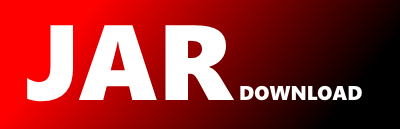
odata.msgraph.client.entity.MobileLobApp Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.util.List;
import java.util.Optional;
import odata.msgraph.client.entity.collection.request.MobileAppContentCollectionRequest;
/**
* “An abstract base class containing properties for all mobile line of business
* apps.”
*/@JsonPropertyOrder({
"@odata.type",
"committedContentVersion",
"fileName",
"size",
"contentVersions"})
@JsonInclude(Include.NON_NULL)
public class MobileLobApp extends MobileApp implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.mobileLobApp";
}
@JsonProperty("committedContentVersion")
protected String committedContentVersion;
@JsonProperty("fileName")
protected String fileName;
@JsonProperty("size")
protected Long size;
@JsonProperty("contentVersions")
protected List contentVersions;
protected MobileLobApp() {
super();
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
/**
* “The internal committed content version.”
*
* @return property committedContentVersion
*/
@Property(name="committedContentVersion")
@JsonIgnore
public Optional getCommittedContentVersion() {
return Optional.ofNullable(committedContentVersion);
}
/**
* Returns an immutable copy of {@code this} with just the {@code
* committedContentVersion} field changed. Field description below. The field name
* is also added to an internal map of changed fields in the returned object so
* that when {@code this.patch()} is called (if available)on the returned object
* only the changed fields are submitted.
*
* “The internal committed content version.”
*
* @param committedContentVersion
* new value of {@code committedContentVersion} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code committedContentVersion} field changed
*/
public MobileLobApp withCommittedContentVersion(String committedContentVersion) {
MobileLobApp _x = _copy();
_x.changedFields = changedFields.add("committedContentVersion");
_x.odataType = Util.nvl(odataType, "microsoft.graph.mobileLobApp");
_x.committedContentVersion = committedContentVersion;
return _x;
}
/**
* “The name of the main Lob application file.”
*
* @return property fileName
*/
@Property(name="fileName")
@JsonIgnore
public Optional getFileName() {
return Optional.ofNullable(fileName);
}
/**
* Returns an immutable copy of {@code this} with just the {@code fileName} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The name of the main Lob application file.”
*
* @param fileName
* new value of {@code fileName} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code fileName} field changed
*/
public MobileLobApp withFileName(String fileName) {
MobileLobApp _x = _copy();
_x.changedFields = changedFields.add("fileName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.mobileLobApp");
_x.fileName = fileName;
return _x;
}
/**
* “The total size, including all uploaded files.”
*
* @return property size
*/
@Property(name="size")
@JsonIgnore
public Optional getSize() {
return Optional.ofNullable(size);
}
/**
* Returns an immutable copy of {@code this} with just the {@code size} field
* changed. Field description below. The field name is also added to an internal
* map of changed fields in the returned object so that when {@code this.patch()}
* is called (if available)on the returned object only the changed fields are
* submitted.
*
* “The total size, including all uploaded files.”
*
* @param size
* new value of {@code size} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code size} field changed
*/
public MobileLobApp withSize(Long size) {
MobileLobApp _x = _copy();
_x.changedFields = changedFields.add("size");
_x.odataType = Util.nvl(odataType, "microsoft.graph.mobileLobApp");
_x.size = size;
return _x;
}
public MobileLobApp withUnmappedField(String name, Object value) {
MobileLobApp _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
/**
* “The list of content versions for this app.”
*
* @return navigational property contentVersions
*/
@NavigationProperty(name="contentVersions")
@JsonIgnore
public MobileAppContentCollectionRequest getContentVersions() {
return new MobileAppContentCollectionRequest(
contextPath.addSegment("contentVersions"), Optional.ofNullable(contentVersions));
}
/**
* Returns an immutable copy of {@code this} with just the {@code contentVersions}
* field changed. Field description below. The field name is also added to an
* internal map of changed fields in the returned object so that when {@code this.
* patch()} is called (if available)on the returned object only the changed fields
* are submitted.
*
* “The list of content versions for this app.”
*
* @param contentVersions
* new value of {@code contentVersions} field (as defined in service metadata)
* @return immutable copy of {@code this} with just the {@code contentVersions} field changed
*/
public MobileLobApp withContentVersions(List contentVersions) {
MobileLobApp _x = _copy();
_x.changedFields = changedFields.add("contentVersions");
_x.odataType = Util.nvl(odataType, "microsoft.graph.mobileLobApp");
_x.contentVersions = contentVersions;
return _x;
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public MobileLobApp patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
MobileLobApp _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public MobileLobApp put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
MobileLobApp _x = _copy();
_x.changedFields = null;
return _x;
}
private MobileLobApp _copy() {
MobileLobApp _x = new MobileLobApp();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.createdDateTime = createdDateTime;
_x.description = description;
_x.developer = developer;
_x.displayName = displayName;
_x.informationUrl = informationUrl;
_x.isFeatured = isFeatured;
_x.largeIcon = largeIcon;
_x.lastModifiedDateTime = lastModifiedDateTime;
_x.notes = notes;
_x.owner = owner;
_x.privacyInformationUrl = privacyInformationUrl;
_x.publisher = publisher;
_x.publishingState = publishingState;
_x.assignments = assignments;
_x.committedContentVersion = committedContentVersion;
_x.fileName = fileName;
_x.size = size;
_x.contentVersions = contentVersions;
return _x;
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("MobileLobApp[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("createdDateTime=");
b.append(this.createdDateTime);
b.append(", ");
b.append("description=");
b.append(this.description);
b.append(", ");
b.append("developer=");
b.append(this.developer);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("informationUrl=");
b.append(this.informationUrl);
b.append(", ");
b.append("isFeatured=");
b.append(this.isFeatured);
b.append(", ");
b.append("largeIcon=");
b.append(this.largeIcon);
b.append(", ");
b.append("lastModifiedDateTime=");
b.append(this.lastModifiedDateTime);
b.append(", ");
b.append("notes=");
b.append(this.notes);
b.append(", ");
b.append("owner=");
b.append(this.owner);
b.append(", ");
b.append("privacyInformationUrl=");
b.append(this.privacyInformationUrl);
b.append(", ");
b.append("publisher=");
b.append(this.publisher);
b.append(", ");
b.append("publishingState=");
b.append(this.publishingState);
b.append(", ");
b.append("assignments=");
b.append(this.assignments);
b.append(", ");
b.append("committedContentVersion=");
b.append(this.committedContentVersion);
b.append(", ");
b.append("fileName=");
b.append(this.fileName);
b.append(", ");
b.append("size=");
b.append(this.size);
b.append(", ");
b.append("contentVersions=");
b.append(this.contentVersions);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}