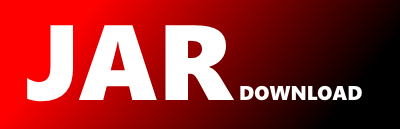
odata.msgraph.client.entity.OrgContact Maven / Gradle / Ivy
Show all versions of odata-client-msgraph Show documentation
package odata.msgraph.client.entity;
import com.fasterxml.jackson.annotation.JsonAnyGetter;
import com.fasterxml.jackson.annotation.JsonAnySetter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.github.davidmoten.odata.client.ActionRequestNoReturn;
import com.github.davidmoten.odata.client.ClientException;
import com.github.davidmoten.odata.client.CollectionPage;
import com.github.davidmoten.odata.client.HttpRequestOptions;
import com.github.davidmoten.odata.client.NameValue;
import com.github.davidmoten.odata.client.ODataEntityType;
import com.github.davidmoten.odata.client.RequestOptions;
import com.github.davidmoten.odata.client.UnmappedFields;
import com.github.davidmoten.odata.client.Util;
import com.github.davidmoten.odata.client.annotation.Action;
import com.github.davidmoten.odata.client.annotation.NavigationProperty;
import com.github.davidmoten.odata.client.annotation.Property;
import com.github.davidmoten.odata.client.internal.ChangedFields;
import com.github.davidmoten.odata.client.internal.ParameterMap;
import com.github.davidmoten.odata.client.internal.RequestHelper;
import com.github.davidmoten.odata.client.internal.TypedObject;
import com.github.davidmoten.odata.client.internal.UnmappedFieldsImpl;
import java.lang.Boolean;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import java.time.OffsetDateTime;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import odata.msgraph.client.complex.OnPremisesProvisioningError;
import odata.msgraph.client.complex.Phone;
import odata.msgraph.client.complex.PhysicalOfficeAddress;
import odata.msgraph.client.complex.ServiceProvisioningError;
import odata.msgraph.client.entity.collection.request.DirectoryObjectCollectionRequest;
import odata.msgraph.client.entity.request.DirectoryObjectRequest;
/**
*
* Org.OData.Capabilities.V1.ChangeTracking
*
* Supported = true
*/@JsonPropertyOrder({
"@odata.type",
"addresses",
"companyName",
"department",
"displayName",
"givenName",
"jobTitle",
"mail",
"mailNickname",
"onPremisesLastSyncDateTime",
"onPremisesProvisioningErrors",
"onPremisesSyncEnabled",
"phones",
"proxyAddresses",
"serviceProvisioningErrors",
"surname"})
@JsonInclude(Include.NON_NULL)
public class OrgContact extends DirectoryObject implements ODataEntityType {
@Override
public String odataTypeName() {
return "microsoft.graph.orgContact";
}
@JsonProperty("addresses")
protected List addresses;
@JsonProperty("addresses@nextLink")
protected String addressesNextLink;
@JsonProperty("companyName")
protected String companyName;
@JsonProperty("department")
protected String department;
@JsonProperty("displayName")
protected String displayName;
@JsonProperty("givenName")
protected String givenName;
@JsonProperty("jobTitle")
protected String jobTitle;
@JsonProperty("mail")
protected String mail;
@JsonProperty("mailNickname")
protected String mailNickname;
@JsonProperty("onPremisesLastSyncDateTime")
protected OffsetDateTime onPremisesLastSyncDateTime;
@JsonProperty("onPremisesProvisioningErrors")
protected List onPremisesProvisioningErrors;
@JsonProperty("onPremisesProvisioningErrors@nextLink")
protected String onPremisesProvisioningErrorsNextLink;
@JsonProperty("onPremisesSyncEnabled")
protected Boolean onPremisesSyncEnabled;
@JsonProperty("phones")
protected List phones;
@JsonProperty("phones@nextLink")
protected String phonesNextLink;
@JsonProperty("proxyAddresses")
protected List proxyAddresses;
@JsonProperty("proxyAddresses@nextLink")
protected String proxyAddressesNextLink;
@JsonProperty("serviceProvisioningErrors")
protected List serviceProvisioningErrors;
@JsonProperty("serviceProvisioningErrors@nextLink")
protected String serviceProvisioningErrorsNextLink;
@JsonProperty("surname")
protected String surname;
protected OrgContact() {
super();
}
/**
* Returns a builder which is used to create a new
* instance of this class (given that this class is immutable).
*
* @return a new Builder for this class
*/
// Suffix used on builder factory method to differentiate the method
// from static builder methods on superclasses
public static Builder builderOrgContact() {
return new Builder();
}
public static final class Builder {
private String id;
private OffsetDateTime deletedDateTime;
private List addresses;
private String addressesNextLink;
private String companyName;
private String department;
private String displayName;
private String givenName;
private String jobTitle;
private String mail;
private String mailNickname;
private OffsetDateTime onPremisesLastSyncDateTime;
private List onPremisesProvisioningErrors;
private String onPremisesProvisioningErrorsNextLink;
private Boolean onPremisesSyncEnabled;
private List phones;
private String phonesNextLink;
private List proxyAddresses;
private String proxyAddressesNextLink;
private List serviceProvisioningErrors;
private String serviceProvisioningErrorsNextLink;
private String surname;
private ChangedFields changedFields = ChangedFields.EMPTY;
Builder() {
// prevent instantiation
}
public Builder id(String id) {
this.id = id;
this.changedFields = changedFields.add("id");
return this;
}
public Builder deletedDateTime(OffsetDateTime deletedDateTime) {
this.deletedDateTime = deletedDateTime;
this.changedFields = changedFields.add("deletedDateTime");
return this;
}
public Builder addresses(List addresses) {
this.addresses = addresses;
this.changedFields = changedFields.add("addresses");
return this;
}
public Builder addresses(PhysicalOfficeAddress... addresses) {
return addresses(Arrays.asList(addresses));
}
public Builder addressesNextLink(String addressesNextLink) {
this.addressesNextLink = addressesNextLink;
this.changedFields = changedFields.add("addresses");
return this;
}
public Builder companyName(String companyName) {
this.companyName = companyName;
this.changedFields = changedFields.add("companyName");
return this;
}
public Builder department(String department) {
this.department = department;
this.changedFields = changedFields.add("department");
return this;
}
public Builder displayName(String displayName) {
this.displayName = displayName;
this.changedFields = changedFields.add("displayName");
return this;
}
public Builder givenName(String givenName) {
this.givenName = givenName;
this.changedFields = changedFields.add("givenName");
return this;
}
public Builder jobTitle(String jobTitle) {
this.jobTitle = jobTitle;
this.changedFields = changedFields.add("jobTitle");
return this;
}
public Builder mail(String mail) {
this.mail = mail;
this.changedFields = changedFields.add("mail");
return this;
}
public Builder mailNickname(String mailNickname) {
this.mailNickname = mailNickname;
this.changedFields = changedFields.add("mailNickname");
return this;
}
public Builder onPremisesLastSyncDateTime(OffsetDateTime onPremisesLastSyncDateTime) {
this.onPremisesLastSyncDateTime = onPremisesLastSyncDateTime;
this.changedFields = changedFields.add("onPremisesLastSyncDateTime");
return this;
}
public Builder onPremisesProvisioningErrors(List onPremisesProvisioningErrors) {
this.onPremisesProvisioningErrors = onPremisesProvisioningErrors;
this.changedFields = changedFields.add("onPremisesProvisioningErrors");
return this;
}
public Builder onPremisesProvisioningErrors(OnPremisesProvisioningError... onPremisesProvisioningErrors) {
return onPremisesProvisioningErrors(Arrays.asList(onPremisesProvisioningErrors));
}
public Builder onPremisesProvisioningErrorsNextLink(String onPremisesProvisioningErrorsNextLink) {
this.onPremisesProvisioningErrorsNextLink = onPremisesProvisioningErrorsNextLink;
this.changedFields = changedFields.add("onPremisesProvisioningErrors");
return this;
}
public Builder onPremisesSyncEnabled(Boolean onPremisesSyncEnabled) {
this.onPremisesSyncEnabled = onPremisesSyncEnabled;
this.changedFields = changedFields.add("onPremisesSyncEnabled");
return this;
}
public Builder phones(List phones) {
this.phones = phones;
this.changedFields = changedFields.add("phones");
return this;
}
public Builder phones(Phone... phones) {
return phones(Arrays.asList(phones));
}
public Builder phonesNextLink(String phonesNextLink) {
this.phonesNextLink = phonesNextLink;
this.changedFields = changedFields.add("phones");
return this;
}
public Builder proxyAddresses(List proxyAddresses) {
this.proxyAddresses = proxyAddresses;
this.changedFields = changedFields.add("proxyAddresses");
return this;
}
public Builder proxyAddresses(String... proxyAddresses) {
return proxyAddresses(Arrays.asList(proxyAddresses));
}
public Builder proxyAddressesNextLink(String proxyAddressesNextLink) {
this.proxyAddressesNextLink = proxyAddressesNextLink;
this.changedFields = changedFields.add("proxyAddresses");
return this;
}
public Builder serviceProvisioningErrors(List serviceProvisioningErrors) {
this.serviceProvisioningErrors = serviceProvisioningErrors;
this.changedFields = changedFields.add("serviceProvisioningErrors");
return this;
}
public Builder serviceProvisioningErrors(ServiceProvisioningError... serviceProvisioningErrors) {
return serviceProvisioningErrors(Arrays.asList(serviceProvisioningErrors));
}
public Builder serviceProvisioningErrorsNextLink(String serviceProvisioningErrorsNextLink) {
this.serviceProvisioningErrorsNextLink = serviceProvisioningErrorsNextLink;
this.changedFields = changedFields.add("serviceProvisioningErrors");
return this;
}
public Builder surname(String surname) {
this.surname = surname;
this.changedFields = changedFields.add("surname");
return this;
}
public OrgContact build() {
OrgContact _x = new OrgContact();
_x.contextPath = null;
_x.changedFields = changedFields;
_x.unmappedFields = new UnmappedFieldsImpl();
_x.odataType = "microsoft.graph.orgContact";
_x.id = id;
_x.deletedDateTime = deletedDateTime;
_x.addresses = addresses;
_x.addressesNextLink = addressesNextLink;
_x.companyName = companyName;
_x.department = department;
_x.displayName = displayName;
_x.givenName = givenName;
_x.jobTitle = jobTitle;
_x.mail = mail;
_x.mailNickname = mailNickname;
_x.onPremisesLastSyncDateTime = onPremisesLastSyncDateTime;
_x.onPremisesProvisioningErrors = onPremisesProvisioningErrors;
_x.onPremisesProvisioningErrorsNextLink = onPremisesProvisioningErrorsNextLink;
_x.onPremisesSyncEnabled = onPremisesSyncEnabled;
_x.phones = phones;
_x.phonesNextLink = phonesNextLink;
_x.proxyAddresses = proxyAddresses;
_x.proxyAddressesNextLink = proxyAddressesNextLink;
_x.serviceProvisioningErrors = serviceProvisioningErrors;
_x.serviceProvisioningErrorsNextLink = serviceProvisioningErrorsNextLink;
_x.surname = surname;
return _x;
}
}
@Override
@JsonIgnore
public ChangedFields getChangedFields() {
return changedFields;
}
@Override
public void postInject(boolean addKeysToContextPath) {
if (addKeysToContextPath && id != null) {
contextPath = contextPath.clearQueries().addKeys(new NameValue(id, String.class));
}
}
@Property(name="addresses")
@JsonIgnore
public CollectionPage getAddresses() {
return new CollectionPage(contextPath, PhysicalOfficeAddress.class, this.addresses, Optional.ofNullable(addressesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public OrgContact withAddresses(List addresses) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("addresses");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.addresses = addresses;
return _x;
}
@Property(name="addresses")
@JsonIgnore
public CollectionPage getAddresses(HttpRequestOptions options) {
return new CollectionPage(contextPath, PhysicalOfficeAddress.class, this.addresses, Optional.ofNullable(addressesNextLink), Collections.emptyList(), options);
}
@Property(name="companyName")
@JsonIgnore
public Optional getCompanyName() {
return Optional.ofNullable(companyName);
}
public OrgContact withCompanyName(String companyName) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("companyName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.companyName = companyName;
return _x;
}
@Property(name="department")
@JsonIgnore
public Optional getDepartment() {
return Optional.ofNullable(department);
}
public OrgContact withDepartment(String department) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("department");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.department = department;
return _x;
}
@Property(name="displayName")
@JsonIgnore
public Optional getDisplayName() {
return Optional.ofNullable(displayName);
}
public OrgContact withDisplayName(String displayName) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("displayName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.displayName = displayName;
return _x;
}
@Property(name="givenName")
@JsonIgnore
public Optional getGivenName() {
return Optional.ofNullable(givenName);
}
public OrgContact withGivenName(String givenName) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("givenName");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.givenName = givenName;
return _x;
}
@Property(name="jobTitle")
@JsonIgnore
public Optional getJobTitle() {
return Optional.ofNullable(jobTitle);
}
public OrgContact withJobTitle(String jobTitle) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("jobTitle");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.jobTitle = jobTitle;
return _x;
}
@Property(name="mail")
@JsonIgnore
public Optional getMail() {
return Optional.ofNullable(mail);
}
public OrgContact withMail(String mail) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("mail");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.mail = mail;
return _x;
}
@Property(name="mailNickname")
@JsonIgnore
public Optional getMailNickname() {
return Optional.ofNullable(mailNickname);
}
public OrgContact withMailNickname(String mailNickname) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("mailNickname");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.mailNickname = mailNickname;
return _x;
}
@Property(name="onPremisesLastSyncDateTime")
@JsonIgnore
public Optional getOnPremisesLastSyncDateTime() {
return Optional.ofNullable(onPremisesLastSyncDateTime);
}
public OrgContact withOnPremisesLastSyncDateTime(OffsetDateTime onPremisesLastSyncDateTime) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("onPremisesLastSyncDateTime");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.onPremisesLastSyncDateTime = onPremisesLastSyncDateTime;
return _x;
}
@Property(name="onPremisesProvisioningErrors")
@JsonIgnore
public CollectionPage getOnPremisesProvisioningErrors() {
return new CollectionPage(contextPath, OnPremisesProvisioningError.class, this.onPremisesProvisioningErrors, Optional.ofNullable(onPremisesProvisioningErrorsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public OrgContact withOnPremisesProvisioningErrors(List onPremisesProvisioningErrors) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("onPremisesProvisioningErrors");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.onPremisesProvisioningErrors = onPremisesProvisioningErrors;
return _x;
}
@Property(name="onPremisesProvisioningErrors")
@JsonIgnore
public CollectionPage getOnPremisesProvisioningErrors(HttpRequestOptions options) {
return new CollectionPage(contextPath, OnPremisesProvisioningError.class, this.onPremisesProvisioningErrors, Optional.ofNullable(onPremisesProvisioningErrorsNextLink), Collections.emptyList(), options);
}
@Property(name="onPremisesSyncEnabled")
@JsonIgnore
public Optional getOnPremisesSyncEnabled() {
return Optional.ofNullable(onPremisesSyncEnabled);
}
public OrgContact withOnPremisesSyncEnabled(Boolean onPremisesSyncEnabled) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("onPremisesSyncEnabled");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.onPremisesSyncEnabled = onPremisesSyncEnabled;
return _x;
}
@Property(name="phones")
@JsonIgnore
public CollectionPage getPhones() {
return new CollectionPage(contextPath, Phone.class, this.phones, Optional.ofNullable(phonesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public OrgContact withPhones(List phones) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("phones");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.phones = phones;
return _x;
}
@Property(name="phones")
@JsonIgnore
public CollectionPage getPhones(HttpRequestOptions options) {
return new CollectionPage(contextPath, Phone.class, this.phones, Optional.ofNullable(phonesNextLink), Collections.emptyList(), options);
}
@Property(name="proxyAddresses")
@JsonIgnore
public CollectionPage getProxyAddresses() {
return new CollectionPage(contextPath, String.class, this.proxyAddresses, Optional.ofNullable(proxyAddressesNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public OrgContact withProxyAddresses(List proxyAddresses) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("proxyAddresses");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.proxyAddresses = proxyAddresses;
return _x;
}
@Property(name="proxyAddresses")
@JsonIgnore
public CollectionPage getProxyAddresses(HttpRequestOptions options) {
return new CollectionPage(contextPath, String.class, this.proxyAddresses, Optional.ofNullable(proxyAddressesNextLink), Collections.emptyList(), options);
}
@Property(name="serviceProvisioningErrors")
@JsonIgnore
public CollectionPage getServiceProvisioningErrors() {
return new CollectionPage(contextPath, ServiceProvisioningError.class, this.serviceProvisioningErrors, Optional.ofNullable(serviceProvisioningErrorsNextLink), Collections.emptyList(), HttpRequestOptions.EMPTY);
}
public OrgContact withServiceProvisioningErrors(List serviceProvisioningErrors) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("serviceProvisioningErrors");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.serviceProvisioningErrors = serviceProvisioningErrors;
return _x;
}
@Property(name="serviceProvisioningErrors")
@JsonIgnore
public CollectionPage getServiceProvisioningErrors(HttpRequestOptions options) {
return new CollectionPage(contextPath, ServiceProvisioningError.class, this.serviceProvisioningErrors, Optional.ofNullable(serviceProvisioningErrorsNextLink), Collections.emptyList(), options);
}
@Property(name="surname")
@JsonIgnore
public Optional getSurname() {
return Optional.ofNullable(surname);
}
public OrgContact withSurname(String surname) {
OrgContact _x = _copy();
_x.changedFields = changedFields.add("surname");
_x.odataType = Util.nvl(odataType, "microsoft.graph.orgContact");
_x.surname = surname;
return _x;
}
public OrgContact withUnmappedField(String name, Object value) {
OrgContact _x = _copy();
_x.setUnmappedField(name, value);
return _x;
}
@NavigationProperty(name="directReports")
@JsonIgnore
public DirectoryObjectCollectionRequest getDirectReports() {
return new DirectoryObjectCollectionRequest(
contextPath.addSegment("directReports"), RequestHelper.getValue(unmappedFields, "directReports"));
}
@NavigationProperty(name="manager")
@JsonIgnore
public DirectoryObjectRequest getManager() {
return new DirectoryObjectRequest(contextPath.addSegment("manager"), RequestHelper.getValue(unmappedFields, "manager"));
}
@NavigationProperty(name="memberOf")
@JsonIgnore
public DirectoryObjectCollectionRequest getMemberOf() {
return new DirectoryObjectCollectionRequest(
contextPath.addSegment("memberOf"), RequestHelper.getValue(unmappedFields, "memberOf"));
}
@NavigationProperty(name="transitiveMemberOf")
@JsonIgnore
public DirectoryObjectCollectionRequest getTransitiveMemberOf() {
return new DirectoryObjectCollectionRequest(
contextPath.addSegment("transitiveMemberOf"), RequestHelper.getValue(unmappedFields, "transitiveMemberOf"));
}
@JsonAnySetter
private void setUnmappedField(String name, Object value) {
if (unmappedFields == null) {
unmappedFields = new UnmappedFieldsImpl();
}
unmappedFields.put(name, value);
}
@JsonAnyGetter
private UnmappedFieldsImpl unmappedFields() {
return unmappedFields == null ? UnmappedFieldsImpl.EMPTY : unmappedFields;
}
@Override
public UnmappedFields getUnmappedFields() {
return unmappedFields();
}
/**
* Submits only changed fields for update and returns an
* immutable copy of {@code this} with changed fields reset.
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public OrgContact patch() {
RequestHelper.patch(this, contextPath, RequestOptions.EMPTY);
OrgContact _x = _copy();
_x.changedFields = null;
return _x;
}
/**
* Submits all fields for update and returns an immutable copy of {@code this}
* with changed fields reset (they were ignored anyway).
*
* @return a copy of {@code this} with changed fields reset
* @throws ClientException if HTTP response is not as expected
*/
public OrgContact put() {
RequestHelper.put(this, contextPath, RequestOptions.EMPTY);
OrgContact _x = _copy();
_x.changedFields = null;
return _x;
}
private OrgContact _copy() {
OrgContact _x = new OrgContact();
_x.contextPath = contextPath;
_x.changedFields = changedFields;
_x.unmappedFields = unmappedFields.copy();
_x.odataType = odataType;
_x.id = id;
_x.deletedDateTime = deletedDateTime;
_x.addresses = addresses;
_x.companyName = companyName;
_x.department = department;
_x.displayName = displayName;
_x.givenName = givenName;
_x.jobTitle = jobTitle;
_x.mail = mail;
_x.mailNickname = mailNickname;
_x.onPremisesLastSyncDateTime = onPremisesLastSyncDateTime;
_x.onPremisesProvisioningErrors = onPremisesProvisioningErrors;
_x.onPremisesSyncEnabled = onPremisesSyncEnabled;
_x.phones = phones;
_x.proxyAddresses = proxyAddresses;
_x.serviceProvisioningErrors = serviceProvisioningErrors;
_x.surname = surname;
return _x;
}
@Action(name = "retryServiceProvisioning")
@JsonIgnore
public ActionRequestNoReturn retryServiceProvisioning() {
Map _parameters = ParameterMap.empty();
return new ActionRequestNoReturn(this.contextPath.addActionOrFunctionSegment("microsoft.graph.retryServiceProvisioning"), _parameters);
}
@Override
public String toString() {
StringBuilder b = new StringBuilder();
b.append("OrgContact[");
b.append("id=");
b.append(this.id);
b.append(", ");
b.append("deletedDateTime=");
b.append(this.deletedDateTime);
b.append(", ");
b.append("addresses=");
b.append(this.addresses);
b.append(", ");
b.append("companyName=");
b.append(this.companyName);
b.append(", ");
b.append("department=");
b.append(this.department);
b.append(", ");
b.append("displayName=");
b.append(this.displayName);
b.append(", ");
b.append("givenName=");
b.append(this.givenName);
b.append(", ");
b.append("jobTitle=");
b.append(this.jobTitle);
b.append(", ");
b.append("mail=");
b.append(this.mail);
b.append(", ");
b.append("mailNickname=");
b.append(this.mailNickname);
b.append(", ");
b.append("onPremisesLastSyncDateTime=");
b.append(this.onPremisesLastSyncDateTime);
b.append(", ");
b.append("onPremisesProvisioningErrors=");
b.append(this.onPremisesProvisioningErrors);
b.append(", ");
b.append("onPremisesSyncEnabled=");
b.append(this.onPremisesSyncEnabled);
b.append(", ");
b.append("phones=");
b.append(this.phones);
b.append(", ");
b.append("proxyAddresses=");
b.append(this.proxyAddresses);
b.append(", ");
b.append("serviceProvisioningErrors=");
b.append(this.serviceProvisioningErrors);
b.append(", ");
b.append("surname=");
b.append(this.surname);
b.append("]");
b.append(",unmappedFields=");
b.append(unmappedFields);
b.append(",odataType=");
b.append(odataType);
return b.toString();
}
}