property(name="appIdentifier")
@JsonIgnore
public Optional getAppIdentifier() {
return Optional.ofNullable(appIdentifier);
}
/**
* Returns an immutable copy of {@code this} with just the {@code appIdentifier}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The app package Identifier”
*
* @param appIdentifier
* new value of {@code appIdentifier} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code appIdentifier} field changed
*/
public ManagedAppRegistration withAppIdentifier(MobileAppIdentifier appIdentifier) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("appIdentifier");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.appIdentifier = appIdentifier;
return _x;
}
/**
* “App version”
*
* @return property applicationVersion
*/
@Property(name="applicationVersion")
@JsonIgnore
public Optional getApplicationVersion() {
return Optional.ofNullable(applicationVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* applicationVersion} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “App version”
*
* @param applicationVersion
* new value of {@code applicationVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code applicationVersion} field changed
*/
public ManagedAppRegistration withApplicationVersion(String applicationVersion) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("applicationVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.applicationVersion = applicationVersion;
return _x;
}
/**
* “Date and time of creation”
*
* @return property createdDateTime
*/
@Property(name="createdDateTime")
@JsonIgnore
public Optional getCreatedDateTime() {
return Optional.ofNullable(createdDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code createdDateTime}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Date and time of creation”
*
* @param createdDateTime
* new value of {@code createdDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code createdDateTime} field changed
*/
public ManagedAppRegistration withCreatedDateTime(OffsetDateTime createdDateTime) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("createdDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.createdDateTime = createdDateTime;
return _x;
}
/**
* “Host device name”
*
* @return property deviceName
*/
@Property(name="deviceName")
@JsonIgnore
public Optional getDeviceName() {
return Optional.ofNullable(deviceName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceName} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Host device name”
*
* @param deviceName
* new value of {@code deviceName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceName} field changed
*/
public ManagedAppRegistration withDeviceName(String deviceName) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("deviceName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.deviceName = deviceName;
return _x;
}
/**
* “App management SDK generated tag, which helps relate apps hosted on the same
* device. Not guaranteed to relate apps in all conditions.”
*
* @return property deviceTag
*/
@Property(name="deviceTag")
@JsonIgnore
public Optional getDeviceTag() {
return Optional.ofNullable(deviceTag);
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceTag} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “App management SDK generated tag, which helps relate apps hosted on the same
* device. Not guaranteed to relate apps in all conditions.”
*
* @param deviceTag
* new value of {@code deviceTag} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceTag} field changed
*/
public ManagedAppRegistration withDeviceTag(String deviceTag) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("deviceTag");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.deviceTag = deviceTag;
return _x;
}
/**
* “Host device type”
*
* @return property deviceType
*/
@Property(name="deviceType")
@JsonIgnore
public Optional getDeviceType() {
return Optional.ofNullable(deviceType);
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceType} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Host device type”
*
* @param deviceType
* new value of {@code deviceType} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceType} field changed
*/
public ManagedAppRegistration withDeviceType(String deviceType) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("deviceType");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.deviceType = deviceType;
return _x;
}
/**
* “Zero or more reasons an app registration is flagged. E.g. app running on rooted
* device”
*
* @return property flaggedReasons
*/
@Property(name="flaggedReasons")
@JsonIgnore
public CollectionPage getFlaggedReasons() {
return new CollectionPage(contextPath, ManagedAppFlaggedReason.class, this.flaggedReasons, Optional.ofNullable(flaggedReasonsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
/**
* Returns an immutable copy of {@code this} with just the {@code flaggedReasons}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Zero or more reasons an app registration is flagged. E.g. app running on rooted
* device”
*
* @param flaggedReasons
* new value of {@code flaggedReasons} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code flaggedReasons} field changed
*/
public ManagedAppRegistration withFlaggedReasons(List flaggedReasons) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("flaggedReasons");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.flaggedReasons = flaggedReasons;
return _x;
}
/**
* “Zero or more reasons an app registration is flagged. E.g. app running on rooted
* device”
*
* @param options
* specify connect and read timeouts
* @return property flaggedReasons
*/
@Property(name="flaggedReasons")
@JsonIgnore
public CollectionPage getFlaggedReasons(HttpRequestOptions options) {
return new CollectionPage(contextPath, ManagedAppFlaggedReason.class, this.flaggedReasons, Optional.ofNullable(flaggedReasonsNextLink), Collections.emptyList(), options);
}
/**
* “Date and time of last the app synced with management service.”
*
* @return property lastSyncDateTime
*/
@Property(name="lastSyncDateTime")
@JsonIgnore
public Optional getLastSyncDateTime() {
return Optional.ofNullable(lastSyncDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code lastSyncDateTime}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Date and time of last the app synced with management service.”
*
* @param lastSyncDateTime
* new value of {@code lastSyncDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code lastSyncDateTime} field changed
*/
public ManagedAppRegistration withLastSyncDateTime(OffsetDateTime lastSyncDateTime) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("lastSyncDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.lastSyncDateTime = lastSyncDateTime;
return _x;
}
/**
* “App management SDK version”
*
* @return property managementSdkVersion
*/
@Property(name="managementSdkVersion")
@JsonIgnore
public Optional getManagementSdkVersion() {
return Optional.ofNullable(managementSdkVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* managementSdkVersion} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “App management SDK version”
*
* @param managementSdkVersion
* new value of {@code managementSdkVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code managementSdkVersion} field changed
*/
public ManagedAppRegistration withManagementSdkVersion(String managementSdkVersion) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("managementSdkVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.managementSdkVersion = managementSdkVersion;
return _x;
}
/**
* “Operating System version”
*
* @return property platformVersion
*/
@Property(name="platformVersion")
@JsonIgnore
public Optional getPlatformVersion() {
return Optional.ofNullable(platformVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code platformVersion}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Operating System version”
*
* @param platformVersion
* new value of {@code platformVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code platformVersion} field changed
*/
public ManagedAppRegistration withPlatformVersion(String platformVersion) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("platformVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.platformVersion = platformVersion;
return _x;
}
/**
* “The user Id to who this app registration belongs.”
*
* @return property userId
*/
@Property(name="userId")
@JsonIgnore
public Optional getUserId() {
return Optional.ofNullable(userId);
}
/**
* Returns an immutable copy of {@code this} with just the {@code userId} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The user Id to who this app registration belongs.”
*
* @param userId
* new value of {@code userId} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userId} field changed
*/
public ManagedAppRegistration withUserId(String userId) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("userId");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.userId = userId;
return _x;
}
/**
* “Version of the entity.”
*
* @return property version
*/
@Property(name="version")
@JsonIgnore
public Optional getVersion() {
return Optional.ofNullable(version);
}
/**
* Returns an immutable copy of {@code this} with just the {@code version} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Version of the entity.”
*
* @param version
* new value of {@code version} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code version} field changed
*/
public ManagedAppRegistration withVersion(String version) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("version");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.version = version;
return _x;
}
public ManagedAppRegistration withUnmappedField(String name, Object value) {
ManagedAppRegistration _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “Zero or more policys already applied on the registered app when it last
* synchronized with managment service.”
*
* @return navigational property appliedPolicies
*/
@NavigationProperty(name="appliedPolicies")
@JsonIgnore
public ManagedAppPolicyCollectionRequest getAppliedPolicies() {
return new ManagedAppPolicyCollectionRequest(
contextPath.addSegment("appliedPolicies"), Optional.ofNullable(appliedPolicies));
}
/**
* “Zero or more policies admin intended for the app as of now.”
*
* @return navigational property intendedPolicies
*/
@NavigationProperty(name="intendedPolicies")
@JsonIgnore
public ManagedAppPolicyCollectionRequest getIntendedPolicies() {
return new ManagedAppPolicyCollectionRequest(
contextPath.addSegment("intendedPolicies"), Optional.ofNullable(intendedPolicies));
}
/**
* “Zero or more long running operations triggered on the app registration.”
*
* @return navigational property operations
*/
@NavigationProperty(name="operations")
@JsonIgnore
public ManagedAppOperationCollectionRequest getOperations() {
return new ManagedAppOperationCollectionRequest(
contextPath.addSegment("operations"), Optional.ofNullable(operations));
}
/**
* Returns an immutable copy of {@code this} with just the {@code appliedPolicies}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Zero or more policys already applied on the registered app when it last
* synchronized with managment service.”
*
* @param appliedPolicies
* new value of {@code appliedPolicies} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code appliedPolicies} field changed
*/
public ManagedAppRegistration withAppliedPolicies(List appliedPolicies) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("appliedPolicies");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.appliedPolicies = appliedPolicies;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code intendedPolicies}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Zero or more policies admin intended for the app as of now.”
*
* @param intendedPolicies
* new value of {@code intendedPolicies} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code intendedPolicies} field changed
*/
public ManagedAppRegistration withIntendedPolicies(List intendedPolicies) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("intendedPolicies");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.intendedPolicies = intendedPolicies;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code operations} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Zero or more long running operations triggered on the app registration.”
*
* @param operations
* new value of {@code operations} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code operations} field changed
*/
public ManagedAppRegistration withOperations(List operations) {
ManagedAppRegistration _x = _copy();
_x.changedFields = changedFields.add("operations");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedAppRegistration");
_x.operations = operations;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ManagedAppRegistration patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
ManagedAppRegistration _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ManagedAppRegistration put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
ManagedAppRegistration _x = _copy();
_x.changedFields = null;
return _x;
}
private ManagedAppRegistration _copy() {
ManagedAppRegistration _x = new ManagedAppRegistration();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.appIdentifier = appIdentifier;
_x.applicationVersion = applicationVersion;
_x.createdDateTime = createdDateTime;
_x.deviceName = deviceName;
_x.deviceTag = deviceTag;
_x.deviceType = deviceType;
_x.flaggedReasons = flaggedReasons;
_x.lastSyncDateTime = lastSyncDateTime;
_x.managementSdkVersion = managementSdkVersion;
_x.platformVersion = platformVersion;
_x.userId = userId;
_x.version = version;
_x.appliedPolicies = appliedPolicies;
_x.intendedPolicies = intendedPolicies;
_x.operations = operations;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ManagedAppRegistration[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("appIdentifier=");
b.append(this.appIdentifier);
b.append(", ");
b.append("applicationVersion=");
b.append(this.applicationVersion);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("deviceName=");
b.append(this.deviceName);
b.append(", ");
b.append("deviceTag=");
b.append(this.deviceTag);
b.append(", ");
b.append("deviceType=");
b.append(this.deviceType);
b.append(", ");
b.append("flaggedReasons=");
b.append(this.flaggedReasons);
b.append(", ");
b.append("lastSyncDateTime=");
b.append(this.lastSyncDateTime);
b.append(", ");
b.append("managementSdkVersion=");
b.append(this.managementSdkVersion);
b.append(", ");
b.append("platformVersion=");
b.append(this.platformVersion);
b.append(", ");
b.append("userId=");
b.append(this.userId);
b.append(", ");
b.append("version=");
b.append(this.version);
b.append(", ");
b.append("appliedPolicies=");
b.append(this.appliedPolicies);
b.append(", ");
b.append("intendedPolicies=");
b.append(this.intendedPolicies);
b.append(", ");
b.append("operations=");
b.append(this.operations);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}