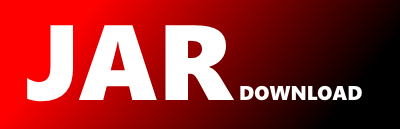
odata.msgraph.client.entity.ManagedEBook Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import odata.msgraph.client.complex.MimeContent;
import odata.msgraph.client.entity.collection.request.DeviceInstallStateCollectionRequest;
import odata.msgraph.client.entity.collection.request.ManagedEBookAssignmentCollectionRequest;
import odata.msgraph.client.entity.collection.request.UserInstallStateSummaryCollectionRequest;
import odata.msgraph.client.entity.request.EBookInstallSummaryRequest;
/**
* “An abstract class containing the base properties for Managed eBook.”
*/@JsonPropertyOrder({
"@odata.type",
"createdDateTime",
"description",
"displayName",
"informationUrl",
"largeCover",
"lastModifiedDateTime",
"privacyInformationUrl",
"publishedDateTime",
"publisher",
"assignments",
"deviceStates",
"installSummary",
"userStateSummary"})
@JsonInclude(Include.NON_NULL)
public class ManagedEBook extends Entity implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.managedEBook";
}
@JsonProperty("createdDateTime")
protected OffsetDateTime createdDateTime;
@JsonProperty("description")
protected String description;
@JsonProperty("displayName")
protected String displayName;
@JsonProperty("informationUrl")
protected String informationUrl;
@JsonProperty("largeCover")
protected MimeContent largeCover;
@JsonProperty("lastModifiedDateTime")
protected OffsetDateTime lastModifiedDateTime;
@JsonProperty("privacyInformationUrl")
protected String privacyInformationUrl;
@JsonProperty("publishedDateTime")
protected OffsetDateTime publishedDateTime;
@JsonProperty("publisher")
protected String publisher;
@JsonProperty("assignments")
protected List assignments;
@JsonProperty("deviceStates")
protected List deviceStates;
@JsonProperty("installSummary")
protected EBookInstallSummary installSummary;
@JsonProperty("userStateSummary")
protected List userStateSummary;
protected ManagedEBook() {
super();
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “The date and time when the eBook file was created.”
*
* @return property createdDateTime
*/
@Property(name="createdDateTime")
@JsonIgnore
public Optional getCreatedDateTime() {
return Optional.ofNullable(createdDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code createdDateTime}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The date and time when the eBook file was created.”
*
* @param createdDateTime
* new value of {@code createdDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code createdDateTime} field changed
*/
public ManagedEBook withCreatedDateTime(OffsetDateTime createdDateTime) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("createdDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.createdDateTime = createdDateTime;
return _x;
}
/**
* “Description.”
*
* @return property description
*/
@Property(name="description")
@JsonIgnore
public Optional getDescription() {
return Optional.ofNullable(description);
}
/**
* Returns an immutable copy of {@code this} with just the {@code description}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Description.”
*
* @param description
* new value of {@code description} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code description} field changed
*/
public ManagedEBook withDescription(String description) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("description");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.description = description;
return _x;
}
/**
* “Name of the eBook.”
*
* @return property displayName
*/
@Property(name="displayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code displayName}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Name of the eBook.”
*
* @param displayName
* new value of {@code displayName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code displayName} field changed
*/
public ManagedEBook withDisplayName(String displayName) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("displayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.displayName = displayName;
return _x;
}
/**
* “The more information Url.”
*
* @return property informationUrl
*/
@Property(name="informationUrl")
@JsonIgnore
public Optional getInformationUrl() {
return Optional.ofNullable(informationUrl);
}
/**
* Returns an immutable copy of {@code this} with just the {@code informationUrl}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The more information Url.”
*
* @param informationUrl
* new value of {@code informationUrl} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code informationUrl} field changed
*/
public ManagedEBook withInformationUrl(String informationUrl) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("informationUrl");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.informationUrl = informationUrl;
return _x;
}
/**
* “Book cover.”
*
* @return property largeCover
*/
@Property(name="largeCover")
@JsonIgnore
public Optional getLargeCover() {
return Optional.ofNullable(largeCover);
}
/**
* Returns an immutable copy of {@code this} with just the {@code largeCover} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Book cover.”
*
* @param largeCover
* new value of {@code largeCover} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code largeCover} field changed
*/
public ManagedEBook withLargeCover(MimeContent largeCover) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("largeCover");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.largeCover = largeCover;
return _x;
}
/**
* “The date and time when the eBook was last modified.”
*
* @return property lastModifiedDateTime
*/
@Property(name="lastModifiedDateTime")
@JsonIgnore
public Optional getLastModifiedDateTime() {
return Optional.ofNullable(lastModifiedDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* lastModifiedDateTime} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The date and time when the eBook was last modified.”
*
* @param lastModifiedDateTime
* new value of {@code lastModifiedDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code lastModifiedDateTime} field changed
*/
public ManagedEBook withLastModifiedDateTime(OffsetDateTime lastModifiedDateTime) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("lastModifiedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.lastModifiedDateTime = lastModifiedDateTime;
return _x;
}
/**
* “The privacy statement Url.”
*
* @return property privacyInformationUrl
*/
@Property(name="privacyInformationUrl")
@JsonIgnore
public Optional getPrivacyInformationUrl() {
return Optional.ofNullable(privacyInformationUrl);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* privacyInformationUrl} field changed. Field description below. The field name is
* also added to an internal map of changed fields in the returned object so that
* when {@code this.patch()} is called (if available)on the returned object only
* the changed fields are submitted.
*
* “The privacy statement Url.”
*
* @param privacyInformationUrl
* new value of {@code privacyInformationUrl} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code privacyInformationUrl} field changed
*/
public ManagedEBook withPrivacyInformationUrl(String privacyInformationUrl) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("privacyInformationUrl");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.privacyInformationUrl = privacyInformationUrl;
return _x;
}
/**
* “The date and time when the eBook was published.”
*
* @return property publishedDateTime
*/
@Property(name="publishedDateTime")
@JsonIgnore
public Optional getPublishedDateTime() {
return Optional.ofNullable(publishedDateTime);
}
/**
* Returns an immutable copy of {@code this} with just the {@code publishedDateTime
* } field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The date and time when the eBook was published.”
*
* @param publishedDateTime
* new value of {@code publishedDateTime} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code publishedDateTime} field changed
*/
public ManagedEBook withPublishedDateTime(OffsetDateTime publishedDateTime) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("publishedDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.publishedDateTime = publishedDateTime;
return _x;
}
/**
* “Publisher.”
*
* @return property publisher
*/
@Property(name="publisher")
@JsonIgnore
public Optional getPublisher() {
return Optional.ofNullable(publisher);
}
/**
* Returns an immutable copy of {@code this} with just the {@code publisher} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “Publisher.”
*
* @param publisher
* new value of {@code publisher} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code publisher} field changed
*/
public ManagedEBook withPublisher(String publisher) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("publisher");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.publisher = publisher;
return _x;
}
public ManagedEBook withUnmappedField(String name, Object value) {
ManagedEBook _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “The list of assignments for this eBook.”
*
* @return navigational property assignments
*/
@NavigationProperty(name="assignments")
@JsonIgnore
public ManagedEBookAssignmentCollectionRequest getAssignments() {
return new ManagedEBookAssignmentCollectionRequest(
contextPath.addSegment("assignments"), Optional.ofNullable(assignments));
}
/**
* “The list of installation states for this eBook.”
*
* @return navigational property deviceStates
*/
@NavigationProperty(name="deviceStates")
@JsonIgnore
public DeviceInstallStateCollectionRequest getDeviceStates() {
return new DeviceInstallStateCollectionRequest(
contextPath.addSegment("deviceStates"), Optional.ofNullable(deviceStates));
}
/**
* “Mobile App Install Summary.”
*
* @return navigational property installSummary
*/
@NavigationProperty(name="installSummary")
@JsonIgnore
public EBookInstallSummaryRequest getInstallSummary() {
return new EBookInstallSummaryRequest(contextPath.addSegment("installSummary"), Optional.ofNullable(installSummary));
}
/**
* “The list of installation states for this eBook.”
*
* @return navigational property userStateSummary
*/
@NavigationProperty(name="userStateSummary")
@JsonIgnore
public UserInstallStateSummaryCollectionRequest getUserStateSummary() {
return new UserInstallStateSummaryCollectionRequest(
contextPath.addSegment("userStateSummary"), Optional.ofNullable(userStateSummary));
}
/**
* Returns an immutable copy of {@code this} with just the {@code assignments}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The list of assignments for this eBook.”
*
* @param assignments
* new value of {@code assignments} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code assignments} field changed
*/
public ManagedEBook withAssignments(List assignments) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("assignments");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.assignments = assignments;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code deviceStates}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The list of installation states for this eBook.”
*
* @param deviceStates
* new value of {@code deviceStates} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code deviceStates} field changed
*/
public ManagedEBook withDeviceStates(List deviceStates) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("deviceStates");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.deviceStates = deviceStates;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code installSummary}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “Mobile App Install Summary.”
*
* @param installSummary
* new value of {@code installSummary} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code installSummary} field changed
*/
public ManagedEBook withInstallSummary(EBookInstallSummary installSummary) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("installSummary");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.installSummary = installSummary;
return _x;
}
/**
* Returns an immutable copy of {@code this} with just the {@code userStateSummary}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The list of installation states for this eBook.”
*
* @param userStateSummary
* new value of {@code userStateSummary} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code userStateSummary} field changed
*/
public ManagedEBook withUserStateSummary(List userStateSummary) {
ManagedEBook _x = _copy();
_x.changedFields = changedFields.add("userStateSummary");
_x.odataType = Util.nvl(odataType, "microsoft.graph.managedEBook");
_x.userStateSummary = userStateSummary;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ManagedEBook patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
ManagedEBook _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public ManagedEBook put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
ManagedEBook _x = _copy();
_x.changedFields = null;
return _x;
}
private ManagedEBook _copy() {
ManagedEBook _x = new ManagedEBook();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.displayName = displayName;
_x.informationUrl = informationUrl;
_x.largeCover = largeCover;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.privacyInformationUrl = privacyInformationUrl;
_x.publishedDateTime = publishedDateTime;
_x.publisher = publisher;
_x.assignments = assignments;
_x.deviceStates = deviceStates;
_x.installSummary = installSummary;
_x.userStateSummary = userStateSummary;
return _x;
}
@Action(name = "assign")
@JsonIgnore
public ActionRequestNoReturn assign(List managedEBookAssignments) {
Map _parameters = ParameterMap
.put("managedEBookAssignments", "Collection(microsoft.graph.managedEBookAssignment)", managedEBookAssignments)
.build();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("microsoft.graph.assign"), _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("ManagedEBook[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("informationUrl=");
b.append(this.informationUrl);
b.append(", ");
b.append("largeCover=");
b.append(this.largeCover);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("privacyInformationUrl=");
b.append(this.privacyInformationUrl);
b.append(", ");
b.append("publishedDateTime=");
b.append(this.publishedDateTime);
b.append(", ");
b.append("publisher=");
b.append(this.publisher);
b.append(", ");
b.append("assignments=");
b.append(this.assignments);
b.append(", ");
b.append("deviceStates=");
b.append(this.deviceStates);
b.append(", ");
b.append("installSummary=");
b.append(this.installSummary);
b.append(", ");
b.append("userStateSummary=");
b.append(this.userStateSummary);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}